/*
* All content copyright Terracotta, Inc., unless otherwise indicated. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*
*/
package com.vctgo.job.core.cron;
import java.io.Serializable;
import java.text.ParseException;
import java.util.Calendar;
import java.util.Date;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Locale;
import java.util.Map;
import java.util.SortedSet;
import java.util.StringTokenizer;
import java.util.TimeZone;
import java.util.TreeSet;
/**
* Provides a parser and evaluator for unix-like cron expressions. Cron
* expressions provide the ability to specify complex time combinations such as
* "At 8:00am every Monday through Friday" or "At 1:30am every
* last Friday of the month".
* <P>
* Cron expressions are comprised of 6 required fields and one optional field
* separated by white space. The fields respectively are described as follows:
*
* <table cellspacing="8">
* <tr>
* <th align="left">Field Name</th>
* <th align="left"> </th>
* <th align="left">Allowed Values</th>
* <th align="left"> </th>
* <th align="left">Allowed Special Characters</th>
* </tr>
* <tr>
* <td align="left"><code>Seconds</code></td>
* <td align="left"> </th>
* <td align="left"><code>0-59</code></td>
* <td align="left"> </th>
* <td align="left"><code>, - * /</code></td>
* </tr>
* <tr>
* <td align="left"><code>Minutes</code></td>
* <td align="left"> </th>
* <td align="left"><code>0-59</code></td>
* <td align="left"> </th>
* <td align="left"><code>, - * /</code></td>
* </tr>
* <tr>
* <td align="left"><code>Hours</code></td>
* <td align="left"> </th>
* <td align="left"><code>0-23</code></td>
* <td align="left"> </th>
* <td align="left"><code>, - * /</code></td>
* </tr>
* <tr>
* <td align="left"><code>Day-of-month</code></td>
* <td align="left"> </th>
* <td align="left"><code>1-31</code></td>
* <td align="left"> </th>
* <td align="left"><code>, - * ? / L W</code></td>
* </tr>
* <tr>
* <td align="left"><code>Month</code></td>
* <td align="left"> </th>
* <td align="left"><code>0-11 or JAN-DEC</code></td>
* <td align="left"> </th>
* <td align="left"><code>, - * /</code></td>
* </tr>
* <tr>
* <td align="left"><code>Day-of-Week</code></td>
* <td align="left"> </th>
* <td align="left"><code>1-7 or SUN-SAT</code></td>
* <td align="left"> </th>
* <td align="left"><code>, - * ? / L #</code></td>
* </tr>
* <tr>
* <td align="left"><code>Year (Optional)</code></td>
* <td align="left"> </th>
* <td align="left"><code>empty, 1970-2199</code></td>
* <td align="left"> </th>
* <td align="left"><code>, - * /</code></td>
* </tr>
* </table>
* <P>
* The '*' character is used to specify all values. For example, "*"
* in the minute field means "every minute".
* <P>
* The '?' character is allowed for the day-of-month and day-of-week fields. It
* is used to specify 'no specific value'. This is useful when you need to
* specify something in one of the two fields, but not the other.
* <P>
* The '-' character is used to specify ranges For example "10-12" in
* the hour field means "the hours 10, 11 and 12".
* <P>
* The ',' character is used to specify additional values. For example
* "MON,WED,FRI" in the day-of-week field means "the days Monday,
* Wednesday, and Friday".
* <P>
* The '/' character is used to specify increments. For example "0/15"
* in the seconds field means "the seconds 0, 15, 30, and 45". And
* "5/15" in the seconds field means "the seconds 5, 20, 35, and
* 50". Specifying '*' before the '/' is equivalent to specifying 0 is
* the value to start with. Essentially, for each field in the expression, there
* is a set of numbers that can be turned on or off. For seconds and minutes,
* the numbers range from 0 to 59. For hours 0 to 23, for days of the month 0 to
* 31, and for months 0 to 11 (JAN to DEC). The "/" character simply helps you turn
* on every "nth" value in the given set. Thus "7/6" in the
* month field only turns on month "7", it does NOT mean every 6th
* month, please note that subtlety.
* <P>
* The 'L' character is allowed for the day-of-month and day-of-week fields.
