# Inputmask 3.x
Copyright (c) 2010 - 2017 Robin Herbots Licensed under the MIT license ([http://opensource.org/licenses/mit-license.php](http://opensource.org/licenses/mit-license.php))
[![NPM Version][npm-image]][npm-url] [![Dependency Status][david-image]][david-url] [![devDependency Status][david-dev-image]][david-dev-url]
Inputmask is a javascript library which creates an input mask. Inputmask can run against vanilla javascript, jQuery and jqlite.
An inputmask helps the user with the input by ensuring a predefined format. This can be useful for dates, numerics, phone numbers, ...
Highlights:
- easy to use
- optional parts anywere in the mask
- possibility to define aliases which hide complexity
- date / datetime masks
- numeric masks
- lots of callbacks
- non-greedy masks
- many features can be enabled/disabled/configured by options
- supports readonly/disabled/dir="rtl" attributes
- support data-inputmask attribute(s)
- alternator-mask
- regex-mask
- dynamic-mask
- preprocessing-mask
- JIT-masking
- value formatting / validating without input element
- AMD/CommonJS support
- dependencyLibs: vanilla javascript, jQuery, jqlite
- <strike>[Android support](README_android.md)</strike>
Demo page see [http://robinherbots.github.io/Inputmask](http://robinherbots.github.io/Inputmask)
[](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=ZNR3EB6JTMMSS)
## Setup
### dependencyLibs
Inputmask can run against different javascript libraries.
You can choose between:
- inputmask.dependencyLib (vanilla)
- inputmask.dependencyLib.jquery
- inputmask.dependencyLib.jqlite
- .... (others are welcome)
### Classic web with <script\> tag
Include the js-files which you can find in the `dist` folder.
If you want to include the Inputmask and all extensions. (with jQuery as dependencylib)
```html
<script src="jquery.js"></script>
<script src="dist/jquery.inputmask.bundle.js"></script>
<script src="dist/inputmask/phone-codes/phone.js"></script>
<script src="dist/inputmask/phone-codes/phone-be.js"></script>
<script src="dist/inputmask/phone-codes/phone-ru.js"></script>
```
For individual extensions. (with jQuery as dependencylib)
```html
<script src="jquery.js"></script>
<script src="dist/inputmask/inputmask.js"></script>
<script src="dist/inputmask/inputmask.extensions.js"></script>
<script src="dist/inputmask/inputmask.numeric.extensions.js"></script>
<script src="dist/inputmask/inputmask.date.extensions.js"></script>
<script src="dist/inputmask/inputmask.phone.extensions.js"></script>
<script src="dist/inputmask/jquery.inputmask.js"></script>
<script src="dist/inputmask/phone-codes/phone.js"></script>
<script src="dist/inputmask/phone-codes/phone-be.js"></script>
<script src="dist/inputmask/phone-codes/phone-ru.js"></script>
```
For individual extensions. (with vanilla dependencylib)
```html
<script src="dist/inputmask/dependencyLibs/inputmask.dependencyLib.js"></script>
<script src="dist/inputmask/inputmask.js"></script>
<script src="dist/inputmask/inputmask.extensions.js"></script>
<script src="dist/inputmask/inputmask.numeric.extensions.js"></script>
<script src="dist/inputmask/inputmask.date.extensions.js"></script>
<script src="dist/inputmask/inputmask.phone.extensions.js"></script>
<script src="dist/inputmask/phone-codes/phone.js"></script>
<script src="dist/inputmask/phone-codes/phone-be.js"></script>
<script src="dist/inputmask/phone-codes/phone-ru.js"></script>
```
If you like to automatically bind the inputmask to the inputs marked with the data-inputmask- ... attributes you may also want to include the inputmask.binding.js
```html
<script src="dist/inputmask/bindings/inputmask.binding.js"></script>
```
### webpack
#### Install the package
```
npm install inputmask --save-dev
```
#### In your modules
If you want to include the Inputmask and all extensions.
