package com.agileai.hr.module.salary.service;
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import org.springframework.dao.DataAccessException;
import com.agileai.common.KeyGenerator;
import com.agileai.domain.DataParam;
import com.agileai.domain.DataRow;
import com.agileai.hotweb.bizmoduler.core.StandardServiceImpl;
import com.agileai.hr.cxmodule.HrSalaryManage;
import com.agileai.util.DateUtil;
import com.agileai.util.ListUtil;
import com.agileai.util.MapUtil;
import com.agileai.util.StringUtil;
public class HrSalaryManageImpl extends StandardServiceImpl implements
HrSalaryManage {
public HrSalaryManageImpl() {
super();
}
private HashMap<String,List<DataRow>> queryRecords(String indexFieldName,String statementId,DataParam param) throws DataAccessException {
HashMap<String,List<DataRow>> result = new HashMap<String,List<DataRow>>();
List<DataRow> recordList = this.daoHelper.queryRecords(statementId, param);
HashMap<String, DataRow> recordMap = this.daoHelper.queryRecords(indexFieldName, statementId, param);
if (recordList != null && recordList.size() > 0){
int count = recordList.size();
Iterator<String> it = recordMap.keySet().iterator();
while (it.hasNext()) {
String indexField = it.next();
List<DataRow> list = new ArrayList<DataRow>();
for (int i = 0; i < count; i++) {
if (indexField.equals(String.valueOf(recordList.get(i).get(indexFieldName)))) {
list.add(recordList.get(i));
}
}
result.put(indexField, list);
}
}
return result;
}
@Override
public DataRow retrieveValidDays(String year, String month) {
DataParam param = new DataParam("year", year, "month", month);
String statementId = sqlNameSpace + "." + "retrieveValidDays";
DataRow row = this.daoHelper.getRecord(statementId, param);
return row;
}
@Override
public DataRow getInductionAndCreateTime(String userId){
DataParam param = new DataParam("userId",userId);
