<?php
/*
$Id: nusoap.php,v 1.75 2004/05/05 12:15:04 snichol Exp $
NuSOAP - Web Services Toolkit for PHP
Copyright (c) 2002 NuSphere Corporation
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with this library; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
If you have any questions or comments, please email:
Dietrich Ayala
dietrich@ganx4.com
http://dietrich.ganx4.com/nusoap
NuSphere Corporation
http://www.nusphere.com
*/
/* load classes
// necessary classes
require_once('class.soapclient.php');
require_once('class.soap_val.php');
require_once('class.soap_parser.php');
require_once('class.soap_fault.php');
// transport classes
require_once('class.soap_transport_http.php');
// optional add-on classes
require_once('class.xmlschema.php');
require_once('class.wsdl.php');
// server class
require_once('class.soap_server.php');*/
/**
*
* nusoap_base
*
* @author Dietrich Ayala <dietrich@ganx4.com>
* @version $Id: nusoap.php,v 1.75 2004/05/05 12:15:04 snichol Exp $
* @access public
*/
class nusoap_base {
var $title = 'NuSOAP';
var $version = '0.6.7';
var $revision = '$Revision: 1.75 $';
var $error_str = false;
var $debug_str = '';
// toggles automatic encoding of special characters as entities
// (should always be true, I think)
var $charencoding = true;
/**
* set schema version
*
* @var XMLSchemaVersion
* @access public
*/
var $XMLSchemaVersion = 'http://www.w3.org/2001/XMLSchema';
/**
* set charset encoding for outgoing messages
*
* @var soap_defencoding
* @access public
*/
//var $soap_defencoding = 'UTF-8';
var $soap_defencoding = 'ISO-8859-1';
/**
* load namespace uris into an array of uri => prefix
*
* @var namespaces
* @access public
*/
var $namespaces = array(
'SOAP-ENV' => 'http://schemas.xmlsoap.org/soap/envelope/',
'xsd' => 'http://www.w3.org/2001/XMLSchema',
'xsi' => 'http://www.w3.org/2001/XMLSchema-instance',
'SOAP-ENC' => 'http://schemas.xmlsoap.org/soap/encoding/',
'si' => 'http://soapinterop.org/xsd');
var $usedNamespaces = array();
/**
* load types into typemap array
* is this legacy yet?
* no, this is used by the xmlschema class to verify type => namespace mappings.
* @var typemap
* @access public
*/
var $typemap = array(
'http://www.w3.org/2001/XMLSchema' => array(
'string'=>'string','boolean'=>'boolean','float'=>'double','double'=>'double','decimal'=>'double',
'duration'=>'','dateTime'=>'string','time'=>'string','date'=>'string','gYearMonth'=>'',
'gYear'=>'','gMonthDay'=>'','gDay'=>'','gMonth'=>'','hexBinary'=>'string','base64Binary'=>'string',
// derived datatypes
'normalizedString'=>'string','token'=>'string','language'=>'','NMTOKEN'=>'','NMTOKENS'=>'','Name'=>'','NCName'=>'','ID'=>'',
'IDREF'=>'','IDREFS'=>'','ENTITY'=>'','ENTITIES'=>'','integer'=>'integer','nonPositiveInteger'=>'integer',
'negativeInteger'=>'integer','long'=>'integer','int'=>'integer','short'=>'integer','byte'=>'integer','nonNegativeInteger'=>'integer',
'unsignedLong'=>'','unsignedInt'=>'','unsignedShort'=>'','unsignedByte'=>'','positiveInteger'=>''),
'http://www.w3.org/1999/XMLSchema' => array(
'i4'=>'','int'=>'integer','boolean'=>'boolean','string'=>'string','double'=>'double',
'float'=>'double','dateTime'=>'string',
'timeInstant'=>'string','base64Binary'=>'string','base64'=>'string','ur-type'=>'array'),
'http://soapinterop.org/xsd' => array('SOAPStruct'=>'struct'),
'http://schemas.xmlsoap.org/soap/encoding/' => array('base64'=>'string','array'=>'array','Array'=>'array'),
'http://xml.apache.org/xml-soap' => array('Map')
);
/**
* entities to convert
*
* @var xmlEntities
* @access public
*/
var $xmlEntities = array('quot' => '"','amp' => '&',
'lt' => '<','gt' => '>','apos' => "'");
/**
* adds debug data to the class level debug string
*
* @param string $string debug data
* @access private
*/
function debug($string){
$this->debug_str .= get_class($this).": $string\n";
}
/**
* expands entities, e.g. changes '<' to '<'.
