/*
* Copyright (c) 2012-2018 Red Hat, Inc.
* This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0
* which is available at https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* Red Hat, Inc. - initial API and implementation
*/
package org.eclipse.che.ide.ext.git.client;
import static java.util.Arrays.stream;
import static java.util.stream.Collectors.toList;
import static org.eclipse.che.api.git.shared.AddRequest.DEFAULT_PATTERN;
import static org.eclipse.che.ide.MimeType.APPLICATION_JSON;
import static org.eclipse.che.ide.MimeType.TEXT_PLAIN;
import static org.eclipse.che.ide.resource.Path.valueOf;
import static org.eclipse.che.ide.rest.HTTPHeader.ACCEPT;
import static org.eclipse.che.ide.rest.HTTPHeader.CONTENTTYPE;
import static org.eclipse.che.ide.util.PathEncoder.encodePath;
import com.google.inject.Inject;
import com.google.inject.Singleton;
import java.util.List;
import java.util.Map;
import org.eclipse.che.api.git.shared.AddRequest;
import org.eclipse.che.api.git.shared.Branch;
import org.eclipse.che.api.git.shared.BranchCreateRequest;
import org.eclipse.che.api.git.shared.BranchListMode;
import org.eclipse.che.api.git.shared.CheckoutRequest;
import org.eclipse.che.api.git.shared.CloneRequest;
import org.eclipse.che.api.git.shared.CommitRequest;
import org.eclipse.che.api.git.shared.DiffType;
import org.eclipse.che.api.git.shared.EditedRegion;
import org.eclipse.che.api.git.shared.FetchRequest;
import org.eclipse.che.api.git.shared.LogResponse;
import org.eclipse.che.api.git.shared.MergeRequest;
import org.eclipse.che.api.git.shared.MergeResult;
import org.eclipse.che.api.git.shared.PullRequest;
import org.eclipse.che.api.git.shared.PullResponse;
import org.eclipse.che.api.git.shared.PushRequest;
import org.eclipse.che.api.git.shared.PushResponse;
import org.eclipse.che.api.git.shared.Remote;
import org.eclipse.che.api.git.shared.RemoteAddRequest;
import org.eclipse.che.api.git.shared.ResetRequest;
import org.eclipse.che.api.git.shared.RevertRequest;
import org.eclipse.che.api.git.shared.RevertResult;
import org.eclipse.che.api.git.shared.Revision;
import org.eclipse.che.api.git.shared.ShowFileContentResponse;
import org.eclipse.che.api.git.shared.Status;
import org.eclipse.che.api.promises.client.Promise;
import org.eclipse.che.ide.MimeType;
import org.eclipse.che.ide.api.app.AppContext;
import org.eclipse.che.ide.api.auth.Credentials;
import org.eclipse.che.ide.dto.DtoFactory;
import org.eclipse.che.ide.resource.Path;
import org.eclipse.che.ide.rest.AsyncRequest;
import org.eclipse.che.ide.rest.AsyncRequestFactory;
import org.eclipse.che.ide.rest.AsyncRequestLoader;
import org.eclipse.che.ide.rest.DtoUnmarshallerFactory;
import org.eclipse.che.ide.rest.StringMapUnmarshaller;
import org.eclipse.che.ide.rest.StringUnmarshaller;
import org.eclipse.che.ide.ui.loaders.request.LoaderFactory;
/**
* Implementation of the {@link GitServiceClient}.
