import cv2
import numpy as np
import os
import random
from PIL import Image
import matplotlib.pyplot as plt
# https://blog.csdn.net/qq_44886601/article/details/135764589
# 将mask绘制在原图
def draw_image(im, ms, brg, opacity):
image_mask = im.copy()
contours, _ = cv2.findContours(ms, cv2.RETR_TREE, cv2.CHAIN_APPROX_NONE) # 查找轮廓
image_mask = cv2.drawContours(image_mask, contours, -1, (0, 255, 0), 2) # 绘制边界
image_mask = cv2.fillPoly(image_mask, contours, color=brg) # 填充
img_bgr = cv2.addWeighted(im, opacity, image_mask, 1 - opacity, 0)
return im, ms, img_bgr
def main(imagePath, labelPath, bgr, opacity):
image = np.array(Image.open(imagePath).convert('RGB'))
label = np.array(Image.open(labelPath).convert('L'))
a, b, c = draw_image(image, label, bgr, opacity)
plt.figure(figsize=(12, 8))
for index, i in enumerate((a, b, c)):
plt.subplot(1, 3, index + 1)
plt.imshow(i)
plt.savefig('./result.png')
# plt.show()
if __name__ == '__main__':
root = './data/train/images'
images_path = [os.path.join(root, i) for i in os.listdir(root)]
r = random.randint(0, len(images_path) - 1)
img_path = images_path[r] # 随机取出一张图片
mask_path = img_path.replace('images', 'masks')
# opacity 越小,掩膜效果越深
main(imagePath=img_path, labelPath=mask_path, bgr=(0, 0, 255), opacity=0.5)
