<h1 align="center">
<b>
<a href="https://axios-http.com"><img src="https://axios-http.com/assets/logo.svg" /></a><br>
</b>
</h1>
<p align="center">Promise based HTTP client for the browser and node.js</p>
<p align="center">
<a href="https://axios-http.com/"><b>Website</b></a> â¢
<a href="https://axios-http.com/docs/intro"><b>Documentation</b></a>
</p>
<div align="center">
[](https://www.npmjs.org/package/axios)
[](https://cdnjs.com/libraries/axios)
[](https://github.com/axios/axios/actions/workflows/ci.yml)
[](https://gitpod.io/#https://github.com/axios/axios)
[](https://coveralls.io/r/mzabriskie/axios)
[](https://packagephobia.now.sh/result?p=axios)
[](https://bundlephobia.com/package/axios@latest)
[](https://npm-stat.com/charts.html?package=axios)
[](https://gitter.im/mzabriskie/axios)
[](https://www.codetriage.com/axios/axios)
[](https://snyk.io/test/npm/axios)
</div>
## Table of Contents
- [Features](#features)
- [Browser Support](#browser-support)
- [Installing](#installing)
- [Package manager](#package-manager)
- [CDN](#cdn)
- [Example](#example)
- [Axios API](#axios-api)
- [Request method aliases](#request-method-aliases)
- [Concurrency ð](#concurrency-deprecated)
- [Creating an instance](#creating-an-instance)
- [Instance methods](#instance-methods)
- [Request Config](#request-config)
- [Response Schema](#response-schema)
- [Config Defaults](#config-defaults)
- [Global axios defaults](#global-axios-defaults)
- [Custom instance defaults](#custom-instance-defaults)
- [Config order of precedence](#config-order-of-precedence)
- [Interceptors](#interceptors)
