# Copyright (c) 2020-2022 by Fraunhofer Institute for Energy Economics
# and Energy System Technology (IEE), Kassel, and University of Kassel. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be found in the LICENSE file.
import numpy as np
import pandas as pd
from pandapipes.component_models import Junction, Sink, Source, Pump, Pipe, ExtGrid, \
HeatExchanger, Valve, CirculationPumpPressure, CirculationPumpMass, PressureControlComponent, \
Compressor
from pandapipes.component_models.component_toolbox import add_new_component
from pandapipes.pandapipes_net import pandapipesNet, get_basic_net_entries, add_default_components
from pandapipes.properties import call_lib
from pandapipes.properties.fluids import Fluid, _add_fluid_to_net
from pandapipes.std_types.std_types import add_basic_std_types, create_pump_std_type, \
load_std_type
from pandapipes.std_types.std_type_class import regression_function, PumpStdType
from pandapower.create import _get_multiple_index_with_check, _get_index_with_check, _set_entries, \
_check_node_element, _check_multiple_node_elements, _set_multiple_entries, \
_add_multiple_branch_geodata, _check_branch_element, _check_multiple_branch_elements
try:
import pandaplan.core.pplog as logging
except ImportError:
import logging
logger = logging.getLogger(__name__)
def create_empty_network(name="", fluid=None, add_stdtypes=True):
"""
This function initializes the pandapipes datastructure.
:param name: Name for the network
:type name: string, default None
:param fluid: A fluid that can be added to the net from the start. Should be either of type\
Fluid (c.f. pandapipes.properties.fluids.Fluid) or a string which refers to a standard\
fluid type used to call `create_fluid_from_lib`. A fluid is required for pipeflow\
calculations, but can also be added later.
:type fluid: Fluid or str, default None
:param add_stdtypes: Flag whether to add a dictionary of typical pump and pipe std types
:type add_stdtypes: bool, default True
:return: net - pandapipesNet with empty tables
:rtype: pandapipesNet
:Example:
>>> net1 = create_empty_network("my_first_pandapipesNet", "lgas")
>>> net2 = create_empty_network()
"""
net = pandapipesNet(get_basic_net_entries())
add_default_components(net, True)
net['name'] = name
if add_stdtypes:
add_basic_std_types(net)
if fluid is not None:
if isinstance(fluid, Fluid):
net["fluid"] = fluid
elif isinstance(fluid, str):
create_fluid_from_lib(net, fluid)
else:
logger.warning("The fluid %s cannot be added to the net Only fluids of type Fluid or "
"strings can be used." % fluid)
return net
def create_junction(net, pn_bar, tfluid_k, height_m=0, name=None, index=None, in_service=True,
type="junction", geodata=None, **kwargs):
"""
Adds one junction in table net["junction"]. Junctions are the nodes of the network that
all other elements connect to.
:param net: The pandapipes network in which the element is created
:type net: pandapipesNet
:param pn_bar: The nominal pressure in [bar]. Used as an initial value for pressure calculation.
:type pn_bar: float
:param tfluid_k: The fluid temperature in [K]. Used as parameter for gas calculations and as\
initial value for temperature calculations.
:type tfluid_k: float
:param height_m: Height of node above sea level in [m]
:type height_m: float, default 0
:param name: The name for this junction
:type name: string, default None
:param index: Force a specified ID if it is available. If None, the index one higher than the\
highest already existing index is selected.
:type index: int, default None
:param in_service: True for in_service or False for out of service
:type in_service: boolean, default True
:param type: not used yet - Designed for type differentiation on pandas lookups (e.g. household\
connection vs. crossing)
:type type: string, default "junction"
:param geodata: Coordinates used for plotting
:type geodata: (x,y)-tuple, default None
:param kwargs: Additional keyword arguments will be added as further columns to the\
net["junction"] table
:return: index - The unique ID of the created element
:rtype: int
:Example:
>>> create_junction(net, pn_bar=5, tfluid_k=320)
"""
add_new_component(net, Junction)
index = _get_index_with_check(net, "junction", index)
cols = ["name", "pn_bar", "tfluid_k", "height_m", "in_service", "type"]
vals = [name, pn_bar, tfluid_k, height_m, bool(in_service), type]
_set_entries(net, "junction", index, **dict(zip(cols, vals)), **kwargs)
if geodata is not None:
if len(geodata) != 2:
raise UserWarning("geodata must be given as (x, y) tuple")
net["junction_geodata"].loc[index, ["x", "y"]] = geodata
return index
def create_sink(net, junction, mdot_kg_per_s, scaling=1., name=None, index=None, in_service=True,
type='sink', **kwargs):
"""
Adds one sink in table net["sink"].
