from django.db.models.functions import TruncDate
from django.views.generic import TemplateView
from django.shortcuts import render, HttpResponse
from .models import WebPage, Type, HistoryInfo
from django.views import View
import requests
from django.http import JsonResponse
import datetime
from django.utils import timezone
from datetime import timedelta
from django.db.models import Count
class IndexViews(View):
def get(self, request):
try:
# 记录历史信息
self.record_history_info(request)
# 获取网页和分类信息
web_models = WebPage.objects.order_by('sort')
types = Type.objects.order_by('sort')
context = {
'web_models': web_models,
'types': types,
}
# 渲染模板
return render(request, 'index/index.html', context)
except Exception as e:
return HttpResponse(f"An error occurred: {str(e)}")
def get_real_ip(self, request):
x_forwarded_for = request.META.get('HTTP_X_FORWARDED_FOR')
if x_forwarded_for:
ip = x_forwarded_for.split(',')[0] # 所以这里是真实的ip
else:
ip = request.META.get('REMOTE_ADDR') # 这里获得代理ip
return ip
def record_history_info(self, request):
# 获取真实的客户端 IP
ip = self.get_real_ip(request)
# 初始化地理位置信息
nation, province, city = '', '', ''
# 尝试获取地理信息
if ip:
try:
response = requests.get(f'http://ip-api.com/json/{ip}?lang=zh-CN', timeout=5)
if response.status_code == 200:
data = response.json()
nation = data.get('country', '')
province = data.get('regionName', '')
city = data.get('city', '')
except requests.RequestException as e:
# 记录错误日志,或者其他错误处理
print(f"Error fetching location data for IP {ip}: {e}")
# 获取当前时间并转换为北京时间
# 将 UTC 时间转换为北京时间
beijing_time = datetime.datetime.now()
# 使用 24 小时制格式化时间
formatted_time = beijing_time.strftime('%Y-%m-%d %H:%M:%S')
print("=========================")
print(formatted_time)
# 创建历史记录
HistoryInfo.objects.create(
ip=ip,
nation=nation,
province=province,
city=city,
create_time=formatted_time
)
class DisplayViews(TemplateView):
template_name = 'index/display.html'
def get(self, request, *args, **kwargs):
start_date = request.GET.get('start_date')
end_date = request.GET.get('end_date')
# 如果没有传递起始和结束日期,默认选择最近7天
if not start_date or not end_date:
end_date = timezone.now().date()
start_date = end_date - timedelta(days=7)
else:
start_date = timezone.datetime.strptime(start_date, '%Y-%m-%d').date()
end_date = timezone.datetime.strptime(end_date, '%Y-%m-%d').date()
# 按天统计访问量
daily_stats = (
HistoryInfo.objects
.filter(create_time__date__gte=start_date, create_time__date__lte=end_date)
.annotate(date=TruncDate('create_time'))
.values('date')
.annotate(count=Count('id'))
.order_by('date')
)
# 转换为列表
daily_stats = list(daily_stats)
# 创建一个字典来存储日期和对应的访问量
stats_dict = {stat['date']: stat['count'] for stat in daily_stats}
# 创建一个包含最近 7 天日期的列表,并确保没有数据的日期访问量为 0
complete_stats = []
current_date = start_date
while current_date <= end_date:
complete_stats.append({
'date': current_date.isoformat(),
'count': stats_dict.get(current_date, 0) # 如果没有数据则为 0
})
current_date += timedelta(days=1)
# 统计国家和省市的访问量
country_stats = (
HistoryInfo.objects
.filter(create_time__date__gte=start_date, create_time__date__lte=end_date)
.values('nation', 'province', 'city') # 使用 'nation' 替换 'country'
.annotate(count=Count('id'))
)
# 将查询到的日期和访问量转换为字典
country_stats = [
{'nation': stat['nation'], 'province': stat['province'], 'city': stat['city'], 'count': stat['count']} for
stat in country_stats]
# 判断是否为 AJAX 请求
if request.headers.get('x-requested-with') == 'XMLHttpRequest':
# print("返回的统计数据:", complete_stats) # 打印返回的数据
return JsonResponse({'daily_stats': complete_stats, 'country_stats': country_stats}, safe=False)
else:
# print("2")
context = self.get_context_data(complete_stats=complete_stats)
return self.render_to_response(context)
没有合适的资源?快使用搜索试试~ 我知道了~
收起资源包目录



