<?php
namespace EasyTask;
use EasyTask\Exception\ErrorException;
use \Exception as Exception;
use \Throwable as Throwable;
/**
* Class Helper
* @package EasyTask
*/
class Helper
{
/**
* 睡眠函数
* @param int $time 时间
* @param int $type 类型:1秒 2毫秒
*/
public static function sleep($time, $type = 1)
{
if ($type == 2) {
$time *= 1000;
}
$type == 1 ? sleep($time) : usleep($time);
}
/**
* 设置进程标题
* @param string $title
*/
public static function cli_set_process_title($title)
{
set_error_handler(
function () {
}
);
if (function_exists('cli_set_process_title')) {
cli_set_process_title($title);
}
restore_error_handler();
}
/**
* 设置掩码
*/
public static function setMask()
{
umask(0);
}
/**
* 设置代码页
* @param int $code
*/
public static function setCodePage($code = 65001)
{
$ds = DIRECTORY_SEPARATOR;
$codePageBinary = "C:{$ds}Windows{$ds}System32{$ds}chcp.com";
if (file_exists($codePageBinary) && static::canUseExcCommand()) {
@shell_exec("{$codePageBinary} {$code}");
}
}
/**
* 获取命令行输入
* @param int $type
* @return string|array
*/
public static function getCliInput($type = 1)
{
// 输入参数
$argv = $_SERVER['argv'];
// 组装PHP路径
array_unshift($argv, Env::get('phpPath'));
// 自动校正
foreach ($argv as $key => $value) {
if (file_exists($value)) {
$argv[$key] = realpath($value);
}
}
// 返回
if ($type == 1) {
return join(' ', $argv);
}
return $argv;
}
/**
* 设置PHP二进制文件
* @param string $path
*/
public static function setPhpPath($path = '')
{
if (!$path) {
$path = self::getBinary();
}
Env::set('phpPath', $path);
}
/**
* 获取进程二进制文件
* @return string
*/
public static function getBinary()
{
return PHP_BINARY;
}
/**
* 是否Win平台
* @return bool
*/
public static function isWin()
{
return DIRECTORY_SEPARATOR == '\\';
}
/**
* 开启异步信号
* @return bool
*/
public static function openAsyncSignal()
{
return pcntl_async_signals(true);
}
/**
* 是否支持异步信号
* @return bool
*/
public static function canUseAsyncSignal()
{
return (function_exists('pcntl_async_signals'));
}
/**
* 是否支持event事件
* @return bool
*/
public static function canUseEvent()
{
return (extension_loaded('event'));
}
/**
* 是否可执行命令
* @return bool
*/
public static function canUseExcCommand()
{
return function_exists('shell_exec');
}
/**
* 获取运行时目录
* @return string
*/
public static function getRunTimePath()
{
$path = Env::get('runTimePath') ? Env::get('runTimePath') : sys_get_temp_dir();
if (!is_dir($path)) {
static::showSysError('please set runTimePath');
}
$path = $path . DIRECTORY_SEPARATOR . Env::get('prefix') . DIRECTORY_SEPARATOR;
$path = str_replace(DIRECTORY_SEPARATOR . DIRECTORY_SEPARATOR, DIRECTORY_SEPARATOR, $path);
return $path;
}
/**
* 获取Win进程目录
* @return string
*/
public static function getWinPath()
{
return Helper::getRunTimePath() . 'Win' . DIRECTORY_SEPARATOR;
}
/**
* 获取日志目录
* @return string
*/
public static function getLogPath()
{
return Helper::getRunTimePath() . 'Log' . DIRECTORY_SEPARATOR;
}
/**
* 获取进程命令通信目录
* @return string
*/
public static function getCsgPath()
{
return Helper::getRunTimePath() . 'Csg' . DIRECTORY_SEPARATOR;
}
/**
* 获取进程队列目录
* @return string
*/
public static function getQuePath()
{
return Helper::getRunTimePath() . 'Que' . DIRECTORY_SEPARATOR;
}
/**
* 获取进程锁目录
* @return string
*/
public static function getLokPath()
{
return Helper::getRunTimePath() . 'Lok' . DIRECTORY_SEPARATOR;
}
/**
* 获取标准输入输出目录
* @return string
*/
public static function getStdPath()
{
return Helper::getRunTimePath() . 'Std' . DIRECTORY_SEPARATOR;
}
/**
* 初始化所有目录
*/
public static function initAllPath()
{
$paths = [
