This folder contains a [jsonstore](https://github.com/bluzi/jsonstore)-like storage service. But is multi-threaded and stores everything in memory. Serving as a showcase on how to build a minimally useful RESTful APIs in Drogon.
## API
#### /get-token
Generate a token that the user can use to create, read, modify and delete records.
* **method**: GET
* **URL params**: None
* **Body**: None
* **Success response**
* **Code**: 200
* **Content**: `{"token":"3a322920d42ef0763152a6efff2ed51985530aedd45370f92fd0f0b8dcc30220"}`
* **Sample call**
```bash
❯ curl -XGET http://localhost:8848/get-token
{"token":"3a322920d42ef0763152a6efff2ed51985530aedd45370f92fd0f0b8dcc30220"}
```
#### /{token}
Create a new JSON object associated with the token
* **method**: POST
* **URL params**: None
* **Body**: The initial JSON object to store
* **Success response**
* **Code**: 200
* **Content**: `{"ok":true}`
* **Failed response**:
* **Code**: 500
* **Why**: Either the token already is associated with the data or request body/content-type is not JSON.
* **Content**: `{"ok":false}`
* **Sample call**
```bash
❯ curl -XPOST http://localhost:8848/3a322920d42ef0763152a6efff2ed51985530aedd45370f92fd0f0b8dcc30220 \
-H 'content-type: application/json' -d '{"foo":{"bar":42}}'
{"ok":true}
```
Delete the JSON object associated with the token
* **method**: DELETE
* **URL params**: None
* **Body**: None
* **Success response**
* **Code**: 200
* **Content**: `{"ok":true}`
* **Sample call**
```bash
❯ curl -XDELETE http://localhost:8848/3a322920d42ef0763152a6efff2ed51985530aedd45370f92fd0f0b8dcc30220
{"ok":true}
```
#### /{token}/{some/path/to/data}
Retrieve data at and below the specified path
* **method**: GET
* **URL params**: None
* **Body**: None
* **Success response**
* **Code**: 200
* **Content**: `{"foo":{"bar":42}}`
* **Failed response**:
* **Code**: 500
* **Why**: No data associated with the provided token, or it does not exist in the JSON object.
* **Content**: `{"ok":false}`
* **Sample call**
```bash
❯ curl -XGET http://localhost:8848/3a322920d42ef0763152a6efff2ed51985530aedd45370f92fd0f0b8dcc30220/
{"foo":{"bar":42}}
```
Update data at the specified path
* **method**: PUT
* **URL params**: None
* **Body**: The JSON object you wish to replace to
* **Success response**
* **Code**: 200
* **Content**: `{"ok":true}`
* **Failed response**:
* **Code**: 500
* **Why**: No data associated with the provided token or it does not exist in the JSON object.
