package cn.zwz.basics.redis;
import io.swagger.annotations.ApiOperation;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.connection.DataType;
import org.springframework.data.redis.connection.RedisConnection;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.Cursor;
import org.springframework.data.redis.core.ScanOptions;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.core.ZSetOperations;
import org.springframework.stereotype.Component;
import java.nio.charset.StandardCharsets;
import java.util.*;
import java.util.concurrent.TimeUnit;
import java.util.function.Consumer;
/**
* Redis工具类
*/
@Component
public class RedisTemplateHelper {
@Autowired
private StringRedisTemplate redisTemplate;
@ApiOperation(value = "scan实现")
private void scan(String wayForScan, Consumer<byte[]> consumableList) {
redisTemplate.execute((RedisConnection connection) -> {
try (Cursor<byte[]> cursor = connection.scan(ScanOptions.scanOptions().count(Long.MAX_VALUE).match(wayForScan).build())) {
cursor.forEachRemaining(consumableList);
return null;
} catch (Exception exception) {
throw new RuntimeException(exception);
}
});
}
@ApiOperation(value = "scan获取符合条件的key")
public Set<String> scan(String pattern) {
Set<String> keys = new HashSet<>();
this.scan(pattern, item -> {
String key = new String(item, StandardCharsets.UTF_8);
keys.add(key);
});
return keys;
}
@ApiOperation(value = "通过通配符表达式删除所有")
public void deleteByPattern(String pattern) {
Set<String> keys = this.scan(pattern);
redisTemplate.delete(keys);
}
@ApiOperation(value = "删除key")
public void delete(String key) {
redisTemplate.delete(key);
}
@ApiOperation(value = "批量删除key")
public void delete(Collection<String> keys) {
redisTemplate.delete(keys);
}
@ApiOperation(value = "序列化key")
