#!/usr/bin/env python
#
# Hi There!
#
# You may be wondering what this giant blob of binary data here is, you might
# even be worried that we're up to something nefarious (good for you for being
# paranoid!). This is a base85 encoding of a zip file, this zip file contains
# an entire copy of pip (version 22.2.2).
#
# Pip is a thing that installs packages, pip itself is a package that someone
# might want to install, especially if they're looking to run this get-pip.py
# script. Pip has a lot of code to deal with the security of installing
# packages, various edge cases on various platforms, and other such sort of
# "tribal knowledge" that has been encoded in its code base. Because of this
# we basically include an entire copy of pip inside this blob. We do this
# because the alternatives are attempt to implement a "minipip" that probably
# doesn't do things correctly and has weird edge cases, or compress pip itself
# down into a single file.
#
# If you're wondering how this is created, it is generated using
# `scripts/generate.py` in https://github.com/pypa/get-pip.
import sys
this_python = sys.version_info[:2]
min_version = (3, 7)
if this_python < min_version:
message_parts = [
"This script does not work on Python {}.{}".format(*this_python),
"The minimum supported Python version is {}.{}.".format(*min_version),
"Please use https://bootstrap.pypa.io/pip/{}.{}/get-pip.py instead.".format(*this_python),
]
print("ERROR: " + " ".join(message_parts))
sys.exit(1)
import os.path
import pkgutil
import shutil
import tempfile
import argparse
import importlib
