【ESP32环境监测系统详解】 ESP32是一款高性能、低成本、低功耗的微控制器,集成Wi-Fi和蓝牙双模无线通信功能,是物联网(IoT)应用的理想选择。在"基于esp32开发的环境监测系统"项目中,开发者充分利用了ESP32的这些特性,构建了一个能够实时监测环境温湿度的智能系统。 该系统的核心部分是ESP32微控制器,它内置有丰富的传感器接口,可以方便地连接各种环境传感器,如DHT11或DHT22等温湿度传感器。这些传感器能够实时采集环境中的温度和湿度信息,并将数据传输给ESP32。 ESP32的高性能处理器能够快速处理这些数据,并通过其内置的Wi-Fi模块将数据上传至云端服务器。 服务器端一般采用RESTful API架构,接收并存储来自ESP32设备的数据。为了保证数据的安全性和可靠性,通常会使用HTTPS协议进行数据传输,并实现身份验证机制,如OAuth 2.0或API密钥。此外,服务器可能会使用数据库(如MySQL或MongoDB)来持久化存储这些环境数据,便于后期分析和查询。 在前端,Android应用程序扮演着用户界面的角色。通过订阅服务器的发布消息,Android应用可以实时获取最新的环境数据。通常,这会涉及到WebSocket或Long Polling等实时通信技术。用户界面设计应当直观易用,展示当前的温湿度数据,并可能包含历史数据图表,以便用户了解环境变化趋势。 在实现这一系统时,开发者可能需要掌握以下关键技术: 1. ESP32编程:使用MicroPython或C/C++,利用Arduino或PlatformIO等框架进行开发。 2. Wi-Fi通信:理解TCP/IP协议栈,设置Wi-Fi连接,并实现HTTP/HTTPS请求。 3. 传感器交互:熟悉DHT系列或其他温湿度传感器的接口和通信协议。 4. 云服务:搭建和配置云服务器,部署RESTful API。 5. 安全性:了解HTTPS加密原理,实施身份验证和授权策略。 6. Android开发:使用Java或Kotlin编写Android应用,实现WebSocket或Long Polling通信,设计用户界面。 7. 数据可视化:利用Android SDK中的图表库,如MPAndroidChart,将环境数据以图形形式展示。 总结来说,"基于esp32开发的环境监测系统"是一个典型的物联网应用实例,涵盖了硬件接口、无线通信、云服务、移动应用开发等多个技术领域,对于学习和实践物联网技术具有很高的参考价值。通过这样的系统,我们可以实时监控环境条件,为智能家居、农业监测、仓储管理等领域提供有价值的数据支持。
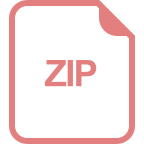
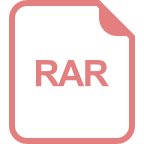
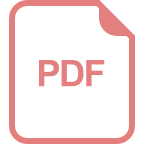
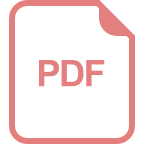
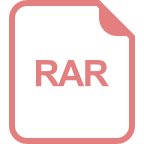
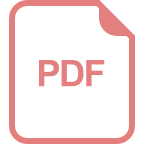
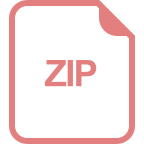
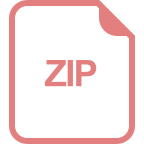
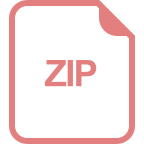
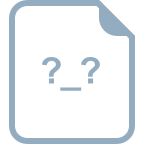
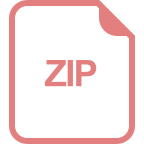
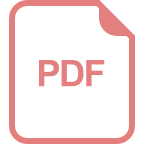
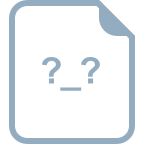
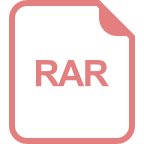
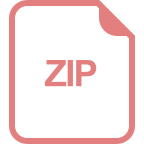
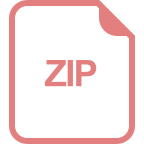
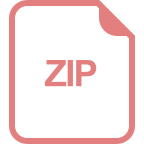
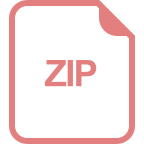
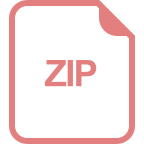
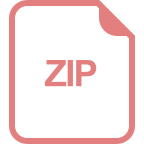
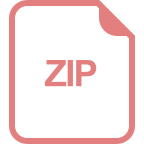
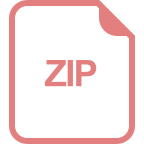
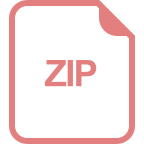
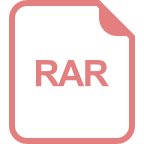
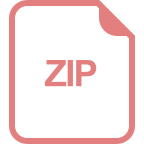