*
* @param string $val The string in which to expand entities.
* @access private
*/
function expandEntities($val) {
if ($this->charencoding) {
$val = str_replace('&', '&', $val);
$val = str_replace("'", ''', $val);
$val = str_replace('"', '"', $val);
$val = str_replace('<', '<', $val);
$val = str_replace('>', '>', $val);
}
return $val;
}
/**
* returns error string if present
*
* @return boolean $string error string
* @access public
*/
function getError(){
if($this->error_str != ''){
return $this->error_str;
}
return false;
}
/**
* sets error string
*
* @return boolean $string error string
* @access private
*/
function setError($str){
$this->error_str = $str;
}
/**
* detect if array is a simple array or a struct (associative array)
*
* @param $val The PHP array
* @return string (arraySimple|arrayStruct)
* @access private
*/
function isArraySimpleOrStruct($val) {
$keyList = array_keys($val);
foreach ($keyList as $keyListValue) {
if (!is_int($keyListValue)) {
return 'arrayStruct';
}
}
return 'arraySimple';
}
/**
* serializes PHP values in accordance w/ section 5. Type information is
* not serialized if $use == 'literal'.
*
* @return string
* @access public
*/
function serialize_val($val,$name=false,$type=false,$name_ns=false,$type_ns=false,$attributes=false,$use='encoded'){
if(is_object($val) && get_class($val) == 'soapval'){
