<?php
/**
* Experimental HTML5-based parser using Jeroen van der Meer's PH5P library.
* Occupies space in the HTML5 pseudo-namespace, which may cause conflicts.
*
* @note
* Recent changes to PHP's DOM extension have resulted in some fatal
* error conditions with the original version of PH5P. Pending changes,
* this lexer will punt to DirectLex if DOM throughs an exception.
*/
class HTMLPurifier_Lexer_PH5P extends HTMLPurifier_Lexer_DOMLex {
public function tokenizeHTML($html, $config, $context) {
$new_html = $this->normalize($html, $config, $context);
$new_html = $this->wrapHTML($new_html, $config, $context);
try {
$parser = new HTML5($new_html);
$doc = $parser->save();
} catch (DOMException $e) {
// Uh oh, it failed. Punt to DirectLex.
$lexer = new HTMLPurifier_Lexer_DirectLex();
$context->register('PH5PError', $e); // save the error, so we can detect it
return $lexer->tokenizeHTML($html, $config, $context); // use original HTML
}
$tokens = array();
$this->tokenizeDOM(
$doc->getElementsByTagName('html')->item(0)-> // <html>
getElementsByTagName('body')->item(0)-> // <body>
getElementsByTagName('div')->item(0) // <div>
, $tokens);
return $tokens;
}
}
/*
Copyright 2007 Jeroen van der Meer <http://jero.net/>
Permission is hereby granted, free of charge, to any person obtaining a
copy of this software and associated documentation files (the
"Software"), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be included
in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
class HTML5 {
private $data;
private $char;
private $EOF;
private $state;
private $tree;
private $token;
private $content_model;
private $escape = false;
private $entities = array('AElig;','AElig','AMP;','AMP','Aacute;','Aacute',
'Acirc;','Acirc','Agrave;','Agrave','Alpha;','Aring;','Aring','Atilde;',
'Atilde','Auml;','Auml','Beta;','COPY;','COPY','Ccedil;','Ccedil','Chi;',
'Dagger;','Delta;','ETH;','ETH','Eacute;','Eacute','Ecirc;','Ecirc','Egrave;',
'Egrave','Epsilon;','Eta;','Euml;','Euml','GT;','GT','Gamma;','Iacute;',
'Iacute','Icirc;','Icirc','Igrave;','Igrave','Iota;','Iuml;','Iuml','Kappa;',
'LT;','LT','Lambda;','Mu;','Ntilde;','Ntilde','Nu;','OElig;','Oacute;',
'Oacute','Ocirc;','Ocirc','Ograve;','Ograve','Omega;','Omicron;','Oslash;',
'Oslash','Otilde;','Otilde','Ouml;','Ouml','Phi;','Pi;','Prime;','Psi;',
'QUOT;','QUOT','REG;','REG','Rho;','Scaron;','Sigma;','THORN;','THORN',
'TRADE;','Tau;','Theta;','Uacute;','Uacute','Ucirc;','Ucirc','Ugrave;',
'Ugrave','Upsilon;','Uuml;','Uuml','Xi;','Yacute;','Yacute','Yuml;','Zeta;',
'aacute;','aacute','acirc;','acirc','acute;','acute','aelig;','aelig',
'agrave;','agrave','alefsym;','alpha;','amp;','amp','and;','ang;','apos;',
'aring;','aring','asymp;','atilde;','atilde','auml;','auml','bdquo;','beta;',
'brvbar;','brvbar','bull;','cap;','ccedil;','ccedil','cedil;','cedil',
'cent;','cent','chi;','circ;','clubs;','cong;','copy;','copy','crarr;',
'cup;','curren;','curren','dArr;','dagger;','darr;','deg;','deg','delta;',
'diams;','divide;','divide','eacute;','eacute','ecirc;','ecirc','egrave;',
'egrave','empty;','emsp;','ensp;','epsilon;','equiv;','eta;','eth;','eth',
'euml;','euml','euro;','exist;','fnof;','forall;','frac12;','frac12',
'frac14;','frac14','frac34;','frac34','frasl;','gamma;','ge;','gt;','gt',
'hArr;','harr;','hearts;','hellip;','iacute;','iacute','icirc;','icirc',
'iexcl;','iexcl','igrave;','igrave','image;','infin;','int;','iota;',
'iquest;','iquest','isin;','iuml;','iuml','kappa;','lArr;','lambda;','lang;',
'laquo;','laquo','larr;','lceil;','ldquo;','le;','lfloor;','lowast;','loz;',
'lrm;','lsaquo;','lsquo;','lt;','lt','macr;','macr','mdash;','micro;','micro',