* This character is short-hand for "last", but it has different
* meaning in each of the two fields. For example, the value "L" in
* the day-of-month field means "the last day of the month" - day 31
* for January, day 28 for February on non-leap years. If used in the
* day-of-week field by itself, it simply means "7" or
* "SAT". But if used in the day-of-week field after another value, it
* means "the last xxx day of the month" - for example "6L"
* means "the last friday of the month". You can also specify an offset
* from the last day of the month, such as "L-3" which would mean the third-to-last
* day of the calendar month. <i>When using the 'L' option, it is important not to
* specify lists, or ranges of values, as you'll get confusing/unexpected results.</i>
* <P>
* The 'W' character is allowed for the day-of-month field. This character
* is used to specify the weekday (Monday-Friday) nearest the given day. As an
* example, if you were to specify "15W" as the value for the
* day-of-month field, the meaning is: "the nearest weekday to the 15th of
* the month". So if the 15th is a Saturday, the trigger will fire on
* Friday the 14th. If the 15th is a Sunday, the trigger will fire on Monday the
* 16th. If the 15th is a Tuesday, then it will fire on Tuesday the 15th.
* However if you specify "1W" as the value for day-of-month, and the
* 1st is a Saturday, the trigger will fire on Monday the 3rd, as it will not
* 'jump' over the boundary of a month's days. The 'W' character can only be
* specified when the day-of-month is a single day, not a range or list of days.
* <P>
* The 'L' and 'W' characters can also be combined for the day-of-month
* expression to yield 'LW', which translates to "last weekday of the
* month".
* <P>
* The '#' character is allowed for the day-of-week field. This character is
* used to specify "the nth" XXX day of the month. For example, the
* value of "6#3" in the day-of-week field means the third Friday of
* the month (day 6 = Friday and "#3" = the 3rd one in the month).
* Other examples: "2#1" = the first Monday of the month and
* "4#5" = the fifth Wednesday of the month. Note that if you specify
* "#5" and there is not 5 of the given day-of-week in the month, then
* no firing will occur that month. If the '#' character is used, there can
* only be one expression in the day-of-week field ("3#1,6#3" is
* not valid, since there are two expressions).
* <P>
* <!--The 'C' character is allowed for the day-of-month and day-of-week fields.
* This character is short-hand for "calendar". This means values are
* calculated against the associated calendar, if any. If no calendar is
* associated, then it is equivalent to having an all-inclusive calendar. A
* value of "5C" in the day-of-month field means "the first day included by the
* calendar on or after the 5th". A value of "1C" i