:param net: The net for which this sink should be created
:type net: pandapipesNet
:param junction: The index of the junction to which the sink is connected
:type junction: int
:param mdot_kg_per_s: The required mass flow
:type mdot_kg_per_s: float, default None
:param scaling: An optional scaling factor to be set customly
:type scaling: float, default 1
:param name: A name tag for this sink
:type name: str, default None
:param index: Force a specified ID if it is available. If None, the index one higher than the\
highest already existing index is selected.
:type index: int, default None
:param in_service: True for in service, False for out of service
:type in_service: bool, default True
:param type: Type variable to classify the sink
:type type: str, default None
:param kwargs: Additional keyword arguments will be added as further columns to the\
net["sink"] table
:return: index - The unique ID of the created element
:rtype: int
:Example:
>>> new_sink_id = create_sink(net, junction=2, mdot_kg_per_s=0.1)
"""
add_new_component(net, Sink)
_check_junction_element(net, junction)
index = _get_index_with_check(net, "sink", index)
cols = ["name", "junction", "mdot_kg_per_s", "scaling", "in_service", "type"]
vals = [name, junction, mdot_kg_per_s, scaling, bool(in_service), type]
_set_entries(net, "sink", index, **dict(zip(cols, vals)), **kwargs)
return index
def create_source(net, junction, mdot_kg_per_s, scaling=1., name=None, index=None, in_service=True,
type='source', **kwargs):
"""
Adds one source in table net["source"].
:param net: The net for which this source should be created
:type net: pandapipesNet
:param junction: The index of the junction to which the source is connected
:type junction: int
:param mdot_kg_per_s: The required mass flow
:type mdot_kg_per_s: float, default None
:param scaling: An optional scaling factor to be set customly
:type scaling: float, default 1
:param name: A name tag for this source
:type name: str, default None
:param index: Force a specified ID if it is available. If None, the index one higher than the\
highest already existing index is selected.
:type index: int, default None
:param in_service: True for in service, False for out of service
:type in_service: bool, default True
:param type: Type variable to classify the source
:type type: str, default None
:param kwargs: Additional keyword arguments will be added as further columns to the\
n
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
这是一个管道流量计算工具,在模拟多能源电网(尤其是热力和燃气管网)时对pandapower起到补充作用。更多信息可以在www.pandapipes.org找到。 开始使用: 安装说明 证明文件 github教程 活页夹互动教程 pandapipes是卡塞尔弗劳恩霍夫能源经济学和能源系统技术研究所(IEE)配电系统运行系和卡塞尔大学能源管理和电力系统运行研究小组的发展成果。
资源推荐
资源详情
资源评论
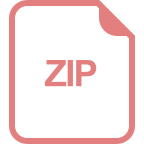
