package com.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import com.entity.CommentInfo;
import com.entity.GoodsInfo;
/**
* 商品操作类
* @author Administrator
*
*/
public class GoodsCtrl {
/**
* 添加商品方法
* @param goods
* @return
*/
public int addGoods(GoodsInfo goods){
int res =0;
Connection conn =ConnDB.getConn();
PreparedStatement stmt =null;
String sql ="insert into GoodsInfo values(?,?,?,?,?,?,?,?,?,?,?,?)";
try {
stmt =conn.prepareStatement(sql);
stmt.setString(1, goods.getGoodsname());
stmt.setString(2, goods.getGoodssort());
stmt.setDouble(3, goods.getGoodsprice());
stmt.setDouble(4, goods.getGoodscarr());
stmt.setString(5, goods.getGoodsaddr());
stmt.setString(6, goods.getGoodsimg());
stmt.setString(7, goods.getGoodstuijian());
stmt.setString(8, goods.getGoodsxianliang());
stmt.setString(9, goods.getGoodstejia());
stmt.setString(10, goods.getGoodscuxiao());
stmt.setString(11, goods.getGoodszt());
stmt.setString(12, goods.getGoodsintro());
res =stmt.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
ConnDB.close(null, stmt, conn);
}
return res;
}
/*==商品列表数据分页==*/
/**
* 根据当前页数和每页显示的行数来查询相应的结果
*
* @param pageSize
* 每页显示的行数
* @param pageNow
* 当前的页数
* @return
*/
public ArrayList<GoodsInfo> getGoodsForPage(int pageSize, int pageNow) {
ArrayList<GoodsInfo> agoods = null;
Connection conn = ConnDB.getConn();
Statement stmt = null;
ResultSet rs = null;
String sql = "select Top " + pageSize
+ " * from GoodsInfo where goodsId not in(select Top (" + pageSize
+ " * (" + pageNow + "- 1)) goodsId from GoodsInfo)";
try {
stmt=conn.createStatement();
rs=stmt.executeQuery(sql);