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于Ruoyi-Cloud版本改造的的多租户SaaS开发框架。 首先感谢若依提供的开源支持!请大家继续关注若依项目! 本项目基于 RuoYi-Cloud 进行二次开发的租户版本 目标在于精简脚手架,致力于中小企业的快速开发项目搭建,让大家不必再耗费精力于各种开源框架里集成的复杂功能如何抉择保留
资源推荐
资源详情
资源评论
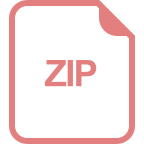
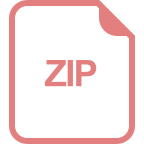
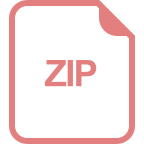
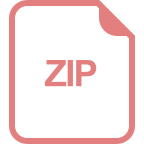
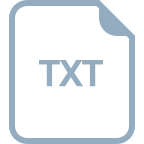
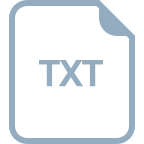
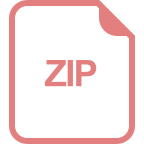
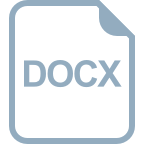
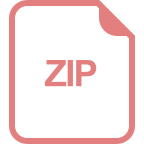
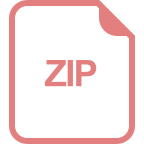
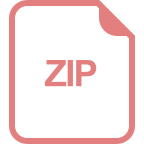
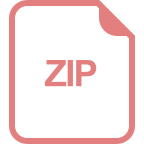
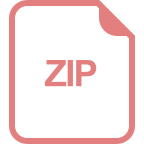
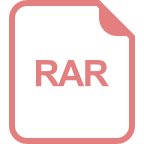
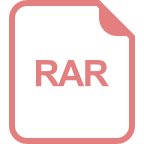
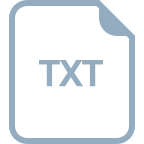
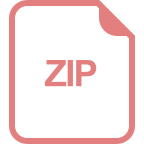
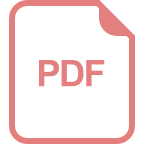
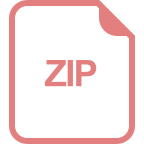
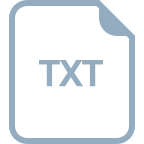
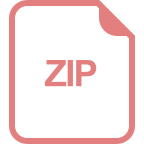
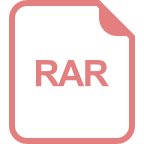
收起资源包目录

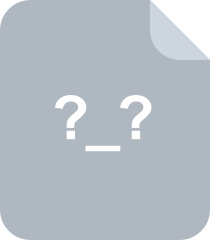
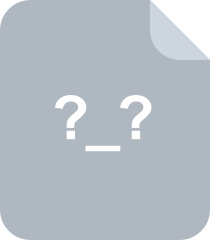
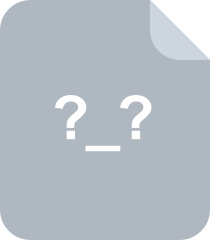
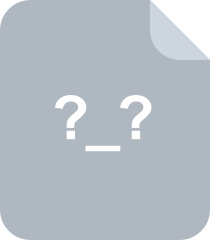
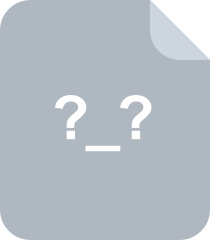
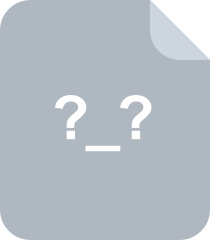
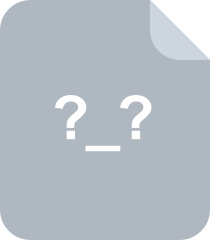
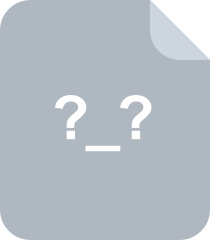