没有合适的资源?快使用搜索试试~ 我知道了~
医学图像分割数据集:腹部CT肺分割(包含训练集和测试集)
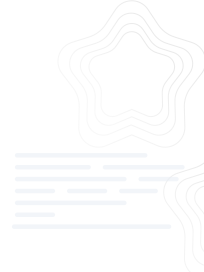
共536个文件
tif:534个
py:1个
png:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
项目包含:腹部CT肺分割(包含训练集和测试集) 数据集为512*512 分辨率下的肺部分割。分割前景为肺等等。标签的mask图像为前景为255的二值图像,方便观察 数据集介绍:分为训练集、测试集 训练集:images图片目录+masks模板目录,214张图片和214个对应的mask图片 测试集:images图片目录+masks模板目录,53图片和53个对应的mask图片 除此之外,包含一个图像分割的可视化脚本,随机提取一张图片,将其原始图片、GT图像、GT在原图蒙板的图像展示,并保存在当前目录下
资源推荐
资源详情
资源评论
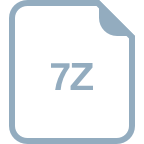
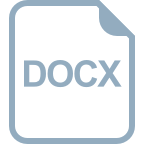
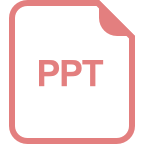
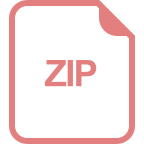
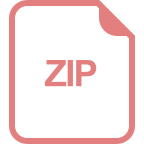
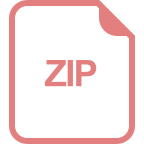
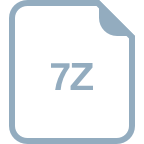
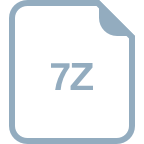
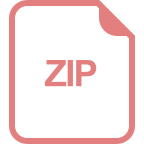
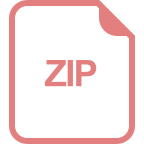
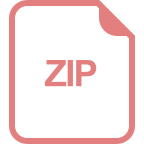
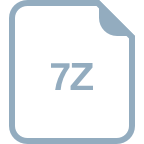
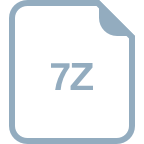
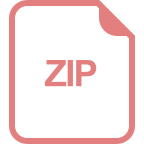
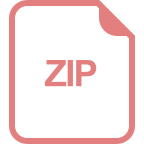
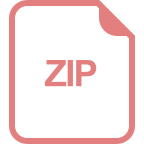
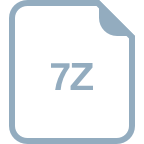
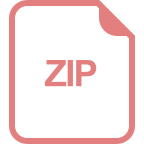
收起资源包目录

