"undefined"!=typeof navigator&&function(t,e){"object"==typeof exports&&"undefined"!=typeof module?module.exports=e():"function"==typeof define&&define.amd?define(e):(t="undefined"!=typeof globalThis?globalThis:t||self).lottie=e()}(this,(function(){"use strict";var svgNS="http://www.w3.org/2000/svg",locationHref="",_useWebWorker=!1,initialDefaultFrame=-999999,setWebWorker=function(t){_useWebWorker=!!t},getWebWorker=function(){return _useWebWorker},setLocationHref=function(t){locationHref=t},getLocationHref=function(){return locationHref};function createTag(t){return document.createElement(t)}function extendPrototype(t,e){var i,r,s=t.length;for(i=0;i<s;i+=1)for(var a in r=t[i].prototype)Object.prototype.hasOwnProperty.call(r,a)&&(e.prototype[a]=r[a])}function getDescriptor(t,e){return Object.getOwnPropertyDescriptor(t,e)}function createProxyFunction(t){function e(){}return e.prototype=t,e}var audioControllerFactory=function(){function t(t){this.audios=[],this.audioFactory=t,this._volume=1,this._isMuted=!1}return t.prototype={addAudio:function(t){this.audios.push(t)},pause:function(){var t,e=this.audios.length;for(t=0;t<e;t+=1)this.audios[t].pause()},resume:function(){var t,e=this.audios.length;for(t=0;t<e;t+=1)this.audios[t].resume()},setRate:function(t){var e,i=this.audios.length;for(e=0;e<i;e+=1)this.audios[e].setRate(t)},createAudio:function(t){return this.audioFactory?this.audioFactory(t):window.Howl?new window.Howl({src:[t]}):{isPlaying:!1,play:function(){this.isPlaying=!0},seek:function(){this.isPlaying=!1},playing:function(){},rate:function(){},setVolume:function(){}}},setAudioFactory:function(t){this.audioFactory=t},setVolume:function(t){this._volume=t,this._updateVolume()},mute:function(){this._isMuted=!0,this._updateVolume()},unmute:function(){this._isMuted=!1,this._updateVolume()},getVolume:function(){return this._volume},_updateVolume:function(){var t,e=this.audios.length;for(t=0;t<e;t+=1)this.audios[t].volume(this._volume*(this._isMuted?0:1))}},function(){return new t}}(),createTypedArray=function(){function t(t,e){var i,r=0,s=[];switch(t){case"int16":case"uint8c":i=1;break;default:i=1.1}for(r=0;r<e;r+=1)s.push(i);return s}return"function"==typeof Uint8ClampedArray&&"function"==typeof Float32Array?function(e,i){return"float32"===e?new Float32Array(i):"int16"===e?new Int16Array(i):"uint8c"===e?new Uint8ClampedArray(i):t(e,i)}:t}();function createSizedArray(t){return Array.apply(null,{length:t})}function _typeof$6(t){return _typeof$6="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(t){return typeof t}:function(t){return t&&"function"==typeof Symbol&&t.constructor===Symbol&&t!==Symbol.prototype?"symbol":typeof t},_typeof$6(t)}var subframeEnabled=!0,expressionsPlugin=null,expressionsInterfaces=null,idPrefix$1="",isSafari=/^((?!chrome|android).)*safari/i.test(navigator.userAgent),_shouldRoundValues=!1,bmPow=Math.pow,bmSqrt=Math.sqrt,bmFloor=Math.floor,bmMax=Math.max,bmMin=Math.min,BMMath={};function ProjectInterface$1(){return{}}!function(){var t,e=["abs","acos","acosh","asin","asinh","atan","atanh","atan2","ceil","cbrt","expm1","clz32","cos","cosh","exp","floor","fround","hypot","imul","log","log1p","log2","log10","max","min","pow","random","round","sign","sin","sinh","sqrt","tan","tanh","trunc","E","LN10","LN2","LOG10E","LOG2E","PI","SQRT1_2","SQRT2"],i=e.length;for(t=0;t<i;t+=1)BMMath[e[t]]=Math[e[t]]}(),BMMath.random=Math.random,BMMath.abs=function(t){if("object"===_typeof$6(t)&&t.length){var e,i=createSizedArray(t.length),r=t.length;for(e=0;e<r;e+=1)i[e]=Math.abs(t[e]);return i}return Math.abs(t)};var defaultCurveSegments=150,degToRads=Math.PI/180,roundCorner=.5519;function roundValues(t){_shouldRoundValues=!!t}function bmRnd(t){return _shouldRoundValues?Math.round(t):t}function styleDiv(t){t.style.position="absolute",t.style.top=0,t.style.left=0,t.style.display="block",t.style.transformOrigin="0 0",t.style.webkitTransformOrigin="0 0",t.style.backfaceVisibility="visible",t.style.webkitBackfaceVisibility="visible",t.style.transformStyle="preserve-3d",t.style.webkitTransformStyle="preserve-3d",t.style.mozTransformStyle="preserve-3d"}function BMEnterFrameEvent(t,e,i,r){this.type=t,this.currentTime=e,this.totalTime=i,this.direction=r<0?-1:1}function BMCompleteEvent(t,e){this.type=t,this.direction=e<0?-1:1}function BMCompleteLoopEvent(t,e,i,r){this.type=t,this.currentLoop=i,this.totalLoops=e,this.direction=r<0?