package com.pig4cloud.pig.common.core.util;
import cn.hutool.core.convert.Convert;
import lombok.experimental.UtilityClass;
import org.springframework.data.redis.connection.RedisConnection;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.*;
import org.springframework.data.redis.core.script.DefaultRedisScript;
import org.springframework.data.redis.core.script.RedisScript;
import java.util.*;
import java.util.concurrent.TimeUnit;
/**
* 缓存工具类
*
* @author XX
* @date 2023/05/12
*/
@UtilityClass
public class RedisUtils {
private static final Long SUCCESS = 1L;
/**
* 指定缓存失效时间
* @param key 键
* @param time 时间(秒)
*/
public boolean expire(String key, long time) {
RedisTemplate<Object, Object> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
Optional.ofNullable(redisTemplate)
.filter(template -> time > 0)
.ifPresent(template -> template.expire(key, time, TimeUnit.SECONDS));
return true;
}
/**
* 根据 key 获取过期时间
* @param key 键 不能为null
* @return 时间(秒) 返回0代表为永久有效
*/
public long getExpire(Object key) {
RedisTemplate<Object, Object> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
return Optional.ofNullable(redisTemplate)
.map(template -> template.getExpire(key, TimeUnit.SECONDS))
.orElse(-1L);
}
/**
* 查找匹配key
* @param pattern key
* @return /
*/
public List<String> scan(String pattern) {
RedisTemplate<Object, Object> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
ScanOptions options = ScanOptions.scanOptions().match(pattern).build();
return Optional.ofNullable(redisTemplate).map(template -> {
RedisConnectionFactory factory = template.getConnectionFactory();
RedisConnection rc = Objects.requireNonNull(factory).getConnection();
Cursor<byte[]> cursor = rc.scan(options);
List<String> result = new ArrayList<>();
while (cursor.hasNext()) {