*
* @author Ann Zhuleva
* @author Valeriy Svydenko
*/
@Singleton
public class GitServiceClientImpl implements GitServiceClient {
private static final String ADD = "/git/add";
private static final String BRANCH = "/git/branch";
private static final String CHECKOUT = "/git/checkout";
private static final String CLONE = "/git/clone";
private static final String COMMIT = "/git/commit";
private static final String CONFIG = "/git/config";
private static final String DIFF = "/git/diff";
private static final String EDITS = "/git/edits";
private static final String FETCH = "/git/fetch";
private static final String INIT = "/git/init";
private static final String LOG = "/git/log";
private static final String SHOW = "/git/show";
private static final String MERGE = "/git/merge";
private static final String STATUS = "/git/status";
private static final String PUSH = "/git/push";
private static final String PULL = "/git/pull";
private static final String REMOTE = "/git/remote";
private static final String REMOVE = "/git/remove";
private static final String RESET = "/git/reset";
private static final String REPOSITORY = "/git/repository";
private static final String REVERT = "/git/revert";
/** Loader to be displayed. */
private final AsyncRequestLoader loader;
private final DtoFactory dtoFactory;
private final DtoUnmarshallerFactory dtoUnmarshallerFactory;
private final AsyncRequestFactory asyncRequestFactory;
private final AppContext appContext;
@Inject
protected GitServiceClientImpl(
LoaderFactory loaderFactory,
DtoFactory dtoFactory,
AsyncRequestFactory asyncRequestFactory,
DtoUnmarshallerFactory dtoUnmarshallerFactory,
AppContext appContext) {