while(rs.next()){
if(agoods==null){
agoods=new ArrayList<GoodsInfo>();
}
GoodsInfo goods = new GoodsInfo();
goods.setGoodsId(rs.getInt("goodsId"));
goods.setGoodsname(rs.getString("goodsName"));
goods.setGoodssort(rs.getString("goodsSort"));
goods.setGoodsprice(rs.getDouble("goodsPrice"));
goods.setGoodscarr(rs.getDouble("goodsCarr"));
goods.setGoodsaddr(rs.getString("goodsAddr"));
goods.setGoodsimg(rs.getString("goodsImg"));
goods.setGoodszt(rs.getString("goodsZt"));
agoods.add(goods);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
ConnDB.close(rs, stmt, conn);
}
return agoods;
}
/**
* 获取总页数
* @param pageSize
* @return
*/
public int getGoodsCount(int pageSize){
int pageCount = 0;
int goodsCount = 0;//获取到商品的总数
Connection conn = ConnDB.getConn();
Statement stmt = null;
ResultSet rs = null;
String sql = "select count(*) as goodsCount from GoodsInfo";
try {
stmt = conn.createStatement();
rs = stmt.executeQuery(sql);
if(rs.next()){
goodsCount = rs.getInt("goodsCount");
}
//页数计算
if(goodsCount % pageSize == 0){
pageCount = goodsCount / pageSize;
}else{
pageCount = goodsCount / pageSize + 1;
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
ConnDB.close(rs, stmt, conn);
}
return pageCount;
}
/**
* 根据ID查询商品方法
* @param goodsId
* @return
*/
public GoodsInfo selgoods(int goodsId){
GoodsInfo goods = null;
Connection conn = ConnDB.getConn();
Statement stmt = null;
ResultSet rs = null;
String sql = "select * from GoodsInfo where goodsId=" + goodsId;
try {
stmt = conn.createStatement();
rs = stmt.executeQuery(sql);
if(rs.next()){
goods = new GoodsInfo();
goods.setGoodsId(rs.getInt("goodsId"));
