<?php
defined('BASE_PATH') OR exit('No direct script access allowed');
/**
* YanPHP
* User: weilongjiang(江炜隆)<[email protected]>
*/
use Yan\Core\Log;
use Yan\Core\ReturnCode;
use Yan\Core\Exception;
use Yan\Core\Config;
if (!function_exists('isCli')) {
/**
* 判断是否为cli访问
*
* @return bool
*/
function isCli()
{
return defined('STDIN') || PHP_SAPI === 'cli' ? true : false;
}
}
if (!function_exists('setHeader')) {
/**
* @param int $code
*/
function setHeader($code = 200)
{
if (isCli()) return;
$code = intval($code);
$status = array(
100 => 'Continue',
101 => 'Switching Protocols',
200 => 'OK',
201 => 'Created',
202 => 'Accepted',
203 => 'Non-Authoritative Information',
204 => 'No Content',
205 => 'Reset Content',
206 => 'Partial Content',
300 => 'Multiple Choices',
301 => 'Moved Permanently',
302 => 'Found',
303 => 'See Other',
304 => 'Not Modified',
305 => 'Use Proxy',
307 => 'Temporary Redirect',
400 => 'Bad Request',
401 => 'Unauthorized',
402 => 'Payment Required',
403 => 'Forbidden',
404 => 'Not Found',
405 => 'Method Not Allowed',
406 => 'Not Acceptable',
407 => 'Proxy Authentication Required',
408 => 'Request Timeout',
409 => 'Conflict',
410 => 'Gone',
411 => 'Length Required',
412 => 'Precondition Failed',
413 => 'Request Entity Too Large',
414 => 'Request-URI Too Sys',
415 => 'Unsupported Media Type',
416 => 'Requested Range Not Satisfiable',
417 => 'Expectation Failed',
422 => 'Unprocessable Entity',
500 => 'Internal Server Error',
501 => 'Not Implemented',
502 => 'Bad Gateway',
503 => 'Service Unavailable',
504 => 'Gateway Timeout',
505 => 'HTTP Version Not Supported'
);
if (!isset($status[$code])) {
throwErr('Invalid error code', 500, Exception\InvalidArgumentException::class);
}
if (strpos(PHP_SAPI, 'Cgi') === 0) {
header('Status:' . $code . ' ' . $status[$code], true);
} else {
$protocol = isset($_SERVER['SERVER_PROTOCOL']) ? $_SERVER['SERVER_PROTOCOL'] : 'HTTP/1.1';
header($protocol . ' ' . $code . ' ' . $status[$code], true, $code);
}
}
}
if (!function_exists('errorHandler')) {
function errorHandler($severity, $errMsg, $errFile, $errLine, $errContext)
{
$is_error = (((E_ERROR | E_USER_ERROR | E_COMPILE_ERROR | E_CORE_ERROR | E_USER_ERROR) & $severity) === $severity);
if (($severity & error_reporting()) !== $severity) return;
Log::error($errMsg, ['file' => $errFile, 'line' => $errLine, 'msg' => $errMsg]);
/**
* 判断是否为致命错误
*/
if ($is_error) {
$namespace = Config::get('namespace') ?: '\\Yan\\Core';
$namespace .= '\\Compo\\Result';
$result = new $namespace(ReturnCode::SYSTEM_ERROR, $errMsg);
showResult($result);
}
}
}
if (!function_exists('exceptionHandler')) {
/**
* 异常处理
* @param \Exception $exception
*/
function exceptionHandler($exception)
{
//TODO handle db exception
$code = $exception->getCode() == 0 || !is_numeric($exception->getCode()) ? ReturnCode::SYSTEM_ERROR : $exception->getCode();
$code = intval($code);
\Yan\Core\Log::error($exception->getMessage(), $exception->getTrace());
$namespace = Config::get('namespace') ?: '\\Yan\\Core';
$namespace .= '\\Compo\\Result';
$result = new $namespace($code, $exception->getMessage());
showResult($result);
}
}
if (!function_exists('showResult')) {
/**
* 打印输出结果
* @param \Yan\Core\Compo\ResultInterface $result
*/
