package com.thinkit.cms.service.content;
import cn.hutool.core.io.FileUtil;
import cn.hutool.json.JSONUtil;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.google.common.collect.Lists;
import com.thinkit.cms.api.category.CategoryModelRelationService;
import com.thinkit.cms.api.category.CategoryService;
import com.thinkit.cms.api.content.ContentAttachService;
import com.thinkit.cms.api.content.ContentAttrService;
import com.thinkit.cms.api.content.ContentRelatedService;
import com.thinkit.cms.api.content.ContentService;
import com.thinkit.cms.api.model.ModelService;
import com.thinkit.cms.api.site.SiteService;
import com.thinkit.cms.api.tag.TagService;
import com.thinkit.cms.config.ActuatorComponent;
import com.thinkit.cms.dto.category.CategoryDto;
import com.thinkit.cms.dto.content.*;
import com.thinkit.cms.dto.model.ModelDto;
import com.thinkit.cms.dto.tag.TagDto;
import com.thinkit.cms.entity.content.Content;
import com.thinkit.cms.mapper.content.ContentMapper;
import com.thinkit.cms.strategy.checker.ContentChecker;
import com.thinkit.cms.strategy.checker.PublishChecker;
import com.thinkit.core.base.BaseContextKit;
import com.thinkit.core.base.BaseServiceImpl;
import com.thinkit.core.constant.Channel;
import com.thinkit.core.constant.Constants;
import com.thinkit.core.handler.CustomException;
import com.thinkit.nosql.base.BaseRedisService;
import com.thinkit.nosql.base.BaseSolrService;
import com.thinkit.nosql.enums.SolrCoreEnum;
import com.thinkit.processor.channel.ChannelThreadLocal;
import com.thinkit.processor.message.MessageSend;
import com.thinkit.utils.enums.*;
import com.thinkit.utils.model.*;