goods.setGoodsname(rs.getString("goodsName"));
goods.setGoodssort(rs.getString("goodsSort"));
goods.setGoodsprice(rs.getDouble("goodsPrice"));
goods.setGoodscarr(rs.getDouble("goodsCarr"));
goods.setGoodsaddr(rs.getString("goodsAddr"));
goods.setGoodsimg(rs.getString("goodsImg"));
goods.setGoodstuijian(rs.getString("goodsTuijian"));
goods.setGoodsxianliang(rs.getString("goodsXianliang"));
goods.setGoodstejia(rs.getString("goodsTejia"));
goods.setGoodscuxiao(rs.getString("goodsCuxiao"));
goods.setGoodszt(rs.getString("goodsZt"));
goods.setGoodsintro(rs.getString("goodsIntro"));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
ConnDB.close(rs, stmt, conn);
}
return goods;
}
/**
* 修改商品方法
*/
public int updateGoods(GoodsInfo goods){
int res = 0;
Connection conn =ConnDB.getConn();
PreparedStatement stmt = null;
String sql = "update GoodsInfo set goodsName=?,goodsSort=?,goodsPrice=?,goodsCarr=?,goodsAddr=?,goodsImg=?,goodsTuijian=?,goodsXianliang=?,goodsTejia=?,goodsCuxiao=?,goodsZt=?,goodsIntro=?" +" where goodsId=?";
try {
stmt =conn.prepareStatement(sql);
stmt.setString(1, goods.getGoodsname());
stmt.setString(2, goods.getGoodssort());
stmt.setDouble(3, goods.getGoodsprice());
stmt.setDouble(4, goods.getGoodscarr());
stmt.setString(5, goods.getGoodsaddr());
stmt.setString(6, goods.getGoodsimg());
stmt.setString(7, goods.getGoodstuijian());
stmt.setString(8, goods.getGoodsxianliang());
stmt.setString(9, goods.getGoodstejia());
stmt.setString(10, goods.getGoodscuxiao());
stmt.setString(11, goods.getGoodszt());
stmt.setString(12, goods.getGoodsintro());
stmt.setInt(13, goods.getGoodsId());