* **Content**: `{"ok":false}`
* **Sample call**
```bash
❯ curl -XPUT http://localhost:8848/3a322920d42ef0763152a6efff2ed51985530aedd45370f92fd0f0b8dcc30220/foo \
-H 'content-type: application/json' -d '{"fruit":"apple"}'
{"ok":true}
```
## Example use
```bash
export URL="http://localhost:8848"
export TOKEN=`curl $URL/get-token -s | sed 's/.*"\([0-9a-f]*\)".*/\1/'`
printf "Token is: $TOKEN\n"
printf 'Creating new data \n> '
curl -XPOST $URL/$TOKEN -H 'content-type: application/json' -d '{"foo":{"bar":42}}'
printf '\nRetrieving value of data["foo"]["bar"] \n> '
curl $URL/$TOKEN/foo/bar
printf '\nModifing data \n> '
curl -XPUT $URL/$TOKEN/foo -H 'content-type: application/json' -d '{"zoo":"zebra"}'
printf '\nNow data["foo"]["bar"] no longer exists \n> '
curl $URL/$TOKEN/foo/bar
printf '\nDelete data \n> '
curl -XDELETE $URL/$TOKEN
echo
```
Output:
```
Token is: 5e73ba044b45e68b4856925faea268391091f39fc62ab8c58955cf20957018fa
Creating new data
> {"ok":true}
Retrieving value of data["foo"]["bar"]
> 42
Modifying data
> {"ok":true}
Now data["foo"]["bar"] no longer exists
> {"ok":false}
Delete data
> {"ok":true}
```