- [Multiple Interceptors](#multiple-interceptors)
- [Handling Errors](#handling-errors)
- [Cancellation](#cancellation)
- [AbortController](#abortcontroller)
- [CancelToken ð](#canceltoken-deprecated)
- [Using application/x-www-form-urlencoded format](#using-applicationx-www-form-urlencoded-format)
- [URLSearchParams](#urlsearchparams)
- [Query string](#query-string-older-browsers)
- [ð Automatic serialization](#-automatic-serialization-to-urlsearchparams)
- [Using multipart/form-data format](#using-multipartform-data-format)
- [FormData](#formdata)
- [ð Automatic serialization](#-automatic-serialization-to-formdata)
- [Files Posting](#files-posting)
- [HTML Form Posting](#-html-form-posting-browser)
- [ð Progress capturing](#-progress-capturing)
- [ð Rate limiting](#-progress-capturing)
- [Semver](#semver)
- [Promises](#promises)
- [TypeScript](#typescript)
- [Resources](#resources)
- [Credits](#credits)
- [License](#license)
## Features
- Make [XMLHttpRequests](https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest) from the browser
- Make [http](https://nodejs.org/api/http.html) requests from node.js
- Supports the [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) API
- Intercept request and response
- Transform request and response data
- Cancel requests
- Automatic transforms for [JSON](https://www.json.org/json-en.html) data
- ð Automatic data object serialization to `multipart/form-data` and `x-www-form-urlencoded` body encodings
- Client side support for protecting against [XSRF](https://en.wikipedia.org/wiki/Cross-site_request_forgery)
## Browser Support
 |  |  |  |  |  |
