# amCharts Export
Version: 1.4.43
## Description
This plugin adds export capabilities to all amCharts products - charts and maps.
It allows annotating and exporting chart or related data to various bitmap,
vector, document or data formats, such as PNG, JPG, PDF, SVG, JSON, XLSX and
many more.
## Important notice
Please note that due to security measures implemented in modern browsers, some
or all export options might not work if the web page is loaded locally (via
file:///) or contain images loaded from different host than the web page itself.
## Usage
### 1) Include the minified version of file of this plugin as well as the
bundled CSS file. I.e.:
```
<script src="amcharts/plugins/export/export.min.js"></script>
<link type="text/css" href="amcharts/plugins/export/export.css" rel="stylesheet">
```
Or if you'd rather use amCharts CDN:
```
<script src="//cdn.amcharts.com/lib/3/plugins/export/export.min.js"></script>
<link type="text/css" href="//cdn.amcharts.com/lib/3/plugins/export/export.css" rel="stylesheet">
```
(this needs to go after all the other amCharts includes)
### 2) Enable `export` with default options:
```
AmCharts.makeChart( "chartdiv", {
...,
"export": {
"enabled": true
}
} );
```
### ... OR set your own custom options:
```
AmCharts.makeChart( "chartdiv", {
...,
"export": {
"enabled": true,
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download",
"menu": [ "PNG", "JPG", "CSV" ]
}, {
"label": "Annotate",
"action": "draw",
"menu": [ {
"class": "export-drawing",
"menu": [ "PNG", "JPG" ]
} ]
} ]
} ]
}
} );
```
## Loading external libraries needed for operation of this plugin
The plugin relies on a number of different libraries, to export images, draw
annotations or generate download files.
Those libraries need to be loaded for the plugin to work properly.
There are two ways to load them. Choose the one that is right:
### 1) Automatic (preferred)
All libraries required for plugin operation are included withing plugins */libs*
subdirectory.
The plugin will automatically try to look in chart's [`path`](http://docs.amcharts.com/3/javascriptcharts/AmSerialChart#path)
property. If your plugin files are located within plugins folder under amcharts
(as is the case with the default distributions), you don't need to do anything -
the libraries will load on-demand.
If you are using relative url, note that it is relative to the web page you are
displaying your chart on, not the export.js library.
In case you've moved the libs folder you need to tell the plugin where it is
`"libs": { "path": "../libs/" }`
### 2) Manual
You can also load all those JavaScript libraries by `<script>` tags. Since
loading of libraries is on by default you will need to turn it off by setting
`"libs": { "autoLoad": false }`
Here is a full list of the files that need to be loaded for each operation:
File | Located in | Required for
---- | ---------- | ------------
fabric.min.js | libs/fabric.js/ | Any export operation
FileSaver.js | libs/FileSaver.js/ | Used to offer download files
pdfmake.min.js | libs/pdfmake/ | Export to PDF format
vfs_fonts.js | libs/pdfmake/ | Export to PDF format
jszip.js | libs/jszip/ | Export to XLSX format
xlsx.js | libs/xlsx/ | Export to XLSX format
## Complete list of available export settings
Property | Default | Description
-------- | ------- | -----------
backgroundColor | #FFFFFF | RGB code of the color for the background of the exported image
enabled | true | Enables or disables export functionality
divId | | ID or a reference to div object in case you want the menu in a separate container.
fabric | {} | Overwrites the default drawing settings (fabricJS library)
fallback | {} | Holds the messages to guide the user to copy the generated output; `false` will disable the fallback feature
fileName | amCharts | A file name to use for generated export files (an extension will be appended to it based on the export format)
legend | {} | Places the legend in case it is within an external container ([skip to chapter](#adding-external-legend))
libs | | 3rd party required library settings (see the above section)
menu | [] | A list of menu or submenu items (see the next chapter for details)
pdfMake | {} | Overwrites the default settings for PDF export (pdfMake library)
position | top-right | A position of export icon. Possible values: "top-left", "top-right" (default), "bottom-left", "bottom-right"
removeImages | true | If true export checks for and removes "tainted" images that area lodead from different domains
delay | | General setting to delay the capturing of the chart ([skip to chapter](#delay-the-capturing-before-export))
exportFields | [] | If set, only fields in this array will be exported ( data export only )
exportTitles | false | Exchanges the data field names with it's dedicated title ( data export only )
columnNames | {} | An object of key/value pairs to use as column names when exporting to data formats. `exportTitles` needs to be set for this to work as well.