function showResult(\Yan\Core\Compo\ResultInterface $result)
{
Log::info('response=' . $result->getMessage(), $result->jsonSerialize());
exit(json_encode($result->jsonSerialize()));
}
}
if (!function_exists('throwErr')) {
function throwErr(string $message = '', int $code, $exceptionClass = '\\Exception')
{
/** @var \Exception $exception */
$exception = new $exceptionClass($message, $code);
throw $exception;
}
}
if (!function_exists('isPHP')) {
/**
* Determines if the current version of PHP is equal to or greater than the supplied value
*
* @param string
* @return bool TRUE if the current version is $version or higher
*/
function isPHP($version)
{
static $_isPHP;
$version = (string)$version;
if (!isset($_isPHP[$version])) {
$_isPHP[$version] = version_compare(PHP_VERSION, $version, '>=');
}
return $_isPHP[$version];
}
}
if (!function_exists('input')) {
/**
* get input params
* @param string $key format:get.a(return Input::get('a')) post.b(return Input::post('b')) c(return Input::input('c'))
* @return array|null|string
*/
function input($key = '')
{
$key = $key ?: '';
return Yan\Core\Input::get($key);
}
}
if (!function_exists('genResult')) {
function genResult(int $code, string $msg, array $data = []): \Yan\Core\Compo\ResultInterface
{
$namespace = Config::get('namespace') ?: '\\Yan\\Core';
$namespace .= '\\Compo\\Result';
return new $namespace($code, $msg, $data);
}
}
if (!function_exists('show404')) {
function show404()
{
$code = ReturnCode::REQUEST_404;
$msg = '404 Not Found';
header("HTTP/1.1 404 Not Found");
$result = genResult($code, $msg);
showResult($result);
}
}
if (!function_exists('getClassName')) {
/**
* 获取短类名
*
* @param object|string $classNameWithNamespace
* @return bool|string
*/
function getClassName($classNameWithNamespace)
{
if (is_object($classNameWithNamespace)) {
return substr(strrchr(get_class($classNameWithNamespace), '\\'), 1);
} elseif (is_string($classNameWithNamespace)) {
return substr(strrchr($classNameWithNamespace, '\\'), 1);
}
return $classNameWithNamespace;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
这是一个为API开发而设计的高性能轻量级框架。 框架为你集成了一些常用的类库,让你开发更加便捷。 另外引入了Composer,可以让你更加轻松地管理依赖以及处理命名空间问题。 让语法更加贴近原生PHP,并且在此基础上让你实现自己的定制化功能。 因为封装程度不高,所以框架的性能十分之优秀
资源推荐
资源详情
资源评论
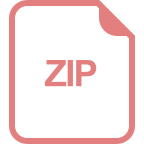
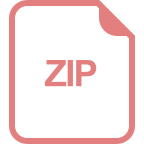
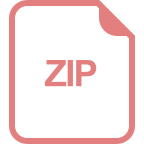
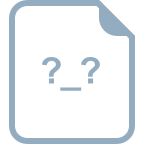
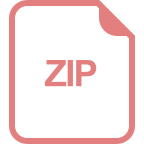
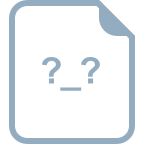
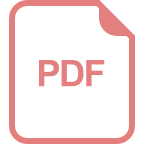
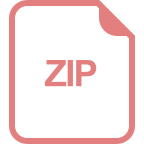
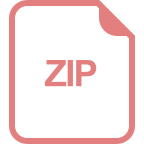
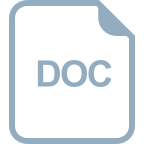
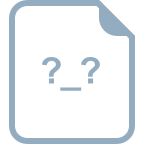
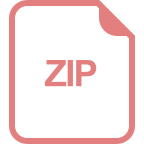
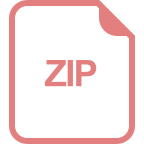
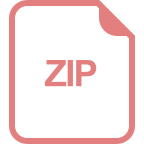
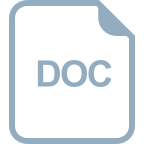
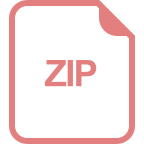
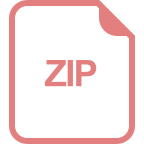
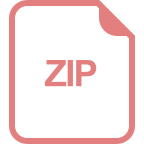
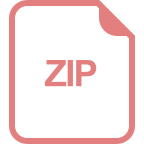
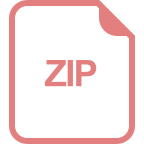
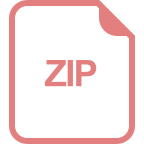
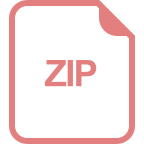
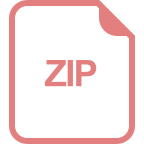
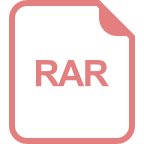
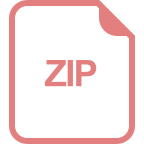
收起资源包目录