public byte[] dump(String key) {
return redisTemplate.dump(key);
}
@ApiOperation(value = "是否存在key")
public Boolean hasKey(String key) {
return redisTemplate.hasKey(key);
}
@ApiOperation(value = "设置过期时间")
public Boolean expire(String key, long timeout, TimeUnit unit) {
return redisTemplate.expire(key, timeout, unit);
}
@ApiOperation(value = "设置过期时间")
public Boolean expireAt(String key, Date date) {
return redisTemplate.expireAt(key, date);
}
@ApiOperation(value = "查找匹配的key")
public Set<String> keys(String pattern) {
return redisTemplate.keys(pattern);
}
@ApiOperation(value = "将当前数据库的 key 移动到给定的数据库 db 当中")
public Boolean move(String key, int dbIndex) {
return redisTemplate.move(key, dbIndex);
}
@ApiOperation(value = "移除 key 的过期时间,key 将持久保持")
public Boolean persist(String key) {
return redisTemplate.persist(key);
}
@ApiOperation(value = "返回 key 的剩余的过期时间")
public Long getExpire(String key, TimeUnit unit) {
return redisTemplate.getExpire(key, unit);
}
@ApiOperation(value = "返回 key 的剩余的过期时间")
public Long getExpire(String key) {
return redisTemplate.getExpire(key);
}
@ApiOperation(value = "从当前数据库中随机返回一个 key")
public String randomKey() {
return redisTemplate.randomKey();
}
@ApiOperation(value = "修改 key 的名称")
public void rename(String oldKey, String newKey) {
redisTemplate.rename(oldKey, newKey);
}
@ApiOperation(value = "仅当 newkey 不存在时,将 oldKey 改名为 newkey")
public Boolean renameIfAbsent(String oldKey, String newKey) {
return redisTemplate.renameIfAbsent(oldKey, newKey);
}
@ApiOperation(value = "返回 key 所储存的值的类型")
public DataType type(String key) {
return redisTemplate.type(key);
}
/** -------------------string相关操作--------------------- */
@ApiOperation(value = "设置指定 key 的值")
public void set(String key, String value) {
redisTemplate.opsForValue().set(key, value);