res = stmt.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
ConnDB.close(null, stmt, conn);
}
return res;
}
/**
* 根据ID删除商品
* @param userId
* @return
*/
public int deleteGoods(int goodsId){
int res = 0;
String sql = "delete GoodsInfo where goodsId=?";
Connection conn = ConnDB.getConn();
PreparedStatement stmt = null;
try {
stmt = conn.prepareStatement(sql);
stmt.setInt(1, goodsId);
res = stmt.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
ConnDB.close(null, stmt, conn);
}
return res;
}
/*==推荐、限量、特价、促销商品搜索数据分页==*/
/**
* 根据导航栏连接搜索商品 *
* 根据当前页数和每页显示的行数来查询相应的结果
*
* @param pageSize
* 每页显示的行数
* @param pageNow
* 当前的页数
* @param tj
* 查询条件:(推荐、特价等)
* @return
*/
public ArrayList<GoodsInfo> getDaohlGoods(int pageSize, int pageNow,String tj) {
ArrayList<GoodsInfo> agoods = null;
Connection conn = ConnDB.getConn();
Statement stmt = null;
ResultSet rs = null;
String sql = "select Top " + pageSize
+ " * from GoodsInfo where goodsId not in(select Top (" + pageSize
+ " * (" + pageNow + "- 1)) goodsId from GoodsInfo) and "+tj+"='true' and goodsZt='true' order by goodsId desc";
try {
stmt=conn.createStatement();
rs=stmt.executeQuery(sql);
while(rs.next()){
if(agoods==null){
agoods=new ArrayList<GoodsInfo>();
}
GoodsInfo goods = new GoodsInfo();
goods.setGoodsId(rs.getInt("goodsId"));
goods.setGoodsname(rs.getString("goodsName"));
goods.setGoodsprice(rs.getDouble("goodsPrice"));
goods.setGoodsimg(rs.getString("goodsImg"));
agoods.add(goods);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
ConnDB.close(rs, stmt