result.add(new String(cursor.next()));
}
RedisConnectionUtils.releaseConnection(rc, factory);
return result;
}).orElse(Collections.emptyList());
}
/**
* 分页查询 key
* @param patternKey key
* @param page 页码
* @param size 每页数目
* @return /
*/
public List<String> findKeysForPage(String patternKey, int page, int size) {
RedisTemplate redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
ScanOptions options = ScanOptions.scanOptions().match(patternKey).build();
RedisConnectionFactory factory = redisTemplate.getConnectionFactory();
RedisConnection rc = Objects.requireNonNull(factory).getConnection();
Cursor<byte[]> cursor = rc.scan(options);
List<String> result = new ArrayList<>(size);
int tmpIndex = 0;
int fromIndex = page * size;
int toIndex = page * size + size;
while (cursor.hasNext()) {
if (tmpIndex >= fromIndex && tmpIndex < toIndex) {
result.add(new String(cursor.next()));
tmpIndex++;
continue;
}
// 获取到满足条件的数据后,就可以退出了
if (tmpIndex >= toIndex) {
break;
}
tmpIndex++;
cursor.next();
}
RedisConnectionUtils.releaseConnection(rc, factory);
return result;
}
/**
* 判断key是否存在
* @param key 键
* @return true 存在 false不存在
*/
public boolean hasKey(String key) {
RedisTemplate<Object, Object> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
return Optional.ofNullable(redisTemplate).map(template -> template.hasKey(key)).orElse(false);
}
/**
* 删除缓存
* @param keys 可以传一个值 或多个
*/
public void del(String... keys) {
RedisTemplate<Object, Object> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
Optional.ofNullable(keys)
.map(Arrays::asList)
.filter(keysList -> !keysList.isEmpty())
.ifPresent(redisTemplate::delete);
}
/**
* 获取锁
* @param lockKey 锁key
* @param value value
* @param expireTime:单位-秒
* @return boolean
*/
public boolean getLock(String lockKey, String value, int expireTime) {