String statementId = sqlNameSpace + "." + "getUserAnnualLeaveDays";
DataRow row = this.daoHelper.getRecord(statementId, param);
return row;
}
public void createValidDayRecord(DataParam param) {
String statementId = sqlNameSpace + "." + "insertValidDaysRecord";
processDataType(param, tableName);
processPrimaryKeys(param);
this.daoHelper.insertRecord(statementId, param);
}
public void updateValidDayRecord(DataParam param) {
String statementId = sqlNameSpace + "." + "updateValidDaysRecord";
processDataType(param, tableName);
this.daoHelper.updateRecord(statementId, param);
}
@Override
public void gatherData(String year, String month) {
String statementId = "";
String yearMonth = year + "-" + month;
String yearMonthDay = yearMonth + "-01";
Date date = DateUtil.getDate(yearMonthDay);
String currentDate = yearMonth + "-31";
Date lastDateMonth = DateUtil.getDate(currentDate);
String beginOfYearDate = DateUtil.format(DateUtil.YYMMDD_HORIZONTAL, DateUtil.getBeginOfYear(date));
String beforeMonthDateStr = DateUtil.format(DateUtil.YYMMDD_HORIZONTAL, DateUtil.getDateAdd(date, DateUtil.MONTH, -1));
String beforeYearDecemberStr = DateUtil.format(DateUtil.YYMMDD_HORIZONTAL, DateUtil.getDateAdd(DateUtil.getBeginOfYear(date), DateUtil.MONTH, -1));
String previousMonth = beforeMonthDateStr.substring(5,7);
String beforeMonth = beforeMonthDateStr.substring(0,7);
String beforeYear = beforeYearDecemberStr.substring(0,4);
String beforeYearDecember = beforeYearDecemberStr.substring(5,7);
DataRow validDaysRow = this.retrieveValidDays(year, month);
DataRow beforeYearDecemberValidDaysRow = this.retrieveValidDays(beforeYear, beforeYearDecember);
statementId = sqlNameSpace + "." + "workDayRecords";
HashMap<String,DataRow> workDayMap = this.daoHelper.queryRecords(
"USER_ID", statementId, new DataParam("yearMonth",yearMonth));
