package com.situ.school.servlet;
import java.io.IOException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.situ.school.entity.Cclass;
import com.situ.school.entity.Course;
import com.situ.school.entity.Student;
import com.situ.school.util.JDBCUtil;
import com.situ.school.util.JSONResult;
import com.situ.school.util.JSONUtil;
import com.situ.school.vo.CclassCount;
@WebServlet("/cclass")
public class CclassServlet extends HttpServlet{
@Override
protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setCharacterEncoding("UTF-8");
String method = req.getParameter("method");
// 如果没有传递method参数,默认执行查找所有
if (method == null || "".equals(method)) {
//method = "findAll";
method = "selectAll";
}
switch (method) {
case "selectAll":
selectAll(req, resp);
break;
case "del":
del(req, resp);
break;
case "add":
add(req, resp);
break;
case "getUpdatePage":
getUpdatePage(req, resp);
break;
case "update":
update(req, resp);
break;
case "selectCclassCount":
selectCclassCount(req, resp);
break;
default:
break;
}
}
private void selectCclassCount(HttpServletRequest req, HttpServletResponse resp) {
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
ArrayList<CclassCount> list = new ArrayList<CclassCount>();
// 加载驱动
try { // DBUtils
connection = JDBCUtil.getConnection();
String sql = "SELECT d.name,COUNT(*) as count FROM student as e INNER JOIN cclass as d ON e.code_dept = d.`code` GROUP BY d.`code` ;";
preparedStatement = connection.prepareStatement(sql);
resultSet = preparedStatement.executeQuery();
System.out.println(preparedStatement);
// 判断下一行有没有,有返回true,并指向这一行,没有返回false
while (resultSet.next()) {
String name = resultSet.getString("name");
int count = resultSet.getInt("count");
CclassCount cclassCount = new CclassCount(name, count);