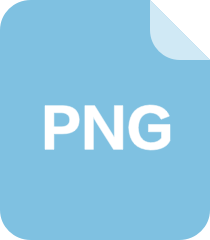
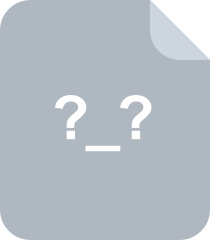
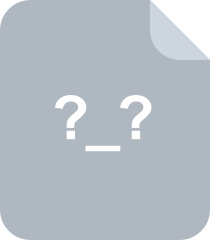
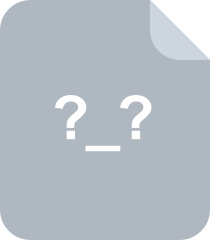
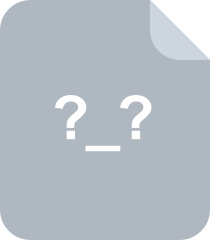
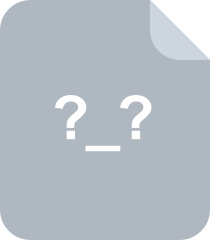
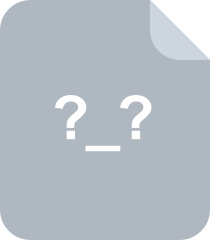
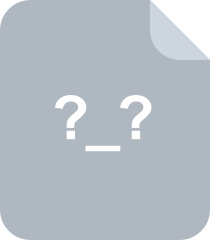
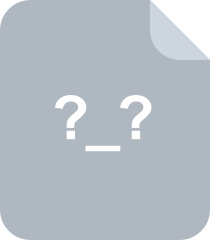
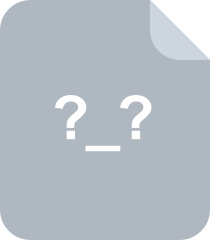
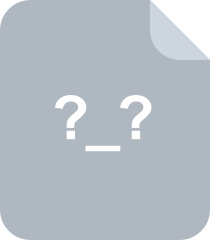
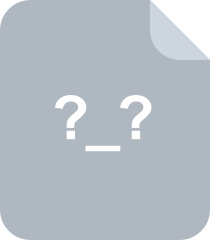
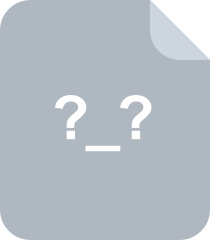
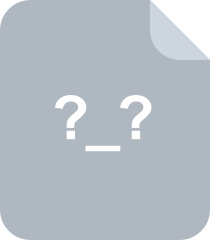
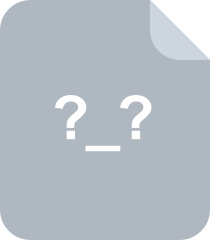
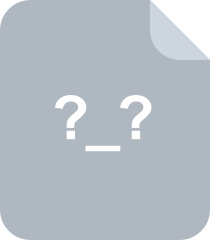
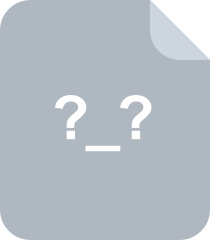
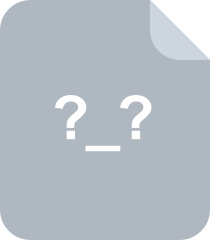
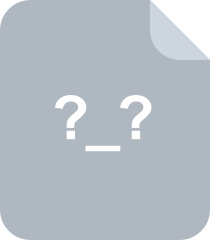
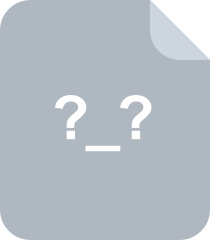
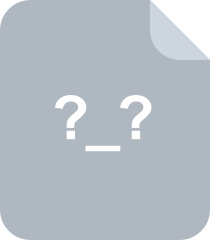
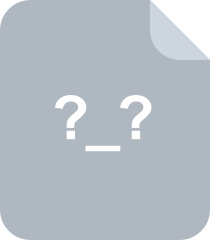
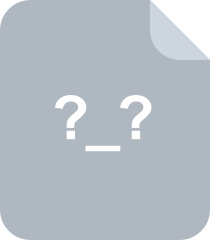
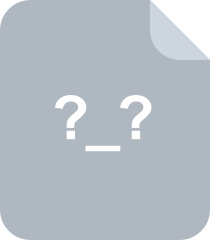
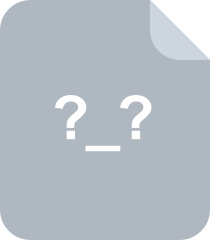
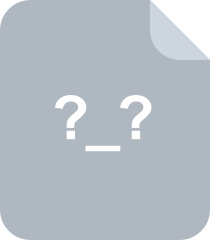
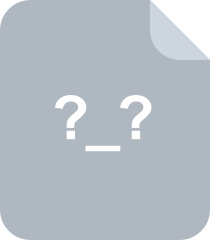
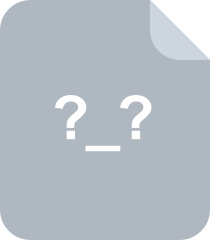
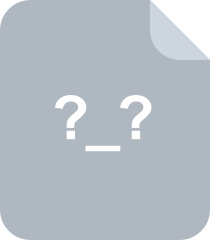
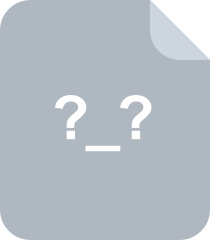
