package com.learn.aop;
import com.learn.emun.LimitType;
import com.learn.exception.BusinessException;
import com.learn.util.IpUtil;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.reflect.MethodSignature;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.script.RedisScript;
import org.springframework.stereotype.Component;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import java.lang.reflect.Method;
import java.util.Collections;
import java.util.List;
/**
* @author linMou
* @Description:
* @Date: 2022/5/25 10:46
* @Version: 1.0
*/
@Aspect
@Component
public class RateLimiterAspect {
private static final Logger log = LoggerFactory.getLogger(RateLimiterAspect.class);
@Autowired
private RedisTemplate<Object, Object> redisTemplate;
@Autowired
private RedisScript<Long> limitScript;
@Before(("@annotation(rateLimiter)"))
public void doBefore(JoinPoint point, RateLimiter rateLimiter) throws Throwable {
String key = rateLimiter.key();
int time = rateLimiter.time();
int count = rateLimiter.count();
Object combineKey = getCombineKey(rateLimiter,point);
List<Object> keys = Collections.singletonList(combineKey);
try {
Long number = redisTemplate.execute(limitScript, keys, count, time);
if (number==null || number.intValue() > count) {
throw new BusinessException("访问过于频繁,请稍候再试");
}
log.info("限制请求'{}',当前请求'{}',缓存key'{}'", count, number.intValue(), key);
} catch (BusinessException e) {
throw e;
} catch (Exception e) {
throw new RuntimeException("服务器限流异常,请稍候再试");
}
}
public String getCombineKey(RateLimiter rateLimiter,JoinPoint point) {
StringBuffer stringBuffer = new StringBuffer();
if(rateLimiter.limitType() == LimitType.IP) {
stringBuffer.append(IpUtil.getIpAddr(((ServletRequestAttributes) RequestContextHolder.currentRequestAttributes()).getRequest())).append("-");
}
MethodSignature signature = (MethodSignature)point.getSignature();
Method method = signature.getMethod();
Class<?> targetClass = method.getDeclaringClass();
stringBuffer.append(targetClass.getName()).append("-").append(method.getName());
return stringBuffer.toString();
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo; java自定义注解接口限流demo;
资源推荐
资源详情
资源评论
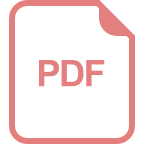
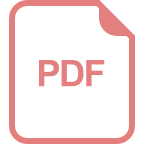
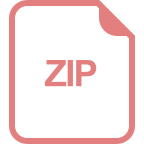
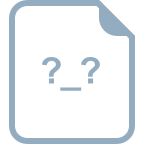
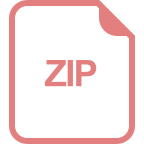
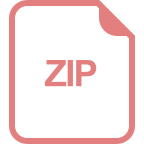
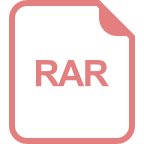
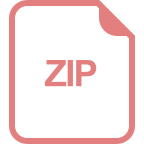
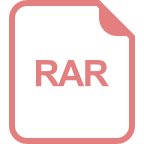
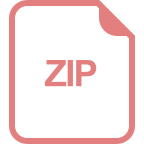
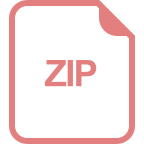
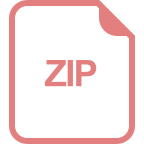
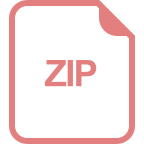
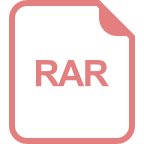
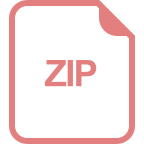
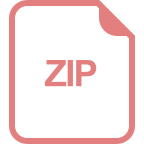
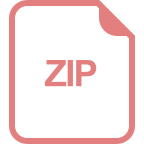
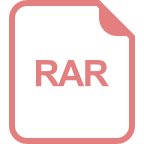
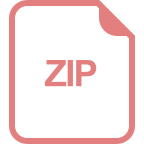
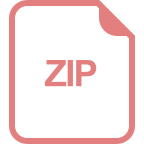
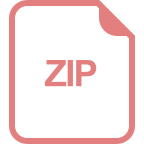
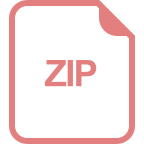
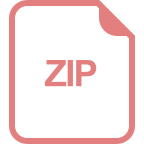
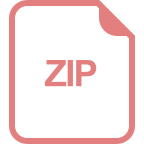
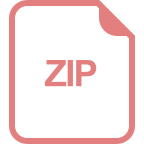
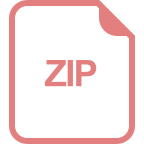
收起资源包目录