HashMap<String,DataRow> probationWorkDayMap = this.daoHelper.queryRecords(
"USER_ID", statementId, new DataParam("sdate",date));
HashMap<String,DataRow> regularWorkDayMap = this.daoHelper.queryRecords(
"USER_ID", statementId, new DataParam("edate",currentDate));
statementId = sqlNameSpace + "." + "overTimeDayRecords";
HashMap<String,DataRow> totalOverTimeDaysMap = this.daoHelper.queryRecords(
"USER_ID", statementId, new DataParam("yearMonth",yearMonth));
statementId = sqlNameSpace + "." + "leaveDayRecords";
HashMap<String,DataRow> totalLeaveDaysMap = this.daoHelper.queryRecords(
"USER_ID", statementId, new DataParam("yearMonth",yearMonth));
HashMap<String,DataRow> currentYearLeaveDaysMap = this.daoHelper.queryRecords(
"USER_ID", statementId, new DataParam("sdate",beginOfYearDate,"edate",currentDate));
statementId = sqlNameSpace + "." + "currentMonthLeaveDayRecords";
HashMap<String,List<DataRow>> currentMonthLeaveDayRecordsMap = queryRecords(
"USER_ID", statementId, new DataParam("yearMonth",yearMonth));
HashMap<String,List<DataRow>> totalLeaveRecordsMap = queryRecords(
"USER_ID",statementId, new DataParam("year",beforeYear));
statementId = sqlNameSpace + "." + "findOverTimeRecords";
HashMap<String,List<DataRow>> beforeMonthOverTimeRecordsMap = queryRecords(
"USER_ID", statementId, new DataParam("yearMonth",beforeMonth));
HashMap<String,List<DataRow>> currentMonthOverTimeRecordsMap = queryRecords(
"USER_ID", statementId, new DataParam("yearMonth",yearMonth));
HashMap<String,List<DataRow>> totalOverTimeRecordsMap = queryRecords(
"USER_ID", statementId, new DataParam("year",beforeYear));
statementId = sqlNameSpace + "." + "findOffsetVacationDayRecords";
HashMap<String,DataRow> beforeYearDecemberOffsetVationDaysMap = this.daoHelper.queryRecords(
"SAL_USER", statementId, new DataParam("year",beforeYear,"month",beforeYearDecember));