return $val->serialize($use);
}
$this->debug( "in serialize_val: $val, $name, $type, $name_ns, $type_ns, $attributes, $use");
// if no name, use item
$name = (!$name|| is_numeric($name)) ? 'soapVal' : $name;
// if name has ns, add ns prefix to name
$xmlns = '';
if($name_ns){
$prefix = 'nu'.rand(1000,9999);
$name = $prefix.':'.$name;
$xmlns .= " xmlns:$prefix=\"$name_ns\"";
}
// if type is prefixed, create type prefix
if($type_ns != '' && $type_ns == $this->namespaces['xsd']){
// need to fix this. shouldn't default to xsd if no ns specified
// w/o checking against typemap
$type_prefix = 'xsd';
} elseif($type_ns){
$type_prefix = 'ns'.rand(1000,9999);
$xmlns .= " xmlns:$type_prefix=\"$type_ns\"";
}
// serialize attributes if present
$atts = '';
if($attributes){
foreach($attributes as $k => $v){
$atts .= " $k=\"$v\"";
}
}
// serialize if an xsd built-in primitive type
if($type != '' && isset($this->typemap[$this->XMLSchemaVersion][$type])){
if (is_bool($val)) {
if ($type == 'boolean') {
$val = $val ? 'true' : 'false';
} elseif (! $val) {
$val = 0;
}
} else if (is_string($val)) {
$val = $this->expandEntities($val);
}
if ($use == 'literal') {
return "<$name$xmlns>$val</$name>";
} else {
return "<$name$xmlns xsi:type=\"xsd:$type\">$val</$name>";
}
}
// detect type and serialize
$xml = '';
switch(true) {
case ($type == '' && is_null($val)):
