/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dromara.cloudeon.controller;
import cn.hutool.core.bean.BeanUtil;
import cn.hutool.core.collection.ListUtil;
import cn.hutool.core.lang.Dict;
import cn.hutool.core.thread.ThreadUtil;
import cn.hutool.core.util.StrUtil;
import com.alibaba.fastjson.JSONObject;
import com.google.common.collect.Lists;
import freemarker.cache.StringTemplateLoader;
import freemarker.template.Configuration;
import freemarker.template.Template;
import freemarker.template.TemplateException;
import io.vertx.core.Vertx;
import lombok.extern.slf4j.Slf4j;
import org.dromara.cloudeon.config.CloudeonConfigProp;
import org.dromara.cloudeon.controller.request.InitServiceRequest;
import org.dromara.cloudeon.controller.request.ServiceConfUpgradeRequest;
import org.dromara.cloudeon.controller.response.*;
import org.dromara.cloudeon.dao.*;
import org.dromara.cloudeon.dto.*;
import org.dromara.cloudeon.entity.*;
import org.dromara.cloudeon.enums.*;
import org.dromara.cloudeon.processor.TaskParam;
import org.dromara.cloudeon.service.CommandHandler;
import org.dromara.cloudeon.utils.DAG;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.web.bind.annotation.*;
import javax.annotation.Resource;
import javax.persistence.EntityManager;
import java.io.File;
import java.io.IOException;
import java.io.StringWriter;
import java.io.Writer;
import java.util.*;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import static org.dromara.cloudeon.utils.Constant.*;
/**
* 集群服务相关接口
*/
@RestController
@RequestMapping("/service")
@Slf4j
public class ClusterServiceController {
ExecutorService flowSchedulerThreadPool = ThreadUtil.newExecutor(5, 10, 1024);
@Resource
private CloudeonConfigProp cloudeonConfigProp;