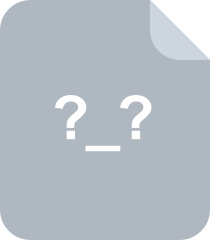
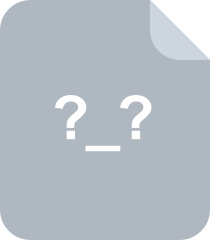
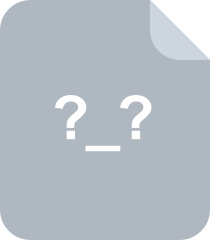
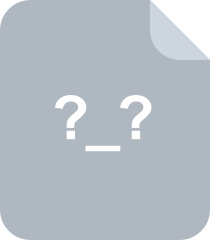
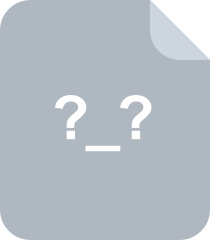
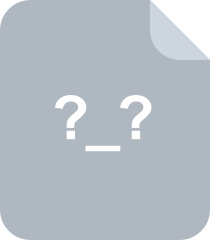
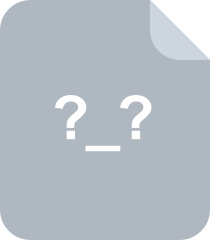
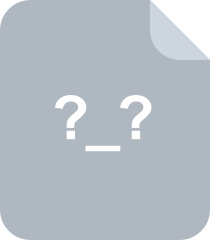
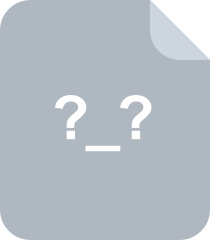
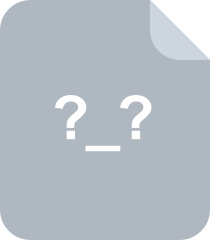
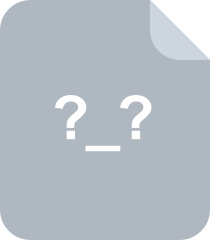
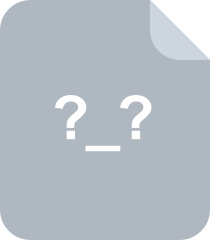
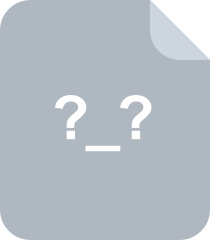
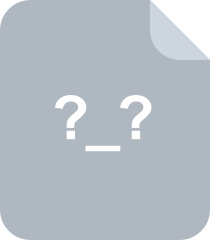
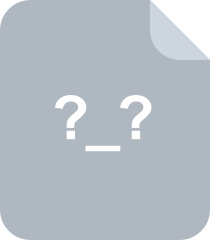
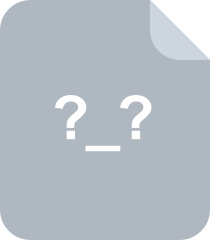
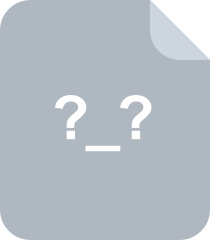
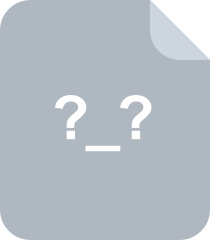
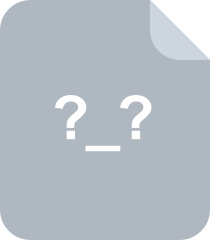
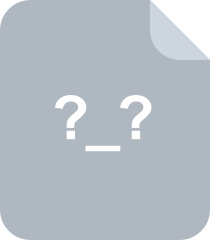
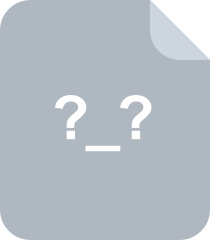
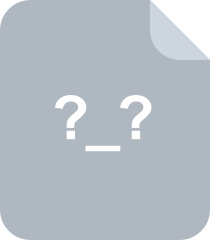
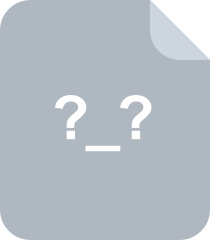
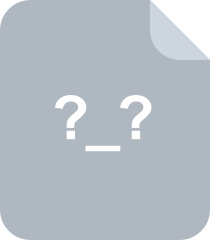
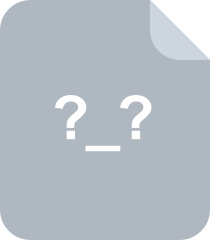
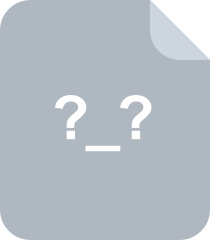
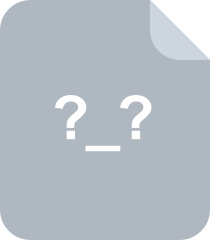
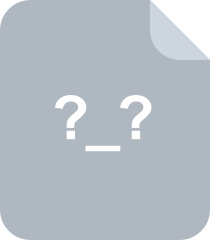
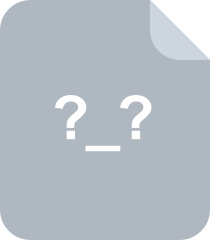
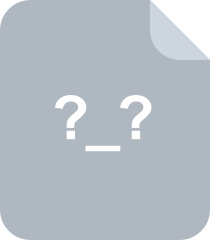
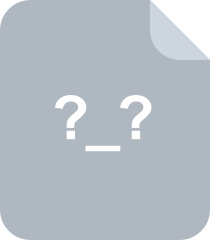
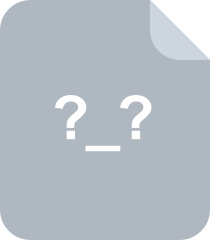
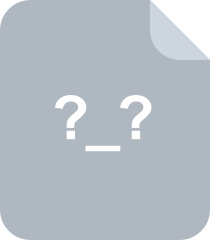
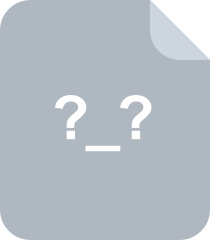