this.appContext = appContext;
this.loader = loaderFactory.newLoader();
this.dtoFactory = dtoFactory;
this.asyncRequestFactory = asyncRequestFactory;
this.dtoUnmarshallerFactory = dtoUnmarshallerFactory;
}
@Override
public Promise<Void> init(Path project, boolean bare) {
String url =
getWsAgentBaseUrl() + INIT + "?projectPath=" + encodePath(project) + "&bare=" + bare;
return asyncRequestFactory.createPostRequest(url, null).loader(loader).send();
}
@Override
public Promise<Void> clone(Path project, String remoteUri, String remoteName) {
CloneRequest cloneRequest =
dtoFactory
.createDto(CloneRequest.class)
.withRemoteName(remoteName)
.withRemoteUri(remoteUri)
.withWorkingDir(project.toString());
String params = "?projectPath=" + encodePath(project);
String url = CLONE + params;
return asyncRequestFactory.createPostRequest(url, cloneRequest).loader(loader).send();
}
@Override
public Promise<String> statusText(Path project) {
String params = "?projectPath=" + encodePath(project);
String url = getWsAgentBaseUrl() + STATUS + params;
return asyncRequestFactory
.createGetRequest(url)
.loader(loader)
.header(CONTENTTYPE, APPLICATION_JSON)
.header(ACCEPT, TEXT_PLAIN)
.send(new StringUnmarshaller());
}
@Override
public Promise<Void> add(Path project, boolean update, Path[] paths) {
AddRequest addRequest = dtoFactory.createDto(AddRequest.class).withUpdate(update);
addRequest.setFilePattern(
paths == null
? DEFAULT_PATTERN
: stream(paths).map(path -> path.isEmpty() ? "." : path.toString()).collect(toList()));
String url = getWsAgentBaseUrl() + ADD + "?projectPath=" + encodePath(project);