'middot;','middot','minus;','mu;','nabla;','nbsp;','nbsp','ndash;','ne;',
'ni;','not;','not','notin;','nsub;','ntilde;','ntilde','nu;','oacute;',
'oacute','ocirc;','ocirc','oelig;','ograve;','ograve','oline;','omega;',
'omicron;','oplus;','or;','ordf;','ordf','ordm;','ordm','oslash;','oslash',
'otilde;','otilde','otimes;','ouml;','ouml','para;','para','part;','permil;',
'perp;','phi;','pi;','piv;','plusmn;','plusmn','pound;','pound','prime;',
'prod;','prop;','psi;','quot;','quot','rArr;','radic;','rang;','raquo;',
'raquo','rarr;','rceil;','rdquo;','real;','reg;','reg','rfloor;','rho;',
'rlm;','rsaquo;','rsquo;','sbquo;','scaron;','sdot;','sect;','sect','shy;',
'shy','sigma;','sigmaf;','sim;','spades;','sub;','sube;','sum;','sup1;',
'sup1','sup2;','sup2','sup3;','sup3','sup;','supe;','szlig;','szlig','tau;',
'there4;','theta;','thetasym;','thinsp;','thorn;','thorn','tilde;','times;',
'times','trade;','uArr;','uacute;','uacute','uarr;','ucirc;','ucirc',
'ugrave;','ugrave','uml;','uml','upsih;','upsilon;','uuml;','uuml','weierp;',
'xi;','yacute;','yacute','yen;','yen','yuml;','yuml','zeta;','zwj;','zwnj;');
const PCDATA = 0;
const RCDATA = 1;
const CDATA = 2;
const PLAINTEXT = 3;
const DOCTYPE = 0;
const STARTTAG = 1;
const ENDTAG = 2;
const COMMENT = 3;
const CHARACTR = 4;
const EOF = 5;
public function __construct($data) {
$data = str_replace("\r\n", "\n", $data);
$data = str_replace("\r", null, $data);
$this->data = $data;
$this->char = -1;
$this->EOF = strlen($data);
$this->tree = new HTML5TreeConstructer;
$this->content_model = self::PCDATA;
$this->state = 'data';
while($this->state !== null) {
$this->{$this->state.'State'}();
}
}
public function save() {
return $this->tree->save();
}
private function char() {
return ($this->char < $this->EOF)
? $this->data[$this->char]
: false;
}
private function character($s, $l = 0) {
if($s + $l < $this->EOF) {
if($l === 0) {
return $this->data[$s];
} else {
return substr($this->data, $s, $l);
}
}
}
private function characters($char_class, $start) {
return preg_replace('#^(['.$char_class.']+).*#s', '\\1', substr($this->data, $start));
}
private function dataState() {
// Consume the next input character
$this->char++;
$char = $this->char();
if($char === '&' && ($this->content_model === self::PCDATA || $this->content_model === self::RCDATA)) {
/* U+0026 AMPERSAND (&)
When the content model flag is set to one of the PCDATA or RCDATA
states: switch to the entity data state. Otherwise: treat it as per
the "anything else" entry below. */
$this->state = 'entityData';
} elseif($char === '-') {
/* If the content model flag is set to either the RCDATA state or
the CDATA state, a

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7454
最新资源
- 【创新无忧】基于星雀优化算法NOA优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 注册程序源码-样式优化
- 【创新无忧】基于星雀优化算法NOA优化相关向量机RVM实现北半球光伏数据预测附matlab代码.rar
- 【创新无忧】基于星雀优化算法NOA优化极限学习机KELM实现故障诊断附matlab代码.rar
- 【创新无忧】基于星雀优化算法NOA优化相关向量机RVM实现数据多输入单输出回归预测附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化广义神经网络GRNN实现光伏预测附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化极限学习机KELM实现故障诊断附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化相关向量机RVM实现北半球光伏数据预测附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化极限学习机ELM实现乳腺肿瘤诊断附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化相关向量机RVM实现数据多输入单输出回归预测附matlab代码.rar
- 【创新无忧】基于遗传算法GA优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于遗传算法GA优化广义神经网络GRNN实现光伏预测附matlab代码.rar
- 【创新无忧】基于遗传算法GA优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 【创新无忧】基于遗传算法GA优化极限学习机KELM实现故障诊断附matlab代码.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


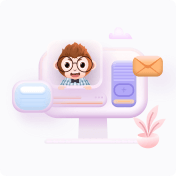