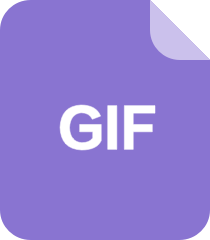
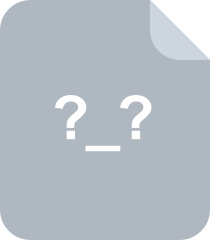
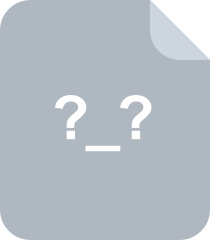
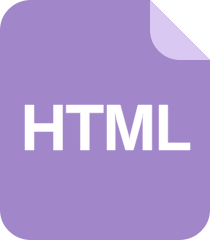
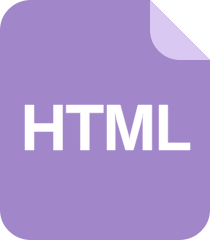
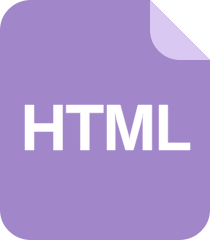
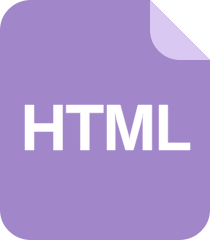
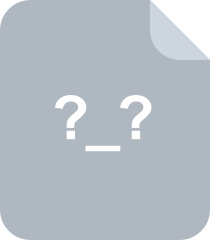
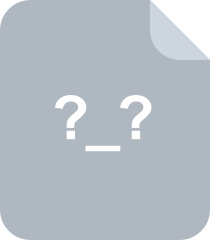
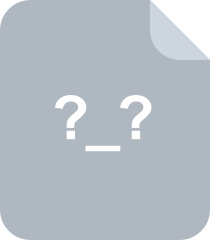
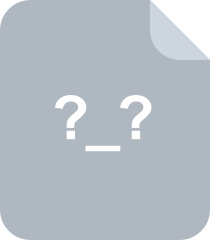
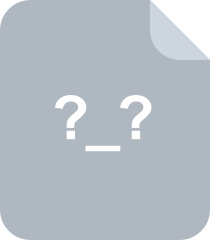
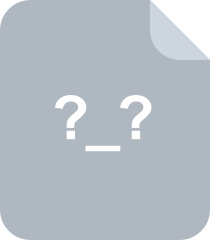
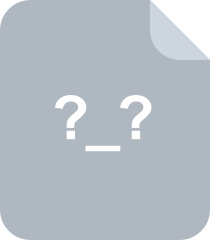
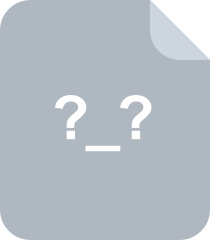
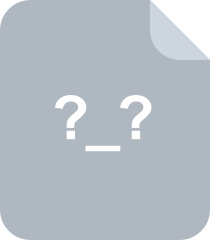
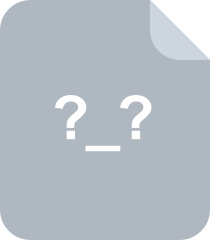
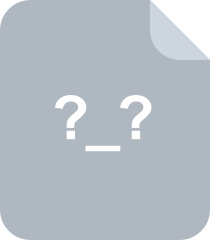
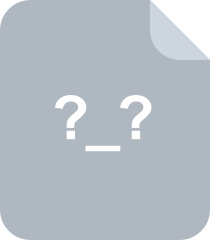
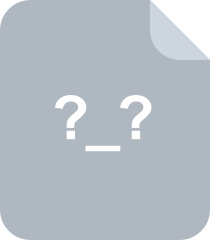
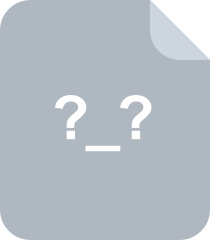
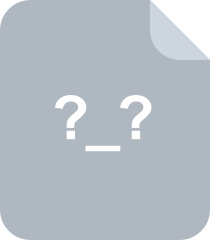
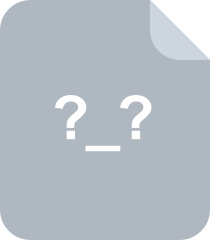
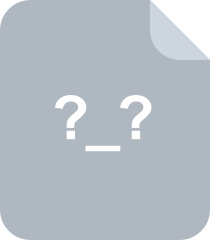
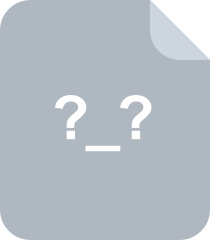
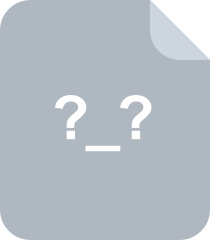
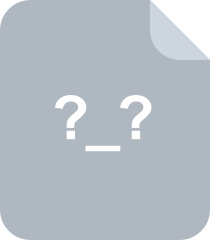
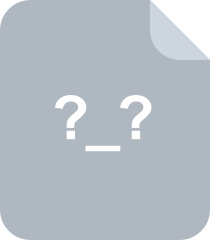
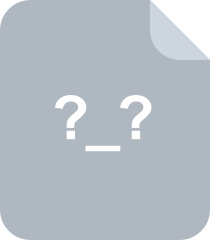
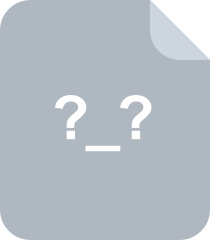
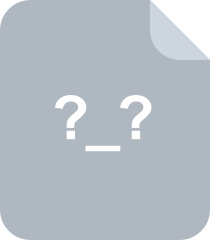
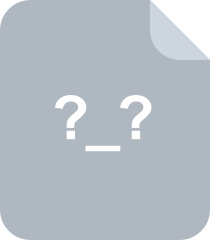
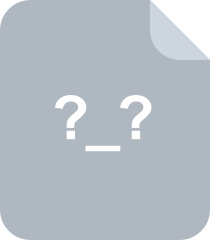
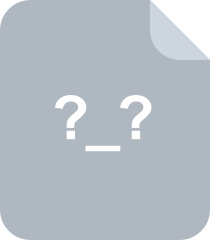
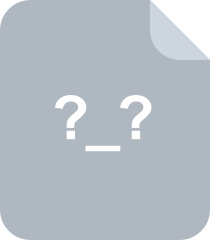