--- | --- | --- | --- | --- | --- |
Latest â | Latest â | Latest â | Latest â | Latest â | 11 â |
[](https://saucelabs.com/u/axios)
## Installing
### Package manager
Using npm:
```bash
$ npm install axios
```
Using bower:
```bash
$ bower install axios
```
Using yarn:
```bash
$ yarn add axios
```
Using pnpm:
```bash
$ pnpm add axios
```
Once the package is installed, you can import the library using `import` or `require` approach:
```js
import axios, {isCancel, AxiosError} from 'axios';
```
You can also use the default export, since the named export is just a re-export from the Axios factory:
```js
import axios from 'axios';
console.log(axios.isCancel('something'));
````
If you use `require` for importing, **only default export is available**:
```js
const axios = require('axios');
console.log(axios.isCancel('something'));
```
For cases where something went wrong when trying to import a module into a custom or legacy environment,
you can try importing the module package directly:
```js
const axios = require('axios/dist/browser/axios.cjs'); // browser commonJS bundle (ES2017)
// const axios = require('axios/dist/node/axios.cjs'); // node commonJS bundle (ES2017)
```
### CDN
Using jsDelivr CDN (ES5 UMD browser module):
```html
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/axios.min.js"></script>
```
Using unpkg CDN:
```html
<script src="https://unpkg.com/[email protected]/dist/axios.min.js"></script>
```
## Example
> **Note** CommonJS usage
> In order to gain the TypeScript typings (for intellisense / autocomplete) while using CommonJS imports with `require()`, use the following approach:
```js
import axios from 'axios';
//const axios = require('axios'); // legacy way
// Make a request for a user with a given ID
axios.get('/user?ID=12345')
.then(function (response) {
// handle success
console.log(response);
})
.catch(function (error) {
// handle error
console.log(error);
})
.finally(function () {
// always executed
});
// Optionally the request above could also be done as
axios.get('/user', {
params: {
ID: 12345
}
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
})
.finally(function () {
// always executed
});
// Want to use async/await? Add the `async` keyword to your outer function/method.
async function getUser() {
try {
const response = await axios.get('/user?ID=12345');
console.log(response);
} catch (error) {
console.error(error);
}
}
```
> **Note** `async/await` is part of ECMAScript 2017 and is not supported in Internet
> Explorer and older browsers, so use with caution.
Performing a `POST` request
```js
axios.post('/user', {
firstName: 'Fred',
lastName: 'Flintstone'
})
.then(function (response
没有合适的资源?快使用搜索试试~ 我知道了~
Mac下Stable Diffusion一键安装好用
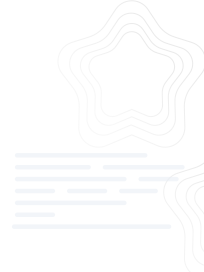
共440个文件
js:211个
pak:58个
md:33个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 133 浏览量
2024-02-05
10:53:01
上传
评论
收藏 90.29MB ZIP 举报
温馨提示
Stable Diffusion 一键安装,国内镜像,无需魔法,Apple Silicon (M1/M2)使用MPS支持GPU,可以根据任何领域的文本输入生成高质量、高分辨率且逼真的图像。 默认选项MAC M1 10秒出图
资源推荐
资源详情
资源评论
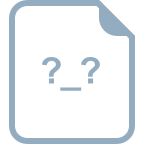
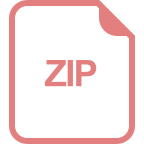
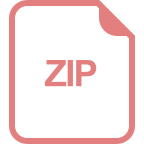
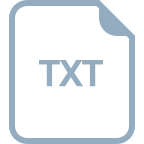