RedisTemplate<Object, Object> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
return Optional.ofNullable(redisTemplate)
.map(template -> template.opsForValue().setIfAbsent(lockKey, value, expireTime, TimeUnit.SECONDS))
.orElse(false);
}
/**
* 释放锁
* @param lockKey 锁key
* @param value value
* @return boolean
*/
public boolean releaseLock(String lockKey, String value) {
RedisTemplate<Object, Object> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
String script = "if redis.call('get', KEYS[1]) == ARGV[1] then return redis.call('del', KEYS[1]) else return 0 end";
RedisScript<Long> redisScript = new DefaultRedisScript<>(script, Long.class);
return Optional.ofNullable(redisTemplate.execute(redisScript, Collections.singletonList(lockKey), value))
.map(Convert::toLong)
.filter(SUCCESS::equals)
.isPresent();
}
// ============================String=============================
/**
* 普通缓存获取
* @param key 键
* @return 值
*/
public <T> T get(String key) {
RedisTemplate<String, T> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
return redisTemplate.opsForValue().get(key);
}
/**
* 批量获取
* @param keys
* @return
*/
public <T> List<T> multiGet(List<String> keys) {
RedisTemplate<String, T> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
return redisTemplate.opsForValue().multiGet(keys);
}
/**
* 普通缓存放入
* @param key 键
* @param value 值
* @return true成功 false失败
*/
public boolean set(String key, Object value) {
RedisTemplate<Object, Object> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
Optional.ofNullable(redisTemplate).map(template -> {
template.opsForValue().set(key, value);
return true;
});
return true;
}
/**
* 普通缓存放入并设置时间
* @param key 键
* @param value 值
* @param time 时间(秒) time要大于0 如果time小于等于0 将设置无限期
* @return true成功 false 失败
*/