from base64 import b85decode
def include_setuptools(args):
"""
Install setuptools only if absent and not excluded.
"""
cli = not args.no_setuptools
env = not os.environ.get("PIP_NO_SETUPTOOLS")
absent = not importlib.util.find_spec("setuptools")
return cli and env and absent
def include_wheel(args):
"""
Install wheel only if absent and not excluded.
"""
cli = not args.no_wheel
env = not os.environ.get("PIP_NO_WHEEL")
absent = not importlib.util.find_spec("wheel")
return cli and env and absent
def determine_pip_install_arguments():
pre_parser = argparse.ArgumentParser()
pre_parser.add_argument("--no-setuptools", action="store_true")
pre_parser.add_argument("--no-wheel", action="store_true")
pre, args = pre_parser.parse_known_args()
args.append("pip")
if include_setuptools(pre):
args.append("setuptools")
if include_wheel(pre):
args.append("wheel")
return ["install", "--upgrade", "--force-reinstall"] + args
def monkeypatch_for_cert(tmpdir):
"""Patches `pip install` to provide default certificate with the lowest priority.
This ensures that the bundled certificates are used unless the user specifies a
custom cert via any of pip's option passing mechanisms (config, env-var, CLI).
A monkeypatch is the easiest way to achieve this, without messing too much with
the rest of pip's internals.
"""
from pip._internal.commands.install import InstallCommand
# We want to be using the internal certificates.
cert_path = os.path.join(tmpdir, "cacert.pem")
with open(cert_path, "wb") as cert:
cert.write(pkgutil.get_data("pip._vendor.certifi", "cacert.pem"))
install_parse_args = InstallCommand.parse_args