exportSelection | false | Exports the current data selection only ( data export only )
dataDateFormat | | Format to convert date strings to date objects, uses by default charts dataDateFormat ( data export only )
dateFormat | YYYY-MM-DD | Formats the category field in given date format ( data export only )
keyListener | false | If true it observes the pressed keys to undo/redo the annotations
fileListener | false | If true it observes the drag and drop feature and loads the dropped image file into the annotation
drawing | {} | Object which holds all possible settings for the annotation mode ([skip to chapter](#annotation-settings))
overflow | true | Flag to overwrite the css attribute 'overflow' of the chart container to avoid cropping the menu on small container
border | {} | An object of key/value pairs to define the overlaying border
processData | | A function which can be used to change the dataProvider when exporting to CSV, XLSX, or JSON
pageOrigin | true | A flag to show / hide the origin of the generated PDF ( pdf export only )
forceRemoveImages | false | If true export removes all images
## Configuring export menu
Plugin includes a way to completely control what is displayed on export menu.
You can set up menus, sub-menus, down to any level. You can even add custom
items there that execute your arbitrary code on click. It's so configurable
it makes us sick with power ;)
The top-level menu is configured via `menu` property under `export`. It should
always be an array, even if you have a single item in it.
The array items could be either objects or format codes. Objects will allow you
to specify labels, action, icon, child items and even custom code to be executed
on click.
Simple format codes will assume you need an export to that format.
### Simple menu setup
Here's a sample of the simple menu setup that allows export to PNG, JPG and CSV:
```
"export": {
"enabled": true,
"menu": [ {
"class": "export-main",
"menu": [ "PNG", "JPG", "CSV" ]
} ]
}
```
The above will display a menu out of three options when you hover on export
icon:
* PNG
* JPG
* CSV
When clicked the plugin will trigger export to a respective format.
If that is all you need, you're all set.
Please note that we have wrapped out menu into another menu item, so that only
the icon is displayed until we roll over the icon. This means that technically
we have a two-level hierarchical menu.
If we opmitted that first step, the chart would simply display a format list
right there on the chart.
### Advanced menu setup
However, you can do so much more with the menu.
Let's add more formats and organize image and data formats into separate
submenus.
To add a submenu to a menu item, simply add a `menu` array as its own property:
```
"export": {
"enabled": true,
