[简体中文](https://github.com/amisadmin/fastapi_amis_admin/blob/master/README.zh.md)
| [English](https://github.com/amisadmin/fastapi_amis_admin)
# Introduction
<h2 align="center">
FastAPI-Amis-Admin
</h2>
<p align="center">
<em>fastapi-amis-admin is a high-performance, efficient and easily extensible FastAPI admin framework.</em><br/>
<em>Inspired by Django-admin, and has as many powerful functions as Django-admin.</em>
</p>
<p align="center">
<a href="https://github.com/amisadmin/fastapi_amis_admin/actions/workflows/pytest.yml" target="_blank">
<img src="https://github.com/amisadmin/fastapi_amis_admin/actions/workflows/pytest.yml/badge.svg" alt="Pytest">
</a>
<a href="https://pypi.org/project/fastapi_amis_admin" target="_blank">
<img src="https://badgen.net/pypi/v/fastapi-amis-admin?color=blue" alt="Package version">
</a>
<a href="https://pepy.tech/project/fastapi-amis-admin" target="_blank">
<img src="https://pepy.tech/badge/fastapi-amis-admin" alt="Downloads">
</a>
<a href="https://gitter.im/amisadmin/fastapi-amis-admin">
<img src="https://badges.gitter.im/amisadmin/fastapi-amis-admin.svg" alt="Chat on Gitter"/>
</a>
</p>
<p align="center">
<a href="https://github.com/amisadmin/fastapi_amis_admin" target="_blank">source code</a>
·
<a href="http://demo.amis.work/admin" target="_blank">online demo</a>
·
<a href="http://docs.gh.amis.work" target="_blank">documentation</a>
·
<a href="http://docs.amis.work" target="_blank">can't open the document?</a>
</p>
------
`fastapi-amis-admin` is a high-performance and efficient framework based on `fastapi` & `amis` with `Python 3.7+`, and
based on standard Python type hints. The original intention of the development is to improve the application ecology and
to quickly generate a visual dashboard for the web application . According to the `Apache2.0` protocol, it is free and
open source . But in order to better operate and maintain this project in the long run, I very much hope to get
everyone's sponsorship and support.
## Features
- **High performance**: Based on [FastAPI](https://fastapi.tiangolo.com/). Enjoy all the benefits.
- **High efficiency**: Perfect code type hints. Higher code reusability.
- **Support asynchronous and synchronous hybrid writing**: `ORM` is based on`SQLModel` & `Sqlalchemy`. Freely customize
database type. Support synchronous and asynchronous mode. Strong scalability.
- **Front-end separation**: The front-end is rendered by `Amis`, the back-end interface is automatically generated
by `fastapi-amis-admin`. The interface is reusable.
- **Strong scalability**: The background page supports `Amis` pages and ordinary `html` pages. Easily customize the
interface freely.
- **Automatic api documentation**: Automatically generate Interface documentation by `FastAPI`. Easily debug and share
interfaces.
## Dependencies
- [FastAPI](https://fastapi.tiangolo.com/): Finish the web part.
- [SQLModel](https://sqlmodel.tiangolo.com/): Finish `ORM` model mapping. Perfectly
combine [SQLAlchemy](https://www.sqlalchemy.org/) with [Pydantic](https://pydantic-docs.helpmanual.io/), and have all
their features .
- [Amis](https://baidu.gitee.io/amis): Finish admin page presentation.
## Composition
`fastapi-amis-admin` consists of three core modules, of which, `amis`, `fastapi-sqlmodel-crud` can be used as separate
modules, `amis_admin` is developed by the former.
- `amis`: Based on the `pydantic` data model building library of `baidu amis`. To generate/parse data rapidly.
- `fastapi-sqlmodel-crud`: Based on `FastAPI` &`SQLModel`. To quickly build Create, Read, Update, Delete common API
interface .
- `admin`: Inspired by `Django-Admin`. Combine `amis` with `fastapi-sqlmodel-crud`. To quickly build Web Admin
dashboard .
