/**
* The MIT License (MIT)
* Copyright (c) 2012-present 铭软科技(mingsoft.net)
* Permission is hereby granted, free of charge, to any person obtaining a copy of
* this software and associated documentation files (the "Software"), to deal in
* the Software without restriction, including without limitation the rights to
* use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
* the Software, and to permit persons to whom the Software is furnished to do so,
* subject to the following conditions:
* <p>
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
* <p>
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
* FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
* COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
* IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package net.mingsoft.cms.action;
import cn.hutool.core.io.file.FileNameUtil;
import cn.hutool.core.util.StrUtil;
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.core.toolkit.Wrappers;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiImplicitParam;
import io.swagger.annotations.ApiImplicitParams;
import io.swagger.annotations.ApiOperation;
import net.mingsoft.base.entity.ResultData;
import net.mingsoft.basic.annotation.LogAnn;
import net.mingsoft.basic.bean.EUListBean;
import net.mingsoft.basic.constant.e.BusinessTypeEnum;
import net.mingsoft.basic.util.BasicUtil;
import net.mingsoft.basic.util.PinYinUtil;
import net.mingsoft.basic.util.StringUtil;
import net.mingsoft.cms.biz.ICategoryBiz;
import net.mingsoft.cms.constant.e.CategoryTypeEnum;
import net.mingsoft.cms.entity.CategoryEntity;
import net.mingsoft.mdiy.util.ParserUtil;
import org.apache.commons.lang3.StringUtils;