"menu": [ {
"class": "export-main",
"menu": [ {
"la
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
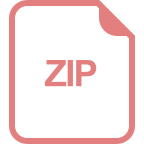
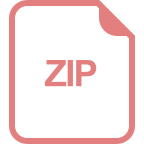
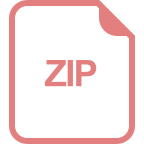
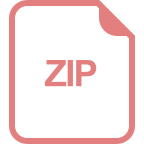
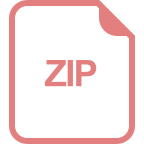
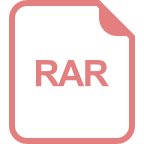
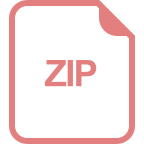
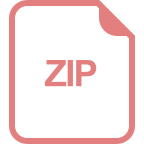
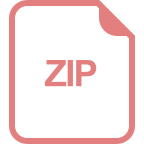
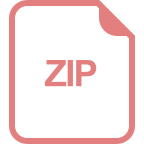
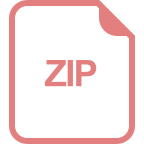
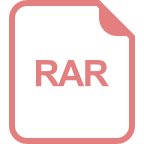
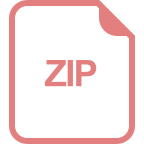
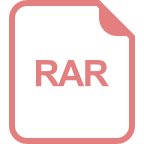
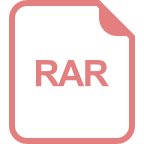
收起资源包目录

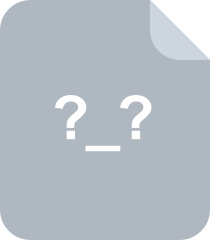
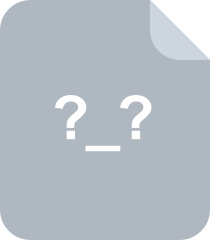
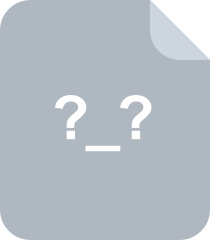
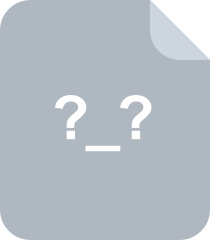
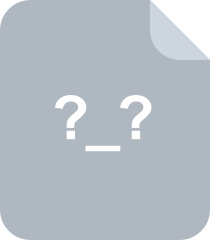
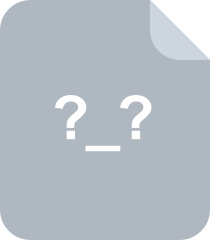
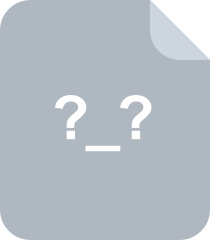
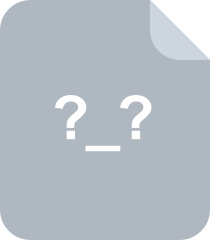
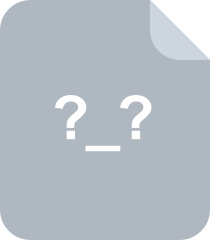
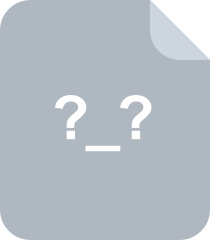
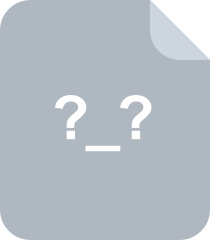
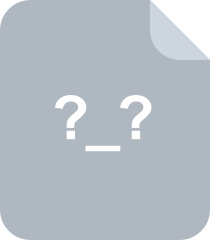
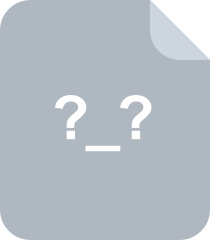
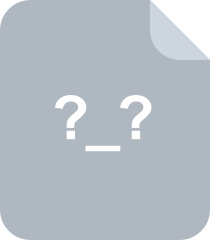
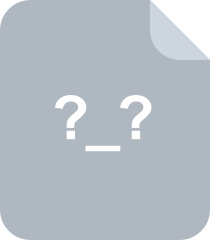
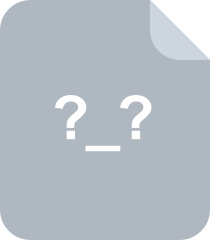