def cert_parse_args(self, args):
if not self.parser.get_default_values().cert:
# There are no user provided cert -- force use of bundled cert
self.parser.defaults["cert"] = cert_path # calculated above
return install_parse_args(self, args)
InstallCommand.parse_args = cert_parse_args
def bootstrap(tmpdir):
monkeypatch_for_cert(tmpdir)
# Execute the included pip and use it to install the latest pip and
# setuptools from PyPI
from pip._internal.cli.main import main as pip_entry_point
args = determine_pip_install_arguments()
sys.exit(pip_entry_point(args))
def main():
tmpdir = None
try:
# Create a temporary working directory
tmpdir = tempfile.mkdtemp()
# Unpack the zipfile into the temporary directory
pip_zip = os.path.join(tmpdir, "pip.zip")
with open(pip_zip, "wb") as fp:
fp.write(b85decode(DATA.replace(b"\n", b"")))
# Add the zipfile to sys.path so that we can import it
sys.path.insert(0, pip_zip)
# Run the bootstrap
bootstrap(tmpdir=tmpdir)
finally:
# Clean up our temporary working directory
if tmpdir:
shutil.rmtree(tmpdir, ignore_errors=True)
DATA = b"""
P)h>@6aWAK2mt4n167QOF#Goa003nH000jF003}la4%n9X>MtBUtcb8c|B0kYQr!LeD_y~yljQehBDX
|=wL5{!Pxe&w^D+eD2X7kjijvP@8`6nwn6x~I~~_@96{$qp#vIIWQ9)>-NJWLiop-YRMj7{<WE(>9*W
)0=35wJ8f;<o6z)BDQr;AY#o!>y$7AMy#ef@lcm)bt8px+8ir=0D^7CPVk+TZkem)?EVTKWXP-r8=Bn
YP&5~VwVcn(m<AaOvDnklJRb1{6+RNpjoT@MYar$M$Lh|sGhDV^&``{`usIJz_^@3N;OkqN~;J-$5KH
Q8mhODUOQt2LdSG+SB>%5#btm9?$v%swULYZOMEINZQXGaK^{P)h>@6aWAK2mt4n163m%<G*bJ006E8
000jF003}la4%n9ZDDC{Utcb8d0kV%Zk#X>z4H|#a#(gnNDl3xQMXd1UUKOns+2=hAs2?lTCkCAmTbS