import com.thinkit.utils.properties.ThinkItProperties;
import com.thinkit.utils.utils.Checker;
import com.thinkit.utils.utils.ModelFieldUtil;
import org.apache.commons.lang3.StringUtils;
import org.apache.solr.client.solrj.SolrServerException;
import org.apache.solr.common.SolrDocument;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Isolation;
import org.springframework.transaction.annotation.Transactional;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.*;
import java.util.concurrent.CompletableFuture;
/**
* <p>
* 内容 服务实现类
* </p>
*
* @author lg
* @since 2020-08-15
*/
@Service
public class ContentServiceImpl extends BaseServiceImpl<ContentDto, Content, ContentMapper> implements ContentService {
@Autowired
CategoryService categoryService;
@Autowired
ContentAttrService contentAttrService;
@Autowired
ContentAttachService attachService;
@Autowired
ModelService modelService;
@Autowired
MessageSend messageSend;
@Autowired
SiteService siteService;
@Autowired
CategoryModelRelationService modelRelationService;
@Autowired
ContentRelatedService relatedService;
@Autowired
TagService tagService;
@Autowired
BaseRedisService baseRedisService;
@Autowired
ActuatorComponent actuatorComponent;
@Autowired
BaseSolrService baseSolrService;
// @Autowired
// ArticleService articleService;
@Autowired
ThinkItProperties thinkItProperties;
@Override
public PageDto<ContentDto> listPage(PageDto<ContentDto> pageDto){
filterCategory(pageDto);
IPage<ContentDto> pages = new Page<>(pageDto.getPageNo(), pageDto.getPageSize());
IPage<ContentDto> result = baseMapper.listPage(pages, pageDto.getDto());
if(Checker.BeNotEmpty(result.getRecords()))filterCover(result.getRecords());