@Resource(name = "cloudeonVertx")
private Vertx cloudeonVertx;
@Resource
private AlertMessageRepository alertMessageRepository;
@Resource
private ServiceInstanceRepository serviceInstanceRepository;
@Resource
private ServiceRoleInstanceRepository roleInstanceRepository;
@Resource
private StackServiceRoleRepository stackServiceRoleRepository;
@Resource
private StackServiceRepository stackServiceRepository;
@Resource
private ServiceRoleInstanceWebuisRepository roleInstanceWebuisRepository;
@Resource
private ServiceInstanceConfigRepository serviceInstanceConfigRepository;
@Resource
private EntityManager entityManager;
@Resource
private CommandRepository commandRepository;
@Resource
private CommandTaskRepository commandTaskRepository;
@Resource
private CommandHandler commandHandler;
@Resource
private ClusterNodeRepository clusterNodeRepository;
@Resource
private StackServiceConfRepository stackServiceConfRepository;
@Resource
private ServiceInstanceSeqRepository serviceInstanceSeqRepository;
@PostMapping("/initService")
public ResultDTO<Void> initService(@RequestBody InitServiceRequest req) {
Integer clusterId = req.getClusterId();
Integer stackId = req.getStackId();
List<Integer> installedServiceInstanceIds = new ArrayList<>();
List<InitServiceRequest.ServiceInfo> serviceInfos = req.getServiceInfos();
// 校验该集群是否已经安装过相同的服务了
String errorServiceInstanceNames = serviceInfos.stream().map(info -> {
ServiceInstanceEntity sameStackServiceInstance = serviceInstanceRepository.findByClusterIdAndStackServiceId(clusterId, info.getStackServiceId());
if (sameStackServiceInstance != null) {
return sameStackServiceInstance.getServiceName();
}
return null;
}).filter(StrUtil::isNotBlank).collect(Collectors.joining(","));