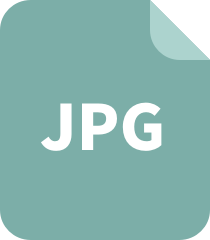
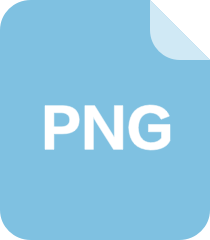
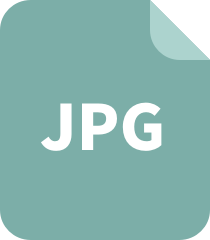
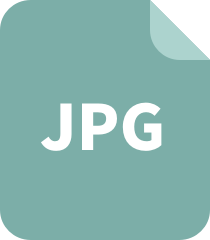
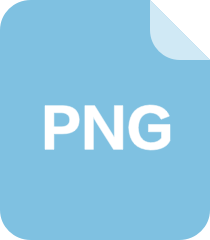
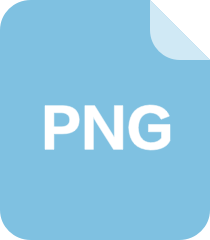

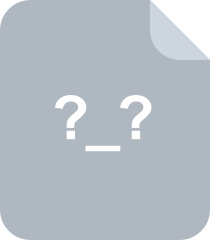
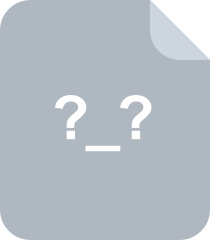
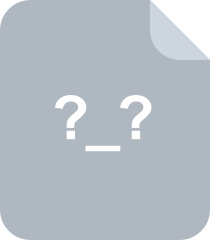
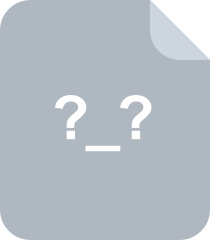

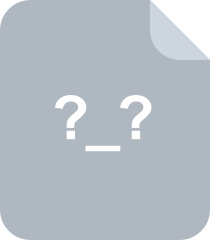
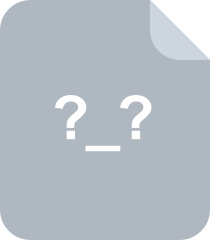
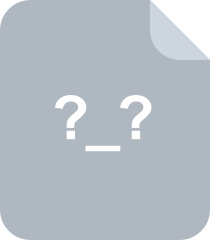
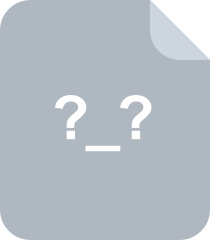
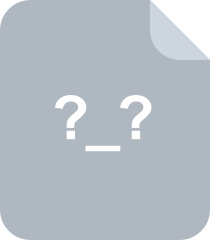


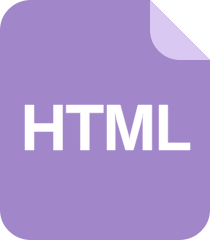
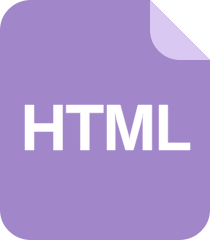
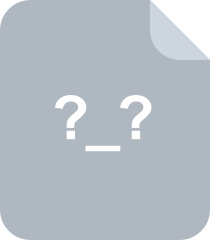
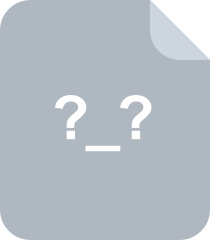

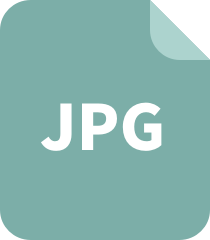
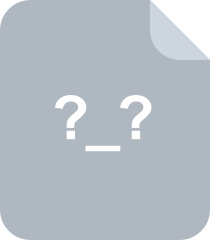
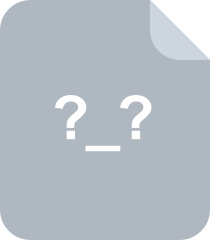