```
var Inputmask = require('inputmask');
//es6
import Inputmask from "inputmask";
```
For individual extensions.
Every extension exports the Inputmask, so you only need to import the extensions.
See example.
```
require("inputmask/dist/inputmask/inputmask.numeric.extensions");
var Inputmask = require("inputmask/dist/inputmask/inputmask.date.extensions");
//es6
import "inputmask/dist/inputmask/inputmask.numeric.extensions";
import Inputmask from "inputmask/dist/inputmask/inputmask.date.extensions";
```
#### Selecting the dependencyLib
By default the vanilla dependencyLib is used. You can select another dependency
by creating an alias in the webpack.config.
```
resolve: {
alias: {
"./dependencyLibs/inputmask.dependencyLib": "./dependencyLibs/inputmask.dependencyLib.jquery"
}
},
```
## Usage
### via Inputmask class
```javascript
var selector = document.getElementById("selector");
var im = new Inputmask("99-9999999");
im.mask(selector);
//or
Inputmask({"mask": "(999) 999-9999", .... other options .....}).mask(selector);
Inputmask("9-a{1,3}9{1,3}").mask(selector);
Inputmask("9", { repeat: 10 }).mask(selector);
```
### via jquery plugin
```javascript
$(document).ready(function(){
$(selector).inputmask("99-9999999"); //static mask
$(selector).inputmask({"mask": "(999) 999-9999"}); //specifying options
$(selector).inputmask("9-a{1,3}9{1,3}"); //mask with dynamic syntax
});
```
### via data-inputmask attribute
```html
<input data-inputmask="'alias': 'date'" />
<input data-inputmask="'mask': '9', 'repeat': 10, 'greedy' : false" />
<input data-inputmask="'mask': '99-9999999'" />
```
```javascript
$(document).ready(function(){
$(":input").inputmask();
or
Inputmask().mask(document.querySelectorAll("input"));
});
```
#### Any option can also be passed through the use of a data attribute. Use data-inputmask-<**_the name of the option_**>="value"
```html
<input id="example1" data-inputmask-clearmaskonlostfocus="false" />
<input id="example2" data-inputmask-regex="[a-za-zA-Z0-9!#$%&'*+/=?^_`{|}~-]+(?:\.[a-zA-Z0-9!#$%&'*+/=?^_`{|}~-]+)*@(?:[a-zA-Z0-9](?:[a-zA-Z0-9-]*[a-zA-Z0-9])?\.)+[a-zA-Z0-9](?:[a-zA-Z0-9-]*[a-zA-Z0-9])?" />
```
```javascript
$(document).ready(function(){
$("#example1").inputmask("99-9999999");
$("#example2").inputmask();
});
```
### Allowed HTML-elements
- `<input type="text">`
- `<input type="tel">`
- `<input type="password">`
- `<div contenteditable="true">` (and all others supported by contenteditable)
- `<textarea>`
- any html-element (mask text content or set maskedvalue with jQuery.val)
The allowed input types are defined in the supportsInputType option. Also see ([input-type-ref])
### Default masking definitions
- `9` : numeric
- `a` : alphabetical
- `*` : alphanumeric
There are more definitions defined within the extensions.<br>You can find info within the js-files or by further exploring the options.
## Masking types
### Static masks
These are the very basic of masking. The mask is defined and will not change during the input.
```javascript
$(document).ready(function(){
$(selector).inputmask("aa-9999"); //static mask
$(selector).inputmask({mask: "aa-9999"}); //static mask
});
```
### Optional masks
It is possible to define some parts in the mask as optional. This is done by using [ ].
Example:
```javascript
$('#test').inputmask('(99) 9999[9]-9999');
```
This mask wil allow input like `(99) 99999-9999` or `(99) 9999-9999`.
Input => 12123451234 mask => (12) 12345-1234 (trigger complete)<br>
Input => 121234-1234 mask => (12) 1234-1234 (trigger complete)<br>
Input => 1212341234 mask => (12) 12341-234_ (trigger incomplete)
#### skipOptionalPartCharacter
As an extra there is another configurable character which is used to skip an optional part in the mask.