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Drogon是一个基于C++17/20的Http应用框架,使用Drogon可以方便的使用C++构建各种类型的Web应用服务端程序。 本版本库是github上Drogon工程的镜像库。Drogon是作者非常喜欢的美剧《权力的游戏》中的一条龙的名字(汉译作卓耿),和龙有关但并不是dragon的误写,为了不至于引起不必要的误会这里说明一下。Drogon是一个跨平台框架,它支持Linux,也支持macOS、FreeBSD,OpenBSD,HaikuOS,和Windows。
资源推荐
资源详情
资源评论
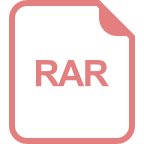
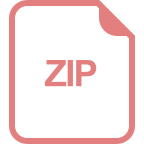
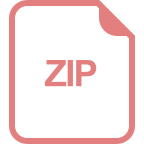
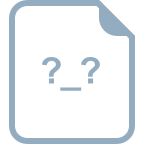
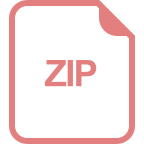
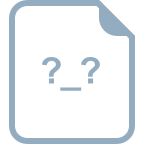
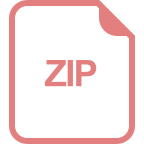
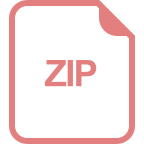
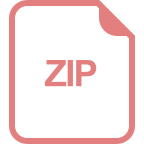
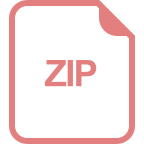
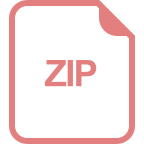
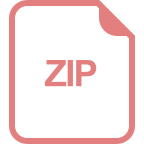
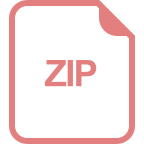
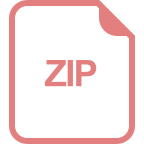
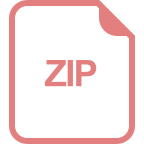
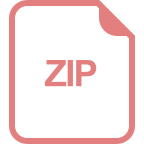
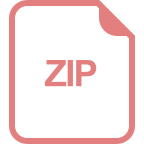
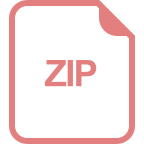
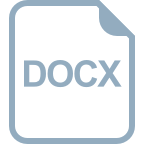
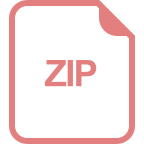
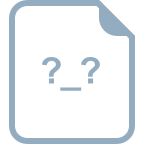
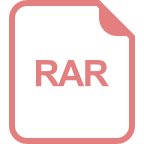
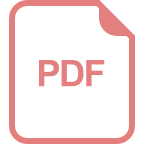