if (StrUtil.isNotBlank(errorServiceInstanceNames)) {
return ResultDTO.failed("该集群已经安装过相同的服务实例:" + errorServiceInstanceNames);
}
// 根据服务间依赖关系调整安装顺序
List<InitServiceRequest.ServiceInfo> sortedServiceInfos = changeInstallSortByDependence(req);
for (InitServiceRequest.ServiceInfo serviceInfo : sortedServiceInfos) {
Integer stackServiceId = serviceInfo.getStackServiceId();
// 查询实例表获取新增的实例序号
ServiceInstanceSeqEntity serviceInstanceSeqEntity = serviceInstanceSeqRepository.findByStackServiceId(stackServiceId);
Integer maxInstanceSeq;
// 不存在该服务的序号,说明之前没安装过
if (serviceInstanceSeqEntity == null) {
maxInstanceSeq = 1;
serviceInstanceSeqEntity = ServiceInstanceSeqEntity.builder().maxSeq(maxInstanceSeq).stackServiceId(stackServiceId).build();
} else {
maxInstanceSeq = serviceInstanceSeqEntity.getMaxSeq() + 1;
serviceInstanceSeqEntity.setMaxSeq(maxInstanceSeq);
}
ServiceInstanceEntity serviceInstanceEntity = new ServiceInstanceEntity();
serviceInstanceEntity.setInstanceSequence(maxInstanceSeq);
String stackServiceName = serviceInfo.getStackServiceName().toLowerCase();
String serviceName = stackServiceName + maxInstanceSeq;
serviceInstanceEntity.setServiceName(serviceName);
serviceInstanceEntity.setLabel(serviceInfo.getStackServiceLabel());
serviceInstanceEntity.setClusterId(clusterId);
serviceInstanceEntity.setCreateTime(new Date());
serviceInstanceEntity.setUpdateTime(new Date());
serviceInstanceEntity.setEnableKerberos(req.getEnableKerberos());
serviceInstanceEntity.setStackServiceId(stackServiceId);
serviceInstanceEntity.setServiceState(ServiceState.INIT_SERVICE);
// 生成持久化宿主机路径
String persistencePaths = stackServiceRepository.findById(stackServiceId).get().getPersistencePaths();
