cocur/slugify
=============
> Converts a string into a slug.
[](https://travis-ci.org/cocur/slugify)
[](https://ci.appveyor.com/project/florianeckerstorfer/slugify)
[](https://scrutinizer-ci.com/g/cocur/slugify/)
[](https://scrutinizer-ci.com/g/cocur/slugify/?branch=master)
[](https://packagist.org/packages/cocur/slugify)
[](http://opensource.org/licenses/MIT)
[](https://packagist.org/packages/cocur/slugify)
Developed by [Florian Eckerstorfer](https://florian.ec) in Vienna, Europe with the help of
[many great contributors](https://github.com/cocur/slugify/graphs/contributors).
Features
--------
- Removes all special characters from a string.
- Provides custom replacements for German, French, Spanish, Russian, Ukrainian, Polish, Czech, Latvian, Greek,
Esperanto¹, Arabian, Vietnamese, Burmese, Danish, Turkish, Finnish, Swedish, and Georgian special characters. Instead of
removing these characters, Slugify approximates them (e.g., `ae` replaces `ä`).
- No external dependencies.
- PSR-4 compatible.
- Compatible with PHP >= 5.5.9, PHP 7 and [HHVM](http://hhvm.com).
- Integrations for [Symfony2](http://symfony.com), [Silex](http://silex.sensiolabs.org), [Laravel](http://laravel.com),
[Twig](http://twig.sensiolabs.org), [Zend Framework 2](http://framework.zend.com/), [Nette Framework](http://nette.org/),
[Latte](http://latte.nette.org/) and [Plum](https://github.com/plumphp/plum).
¹ Some Esperanto transliterations conflict with others. You need to enable the Esperanto ruleset to use these transliterations.
Installation
------------
You can install Slugify through [Composer](https://getcomposer.org):
```shell
$ composer require cocur/slugify
```
Usage
-----
> The documentation you can find here has already been updated for the upcoming 2.0 release. If you are using the
v1.4, the latest stable version, please use the corresponding documentation. You can find it
[here](https://github.com/cocur/slugify/tree/1.4).
Generate a slug:
```php
use Cocur\Slugify\Slugify;
$slugify = new Slugify();
echo $slugify->slugify('Hello World!'); // hello-world
```
You can also change the separator used by `Slugify`:
```php
echo $slugify->slugify('Hello World!', '_'); // hello_world
```
The library also contains `Cocur\Slugify\SlugifyInterface`. Use this interface whenever you need to type hint an
instance of `Slugify`.
To add additional transliteration rules you can use the `addRule()` method.
```php
$slugify->addRule('i', 'ey');
echo $slugify->slugify('Hi'); // hey
```
### Rulesets
Many of the transliterations rules used in Slugify are specific to a language. These rules are therefore categorized
using rulesets. Rules for the most popular are activated by default in a specific order. You can change which rulesets
are activated and the order in which they are activated. The order is important when there are conflicting rules in
different languages. For example, in German `ä` is transliterated with `ae`, in Turkish the correct transliteration is
`a`. By default the German transliteration is used since German is used more often on the internet. If you want to use
prefer the Turkish transliteration you have to possibilities. You can activate it after creating the constructor:
```php
$slugify = new Slugify();
$slugify->slugify('ä'); // -> "ae"
$slugify->activateRuleset('turkish');
$slugify->slugify('ä'); // -> "a"
```
An alternative way would be to pass the rulesets and their order to the constructor.
```php
$slugify = new Slugify(['rulesets' => ['default', 'turkish']]);
$slugify->slugify('ä'); // -> "a"
```
You can find a list of the available rulesets in `Resources/rules`.
### More options
The constructor takes an options array, you have already seen the `rulesets` options above. You can also change the
regular expression that is used to replace characters with the separator.
```php
$slugify = new Slugify(['regexp' => '/([^A-Za-z0-9]|-)+/']);
```
*(The regular expression used in the example above is the default one.)*
By default Slugify will convert the slug to lowercase. If you want to preserve the case of the string you can set the
`lowercase` option to false.
```php
$slugify = new Slugify(['lowercase' => false]);
$slugify->slugify('Hello World'); // -> "Hello-World"
```
### Contributing
Feel free to ask for new rules for languages that is not already here.