import org.apache.shiro.authz.annotation.RequiresPermissions;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.*;
import springfox.documentation.annotations.ApiIgnore;
import java.util.List;
/**
* 分类管理控制层
* @author 铭飞开发团队
* 创建日期:2019-11-28 15:12:32<br/>
* 历史修订:<br/>
*/
@Api(tags = {"后端-内容模块接口"})
@Controller("cmsCategoryAction")
@RequestMapping("/${ms.manager.path}/cms/category")
public class CategoryAction extends BaseAction {
/**
* 注入分类业务层
*/
@Autowired
private ICategoryBiz categoryBiz;
/**
* 返回主界面index
* @return
*/
@ApiIgnore
@GetMapping("/index")
@RequiresPermissions("cms:category:view")
public String index() {
return "/cms/category/index";
}
/**
* 查询分类列表接口
* @param category 栏目实体
* @return
*/
@ApiOperation(value = "查询分类列表接口")
@ApiImplicitParams({
@ApiImplicitParam(name = "categoryTitle", value = "栏目管理名称", required = false, paramType = "query"),
@ApiImplicitParam(name = "categoryParentId", value = "父类型编号", required = false, paramType = "query"),
})
@RequestMapping(value = "/list", method = {RequestMethod.GET, RequestMethod.POST})
@ResponseBody
public ResultData list(@ModelAttribute @ApiIgnore CategoryEntity category) {
List categoryList = categoryBiz.list(new LambdaQueryWrapper<CategoryEntity>(category));
return ResultData.build().success(new EUListBean(categoryList, categoryList.size()));
}
/**
* 返回编辑界面category_form
* @param category 栏目
* @return
*/
@ApiIgnore
@GetMapping("/form")
public String form(@ModelAttribute CategoryEntity category, ModelMap model) {
model.addAttribute("appId", BasicUtil.getApp().getAppId());
return "/cms/category/form";
}
/**
* 获取分类
* @param category 分类实体
*/
@ApiOperation(value = "获取分类详情接口")
@ApiImplicitParam(name = "id", value = "编号", required = true, paramType = "query")
@GetMapping("/get")
@RequiresPermissions("cms:category:view")