HashMap<String,DataRow> beforeMonthOffsetVationDaysMap = this.daoHelper.queryRecords(
"SAL_USER", statementId, new DataParam("year",year,"month",previousMonth));
statementId = sqlNameSpace + "." + "findMasterDaysRecords";
List<DataRow> basicRecords = this.daoHelper.queryRecords(statementId,
new DataParam("year",year,"month",month));
statementId = sqlNameSpace + "." + "findPunishmentRecords";
HashMap<String,DataRow> punishmentMap = this.daoHelper.queryRecords(
"USER_ID",statementId, new DataParam("yearMonth",yearMonth));
statementId = sqlNameSpace + "." + "findRewardtRecords";
HashMap<String,DataRow> rewardMap = this.daoHelper.queryRecords(
"USER_ID",statementId, new DataParam("yearMonth",yearMonth));
statementId = sqlNameSpace + "." + "findAdditionalVationRecords";
HashMap<String,DataRow> additionalVationMap = this.daoHelper.queryRecords(
"USER_ID",statementId, new DataParam("yearMonth",yearMonth,"date",date));
statementId = sqlNameSpace+ "." + "findRecords";
HashMap<String,DataRow> salaryRecordMap = this.daoHelper.queryRecords(
"SAL_USER", statementId, new DataParam("salYear",year,"salMonth",month));
HashMap<String,DataRow> beforeYearDecemberSalaryRecords = this.daoHelper.queryRecords(
"SAL_USER",statementId,new DataParam("salYear",beforeYear,"salMonth",beforeYearDecember));
statementId = sqlNameSpace+ "." + "findBonusPenaltyRecords";
HashMap<String,DataRow> overRunDayRecordMap = this.daoHelper.queryRecords(
"USER_ID", statementId, new DataParam("BP_DATE",date,"BP_TYPE","OVERRUN"));
HashMap<String,DataRow> fullTimeRecordMap = this.daoHelper.queryRecords(
"USER_ID", statementId, new DataParam("BP_DATE",date,"BP_TYPE","FULLTIMEAWARD"));
HashMap<String,DataRow> additionalVationRecordMap = this.daoHelper.queryRecords(
"USER_ID", statementId, new DataParam("BP_DATE",date,"BP_TYPE","ADDITIONALVATION"));
statementId = sqlNameSpace+ "." + "