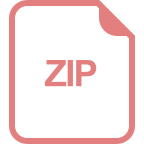
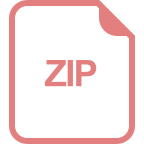
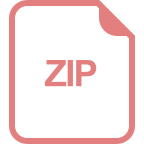
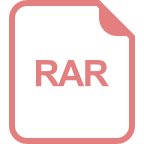
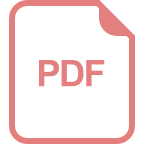
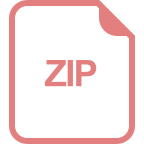
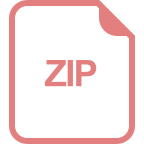
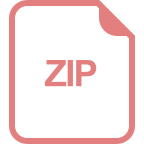
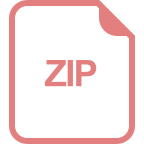
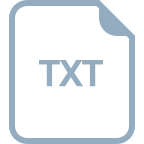
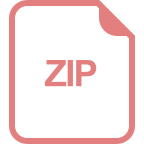
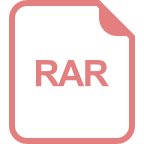
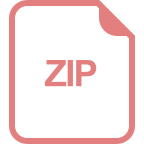
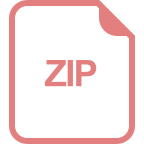
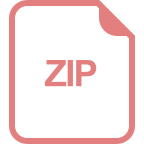
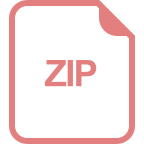
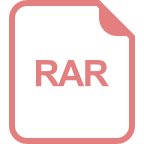
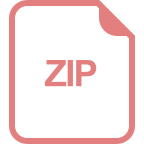
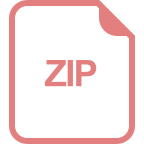
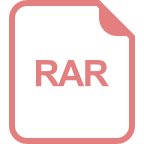
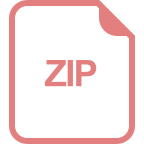
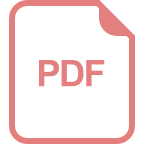
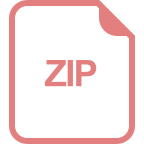
收起资源包目录

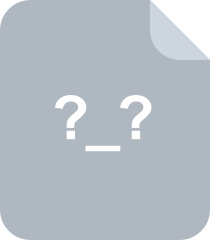
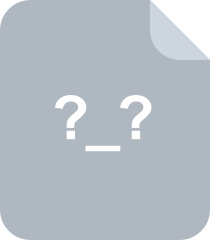
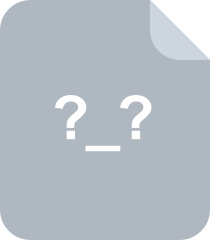
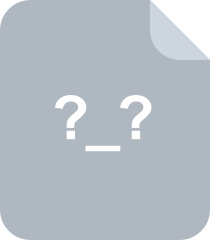
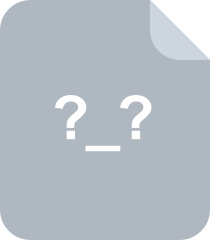
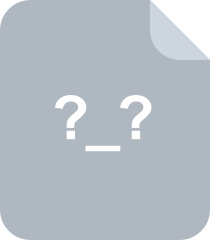
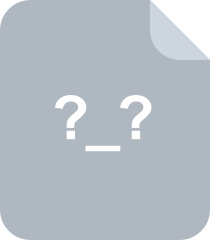
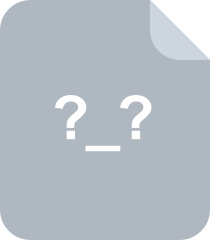
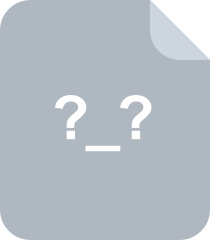
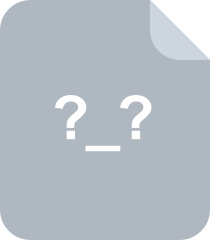