list.add(cclassCount);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
JDBCUtil.close(connection, preparedStatement, resultSet);
}
for (CclassCount cclassCount : list) {
System.out.println(cclassCount);
}
JSONResult jsonResult = JSONResult.success(list);
JSONUtil.obj2Json(jsonResult, resp);
}
private void update(HttpServletRequest req, HttpServletResponse resp) {
String id = req.getParameter("id");
String code = req.getParameter("code");
String name = req.getParameter("name");
String acad = req.getParameter("acad");
Connection connection = null;
PreparedStatement preparedStatement = null;
int count = 0;
try {
connection = JDBCUtil.getConnection();
String sql = "update cclass set code=?,name=?,acad=? where id=?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1, code);
preparedStatement.setString(2, name);
preparedStatement.setString(3, acad);
preparedStatement.setInt(4, Integer.parseInt(id));
count = preparedStatement.executeUpdate();
System.out.println(preparedStatement);
} catch (SQLException e) {
e.printStackTrace();
} finally {
JDBCUtil.close(connection, preparedStatement, null);
}
if (count == 1) {
JSONResult jsonResult = JSONResult.success("修改成功");
JSONUtil.obj2Json(jsonResult, resp);
} else {
JSONResult jsonResult = JSONResult.error("修改失败");
JSONUtil.obj2Json(jsonResult, resp);
}
}
// 通过转发的方式跳转到update.jsp页面
private void getUpdatePage(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String updateId = req.getParameter("id");
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
Cclass cclass = null;
// 加载驱动
try { // DBUtils
connection = JDBCUtil.getConnection();
String sql = "select id,code,name,acad from cclass where id=?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setInt(1, Integer.parseInt(updateId));