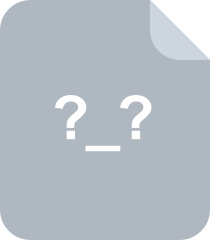
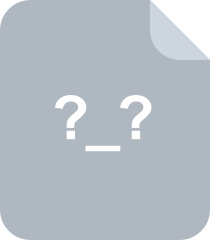


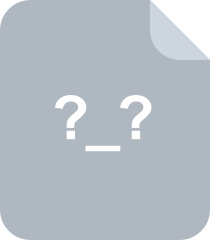
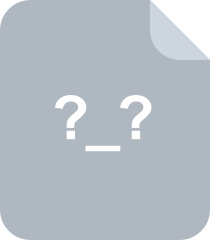
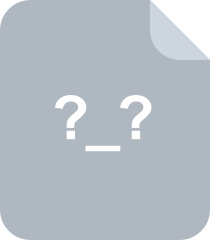

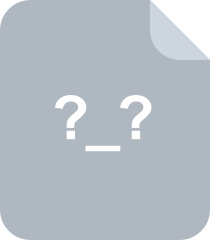
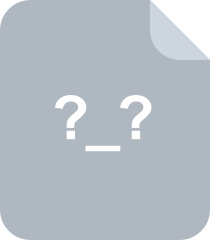
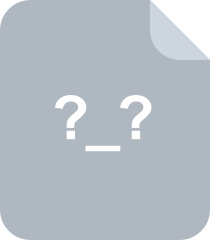
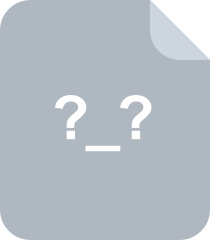
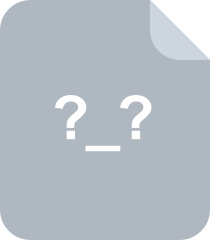
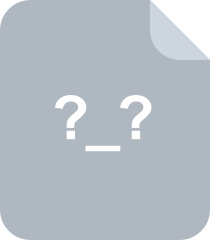
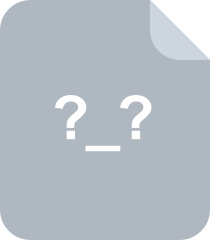
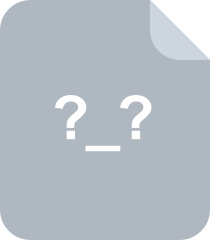
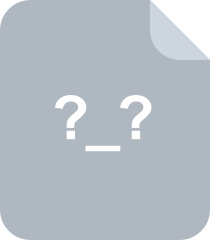
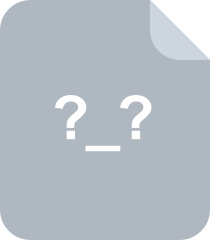
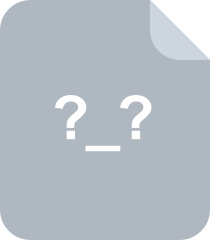