iZw%}v;e_osZ{EC_(fwduAnOx7|M~OfcT&!1bMQ%WLr>h>6Vqlx%G6Yi&anZ)x+4%&YsXcv?o5rdJ%y
I3(ar|~$ej^x8zC+R722G1LxW>41i_LzSzXiUg=gix@F7$i8uUPw?R%v5RJB|kb0lK^#~?F4sIJSY)5
s^{d~2s1fm6!{&nJ82nv|#E99nDWwvT*Y7s79ezH0k@|3~s=X_~{5;=rMr>TJ7xNC@AGDh4}b_gk^$v
7w1cIVLw6F>5wJNpglLRoBstc$8{a4#fUywI@iolaH9us{^kpA@O!sOl@~3VwWPXw<5YM2UQ&rQ<k*f
Q<}~yZ}8CQPHw^fj_0l{d!<cT7Q=bL&TWF<M)gdUB=N!N!Ar|FZv!?td9JJ&yaEIP+ReiC#D468m>?B
Ob}1_ogY0U94xYPa3Hlx#QsJ#R<gM6RDfDk1MP!Ni__~+g#6~Q-vybQ1f$jEv<KQE^klp8z2px<zO33
2jTJOcnXG}QyYezFTlAW`T9E&Q96^7;J%jZu*$flOk6=vHG?BMKcv5@?<fgk8Gh$ZZ0@U`k@L*^9&xC
@BEyXQc=zuZ{IyQO*n7NYrs8MzF!5Y&uSs#MUJnN|%@ftd=cZ9(@~5o3dlryOQQ>tYvk+Vz+vO9D#u!
9W1E0MvZIBJO`&6FtxPoO)Z29q-d(H%}riUtWq6I}cPE+2#hsrGV_B)CLKS>WZq<g?PP6jsGYrHXymQ
jbC-h;vLp7`43P_0|XQR000O8=avIiGk4f42?78B4FmuH6#xJLaA|NaUte%(a4m9mZf<3AUtcb8d3{q
&Z`&{oz3W$Sy37F-W9I-K1{598!<HaPPeC9kHr)uYB~OyO`rnU|zuF$AgDp{fkK`l8Ie&b^vJVLOrpn
L^5boMTX}bYz4V~@;{MBSLprF!qjSfu!G_TrO)6za?>>MGrZV>2iRErfT4?56MK(OTPdxylV!bsoRsw
eMKnXdtE1Zz9%0>NIjV#-Ws@IeI}V{pzHo6N2^-7p{g;OA`^I`ph|ww(wXeal-_Qu64Ane<nRed@3ja
8Tj?Q=1Ci2(v!#$TYiF+Qn+dJ7U-7Wxm>XL0h950Vn*^qbX6qvmp~>ho^%)?Vpbik0*KD@6S-oMA`h8
GO8uzX2TdO8|6J5boZ2~k4Rov1`0N&wqro+M{;X1+-;Q%71pG=sidb;vSf<OV^fReRQF92Bko8`x0+*
-yQPN9T*4()aJdZusd0q3Uayq>Z)<SH2IXEbyhA71^HJ6_?LR^`u>HRJuHs*J3!|==JmE_LoQ96Cw{b
ZPy)%iGt(6iBg<5-<uX4<M0xqB1aTQYun}vyl#W+f7CbG`}v1GR*ri3DbI!H5^Q)iTny5`zMw(OhuPf
xSh`6;ZFky}bAsCFq^rHzza0Dn-%*fj3sL!xYHN0TgNPLGowm;z(XHu_HwinH`Ia{9{Ak|i-k<VaD9_
W@KBh>emj&aS88{sB-+0|XQR000O8=avIi&BwqszyJUM9svLV3;+NCaA|NaaCt6td2nT9C62L9#V`y-
_kP9QSf2V13=C{<Kw>tnX)PsolsJX_Jx&=d9p7_`6i5SMvz$qHBvD4Gc2vqMK2J#u@ySRoi8HJ74pBU