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
AEAI HR人力资源管理系统是数通畅联软件基于AEAI DP开发的开源Java Web系统,用来协助管理公司人力、薪酬等事务, AEAI HR人力资源系统包括一些核心的人力资源管理业务功能,如:薪酬管理、考勤管理、绩效管理等模块,同时支持移动签到、签退(移动支持是V1.5版本新特性),能够满足企业人力资源信息化的基本要求。 AEAI HR人力资源系统内部已预置演示账户,用于快速了解、掌握该系统。AEAIHR系统功能架构包括基本信息模块,考勤模块,加班申请,请假申请,薪资管理和微信签到签退。
资源推荐
资源详情
资源评论
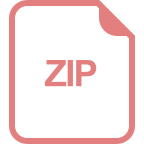
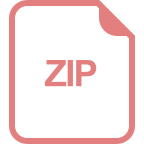
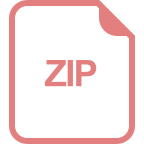
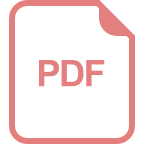
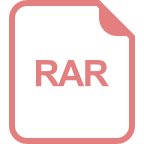
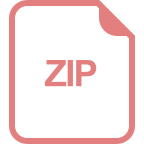
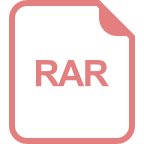
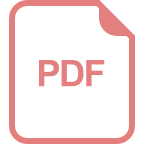
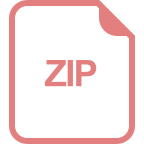
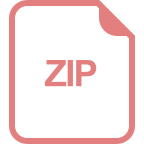
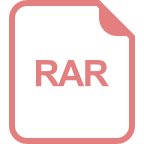
收起资源包目录

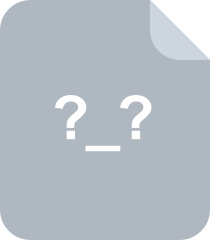
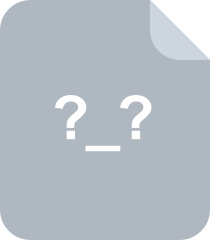
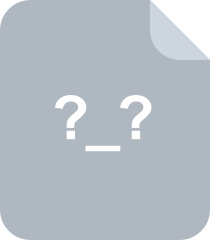
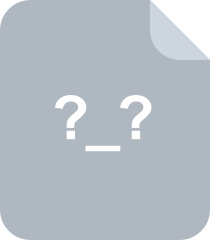
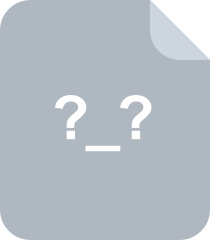
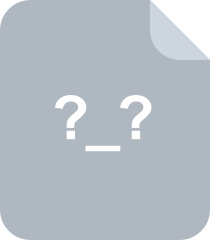
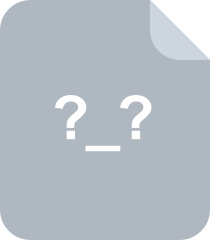
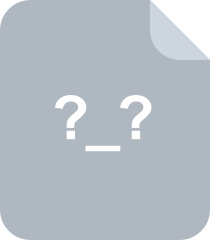
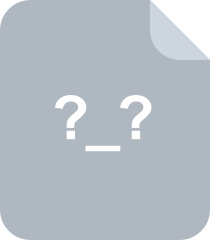
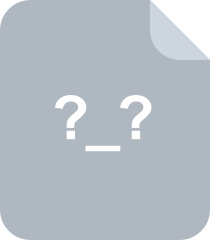
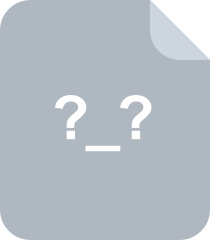
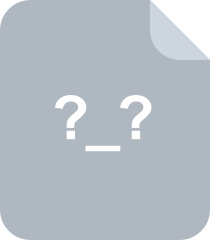
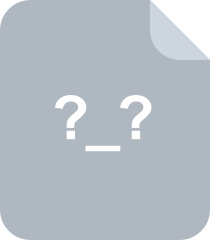
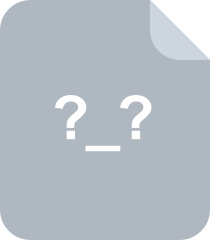
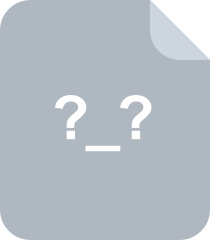
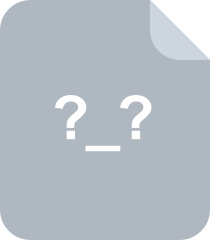
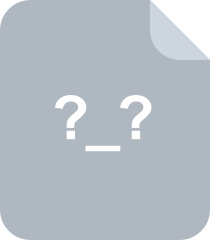
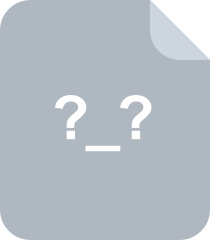
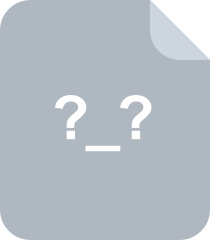
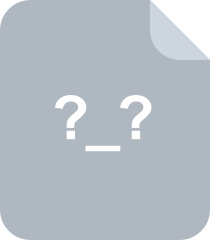
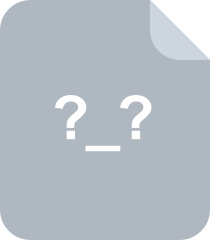
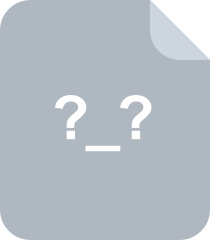
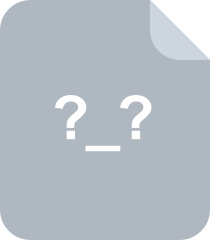
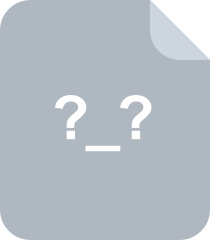
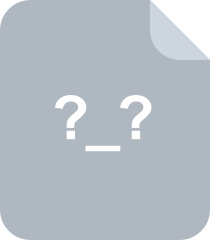
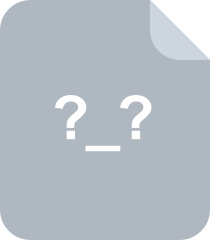
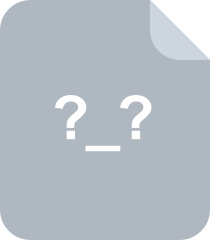
