#include <windows.h>
#include <stdint.h>
#include <tlhelp32.h>
#include <stdio.h>
#include <iostream>
#include <vector>
#include <string>
#include <fstream>
#include <stdlib.h>
#include <ctime>
// opencv2
#include <opencv2\core\core.hpp>
#include <opencv2\highgui\highgui.hpp>
#include <opencv2\opencv.hpp>
using namespace std;
using namespace cv;
#define PATH "C:\\Users\\Administrator\\Desktop\\test\\" // 临时文件保存目录
#define MAPSIZE 100
typedef struct EnumHWndsArg
{
std::vector<HWND> *vecHWnds;
DWORD dwProcessId;
}EnumHWndsArg, *LPEnumHWndsArg;
void ReadF(char* str, char* buffer)
{
HANDLE pfile;
pfile = ::CreateFile(str, GENERIC_READ, 0, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, NULL);
if (pfile == INVALID_HANDLE_VALUE)
{
cout << "打开文件失败" << endl;;
CloseHandle(pfile); // 一定注意在函数退出之前对句柄进行释放
return;
}
DWORD filesize = GetFileSize(pfile, NULL);
DWORD readsize;
ReadFile(pfile, buffer, filesize, &readsize, NULL);
buffer[filesize] = 0;
CloseHandle(pfile); // 关闭句柄
DeleteFile(str);
}
void WriteF(char* str, const char* buffer, int size)
{
HANDLE pfile;
pfile = ::CreateFile(str, GENERIC_WRITE | GENERIC_READ, 0,
NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_HIDDEN | FILE_FLAG_WRITE_THROUGH, NULL);
if (pfile == INVALID_HANDLE_VALUE)
{
cout << "打开文件失败" << endl;;
CloseHandle(pfile); // 一定注意在函数退出之前对句柄进行释放
return;
}
DWORD readsize;
WriteFile(pfile, buffer, size, &readsize, NULL);
CloseHandle(pfile); // 关闭句柄
DeleteFile(str);
}
//通过进程ID获取进程句柄
HANDLE GetProcessHandleByID(int nID)
{
return OpenProcess(PROCESS_ALL_ACCESS, FALSE, nID);
}
// 通过应用程序名称得到进程ID
DWORD GetProcessIDByName(const char* pName)
{
HANDLE hSnapshot = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, 0);
if (INVALID_HANDLE_VALUE == hSnapshot) {
return NULL;
}
PROCESSENTRY32 pe = { sizeof(pe) };
for (BOOL ret = Process32First(hSnapshot, &pe); ret; ret = Process32Next(hSnapshot, &pe)) {
if (strcmp((const char*)pe.szExeFile, pName) == 0) {
CloseHandle(hSnapshot);
return pe.th32ProcessID;
}
//printf("%-6d %s\n", pe.th32ProcessID, pe.szExeFile);
}
CloseHandle(hSnapshot);
return 0;
}
BOOL CALLBACK lpEnumFunc(HWND hwnd, LPARAM lParam)
{
EnumHWndsArg *pArg = (LPEnumHWndsArg)lParam;
DWORD processId;
GetWindowThreadProcessId(hwnd, &processId);
if (processId == pArg->dwProcessId)
{
pArg->vecHWnds->push_back(hwnd);
//printf("%p\n", hwnd);
}
return TRUE;
}
// 对指定句柄窗口进行截屏操作
void ShootScreen(const char* filename, HWND hWnd)
{
HDC hdc = CreateDC("DISPLAY", NULL, NULL, NULL);
int32_t ScrWidth = 0, ScrHeight = 0;
RECT rect = { 0 };