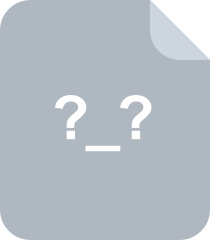
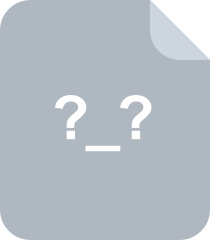
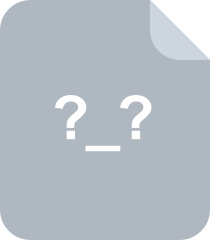
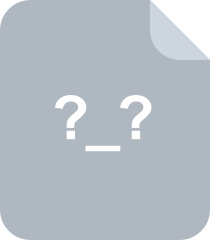
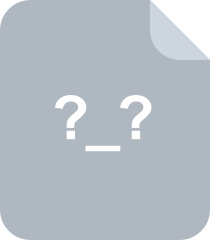
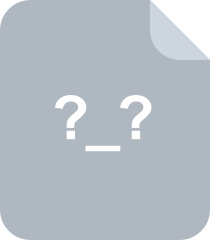
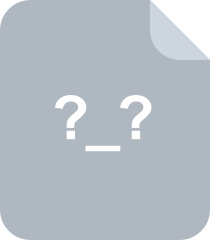
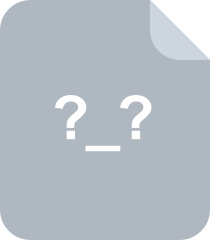
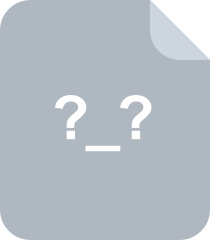
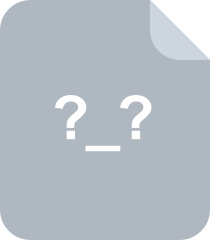
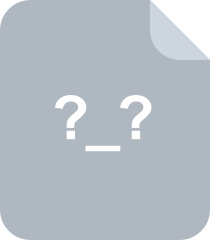
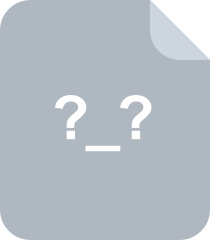
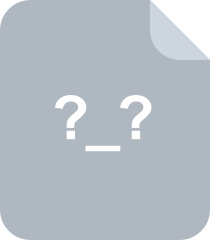
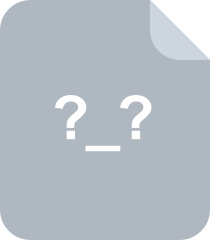
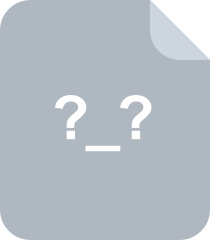
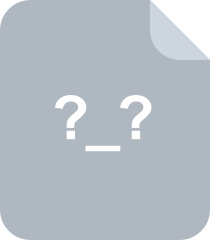
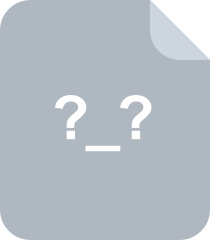
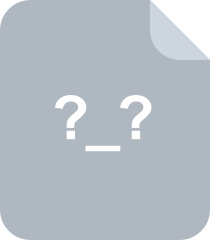
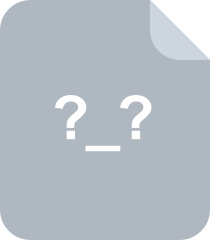
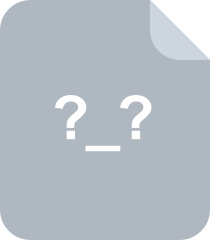
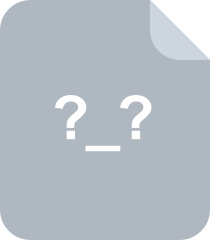
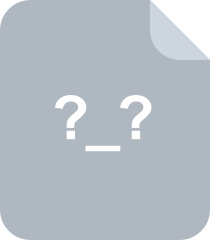
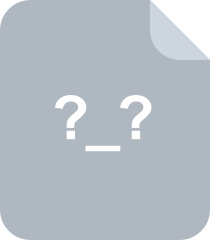
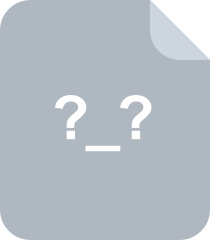
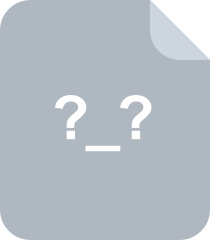
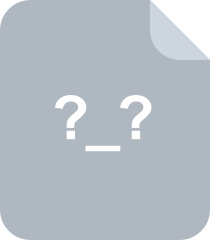
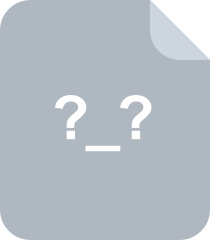
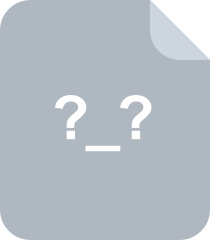
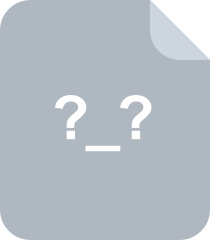
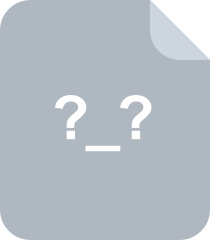