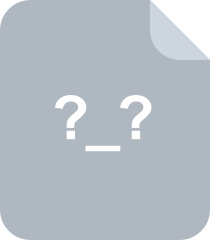
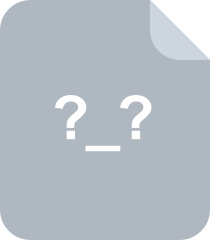
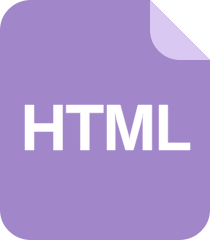
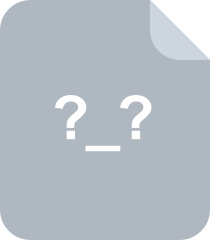
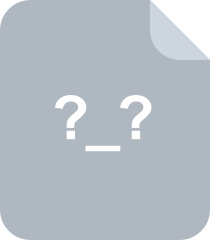
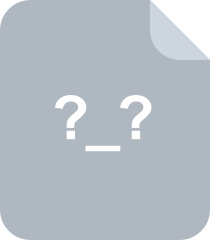
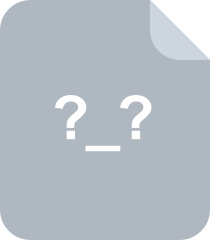

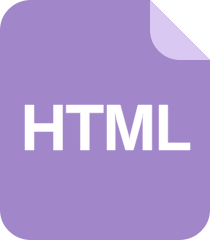
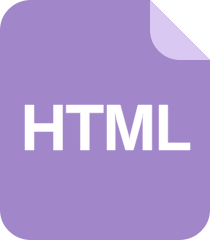

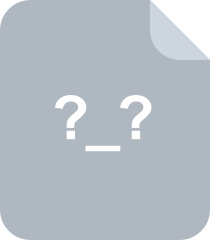
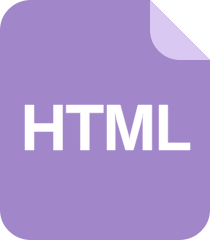

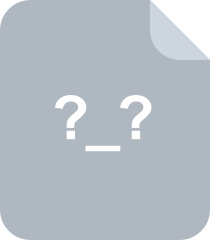
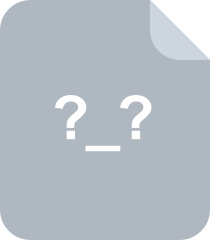
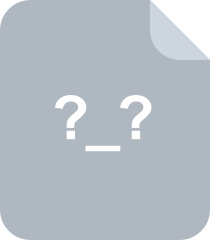
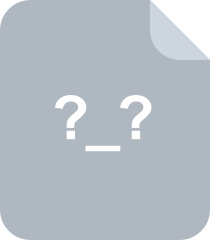
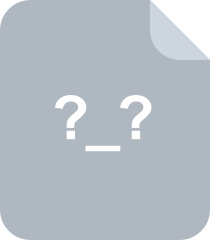

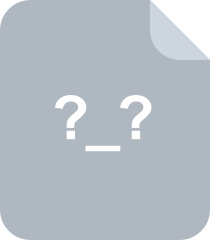

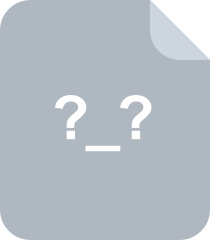
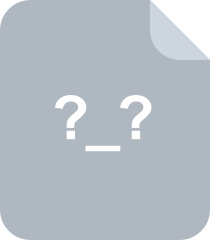
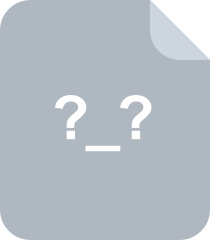
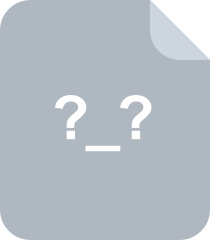
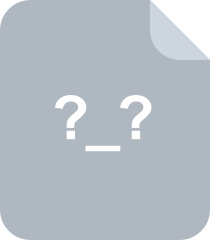
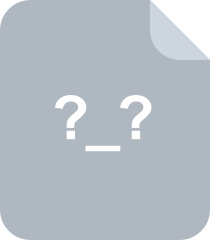
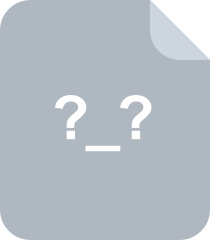
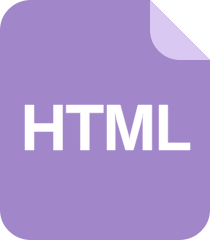
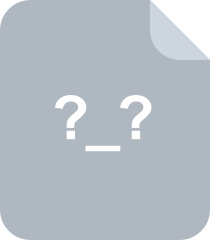
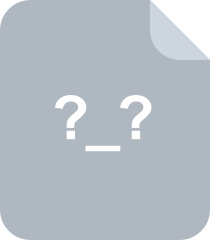