resultSet = preparedStatement.executeQuery();
System.out.println(preparedStatement);
// 判断下一行有没有,有返回true,并指向这一行,没有返回false
while (resultSet.next()) {
int id = resultSet.getInt("id");
String code = resultSet.getString("code");
String name = resultSet.getString("name");
String acad = resultSet.getString("acad");
cclass = new Cclass(id, code, name, acad);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
JDBCUtil.close(connection, preparedStatement, resultSet);
}
req.setAttribute("cclass", cclass);
req.getRequestDispatcher("/web/page/cclass/update.jsp").forward(req, resp);
}
private void add(HttpServletRequest req, HttpServletResponse resp) {
String code = req.getParameter("code");
String name = req.getParameter("name");
String acad = req.getParameter("acad");
Connection connection = null;
PreparedStatement preparedStatement = null;
int count = 0;
try {
connection = JDBCUtil.getConnection();
String sql = "INSERT INTO cclass(code,`name`,acad) VALUES(?,?,?)";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1, code);
preparedStatement.setString(2, name);
preparedStatement.setString(3, acad);
count = preparedStatement.executeUpdate();
System.out.println(preparedStatement);
} catch (SQLException e) {
e.printStackTrace();
} finally {
JDBCUtil.close(connection, preparedStatement, null);
}
if (count == 1) {
JSONResult jsonResult = JSONResult.success("添加成功");
JSONUtil.obj2Json(jsonResult, resp);
} else {
JSONResult jsonResult = JSONResult.error("添加失败");
JSONUtil.obj2Json(jsonResult, resp);
}
}
private void del(HttpServletRequest req, HttpServletResponse resp) {
System.out.println("CclassServlet.del()");
String code = req.getParameter("code");
Connection connection = null;