## Installation
```bash
pip install fastapi_amis_admin
```
## Simple Example
```python
from fastapi import FastAPI
from fastapi_amis_admin.admin.settings import Settings
from fastapi_amis_admin.admin.site import AdminSite
# create FastAPI application
app = FastAPI()
# create AdminSite instance
site = AdminSite(settings=Settings(database_url_async='sqlite+aiosqlite:///amisadmin.db'))
# mount AdminSite instance
site.mount_app(app)
if __name__ == '__main__':
import uvicorn
uvicorn.run(app, debug=True)
```
## ModelAdmin Example
```python
from fastapi import FastAPI
from sqlmodel import SQLModel
from fastapi_amis_admin.admin.settings import Settings
from fastapi_amis_admin.admin.site import AdminSite
from fastapi_amis_admin.admin import admin
from fastapi_amis_admin.models.fields import Field
# create FastAPI application
app = FastAPI()
# create AdminSite instance
site = AdminSite(settings=Settings(database_url_async='sqlite+aiosqlite:///amisadmin.db'))
# Create an SQLModel, see document for details: https://sqlmodel.tiangolo.com/
class Category(SQLModel, table=True):
id: int = Field(default=None, primary_key=True, nullable=False)
name: str = Field(title='CategoryName')
description: str = Field(default='', title='Description')
# register ModelAdmin
@site.register_admin
class CategoryAdmin(admin.ModelAdmin):
page_schema = 'Category'
# set model
model = Category
# mount AdminSite instance
site.mount_app(app)
# create initial database table
@app.on_event("startup")
async def startup():
await site.db.async_run_sync(SQLModel.metadata.create_all, is_session=False)
if __name__ == '__main__':
import uvicorn
uvicorn.run(app, debug=True)
```
## FormAdmin Example
```python
from typing import Any
from fastapi import FastAPI
from pydantic import BaseModel
from starlette.requests import Request
from fastapi_amis_admin.amis.components import Form