All you need to do is:
1. Provide transliteration rules for your language, in any form, e.g. `'ї' => 'ji'`
2. Provide some examples of texts transliterated with this rules e.g. `'Україна' => 'Ukrajina'`
### Further information
- [API docs](http://cocur.co/slugify/api/master/)
Integrations
------------
### Symfony2
Slugify contains a Symfony2 bundle and service definition that allow you to use it as a service in your Symfony2
application. The code resides in the `Cocur\Slugify\Bridge\Symfony` namespace and you only need to add the bundle class
to your `AppKernel.php`:
```php
# app/AppKernel.php
class AppKernel extends Kernel
{
public function registerBundles()
{
$bundles = array(
// ...
new Cocur\Slugify\Bridge\Symfony\CocurSlugifyBundle(),
);
// ...
}
// ...
}
```
You can now use the `cocur_slugify` service everywhere in your application, for example, in your controller:
```php
$slug = $this->get('cocur_slugify')->slugify('Hello World!');
```
The bundle also provides an alias `slugify` for the `cocur_slugify` service:
```php
$slug = $this->get('slugify')->slugify('Hello World!');
```
You can set the following configuration settings in `app/config.yml` to adjust the slugify service:
```yaml
cocur_slugify:
lowercase: <boolean>
regexp: <string>
rulesets: { }
```
### Twig
If you use the Symfony2 framework with Twig you can use the Twig filter `slugify` in your templates after you have setup