PreparedStatement preparedStatement = null;
int count = 0;
try {
connection = JDBCUtil.getConnection();
String sql = "delete from cclass where code=?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1, code);
count = preparedStatement.executeUpdate();
System.out.println(preparedStatement);
} catch (SQLException e) {
e.printStackTrace();
} finally {
JDBCUtil.close(connection, preparedStatement, null);
}
if (count == 1) {
JSONResult jsonResult = JSONResult.success("删除成功");
JSONUtil.obj2Json(jsonResult, resp);
} else {
JSONResult jsonResult = JSONResult.error("删除失败");
JSONUtil.obj2Json(jsonResult, resp);
}
}
private void selectAll(HttpServletRequest req, HttpServletResponse resp) {
System.out.println("CclassServlet.selectAll()");
// 得到搜索的名字
String searchName = req.getParameter("name");
if (searchName == null) {
searchName = "";
}
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
ArrayList<Cclass> list = new ArrayList<Cclass>();
// 加载驱动
try { // DBUtils
connection = JDBCUtil.getConnection();
String sql = "select id,code,name,acad from cclass where name like ?";
prepare
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
对于信息管理系统的一系列要求,对系统提出以下要求: (1)需要进行身份认证登录。学生登录系统后可以进行信息更换、增加、删除,并查询自己的考试成绩;后台操作人员登录系统后,可以根据操作人员所属角色进行指定的系统操作。 (2)具备数据管理功能。数据管理主要是对班级基本信息、学生基本信息、课程基本信息、成绩信息等基础数据进行的管理和维护工作。 (3)具备查询访问计数功能。查询前台网站当前总访问人数和当日访问人数。(此功能待开发) (4)学生参加过完考试后,系统将记录学生考试成绩等信息,系统应具备按班级或按学生查询考试成绩的功能。 (5)具备Excel导出功能。可以将学生信息查询结果、学生成绩等导出到Excel中,供打印输出保存之用。 (6)统计分析功能。统计每个分数段的学生人数,并能够查看该分数段的具体学生信息。 (7)系统运行在Windows平台上,要有较好的图形用户界面。 (8)系统应该有很好的扩展性,在已实现功能的基础上可以扩展其他功能。
资源推荐
资源详情
资源评论
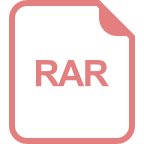
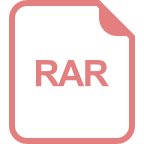
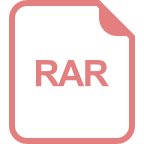
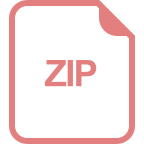