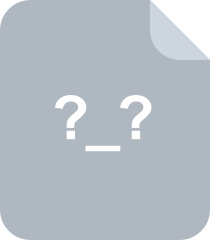
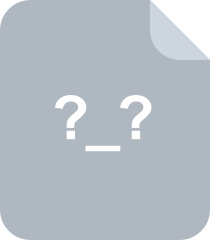
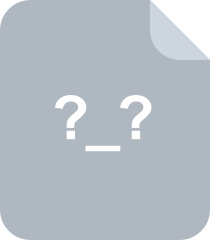
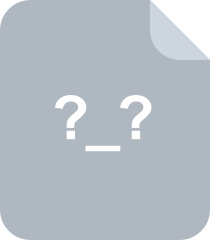
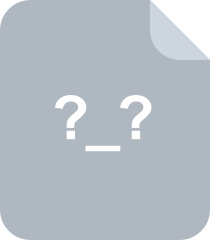
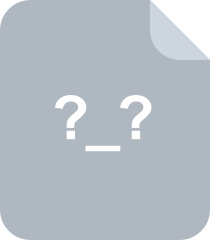
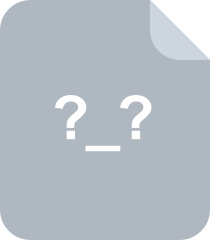
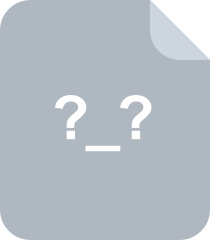
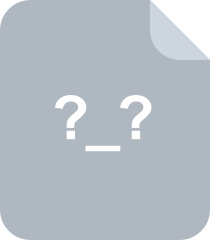
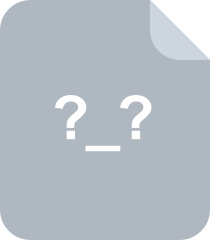
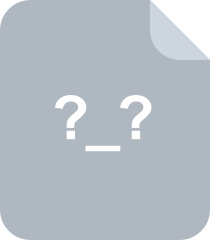
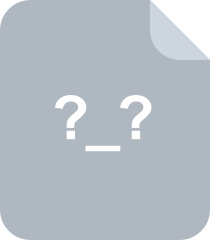
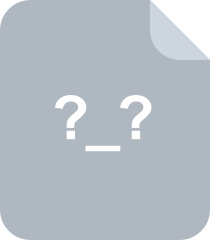
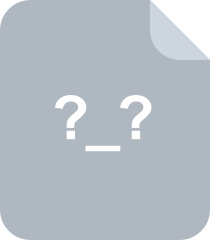
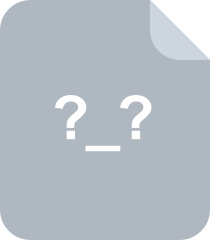
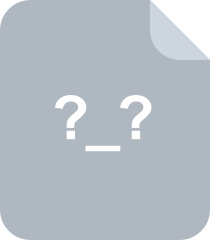
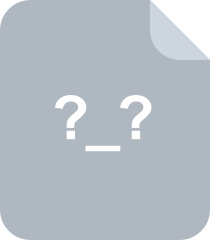
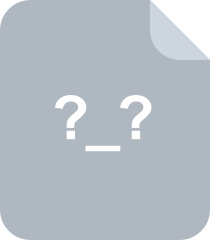
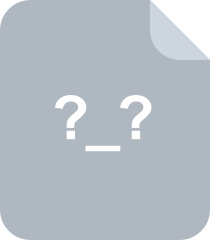
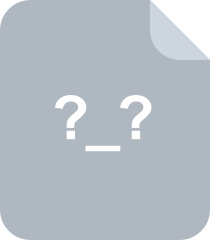
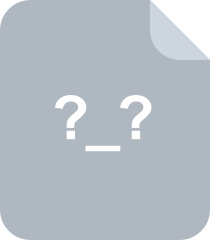
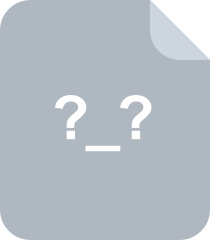
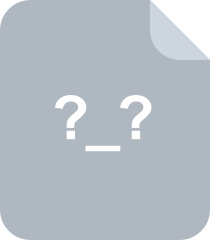
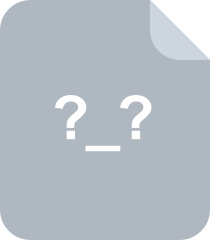
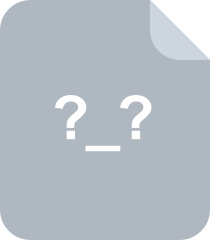
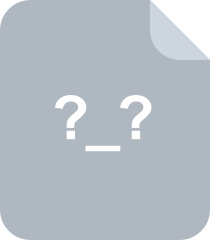
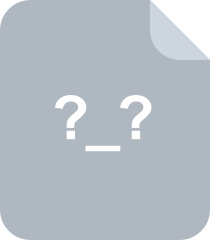
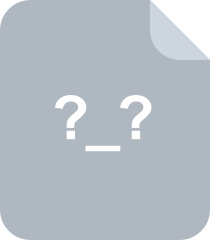
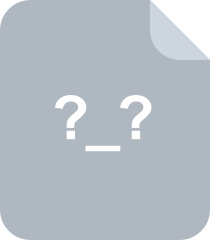
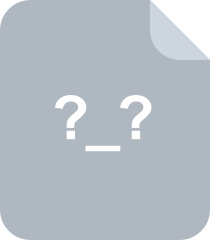
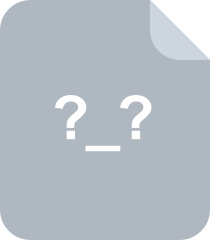
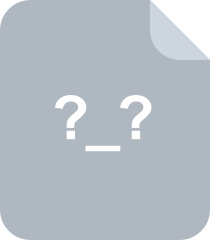
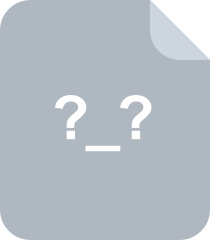
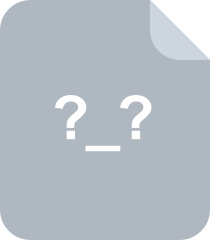
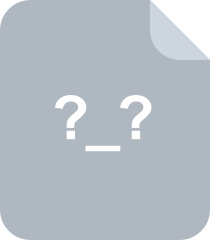
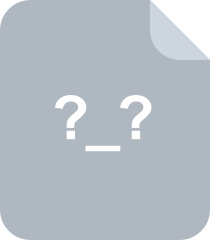
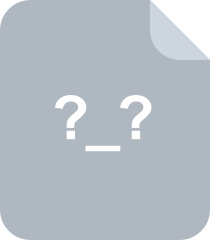
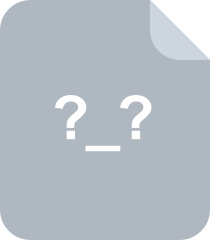
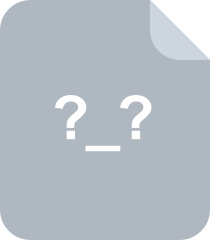
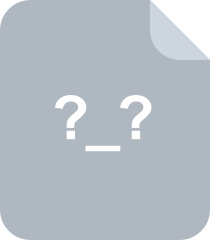
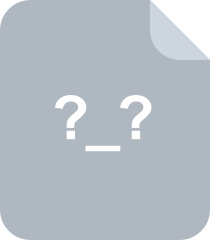
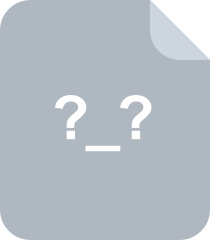
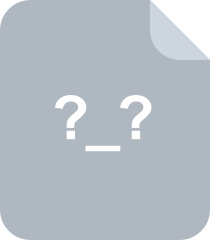
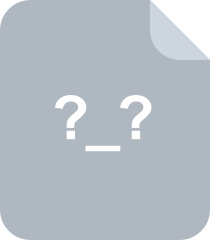
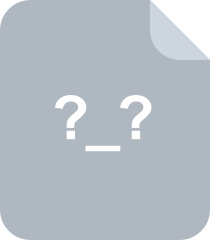