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源概述: 此资源集包含了一套完整的Java实现的购物商城项目源代码、相关的毕业论文以及详尽的使用说明。它旨在提供一个全面、深入的学习和研究工具,适用于本科课程设计、毕业设计以及任何希望深入学习Java编程的学习者。 详细内容: 源代码——提供了一套完整、经过良好注释的Java源代码,实现了购物商城项目的全部功能。代码结构清晰,逻辑严谨,是学习Java编程和项目实践的优秀材料。 毕业论文——包含了一篇探讨购物商城项目背景、技术原理和实现过程的学术论文,可作为论文撰写参考。通过阅读论文,学习者可以了解项目的理论基础,深入理解项目的实现细节,提升分析和解决问题的能力。 使用说明——详细的使用说明文档帮助学习者快速了解项目结构和使用方法,降低学习难度,提高学习效率。 适用对象: 此资源集适用于计算机科学、软件工程等专业的本科生进行课程设计和毕业设计。同时,对于希望提升Java编程技能、了解项目实践过程的学习者,这也是一份不可多得的学习资料。
资源推荐
资源详情
资源评论
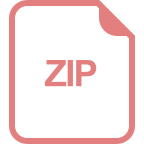
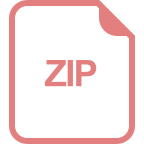
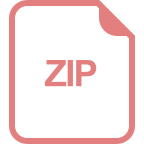
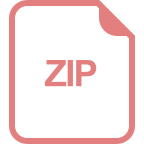
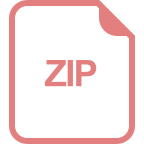
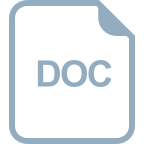
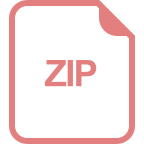
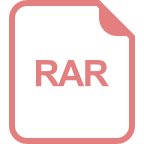
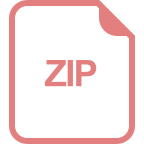
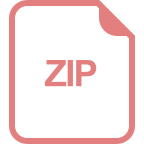
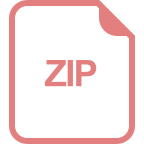
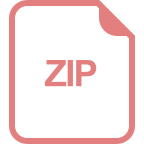
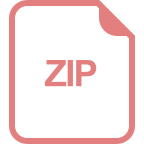
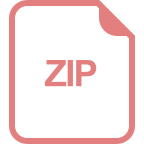
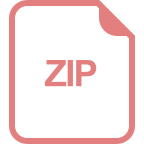
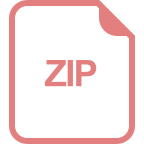
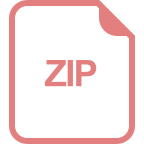
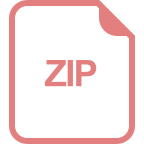
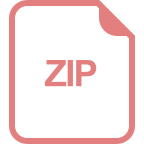
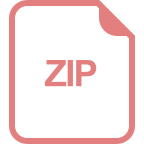
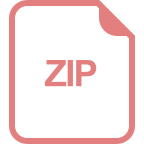
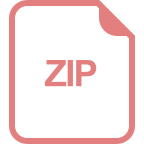
收起资源包目录

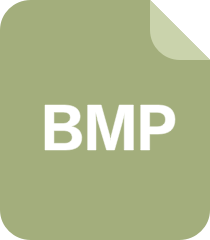
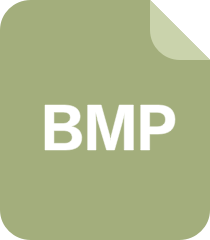
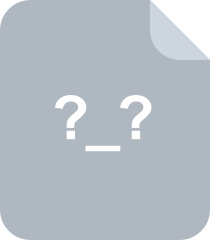
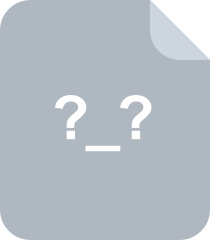
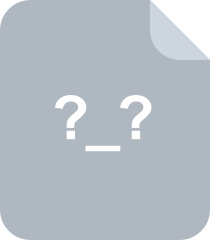
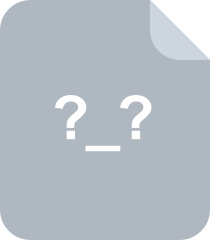
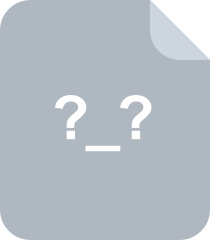