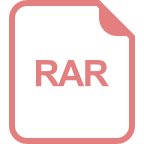
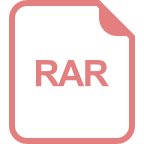
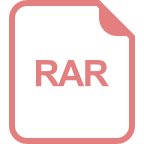
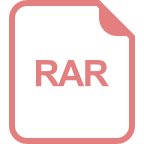
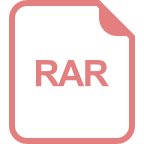
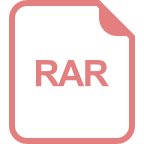
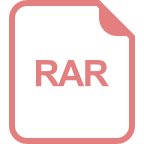
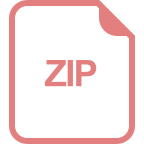
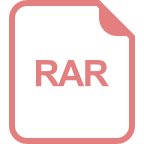
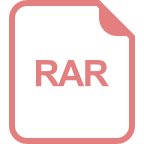
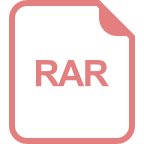
收起资源包目录

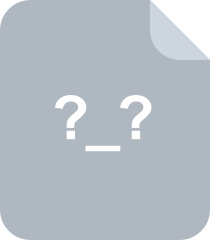
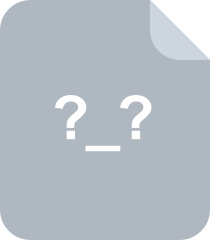
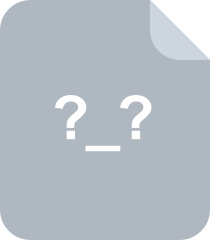
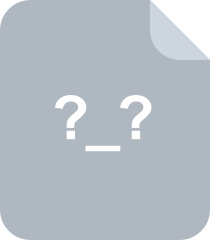
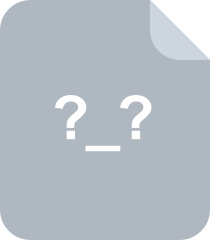
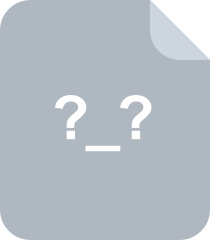
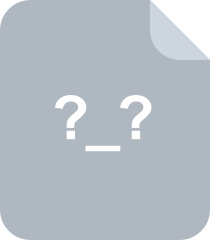
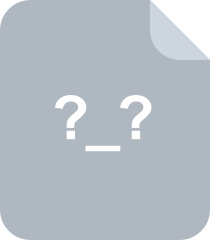
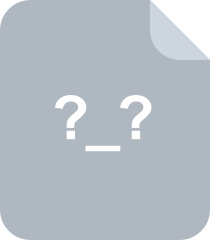
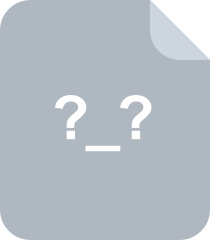
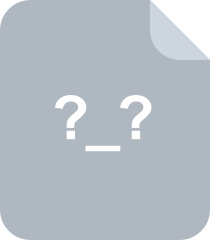
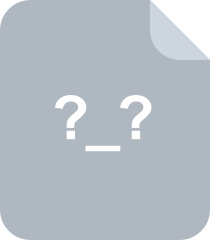
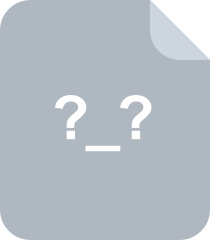
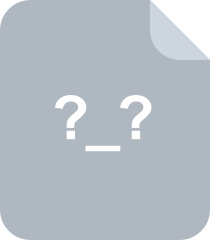
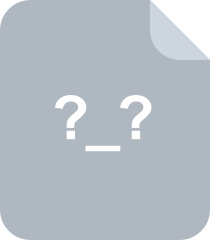
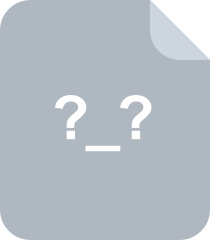
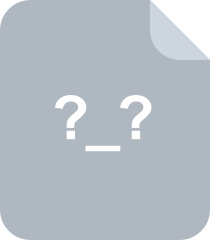
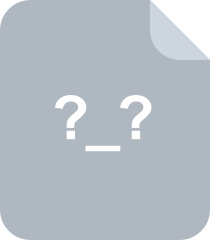
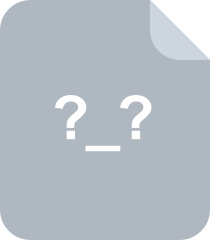
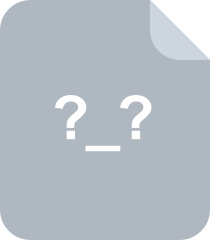
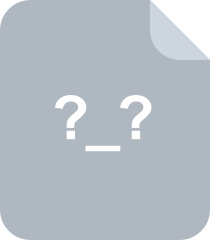
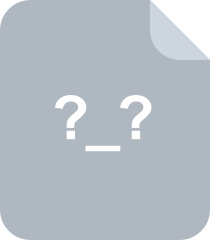
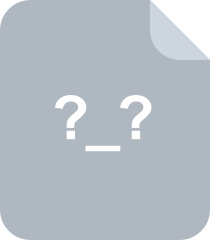
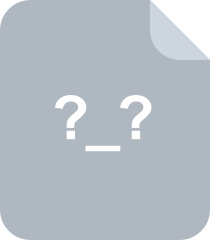
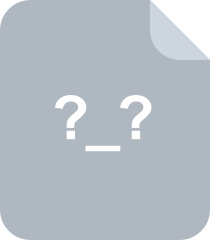
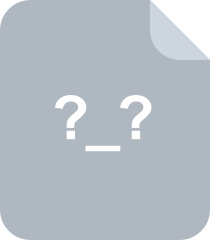
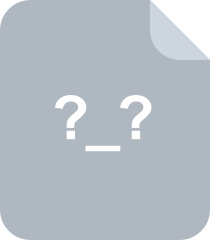
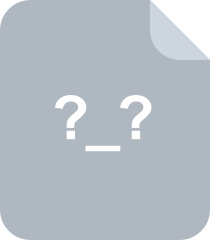
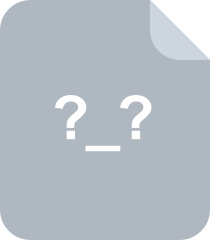
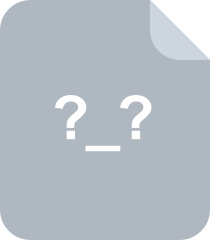
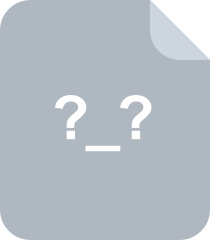