-1:1}function BMSegmentStartEvent(t,e,i){this.type=t,this.firstFrame=e,this.totalFrames=i}function BMDestroyEvent(t,e){this.type=t,this.target=e}function BMRenderFrameErrorEvent(t,e){this.type="renderFrameError",this.nativeError=t,this.currentTime=e}function BMConfigErrorEvent(t){this.type="configError",this.nativeError=t}function BMAnimationConfigErrorEvent(t,e){this.type=t,this.nativeError=e}var createElementID=(_count=0,function(){return idPrefix$1+"__lottie_element_"+(_count+=1)}),_count;function HSVtoRGB(t,e,i){var r,s,a,n,o,h,l,p;switch(h=i*(1-e),l=i*(1-(o=6*t-(n=Math.floor(6*t)))*e),p=i*(1-(1-o)*e),n%6){case 0:r=i,s=p,a=h;break;case 1:r=l,s=i,a=h;break;case 2:r=h,s=i,a=p;break;case 3:r=h,s=l,a=i;break;case 4:r=p,s=h,a=i;break;case 5:r=i,s=h,a=l}return[r,s,a]}function RGBtoHSV(t,e,i){var r,s=Math.max(t,e,i),a=Math.min(t,e,i),n=s-a,o=0===s?0:n/s,h=s/255;switch(s){case a:r=0;break;case t:r=e-i+n*(e<i?6:0),r/=6*n;break;case e:r=i-t+2*n,r/=6*n;break;case i:r=t-e+4*n,r/=6*n}return[r,o,h]}function addSaturationToRGB(t,e){var i=RGBtoHSV(255*t[0],255*t[1],255*t[2]);return i[1]+=e,i[1]>1?i[1]=1:i[1]<=0&&(i[1]=0),HSVtoRGB(i[0],i[1],i[2])}function addBrightnessToRGB(t,e){var i=RGBtoHSV(255*t[0],255*t[1],255*t[2]);return i[2]+=e,i[2]>1?i[2]=1:i[2]<0&&(i[2]=0),HSVtoRGB(i[0],i[1],i[2])}function addHueToRGB(t,e){var i=RGBtoHSV(255*t[0],255*t[1],255*t[2]);return i[0]+=e/360,i[0]>1?i[0]-=1:i[0]<0&&(i[0]+=1),HSVtoRGB(i[0],i[1],i[2])}var rgbToHex=function(){var t,e,i=[];for(t=0;t<256;t+=1)e=t.toString(16),i[t]=1===e.length?"0"+e:e;return function(t,e,r){return t<0&&(t=0),e<0&&(e=0),r<0&&(r=0),"#"+i[t]+i[e]+i[r]}}(),setSubframeEnabled=function(t){subframeEnabled=!!t},getSubframeEnabled=function(){return subframeEnabled},setExpressionsPlugin=function(t){expressionsPlugin=t},getExpressionsPlugin=function(){return expressionsPlugin},setExpressionInterfaces=function(t){expressionsInterfaces=t},getExpressionInterfaces=function(){return expressionsInterfaces},setDefaultCurveSegments=function(t){defaultCurveSegments=t},getDefaultCurveSegments=function(){return defaultCurveSegments},setIdPrefix=function(t){idPrefix$1=t},getIdPrefix=function(){return idPrefix$1};function createNS(t){return document.createElementNS(svgNS,t)}function _typeof$5(t){return _typeof$5="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(t){return typeof t}:function(t){return t&&"function"==typeof Symbol&&t.constructor===Symbol&&t!==Symbol.prototype?"symbol":typeof t},_typeof$5(t)}var dataManager=function(){var t,e,i=1,r=[],s={onmessage:function(){},postMessage:function(e){t({data:e})}},a={postMessage:function(t){s.onmessage({data:t})}};function n(){e||(e=function(e){if(window.Worker&&window.Blob&&getWebWorker()){var i=new Blob(["var _workerSelf = self; self.onmessage = ",e.toString()],{type:"text/javascript"}),r=URL.createObjectURL(i);return new Worker(r)}return t=e,s}((function(t){if(a.dataManager||(a.dataManager=function(){function t(s,a){var n,o,h,l,p,c,m=s.length;for(o=0;o<m;o+=1)if("ks"in(n=s[o])&&!n.completed){if(n.completed=!0,n.hasMask){var d=n.masksProperties;for(l=d.length,h=0;h<l;h+=1)if(d[h].pt.k.i)r(d[h].pt.k);else for(c=d[h].pt.k.length,p=0;p<c;p+=1)d[h].pt.k[p].s&&r(d[h].pt.k[p].s[0]),d[h].pt.k[p].e&&r(d[h].pt.k[p].e[0])}0===n.ty?(n.layers=e(n.refId,a),t(n.layers,a)):4===n.ty?i(n.shapes):5===n.ty&&f(n)}}function e(t,e){var i=function(t,e){for(var i=0,r=e.length;i<r;){if(e[i].id===t)return e[i];i+=1}return null}(t,e);return i?i.layers.__used?JSON.parse(JSON.stringify(i.layers)):(i.layers.__used=!0,i.layers):null}function i(t){var e,s,
没有合适的资源?快使用搜索试试~ 我知道了~
一个将Web动画渲染为视频的框架,基于Node.js+Puppeteer+Chrome+FFmpeg实现,使用最先进浏览器API
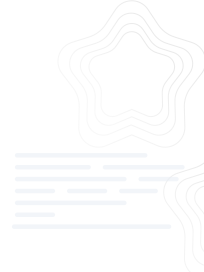
共58个文件
js:44个
md:5个
gif:4个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 182 浏览量
2023-11-04
14:27:18
上传
评论
收藏 6.43MB ZIP 举报
温馨提示
WebVideoCreator(简称WVC)是一个将Web动画渲染为视频的框架,基于 Node.js + Puppeteer + Chrome + FFmpeg 实现,它执行确定性的渲染,准确的以目标帧率捕获任何可在HTML5播放动画(CSS3动画/SVG动画/Lottie动画/GIF动画/APNG动画/WEBP动画)以及任何基于时间轴使用RAF驱动的动画(anime.js是一个不错的选择 :D),当然您也可以调皮的使用setInterval或者setTimeout来控制动画,支持嵌入mp4和透明webm视频,还支持转场合成、音频合成与字体加载等功能。
资源推荐
资源详情
资源评论
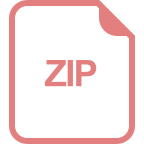
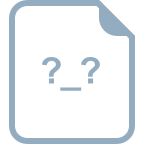
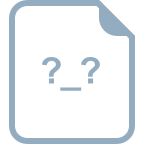
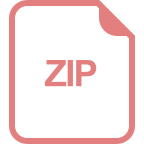
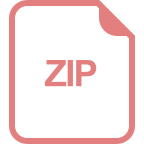
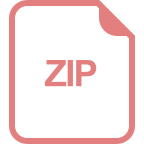
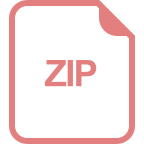
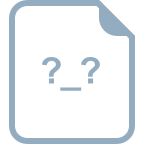
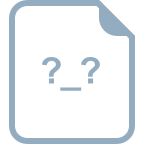
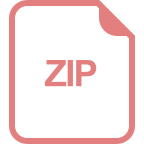
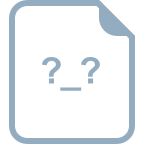
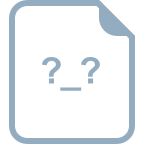
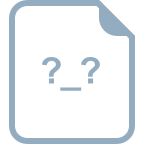
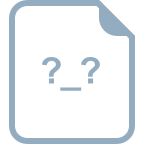
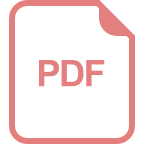
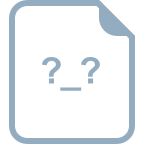
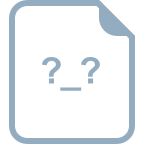
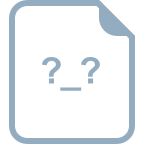
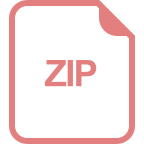
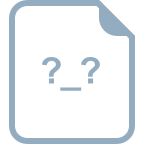
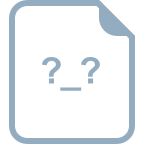
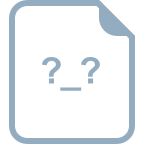
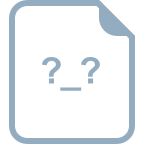
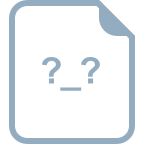
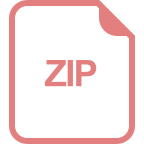
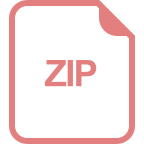
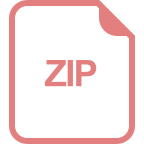
收起资源包目录