}
@ApiOperation(value = "将值 value 关联到 key ,并将 key 的过期时间设为 timeout")
public void set(String key, String value, long timeout, TimeUnit unit) {
redisTemplate.opsForValue().set(key, value, timeout, unit);
}
@ApiOperation(value = "获取指定 key 的值")
public String get(String key) {
return redisTemplate.opsForValue().get(key);
}
@ApiOperation(value = "返回 key 中字符串值的子字符")
public String getRange(String key, long start, long end) {
return redisTemplate.opsForValue().get(key, start, end);
}
@ApiOperation(value = "将给定 key 的值设为 value ,并返回 key 的旧值(old value)")
public String getAndSet(String key, String value) {
return redisTemplate.opsForValue().getAndSet(key, value);
}
@ApiOperation(value = "对 key 所储存的字符串值,获取指定偏移量上的位(bit)")
public Boolean getBit(String key, long offset) {
return redisTemplate.opsForValue().getBit(key, offset);
}
@ApiOperation(value = "批量获取")
public List<String> multiGet(Collection<String> keys) {
return redisTemplate.opsForValue().multiGet(keys);
}
@ApiOperation(value = "设置ASCII码, 字符串'a'的ASCII码是97, 转为二进制是'01100001', 此方法是将二进制第offset位值变为value",notes = "offset 位置, value: 值,true为1, false为0")
public boolean setBit(String key, long offset, boolean value) {
return redisTemplate.opsForValue().setBit(key, offset, value);
}
@ApiOperation(value = "只有在 key 不存在时设置 key 的值",notes = "之前已经存在返回false, 不存在返回true")
public boolean setIfAbsent(String key, String value) {
return redisTemplate.opsForValue().setIfAbsent(key, value);
}
@ApiOperation(value = "用 value 参数覆写给定 key 所储存的字符串值,从偏移量 offset 开始",notes = "offset:从指定位置开始覆写")
public void setRange(String key, String value, long offset) {
redisTemplate.opsForValue().set(key, value, offset);
}
@ApiOperation(value = "获取字符串的长度")
public Long size(String key) {
return redisTemplate.opsForValue().size(key);