```javascript
skipOptionalPartCharacter: " "
```
Input => 121234 1234 mask => (12) 1234-1234 (trigger complete)
When `clearMaskOnLostFocus: true` is set in the options (default), the mask will clear out the optiona
没有合适的资源?快使用搜索试试~ 我知道了~
Leadshop是一款提供持续更新迭代服务的轻量级、高性能开源电商系统,是一款提供持续更新迭代服务的企业级商用电商软件
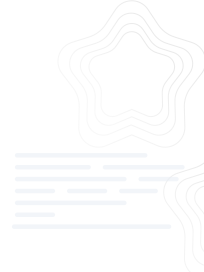
共2000个文件
php:4574个
js:480个
dat:360个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 131 浏览量
2023-05-09
11:18:47
上传
评论
收藏 46.4MB ZIP 举报
温馨提示
Leadshop是一款提供持续更新迭代服务的轻量级、高性能开源电商系统,是一款提供持续更新迭代服务的企业级商用电商软件;前后端分离(uniapp+yii2.0),可视化DIY拖拽装修,旨在打造极致的用户体验! 前端uni-app 、 ES6、vue、vuex、vue-router、vue-cli、axios、element-ui 后端Yii2、Jwt、Mysql、Easy-SMS
资源推荐
资源详情
资源评论
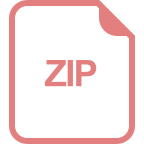
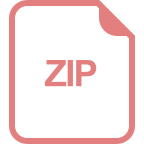
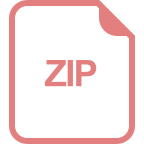
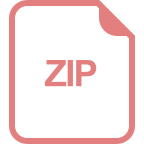
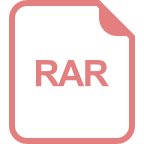
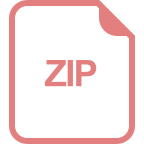
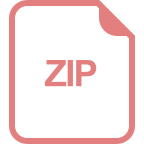
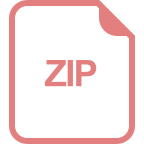
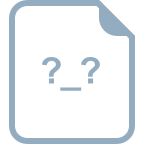