@ResponseBody
public ResultData get(@ModelAttribute @ApiIgnore CategoryEntity category) {
if (StringUtils.isBlank(category.getId())) {
return ResultData.build().error(getResString("err.empty",this.getResString("id")));
}
CategoryEntity _category = (CategoryEntity) categoryBiz.getById(category.getId());
return ResultData.build().success(_category);
}
/**
* 保存分类
* @param category 分类实体
*/
@ApiOperation(value = "保存分类列表接口")
@ApiImplicitParams({
@ApiImplicitParam(name = "categoryTitle", value = "栏目管理名称", required = true, paramType = "query"),
@ApiImplicitParam(name = "categoryType", value = "栏目类型,1:列表,2:单篇,3:链接", required = true, paramType = "query"),
@ApiImplicitParam(name = "categoryDisplay", value = "栏目是否显示,enable:显示 disable:不显示", required = true, paramType = "query"),
@ApiImplicitParam(name = "categoryIsSearch", value = "栏目是否可搜索,enable:可搜索 disable:不可搜索", required = true, paramType = "query"),
@ApiImplicitParam(name = "categoryId", value = "所属栏目", required = false, paramType = "query"),
@ApiImplicitParam(name = "categorySort", value = "自定义顺序", required = false, paramType = "query"),
@ApiImplicitParam(name = "categoryListUrl", value = "列表模板", required = false, paramType = "query"),
@ApiImplicitParam(name = "categoryUrl", value = "内容模板", required = false, paramType = "query"),
@ApiImplicitParam(name = "categoryKeyword", value = "栏目管理关键字", required = false, paramType = "query"),
@ApiImplicitParam(name = "categoryDescrip", value = "栏目管理描述", required = false, paramType = "query"),
@ApiImplicitParam(name = "categoryImg", value = "缩略图", required = false, paramType = "query"),
@ApiImplicitParam(name = "categoryIco", value = "栏目小图", required = false, paramType = "query"),