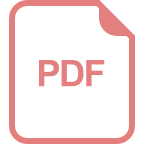
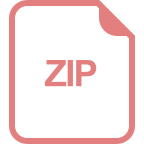
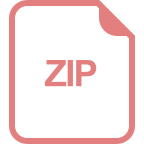
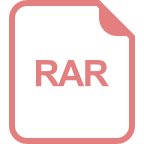
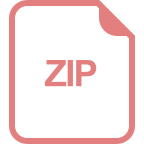
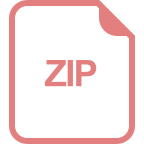
收起资源包目录

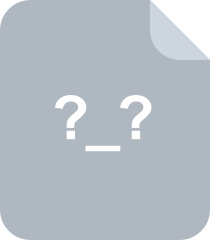
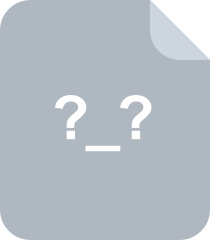
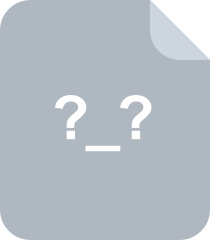
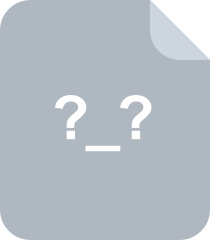
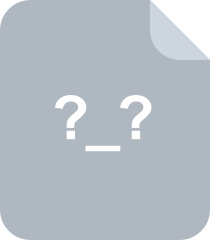
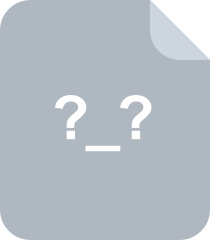
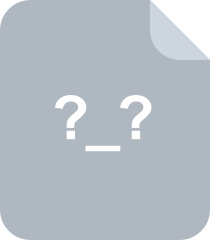
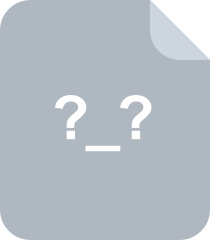
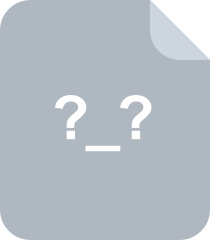
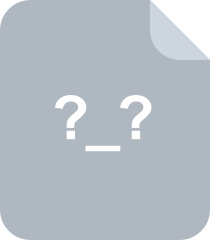
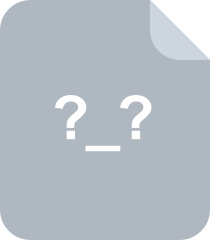
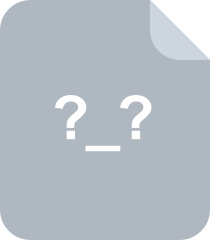
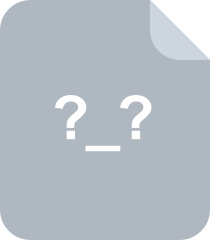
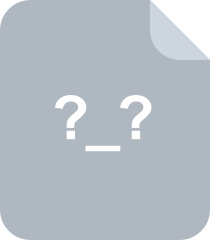
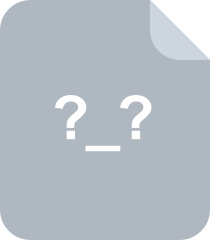
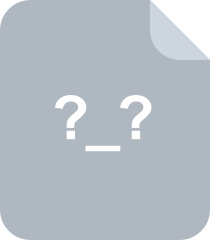
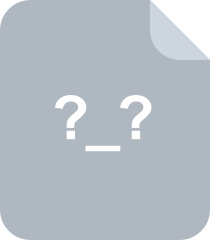
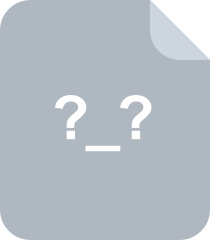
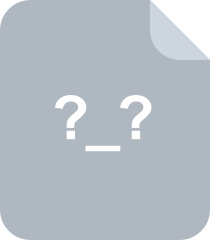
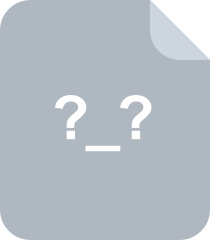
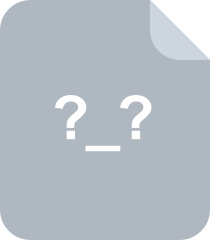
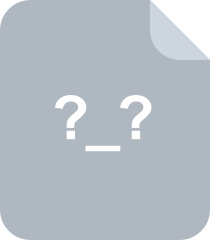
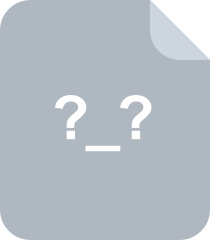