return asyncRequestFactory.createPostRequest(url, addRequest).loader(loader).send();
}
@Override
public Promise<Revision> commit(Path project, String message, boolean all, boolean amend) {
CommitRequest commitRequest =
dtoFactory
.createDto(CommitRequest.class)
.withMessage(message)
.withAmend(amend)
.withAll(all);
String url = getWsAgentBaseUrl() + COMMIT + "?projectPath=" + encodePath(project);
return asyncRequestFactory
.createPostRequest(url, commitRequest)
.loader(loader)
.send(dtoUnmarshallerFactory.newUnmarshaller(Revision.class));
}
@Override
public Promise<Revision> commit(Path project, String message, boolean amend, Path[] files) {
CommitRequest commitRequest =
dtoFactory
.createDto(CommitRequest.class)
.withMessage(message)
.withAmend(amend)
.withFiles(
stream(files)
.filter(file -> !file.isEmpty())
.map(Path::toString)
.collect(toList()));
String url = getWsAgentBaseUrl() + COMMIT + "?projectPath=" + encodePath(project);
return asyncRequestFactory
.createPostRequest(url, commitRequest)
.loader(loader)
.send(dtoUnmarshallerFactory.newUnmarshaller(Revision.class));
}
@Override
pu
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
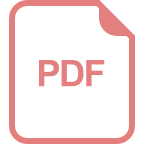
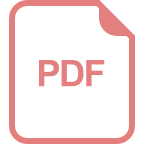
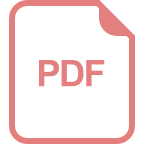
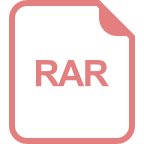
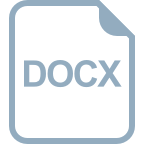
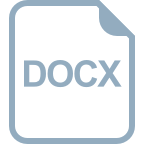
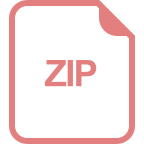
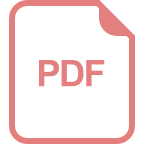
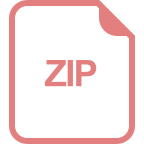
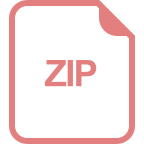
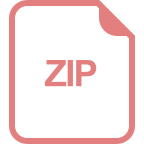
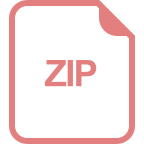
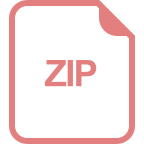