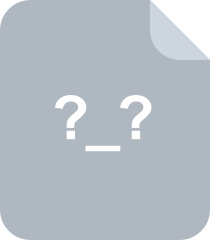
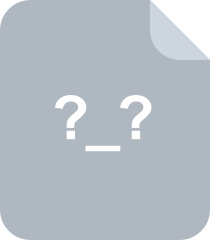
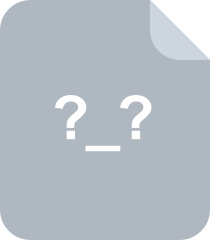
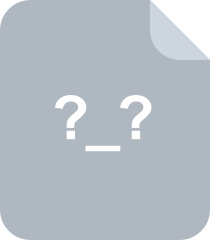
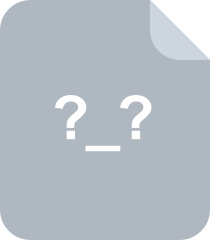
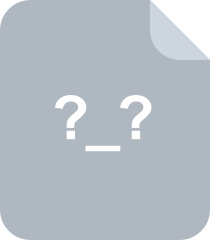
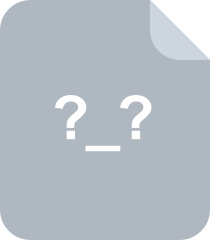
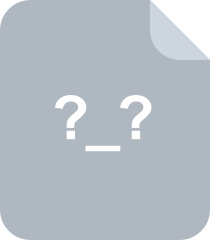
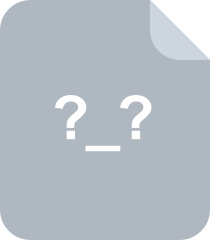
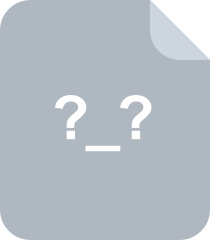
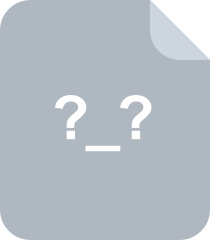
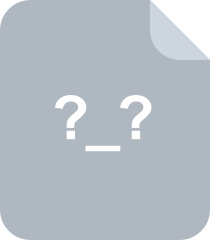
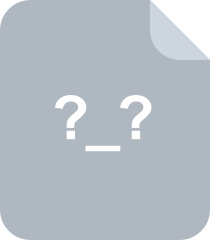
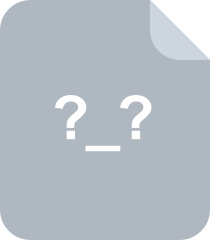
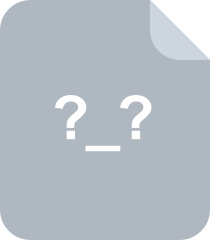
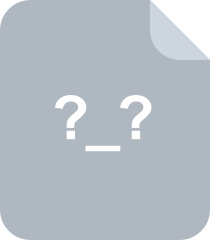
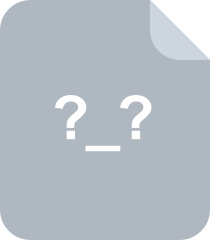
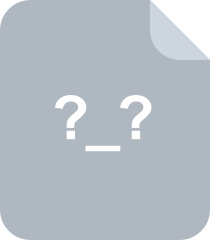
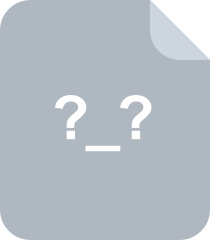
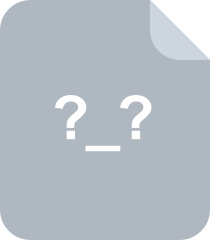
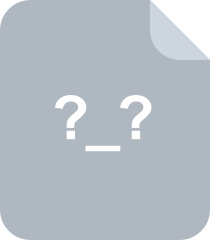
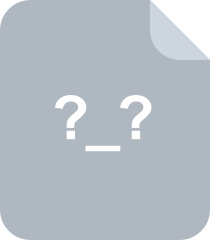
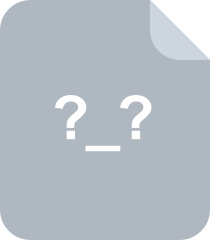
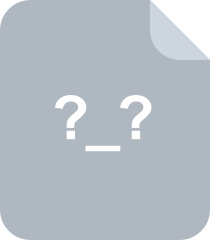
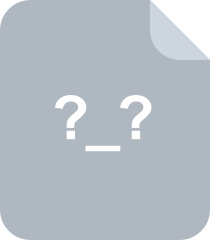
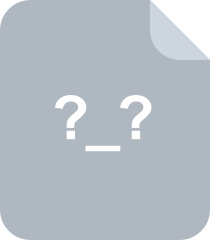
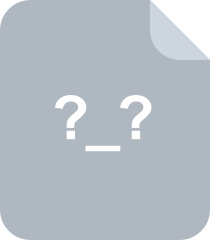
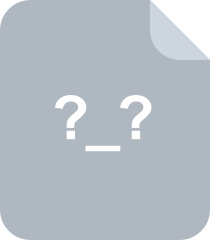
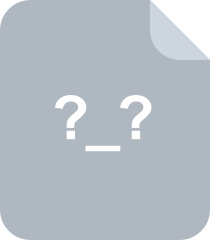
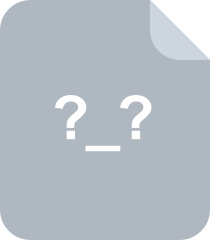
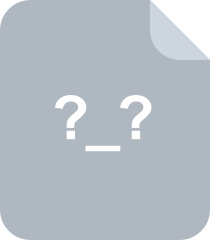
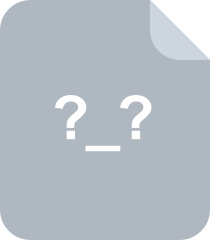
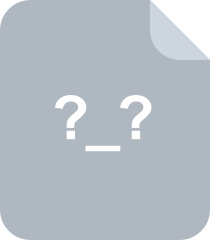
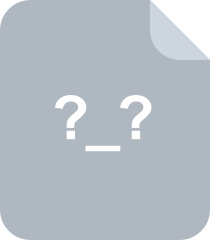
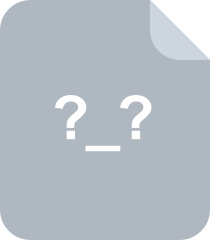
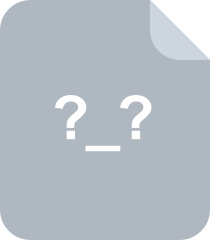
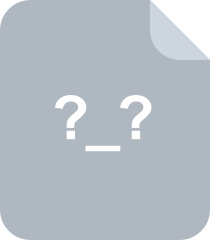
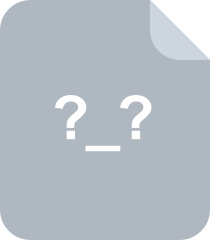
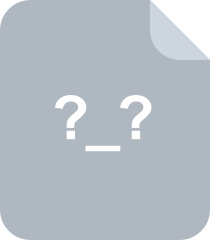