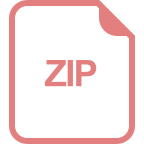
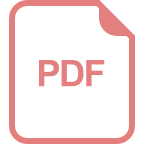
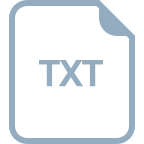
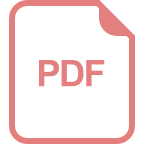
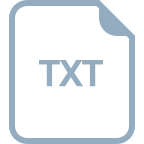
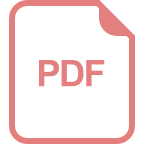
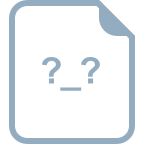
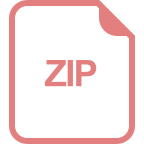
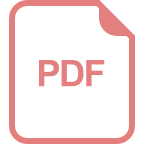
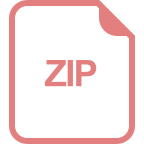
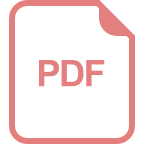
收起资源包目录

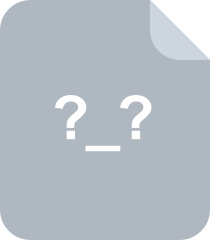
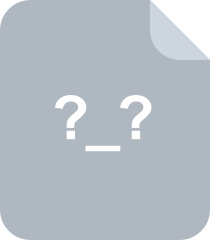
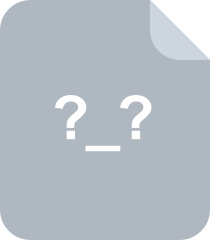
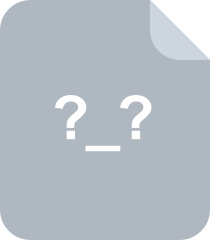
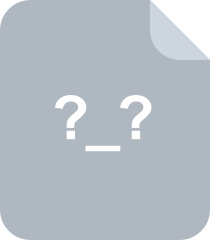
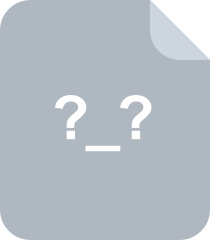
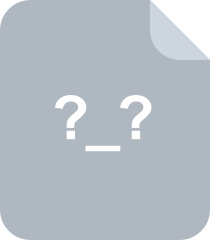
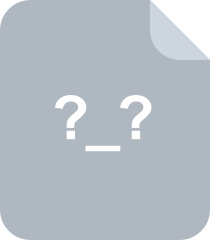
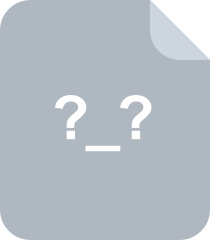
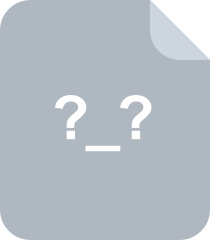
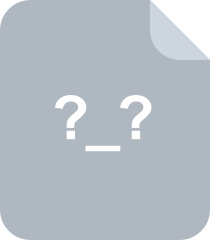
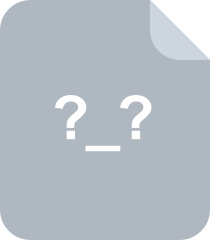
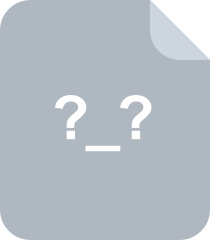
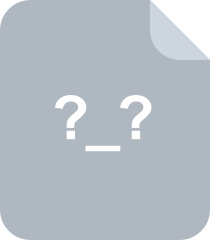
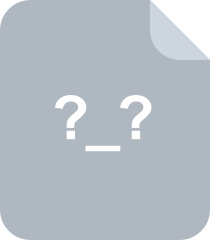
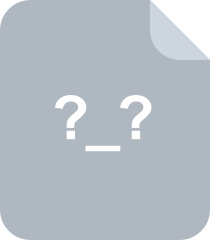
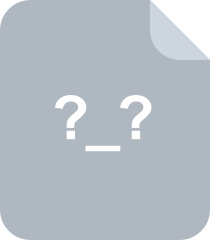
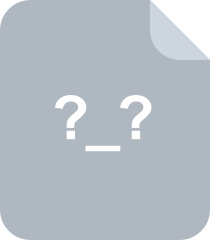
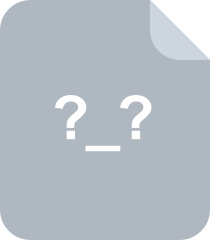
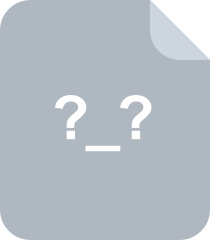
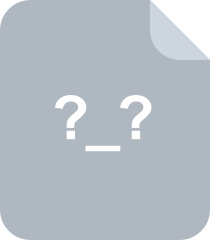
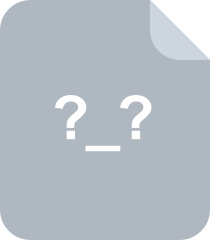
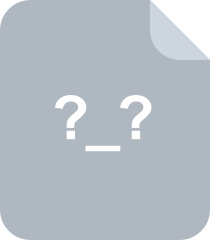
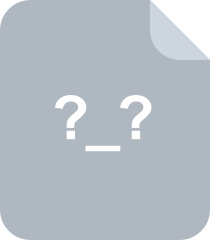
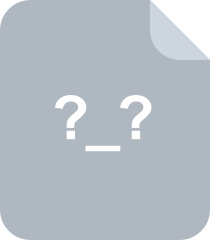
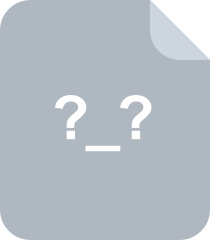
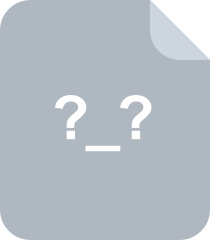
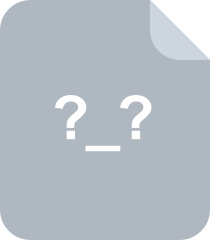