}
@ApiOperation(value = "批量添加")
public void multiSet(Map<String, String> maps) {
redisTemplate.opsForValue().multiSet(maps);
}
@ApiOperation(value = "同时设置一个或多个 key-value 对,当且仅当所有给定 key 都不存在")
public boolean multiSetIfAbsent(Map<String, String> maps) {
return redisTemplate.opsForValue().multiSetIfAbsent(maps);
}
@ApiOperation(value = "增加(自增长), 负数则为自减")
public Long incrBy(String key, long increment) {
return redisTemplate.opsForValue().increment(key, increment);
}
@ApiOperation(value = "增加(自增长)")
public Double incrByFloat(String key, double increment) {
return redisTemplate.opsForValue().increment(key, increment);
}
@ApiOperation(value = "追加到末尾")
public Integer append(String key, String value) {
return redisTemplate.opsForValue
没有合适的资源?快使用搜索试试~ 我知道了~
基于Vue和SpringBoot的资产出入库管理系统(源码+数据库)
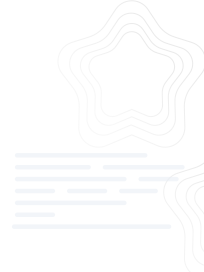
共364个文件
java:169个
vue:103个
js:42个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
本资源是基于Vue和SpringBoot的高校固定资产管理系统,包含源代码+数据库。文件预览贴:https://bbs.csdn.net/topics/612434181,你可以预览压缩包内的所有内容,欢迎下载。 资产出入库管理系统,给公司管理员、仓管员和员工使用,支持资产类型维护、资产入库、资产领用出库、资产出库审核这四大核心业务,适用于中小企业管理行政办公资产。系统给每个资产档案提供一个唯一标识,对物品入库、出库等各个作业环节进行快速批量的数据采集,确保中小企业及时掌握资产库存的真实数据,合理保持和控制企业库存。另外系统还包括员工管理、组织机构管理、文件管理、权限管理、图表分析功能,给中小企业提供更个性化的资产管理模式。 高分毕业设计项目,也可作为课程设计、大作业,下载即可使用,无需修改,确保可以直接运行,主要针对计算机相关专业的正在做毕设的学生和需要项目实战的Java学习者,也可作为课程设计、期末大作业。
资源推荐
资源详情
资源评论
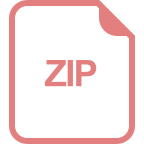
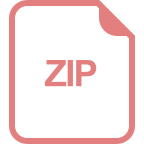
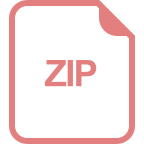
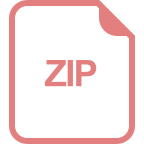
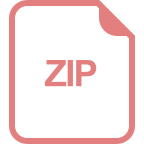
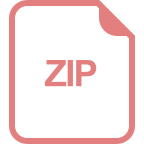
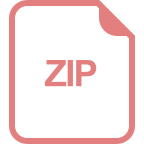
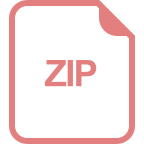
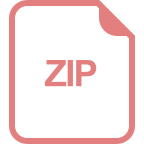