ZpQaDYr)B{xbde<biidBj6SwLQ4C~0fIn*4z#kiE0Sc{#il<@j|pBMZL#}ADaAESsKi)hSbaxtCyXu4
z%H~r`8#VV{D!!(UMBd94M9GxnKfLFZz7T$d6N~+ca-?5#f2RFEdlxM*G?W6ErQaLd-ZtL;~P)h>@6a
WAK2mt4n167u_aG_BF002D#000>P003}la4%nJZggdGZeeUMUtei%X>?y-E^v8ukuguiFbswF{0i$>t
`ejR5^xfXOea{_5ITj{ZH>|-*e<C59=C8HB*+r$$$9?r+;JX3=R&Cm8cSw{J&B&eeNoCOMCZQbLd72_
DYB`4Qi|d!y<npU!DeDq4oTKlIDwR3gX<RaKi(ZD9b)dCI{`|hOWhmAwt{gIg=d5&#E7j`U1o%k=2Zd
B@YU;k)V-C++sbU-2WkcwLMfO8f*!}@4#sSjV{WI2;@vXK{~qd`Yti}wrETC|cCbAr@VEr>D9TSy6<o
tzPFTU&jZy2)ft}4}^DvP8N}w<b@|#f`GvwGplau6#APrMd0UZo%3^Rx&5tnZ=cF33-<5=xTy<3Z0vj
}ZVpBT`h1`F>L1Q7<+BD=coNr&m#H+ihfTtaPW*CaBb)EDPhm;MO2-v8~xV^W?=HuYyW@4N)bpD4E7i
PN{ZMpU^EP)h>@6aWAK2mt4n166RfP;hYz002KF000^Q003}la4%nJZggdGZeeUMVs&Y3WM5@&b}n#v
)f!!M+qm&vzXD|*O1321>(h;<YMVGp;;GN}#Ll%h8V!dgAsaPC@Bq@b&g1{yU3`!LNjbSrUrrB}2<$H
QdlyRwLGZ3)RY}(M0~5U7)4C;{q$SyLL73!K-ZEa3vJ<SnC9-6)B{zrxkBcA(77Mm-xM)emZ*OtUJaT
D&HF<kiu^am+5B*L{pV~)}H;bL%ds6VKq6MBNi2=9}B^9)M&x%&V0CK_W9lNy<x3tah0IB!eLqmJ)Ym
pc9CNJ)<VYS~!F)YAOm+S20)B5C}r{^DIa@tan-&8avXCP%vE*fMeuVQjV;qkg_a8D7_)Ed^L*#oUhF
4BUEmhG4&{dfnAPoWy%CPy`;g8tkwK~dj5!=Hz>*H9m*kZ2Qw^`m|qc(NN=wqq5|GLlPN&MICgV>+~1
4Kjm>X;HCMf^KRcX7PDL>&z_9;aGaoGX^*eSUc7ag6`N8Ei+h=W~mbty<vvlp0;_Jx4BEMq`SPU+Kdk
Fsp&WibF{jaRWg;`O(S?gWzS-fS5-DFA4hHJzR5
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
扬帆测试平台是一款高效、可靠的自动化测试平台,旨在帮助团队提升测试效率、降低测试成本。该平台包括用例管理、定时任务、执行记录等功能模块,支持多种类型的测试用例,目前支持API(http和grpc协议)、性能、CI调用等功能,并且可定制化,灵活满足不同场景的需求。 其中,支持批量执行、并发执行等高级功能。通过用例设置,可以设置用例的基本信息、运行配置、环境变量等,灵活控制用例的执行
资源推荐
资源详情
资源评论
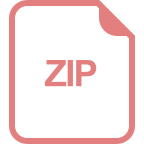
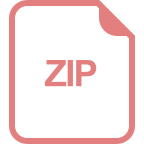