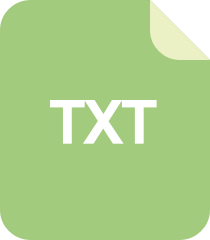
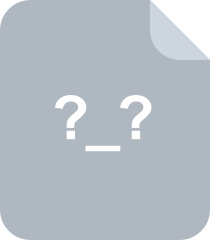
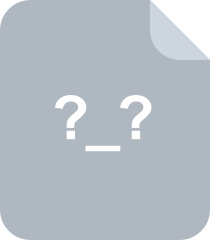
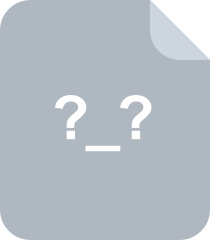
- 1
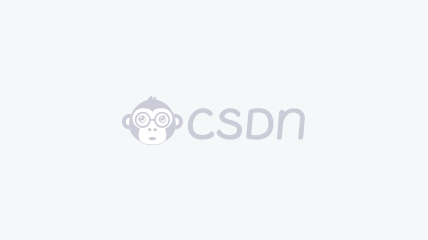

- 粉丝: 1w+
- 资源: 7530
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

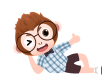
最新资源
- 绿色便携版Python打包版
- Ngrok内网穿透启动隧道工具
- 异步电机matlab仿真模型
- BMS电池管理控制器,开发板资料 电池管理系统策略开发,应用层软件,开发流程开发
- 基于Transformer的各种变体已经是时间序列以及多元时间序列的一大热点,自注意力机制以及多头自注意力机制本团队已经可以基于matlab平台实现
- 基于自适应在线学习的概率负荷预测(代码)
- 出基于滑模的永磁直线同步电机的鲁棒H无穷控制Matlab程序,对于学习SMC和H无穷的结合可以很好参考
- COMSOL 孔隙尺度渗流模拟,单相及多相渗流模拟,案例复现,水平集(LS)和相场(PF)实现两相流模拟,水驱油,水驱气,二氧化碳驱油等模拟
- 可三相LCL型并网逆变器仿真模型,LCL滤波器,电容电流反馈有源阻尼方法 只采用网侧电流环控制方法时,由于系统的固有谐振峰的存在,以及数字控制延时的影响,通常延时时间 Td=1.5Ts(Ts
- 联电SOA软件平台:基于MATLAB/Simulink加速汽车软件开发生态建设与实现
- 柴油发电机仿真 Matlab Simulink 柴油发电机matlab仿真 微电网仿真 柴油发电仿真 风光柴储微电网 光伏发电 柴油发电 风力发电 储能电池 光柴储微电网 风柴储微电网 风机光伏柴油
- IEEE Access模板
- V2G 充电桩,新能源汽车车载充电机, MATLAB仿真模型 ,PFC+CLLC拓扑; 1. V2G,AC DC,DC DC双向充放电; 2. 前级,双向AC DC单相整流器(PWM),输入AC
- ARM发布Armv9.5架构:迈向更强性能与灵活性的新时代
- 汽车行业面向服务架构(SOA)下传统应用向软件定义汽车(SDV)的迁移方案及挑战
- 西门子1200恒压供水程序+PID+触摸屏 此程序样 例为 一拖三恒压供水程序样,S7-1200PLC和KTP1000PN触摸屏 人机执行PID控制变频器实现恒压供水. 供学习参考,用15

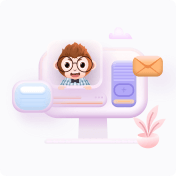