Symfony2 integrations (see above).
```twig
{{ 'Hällo Wörld'|slugify }}
```
If you use Twig outside of the Symfony2 framework you first need to add the extension to your environment:
```php
use Cocur\Slugify\Bridge\Twig\SlugifyExtension;
use Cocur\Slugify\Slugify;
$twig = new Twig_Environment($loader);
$twig->addExtension(new SlugifyExtension(Slugify::create()));
```
To use the Twig filter with [TwigBridge](https://github.com/rcrowe/TwigBridge) for Laravel, you'll need to add the
Slugify extension using a closure:
```php
// laravel/app/config/packages/rcrowe/twigbridge/config.php
'extensions' => array(
//...
function () {
return new \Cocur\Slugify\Bridge\Twig\SlugifyExtension(\Cocur\Slugify\Slugify::create());
},
),
```
You can find more information about registering extensions in the
[Twig documentation](http://twig.sensiolabs.org/doc/advanced.html#creating-an-extension).
### Silex
Slugify also provides a service provider to integrate into Silex.
```php
$app->register(new Cocur\Slugify\Bridge\Silex\SlugifyServiceProvider());
```
You can use the `slugify` method in your controllers:
```php
$app->get('/', function () {
return $app['slugify']->slugify('welcome to the homepage');
});
```
And if you use Silex in combination with Twig you can also use it in y
没有合适的资源?快使用搜索试试~ 我知道了~
08cms房产网站管理系统V8.6.1
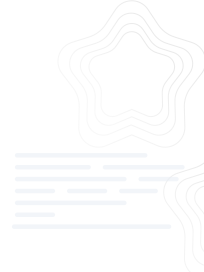