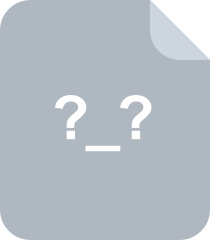
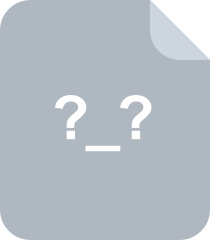
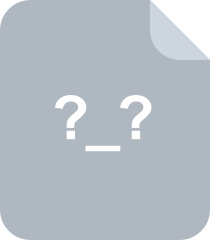
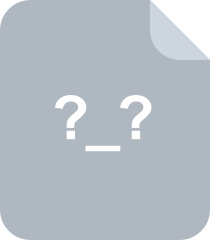
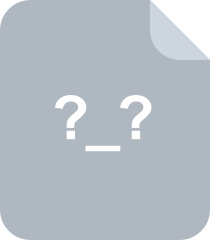
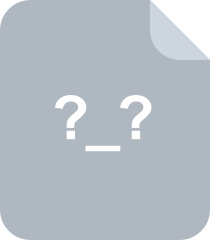
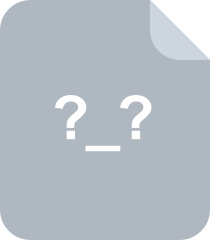
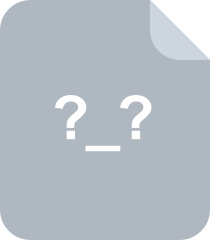
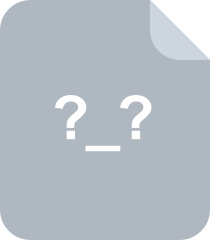
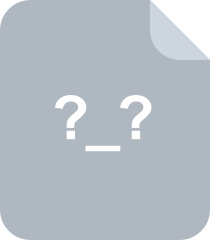
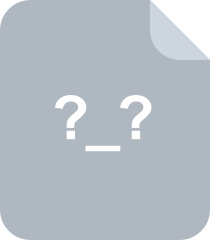
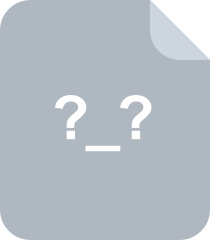
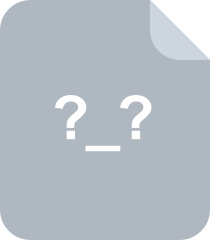
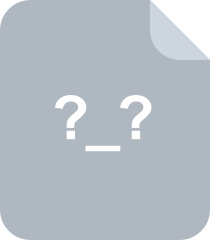
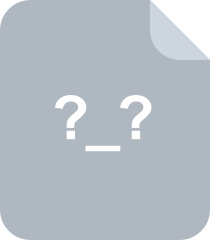
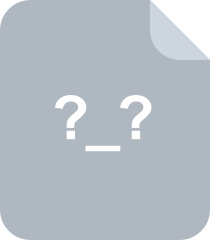
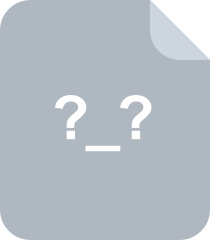
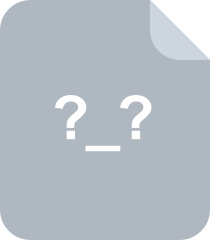
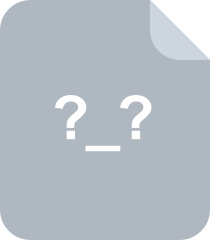
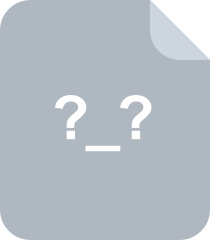
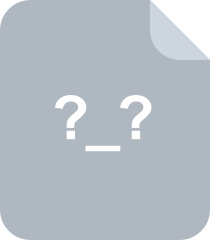
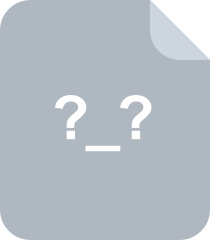
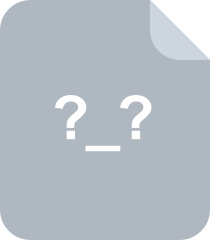
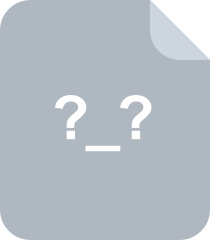
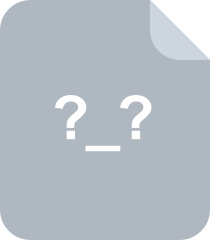
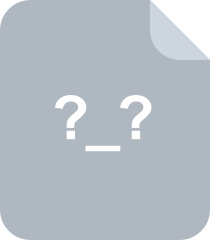
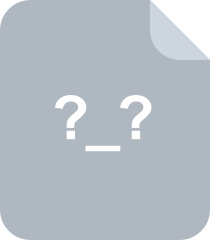
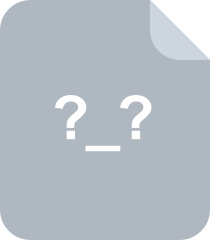
