package xplay;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonElement;
import com.google.gson.JsonParser;
class Rect {
Rect(int _x, int _y, int _width, int _height) {
x = _x;
y = _y;
width = _width;
height = _height;
}
int x;
int y;
int width;
int height;
}
class Dep {
String path; // 素材路径
String type; // 素材类型(video or pic)
int duration; // 素材持续时间(非视频)
}
public class XplayCtl {
XplayCtl(String _host, int _port) {
host = _host;
port = _port;
}
private String host;
private int port;
private Socket socket = null;
private boolean connXplay(String _data) {
try {
if (socket == null || !socket.isConnected())
socket = new Socket(host, port);
OutputStreamWriter os = new OutputStreamWriter(socket.getOutputStream(), "UTF-8");
os.write(_data + "\n#End\n");
os.flush();
BufferedReader br = new BufferedReader(new InputStreamReader(socket.getInputStream(), "UTF-8"));
String data = null;
while ((data = br.readLine()) != null) {
if (data.contains("#End"))
break;
System.out.println(data);
}
} catch (UnknownHostException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return false;
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return false;
}
return true;
}
private boolean playXplay(Map<String, Object> _data) {
Gson gson = new GsonBuilder().setPrettyPrinting().create();
String json = gson.toJson(_data);
JsonParser jp = new JsonParser();
JsonElement je = jp.parse(json);
String prettyJsonString = gson.toJson(je);
System.out.print(prettyJsonString);
return this.connXplay(prettyJsonString);
}
/*
* 序列(视频与图片)
* _content 一组素材,每一个素材为一个 Dep 包含素材路径、类型(libName)、持续时间(非视频)
*/
public boolean playSequence(long _start, String _id, int _index, ArrayList<Dep> _content, Rect _rect, String _mode,
int _rotate) {
Map<String, Object> data = new HashMap<String, Object>();
Map<String, Object> params = new HashMap<String, Object>();
data.put("id", _id);
data.put("type", "sequence");
data.put("start", _start);
data.put("libName", "video");
params.put("zIndex", _index);
params.put("left", _rect.x);
params.put("top", _rect.y);
params.put("width", _rect.width);
params.put("height", _rect.height);
params.put("screen_mode", _mode);
params.put("screen_rotate", _rotate);
data.put("params", params);
data.put("deps", _content);
return this.playXplay(data);
}
/*
* 视频或流媒体等 ...
* _start 该素材开始播放的时间精确到毫秒 (视频预加载问题:
* 提前500ms-1000ms发送指令xplay可以预加载视频)(也就是_start时间是12:00:00则在11:59:59把指令发送xplay)
* _id 该素材唯一标识符(调试使用)
* _index 该素材所使用的层(0-999)(层数越小越靠前,一般1-9层作为保留层,显示一些提示或者LOGO,通知等信息使用 ...)
* _content 素材存储路径
* _rect 素材显示位置与尺寸(素材可以任意缩放拉伸,任意坐标显示)
* _mode 屏幕模式(横屏:landscape、竖屏:portrait)
* _rotate 旋转角度(横屏:0,180、竖屏:90,270)
*/
public boolean playVideo(long _start, String _id, int _index, String _content, Rect _rect, String _mode,
int _rotate) {
Map<String, Object> data = new HashMap<String, Object>();
Map<String, Object> params = new HashMap<String, Object>();
data.put("id", _id);
data.put("type", "play");
data.put("start", _start);
data.put("libName", "video");
params.put("zIndex", _index);
params.put("path", _content);
params.put("left", _rect.x);
params.put("top", _rect.y);
params.put("width", _rect.width);
params.put("height", _rect.height);
params.put("screen_mode", _mode);
params.put("screen_rotate", _rotate);
data.put("params", params);
return this.playXplay(data);
}
/*
* 图片,参数使用与视频相同
*/
public boolean playImage(long _start, String _id, int _index, String _content, Rect _rect, String _mode,
int _rotate) {
Map<String, Object> data = new HashMap<String, Object>();
Map<String, Object> params = new HashMap<String, Object>();
data.put("id", _id);
data.put("type", "play");
data.put("start", _start);
data.put("libName", "pic");
params.put("zIndex", _index);
params.put("path", _content);
params.put("left", _rect.x);
params.put("top", _rect.y);
params.put("width", _rect.width);
params.put("height", _rect.height);
params.put("screen_mode", _mode);
params.put("screen_rotate", _rotate);
data.put("params", params);
return this.playXplay(data);
}
/*