public boolean set(String key, Object value, long time) {
RedisTemplate<Object, Object> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
return Optional.ofNullable(redisTemplate).map(template -> {
if (time > 0) {
template.opsForValue().set(key, value, time, TimeUnit.SECONDS);
}
else {
template.opsForValue().set(key, value);
}
return true;
}).orElse(false);
}
/**
* 普通缓存放入并设置时间
* @param key 键
* @param value 值
* @param time 时间
* @param timeUnit 类型
* @return true成功 false 失败
*/
public <T> boolean set(String key, T value, long time, TimeUnit timeUnit) {
RedisTemplate<String, T> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
Optional.ofNullable(redisTemplate).map(template -> {
if (time > 0) {
template.opsForValue().set(key, value, time, timeUnit);
}
else {
template.opsForValue().set(key, value);
}
return true;
});
return true;
}
// ================================Map=================================
/**
* HashGet
* @param key 键 不能为null
* @param hashKey 项 不能为null
* @return 值
*/
public <HK, HV> HV hget(String key, HK hashKey) {
RedisTemplate<String, HV> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
return redisTemplate.<HK, HV>opsForHash().get(key, hashKey);
}
/**
* 获取hashKey对应的所有键值
* @param key 键
* @return 对应的多个键值
*/
public <HK, HV> Map<HK, HV> hmget(String key) {
RedisTemplate<String, HV> redisTemplate = SpringContextHolder.getBean(RedisTemplate.class);
return redisTemplate.<HK, HV>opsForHash().entries(key);
}
/**
* HashSet
* @param key 键
* @param map 对应多个键值
* @return true 成功 f
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于Spring Boot 3.2、 Spring Cloud 2023 & Alibaba、 SAS OAuth2 的微服务RBAC 权限管理系统。基于数据驱动视图的理念封装 element-plus,即使没有 vue 的使用经验也能快速上手。提供对常见容器化支持 Docker、Kubernetes、Rancher2 支持。提供 lambda 、stream api 、webflux 的生产实践
资源推荐