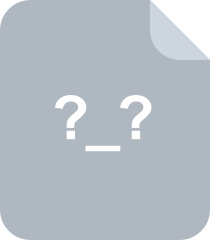
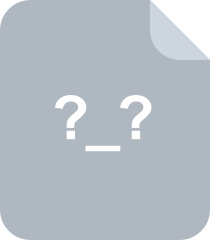
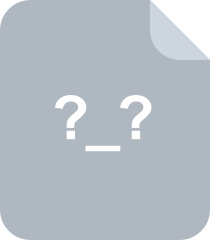
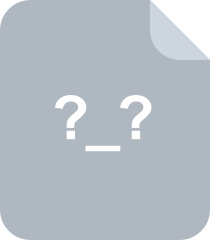
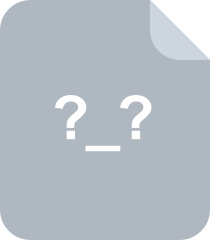
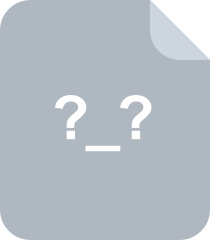
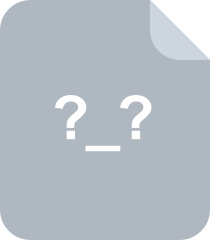
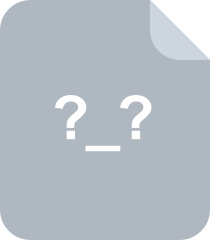
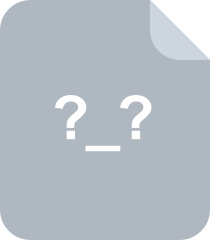
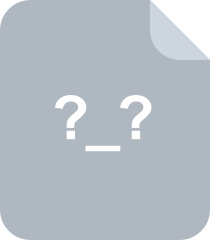
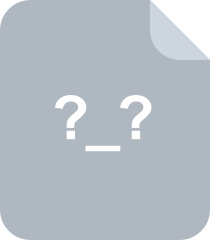
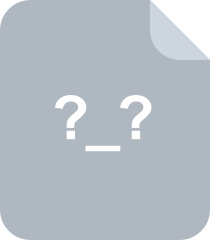
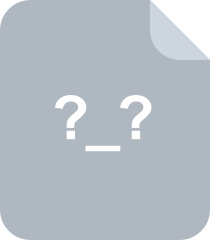
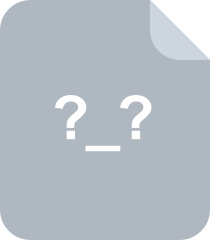
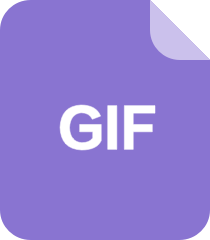
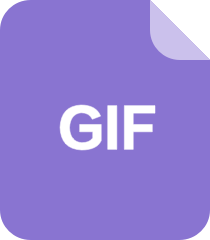
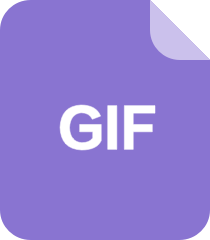
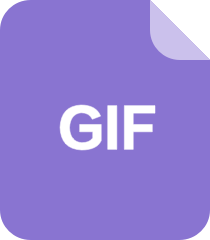
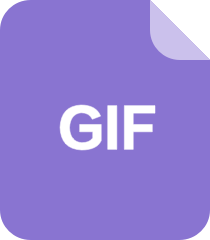
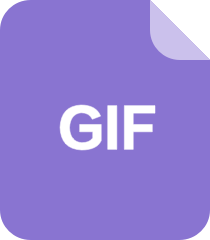
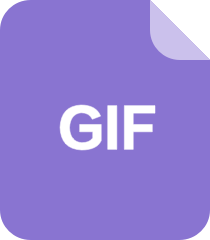
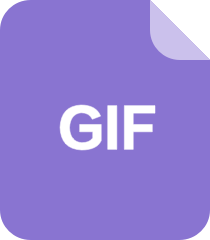
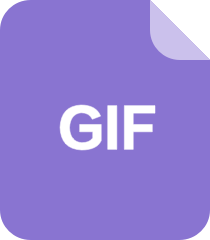
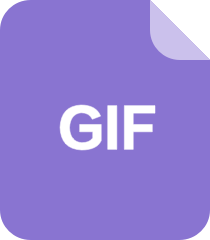
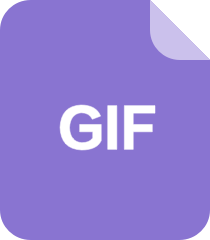
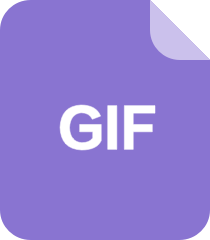
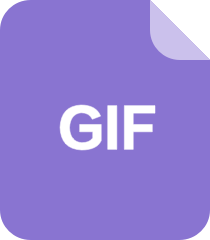
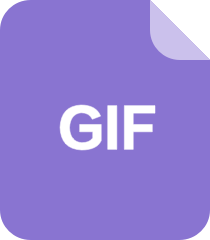
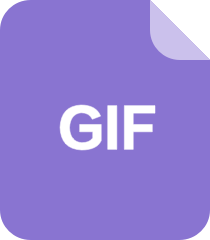
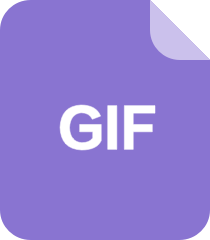
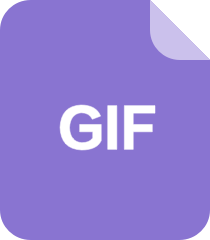
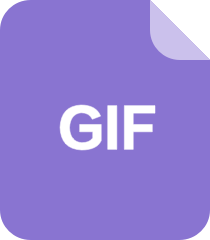
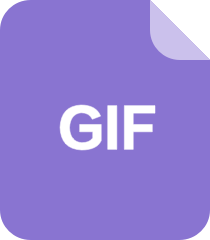
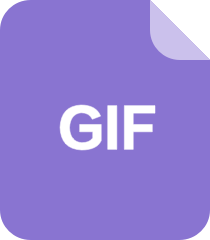
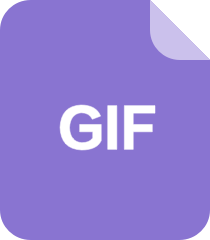
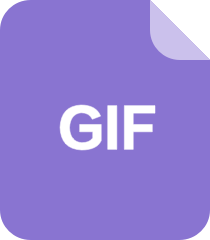
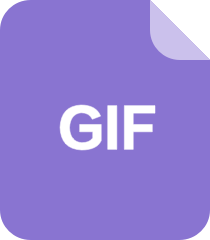
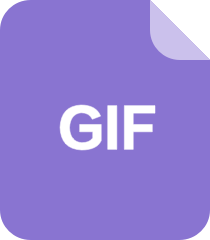
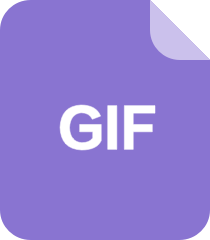
