#include "downwidget.h"
downWidget::downWidget(QWidget *parent) : QWidget(parent)
{
x = 50, y = 50;
n = m = 1;
this->setStyleSheet("background: #ada");
setFocusPolicy(Qt::StrongFocus ); //使得键盘控制此窗口
ball = new QLabel(this); //球
ball->resize(50, 50);
ball->move(100, 100);
ball->setStyleSheet("background-color: red; border-radius: 25px;");
// bat = new QPushButton(this); //底板 换成Qlabel可以??????
bat = new QLabel(this); //底板
bat->resize(70, 40);
bat->move(200, 300);
bat->setStyleSheet("background-color: green; border-radius: 5px;");
timer = new QTimer(this);
timer->start(10);
connect(timer, SIGNAL(timeout()), this, SLOT(mymove()));
}
void downWidget::mymove(){ //球移动
slottime();
bat->setGeometry(this->bat->x(), this->height() - 40, 100, 40); //动态获取设置bat:位置, 大小
if(x < 0 || x > this->width() - 50) //左右相撞
n = -n;
if(y < 0) // 与上面相撞
m = -m;
if(this->ball->y() + this->ball->height() >= this->bat->y() && this->ball->x() + 25 > this->bat->x()
&& this->ball->x() + 25 < this->bat->x() + this->bat->width()){ //与底板相撞
m = -m;
slotClick();
}
if(this->ball->y() > this->height()){ //没接住,则关闭窗口,结束游戏
this->close();
this->slotgameover();
this->timer->stop();
}
x += n; y += m;
this->ball->move(x, y);
}
void downWidget::slotClick(){ //转化信号
emit signalScore(); //发出信号
}
void downWidget::slottime(){
emit signaltime();
}
void downWidget::slotgameover(){
emit signalgameover();
}
void downWidget::paintEvent(QPaintEvent *e){ //使子窗口可以调色
QStyleOption opt;
opt.init(this);
QPainter p(this);
style()->drawPrimitive(QStyle::PE_Widget, &opt, &p, this);
}
void downWidget::keyPressEvent(QKeyEvent *event){
qDebug() << event->key(); //输出所按键
if(event->key() == Qt::Key_A && this->bat->x() >= 10) //a = 65, 按下a左移 // 移动的同时保证木块不移出去
this->bat->move(this->bat->x() - 10, this->bat->y());
if(event->key() == Qt::Key_D && this->bat->x() + this->bat->width() + 5 <= this->width()) //d = 68,按d右移
this->bat->move(this->bat->x() + 10, this->bat->y());
if(event->key() == Qt::Key_Left && this->bat->x() > 5)
this->bat->move(this->bat->x() - 10, this->bat->y());
if(event->key() == Qt::Key_Right && this->bat->x() + this->bat->width() < this->width() - 5)
this->bat->move(this->bat->x() + 10, this->bat->y());
}

ystraw_ah
- 粉丝: 443
- 资源: 51
最新资源
- 立方体、球体、金字塔检测26-YOLO(v5至v11)、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 施耐德电气-KNX-智系列智能家居解决方案20170303.pptx
- 立方体检测30-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 施耐德资料 智能家居 EIBA ETS3安装软件.rar
- VID_20241224_175323.mp4
- 1004202304027刘政阳.zip
- 立方体检测6-YOLO(v5至v11)、COCO、TFRecord、VOC数据集合集.rar
- java基础代码,练手啦,兄弟们!
- 施耐德资料 KNX ETS4-1-5-Setup.rar
- 汽车站售票系统.mdf
- 笔、水杯检测16-YOLO(v7至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 基于node的nodejs电影交流网站源代码(完整前后端+mysql+说明文档+LW).zip
- 基于node的nodejs学院会议纪要管理系统源代码(完整前后端+mysql+说明文档).zip
- 筛子检测23-YOLO(v5至v11)、CreateML、Paligemma、TFRecord数据集合集.rar
- 基于node的在线跑腿系统源代码(完整前后端+mysql+说明文档+LW).zip
- Java(Android开发):异常处理与调试全面解析
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


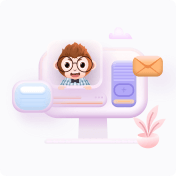