* 二维码 _content 内容为二维码中的内容
*/
public boolean playQRCode(long _start, String _id, int _index, String _content, Rect _rect, String _mode,
int _rotate) {
Map<String, Object> data = new HashMap<String, Object>();
Map<String, Object> params = new HashMap<String, Object>();
data.put("id", _id);
data.put("type", "play");
data.put("start", _start);
data.put("libName", "qrcode");
params.put("zIndex", _index);
params.put("content", _content);
params.put("left", _rect.x);
params.put("top", _rect.y);
params.put("width", _rect.width);
params.put("height", _rect.height);
params.put("screen_mode", _mode);
params.put("screen_rotate", _rotate);
data.put("params", params);
return this.playXplay(data);
}
/*
* GIF,参数使用与视频相同
*/
public boolean playGIF(long _start, String _id, int _index, String _content, Rect _rect, String _mode,
int _rotate) {
Map<String, Object> data = new HashMap<String, Object>();
Map<String, Object> params = new HashMap<String, Object>();
data.put("id", _id);
data.put("type", "play");
data.put("start", _start);
data.put("libName", "gif");
params.put("zIndex", _index);
params.put("path", _content);
params.put("left", _rect.x);
params.put("top", _rect.y);
params.put("width", _rect.width);
params.put("height", _rect.height);
params.put("screen_mode", _mode);
params.put("screen_rotate", _rotate);
data.put("params", params);
return this.playXplay(data);
}
/*
* 信息提示框 _content 提示框内容
* _toast_type 提示框类型(notice、success、warning、error)
* _duration 提示框显示时间(如果为0则永久显示)
*/
public boolean playToast(long _start, String _id, int _index, String _content, String _mode, int _rotate,
String _toast_type, int _duration) {
Map<String, Object> data = new HashMap<String, Object>();
Map<String, Object> params = new HashMap<String, Object>();
data.put("id", _id);
data.put("type", "play");
data.put("start", _start);
data.put("libName", "toast");
params.put("zIndex", _index);
params.put("screen_mode", _mode);
params.put("screen_rotate", _rotate);
params.put("content", _content);
params.put("toast_type", _toast_type);
params.put("duration", _duration);
data.put("params", params);
return this.playXplay(data);
}
/*
* 摄像头
* _camera_width 与 _camera_height 为摄像头采集画面分辨率,建议(1280x720)
*/
public boolean playCamera(long _start, String _id, int _index, String _content, Rect _rect, String _mode,
int _rotate, int _camera_width, int _camera_height) {
Map<String, Object> data = new HashMap<String, Object>();
Map<String, Object> params = new HashMap<String, Object>();
data.put("id", _id);
data.put("type", "play");
data.put("start", _start);
data.put("libName", "camera");
params.put("zIndex", _index);
params.put("device", _content);
params.put("camera_width", _camera_width);
params.put("camera_height", _camera_height);
params.put("left", _rect.x);
params.put("top", _rect.y);
params.put("width", _rect.width);
params.put("height", _rect.height);
params.put("screen_mode", _mode);
params.put("screen_rotate", _rotate);
data.put("params", params);

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7447
最新资源
- MATLAB代码:储能参与调峰调频联合优化模型 关键词:储能 调频 调峰 充放电优化 联合运行 仿真平台:MATLAB+CVX 平台 主要内容:代码主要做的是考虑储能同时参与调峰以及调频的联合调度
- 汇川PLC程序(梯形图)
- 老年公寓信息管理:构建安全舒适的居住环境
- orca多机器人防碰 MATLAB仿真源代码全套技术资料.zip
- chatexcel数据表.zip
- 行驶车辆状态估计,无迹卡尔曼滤波,扩展卡尔曼滤波(EKF UKF) 软件使用:Matlab Simulink 适用场景:采用扩展卡尔曼滤波和无迹卡尔曼滤波EKF UKF进行行驶车辆的车速,质心侧偏
- 椅子、杯子、笔记本电脑、人、手机检测23-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 永磁同步电机(PMSM)基于高阶滑模观测器(HSMO)的无位置传感器速度控制仿真
- 永磁直驱风机MPPT最大功率点跟踪Simulink仿真模型,采用占空比扰动观察法,调整PWM的占空比来调节发电机达到最佳工作点
- U8点击按钮打开生成凭证界面
- Bukkit-BETA1.8.1服务端核心
- IEEE9节点系统Simulink仿真 1.基础功能:基于Matlab simulink平台搭建IEEE9节点仿真模型,对电力系统进行潮流计算(与编程用牛拉法计算潮流结果一致) 2.拓展功能: 可在该
- 椅子人坦克检测24-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord数据集合集.rar
- WRF-Chem 人为排放清单的设计
- ESP8266、ESP32网页配网 支持中文SSID
- lmx2592频率源原理图和程序源码 20MHz-9.8GHz的低噪声锁相环频率源,最小频率步进1MHz,输出功率可调,stm32f103c8t6控制lmx2592一体化,按键操控输出频率和输出功
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


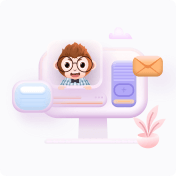