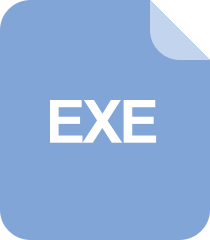
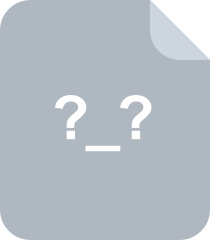
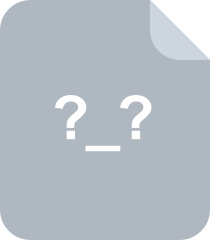
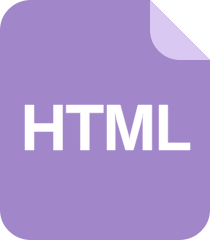
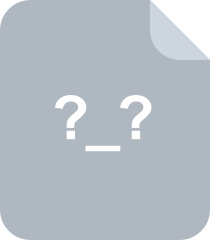
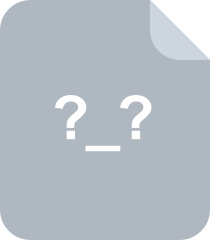
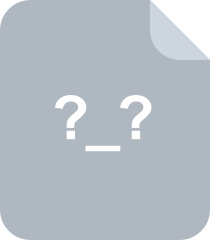
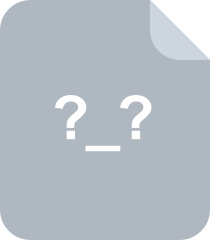
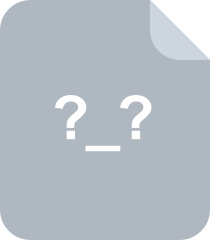
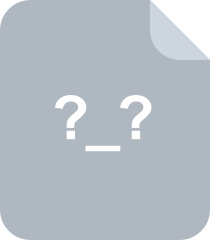
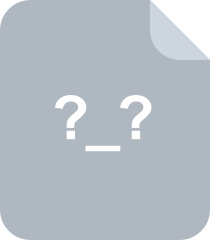
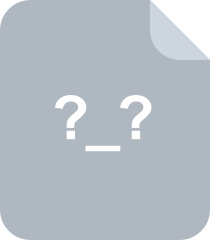
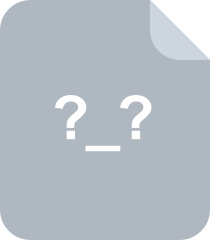
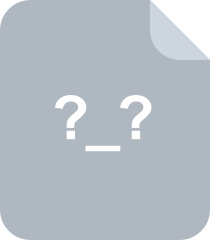
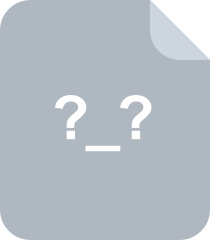
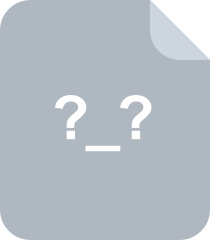
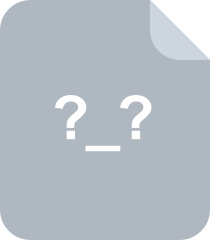
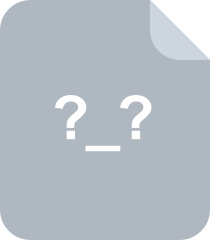
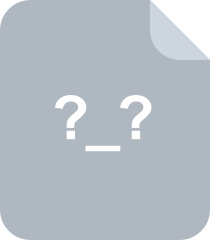
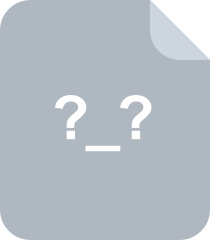
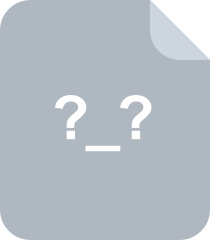
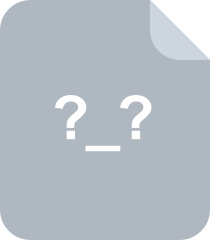
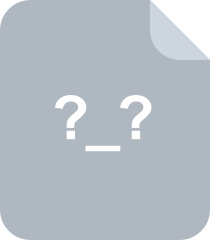
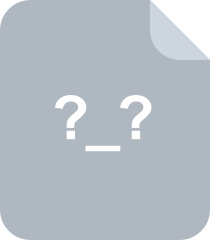
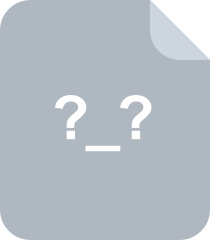
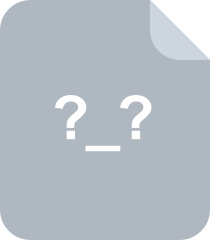
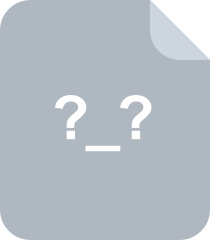
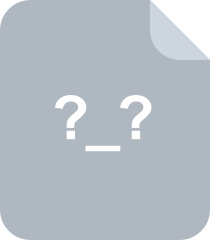
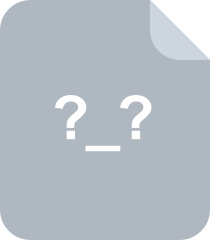
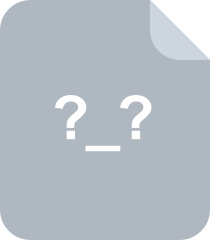
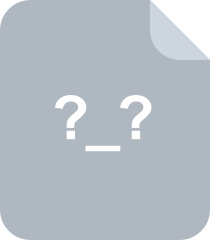
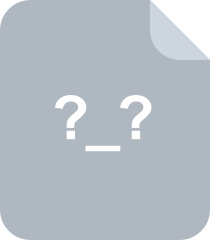
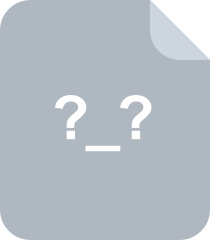
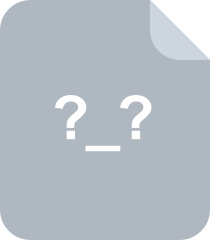
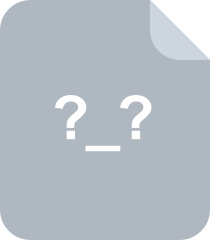
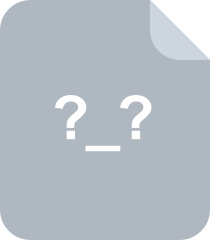
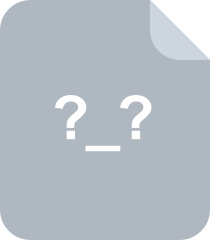
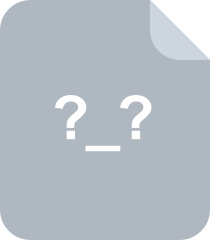
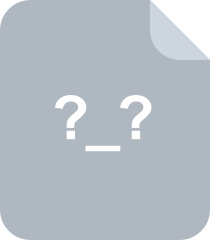