if (hWnd == NULL)
{
ScrWidth = GetDeviceCaps(hdc, HORZRES);
ScrHeight = GetDeviceCaps(hdc, VERTRES);
}
else
{
GetWindowRect(hWnd, &rect);
rect.left += 8;
rect.right -= 8;
rect.bottom -= 8;
rect.top += 1;
ScrWidth = rect.right - rect.left;
ScrHeight = rect.bottom - rect.top;
}
HDC hmdc = CreateCompatibleDC(hdc);
HBITMAP hBmpScreen = CreateCompatibleBitmap(hdc, ScrWidth, ScrHeight);
HBITMAP holdbmp = (HBITMAP)SelectObject(hmdc, hBmpScreen);
BITMAP bm;
GetObject(hBmpScreen, sizeof(bm), &bm);
BITMAPINFOHEADER bi = { 0 };
bi.biSize = sizeof(BITMAPINFOHEADER);
bi.biWidth = bm.bmWidth;
bi.biHeight = bm.bmHeight;
bi.biPlanes = bm.bmPlanes;
bi.biBitCount = bm.bmBitsPixel;
bi.biCompression = BI_RGB;
bi.biSizeImage = bm.bmHeight * bm.bmWidthBytes;
// 图片的像素数据
char *buf = new char[bi.biSizeImage];
BitBlt(hmdc, 0, 0, ScrWidth, ScrHeight, hdc, rect.left, rect.top, SRCCOPY);
GetDIBits(hmdc, hBmpScreen, 0L, (DWORD)ScrHeight, buf, (LPBITMAPINFO)&bi, (DWORD)DIB_RGB_COLORS);
BITMAPFILEHEADER bfh = { 0 };
bfh.bfType = ((WORD)('M' << 8) | 'B');
bfh.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + bi.biSizeImage;
bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER);
HANDLE hFile = CreateFile(filename, GENERIC_WRITE, 0, 0, OPEN_ALWAYS, FILE_ATTRIBUTE_NORMAL, 0);
DWORD dwWrite;
WriteFile(hFile, &bfh, sizeof(BITMAPFILEHEADER), &dwWrite, NULL);
WriteFile(hFile, &bi, sizeof(BITMAPINFOHEADER), &dwWrite, NULL);
WriteFile(hFile, buf, bi.biSizeImage, &dwWrite, NULL);
CloseHandle(hFile);
hBmpScreen = (HBITMAP)SelectObject(hmdc, holdbmp);
}
// 通过进程号得到当前进程中的所有窗口句柄
void GetHWndsByProcessID(DWORD processID, std::vector<HWND> &vecHWnds)
{
EnumHWndsArg wi;
wi.dwProcessId = processID;
wi.vecHWnds = &vecHWnds;
EnumWindows(lpEnumFunc, (LPARAM)&wi);
}
// 得到程序主窗口
// 主要是根据窗口的大小和窗口的可见性进筛选
HWND getMainHWND(vector<HWND> &vec)
{
HWND res;
int size = 0;
for (auto &hdc : vec)
{
if (!IsWindowVisible(hdc)) continue;
LPRECT lrt = new tagRECT();
GetWindowRect(hdc, lrt);
int h = lrt->bottom - lrt->top;
int w = lrt->right - lrt->left;
if (h * w > size)
{
size = h * w;
res = hdc;
}
}
return res;
}
// 模拟鼠标左键点击
void leftClickScreen(int x, int y)
{
SetCursorPos(x, y);
mouse_event(MOUSEEVENTF_LEFTDOWN, 0, 0, 0, 0);
mouse_event(MOUSEEVENTF_LEFTUP, 0, 0, 0, 0);
return;
}
// 模拟鼠标右键点击
void rightClickScreen(int x, int y)
{
SetCursorPos(x, y);
mouse_event(MOUSEEVENTF_RIGHTDOWN, 0, 0, 0, 0);
mouse_event(MOUSEEVENTF_RIGHTUP, 0, 0, 0, 0);
return;
}
void initMap(vector<vector<int>> &mp, int h, int w)
{
// -1 未点击
// 0 空地
// >0 炸弹数量
// -2 炸弹