资源详情
资源评论
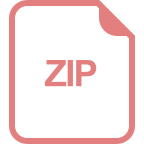
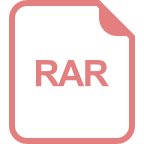
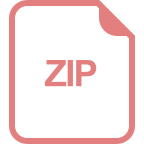
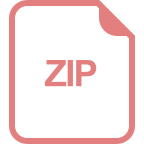
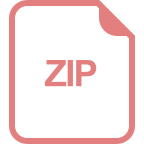
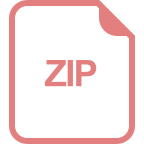
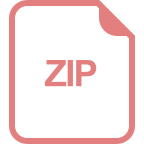
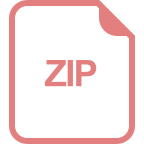
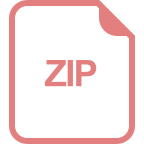
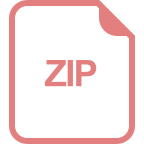
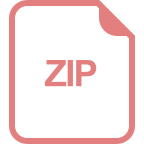
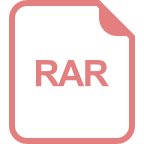
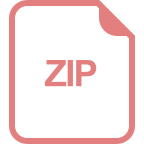
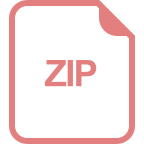
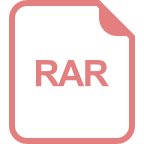
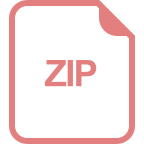
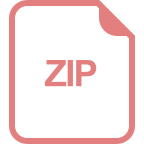
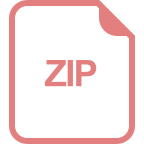
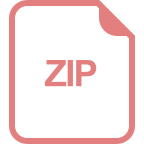
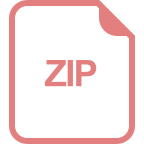
收起资源包目录

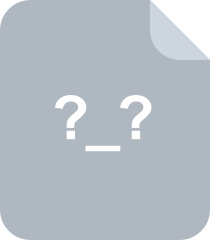
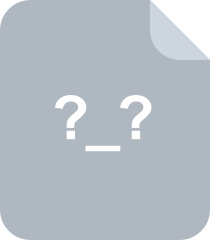
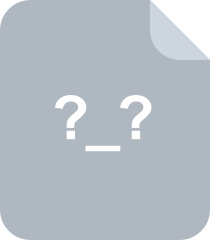
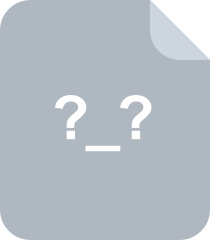
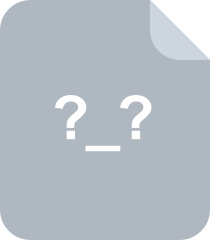
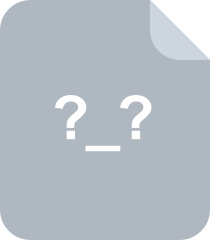
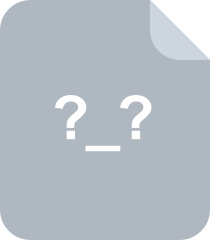
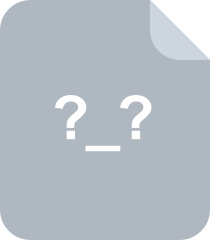
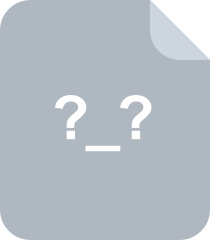
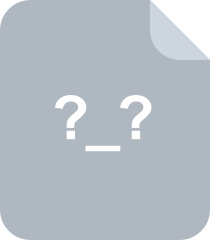
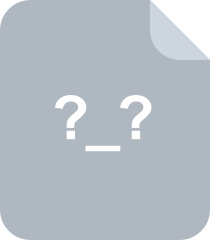
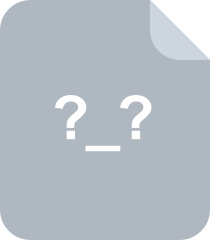
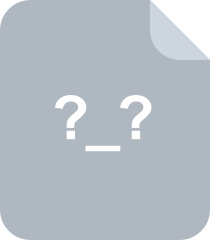
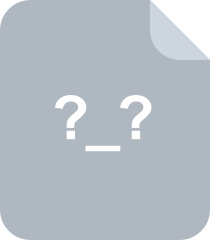
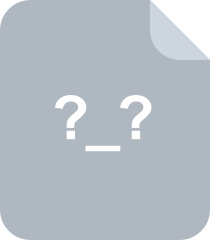
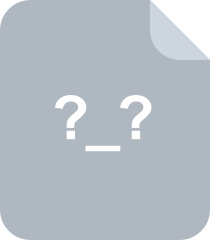
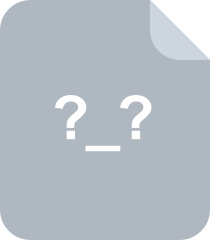
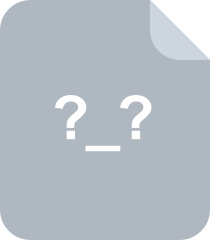
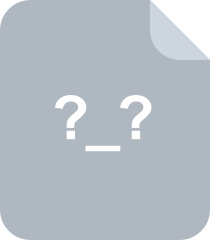
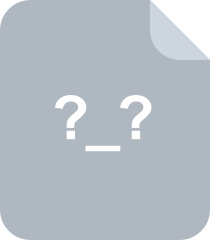
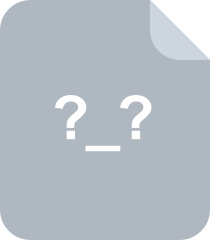
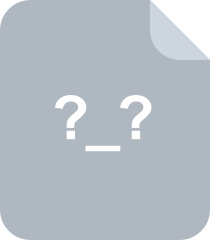
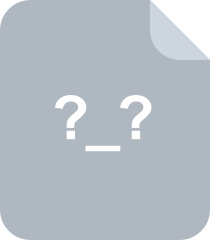