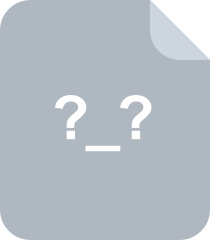
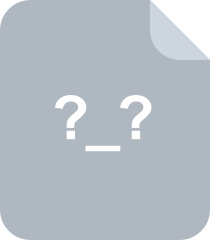
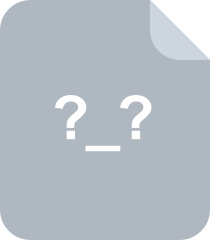
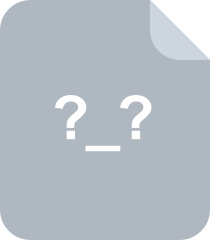
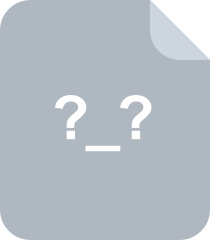
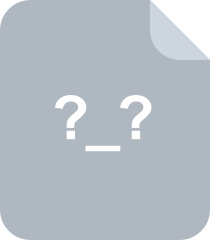
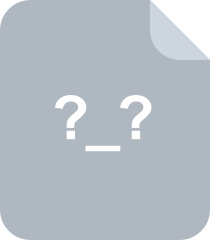
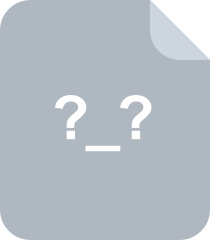
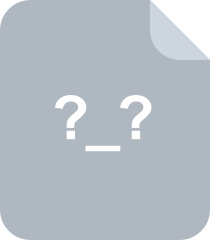
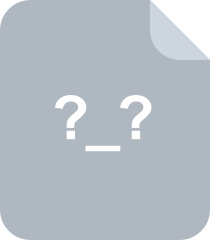
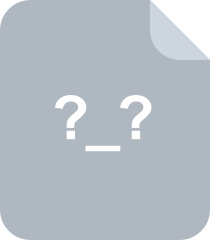