PageDto<ContentDto> resultSearch = new PageDto(result.getTotal(), result.getPages(), result.getCurrent(), Checker.BeNotEmpty(result.getRecords()) ? result.getRecords() : Lists.newArrayList());
return resultSearch;
}
@Override
public PageDto<ContentDto> pageRecycler(PageDto<ContentDto> pageDto) {
filterCategory(pageDto);
IPage<ContentDto> pages = new Page<>(pageDto.getPageNo(), pageDto.getPageSize());
IPage<ContentDto> result = baseMapper.pageRecycler(pages, pageDto.getDto());
if(Checker.BeNotEmpty(result.getRecords()))filterCover(result.getRecords());
PageDto<ContentDto> resultSearch = new PageDto(result.getTotal(), result.getPages(), result.getCurrent(), Checker.BeNotEmpty(result.getRecords()) ? result.getRecords() : Lists.newArrayList());
return resultSearch;
}
private void filterCover(List<ContentDto> contents){
for(ContentDto content:contents){
if(Checker.BeNotBlank(content.getCoverStr())){
content.setCover(JSONUtil.toList(JSONUtil.parseArray(content.getCoverStr()),Map.class));
}
}
}
private void filterCategory(PageDto<ContentDto> pageDto){
if (Checker.BeNotBlank(pageDto.getDto().getCategoryId())) {
List<String> childcategoryIds = new ArrayList<>();
childcategoryIds.add(pageDto.getDto().getCategoryId());
List<String> newChildCategoryIds = new ArrayList<>();
recursionCategoryIds(childcategoryIds, newChildCategoryIds);
pageDto.getDto().setCategoryIds(newChildCategoryIds);
}
}
private void recursionCategoryIds(List<String> childcategoryIds, List<String> newChildcategoryIds) {
if (Checker.BeNotEmpty(childcategoryIds)) {
newChildcategoryIds.addAll(childcategoryIds);
for (String categoryId : childcategoryIds) {