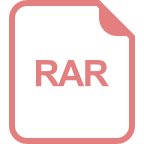
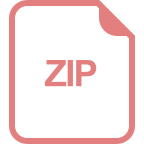
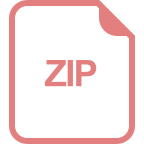
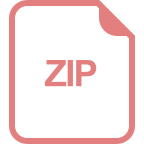
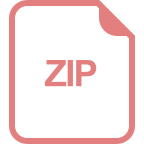
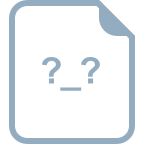
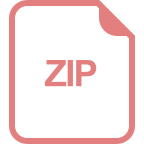
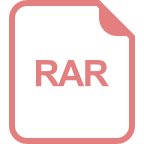
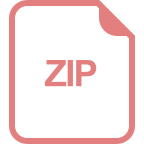
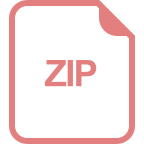
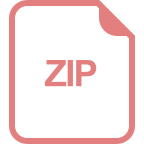
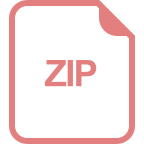
收起资源包目录

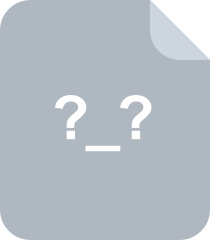
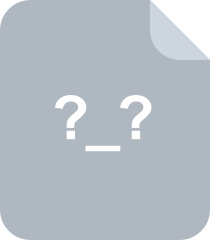
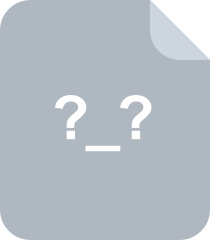
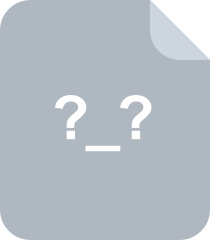
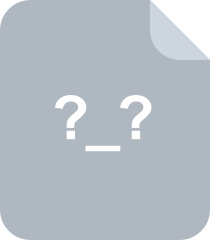
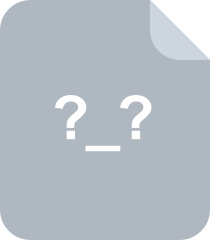
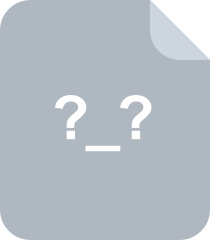
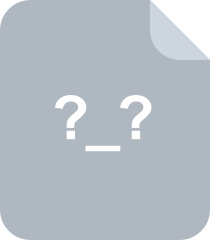
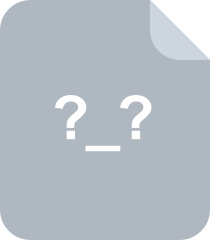
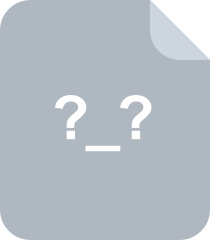
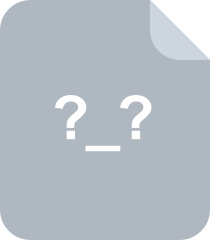
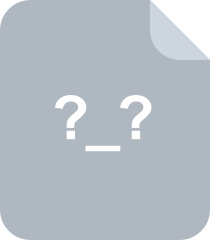
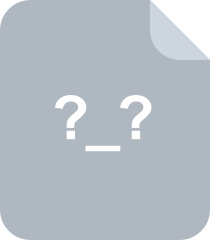
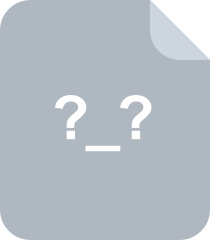