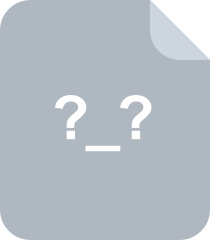
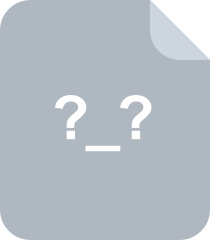

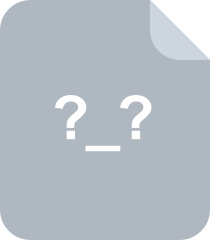
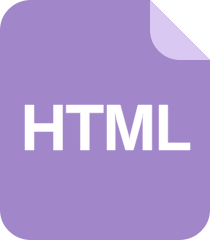

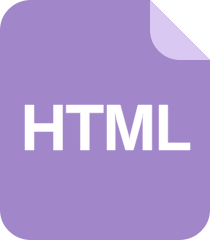

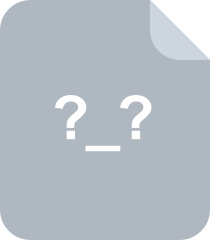

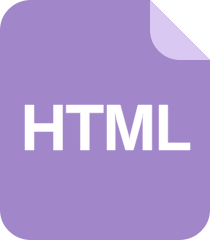

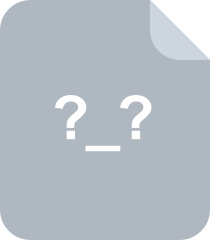
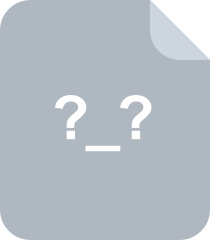
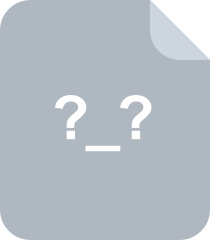
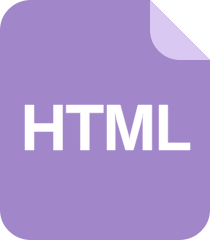

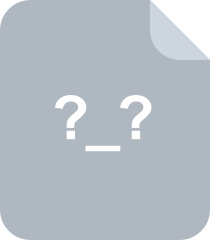
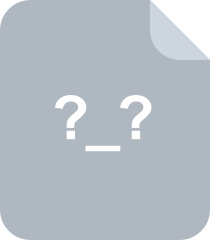
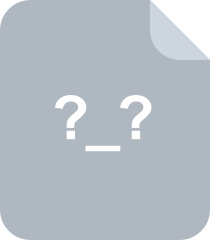
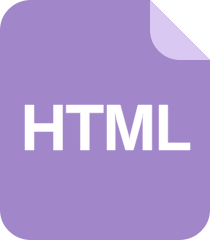

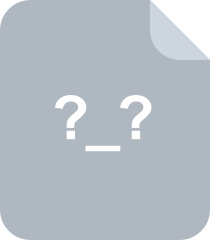
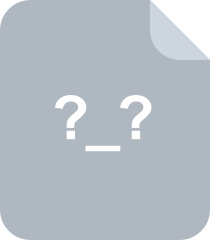
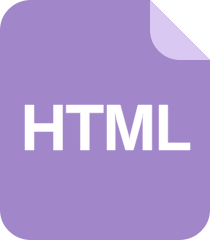
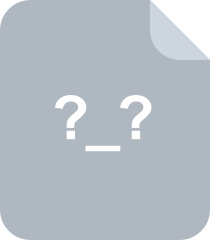

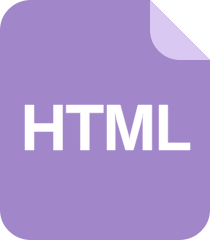

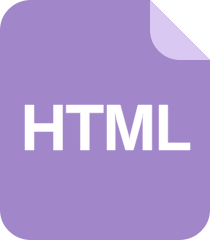

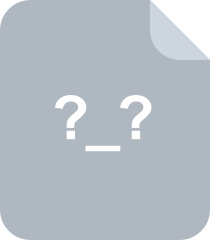
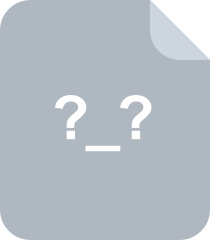