if (Checker.BeNotBlank(categoryId)) {
List<String> childCategs = getCategoryChildByParentId(categoryId);
recursionCategoryIds(childCategs, newChildcategoryIds);
}
}
}
}
private List<String> getCategoryChildByParentId(String categoryId) {
List<String> categoryIds = new ArrayList<>();
List<CategoryDto> cmsCategoryDtos = categoryService.listCategoryByPid(categoryId);
if (Checker.BeNotEmpty(cmsCategoryDtos)) {
for (CategoryDto cmsCategoryDto : cmsCategoryDtos) {
categoryIds.add(cmsCategoryDto.getId());
}
}
return categoryIds;
}
@Override
public IPage<ContentDto> pageContentForNoSql(Integer pageNo, Integer pageSize) {
IPage<Map<String,String>> pages = new Page<>(pageNo, pageSize);
IPage<ContentDto> result = baseMapper.pageContentForNoSql(pages, getSiteId());
if (Checker.BeNotEmpty(result.getRecords())) {
// 处理格式化
for(ContentDto contentDto:result.getRecords()){
if(Checker.BeNotBlank(contentDto.getAttrData())){
Map<String,Object> params = ModelFieldUtil.jsonStrToMap(contentDto.getAttrData());
contentDto.setParams(params);
}
if(Checker.BeNotBlank(contentDto.getCoverStr())){
contentDto.setCover(JSONUtil.toList(JSONUtil.parseArray(contentDto.getCoverStr()),Map.class));
}
}
return result;
}
return null;
}
@Override
public Tree<CategoryDto> treeCategory() {
return categoryService.treeCategoryForContent();
}
@Override
public List<DynamicModel> getFormDesign(String modelId) {
ModelDto model = modelService.getModel(modelId);
Boolean hasfile =model.getHasFiles();
List<DynamicModel> dynamicModels=ModelFieldUtil.jsonStrToModel(model.getAllFieldList());
if(hasfile){
List<DynamicModel> attachField = ModelFieldUtil.loadModel(BelongEnum.ATTACH);