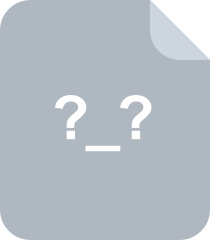
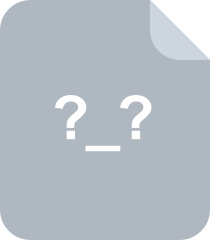
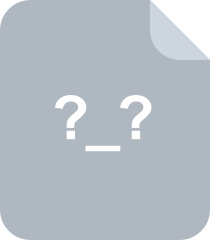
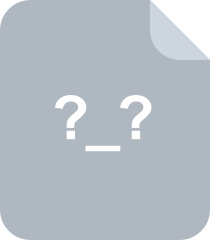
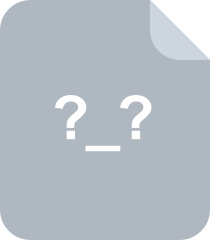
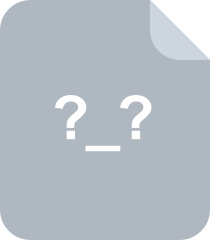
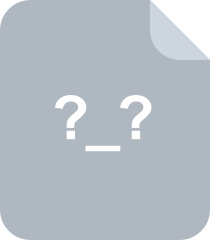
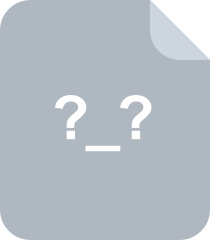
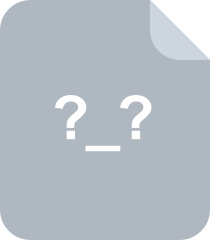
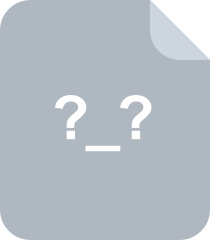
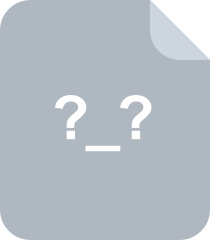
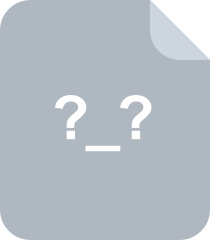
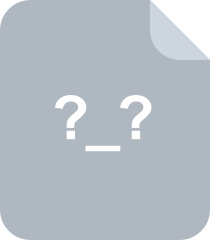
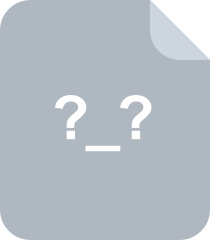
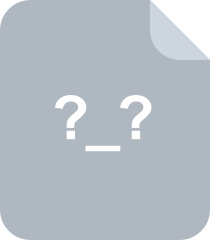
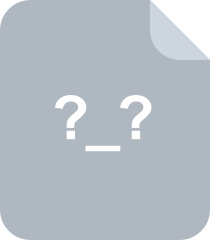
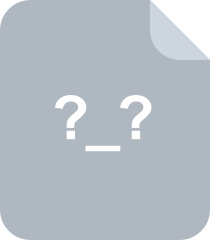
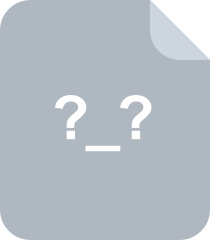
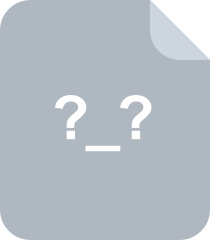
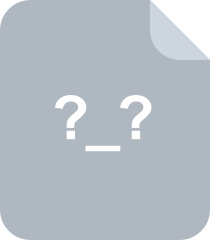
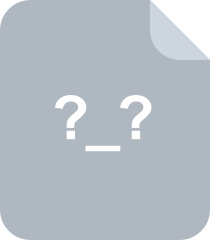
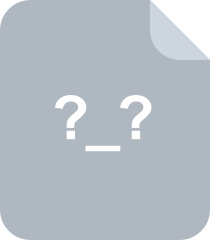
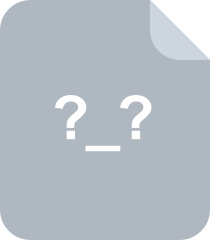
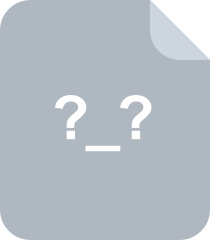
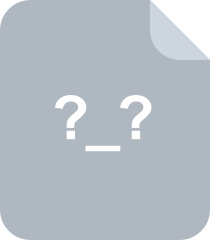
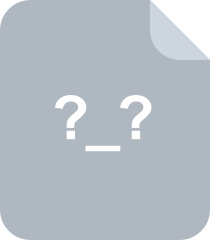