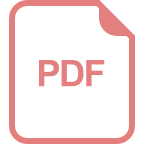
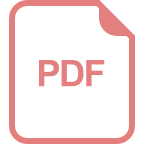
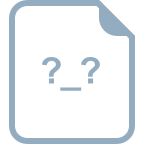
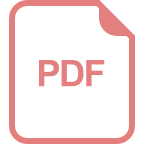
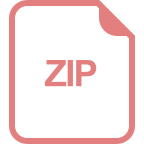
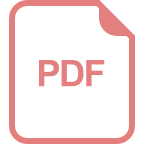
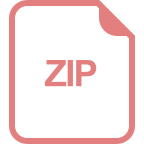
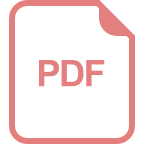
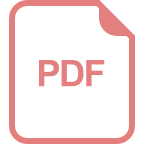
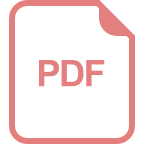
收起资源包目录

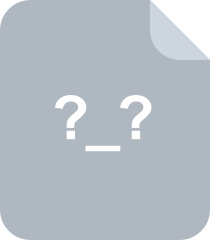
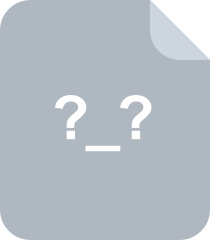
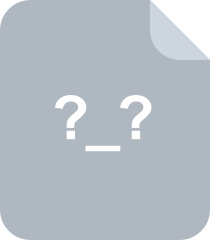
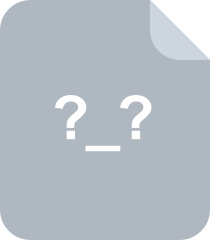
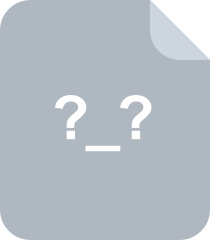
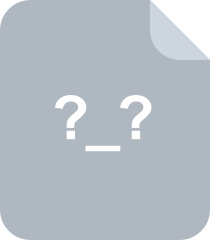
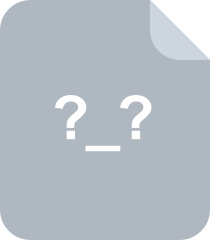
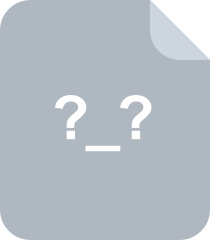
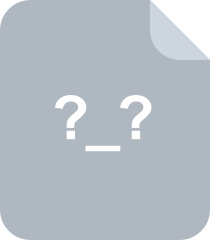
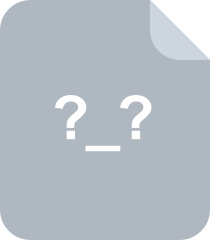
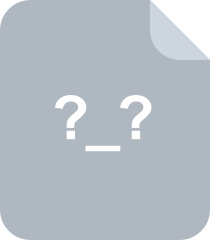
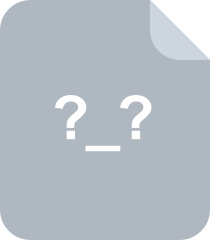
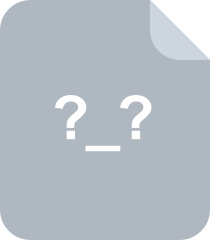
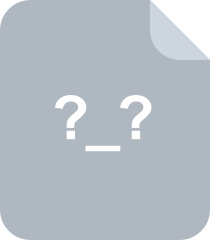
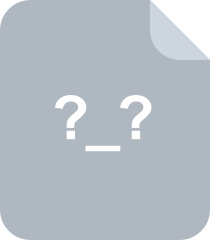
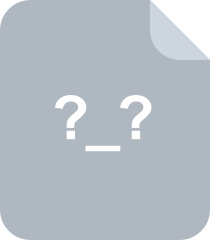
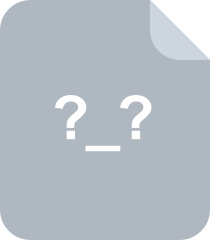
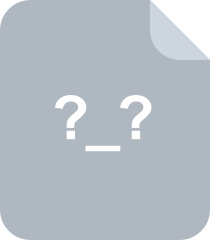
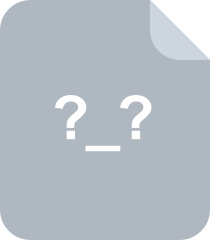
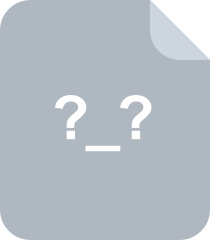
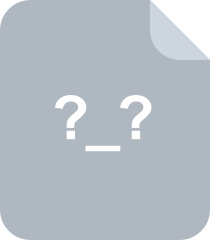
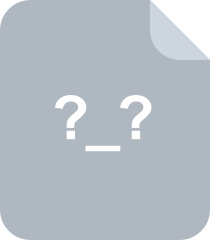
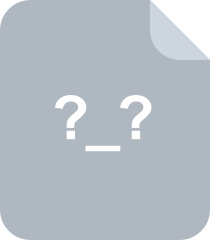
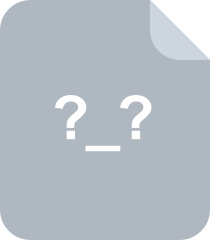
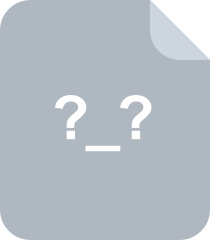
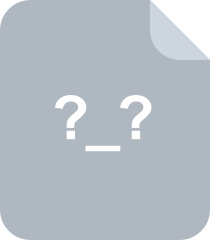
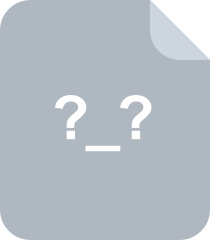
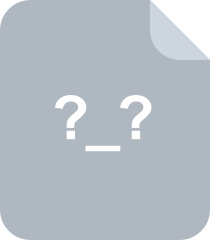
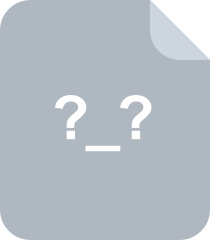