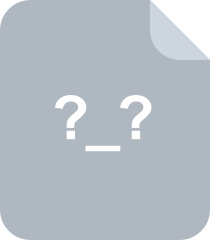
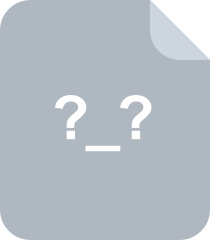
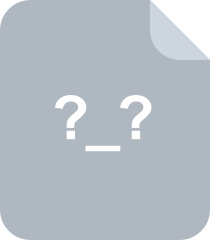
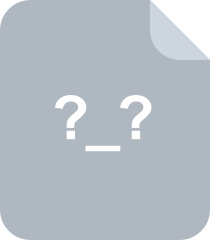
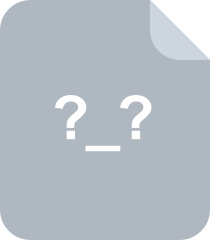

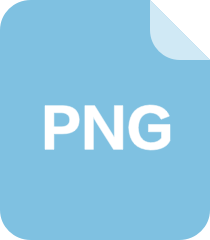
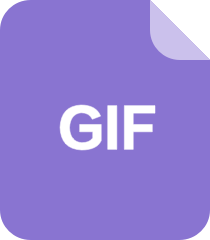
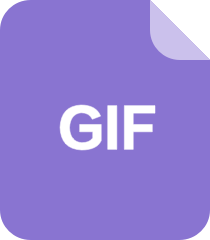
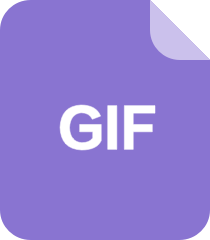
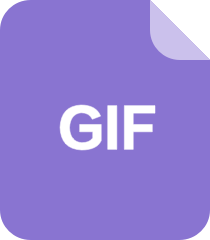