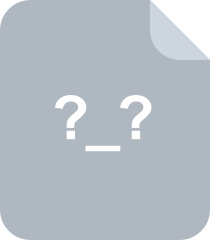


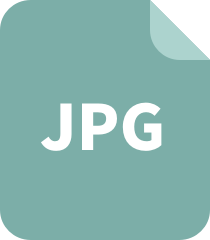
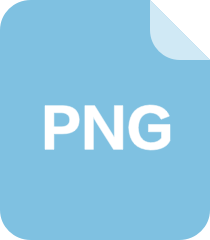
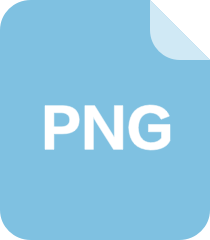

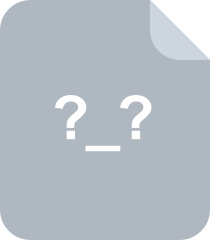

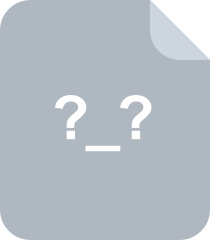
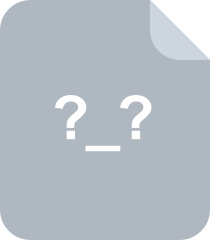

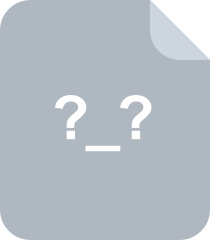

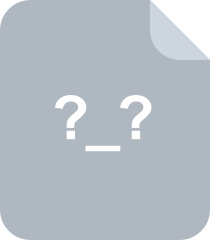
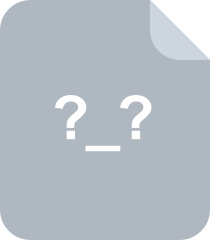
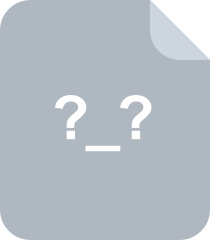

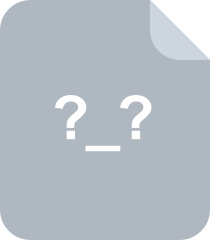
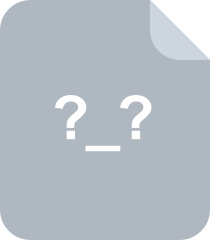
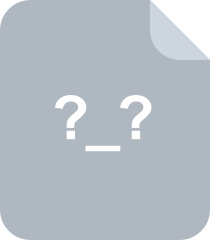

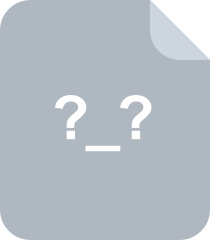
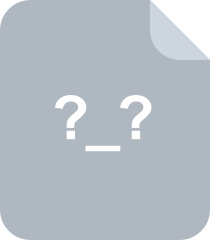
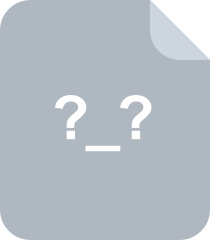
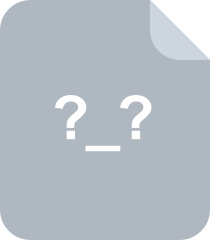
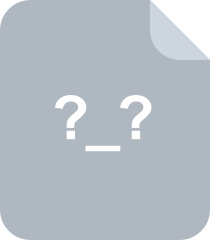
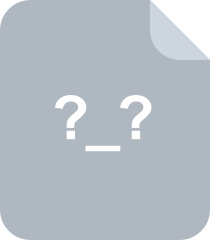