if(Checker.BeNotEmpty(attachField)){
dynamicModels.addAll(attachField);
}
}
return dynamicModels;
}
@Transactional
@Override
public void save(ContentDto
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
ThinkItCMS 是一款面向模板开发,支持静态生成的CMS 系统,其支持前后端分离部署,是一款好用的 cms 系统。 ThinkItCMS 采用 SpringBoot + Mybatis Plus + Redis+ Spring Security + OAuth2 + Freemark 搭建的一套cms 系统,数据库采用 mysql 数据库,文件服务器采用 Fastdfs 全文检索采用 Solr 。 前端架构采用ant design vue 前后端分离的系统架构。 门户系统采用的 静态模板生成技术,直接生成的静态 html 模板,js + jQuery 作相应的辅助。 部署服务 采用 nginx 门户系统和 后台 管理系统 采用正向代理正常部署,服务端采用反向代理暴露接口。ThinkItCMS 的服务端在接口限制方面都可以灵活配置分配权限,保证系统的最大安全。无特殊要求亦可以内网部署服务端。ThinkItCMS 采用目前最流行的 JEE 架构 SpringBoot 开发的一个CMS 系统
资源推荐
资源详情
资源评论
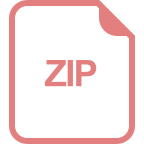
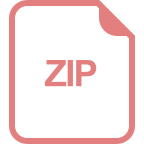
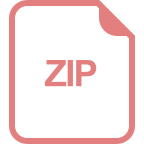
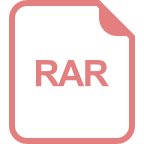
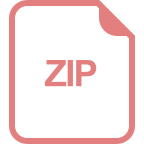
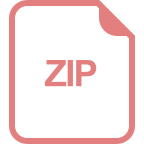
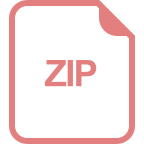
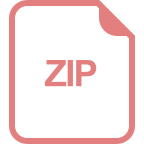
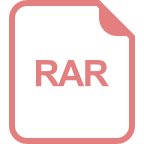
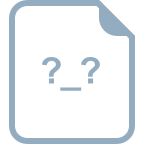
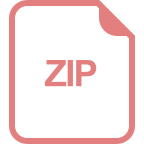
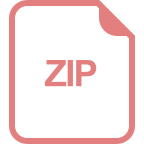
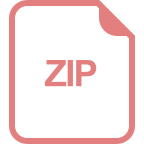
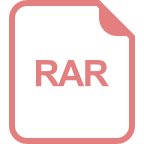
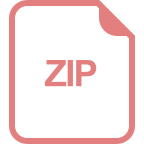
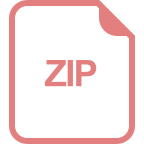
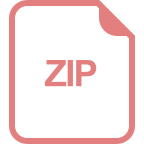
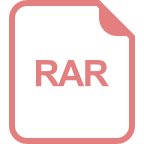
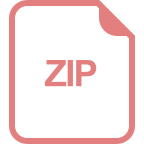
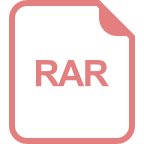
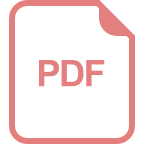
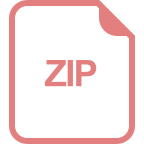
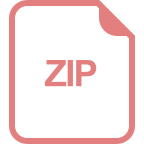