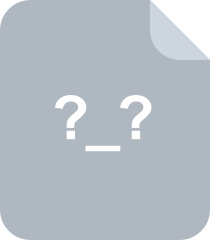
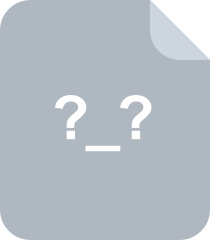
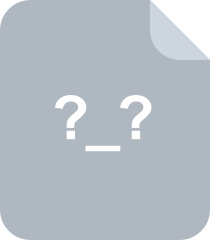
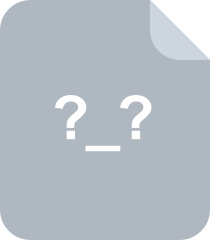
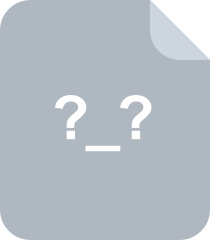
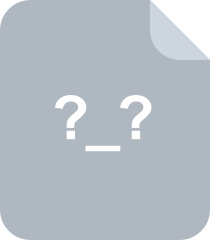
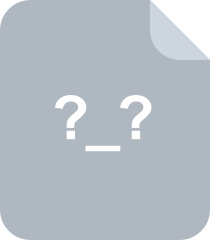
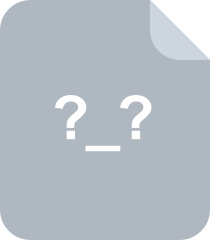
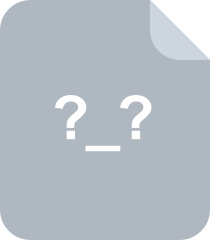
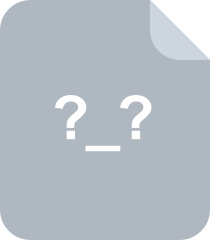
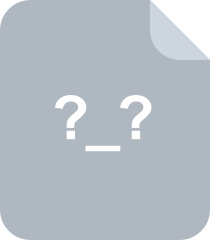
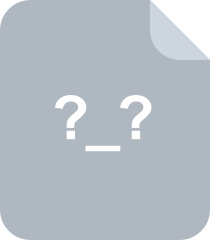
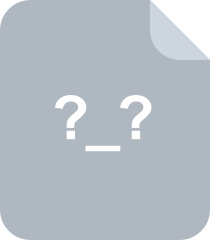
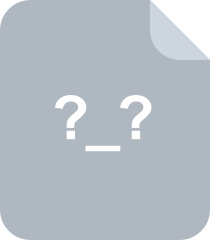
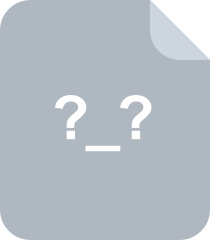
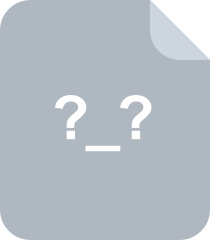
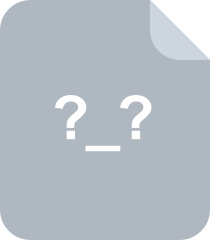
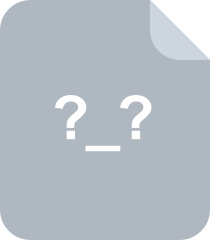
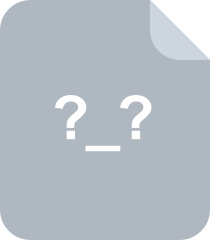
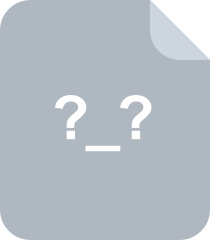
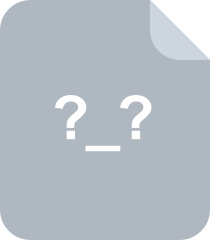
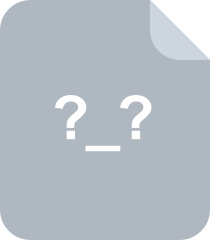
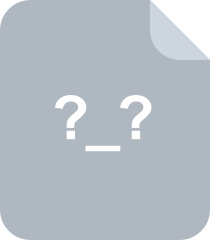
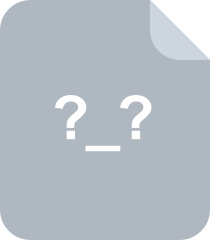
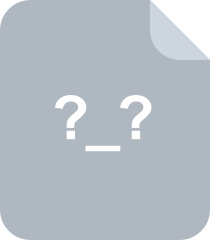
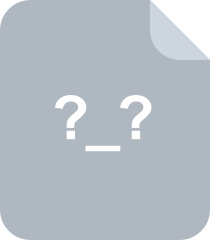
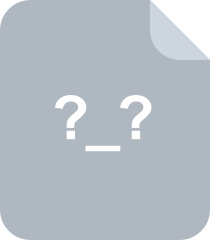
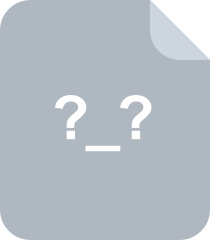
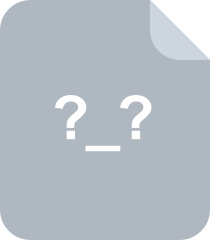
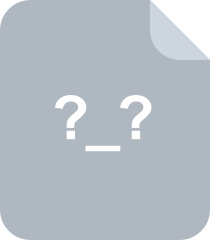
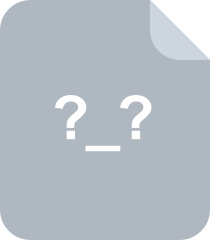
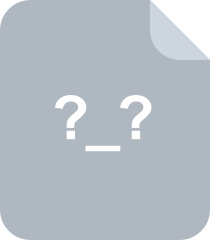
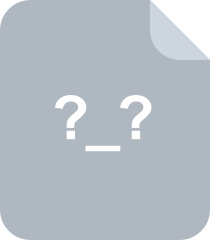
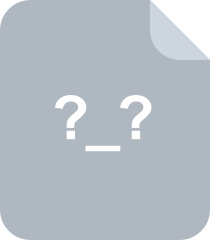
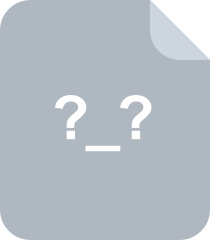
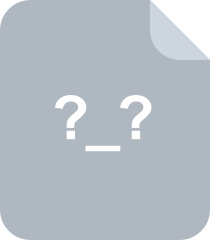
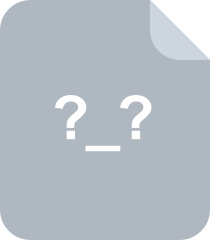
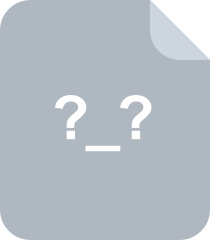
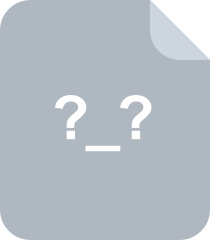
