package services
import (
"ThingsPanel-Go/initialize/psql"
"ThingsPanel-Go/initialize/redis"
"ThingsPanel-Go/models"
cm "ThingsPanel-Go/modules/dataService/mqtt"
tphttp "ThingsPanel-Go/others/http"
"ThingsPanel-Go/utils"
uuid "ThingsPanel-Go/utils"
valid "ThingsPanel-Go/validate"
"encoding/json"
"errors"
"fmt"
"log"
"os"
"reflect"
"strings"
"time"
"github.com/spf13/cast"
"github.com/spf13/viper"
"github.com/beego/beego/v2/core/logs"
simplejson "github.com/bitly/go-simplejson"
"gorm.io/gorm"
)
type DeviceService struct {
}
// Token 获取设备token
func (*DeviceService) Token(id string) (*models.Device, int64) {
var device models.Device
result := psql.Mydb.Where("id = ?", id).First(&device)
if result.Error != nil {
logs.Error(result.Error.Error())
return nil, 0
}
return &device, result.RowsAffected
}
// GetSubDeviceCount 获取子设备数量
func (*DeviceService) GetSubDeviceCount(parentId string) (int64, error) {
var count int64
result := psql.Mydb.Model(models.Device{}).Where("parent_id = ?", parentId).Count(&count)
return count, result.Error
}
// GetDevicesByAssetID 获取设备列表
func (*DeviceService) GetDevicesByAssetID(asset_id string) ([]models.Device, int64) {
var devices []models.Device
var count int64
db := psql.Mydb.Model(&models.Device{}).Where("asset_id = ?", asset_id)
result := db.Find(&devices)
db.Count(&count)
if result.Error != nil {
if errors.Is(result.Error, gorm.ErrRecordNotFound) {
return devices, 0
}
logs.Error(result.Error.Error())
return nil, 0
}
if len(devices) == 0 {
devices = []models.Device{}
}
return devices, count
}
// GetDevicesByAssetID 获取设备列表(business_id string, device_id string, asset_id string, current int, pageSize int,device_type string)
func (*DeviceService) PageGetDevicesByAssetID(business_id string, asset_id string, device_id string, current int, pageSize int, device_type string, token string, name string) ([]map[string]interface{}, int64) {
sqlWhere := `select (with RECURSIVE ast as
(
(select aa.id,cast(aa.name as varchar(255)),aa.parent_id from asset aa where id=a.id)
union
(select tt.id,cast (kk.name||'/'||tt.name as varchar(255))as name ,kk.parent_id from ast tt inner join asset kk on kk.id = tt.parent_id )
)select name from ast where parent_id='0' limit 1)
as asset_name,b.id as business_id ,b."name" as business_name,d.d_id,d.location,a.id as asset_id ,d.id as device ,d."name" as device_name,
d."token" as device_token,d."type" as device_type,d.protocol as protocol ,(select ts from ts_kv_latest tkl where tkl.entity_id = d.id order by ts desc limit 1) as latest_ts
from device d left join asset a on d.asset_id = a.id left join business b on b.id = a.business_id where 1=1 `
sqlWhereCount := `select count(1) from device d left join asset a on d.asset_id = a.id left join business b on b.id = a.business_id where 1=1`
var values []interface{}
var where = ""
if business_id != "" {
values = append(values, business_id)
where += " and b.id = ?"
}
if asset_id != "" {
values = append(values, asset_id)
where += " and a.id = ?"
}
if device_id != "" {
values = append(values, device_id)
where += " and d.id = ?"
}
if device_type != "" {
values = append(values, device_type)
where += " and d.type = ?"
}
if token != "" {
values = append(values, token)
where += " and d.token = ?"
}
if name != "" {
values = append(values, fmt.Sprintf("%%%s%%", name))
where += " and d.name like ?"
}
sqlWhere += where
sqlWhereCount += where
var count int64
result := psql.Mydb.Raw(sqlWhereCount, values...).Count(&count)
if result.Error != nil {
//errors.Is(result.Error, gorm.ErrRecordNotFound)
logs.Error(result.Error.Error())
}
var offset int = (current - 1) * pageSize
var limit int = pageSize
sqlWhere += " offset ? limit ?"