if ($use == 'literal') {
// TODO: depends on nillable
$xml .= "<$name$xmlns/>";
} else {
$xml .= "<$name$xmlns xsi:nil=\"true\"/>";
}
break;
case (is_bool($val) || $type == 'boolean'):
if ($type == 'boolean') {
$
没有合适的资源?快使用搜索试试~ 我知道了~
phpweb代理平台V2.1.2 2017最新版 代理平台9001
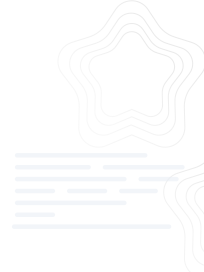
共3182个文件
gif:1115个
htm:621个
php:556个

需积分: 9 13 下载量 62 浏览量
2017-12-20
22:02:11
上传
评论
收藏 4.42MB ZIP 举报
温馨提示
phpweb代理平台V2.1.2 2017最新版 代理平台9001,成品网站源码,成品网站代理平台
资源推荐
资源详情
资源评论
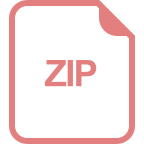
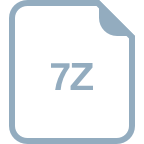
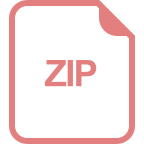
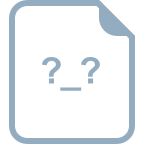
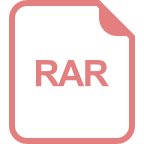
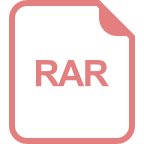
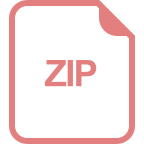
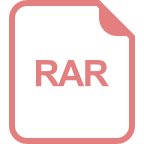
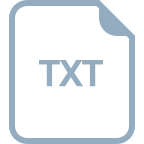
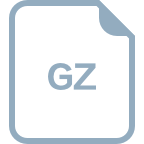
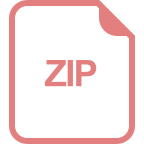
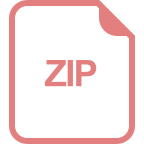
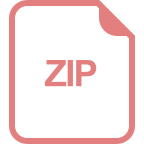
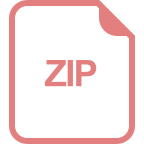
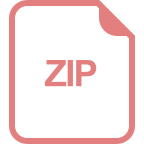
收起资源包目录

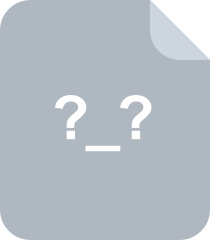
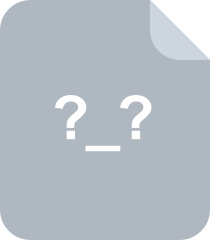
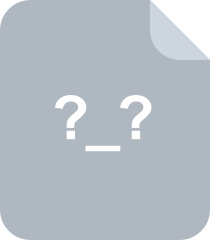
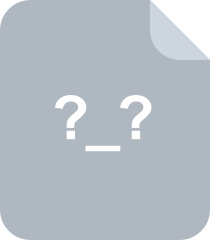
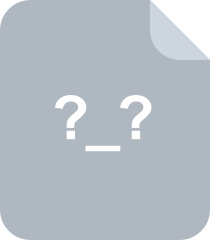
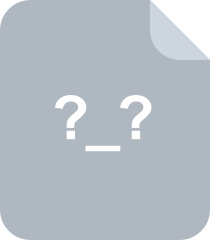