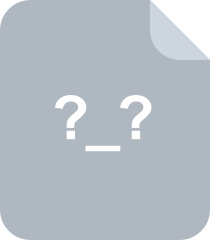
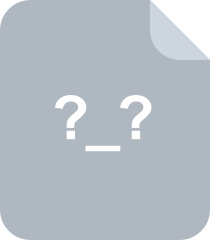
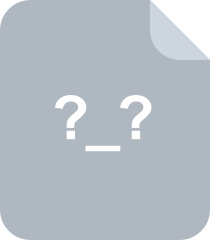
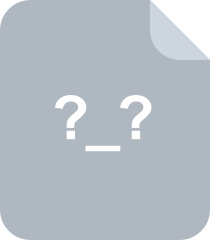
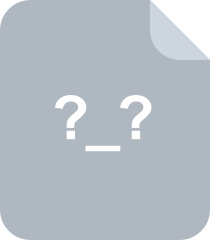
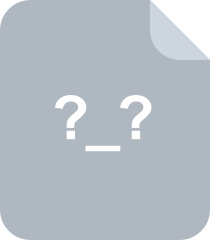
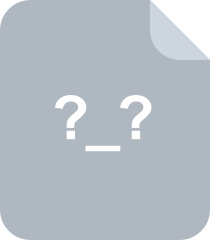
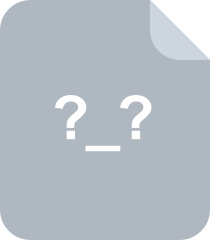
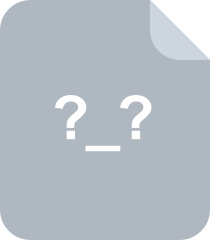
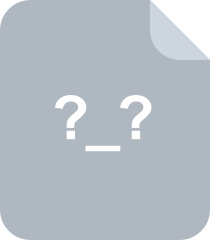
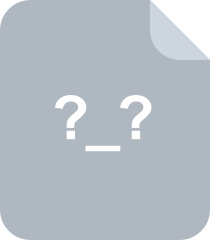
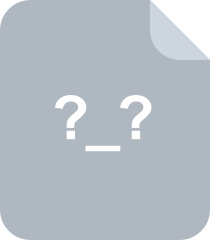
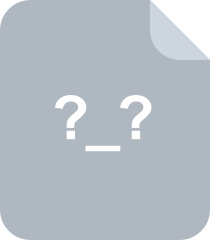
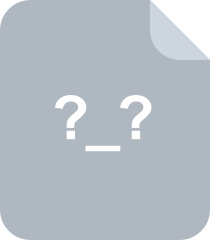
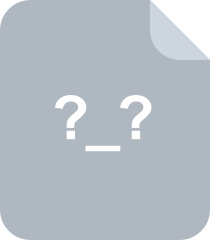
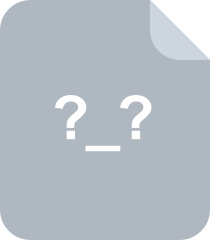
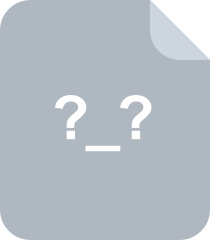
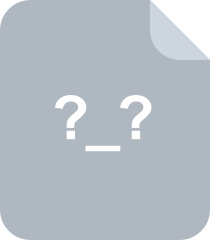
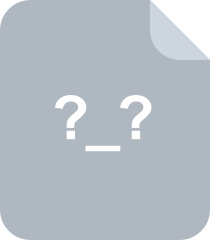
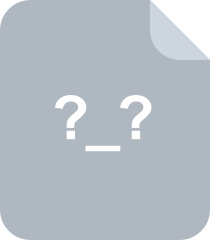
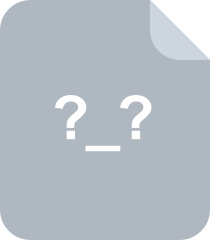
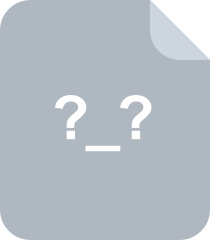
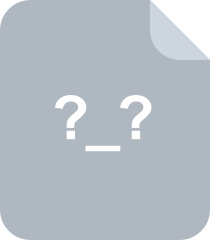
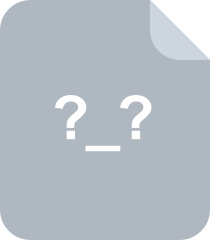
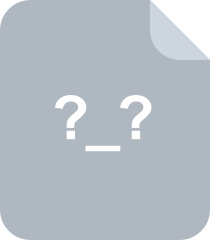
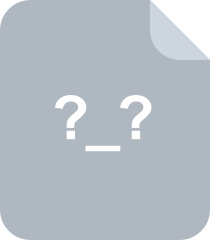
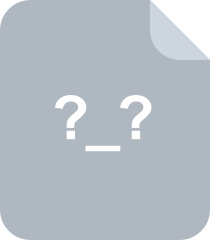
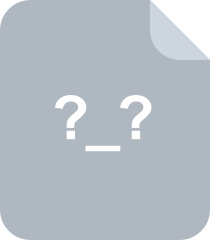
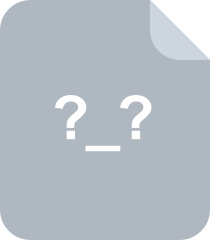