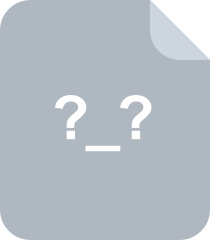
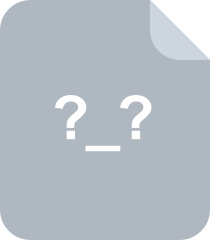
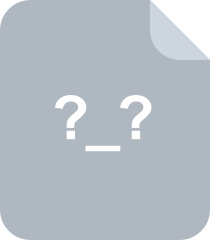
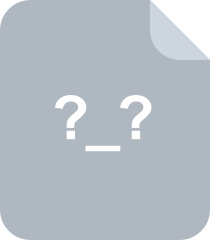
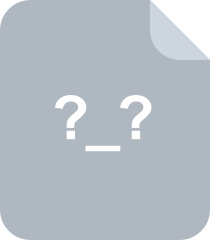
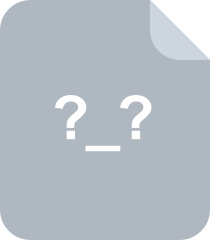
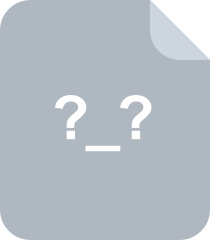
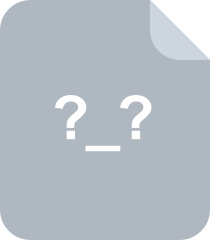
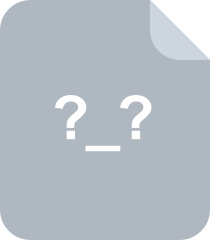
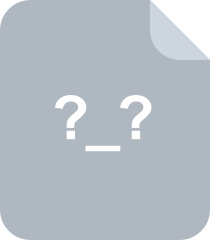
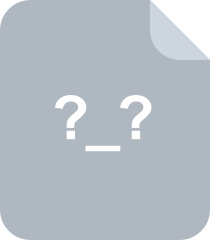
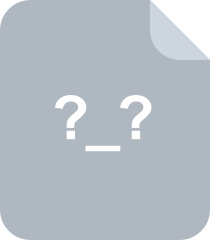
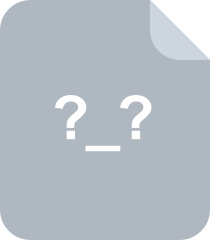
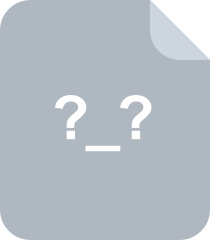
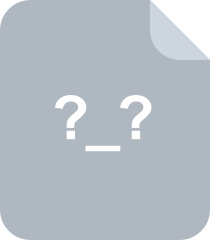
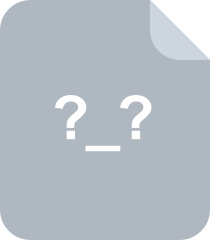
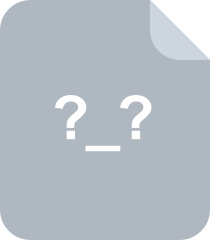
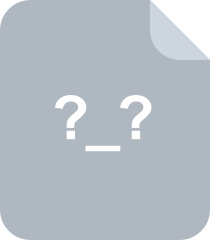
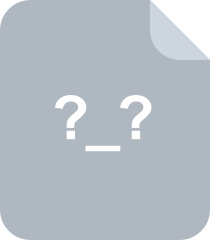
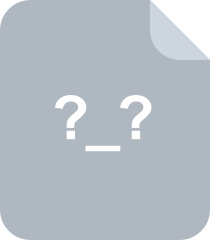
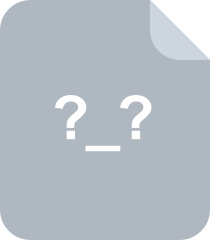
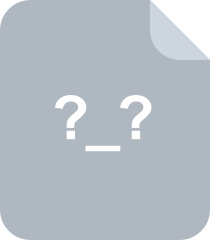
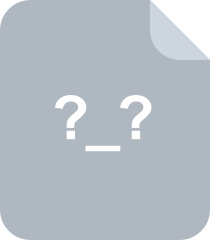
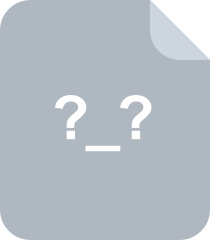
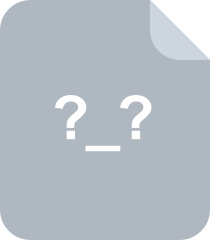
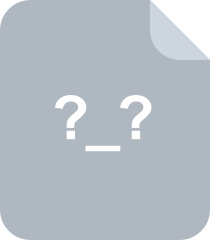