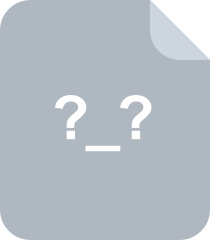
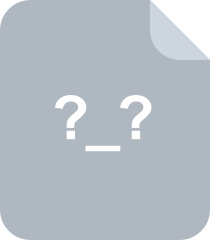
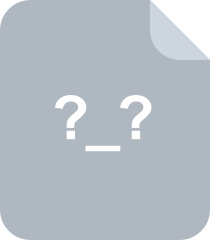





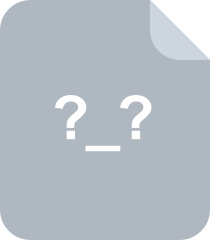



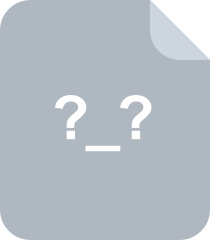
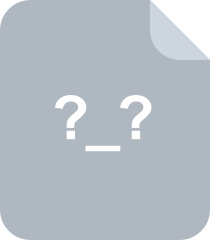




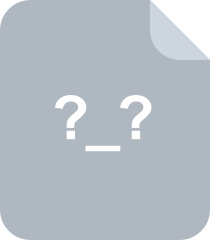
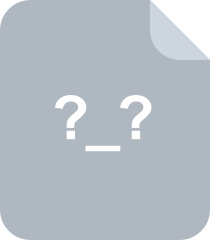

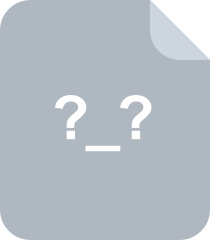

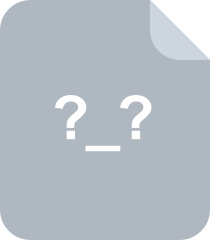
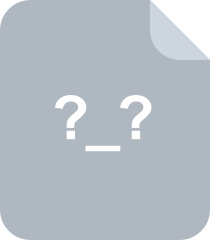

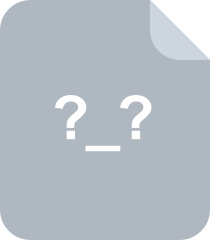

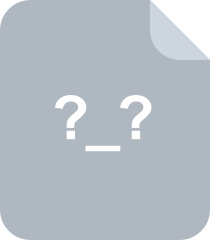

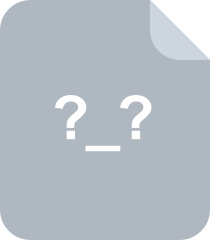
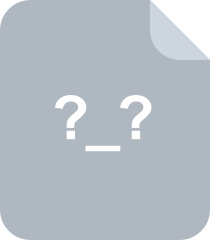


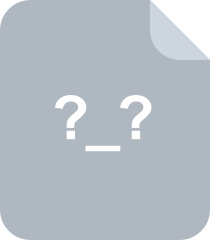
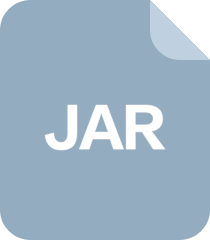
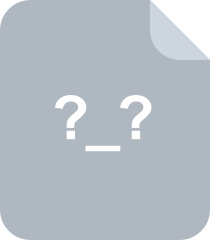
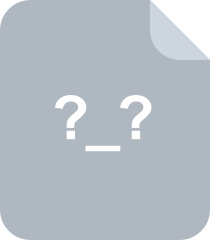
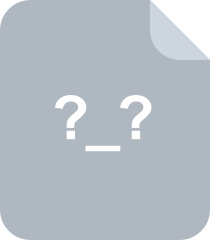
共 20 条
- 1
资源评论
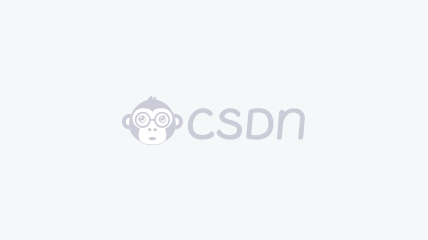

一枚猿人
- 粉丝: 0
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

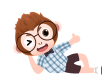
最新资源
- IMG_20241115_051050812.jpg
- 基于javaweb的网上拍卖系统,采用Spring + SpringMvc+Mysql + Hibernate+ JSP技术
- polygon-mumbai
- Chrome代理 switchyOmega
- GVC-全球价值链参与地位指数,基于ICIO表,(Wang等 2017a)计算方法
- 易语言ADS指纹浏览器管理工具
- 易语言奇易模块5.3.6
- cad定制家具平面图工具-(FG)门板覆盖柜体
- asp.net 原生js代码及HTML实现多文件分片上传功能(自定义上传文件大小、文件上传类型)
- whl@pip install pyaudio ERROR: Failed building wheel for pyaudio
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


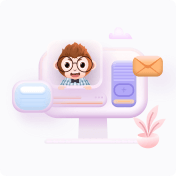
安全验证
文档复制为VIP权益,开通VIP直接复制