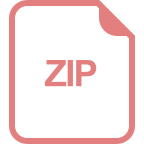
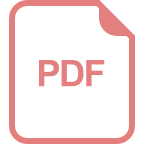
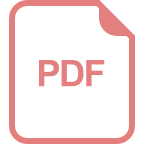
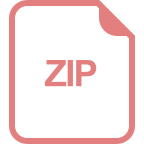
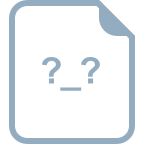
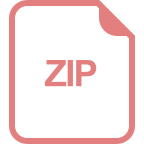
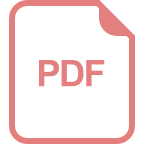
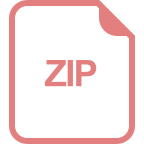
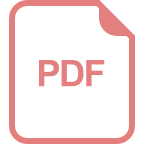
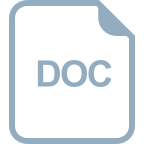
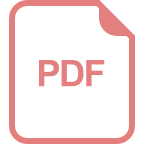
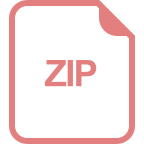
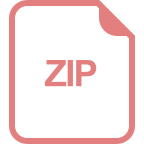
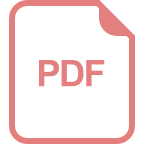
收起资源包目录

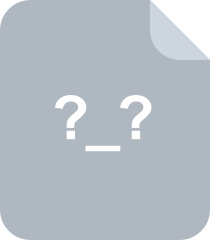
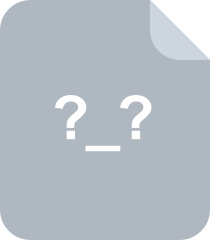
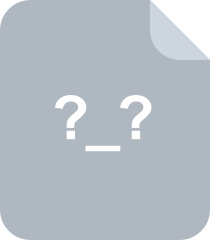
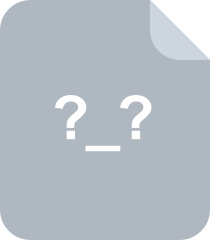
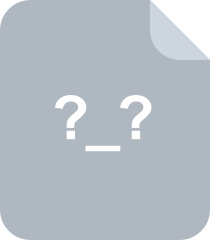
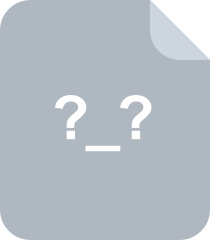
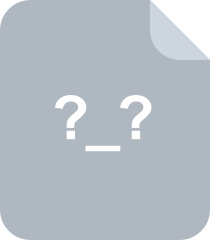
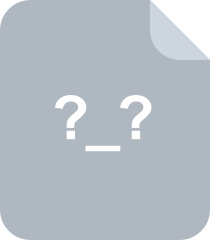
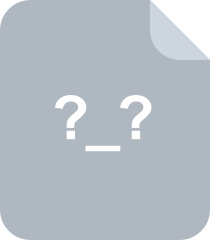
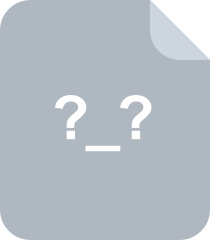
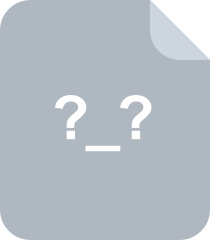
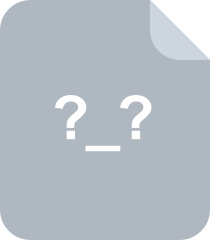
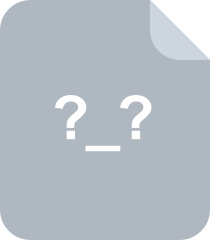
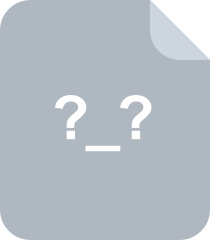
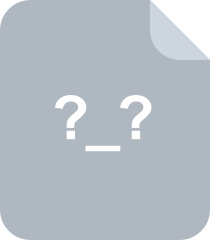
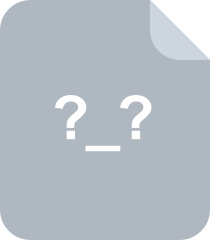
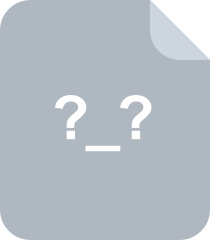
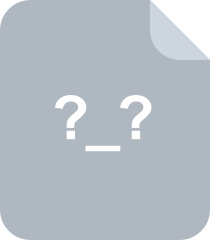
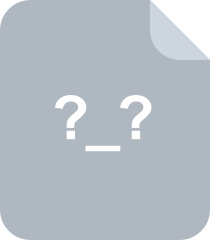
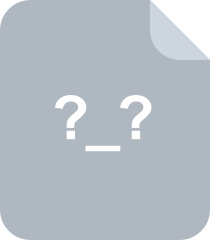
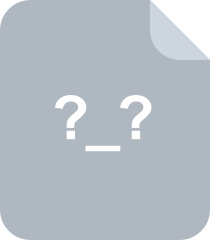
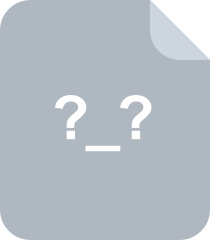
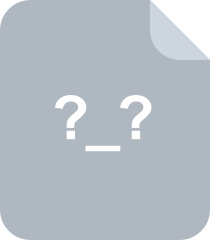
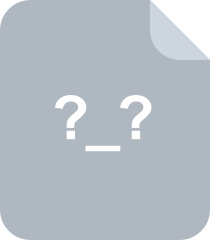
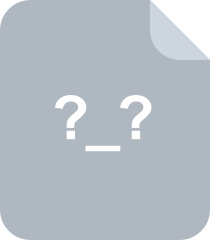
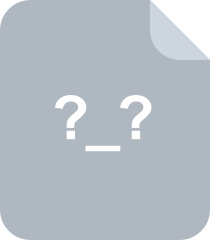