values = append(values, offset, limit)
var deviceList []map[string]interface{}
dataResult := psql.Mydb.Raw(sqlWhere, values...).Scan(&deviceList)
if dataResult.Error != nil {
//errors.Is(dataResult.Error, gorm.ErrRecordNotFound)
logs.Error(dataResult.Error.Error())
}
return deviceList, count
}
// GetDevicesByAssetID 获取设备列表(business_id string, device_id string, asset_id string, current int, pageSize int,device_type string)
func (*DeviceService) PageGetDevicesByAssetIDTree(req valid.DevicePageListValidate) ([]map[string]interface{}, int64) {
sqlWhere := `select (with RECURSIVE ast as
(
(select aa.id,cast(aa.name as varchar(255)),aa.parent_id from asset aa where id=a.id)
union
(select tt.id,cast (kk.name||'/'||tt.name as varchar(255))as name ,kk.parent_id from ast tt inner join asset kk on kk.id = tt.parent_id )
)select name from ast where parent_id='0' limit 1)
as asset_name,b.id as business_id ,b."name" as business_name,d.d_id,d.location,a.id as asset_id ,d.id as device ,d."name" as device_name,d.device_type as device_type,d.parent_id as parent_id,d.protocol_config as protocol_config,
d.additional_info as additional_info,d.sub_device_addr as sub_device_addr,d."token" as device_token,d."type" as "type",d.protocol as protocol ,dm.model_name as plugin_name,(select ts from ts_kv_latest tkl where tkl.entity_id = d.id order by ts desc limit 1) as latest_ts
from device d left join asset a on d.asset_id = a.id left join business b on b.id = a.business_id LEFT JOIN device_model dm ON d.type = dm.id where 1=1 and d.device_type != '3'`
sqlWhereCount := `select count(1) from device d left join asset a on d.asset_id = a.id left join business b on b.id = a.business_id where 1=1 and d.device_type != '3'`
var values []interface{}
var where = ""
if req.BusinessId != "" {
values = append(values, req.BusinessId)
where += " and b.id = ?"
}
if req.AssetId != "" {
values = append(values, req.AssetId)
where += " and a.id = ?"
}
if req.DeviceId != "" {
values = append(values, req.DeviceId)
where += " and d.id = ?"
}
if req.DeviceType != "" {
values = append(values, req.DeviceType)
where += " and d.type = ?"
}
if req.Token != "" {
values = append(values, req.Token)
where += " and d.token = ?"
}
if req.Name != "" {
values = append(values, fmt.Sprintf("%%%s%%", req.Name))
where += " and d.name like ?"
}
sqlWhere += where
sqlWhereCount += where
var count int64
result := psql.Mydb.Raw(sqlWhereCount, values...).Count(&count)
if result.Error != nil {
//errors.Is(result.Error, gorm.ErrRecordNotFound)
logs.Error(result.Error.Error())
}
var offset int = (req.CurrentPage - 1) * req.PerPage
var limit int = req.PerPage
sqlWhere += "order by d.created_at desc offset ? limit ?"
values = append(values, offset, limit)
var deviceList []map[string]interface{}
dataResult := psql.Mydb.Raw(sqlWhere, values...).Scan(&deviceList)
if dataResult.Error != nil {
logs.Error(dataResult.Error.Error())
} else {
for _, device := range deviceList {
if device["type"].(string) != "" {
var deviceModelService DeviceModelService
chartNameList, err := deviceModelService.GetChartNameListByPluginId(device["type"].(string))
if err == nil {
device["chart_names"] = chartNameList
} else {
logs.Error(err.Error())
}
}
//在线离线状态
// if device["device_type"].(string) != "3" {
// var interval int64
// if a, ok := device["additional_info"].(string); ok {
// aJson, err := simplejson.NewJson([]byte(a))
// if err == nil {
// thresholdTime, err := aJson.Get("runningInfo").Get("thresholdTime").Int64()
// if err == nil {
// interval = thresholdTime
// }
// }
// }
// var TSKVService TSKVService
// state, err := TSKVService.DeviceOnline(device["device"].(string), interval)
// if err != nil {
// logs.Error(err.Error())
// }
// device["device_state"] = state
// }
if device["device_type"].(string) == "2" { // 网关设备需要查询子设备
var subDeviceList []map[string]interface{}
sql :=

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7527
最新资源
- 基于hadoop的百度云盘源代码(亲测可用完整项目代码)
- CTF比赛工具合集-多种竞赛场景源码.zip
- 基于深度学习4j价格预测与语义分析源码+实战项目.zip
- Django+MySQL新冠疫情数据可视化平台源码+设计报告.zip
- 基于Django自动化测试管理系统python源码+设计报告(高分项目).zip
- Django高考志愿填报智能推荐系统python源码+设计论文(2024年毕业设计).zip
- Docker和Kubernetes构建的服务管理系统设计与实现 + 设计报告(Java版).zip
- DQN柔性作业车间调度-带插单的动态调度问题(含源码+项目说明+设计报告).zip
- ESP32-CAM+MicroPython+Flask智能Web视频监控与目标检测系统 + 设计报告.zip
- ESP8266墨水屏开发板项目(C++源码+硬件PCB资料+开发手册).zip
- Fisco Bcos支持的NFT数字藏品网站-交易不可篡改且可追溯溯源(含源码及论文资料).zip
- Facenet深度学习人脸识别系统(含源码+项目说明+亚洲人脸数据集+设计报告).zip
- FISCOBCOS匿名投票系统源码+设计报告及全部资料(Paillier加密).zip
- FPGA加速交通标志识别-卷积神经网络实现(含源码+项目说明+硬件设计).zip
- Flask框架YOLOv5检测训练源码+快速搭建手册.zip
- GAILC2024无人机双光检测Rank6源码+项目报告文档.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


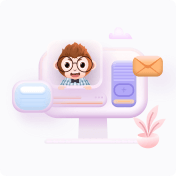