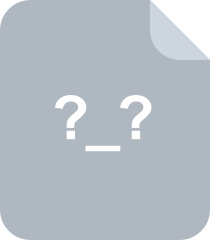
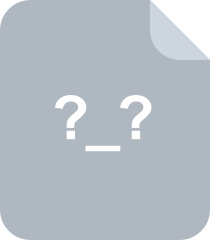
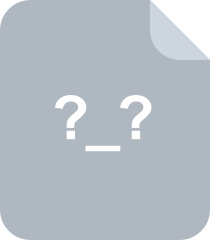
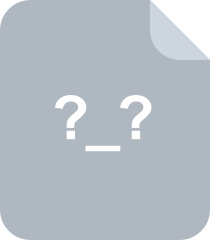
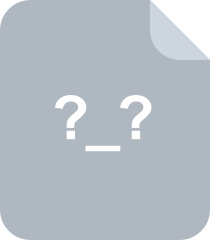
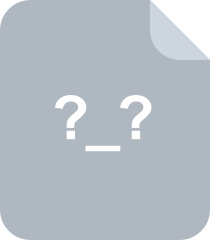
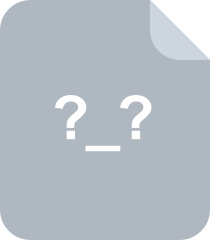
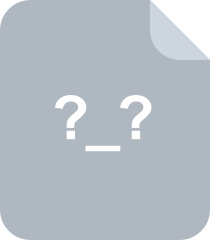
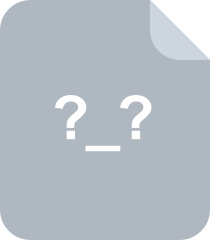
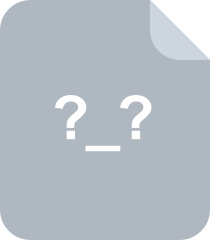
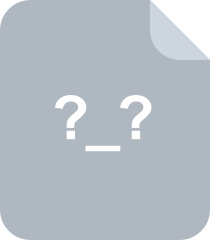
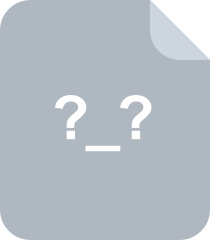
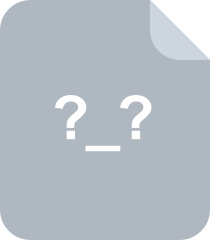
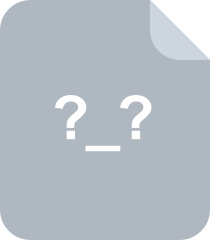
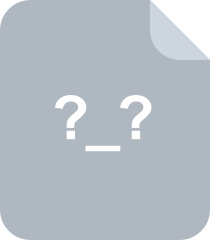
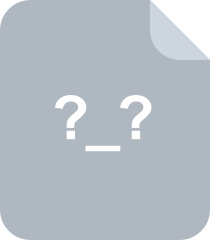
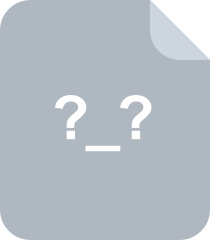
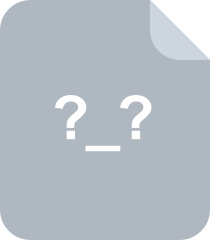
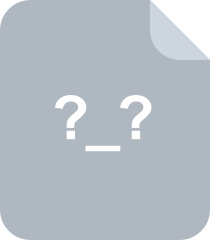
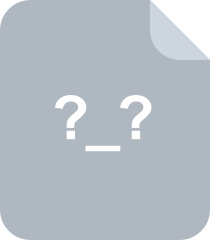
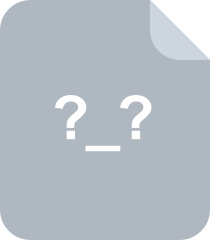
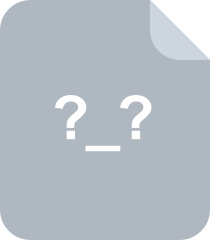
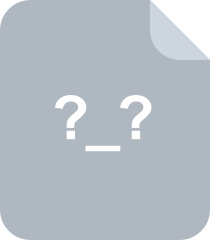
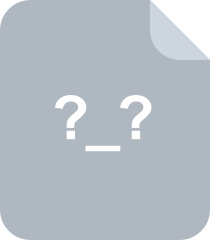
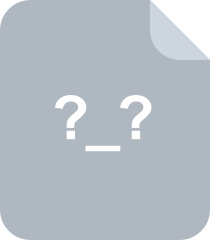
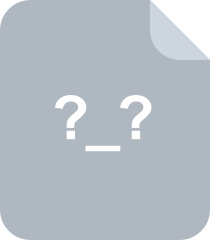