共2000个文件
js:809个
txt:642个
css:247个

需积分: 5 2 下载量 48 浏览量
2024-02-07
11:27:11
上传
评论
收藏 130.12MB ZIP 举报
温馨提示
08cms房产源码v8是一款全新的房产网站管理系统,它基于08cms系统二次开发而成,具有独特的优势和特点,能够为房地产行业带来全方位的解决方案。其基于PHP+MYSQL开源性开发、易于扩展、注重体验、利于SEO。
资源推荐
资源详情
资源评论
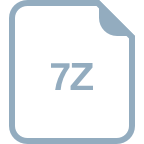
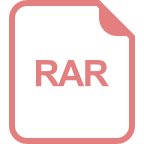
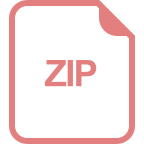
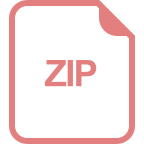
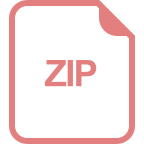
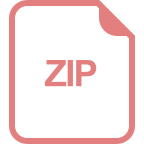
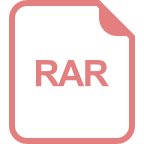
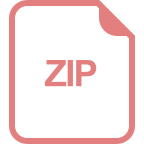
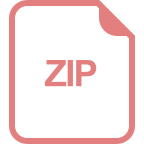
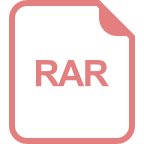
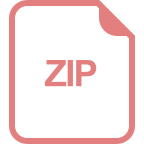
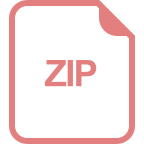
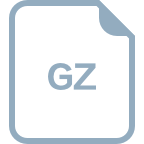
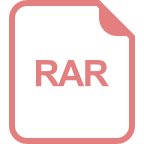
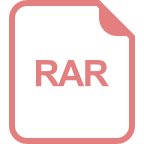
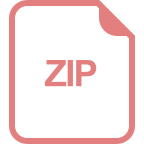
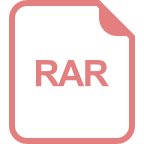
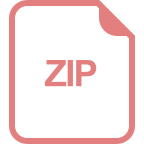
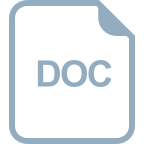
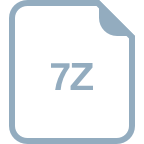
收起资源包目录

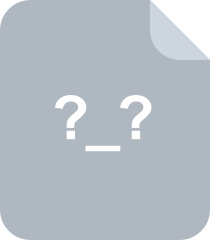
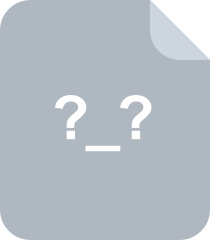
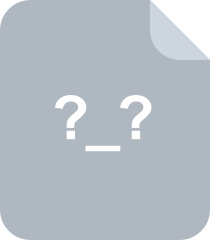
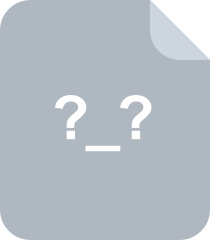
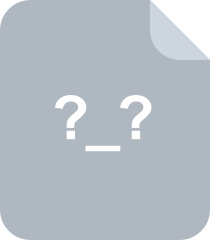
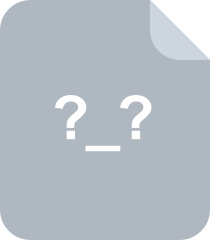
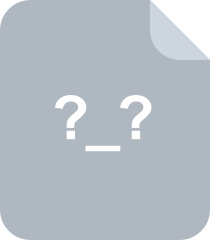
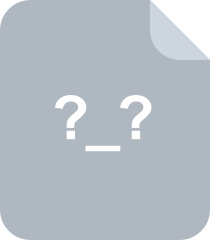