@ApiImplicitParam(name = "categoryDiyUrl", value = "自定义链接", required = false, paramType = "query"),
@ApiImplicitParam(name = "mdiyModelId", value = "栏目管理的内容模型id", required = false, paramType = "query"),
@ApiImplicitParam(name = "dictId", value = "字典对应编号", required = false, paramType = "query"),
@ApiImplicitParam(name = "categoryFlag", value = "栏目属性", required = false, paramType = "query"),
@ApiImplicitParam(name = "categoryPath", value = "栏目路径", required = false, paramType = "query"),
@ApiImplicitParam(name = "categoryParentIds", value = "父类型编号", required = false, paramType = "query"),
})
@PostMapping("/save")
@ResponseBody
@LogAnn(title = "保存分类", businessType = BusinessTypeEnum.INSERT)
@RequiresPermissions("cms:category:save")
public ResultData save(@ModelAttribute @ApiIgnore CategoryEntity category) {
//验证缩略图参数值是否合法
if (category.getCategoryImg() == null || !category.getCategoryImg().matches("^\\[.{1,}]$")) {
category.setCategoryImg("");
}
//验证栏目管理名称的值是否合法
if (StringUtil.isBlank(category.getCategoryTitle())) {
return ResultData.build().error(getResStri
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
免费可商用的开源Java CMS内容管理系统/基于SpringBoot 2/前端Vue3/element plus/提供上百套模板,同时提供实用的插件/每两个月收集issues问题并更新版本/一套简单好用开源免费的Java CMS内容管理系/一整套优质的开源生态内容体系。我们的使命就是降低开发成本提高开发效率,提供全方位的企业级开发解决方案。
资源推荐
资源详情
资源评论
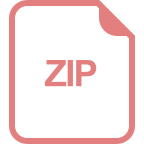
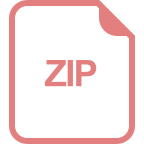
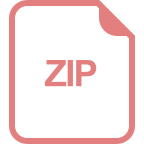
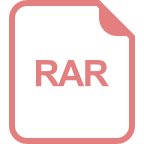
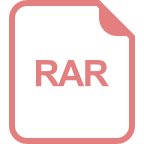
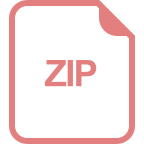
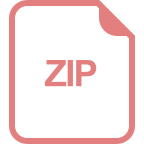
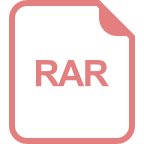
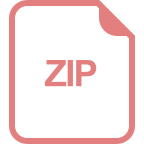
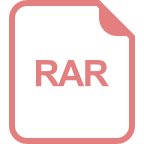
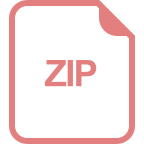