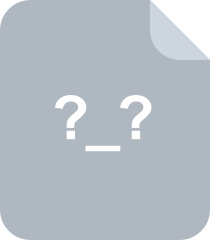
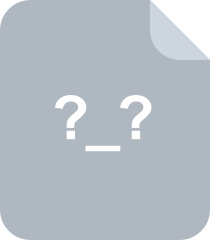
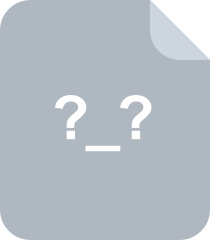
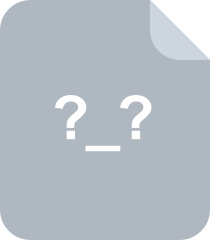
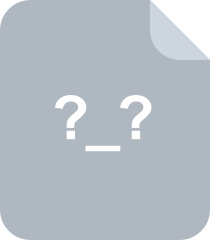
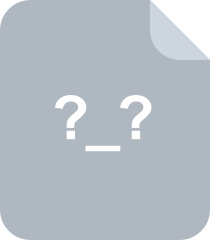
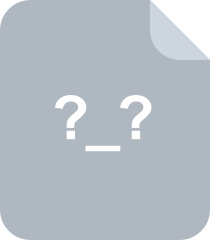
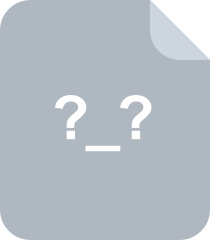
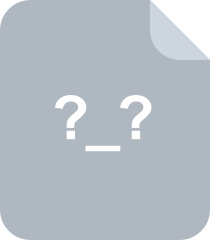
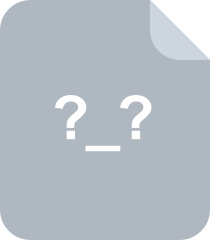
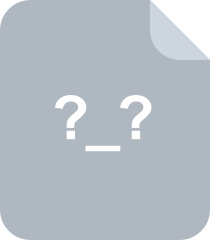
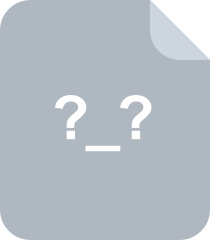
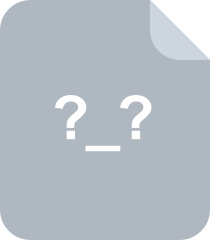
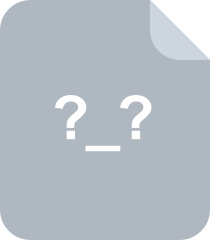
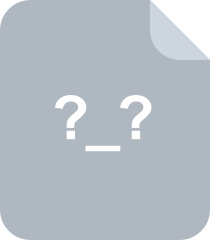
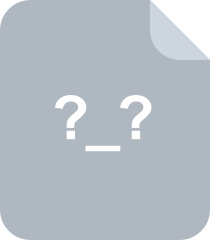
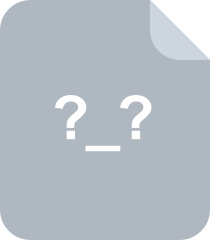
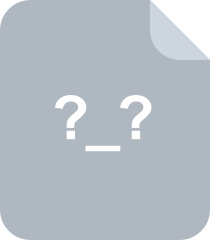
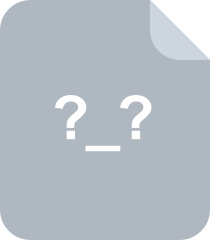
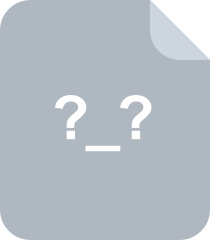
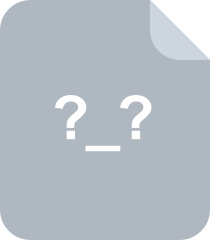
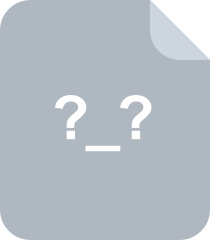
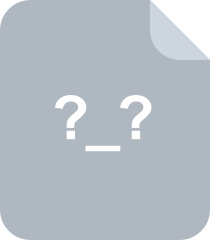
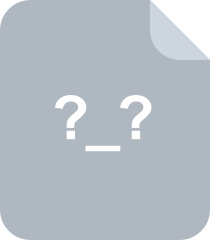
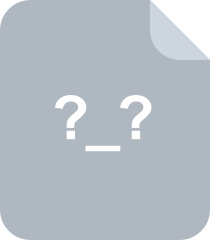
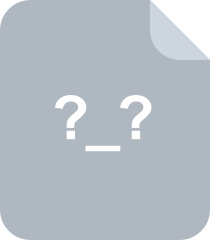
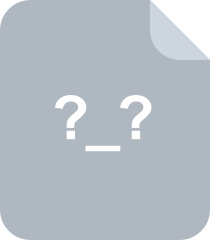
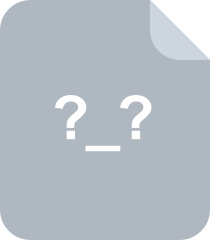
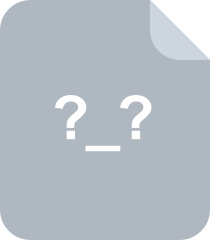
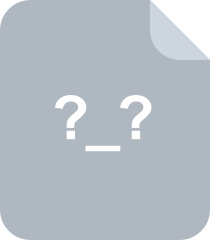
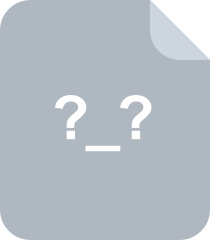
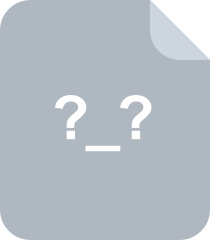