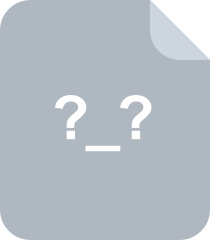
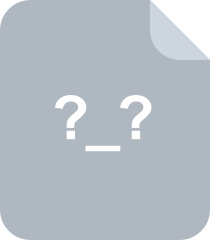
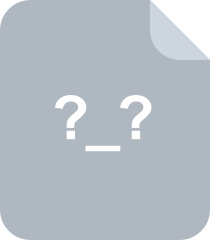
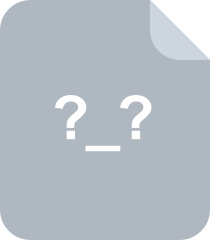
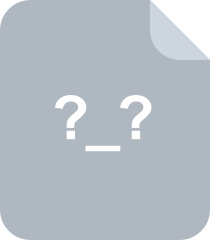
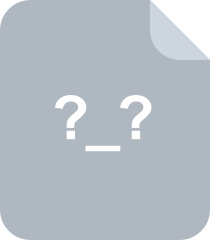
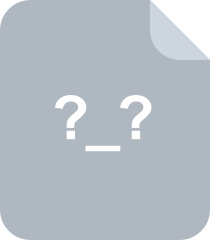
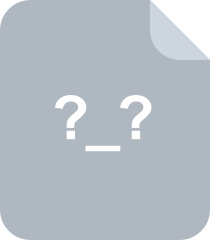
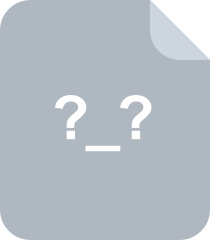
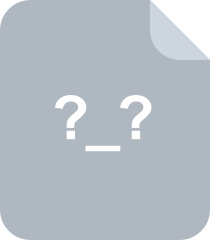
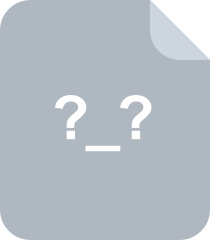
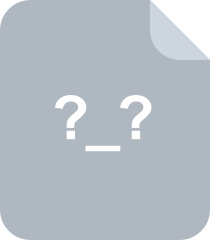
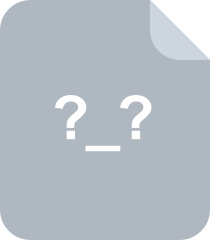
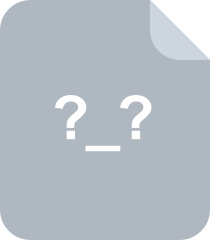
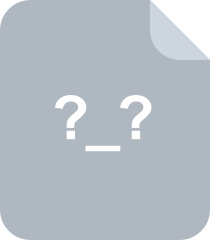
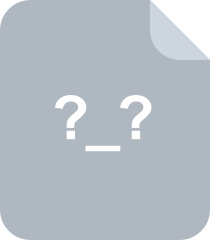
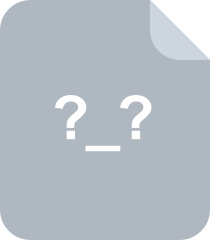
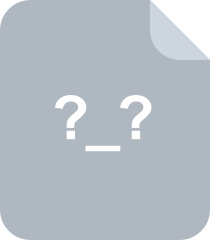
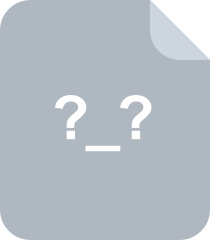
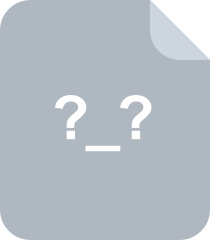
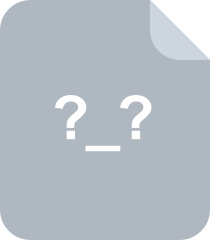
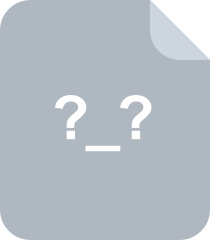
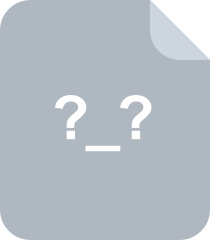
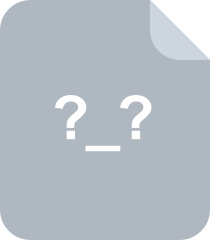
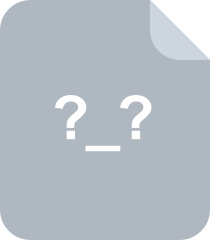
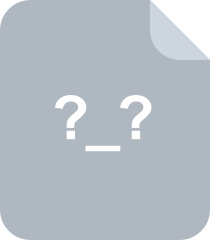
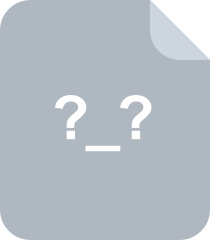
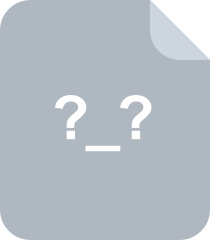
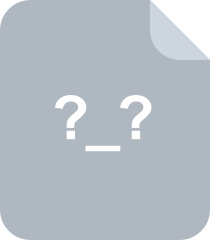
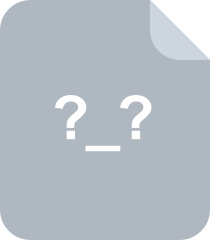
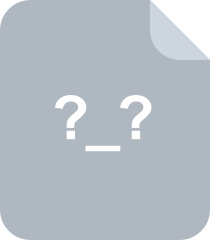
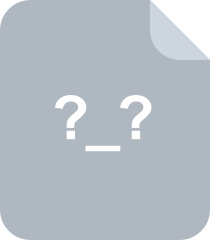
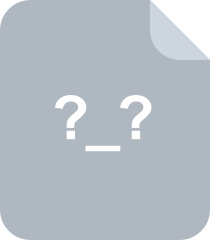