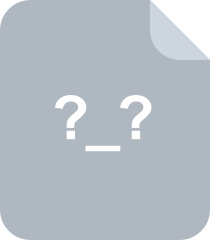
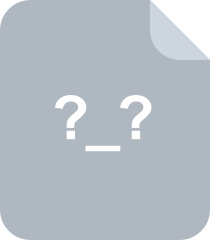
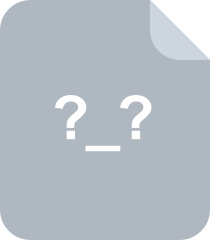
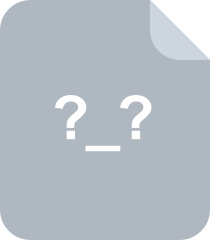
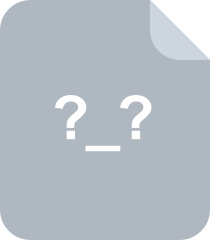
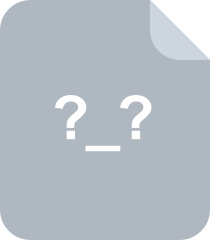
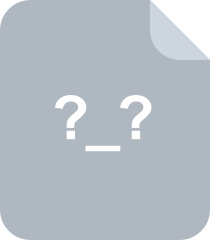
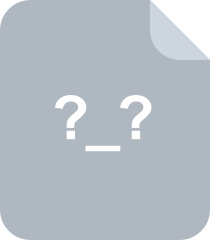
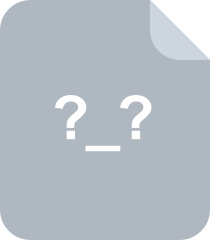
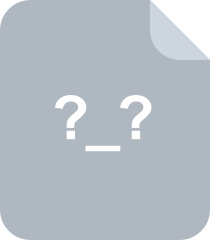
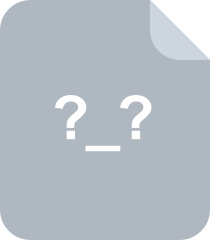
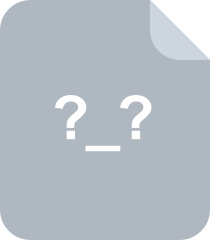
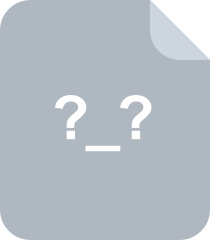
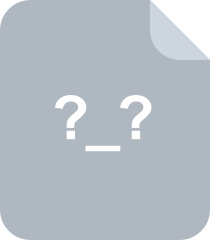
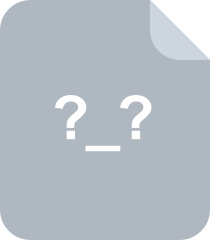
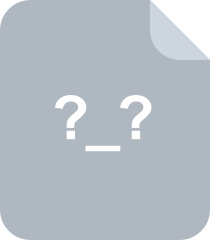
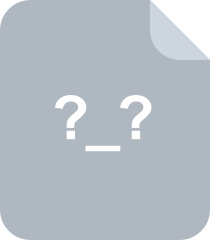
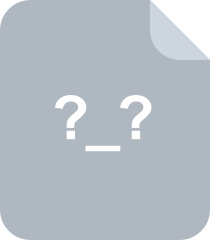
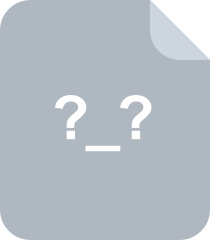
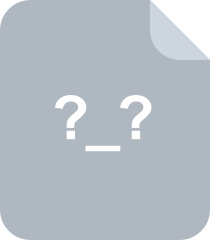
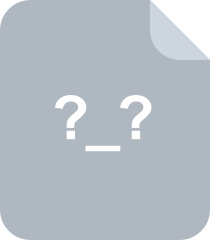
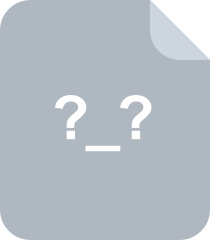
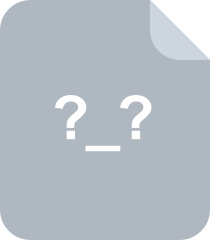
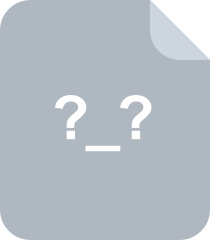
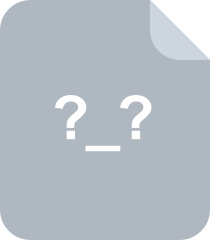
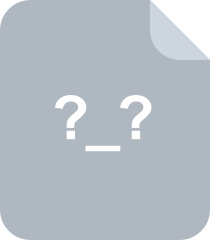
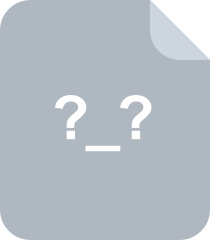
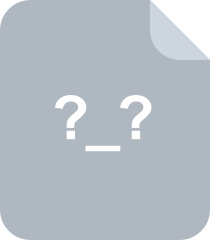
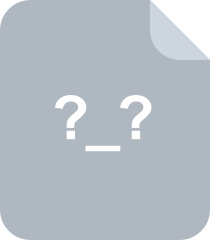
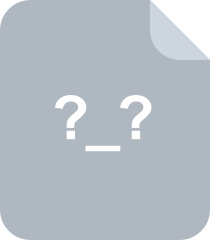
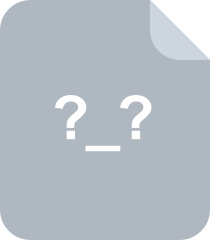
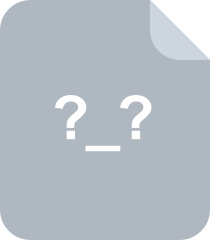
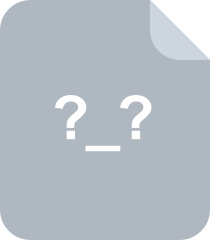
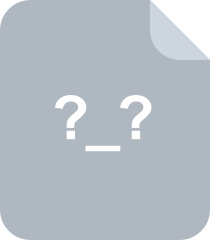