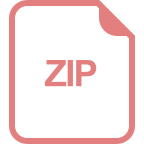
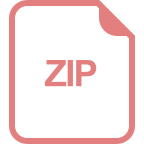
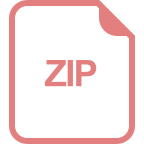
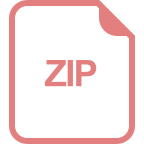
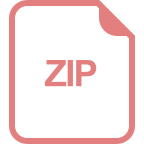
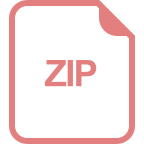
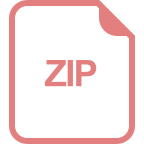
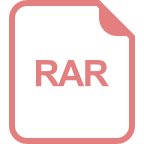
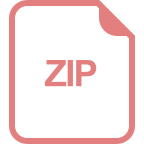
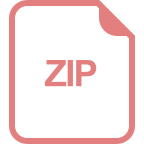
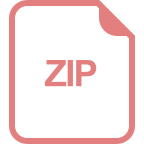
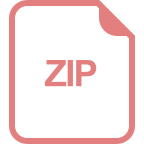
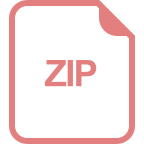
收起资源包目录

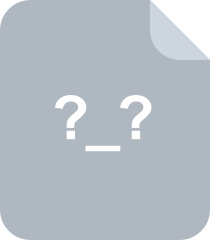
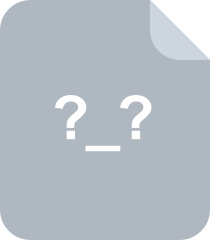
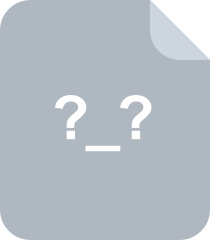
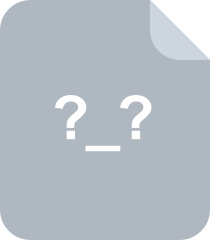
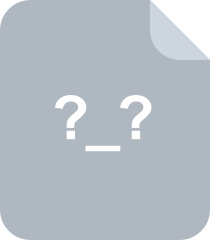
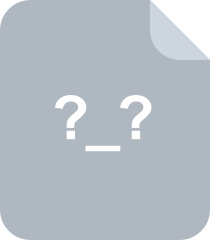
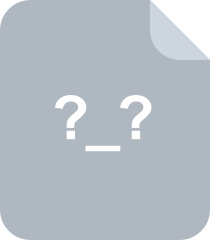
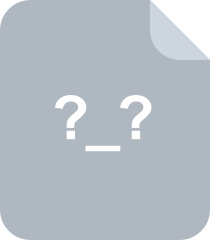
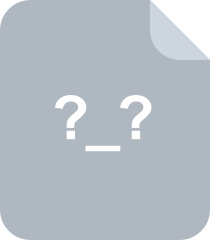
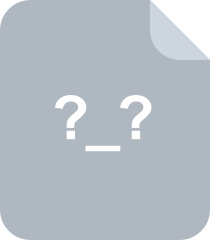
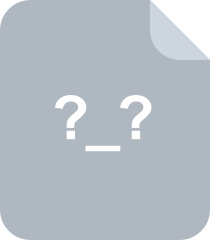
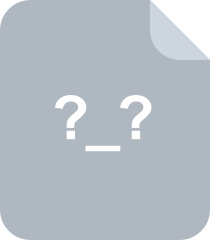
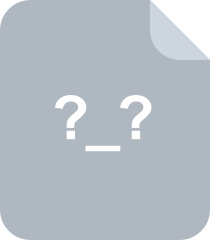
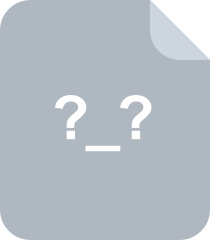
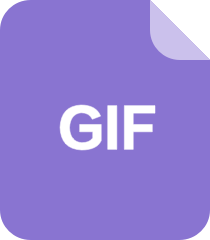
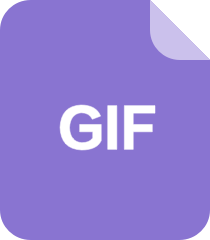
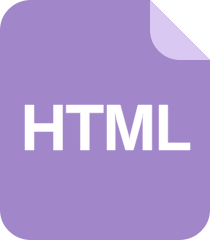
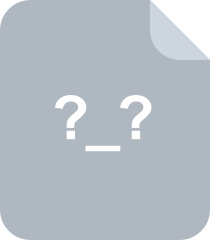
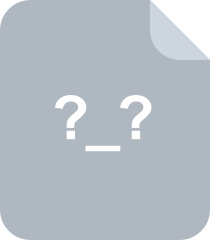
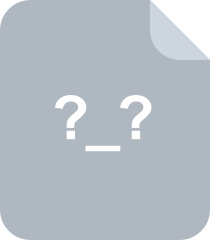
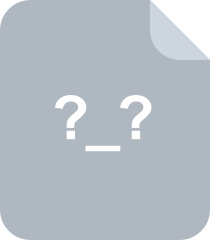
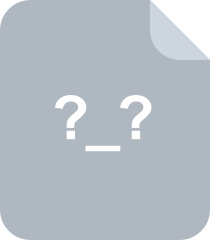
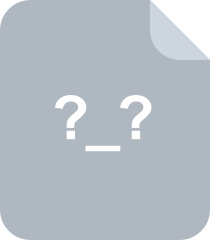
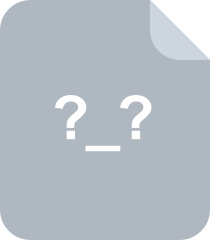
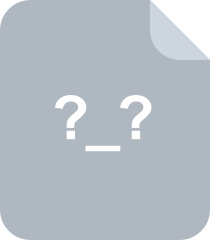
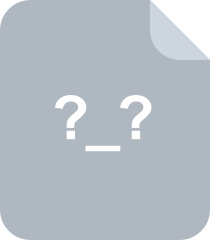
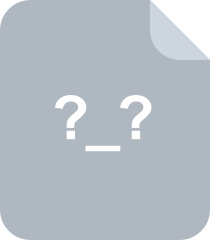
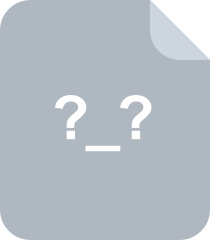
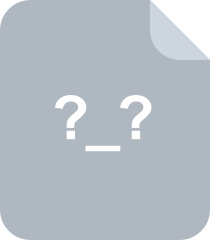
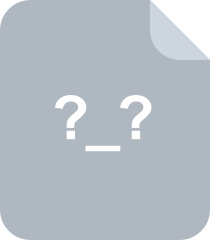