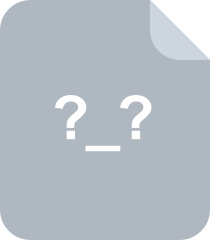
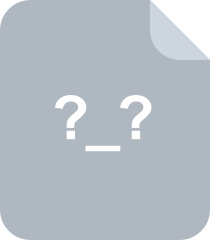
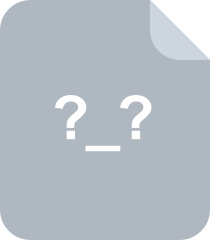
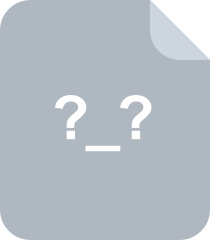
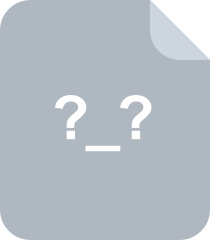
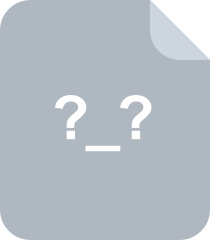
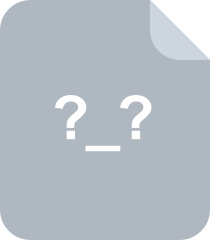
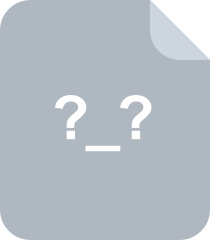
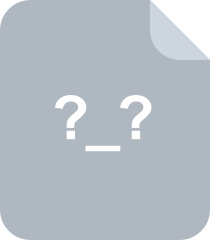
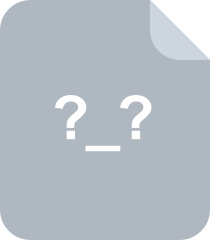
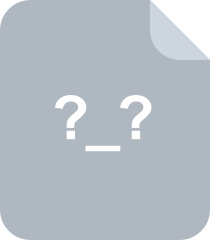
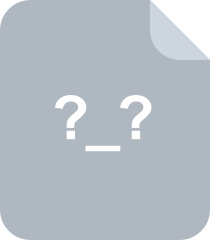
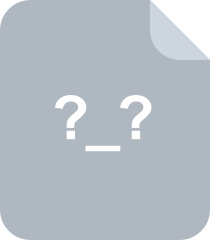
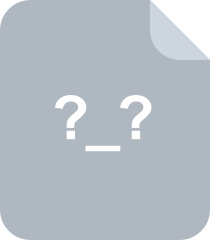
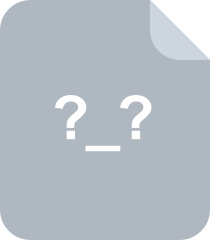
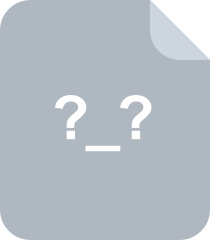
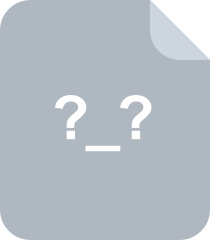
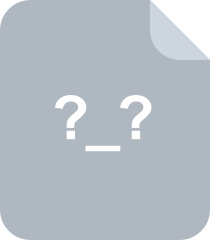
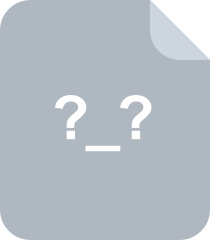
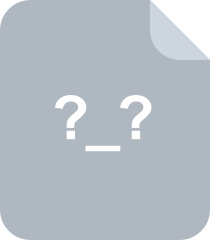
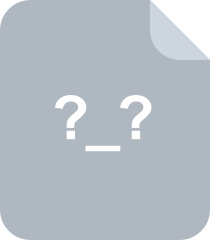
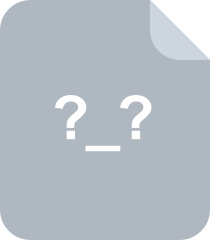
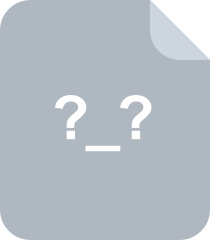
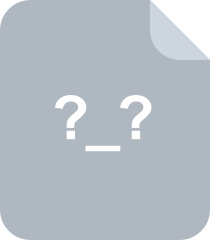
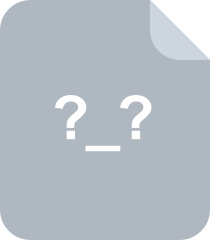
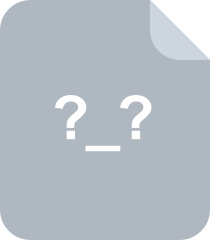
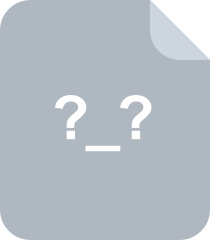
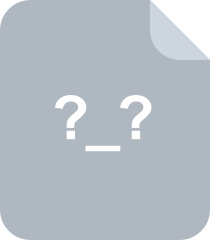
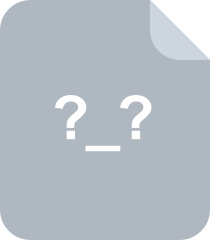
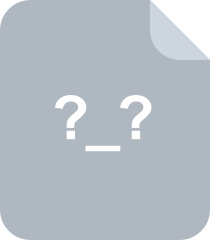
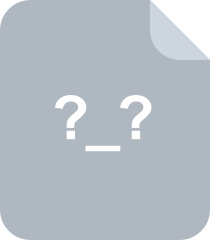
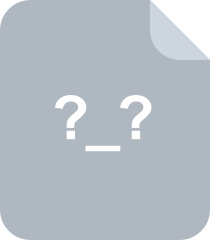
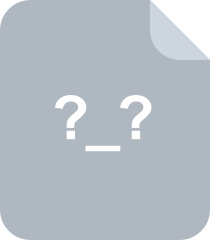