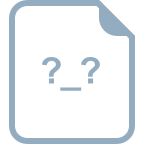
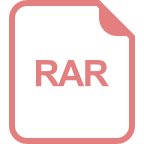
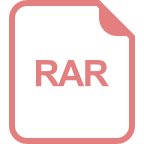
收起资源包目录

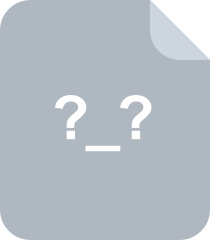
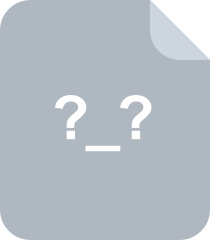
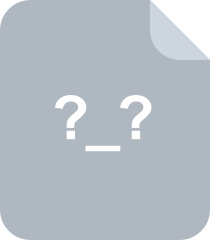
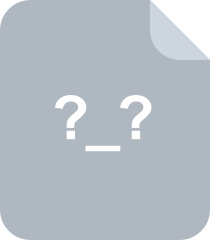
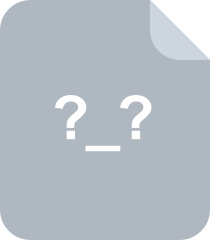
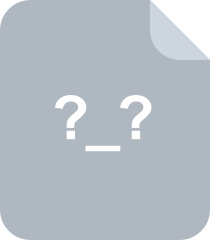
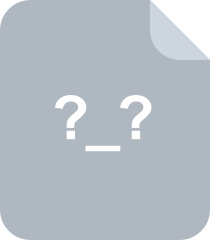
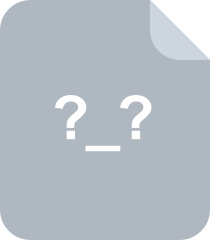
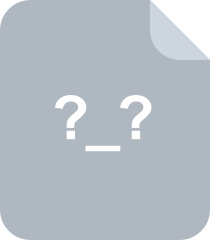
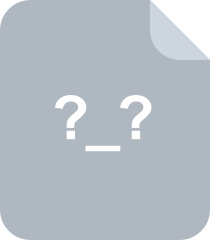
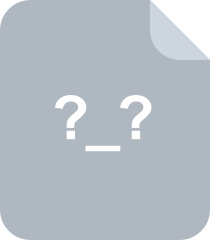
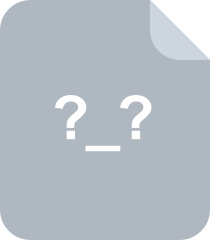
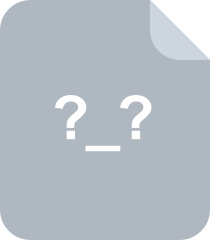
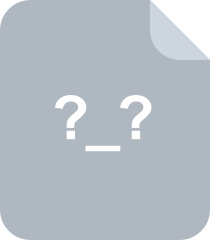
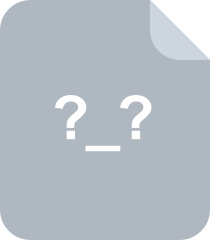
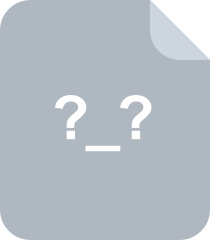
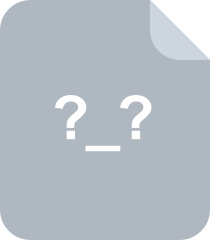
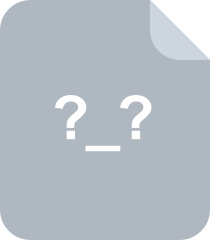
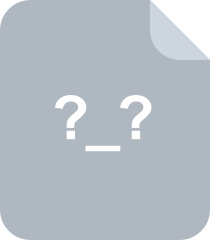
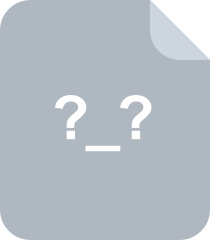
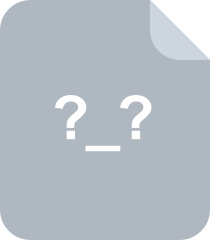
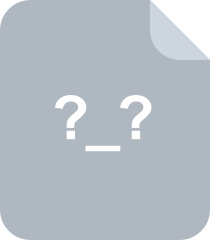
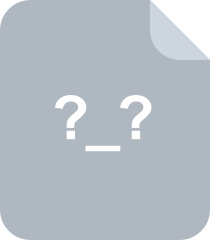
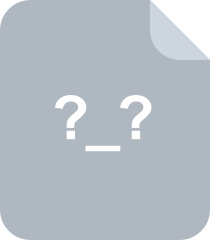
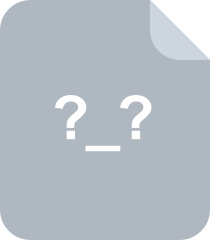
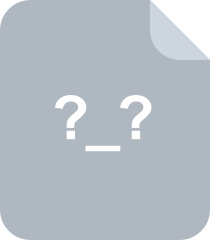
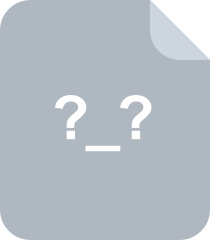
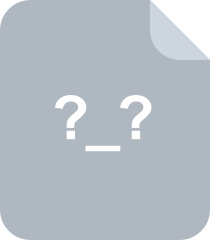
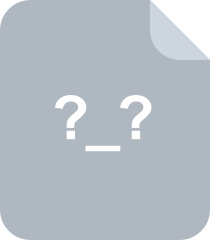
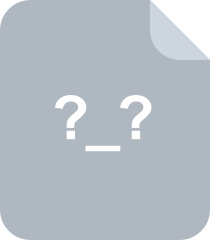
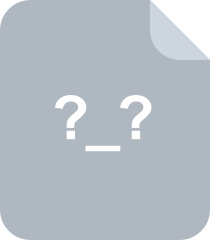
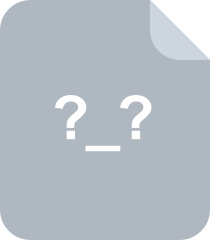
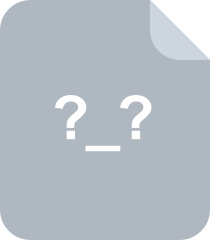
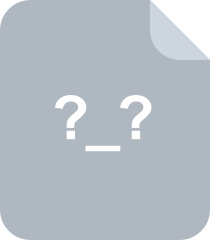
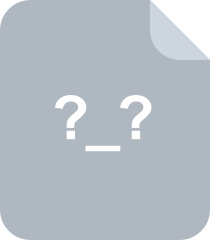
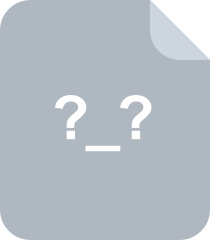
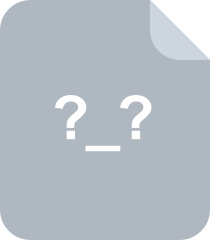