from fastapi_amis_admin.admin import admin
from fastapi_amis_admin.admin.settings import Settings
from fastapi_amis_admin.admin.site import AdminSite
from fastapi_amis_admin.crud.schema import BaseApiOut
from fastapi_amis_admin.models.fields import Field
# create FastAPI application
app = FastAPI()
# create AdminSite instance
site = AdminSite(settings=Settings(database_url_async='sqlite+aiosqlite:///amisadmin.db'))
# register FormAdmin
@site.register_admin
class UserLoginFormAdmin(admin.FormAdmin):
page_schema = 'UserLoginForm'
# set form information, optional
form = Form(title='This is a test login form', submitText='login')
# create form schema
class schema(BaseModel):
username: str = Field(..., title='username', min_length=3, max_length=30)
password: str = Field(..., title='password')
# handle form submission data
async def handle(self, request: Request, data: BaseModel, **kwargs) -> BaseApiOut[Any]:
if data.username == 'amisadmin' and data.password == 'amisadmin':
return BaseApiOut(msg='Login successfully!', data={'token': 'xxxxxx'})
return BaseApiOut(status=-1, msg='Incorrect username or password!')
# mount AdminSite instance
site.mount_app(app)
if __name__ == '__main__':
import uvicorn
uvicorn.run(app, debug=True)
```
## Working with Command
```bash
# Install command line extension
pip install fastapi_amis_admin[cli]
# View help
faa --help
# Initialize a `FastAPI-Amis-Admin` project
faa new project_name --init
# Initialize a `FastAPI-Amis-Admin` application
faa new app_name
# Fast running project
faa run
```
## Preview
- Open `http://127.0.0.1:8000/admin/` in your browser:
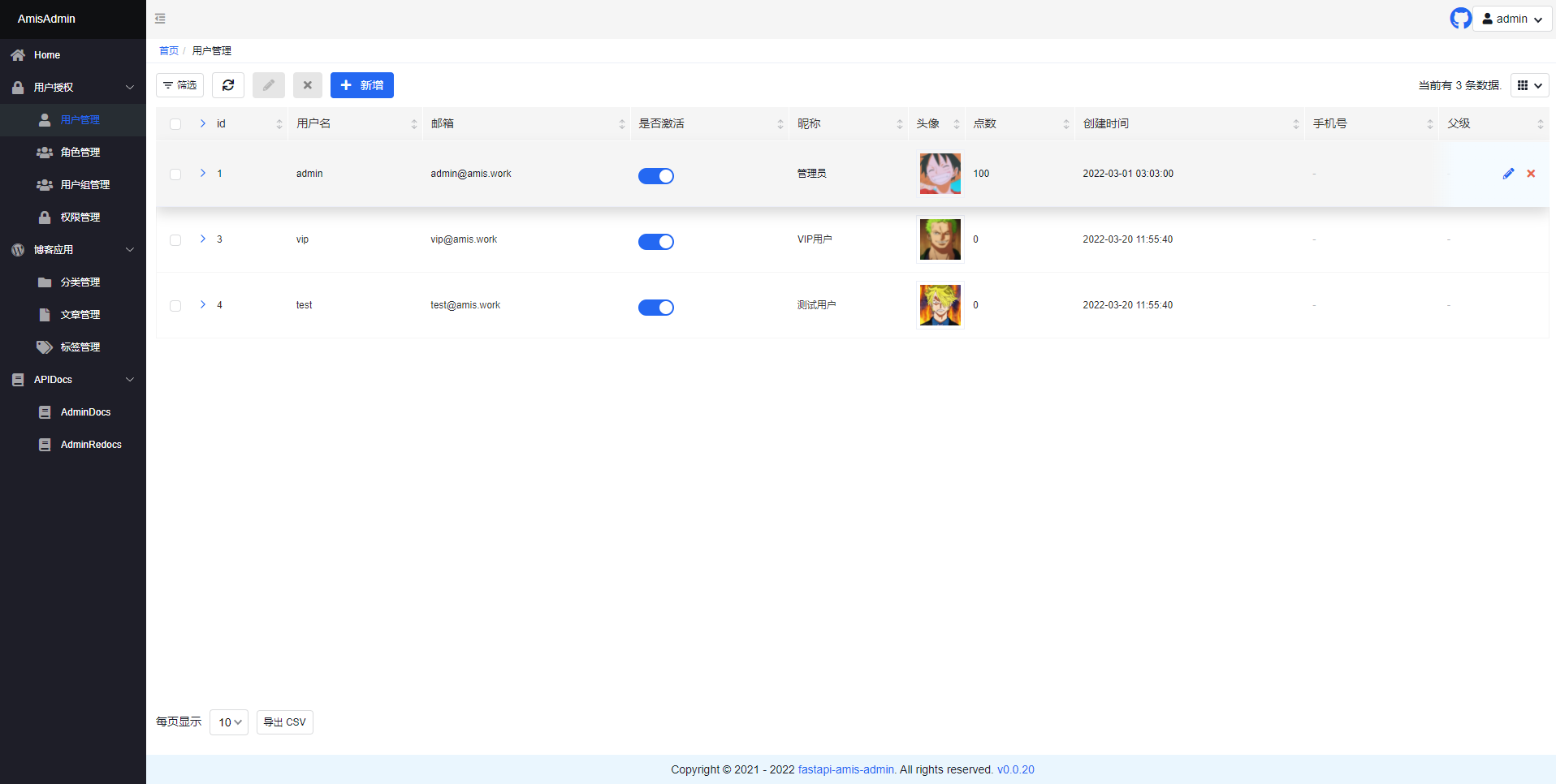
- Open `http://127.0.0.1:8000/admin/docs` in your browser:
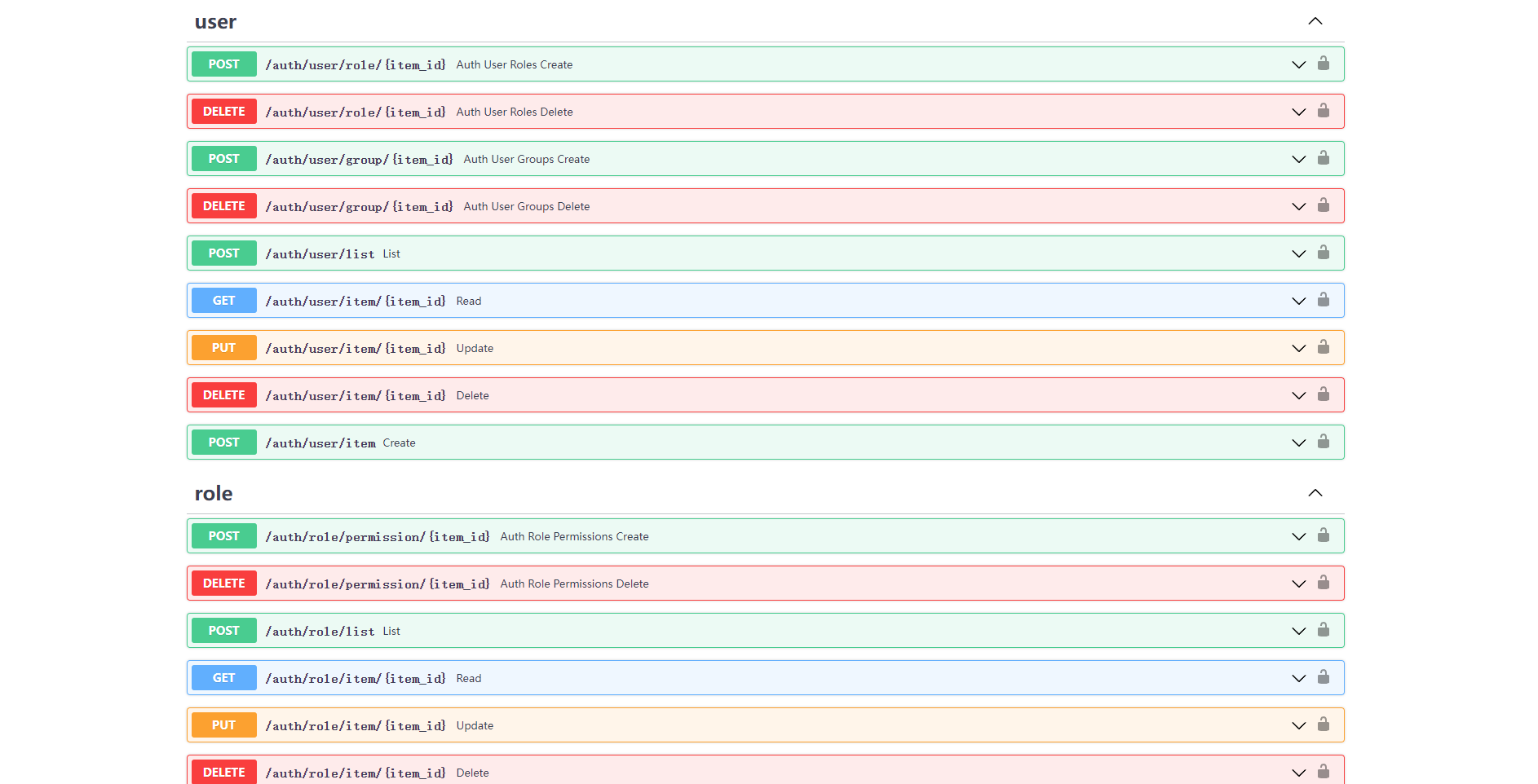
## Project
- [`Amis-Admin-Theme-Editor`](https://github.com/swelcker/amis-admin-theme-editor):Theme-Editor for the fastapi-amis-admin.