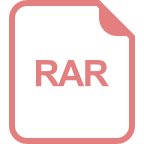
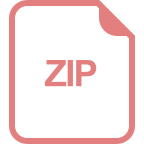
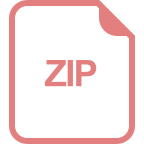
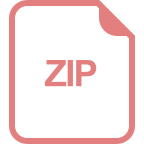
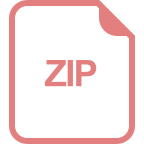
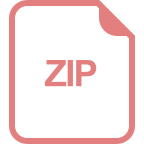
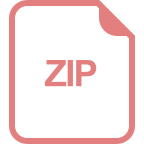
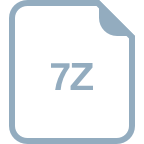
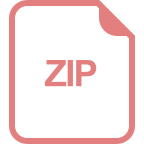
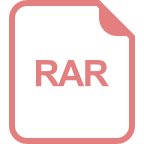
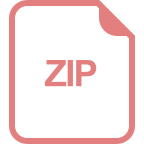
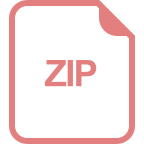
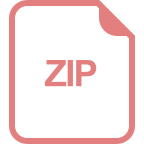
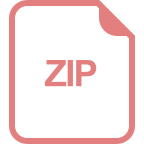
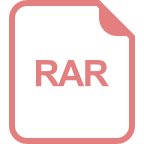
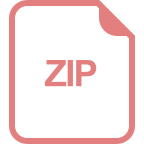
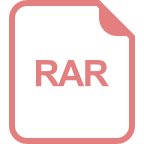
收起资源包目录

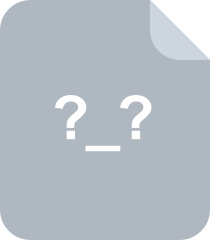
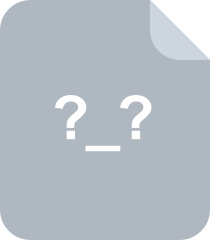
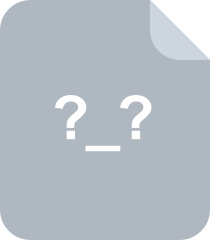
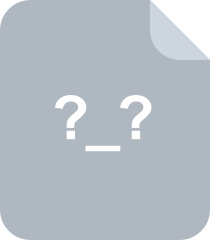
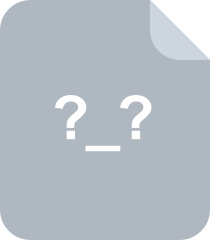
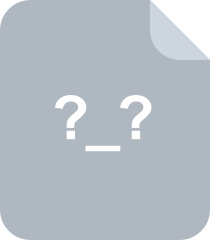
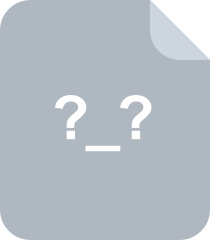
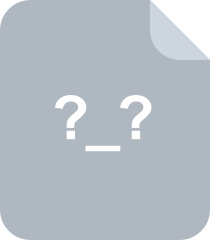
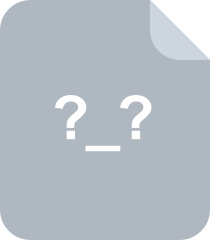
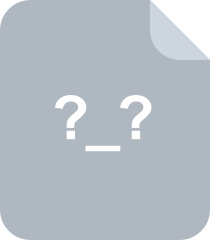
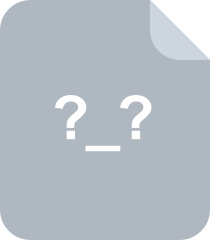
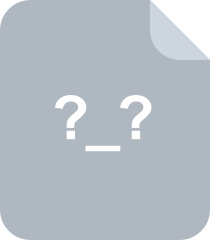
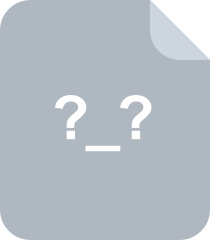
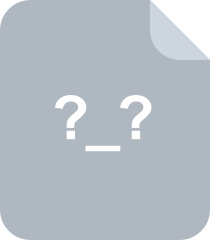
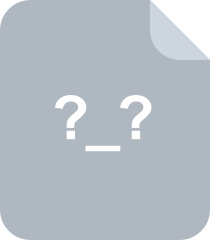
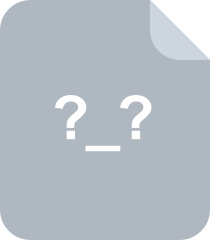
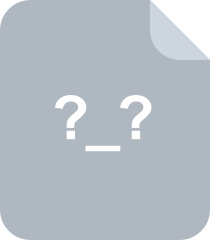
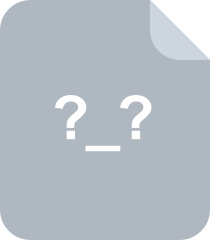
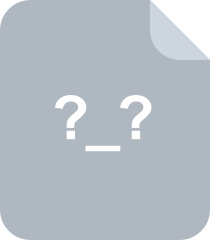
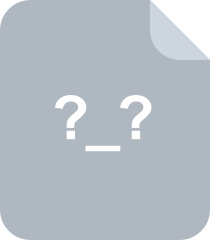
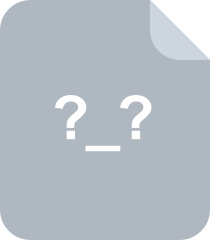
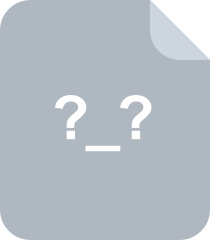
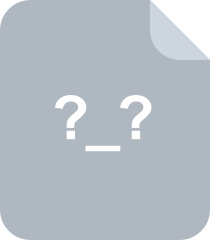
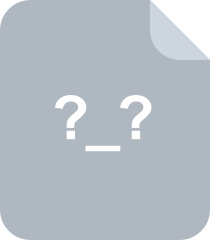
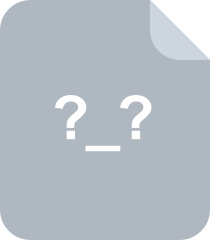