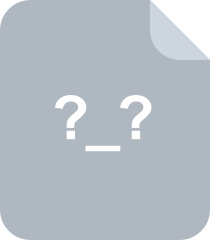
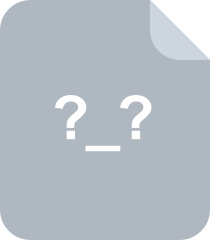
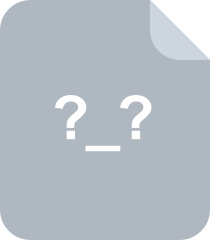
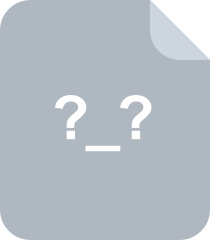
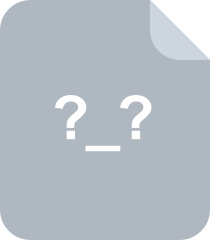
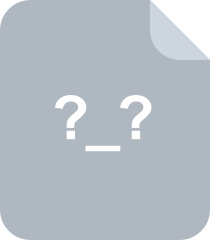
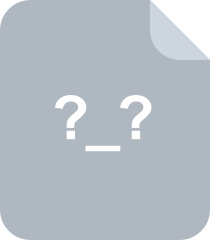
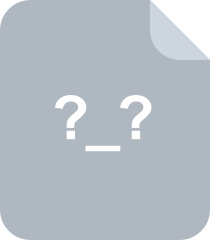
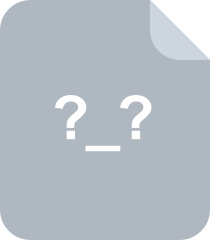
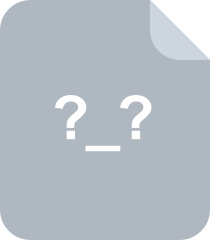
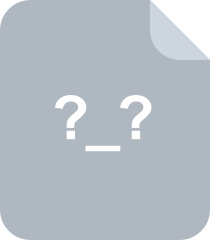
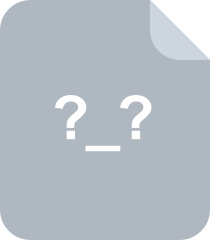
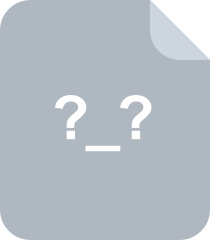
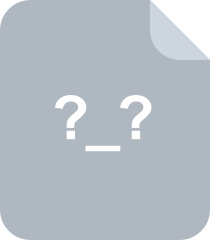
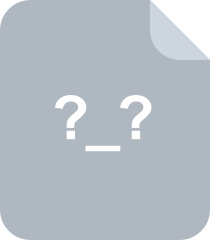
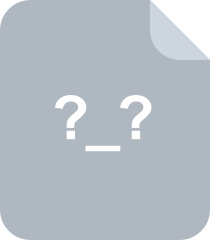
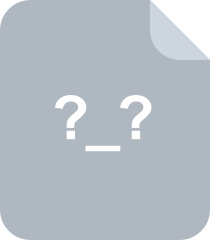
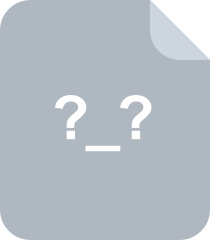
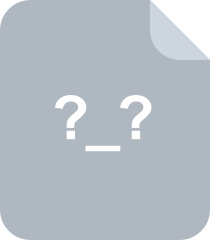
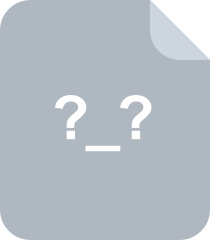
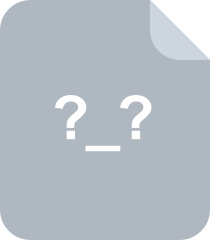
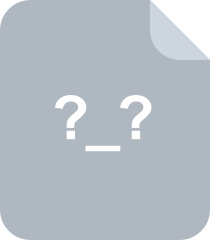
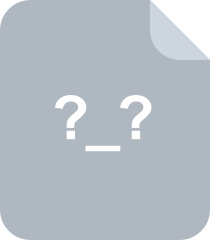
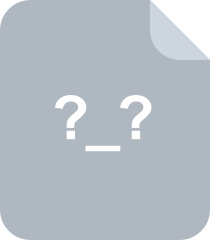
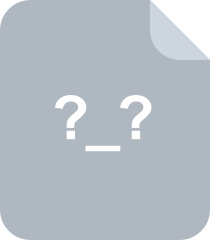
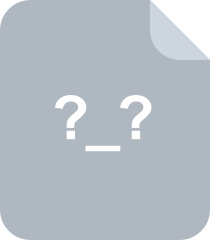
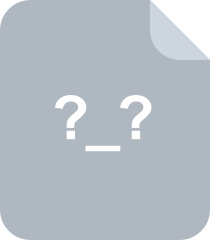
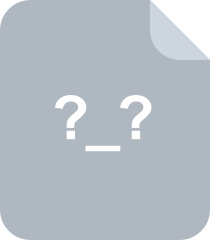
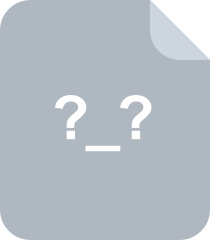
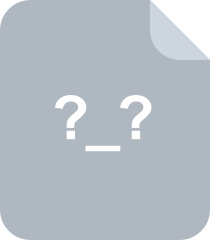
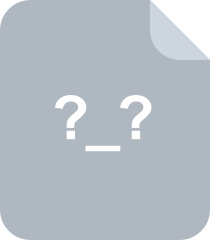
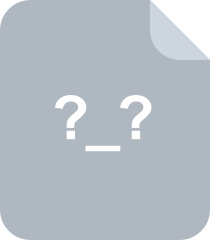
共 1695 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17
资源评论
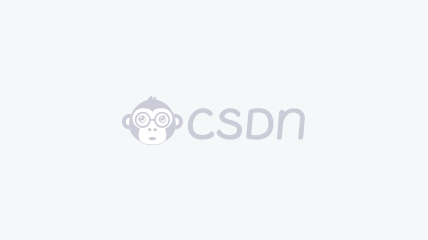

Java程序员-张凯
- 粉丝: 1w+
- 资源: 6649
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

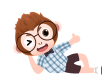
安全验证
文档复制为VIP权益,开通VIP直接复制