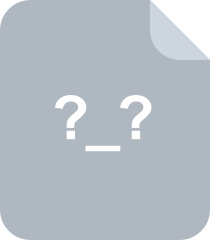
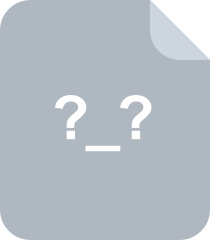
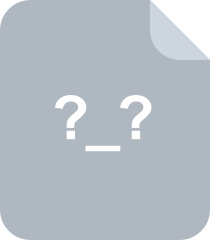
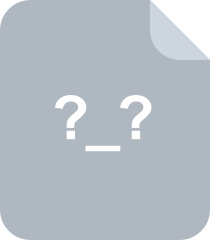
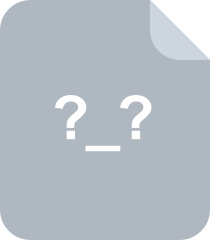
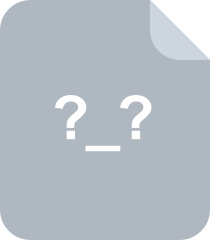
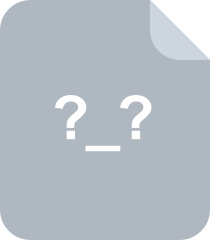
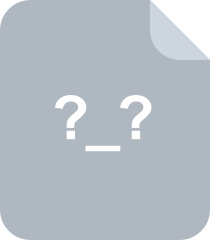
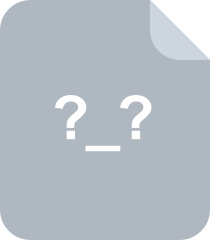
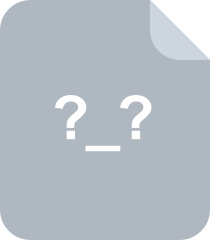
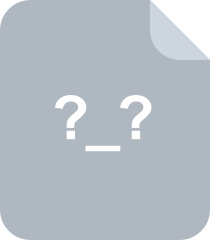
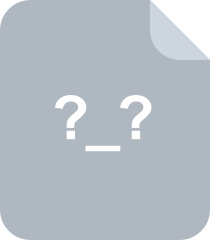
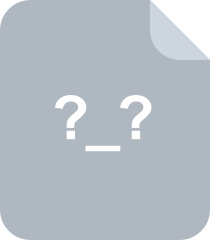
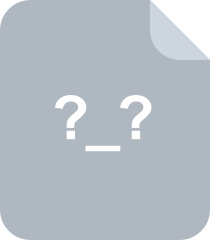
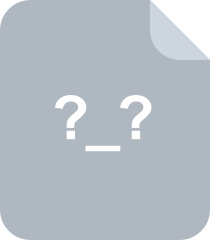
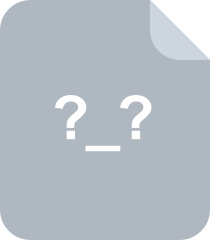
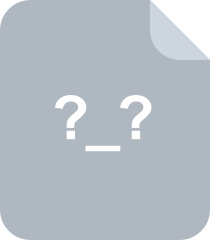
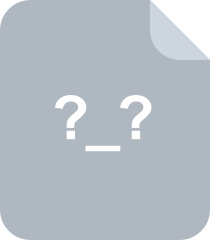
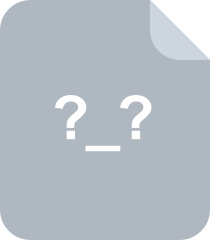
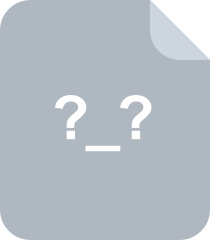
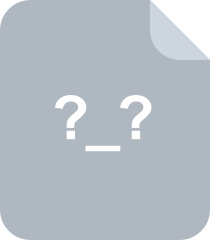
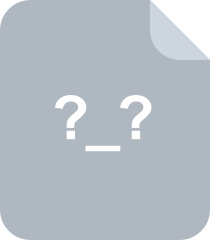
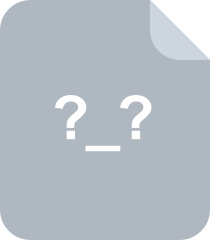
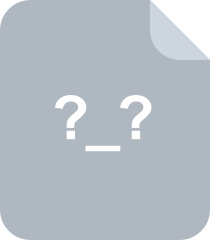
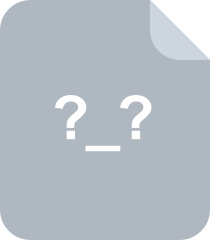
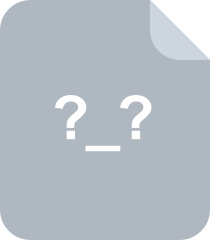
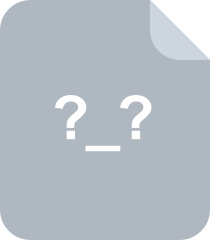
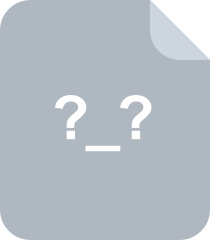
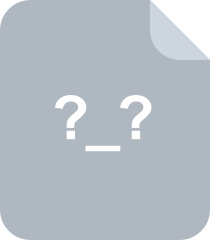
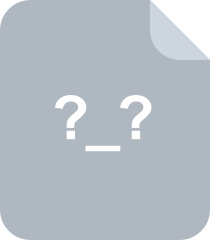
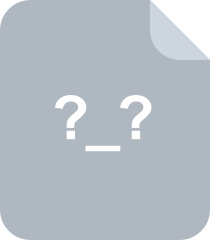
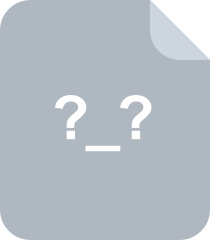
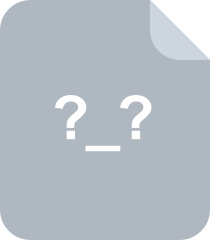
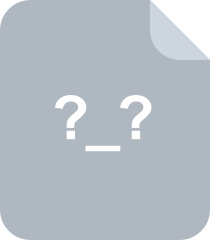