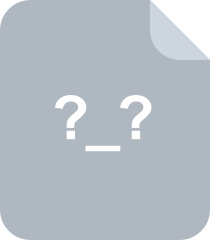
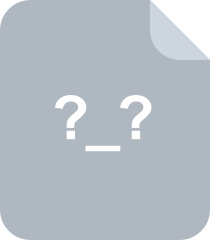
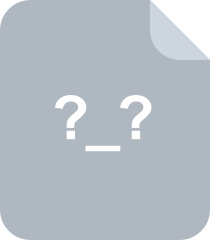
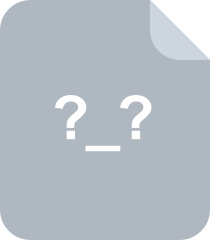
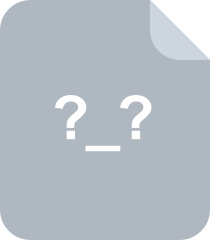
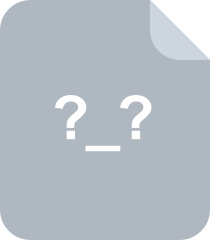
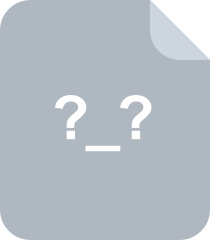
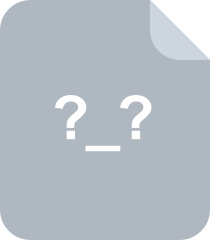
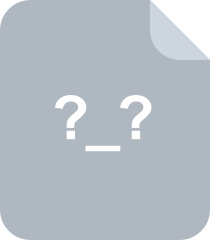
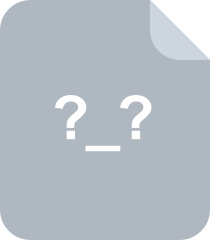
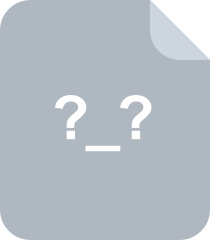
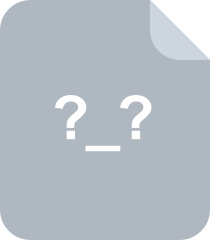
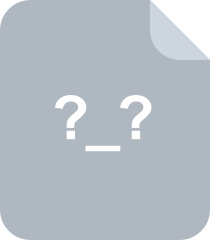
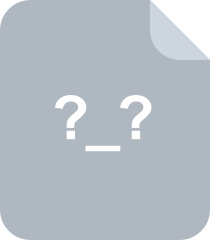
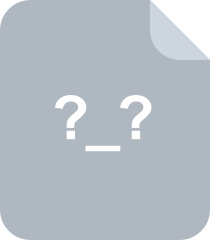
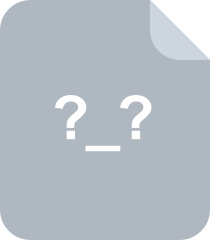
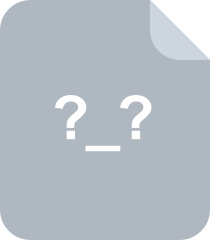
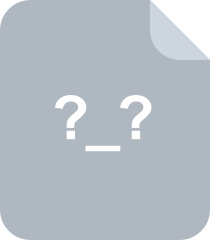
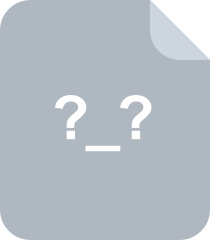
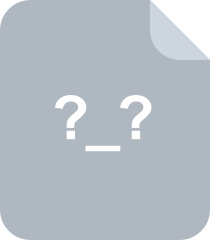
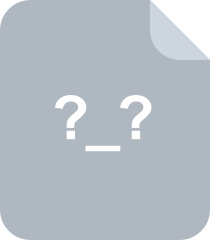
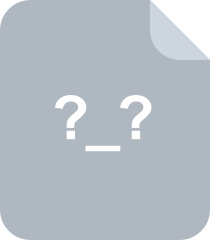
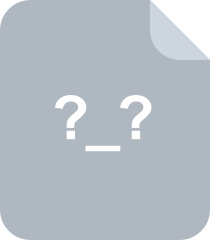
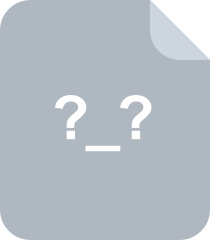
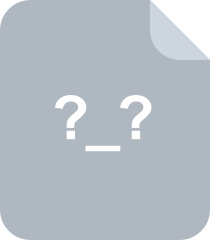
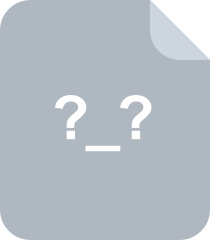
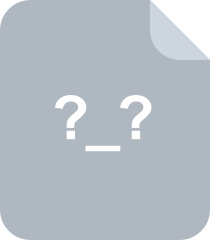
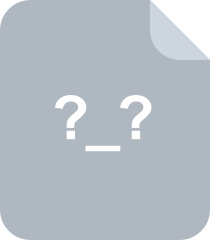
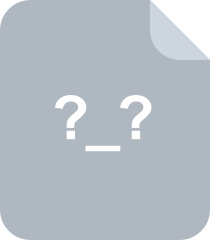
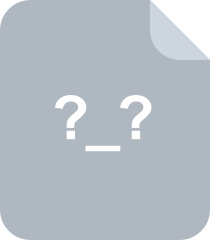
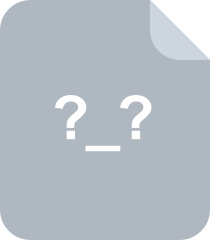
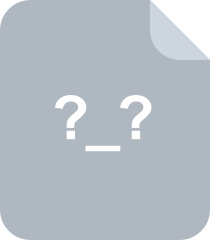
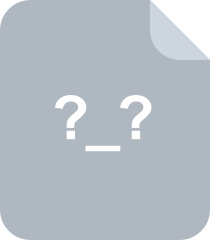
共 440 条
- 1
- 2
- 3
- 4
- 5
资源评论
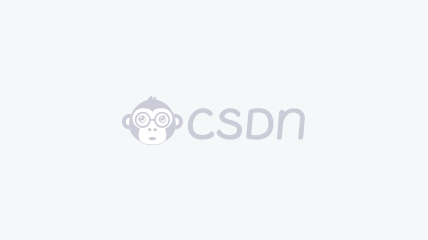


小风飞子
- 粉丝: 329
- 资源: 1511

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

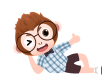
安全验证
文档复制为VIP权益,开通VIP直接复制