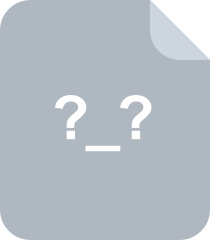
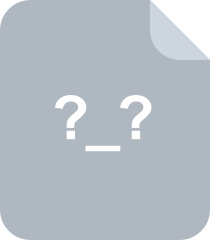
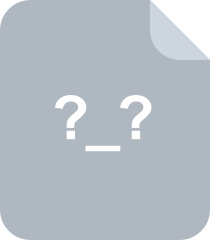
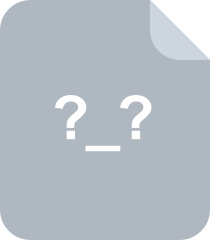
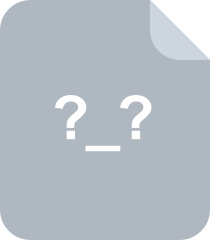
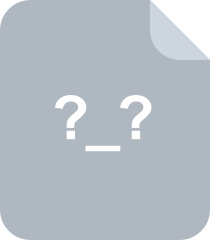
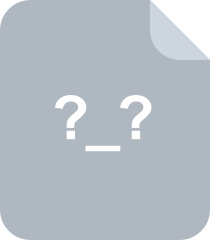
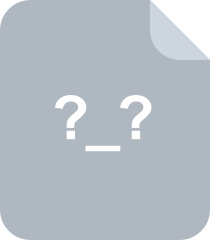
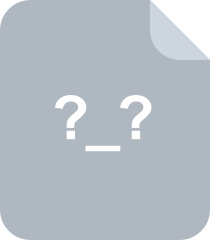
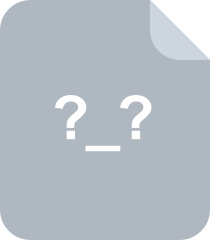
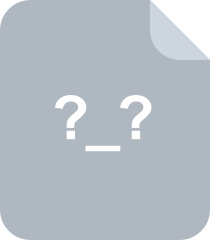
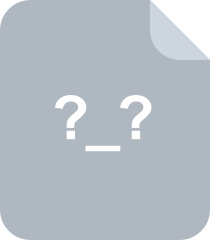
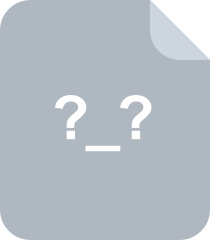
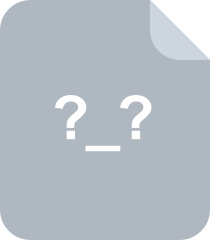
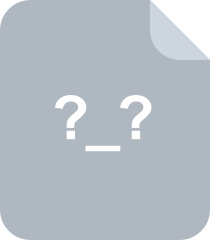
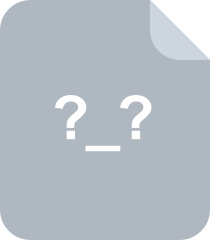
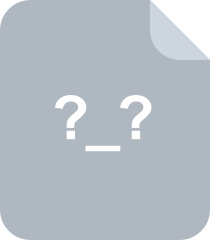
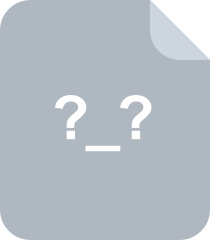
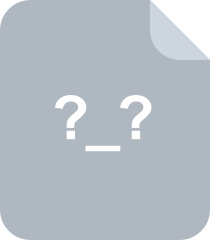
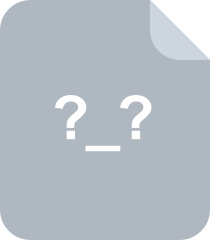
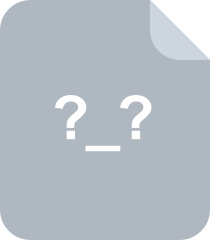
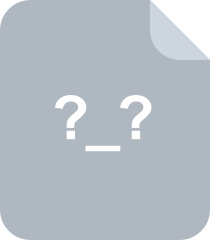
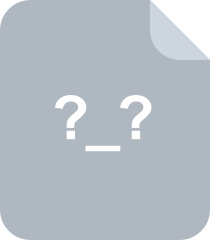
共 952 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
资源评论
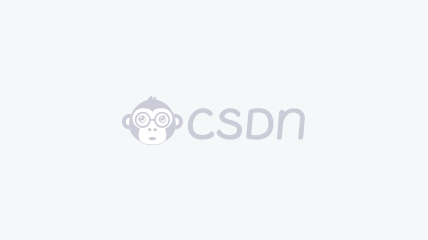
- 笔耕不错,代码不止2024-11-06资源和描述一致,质量不错,解决了我的问题,感谢资源主。
- kefuxiaohui22024-08-01资源有一定的参考价值,与资源描述一致,很实用,能够借鉴的部分挺多的,值得下载。

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7366
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

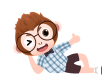
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


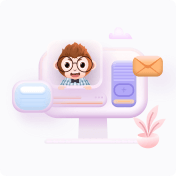
安全验证
文档复制为VIP权益,开通VIP直接复制