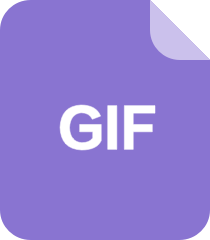
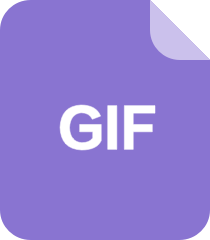
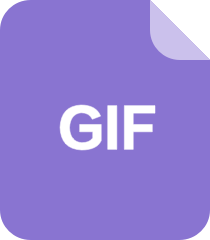
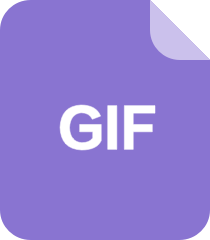
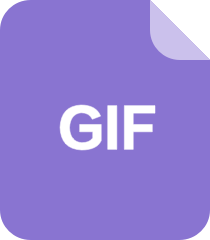
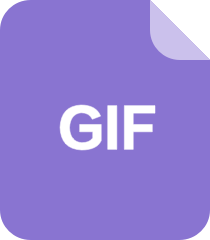
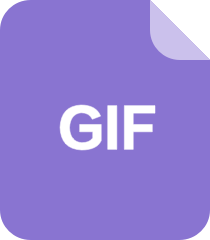
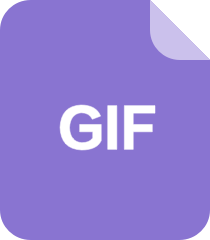
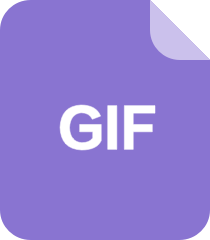
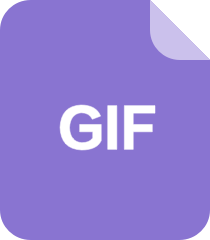
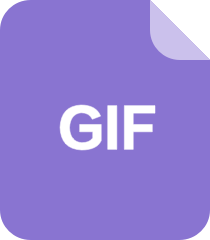
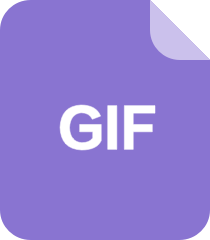
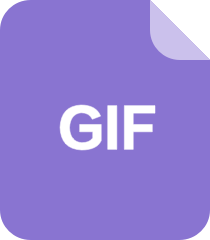
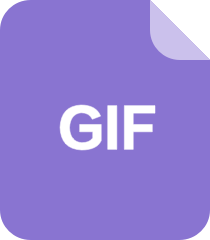
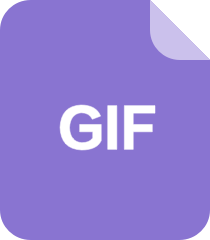
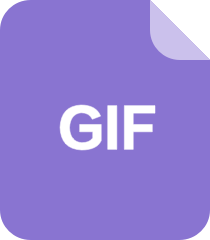
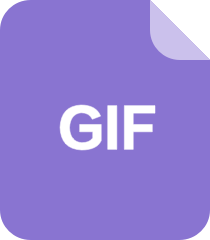
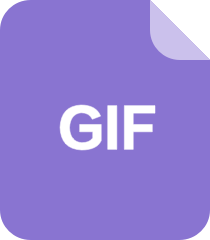
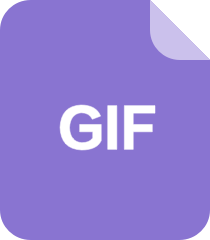
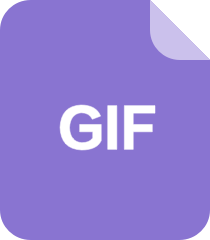
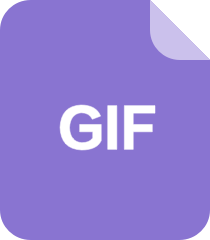
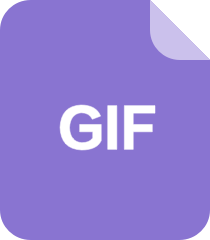
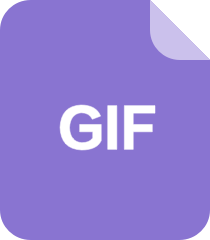
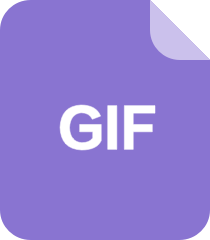
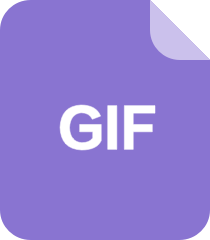
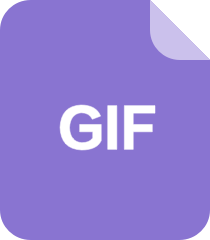
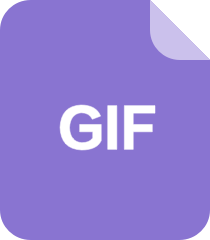
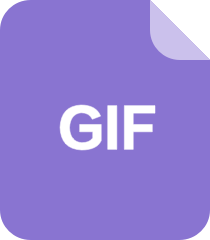
共 866 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
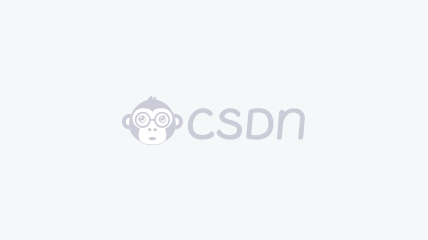

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7361
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

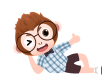
最新资源
- (源码)基于PythonSpleeter的戏曲音频处理系统.zip
- (源码)基于Spring Boot的监控与日志管理系统.zip
- (源码)基于C++的Unix V6++二级文件系统.zip
- (源码)基于Spring Boot和JPA的皮皮虾图片收集系统.zip
- (源码)基于Arduino和Python的实时歌曲信息液晶显示屏展示系统.zip
- (源码)基于C++和C混合模式的操作系统开发项目.zip
- (源码)基于Arduino的全球天气监控系统.zip
- OpenCVForUnity2.6.0.unitypackage
- (源码)基于SimPy和贝叶斯优化的流程仿真系统.zip
- (源码)基于Java Web的个人信息管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


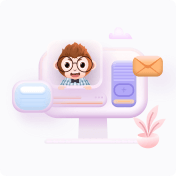
安全验证
文档复制为VIP权益,开通VIP直接复制