serviceInstanceEntity.setPersistencePaths(genPersistencePaths(persistencePaths, serviceInstanceEntity));
// 持久化service信息
serviceInstanceRepository.save(serviceInstanceEntity);
// 持久化service instance 序号
serviceInstanceSeqRepository.save(serviceInstanceSeqEntity);
// 获取持久化后的service 实例id
Integer serviceInstanceEntityId = serviceInstanceEntity.getId();
installedServiceInstanceIds.add(serviceInstanceEntityId);
List<ServiceInstanceConfigEntity> serviceInstanceConfigEntities = new ArrayList<>();
// 页面上的预设配置
List<ServicePresetConf> presetConfList = serviceInfo.getPresetConfList();
// 用户自定义配置
List<ServiceCustomConf> customConfList = serviceInfo.getCustomConfList();
fillInstanceConfigEntities(stackId, stackServiceId, serviceInstanceEntityId, serviceInstanceConfigEntities,
没有合适的资源?快使用搜索试试~ 我知道了~
CloudEon云原生大数据平台,构建于kubernetes集群之上的大数据集群管理平台
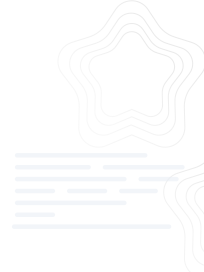
共904个文件
java:194个
ftl:147个
png:124个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 26 浏览量
2023-06-13
11:30:43
上传
评论
收藏 11.01MB ZIP 举报
温馨提示
CloudEon是一款基于kubernetes的开源大数据平台,旨在为用户提供一种简单、高效、可扩展的大数据解决方案。该平台致力于简化多种大数据服务在kubernetes上的部署和管理,如Hadoop、Doris、Spark、Flink、Hive、Kafka等,能够满足不同规模和业务需求下的大数据处理和分析需求。
资源推荐
资源详情
资源评论
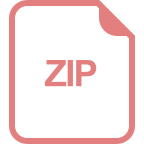
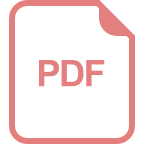
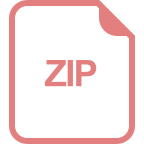
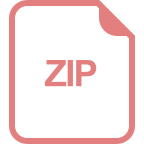
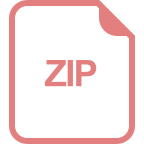
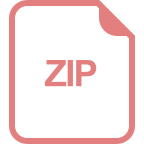
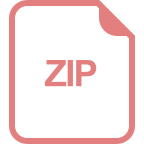
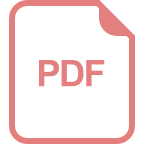
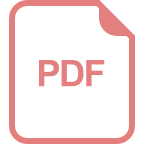
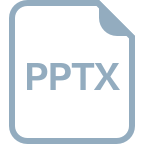
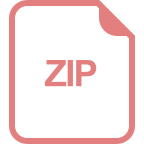
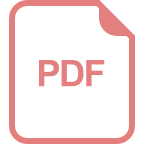
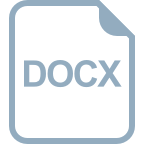