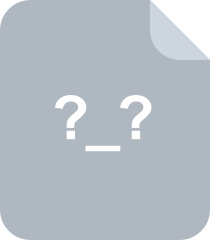
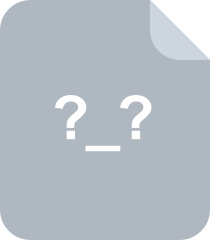
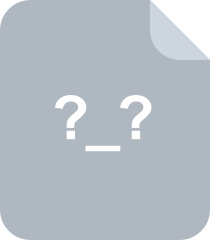
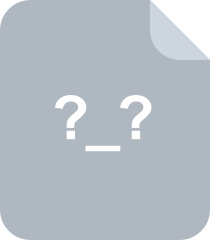
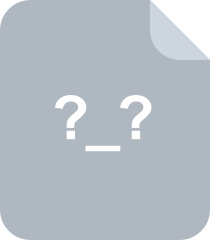
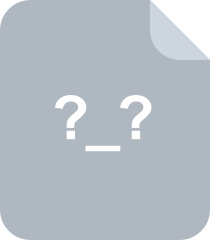
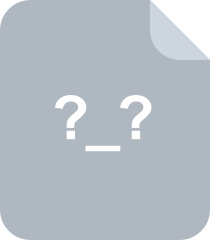
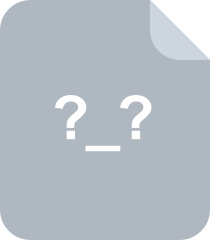
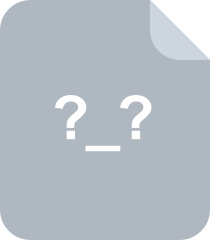
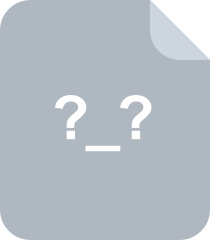
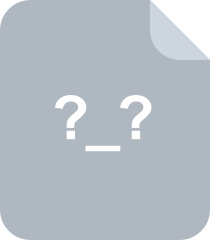
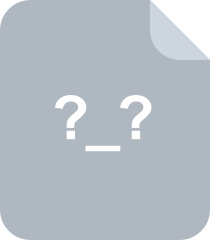
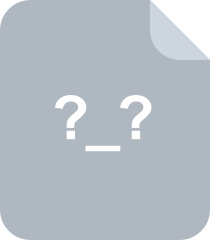
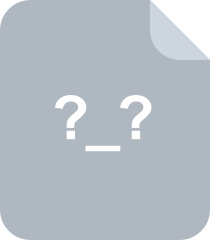
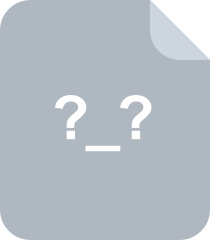
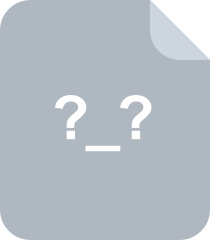
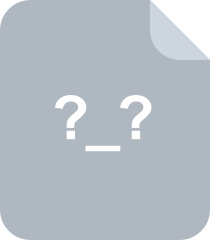
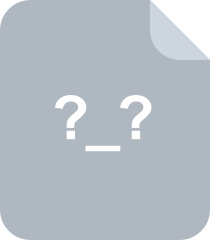
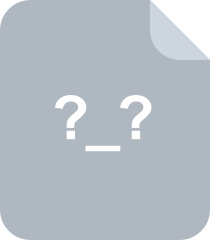
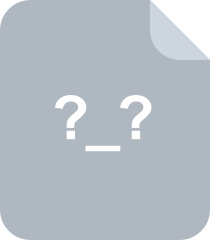
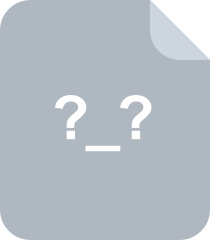
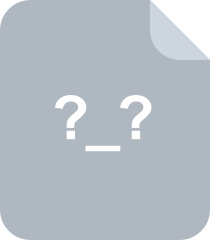
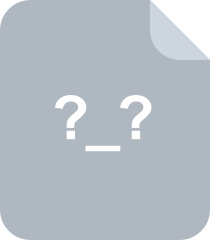
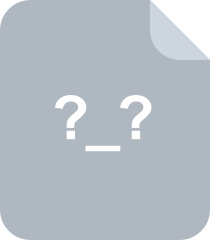
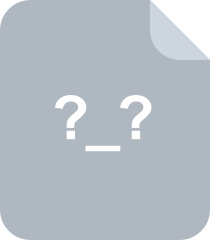
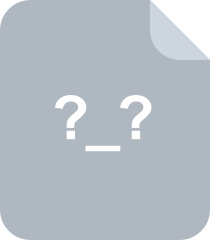
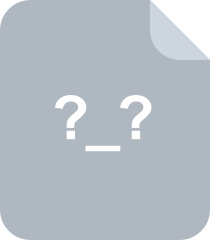
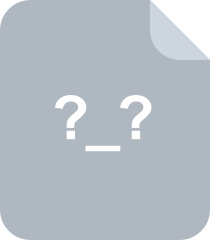
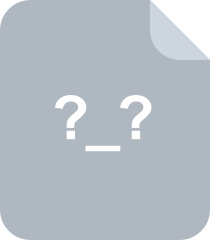
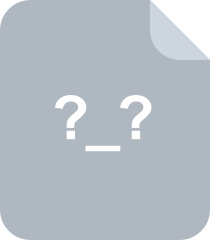
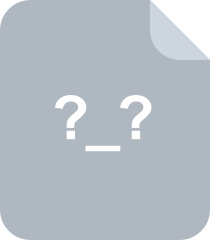
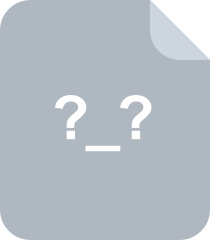
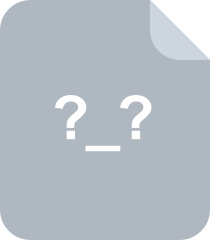
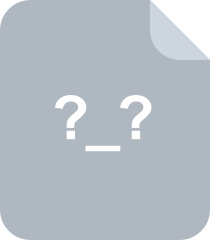
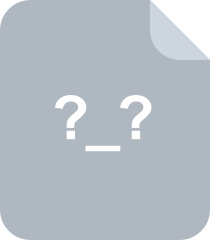
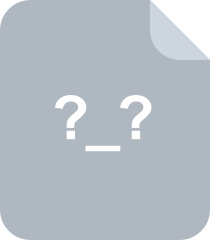
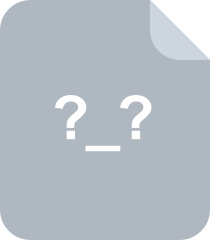
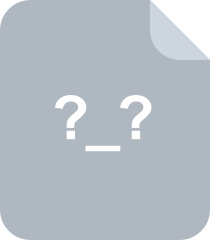
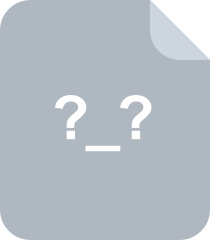
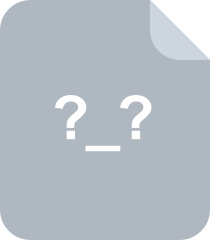