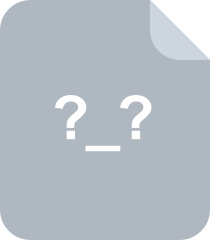
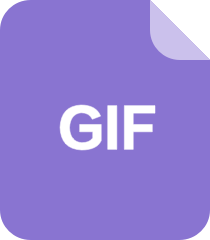
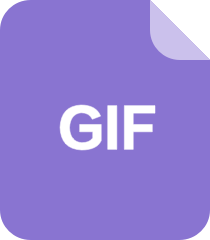
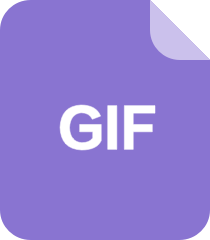
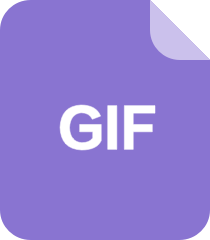
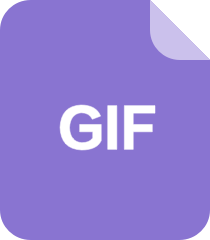
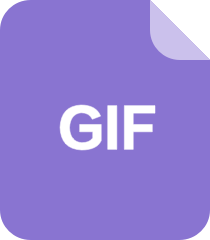
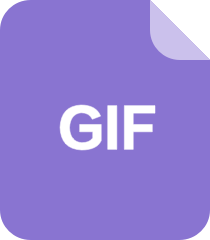
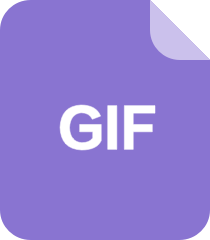
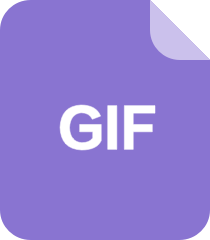
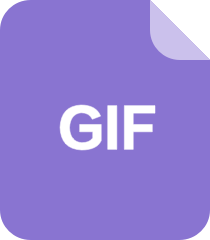
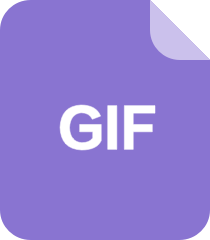
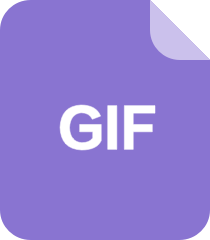
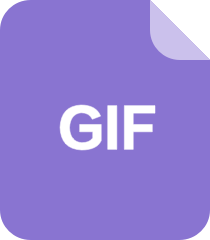
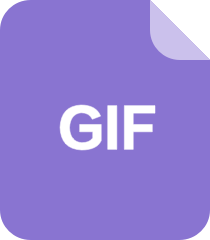
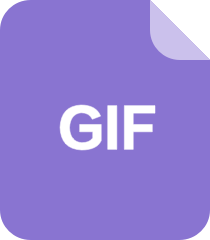
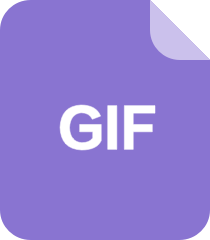
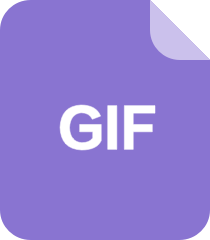
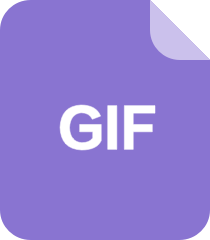
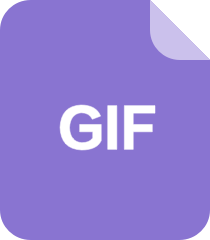
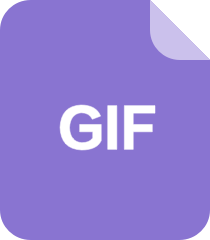
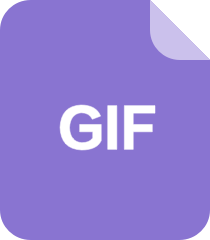
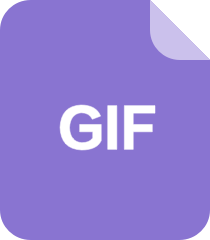
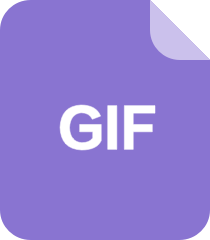
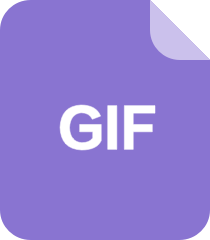
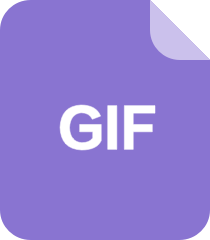
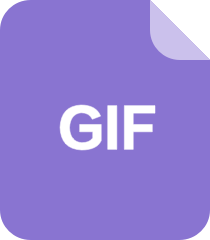
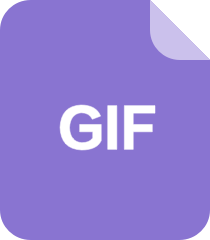
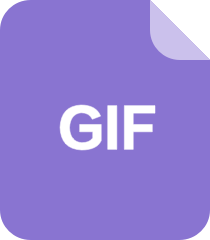
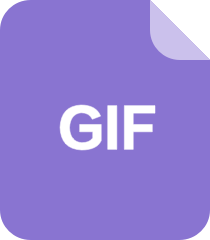
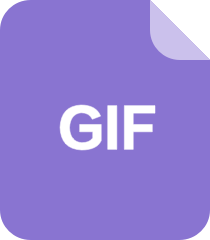
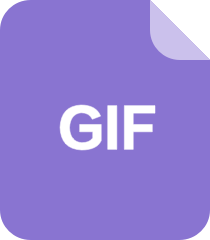
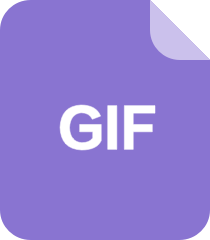
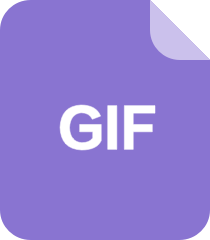
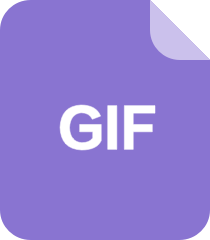
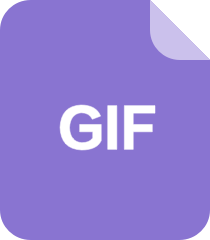
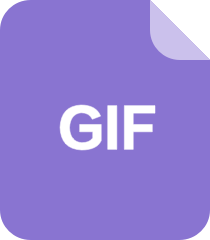
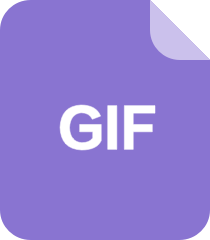
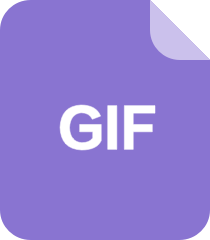
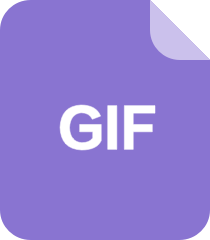
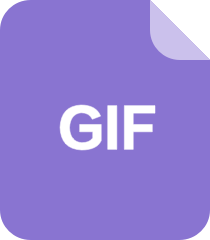
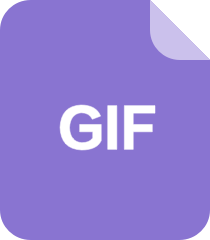
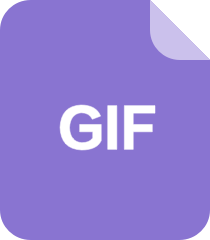