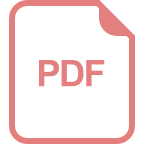
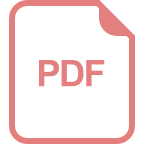
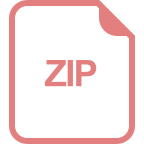
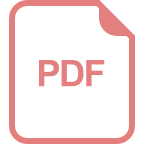
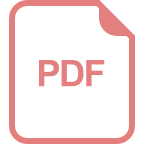
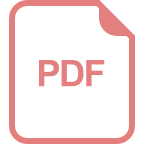
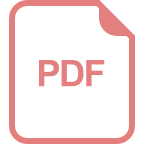
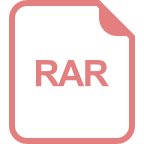
收起资源包目录

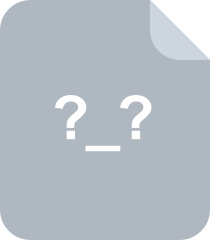
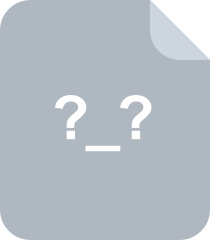
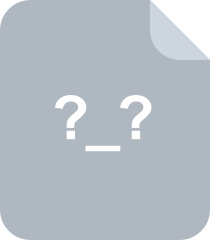
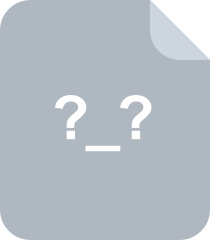
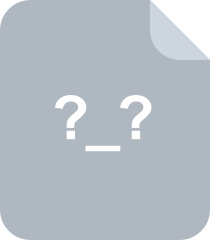
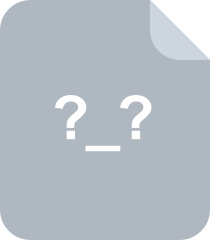
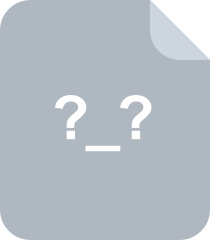
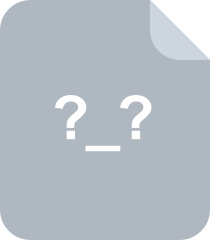
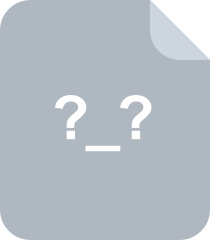
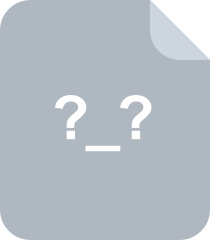
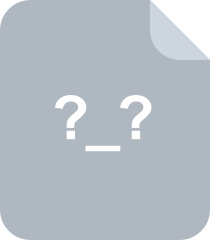
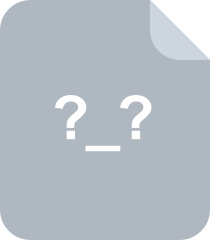
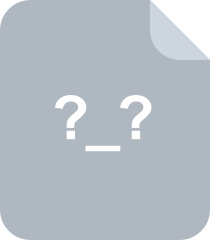
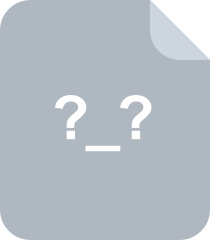
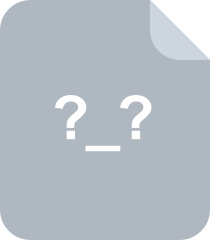
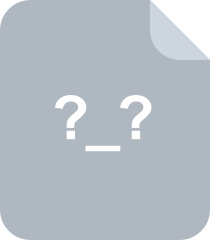
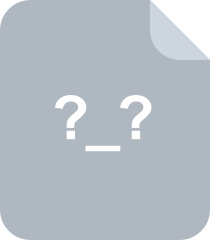
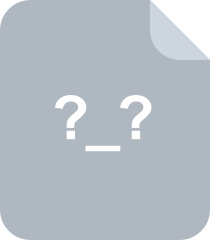
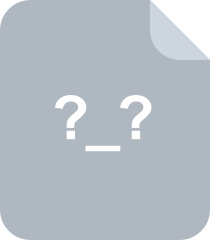
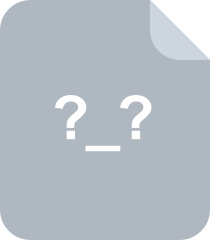
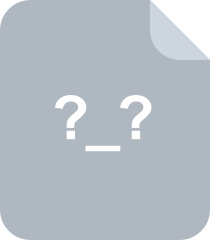
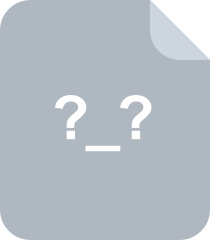
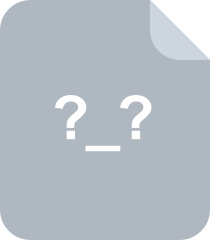
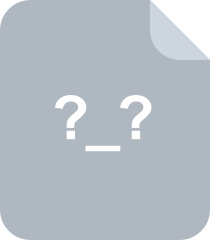
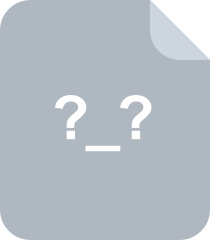
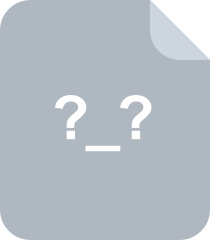
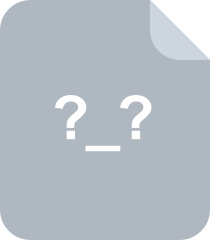
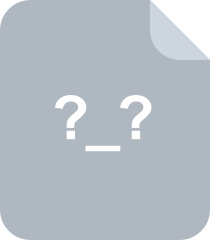
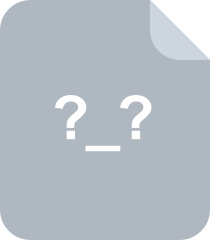
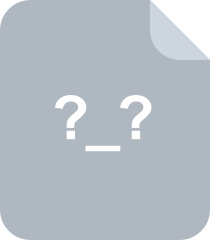
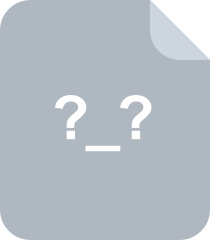
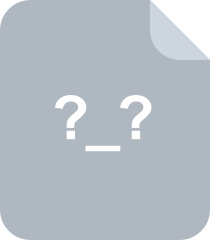
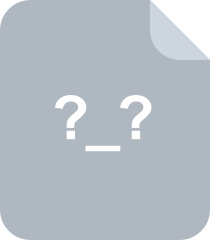