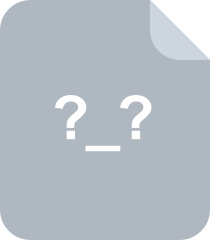
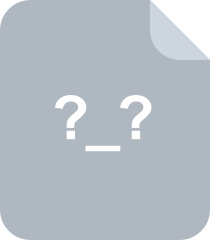
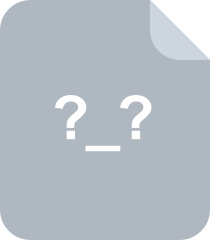
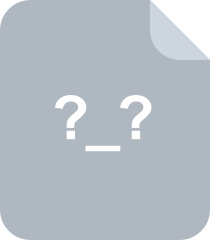
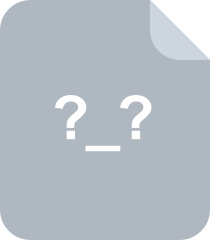
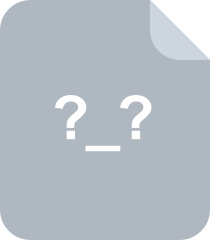
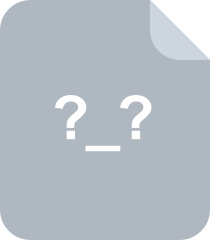
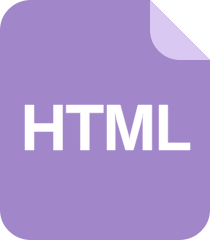
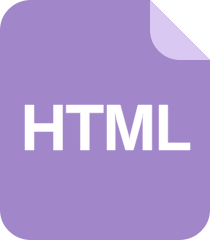
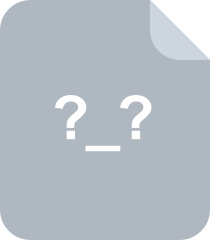
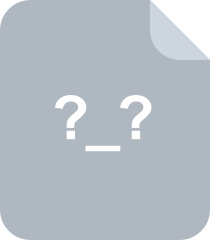
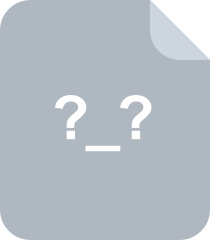
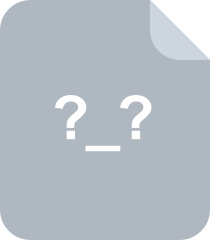
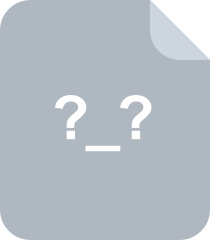
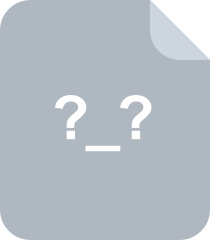
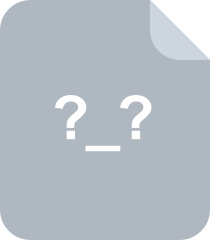
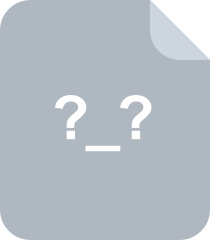
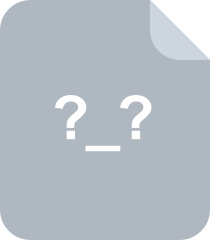
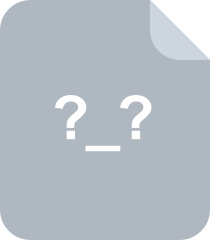
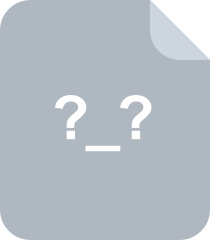
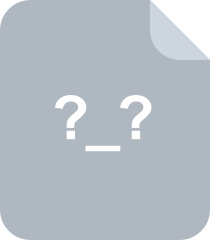
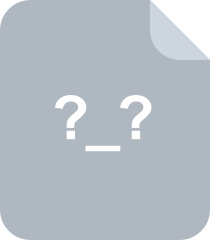
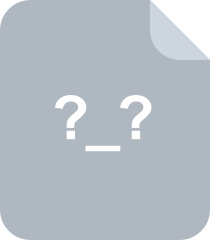
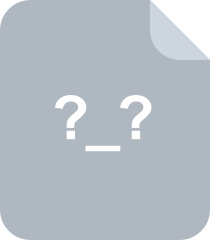
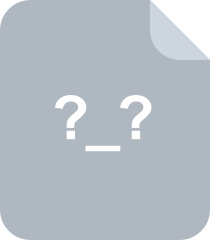
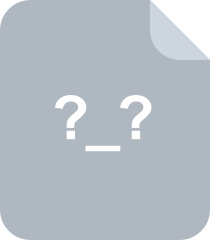
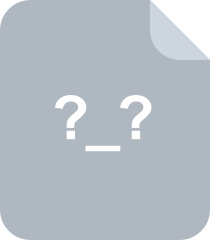
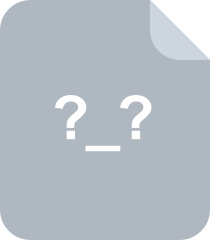
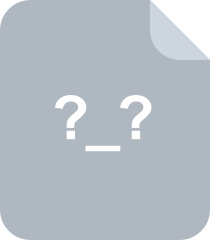
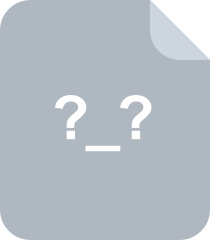
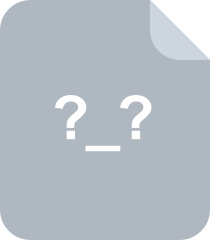
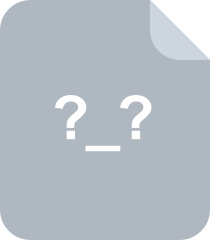
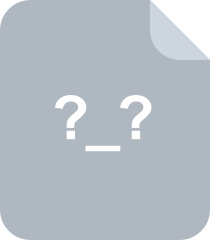
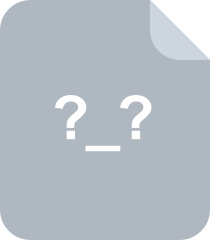
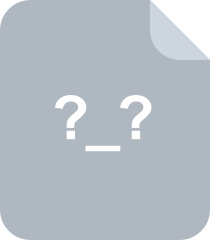
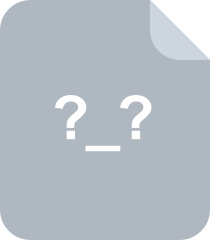
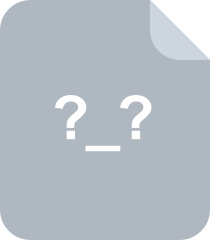
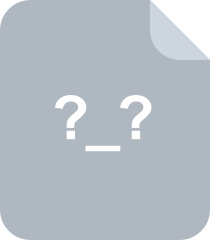
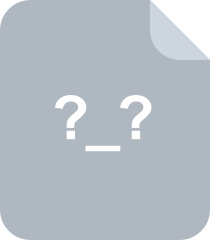
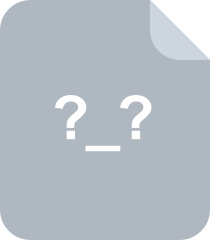
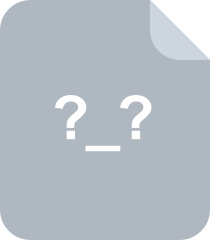
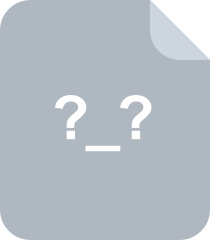
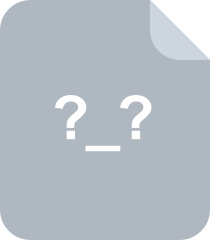