mp = vector<vector<int>>(h, vector<int>(w, -1));
}
// 返回true 代表踩到地雷结束游戏
// 根据图片更新二维矩阵
bool updataMap(
vector<vector<int>> &mp,
string path,
int bx,
int by,
int height,
int width,
int cellheight,
int cellwidth
)
{
Mat image = imread(path);
/*for (int i = 1; i <= height; i++)
{
Point beginPoint(0 + bx, i * cellheight + by);
Point endPoint(width * cellwidth + bx, i * cellheight + by);
line(image, beginPoint, endPoint, Scalar(0, 0, 0));
}
for (int i = 1; i <= width; i++)
{
Point beginPoint(i * cellwidth + bx, 0 + by);
Point endPoint(i * cellwidth + bx, height * cellheight + by);
line(image, beginPoint, endPoint, Scalar(0, 0, 0));
}
*/
for (int i = 0; i < height; i++)
{
for (int j = 0; j < width; j++)
{
Point point(j * cellwidth + bx + cellwidth / 2, i * cellheight + by + cellheight / 2);
Point point1(j * cellwidth + bx + 1, i * cellheight + by + 3);
//circle(image, point, 2, Scalar(100, 100, 100));
auto it = image.at<Vec3b>(point);
int r = it[0], g = it[1], b = it[2];
//printf("(%d: r = %d, g = %d, b = %d)", i * width + j, r, g, b);
if (r == 0 && g == 0 && b == 0) return true;
if (r == 192 && g == 192 && b == 192){
it = image.at<Vec3b>(point1);
r = it[0], g = it[1], b = it[2];
//printf("(r = %d, g = %d, b = %d)", r, g, b);
if (r == 192) mp[i][j] = 0;
}
else
{
if (r == 255 && g == 0 && b == 0) mp[i][j] = 1;
else if (r == 0 && g == 128 && b == 0) mp[i][j] = 2;
else if (r == 0 && g == 0 && b == 255) mp[i][j] = 3;
else if (r == 128 && g == 0 && b == 0) mp[i][j] = 4;
else if (r == 0 && g == 0 && b == 128) mp[i][j] = 5;
else mp[i][j] = 6;
}
//printf("\n");
}
}
for (int i = 0; i < height; i++)
{
for (int j = 0; j < width; j++)
{
printf("%d ", mp[i][j]);
}
printf("\n");
}
printf("\n");
imshow("image", image);
waitKey(1);
return false;
}
/*
[gamename] 扫雷的名称
[bx] 第一个小单元格相对于扫雷界面横坐标的偏移
[by] 第一个小单元格相对于扫雷界面纵坐标的偏移
[height] 地图大小(高度)
[width] 地图大小(宽度)
[cellHeight] 小单元格的高度
[cellWidth] 小单元格的宽度
*/
bool playGame(
string gamename,
int bx,
int by,
int height,
int width,
int cellHeight,
int cellWidth
)
{
DWORD pid = GetPro

特立独行的猪鸭
- 粉丝: 98
- 资源: 8
最新资源
- Проекты и скрипты.zip
- 公开整理-中国各省市级信用体系建设匹配数据集(2010-2024).xls
- [한빛미디어]“与파스트다和파스썬”전체소스코드저장소입니다 .zip
- 汽车行业车载网络安全认证协议 UDS Service 29 解析与应用
- .raw 文件打开方式.pdf
- 760964449620474KivaIxaBeltAllRiderSeries_1.1_apkcombo.com.apk
- 开源的跨平台计算机视觉库opencv-4.10.0-windows
- qt-opensource-windows-x86-msvc2013-5.6.3.rar
- 基于 crossbeam-channel + JNI 实现 Java 与 Rust 的消息传递
- 酒店管理客房管理系统源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


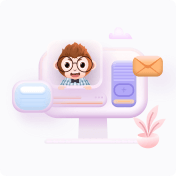