Allows to add custom css styles and to apply theme --vars change on the fly.
- [`FastAPI-User-Auth`](https://github.com/amisadmin/fastapi_user_auth): A simple and powerful `FastAPI` user `RB
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
fastapi-amis-admin是一个拥有高性能,高效率,易拓展的fastapi管理后台框架。启发自Django-Admin,并且拥有不逊色于Django-Admin的强大功能。是一个基于fastapi+amis开发的高性能并且高效率 web-admin 框架,使用 Python 3.7+ 并基于标准的 Python 类型提示. fastapi-amis-admin开发的初衷是为了完善fastapi应用生态,为fastapi web应用程序快速生成一个可视化管理后台。 性能极高:基于FastAPI, 可享受FastAPI的全部优势. 效率更快:完善的编码类型提示, 代码可重用性更高. 支持异步和同步混合编写: ORM基于SQLModel+Sqlalchemy, 可自由定制数据库类型, 支持同步及异步模式, 可拓展性强. 前后端分离: 前端由Amis渲染, 后端接口由fastapi-amis-admin自动生成, 接口可重复利用. 可拓展性强: 后台页面支持Amis页面及普通html页面,开发者可以很方便的自由定制界面. 自动生成API文档: 由FastAPI自动生成接口文
资源推荐
资源详情
资源评论
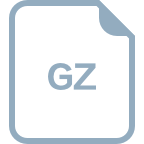
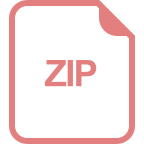
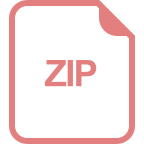
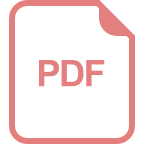
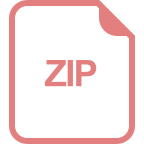
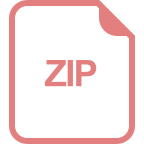
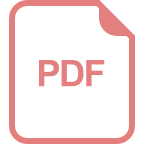
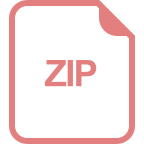
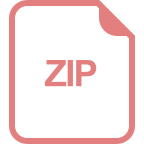
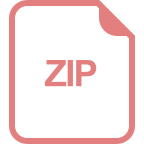