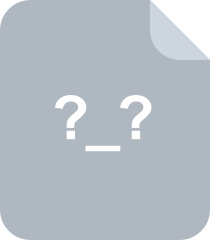
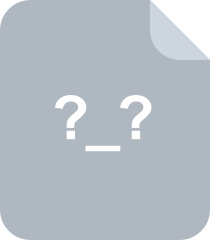
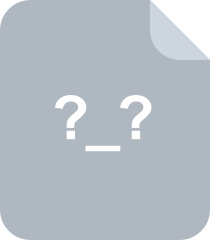
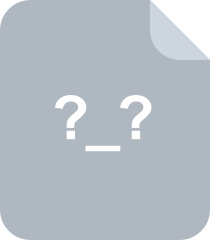
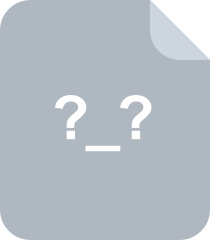
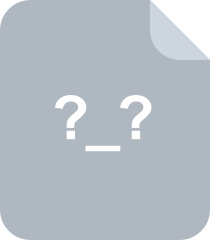
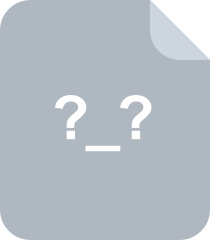
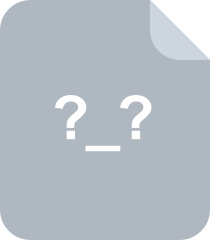
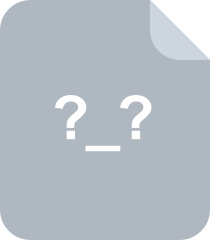
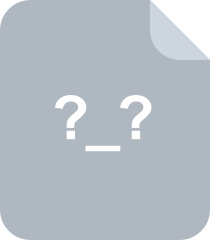
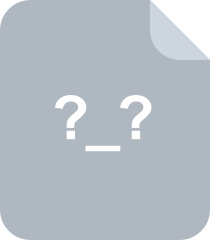
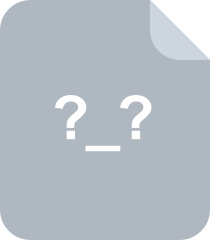
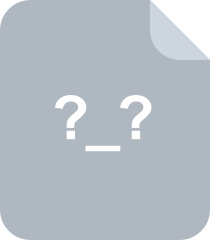
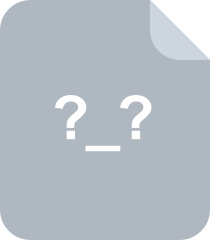
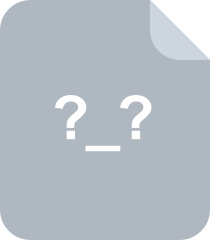
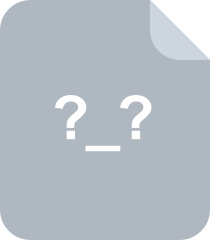
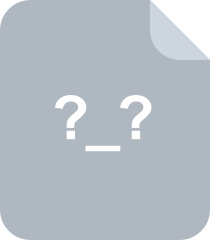
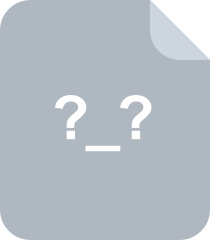
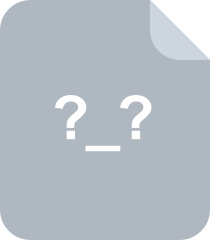
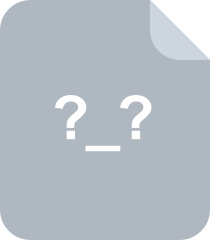
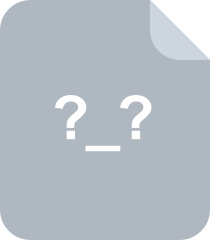
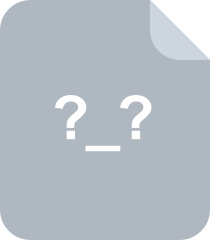
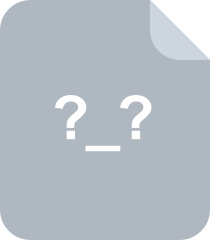
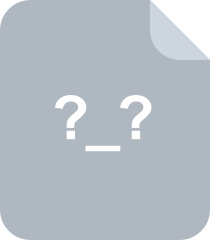
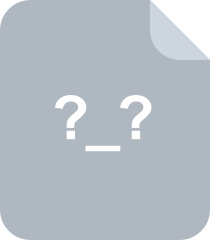
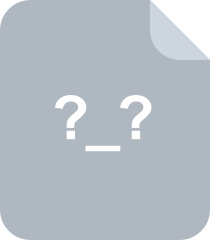
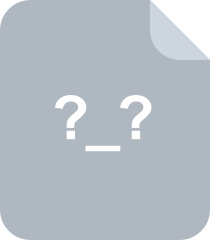
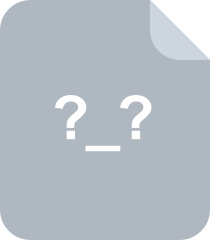
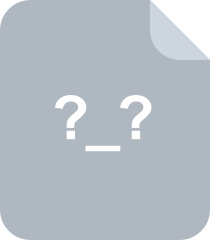
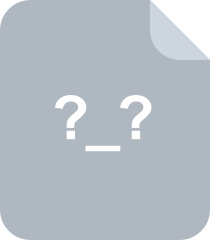
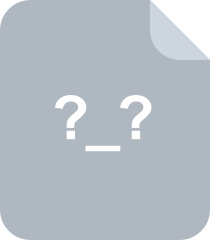
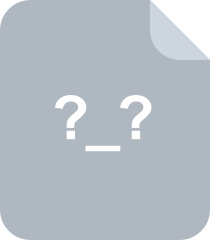
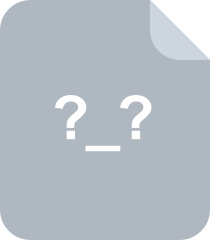