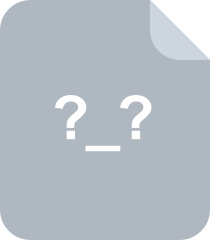
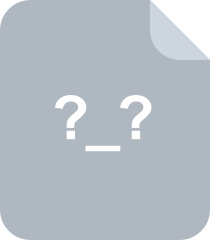
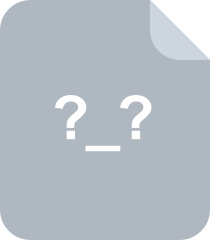
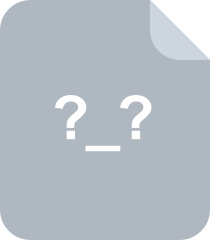
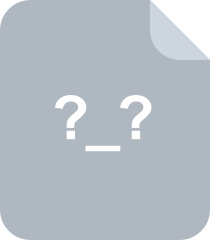
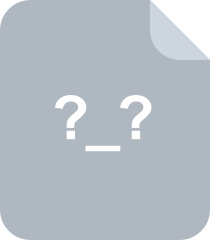
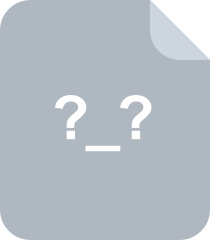
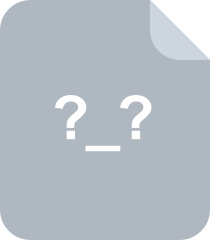
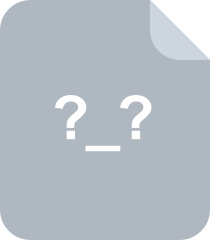
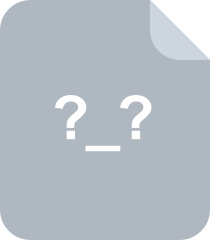
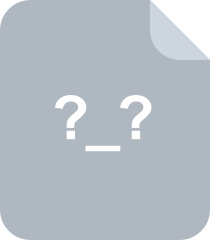
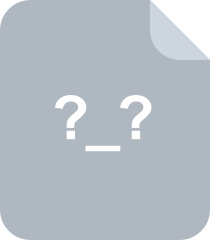
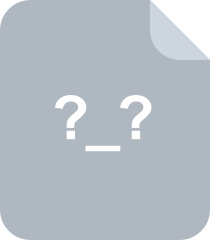
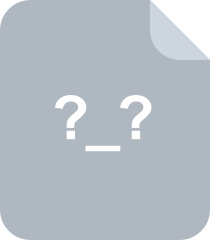
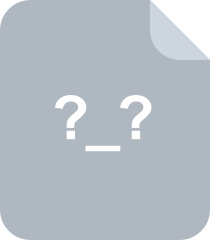
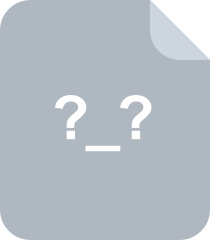
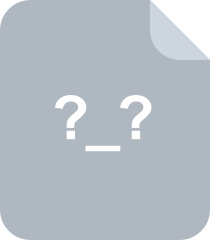
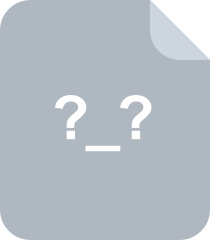
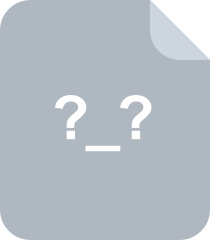
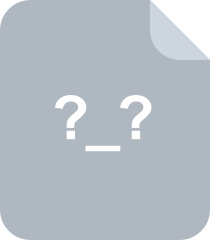
共 541 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
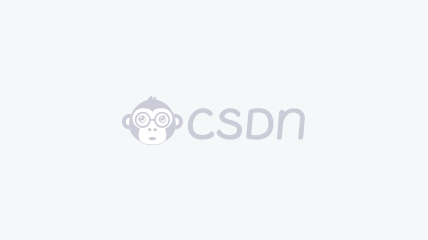

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7364
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

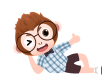
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


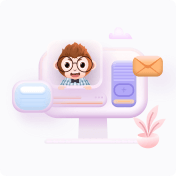
安全验证
文档复制为VIP权益,开通VIP直接复制