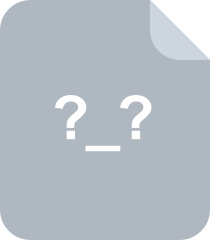
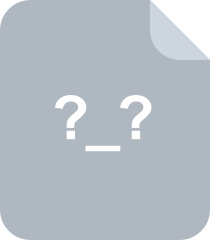
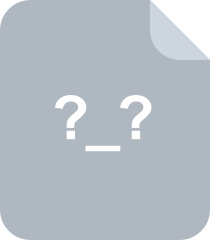
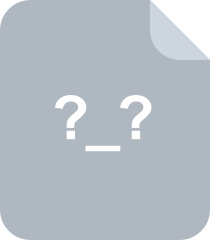
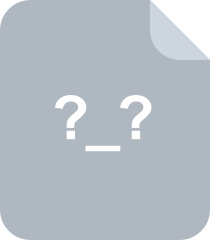
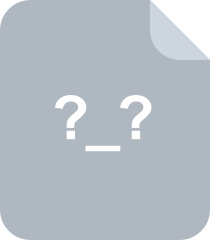
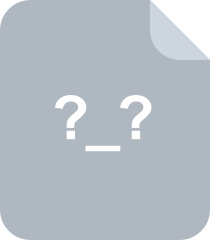
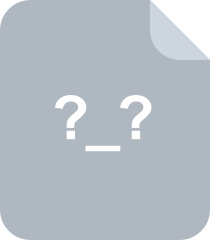
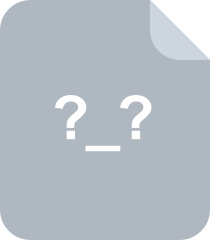
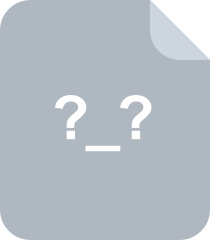
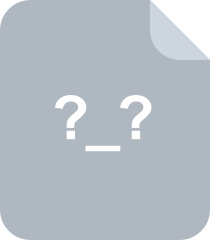
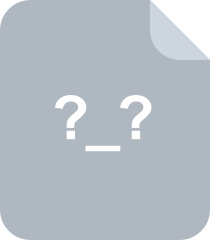
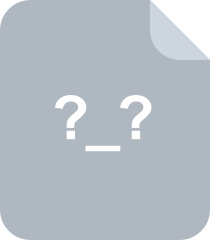
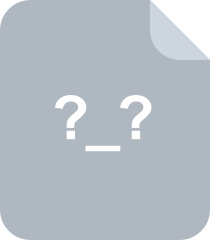
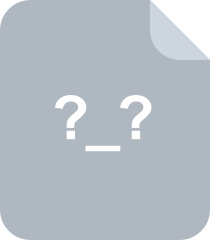
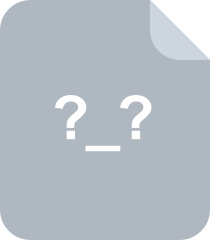
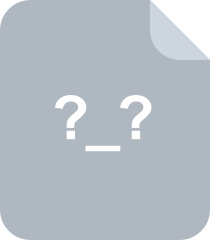
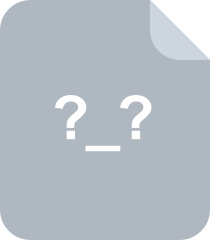
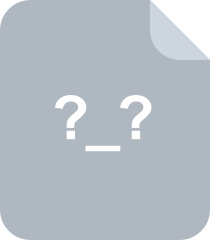
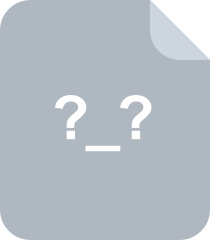
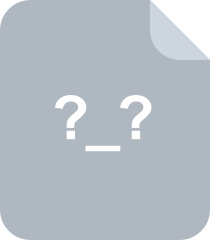
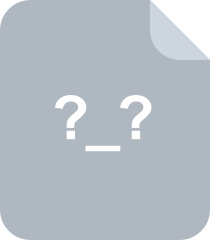
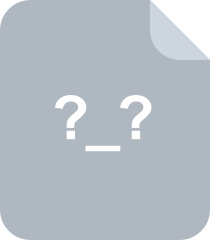
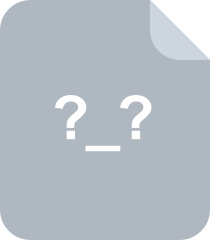
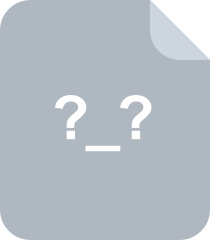
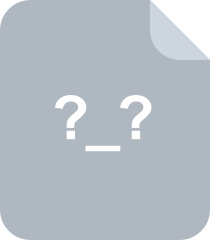
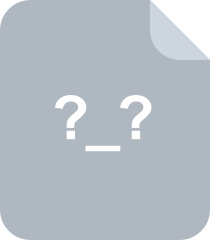
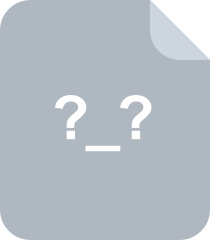
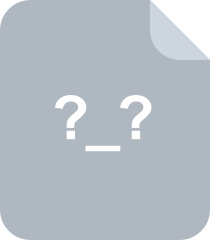
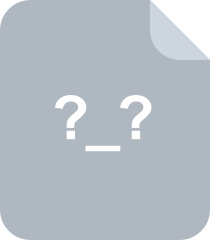
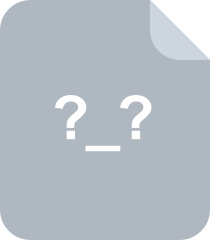
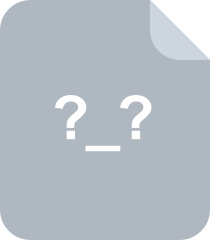
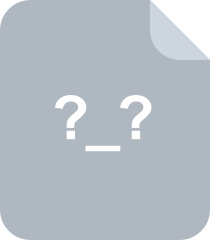
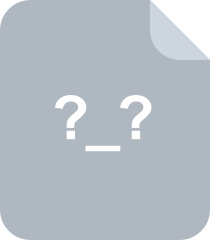
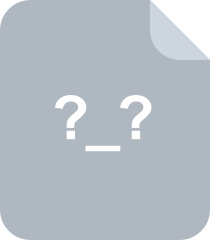
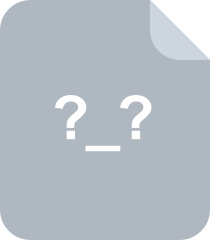
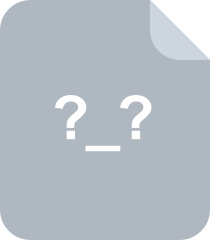
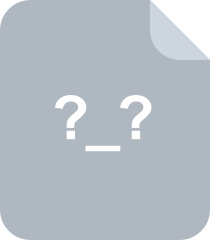
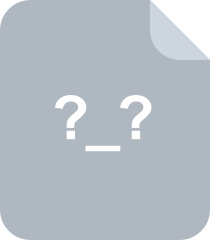
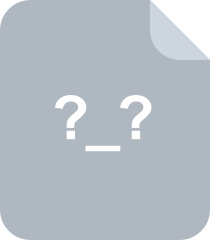
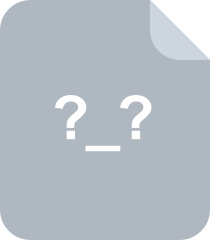
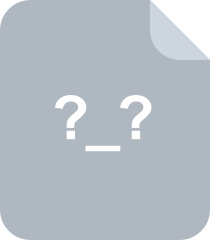