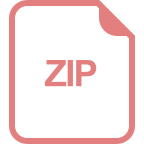
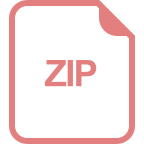
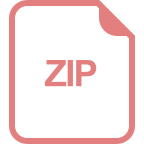
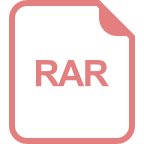
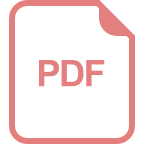
收起资源包目录

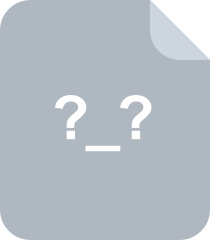
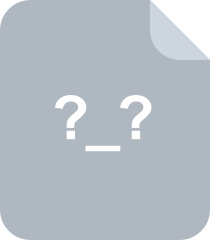
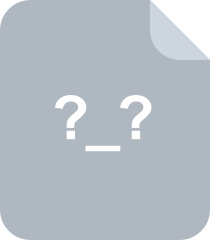
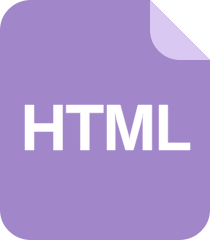
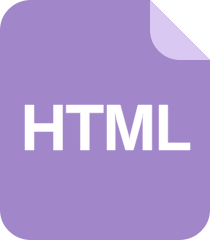
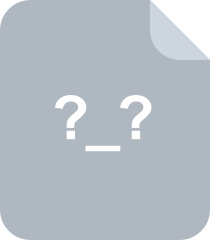
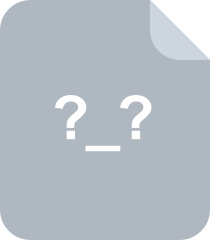
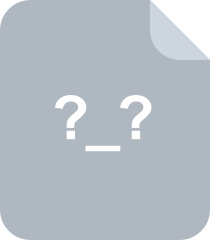
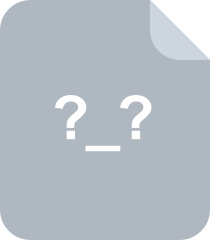
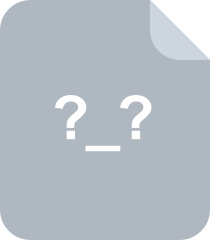
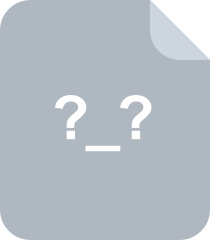
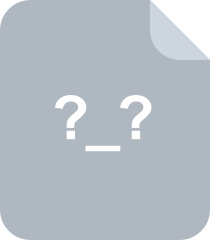
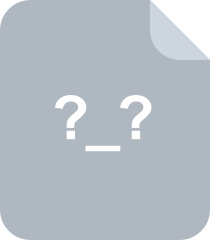
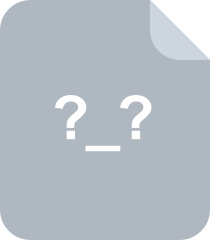
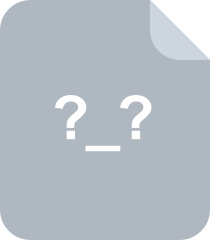
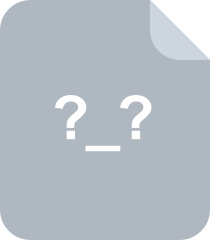
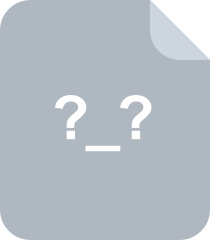
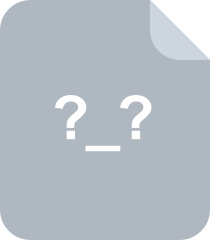
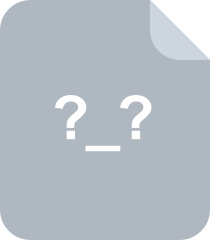
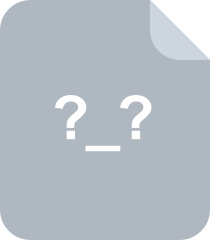
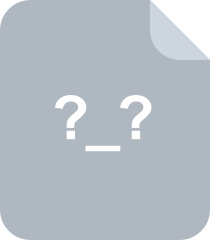
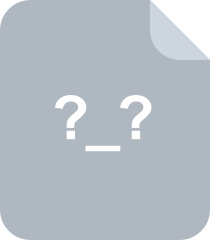
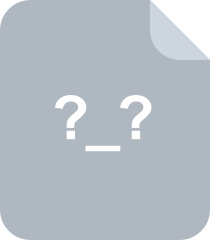