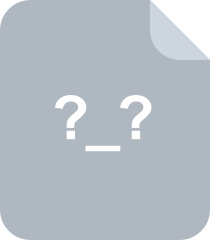
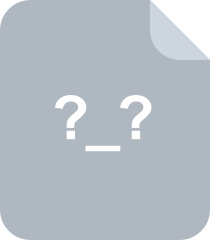
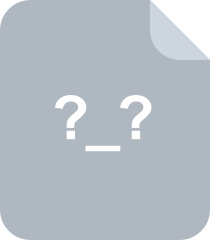
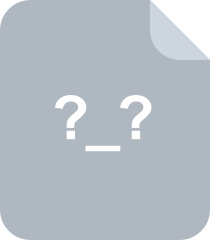
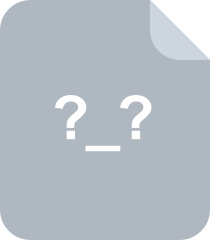
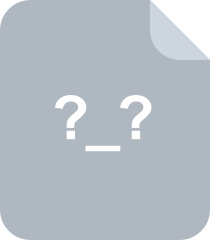
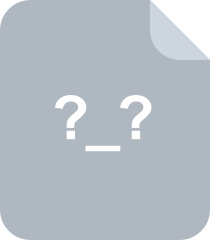
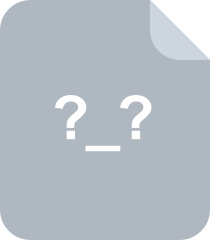
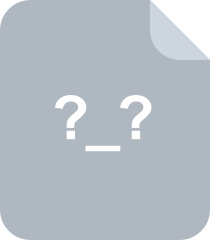
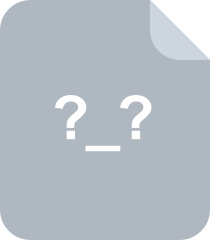
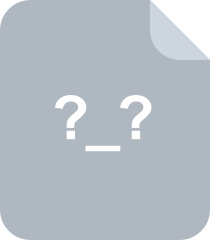
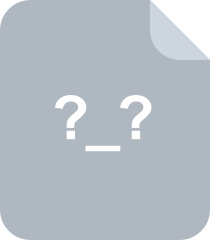
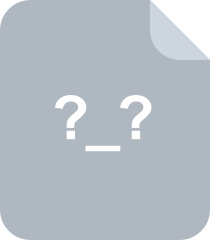
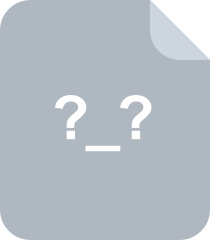
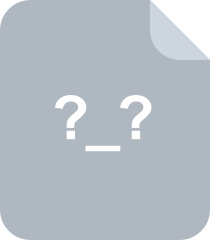
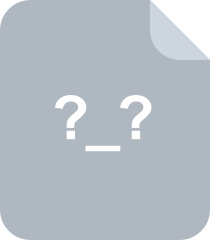
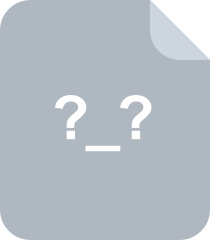
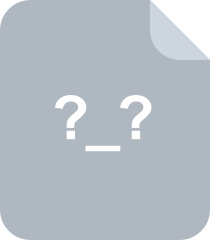
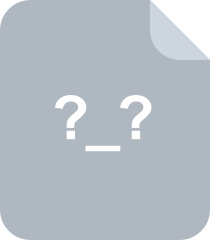
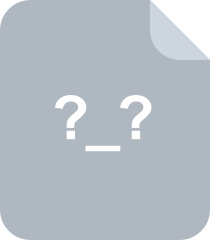
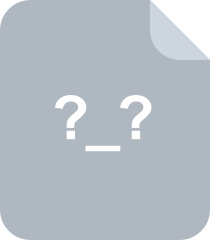
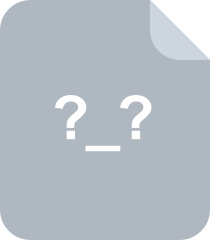
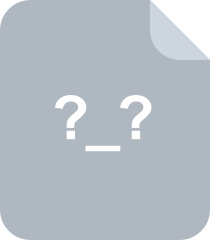
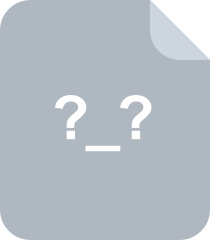
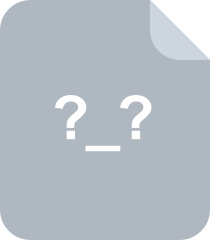
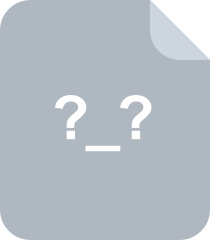
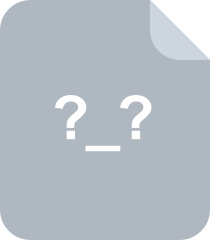
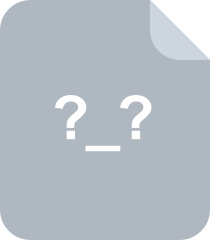
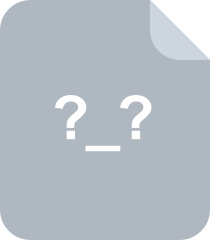
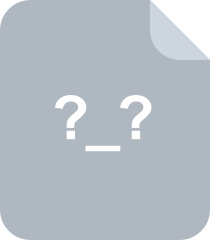
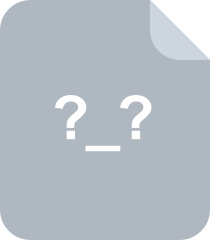
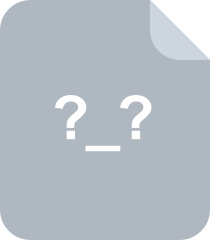
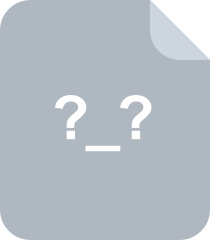