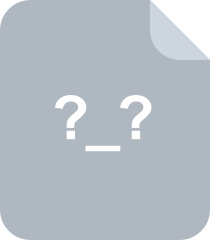
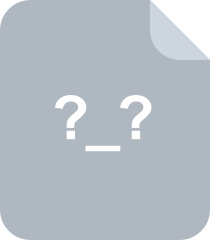
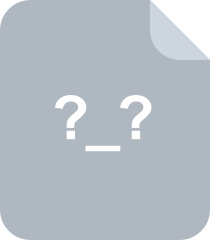
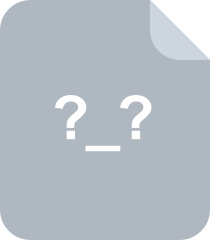
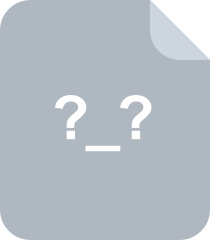
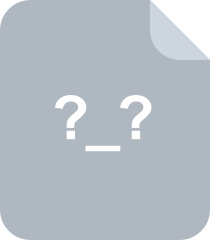
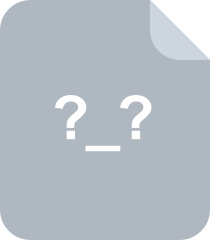
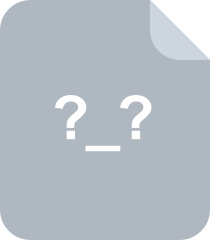
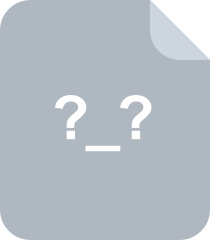
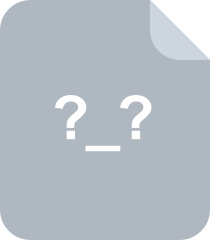
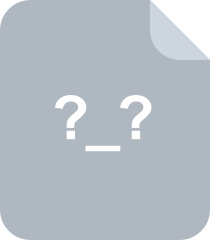
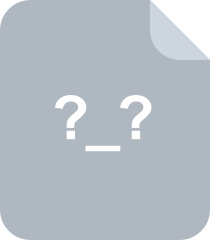
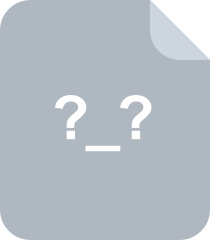
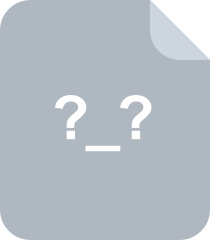
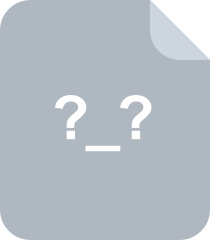
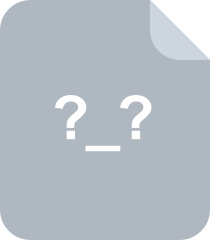
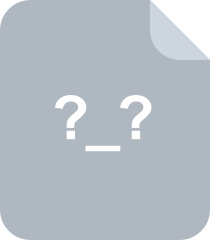
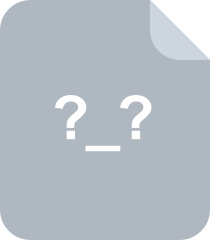
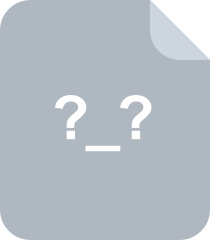
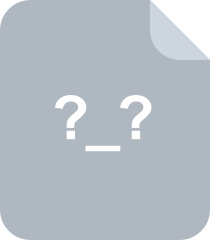
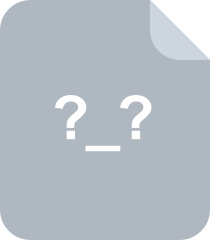
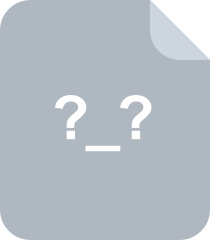
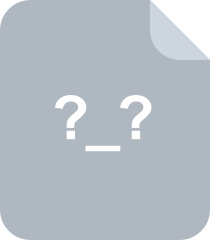
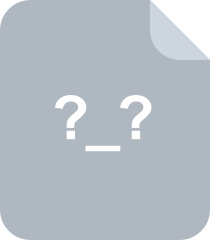
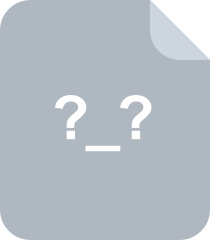
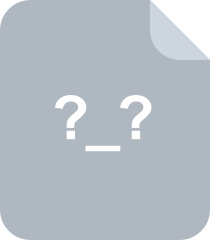
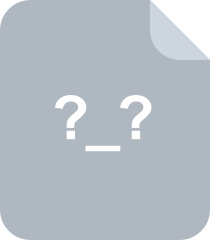
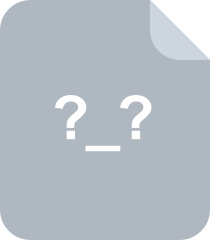
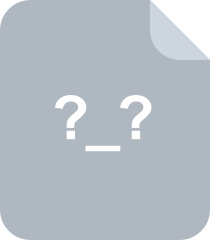
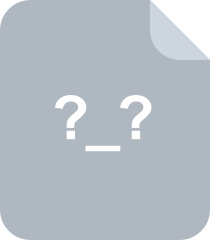
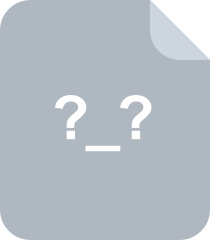
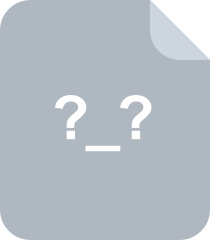
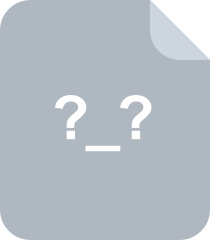
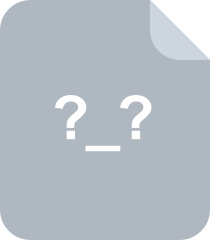
共 583 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
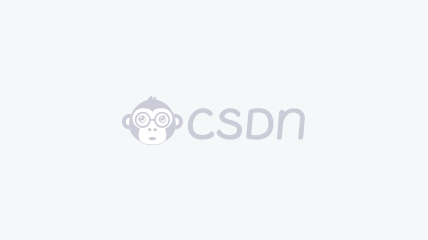

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7363
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

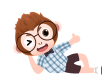
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


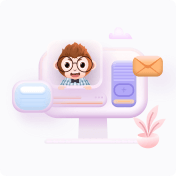
安全验证
文档复制为VIP权益,开通VIP直接复制