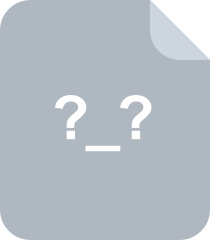
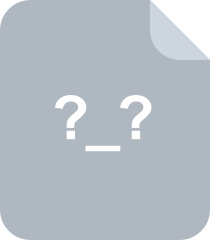
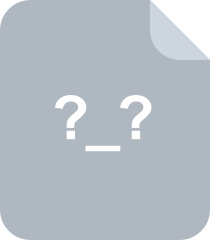
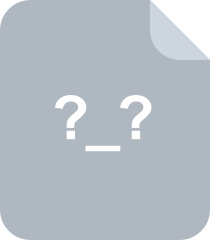
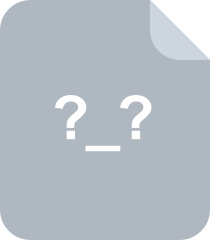
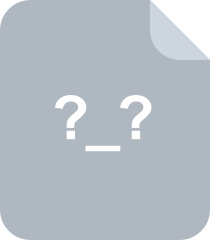
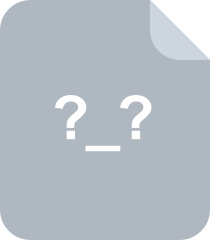

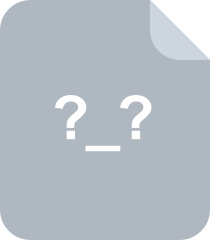
共 50 条
- 1
资源推荐
资源预览
资源评论
641 浏览量
2018-09-02 上传
301 浏览量
198 浏览量
4947 浏览量
375 浏览量
1993 浏览量
395 浏览量
12949 浏览量
518 浏览量
2022-05-08 上传
1226 浏览量
2023-08-21 上传
2022-05-08 上传
2024-03-25 上传
2019-03-14 上传
25576 浏览量
224 浏览量
10613 浏览量
147 浏览量
2384 浏览量
2022-05-04 上传
2020-06-03 上传
657 浏览量
7438 浏览量
2018-06-20 上传
2024-03-25 上传
资源评论
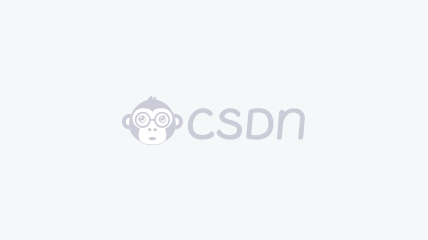

一个小渣渣AI
- 粉丝: 147
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

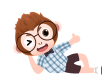
最新资源
- RHEL9环境下Python和PHP的安装及应用指南
- 基于OpenCV与Python的车道线智能检测系统:从图像预处理到UI界面的实现,基于OpenCV与Python的车道线智能检测系统(带UI界面):从图像预处理到霍夫直线检测与拟合的自动驾驶核心技术解
- FMETP STREAM EditionS
- 【大作业-36】基于yolov8和yolo11的绝缘子缺陷检测系统.zip
- 基于LSTM的时间序列预测模型:单输入单输出预测,数据存入Excel,性能评估指标包括决定系数R2、平均绝对误差MAE及平均相对误差MBE的详解代码,基于LSTM的时间序列预测模型:单输入单输出预测
- 清华大学第四弹-deepseek+Research让科研像聊天一样简单
- 使用Android手机的摄像头,通过闪光灯识别手指的血管,完成心率的检测,绘制出心率图
- 基于随机森林算法RF的数据分类预测详解:从代码注释到Excel数据存储的全面指南,基于随机森林算法RF的数据分类预测详解:从代码注释到Excel数据存储之道,基于随机森林算法RF的数据分类预测 代码含
- 呼气式酒精检测仪压力传感器US9411规格书V6.0:参数特性、应用领域及订购指南
- coreutils+wget.zip
- Nginx 服务器安装及配置文件详解.docx
- 资源-网络工程师知识点
- CCNA课件14节课程,pdf课件
- 《免费模电-Multisim电路仿真大合集(120例)-电子类专业实验/毕设实战资源库》
- stm32f103c8
- SDN 架构图.jpg
安全验证
文档复制为VIP权益,开通VIP直接复制