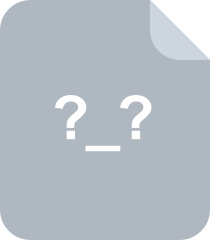
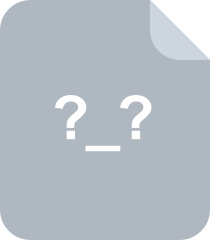
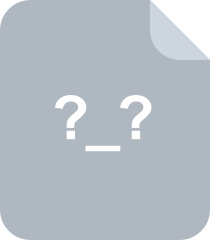
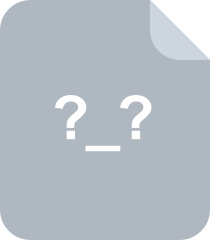
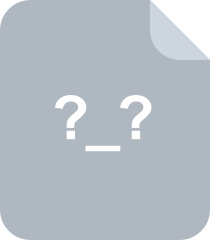
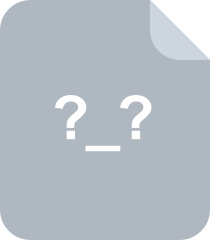
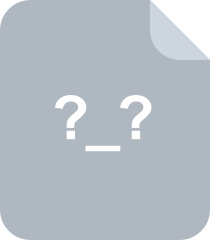
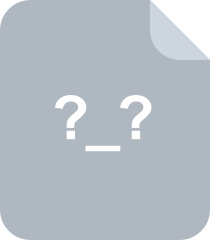
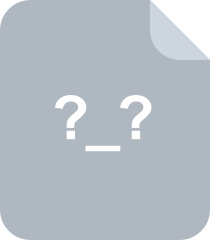
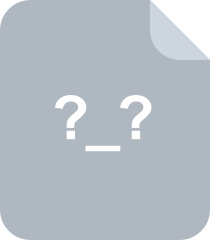
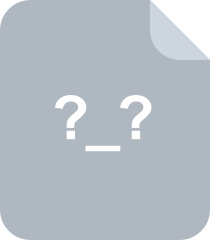
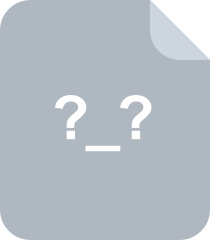
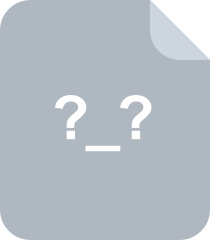
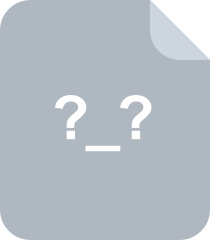
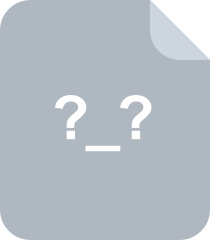
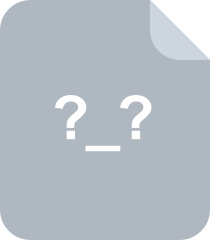
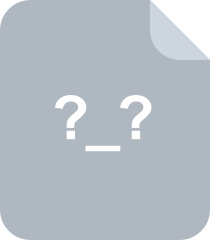
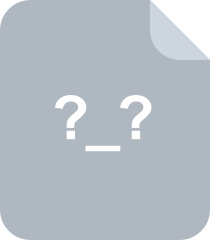
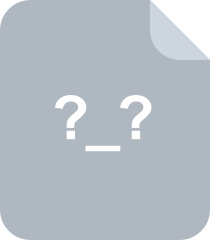
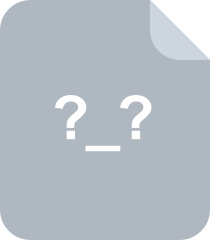
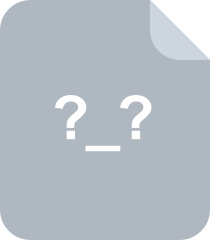
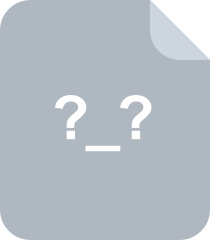
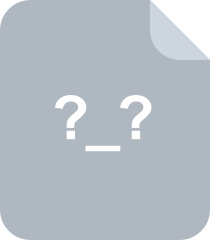
共 548 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
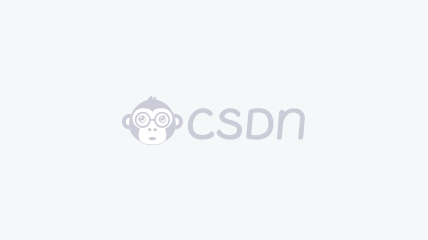

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7363
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

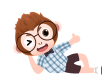
最新资源
- C#仿通达OA系统源码 网络智能办公协同系统源码数据库 SQL2008源码类型 WebForm
- Screenshot_20241118_214455.jpg
- Pi~1.39.0(94).apk
- flinksql专用资源,各种jar包
- CLShanYanSDKDataList.sqlite
- C#ASP.NET销售管理系统源码数据库 SQL2008源码类型 WebForm
- 1111232132132132
- 基于MAPPO算法与DL优化预编码的多用户MISO通信系统双时间尺度传输方案设计源码
- 基于微信拍照功能的ohos开源CameraView控件设计源码
- 基于JavaCV的RTSP转HTTP-FLV流媒体服务设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


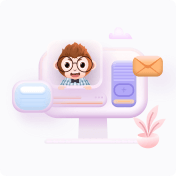
安全验证
文档复制为VIP权益,开通VIP直接复制