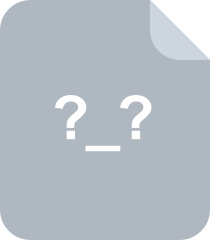
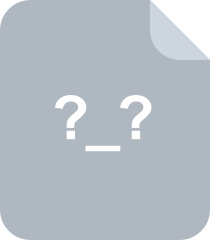
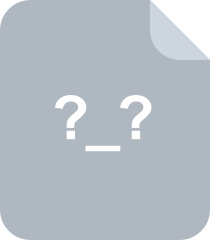
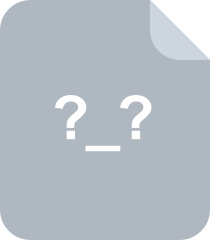

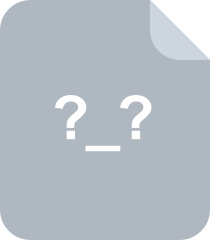
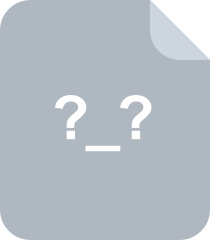
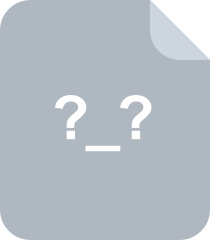
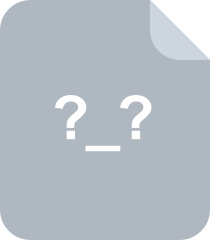
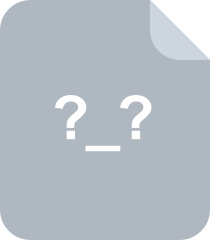

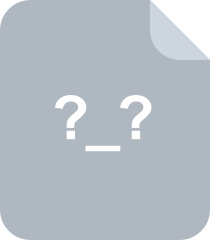
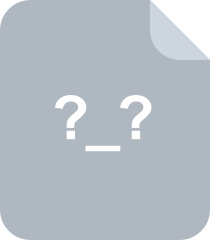
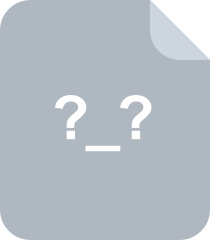
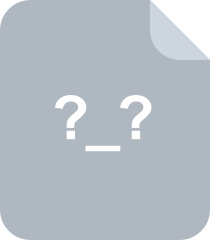
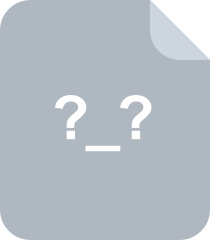

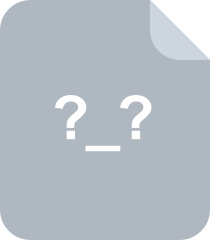
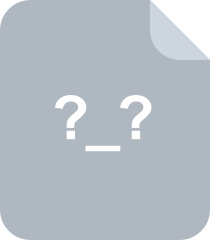
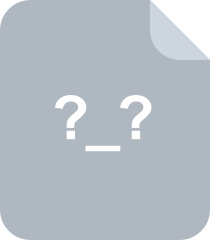

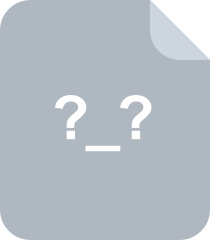
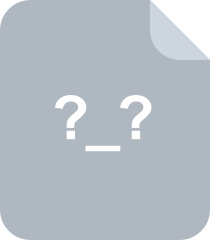
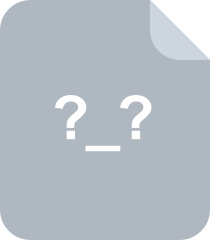
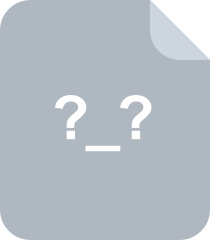
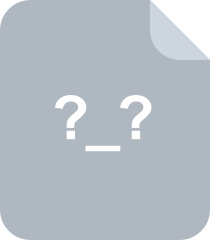
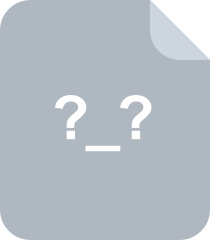