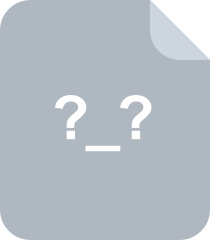
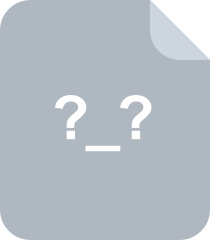
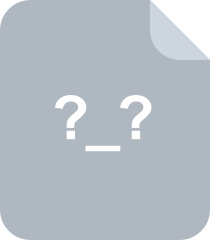
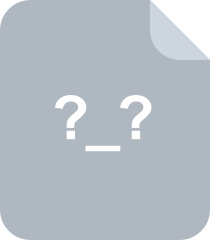
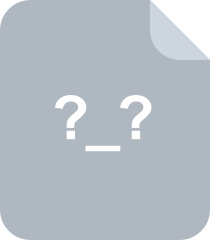
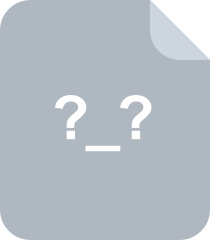
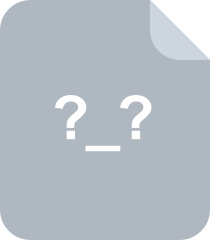
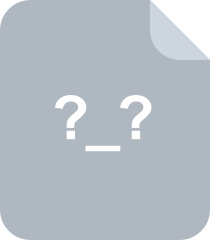
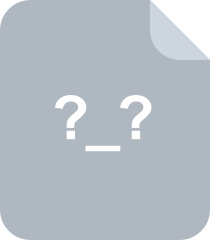
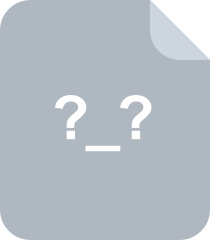
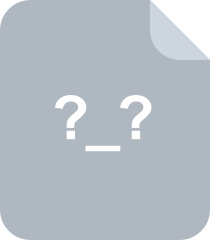
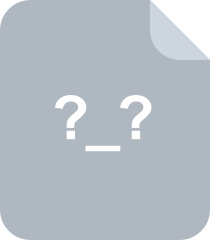
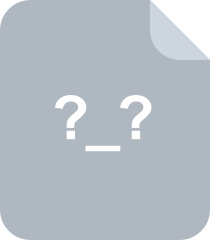
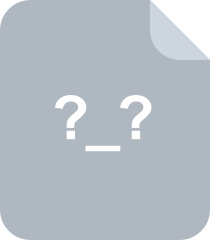
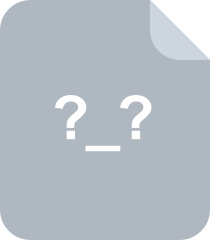
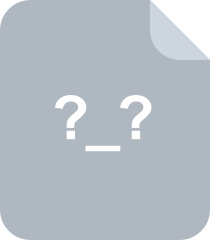
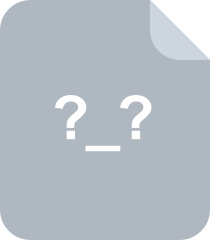
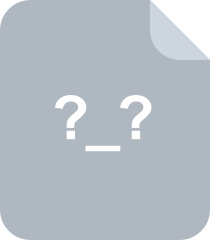
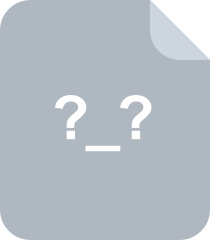
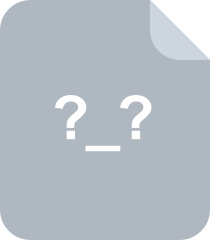
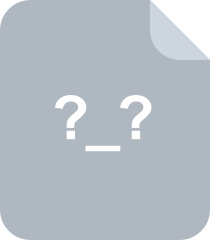
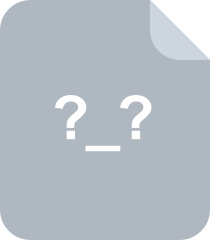
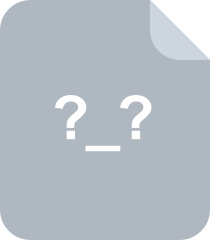
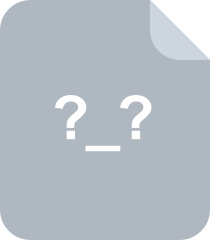
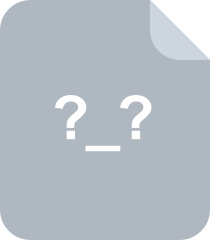
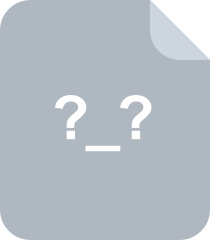
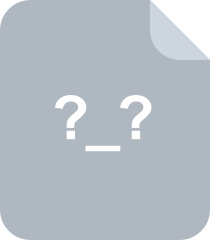
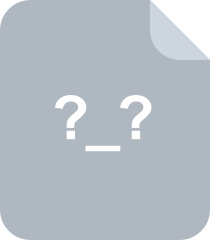
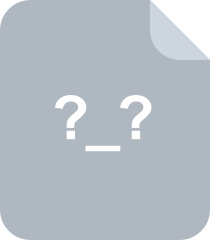
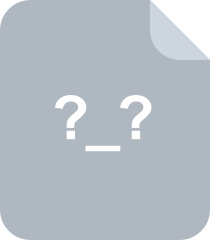
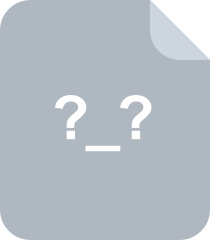
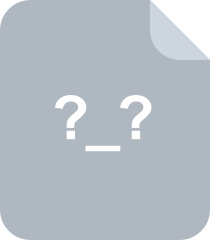
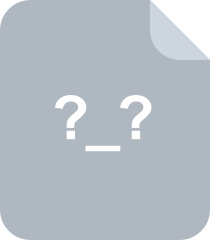
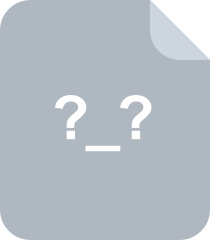
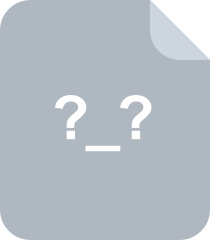
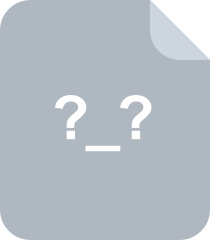
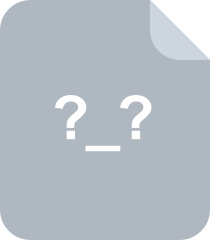
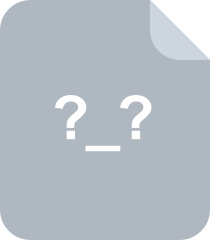
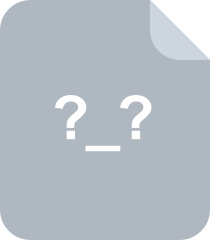
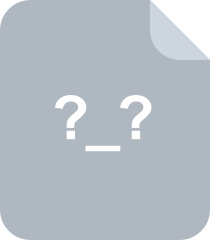
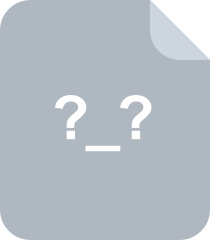
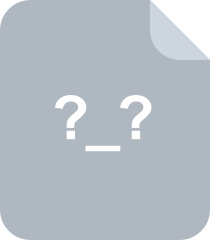
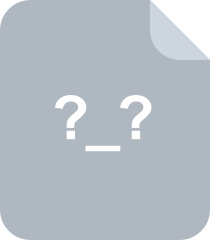