static::getRunTimePath(),
static::getWinPath(),
static::getLogPath(),
static::getLokPath(),
static::getQuePath(),
static::getCsgPath(),
static::getStdPath(),
];
foreach ($paths as $path) {
if (!is_dir($path)) {
mkdir($path, 0777, true);
}
}
}
/**
* 保存标准输入|输出
* @param string $char 输入|输出
*/
public static function saveStdChar($char)
{
$path = static::getStdPath();
$file = $path . date('Y_m_d') . '.std';
$char = static::convert_char($char);
file_put_contents($file, $char, FILE_APPEND);
}
/**
* 保存日志
* @param string $message
*/
public static function writeLog($message)
{
// 日志文件
$path = Helper::getLogPath();
$file = $path . date('Y_m_d') . '.log';
// 加锁保存
$message = static::convert_char($message);
file_put_contents($file, $message, FILE_APPEND | LOCK_EX);
}
/**
* 保存类型日志
* @param string $message
* @param string $type
* @param bool $isExit
*/
public static function writeTypeLog($message, $type = 'info', $isExit = false)
{
// 格式化信息
$text = Helper::formatMessage($message, $type);
// 记录日志
static::writeLog($text);
if ($isExit) {
exit();
}
}
/**
* 编码转换
* @param string $char
* @param string $coding
* @return string
*/
public static function convert_char($char, $coding = 'UTF-8')
{
$encode_arr = ['UTF-8', 'ASCII', 'GBK', 'GB2312', 'BIG5', 'JIS', 'eucjp-win', 'sjis-win', 'EUC-JP'];
$encoded = mb_detect_encoding($char, $encode_arr);
if ($encoded) {
$char = mb_convert_encoding($char, $coding, $encoded);
}
return $char;
}
/**
* 格式化异常信息
* @param ErrorException|Exception|Throwable $exception
* @param string $type
* @return string
*/
public static function formatException($exception, $type = 'exception')
{
// 参数
$pid = getmypid();
$date = date('Y/m/d H:i:s', time());
// 组装
return $date . " [$type] : errStr:" . $exception->getMessage() . ',errFile:' . $exception->getFile() . ',errLine:' . $exception->getLine() . " (pid:$pid)" . PHP_EOL;
}
/**
* 格式化异常信息
* @param string $message
* @param string $type
* @return string
*/
public static function formatMessage($message, $type = 'error')
{
// 参数
$pid = getmypid();
$date = date('Y/m/d H:i:s', time());
// 组装
return $date . " [$type] : " . $message . " (pid:$pid)" . PHP_EOL;
}
/**
* 检查任务时间是否合法
* @param mixed $time
*/
public static function checkTaskTime($time)
{
没有合适的资源?快使用搜索试试~ 我知道了~
PHP常驻内存的多进程任务管理器Composer包
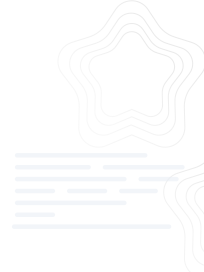
共18个文件
php:16个
license:1个
json:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 24 浏览量
2023-04-19
16:05:51
上传
评论
收藏 25KB ZIP 举报
温馨提示
PHP常驻内存的多进程任务管理器Composer包。以进程管理为出发点,同时也支持为每个进程设置定时执行功能,您可以用它来完成需要重复运行的任务(如订单超时自动取消,短信邮件异步推送,队列/消费者/频道订阅者等等),甚至处理计划任务。内置任务异常上报功能,异常错误您都可以自定义处理(例如实现异常错误自动短信邮件通知);还支持任务异常退出自动重启功能,让您的任务运行更稳定 ,工具包同时支持windows、linux、mac环境运行。
资源推荐
资源详情
资源评论
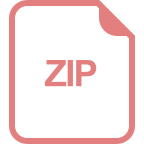
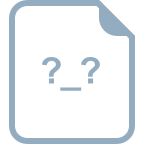
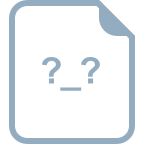
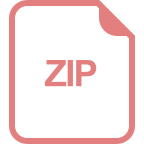
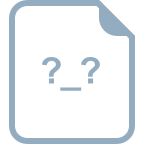
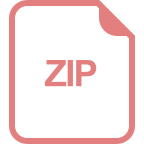
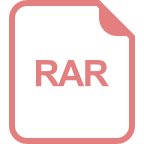
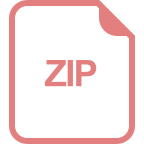
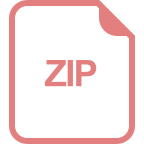
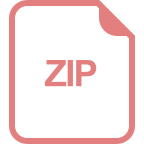
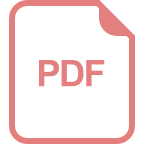
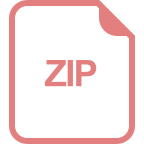
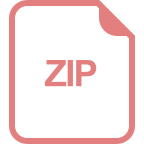
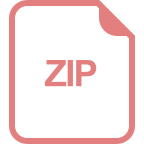
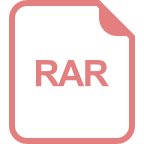
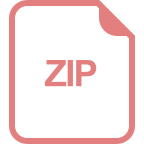
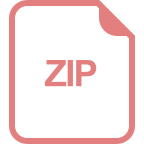
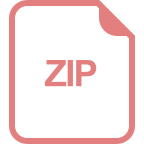
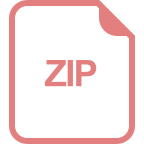
收起资源包目录