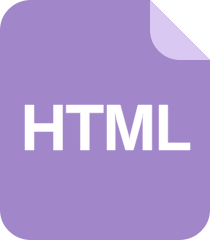

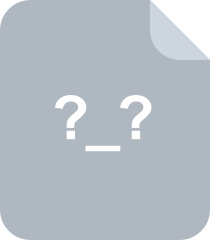

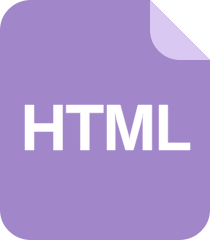

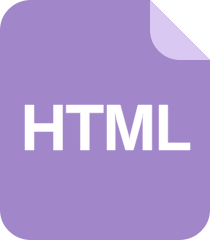

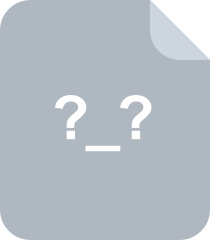
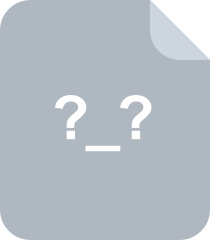
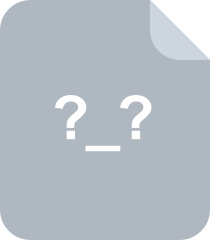
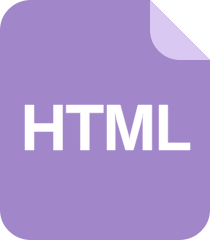

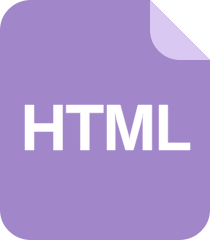

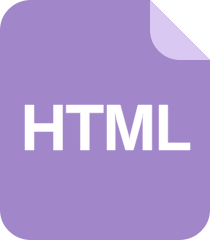

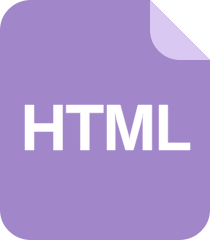
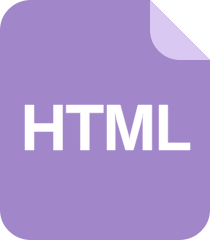
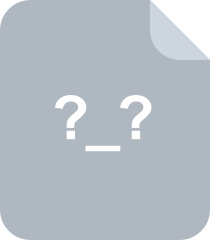
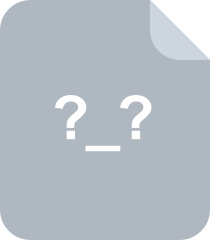
共 80 条
- 1
资源评论
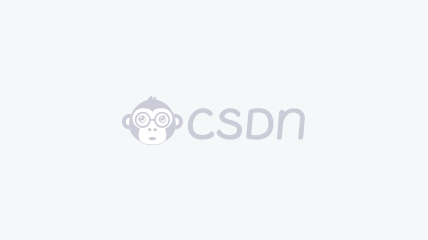

Java程序员-张凯
- 粉丝: 1w+
- 资源: 6727
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

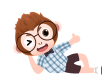
最新资源
- 海信智能电视刷机数据 LED32K20JD(1115)BOM5 生产用软件数据 务必确认机编一致 强制刷机 整机USB升级程序
- 520表白html5爱心代码
- TINY Syntax Tree -setup1.0.0
- mmexport1689832776313.jpg
- 月宝の病理の爱(黑).zi
- 海信智能电视刷机数据 LED32EC510N(2000)BOM22 生产用软件数据 务必确认机编一致 强制刷机 整机USB升级
- 电磁场与波,非常基础的知识总结
- android ios java后台通用DES base64加密
- 华为OD刷题C卷练习记录(300道).rar
- 最新《Vue-框架开发》期末考试试题分享给需要的同学
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


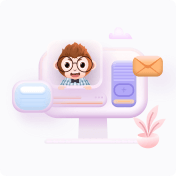
安全验证
文档复制为VIP权益,开通VIP直接复制