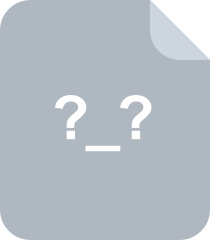
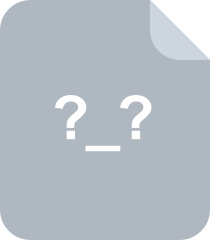
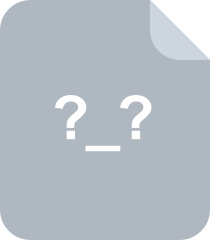
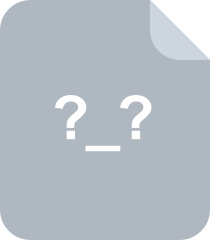
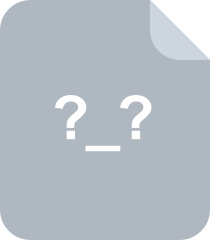
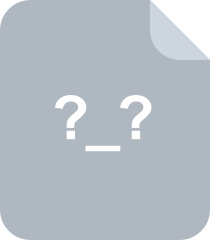
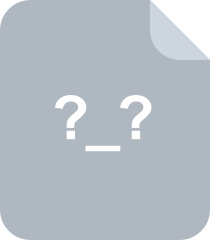
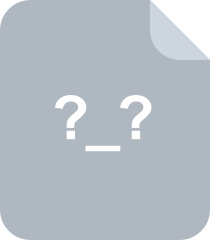
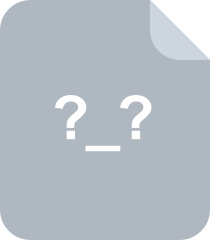
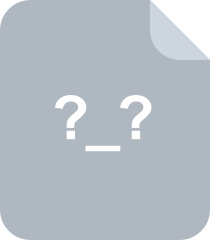
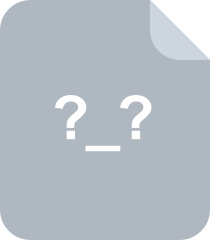
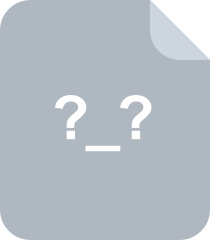
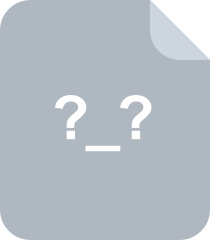
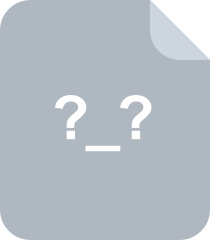
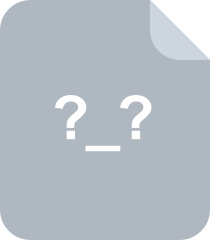
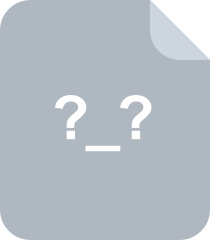
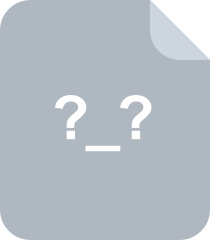
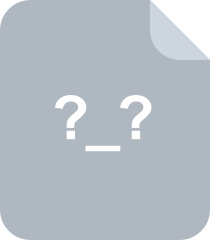
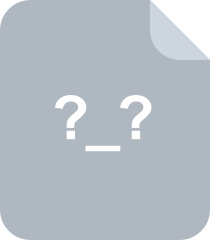
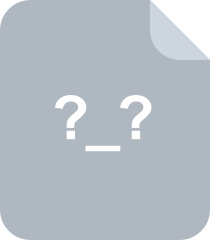
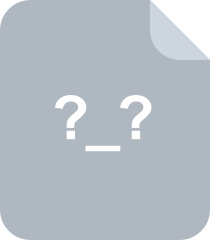
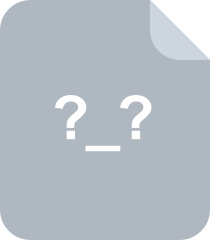
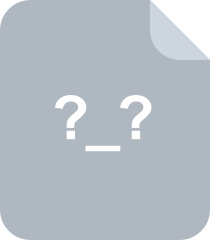
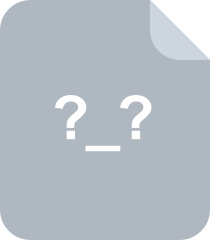
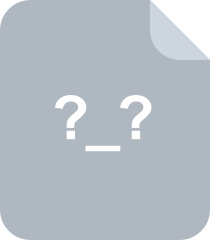
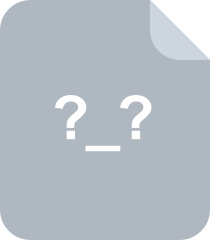
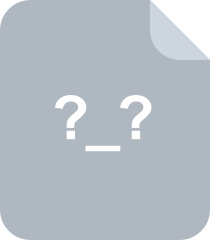
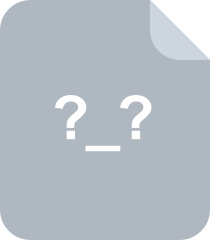
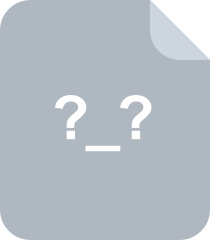
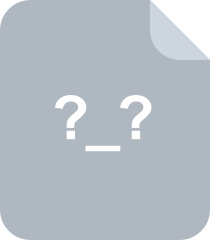
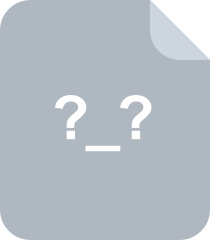
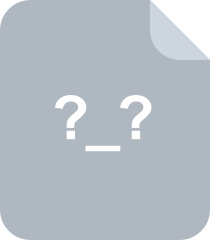
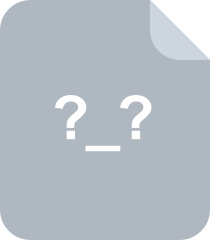
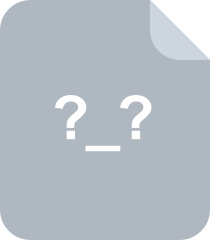
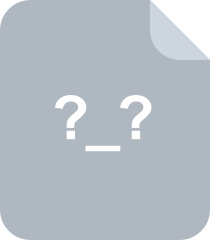
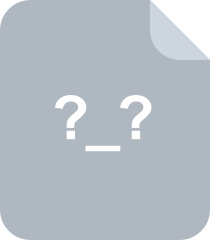
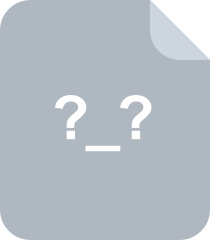
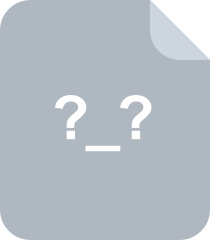
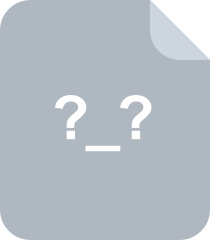
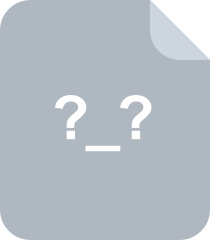