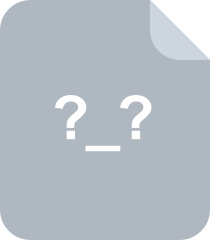
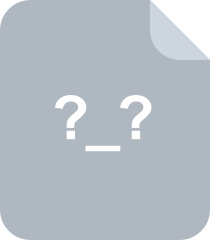
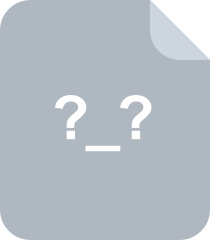
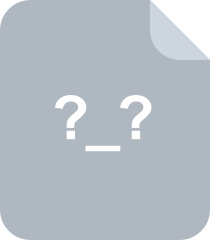
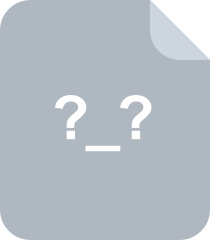
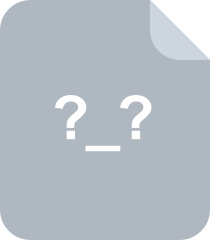
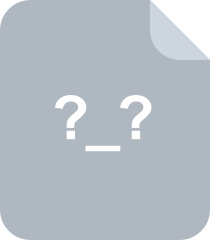
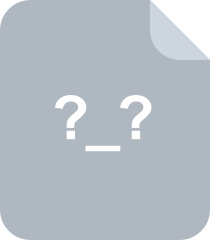
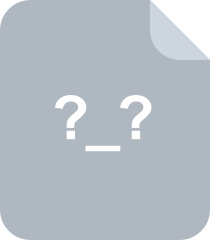
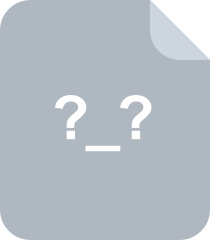
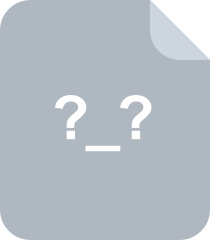
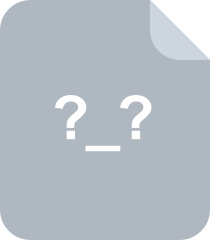
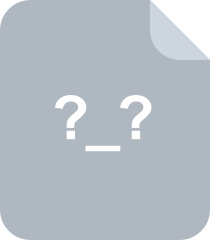
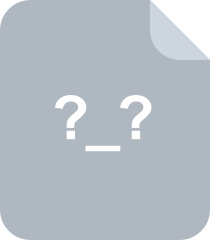
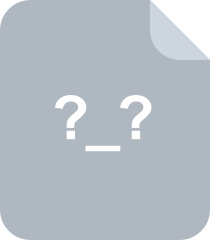
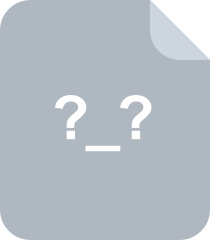
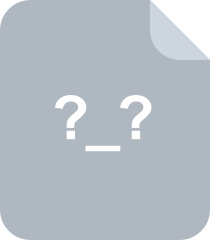
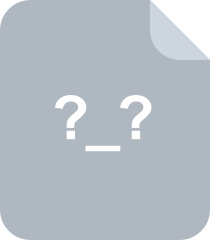
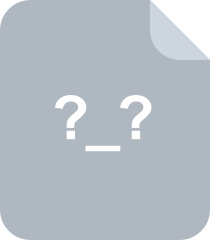
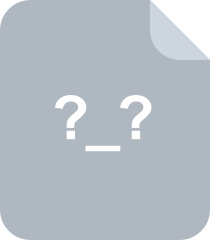
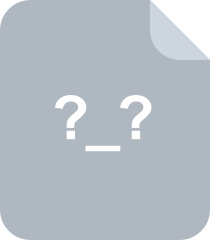
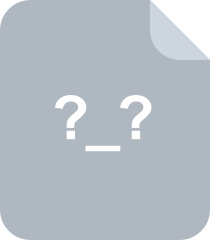
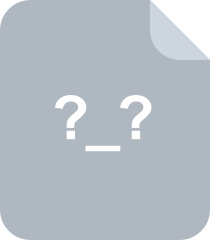
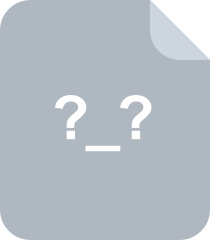
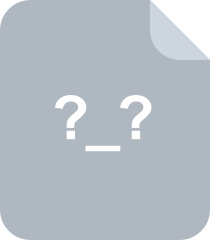
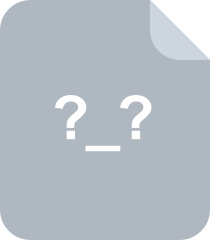
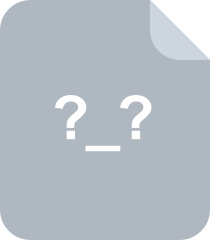
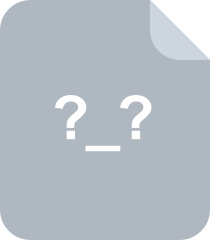
共 3182 条
- 1
- 2
- 3
- 4
- 5
- 6
- 32
资源评论
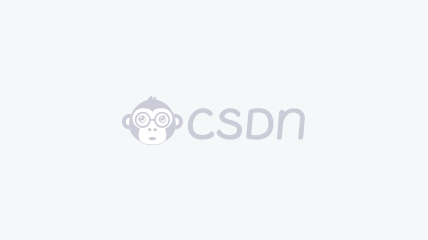

qq_41476222
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

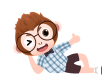
安全验证
文档复制为VIP权益,开通VIP直接复制