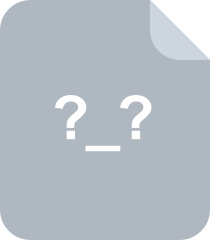
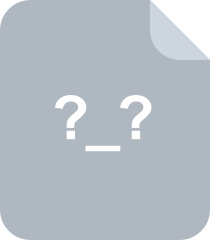
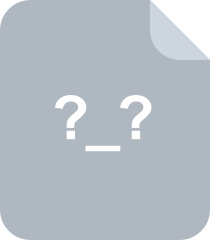
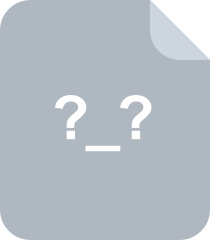
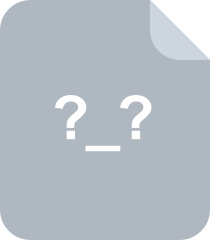
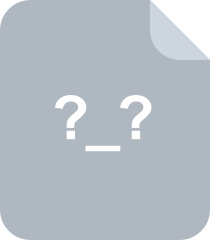
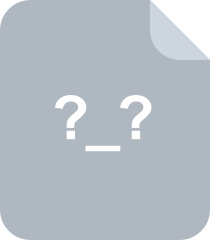
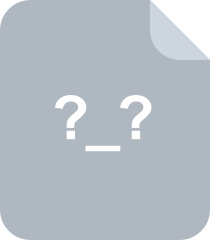
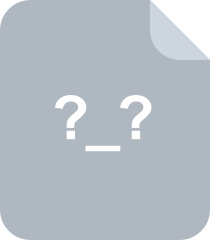
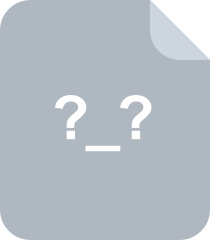
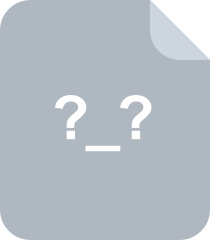
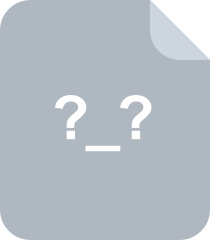
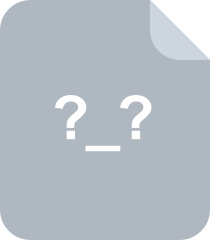
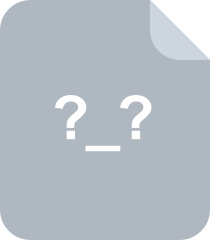
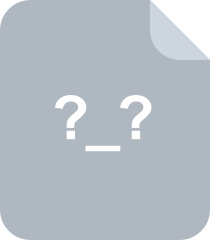
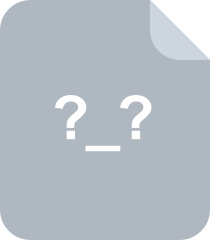
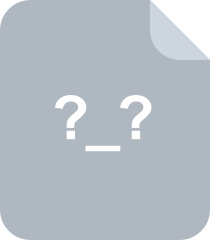
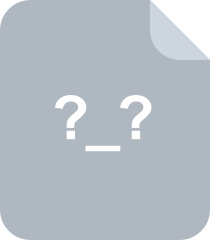
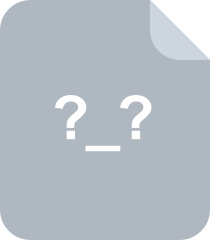
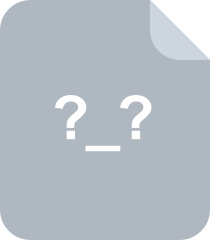
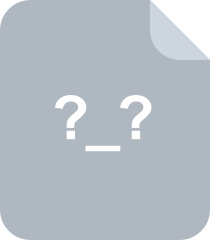
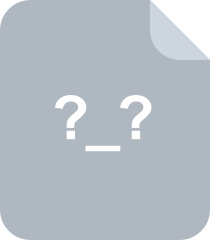
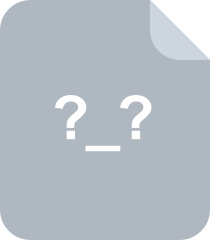
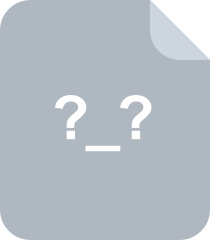
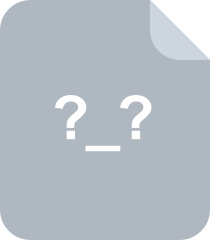
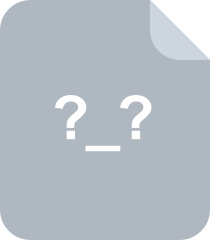
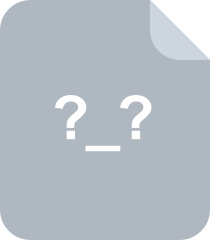
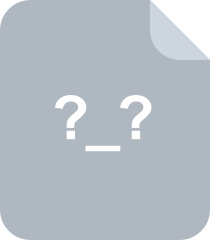