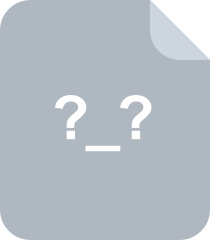
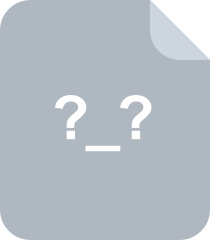
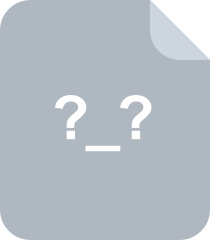
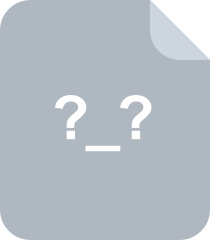
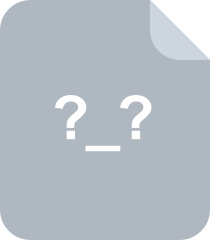
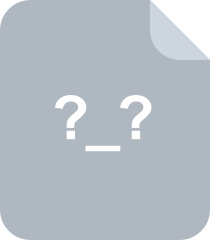
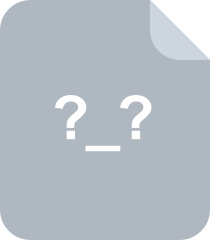
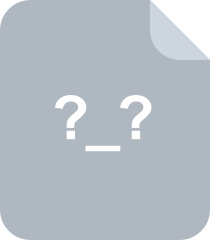
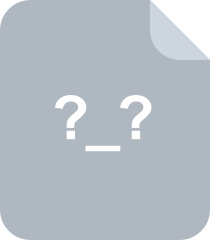
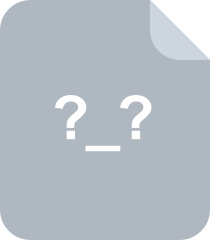
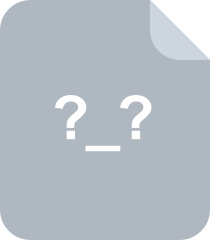
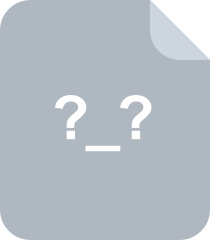
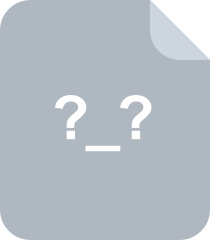
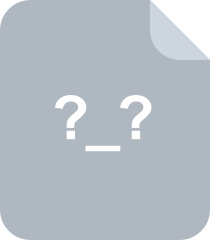
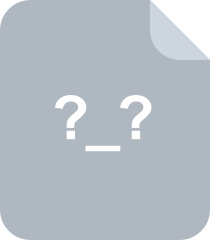
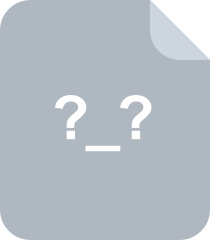
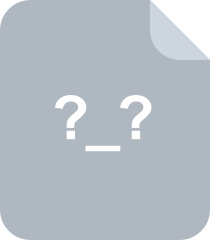
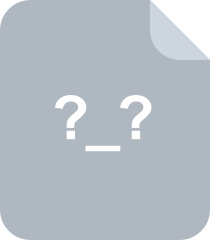
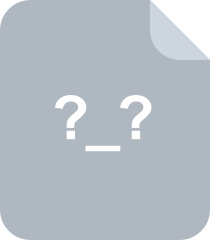
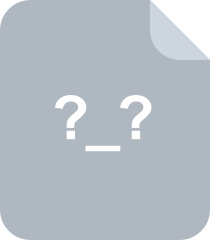
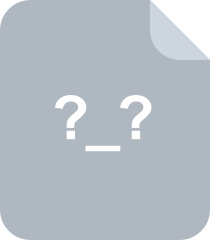
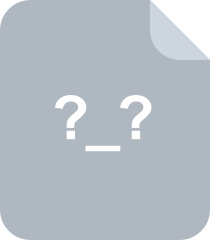
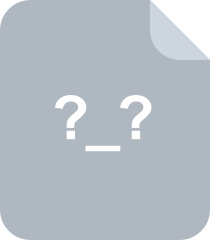
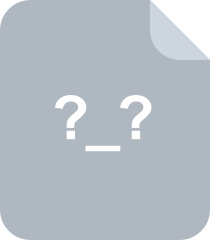
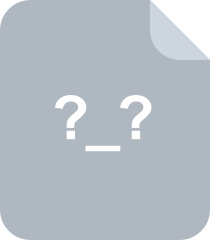
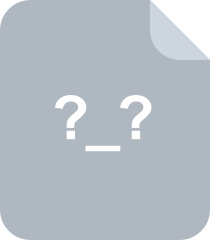
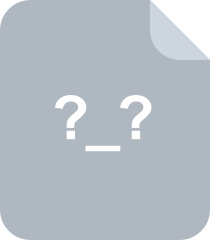
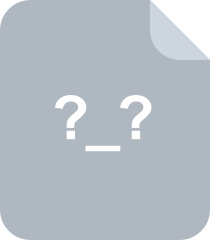
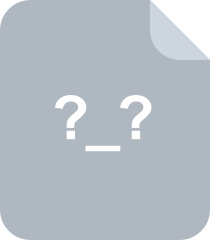
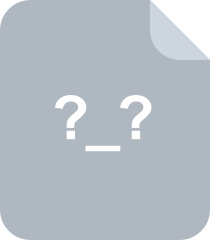