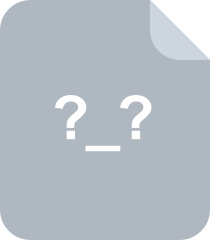
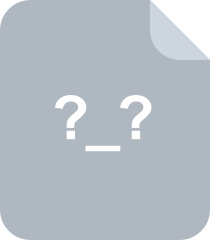
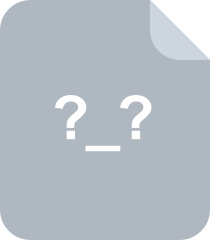
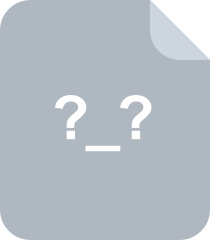
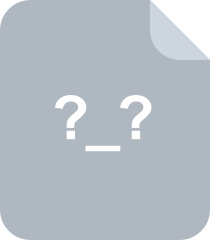
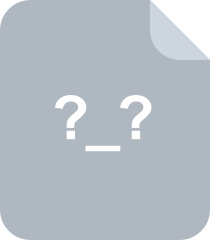
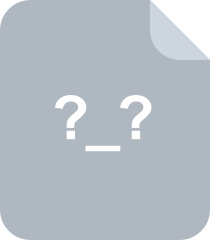
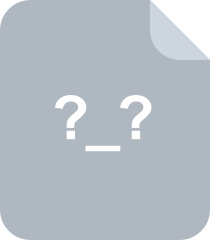
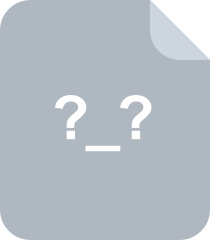
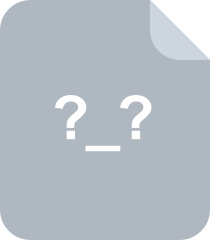
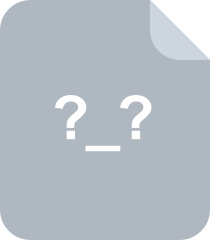
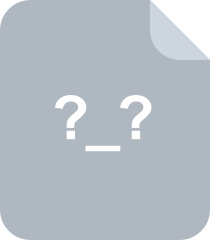
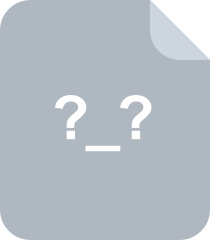
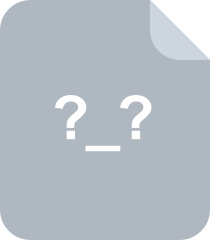
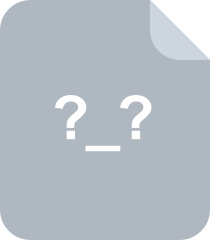
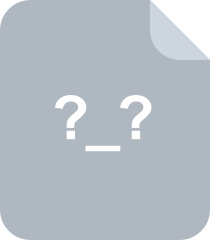
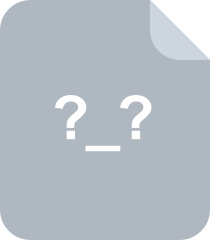
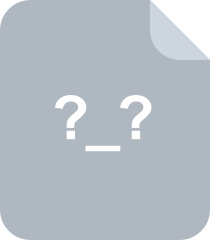
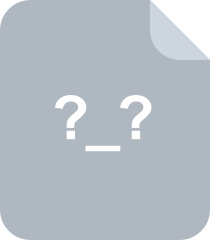
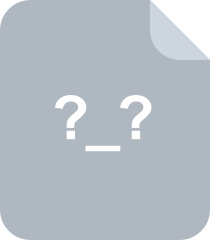
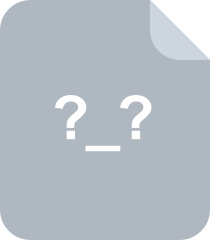
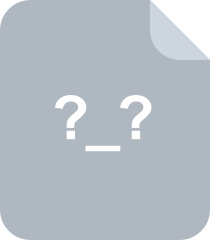
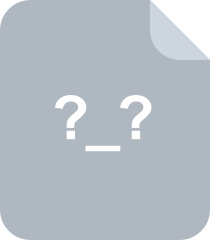
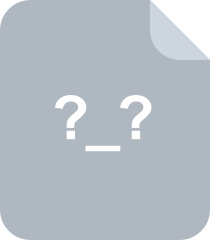
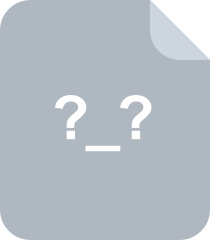
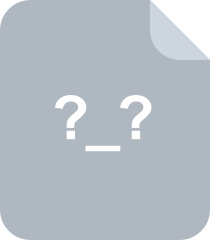
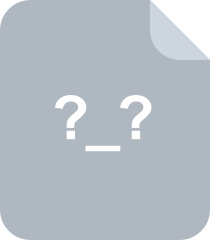
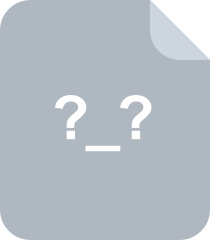
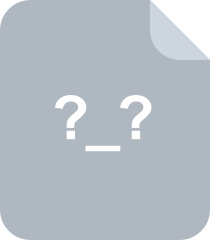
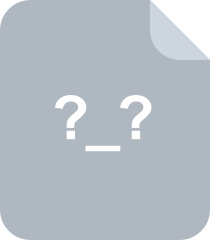
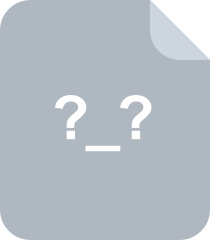
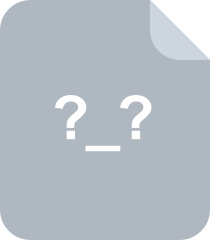
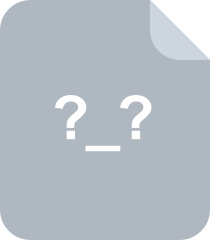