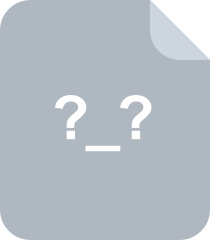
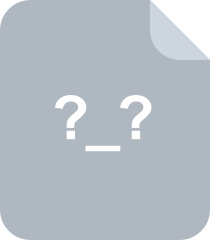
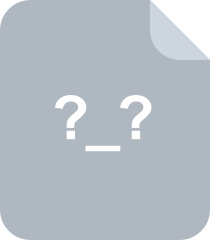
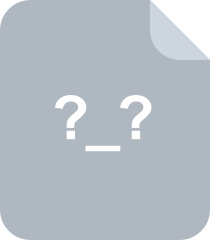
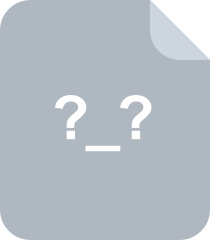
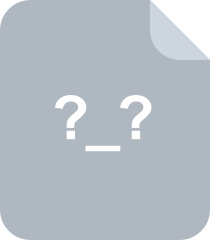
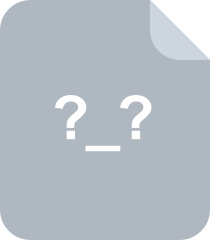
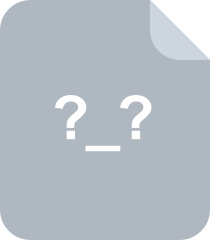
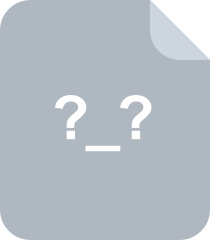
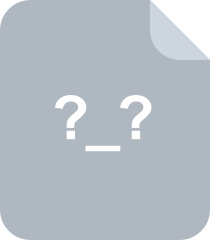
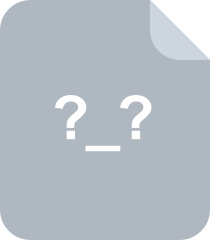
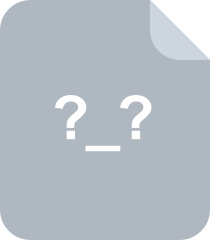
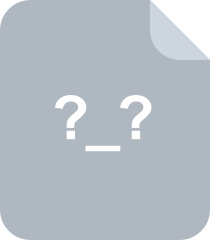
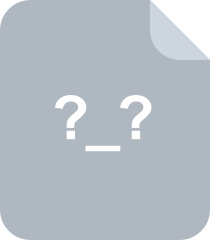
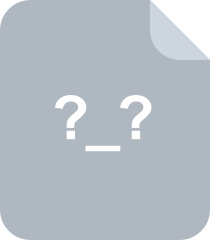
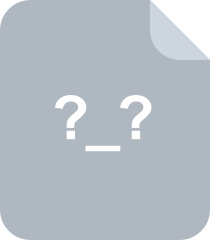
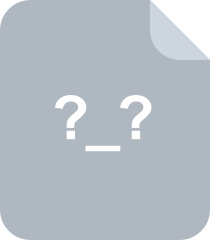
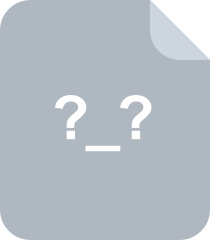
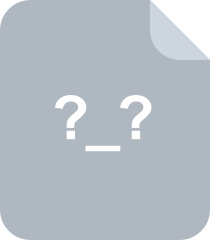
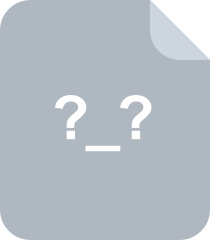
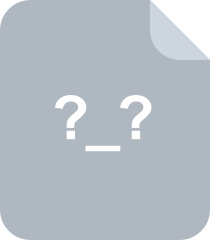
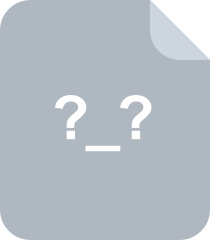
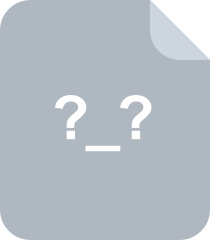
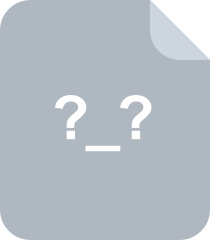
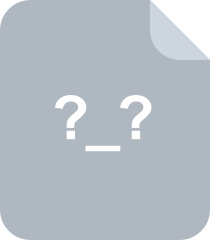
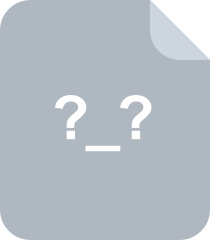
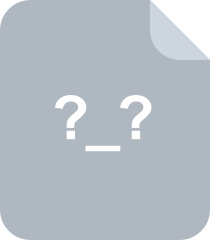
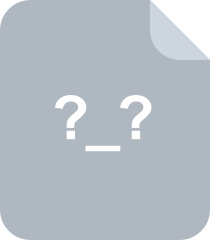
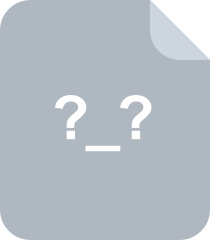
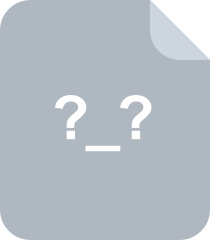
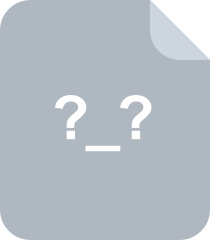
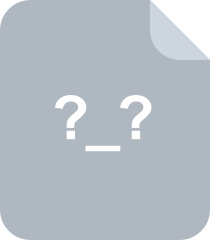
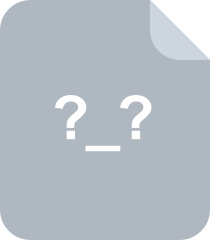
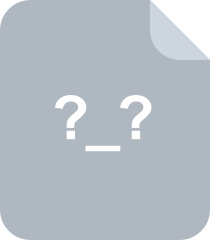
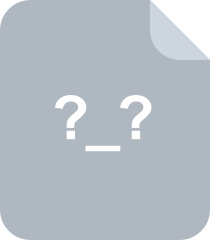
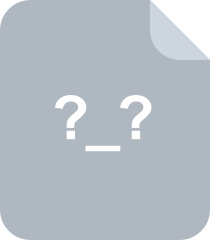
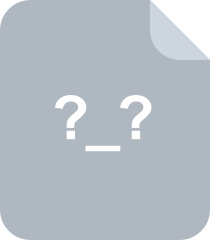
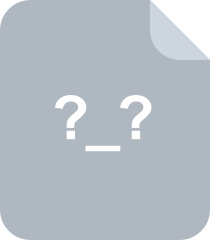
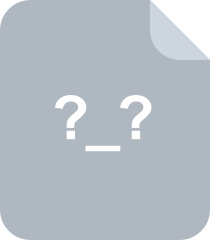
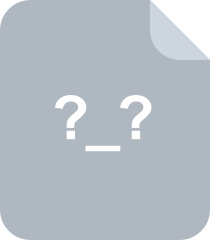
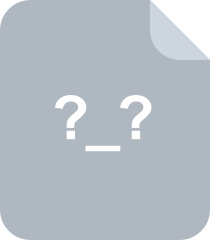
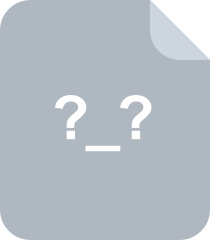