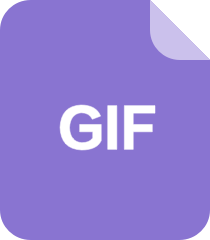
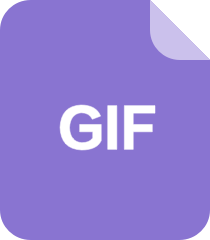
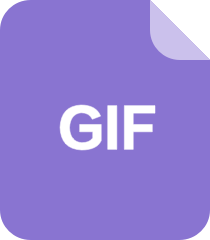
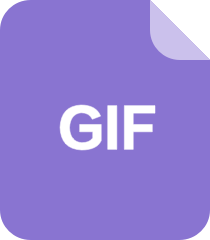
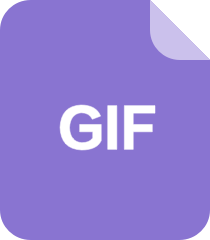
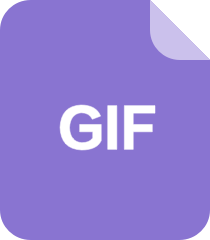
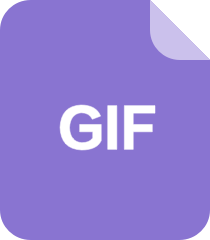
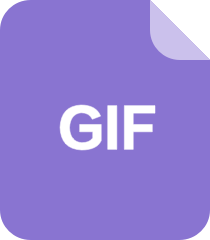
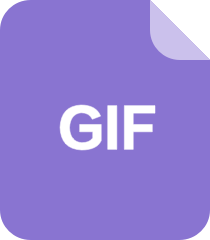
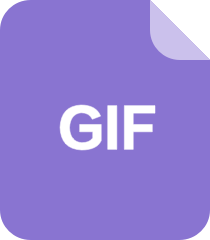
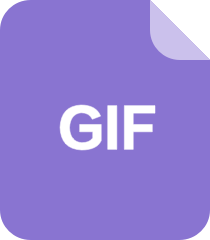
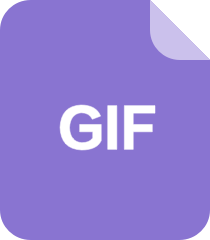
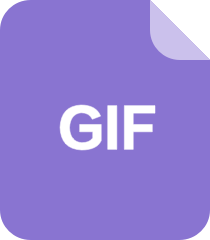
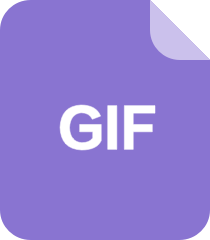
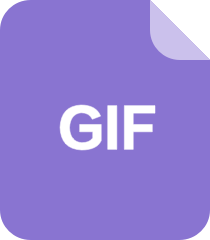
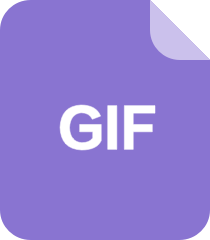
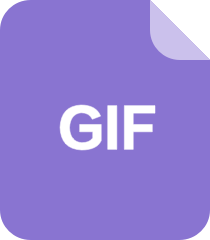
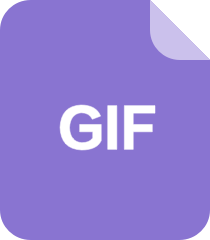
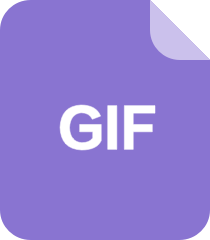
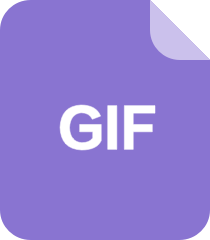
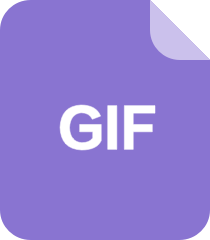
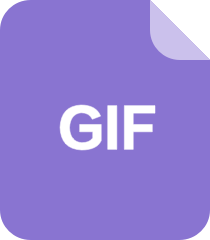
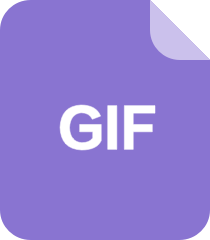
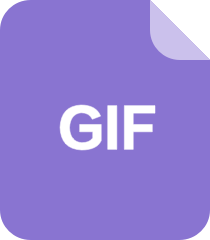
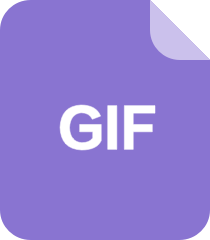
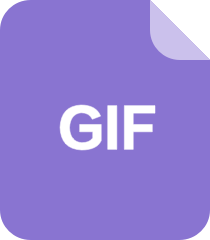
共 205 条
- 1
- 2
- 3

ZZ--瑞hopeACMer
- 粉丝: 50
- 资源: 7

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

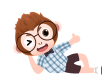
最新资源
- C#通用固定资产管理系统源码带二维码数据库 SQL2008源码类型 WebForm
- Matlab 建立了并具有数字调压功能的空间矢量脉宽调制SVPWM逆变器仿真
- 毕业设计-毕业设计&课设-推荐系统项目:含协同过滤、矩阵分解等算法及相关代码
- 电力系统继电保护整定及其应用-发电机组与变压器保护
- C++极速内存池、跨平台高效C++内存池
- C#WMS仓库管理系统源码 WMS仓储管理系统源码数据库 SQL2008源码类型 WinForm
- 基于51单片机电子称称重压力检测阈值报警系统设计.zip
- C#ASP.NET工程项目管理源码带文档数据库 SQL2008源码类型 WebForm
- 20210203047_卢诗贝_大数据1班.rar
- GL《装成偏执狂攻略清冷师尊翻车后》作者:酸辣离蒿.txt
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


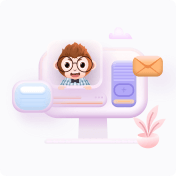
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页