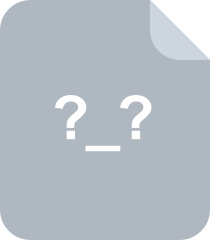
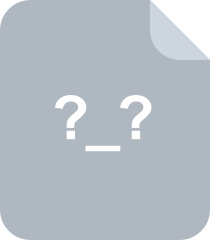
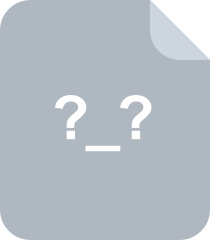
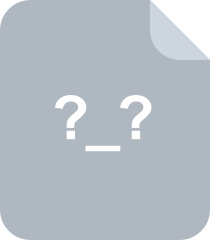
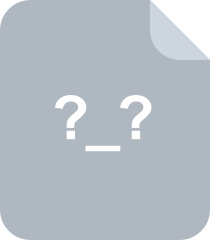
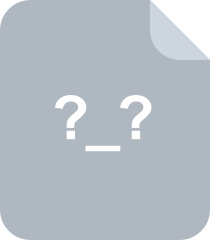
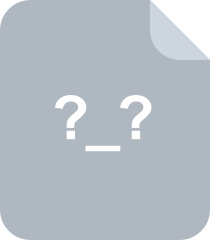
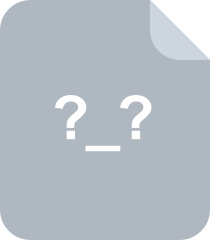
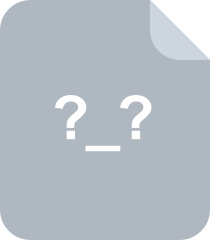
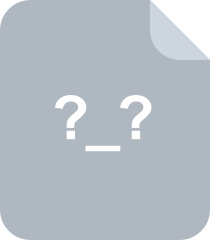
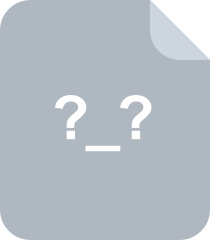
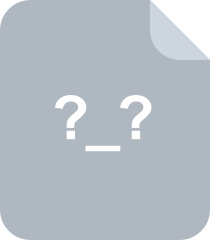
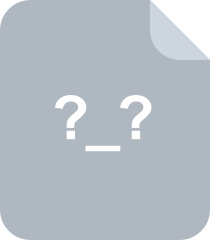
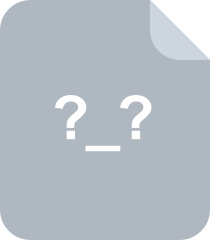
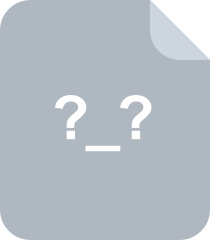
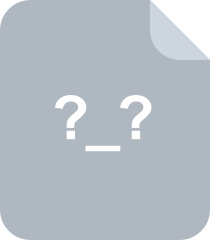
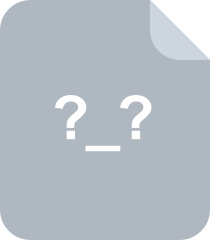
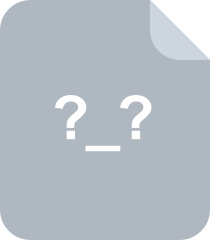
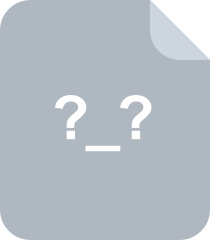
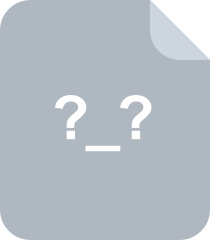
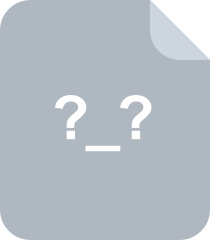
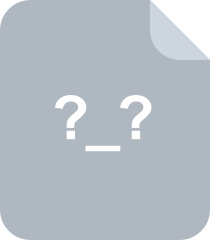
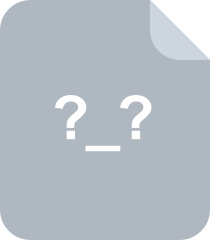
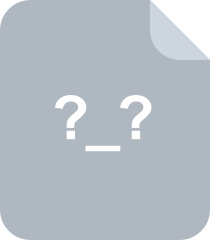
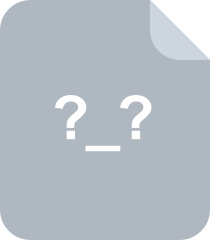
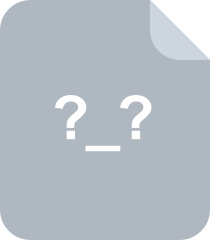
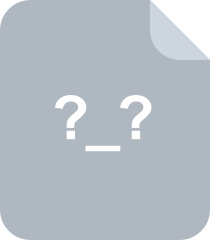
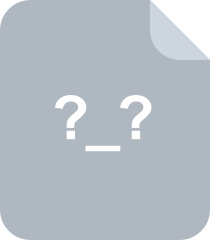