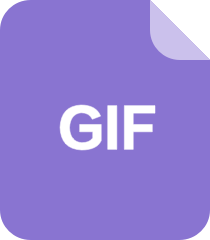
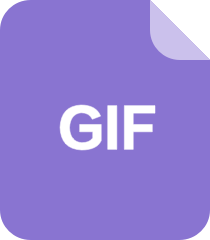
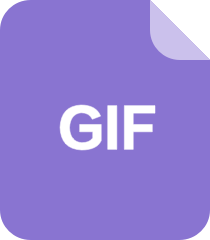
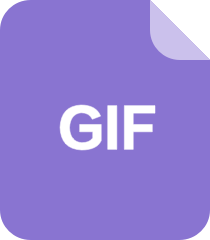
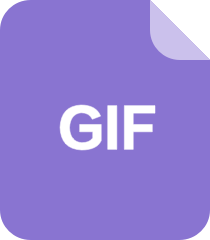
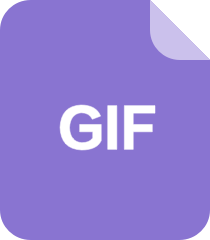
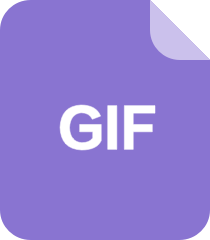
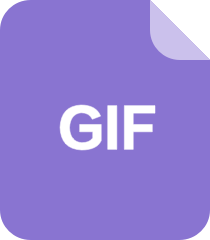
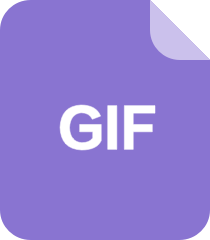
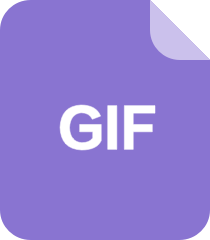
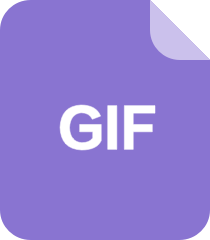
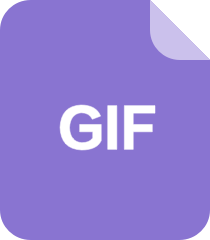
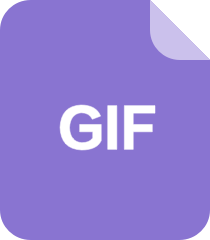
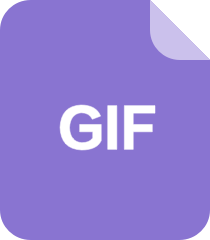
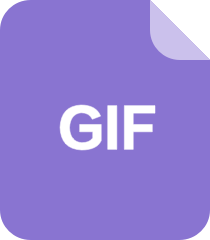
共 318 条
- 1
- 2
- 3
- 4
资源评论
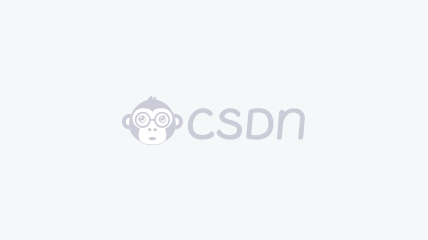

小新要变强
- 粉丝: 3w+
- 资源: 537
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

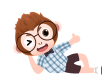
最新资源
- 在贪吃蛇游戏代码中,有关于蛇身操作的函数,如增加蛇块的函数 addnode: 这个函数用于在蛇的前端增加蛇块 如果蛇身存在(if self.body: ),会创建一个新的蛇块(node = pygam
- 诺基亚LTE后台网管操作详解+网络优化
- 台达A2 B2伺服电机编码器改功率软件 台达A2 B2伺服电机编码修改, 用于更编码器写匹配电机参数,更改编码器功率匹配驱动器测试维修用
- HTML和CSS实现简洁圣诞树网页
- fiddler5.0免费永久安装,支持https抓包(内有 fiddlercertmaker.exe),详细安装说明
- java项目,毕业设计-大学生租房系统
- C# 上位机数据上传数据库WebAPI.zip
- MATLAB中创建圣诞树图形的基本实现方法
- 基于PID的四旋翼无人机轨迹跟踪控制 0. 直接运行simulink仿真文件.slx 1. 如果出现文件或变量不能识别的警告或错误,建议将文件夹添加到matlab搜索路径以检索到所需文件,或者进入到
- SAP Query快速报表出具
- 匈牙利算法(简单易懂) - CillyB的博客 - CSDN博客.webarchive
- 温室大棚除雪装置的设计(sw12可编辑+CAD+说明书)全套技术开发资料100%好用.zip
- 学术规范与论文写作.docx
- 套箱封箱贴标签一体化包装线sw12可编辑全套技术开发资料100%好用.zip
- GNSS IMU, INS学习必备教材
- HTML5实现好看的图书音乐点评网站模板.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


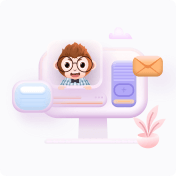
安全验证
文档复制为VIP权益,开通VIP直接复制