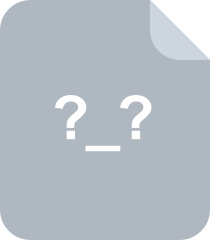
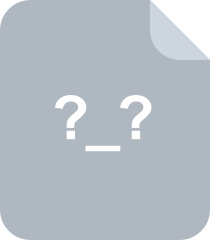
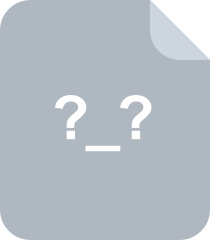
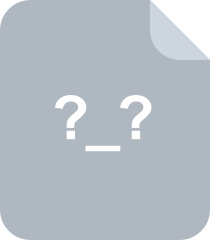
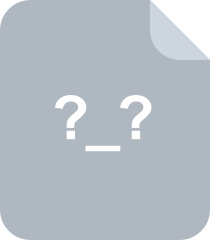
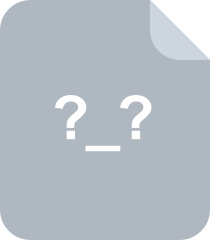
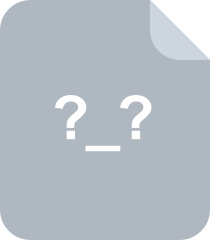
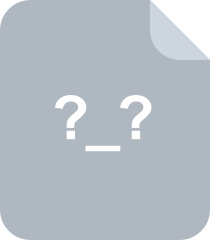
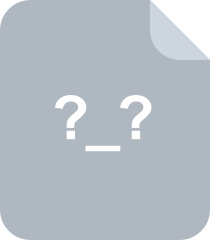
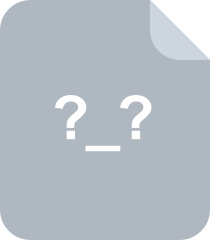
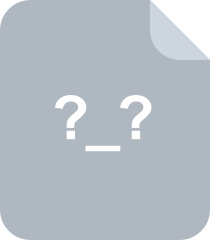
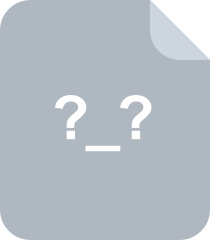
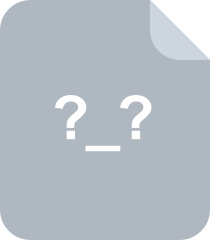
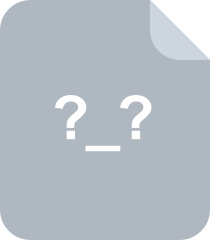
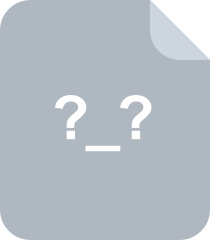
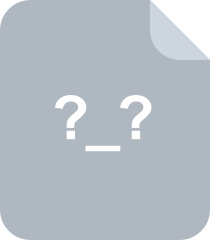
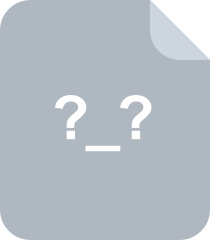
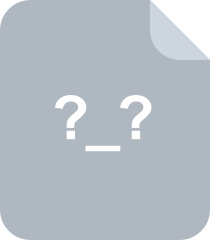
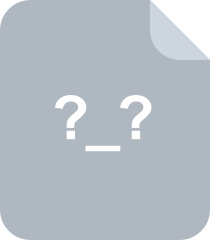
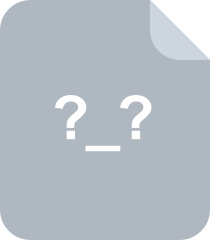
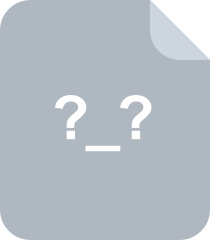
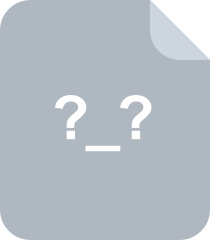
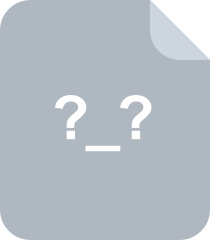
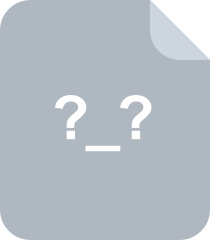
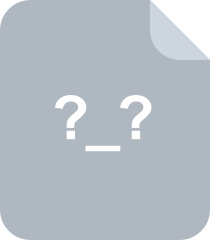
共 904 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
资源评论
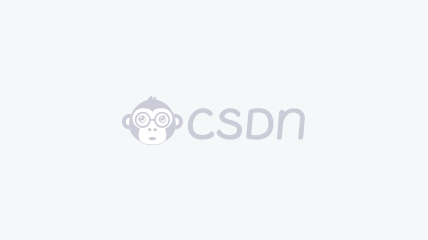

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7454
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

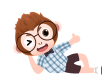
最新资源
- MATLAB实现EMD-iCHOA+GRU基于经验模态分解-改进黑猩猩算法优化门控循环单元的时间序列预测(含完整的程序和代码详解)
- christmasTree-圣诞树html网页代码
- LabVIEW-Version-Selector-labview
- awesome-ios-swift
- Servlet-servlet
- temperature-humidity-monitoring-system-labview
- javakeshe-java课程设计
- HormanyOs-notion鸿蒙版-鸿蒙
- Awesome-BUPT-Projects-自然语言处理课程设计
- JavaTest01-java课程设计
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


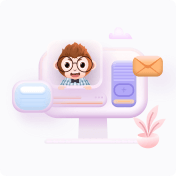
安全验证
文档复制为VIP权益,开通VIP直接复制