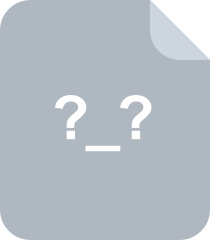
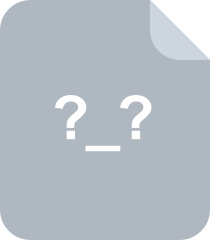
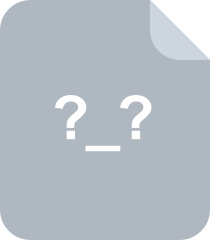
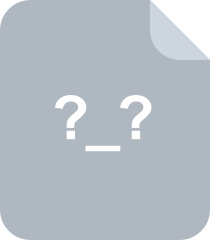
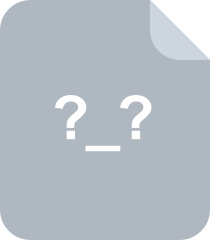
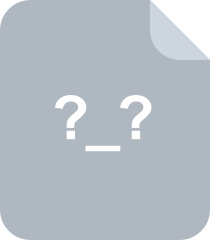
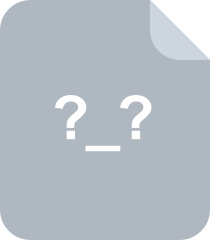
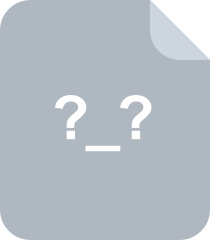
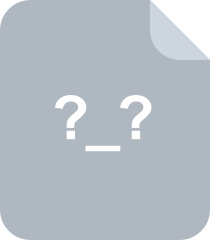
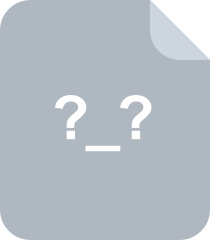
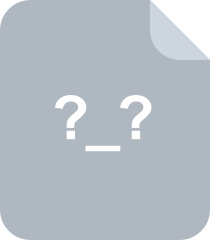
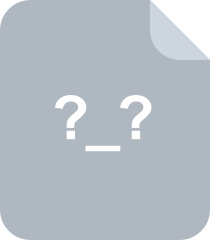
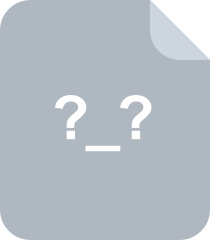
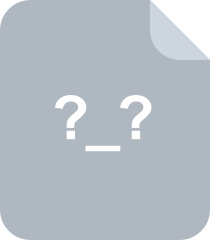
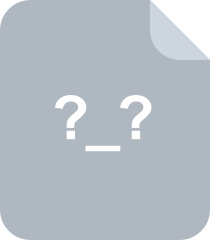
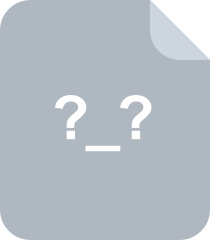
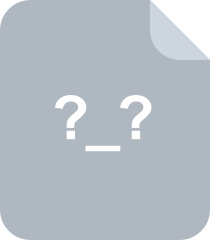
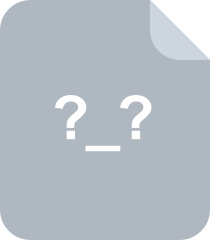
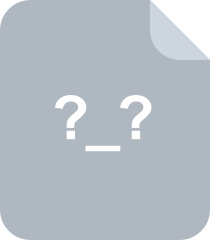
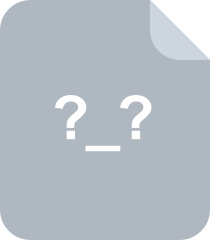
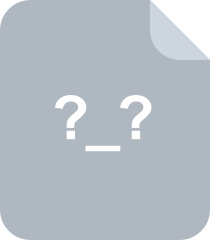
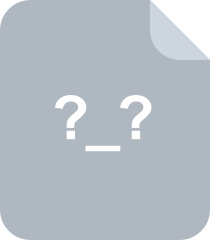
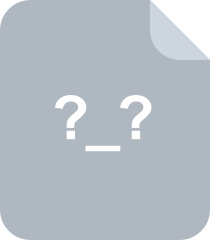
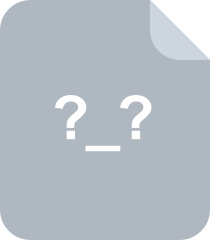
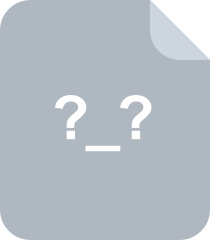
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
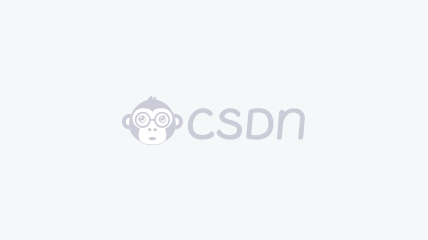

qq_43391237
- 粉丝: 0
- 资源: 9
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

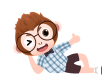
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


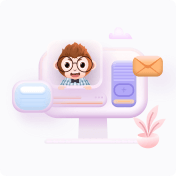
安全验证
文档复制为VIP权益,开通VIP直接复制