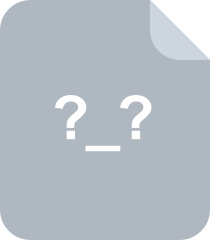
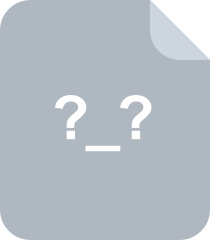
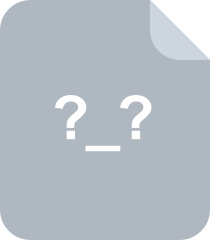
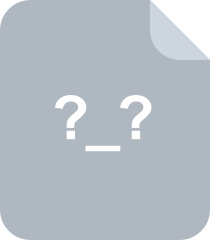
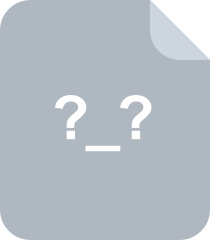
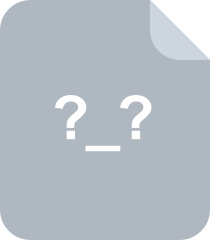
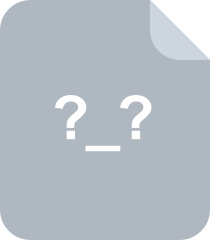
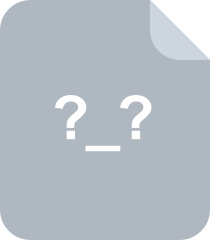
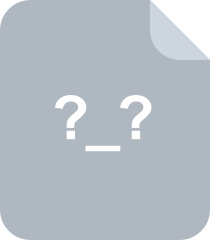
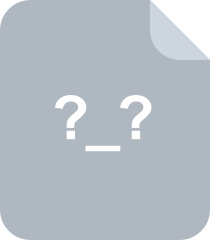
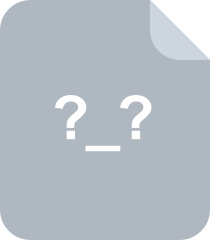
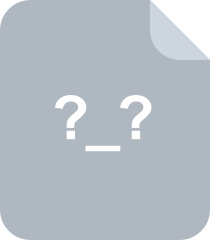
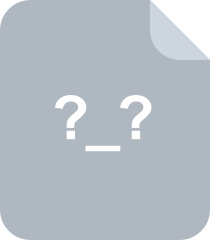
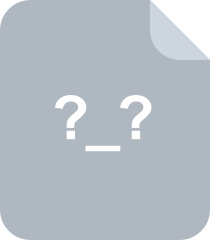
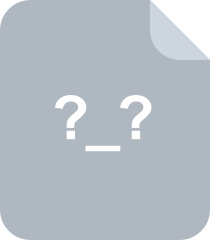
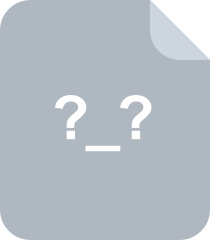
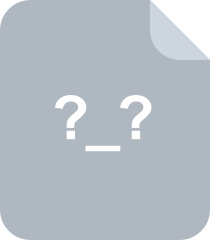
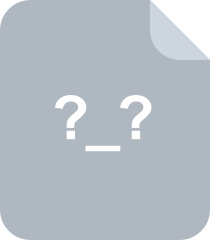
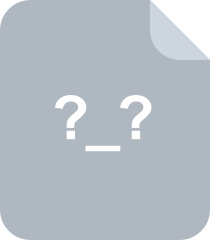
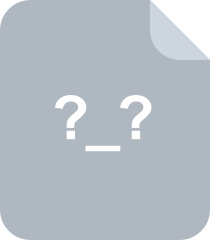
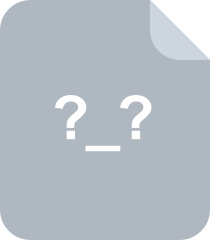
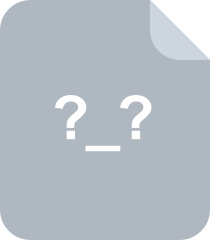
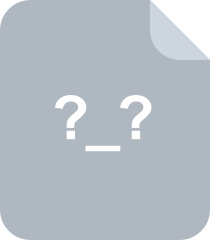
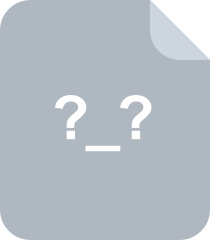
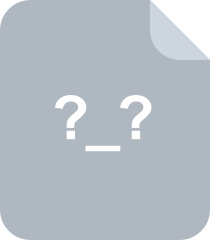
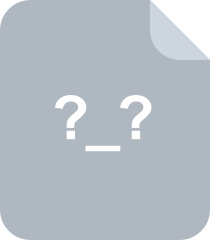
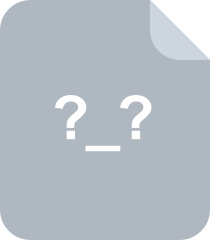
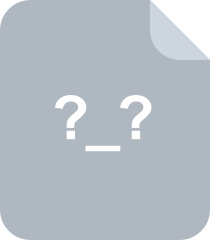
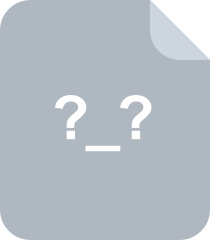
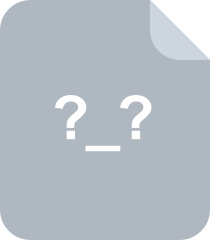
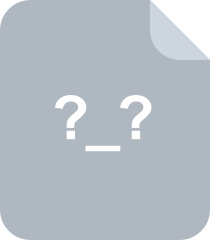
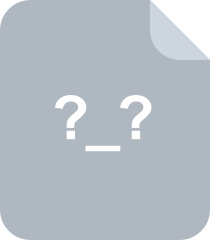
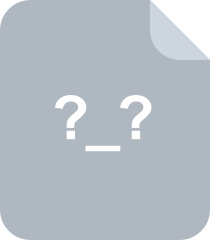
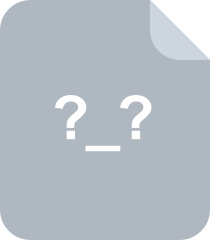
共 387 条
- 1
- 2
- 3
- 4
资源评论
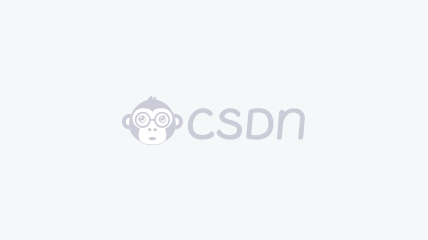

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7451
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

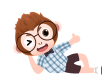
安全验证
文档复制为VIP权益,开通VIP直接复制