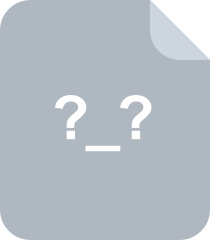
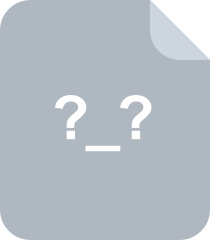
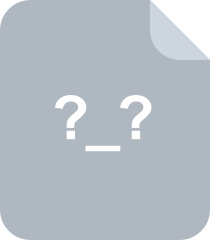
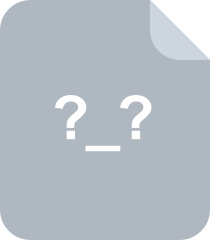
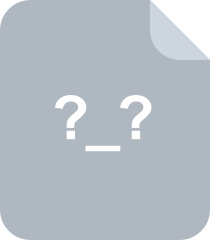
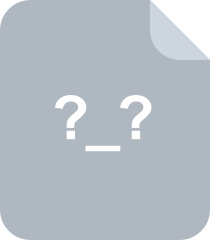
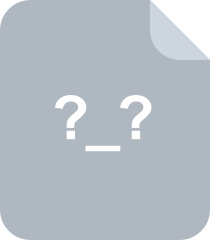
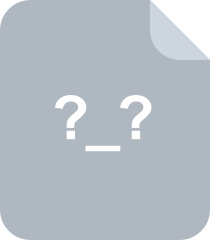
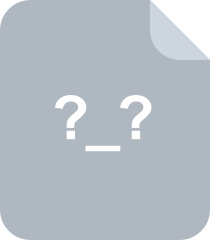
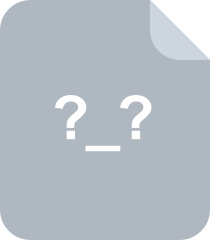
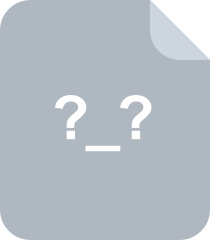
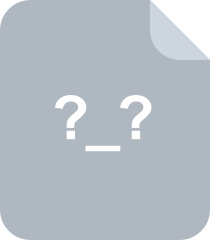
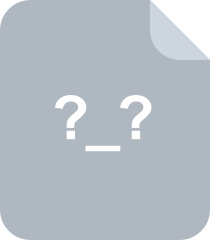
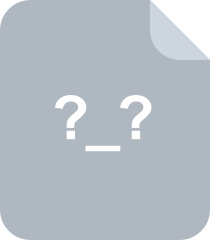
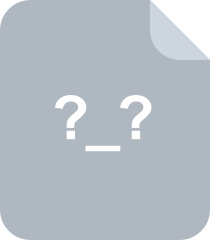
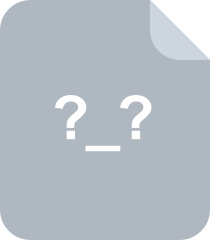
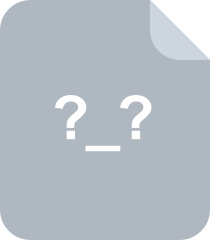
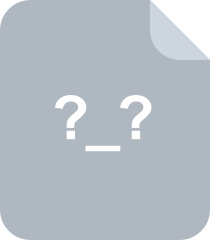
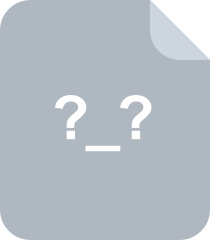
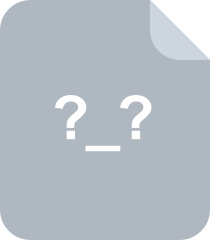
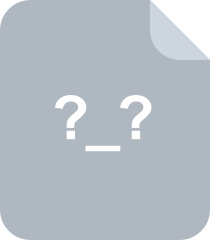
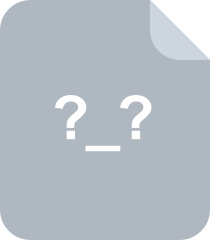
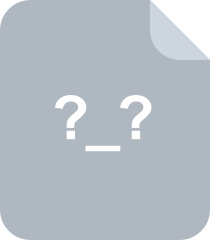
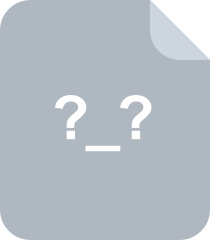
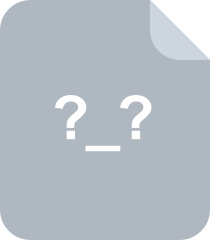
共 530 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
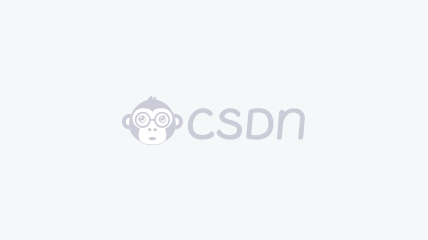

小王学知识
- 粉丝: 33
- 资源: 24
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

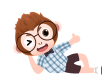
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


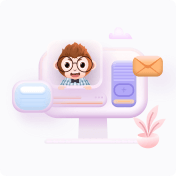
安全验证
文档复制为VIP权益,开通VIP直接复制