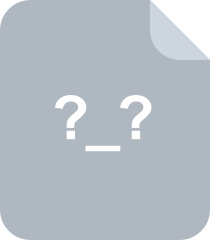
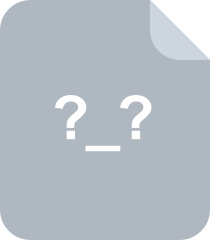
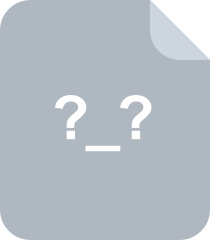
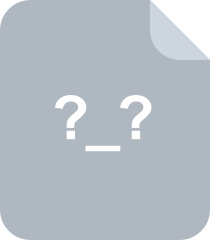
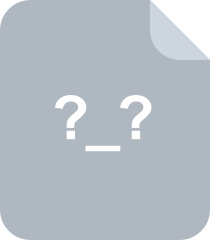
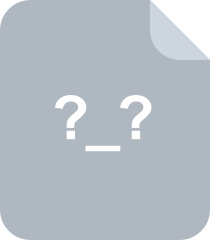
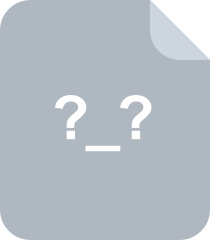
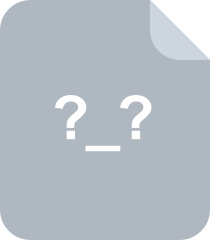
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
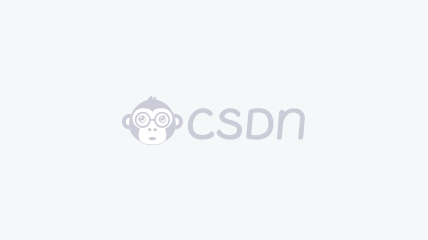

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7364
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

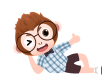
最新资源
- C#ASP.NET企业文件管理系统源码数据库 SQL2008源码类型 WebForm
- 小红书图文下载工具,无水印下载图文
- 飞书文档下载工具,解除飞书文档复制限制
- 7fe9198d9e3a020dd32b09bda2cdd7ab_1731557932240_1
- VaM_Updater.zip
- C#MVC5+EasyUI企业快速开发框架源码 BS开发框架源码数据库 SQL2012源码类型 WebForm
- zblog站群:zblog seo站群高收录排名全地域霸屏
- 【安卓毕业设计】数独联网对战APP源码(完整前后端+mysql+说明文档).zip
- 【安卓毕业设计】Android天气小作业源码(完整前后端+mysql+说明文档).zip
- 【安卓毕业设计】群养猪生长状态远程监测源码(完整前后端+mysql+说明文档).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


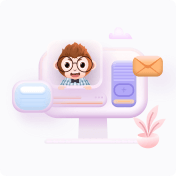
安全验证
文档复制为VIP权益,开通VIP直接复制