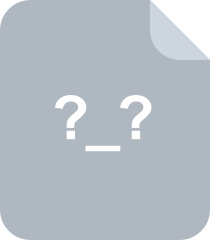
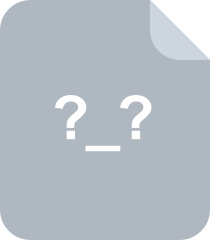
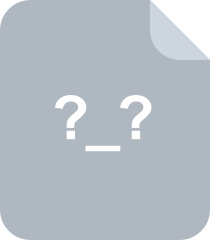
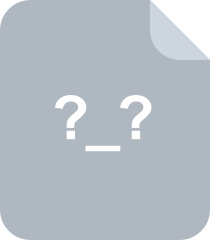
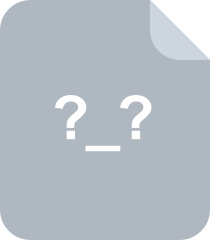
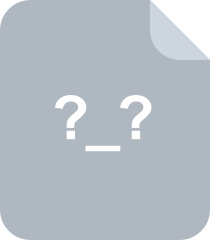
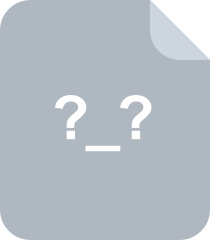
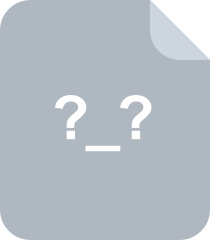

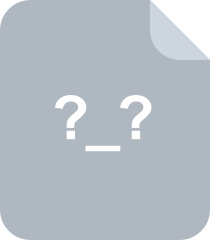
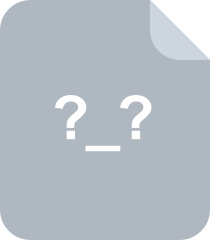
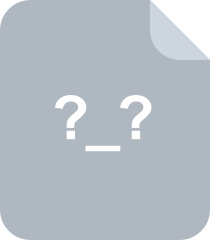
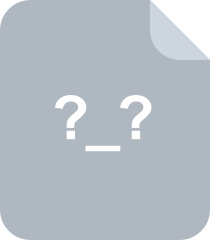
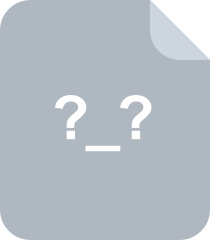
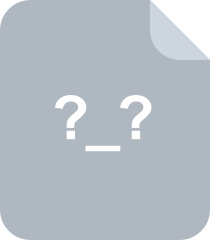
共 58 条
- 1
资源评论
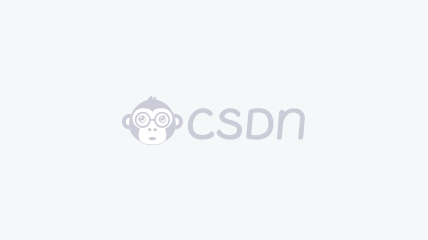

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7364
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

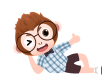
最新资源
- SparkSQL进阶操作相关数据
- java制作的小游戏,作为巩固java知识之用.zip
- Java语言写的围棋小游戏 半成品A Go game written in golang(Semi-finished).zip
- 基于Java-swing的俄罗斯方块游戏:源码+答辩文档+PPT.zip
- florr map详细版
- shiahdifhiahfiqefiwhfi weifwijfiwqufiqweefijeq0jfe
- registry-2.8.3<arm/amd>二进制文件
- Kotlin接口与抽象类详解及其应用
- 51单片机加减乘除计算器系统设计(proteus8.17,keil5),复制粘贴就可以运行
- lv_0_20241114231223.mp4
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


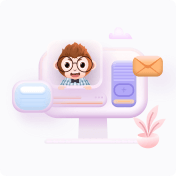
安全验证
文档复制为VIP权益,开通VIP直接复制