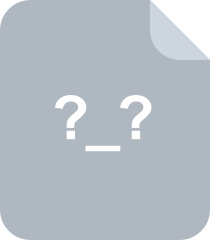

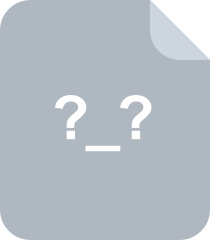
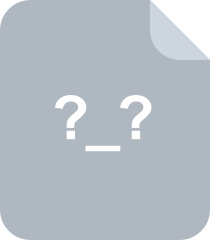
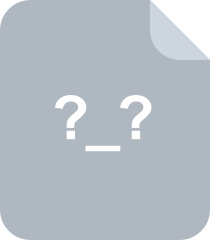
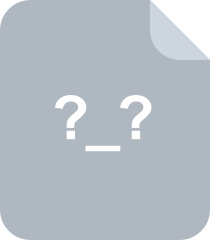

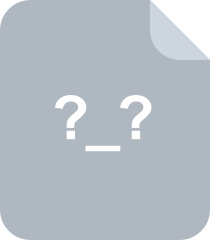
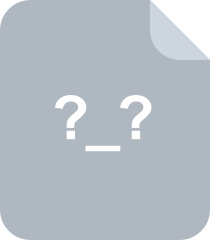
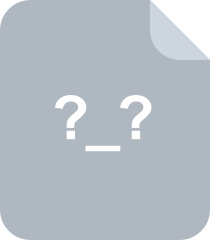
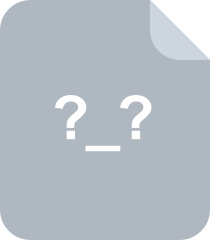
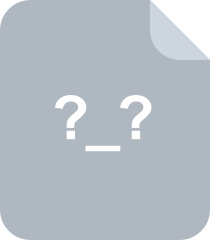
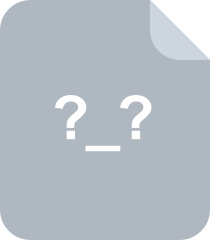
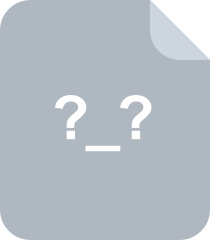
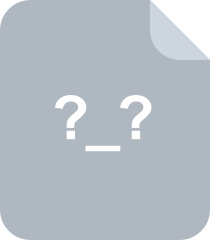
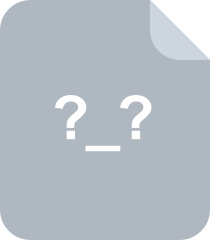
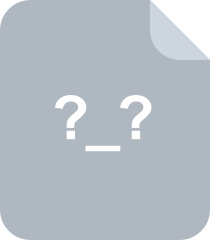
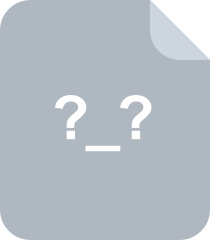
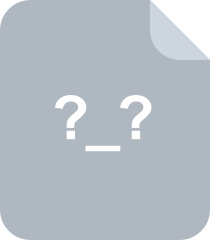

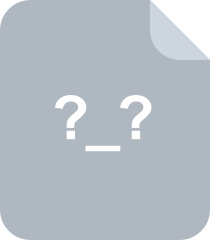
共 18 条
- 1
资源评论
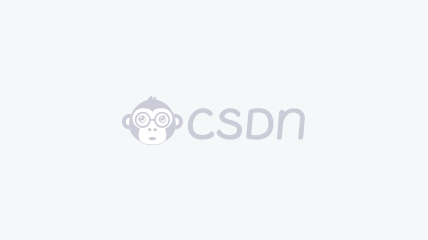

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7451
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

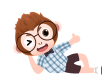
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


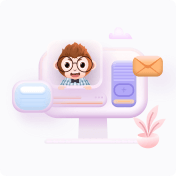
安全验证
文档复制为VIP权益,开通VIP直接复制