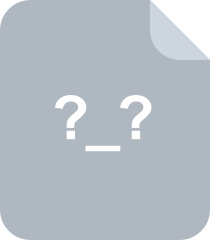
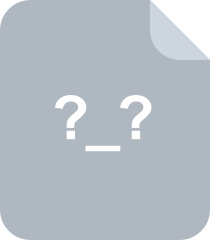
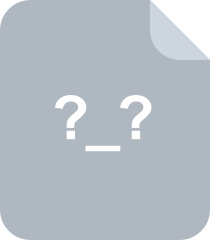
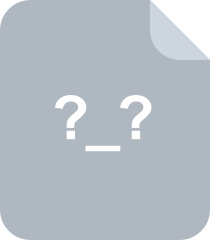
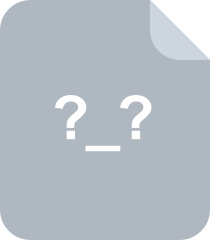
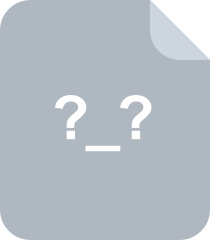
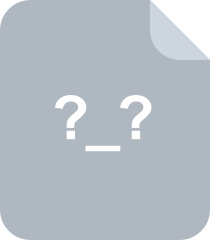
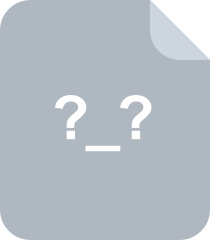
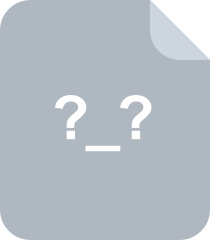
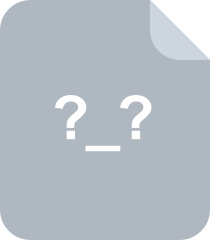
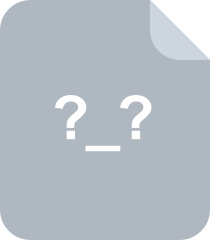
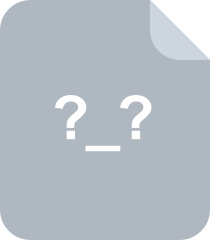
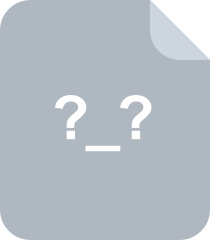
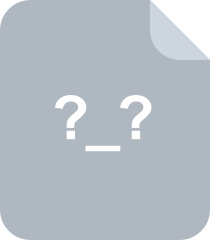
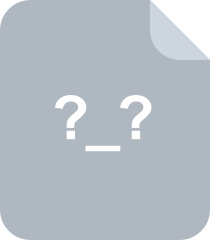
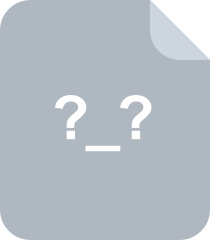
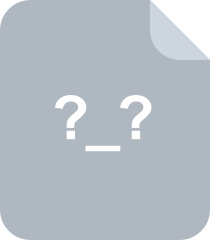
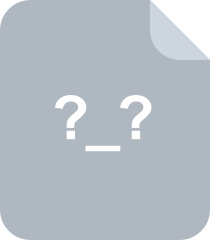
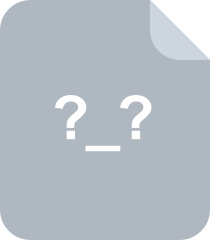
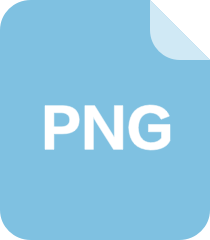
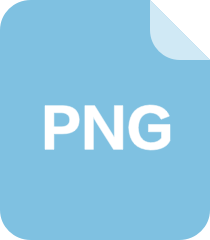
共 162 条
- 1
- 2
资源评论
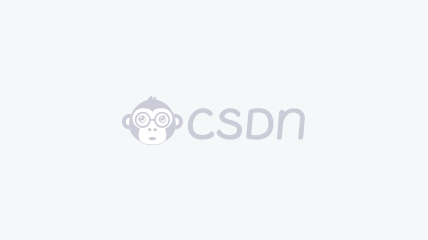

大数据之美
- 粉丝: 3
- 资源: 7
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

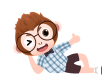
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


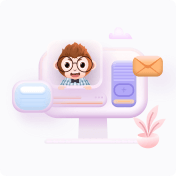
安全验证
文档复制为VIP权益,开通VIP直接复制