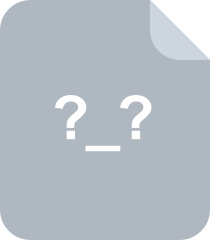
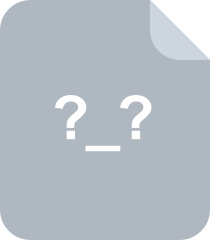
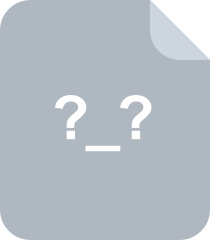
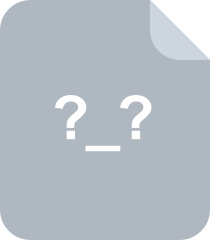
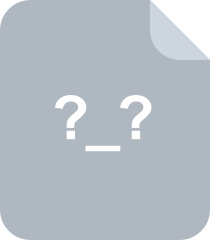
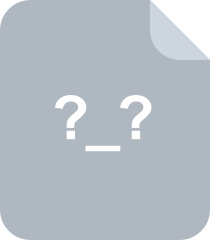
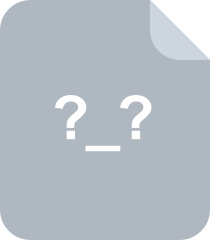
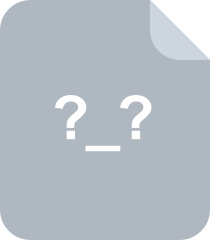
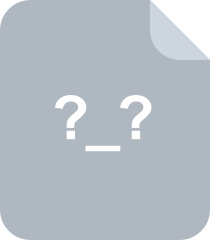
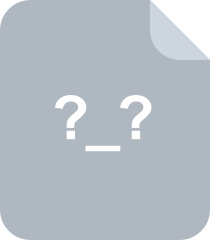
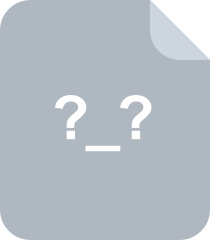
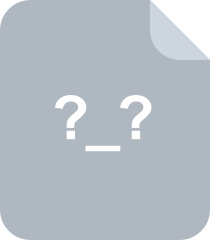
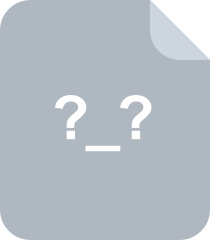
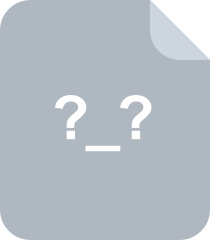
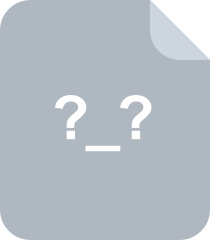
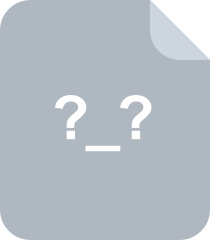
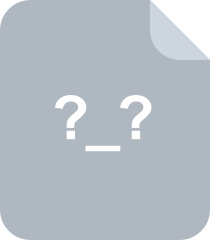
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
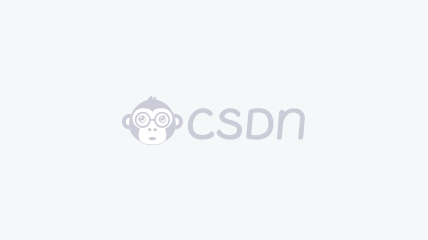


普通网友
- 粉丝: 4733
- 资源: 910
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

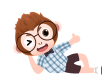
最新资源
- 2024年下半年软考中级网络工程师bfd与静态路由联动配置实验
- 2024年下半年软考中级网络工程师单臂路由配置实验
- 类图与操作系统进程:映射与实现
- vmware虚拟机安装教程的个人学习资料,欢迎使用 内容来源于网络分享,如有侵权请联系我删除
- 数据库数据清洗策略:技术实现与代码实践
- vmware虚拟机安装教程的个人学习资料,欢迎使用 内容来源于网络分享,如有侵权请联系我删除
- vmware虚拟机安装教程的个人学习资料,欢迎使用 内容来源于网络分享,如有侵权请联系我删除
- unity射击游戏模板Low Poly Shooter Pack v4.0.7z
- 行人乱丢垃圾检测数据集VOC+YOLO格式3264张2类别
- 电力场景越线闯入检测数据集VOC+YOLO格式258张1类别.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


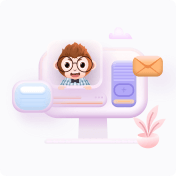
安全验证
文档复制为VIP权益,开通VIP直接复制