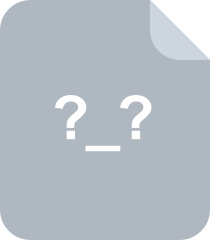
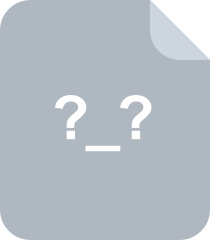
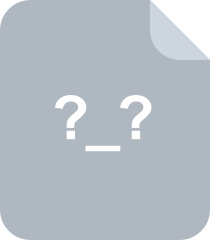
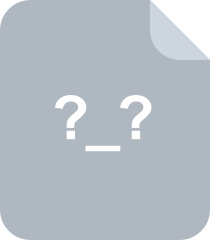
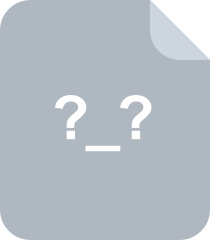
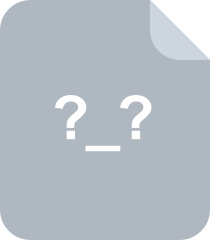
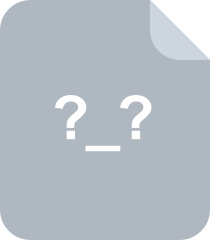
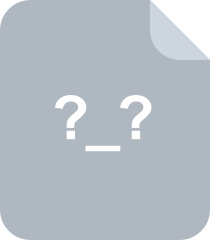
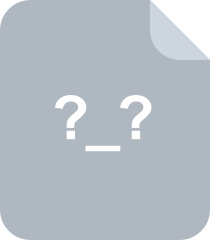
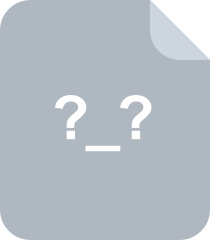
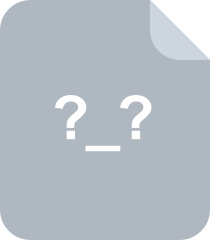
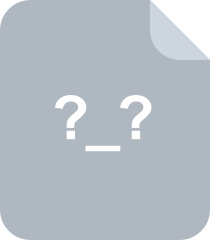
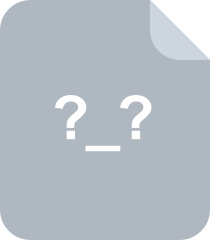
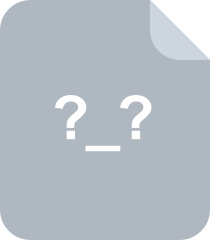
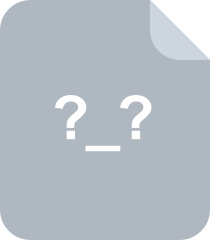
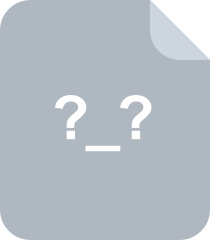
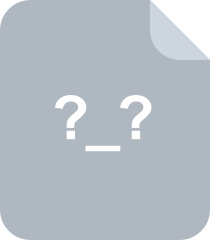
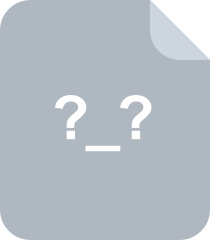
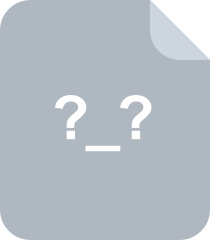
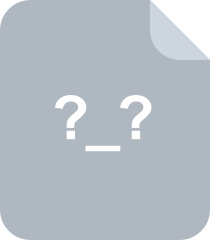
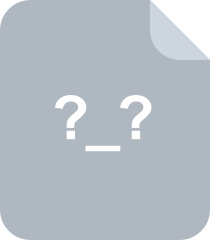
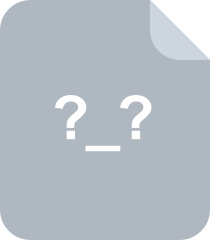
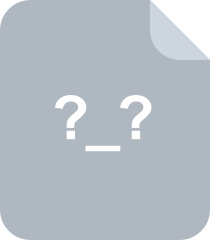
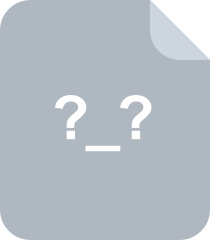
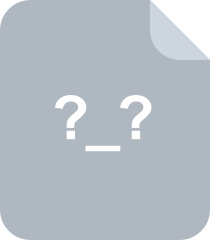
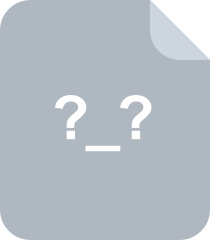
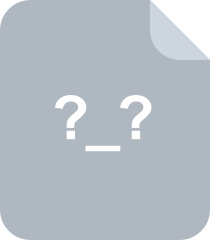
共 364 条
- 1
- 2
- 3
- 4

Designer小郑
- 粉丝: 8w+
- 资源: 156
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

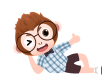
最新资源
- (源码)基于C语言和汇编语言的简单操作系统内核.zip
- (源码)基于Spring Boot框架的AntOA后台管理系统.zip
- (源码)基于Arduino的红外遥控和灯光控制系统.zip
- (源码)基于STM32的简易音乐键盘系统.zip
- (源码)基于Spring Boot和Vue的管理系统.zip
- (源码)基于Spring Boot框架的报表管理系统.zip
- (源码)基于树莓派和TensorFlow Lite的智能厨具环境监测系统.zip
- (源码)基于OpenCV和Arduino的面部追踪系统.zip
- (源码)基于C++和ZeroMQ的分布式系统中间件.zip
- (源码)基于SSM框架的学生信息管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


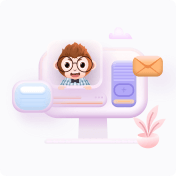
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
前往页