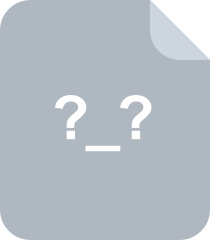
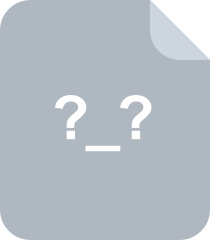
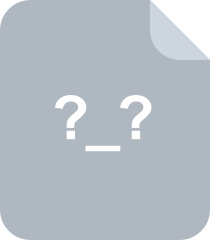
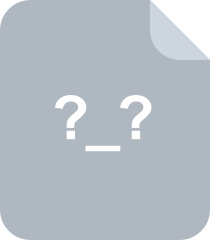
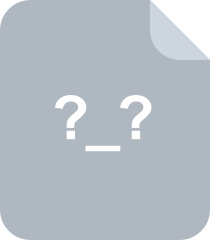
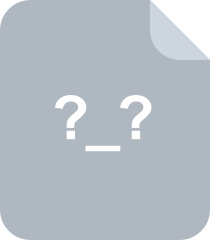
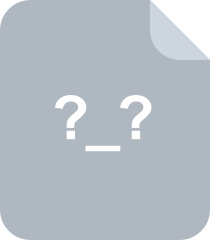
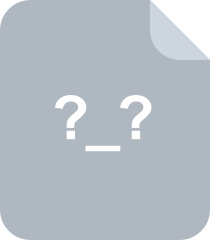
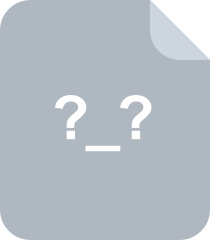
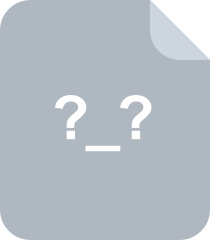
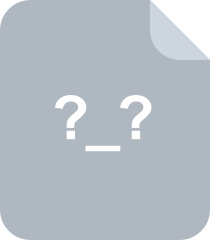
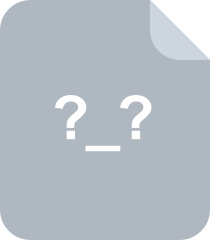
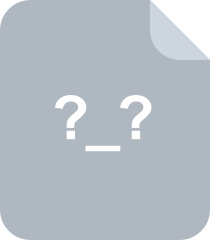
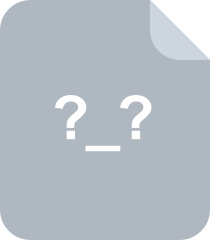
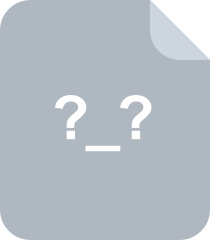
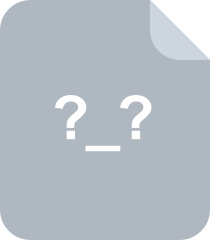
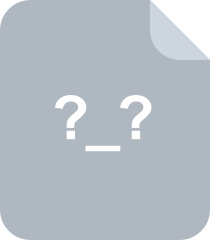
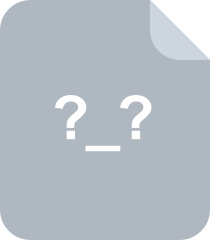
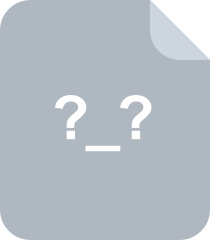
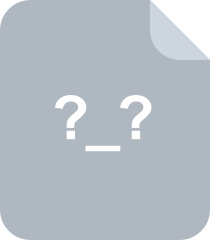
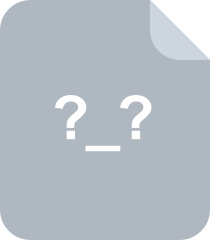
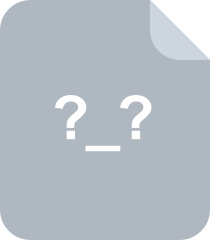
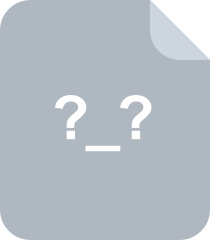
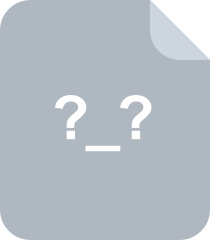
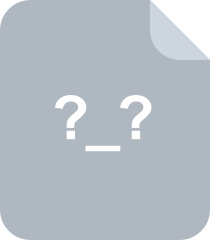
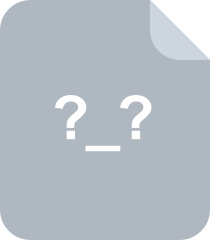
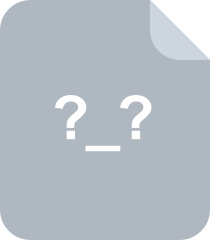
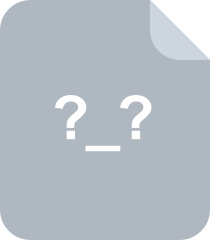
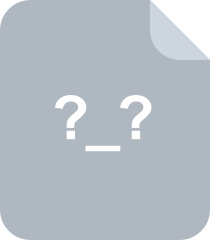
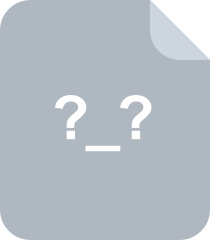
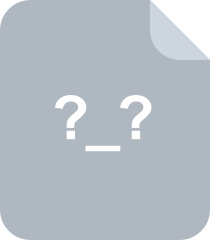
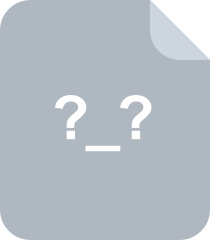
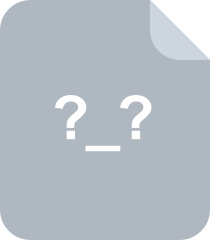
共 1252 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
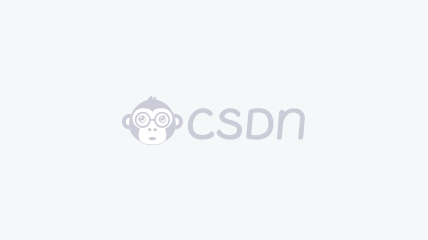

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7266

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

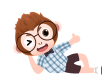
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


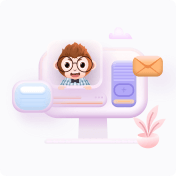
安全验证
文档复制为VIP权益,开通VIP直接复制