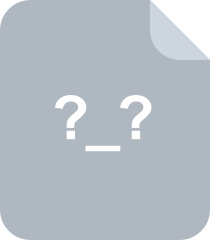
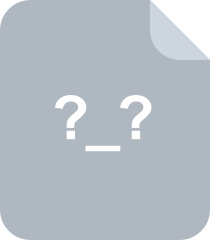
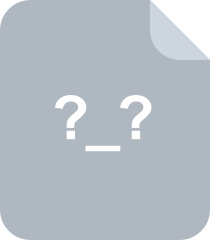
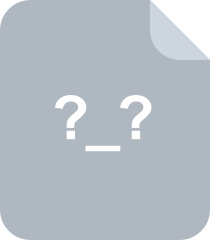
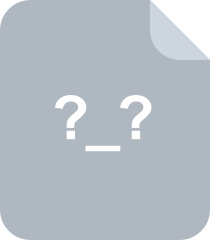
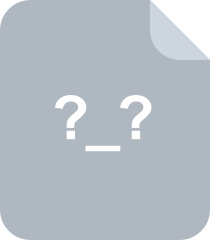
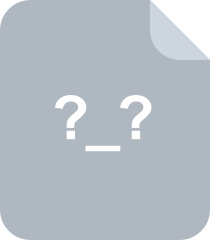
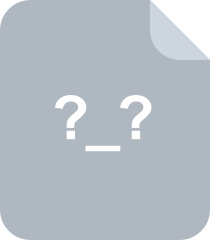
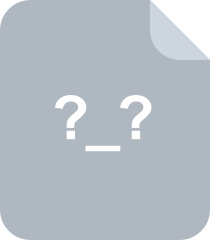
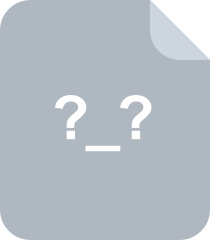
共 536 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
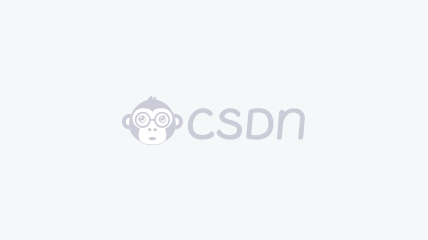
- weixin_464453822024-06-15资源很实用,对我启发很大,有很好的参考价值,内容详细。
- qq_580323712024-06-10发现一个宝藏资源,资源有很高的参考价值,赶紧学起来~

Ai医学图像分割
- 粉丝: 2w+
- 资源: 2128
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

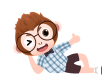
最新资源
- apache-maven-3.6.1-bin.zip
- c593f5fc-d4a7-4b43-8ab2-51afc90f3f62
- IIR滤波器参数计算函数
- WPF树菜单拖拽功能,下级目录拖到上级目录,上级目录拖到下级目录.zip
- CDH6.3.2版本hive2.1.1修复HIVE-14706后的jar包
- 鸿蒙项目实战-天气项目(当前城市天气、温度、湿度,24h天气,未来七天天气预报,生活指数,城市选择等)
- Linux环境下oracle数据库服务器配置中文最新版本
- Linux操作系统中Oracle11g数据库安装步骤详细图解中文最新版本
- SMA中心接触件插合力量(插入力及分离力)仿真
- 变色龙记事本,有NPP功能,JSONview功能
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


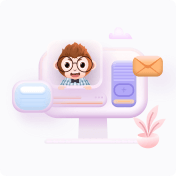
安全验证
文档复制为VIP权益,开通VIP直接复制
