<div align="center">
<br>
<br>
<img width="360" src="media/logo.svg" alt="Got">
<br>
<br>
<br>
<p align="center">Huge thanks to <a href="https://moxy.studio"><img src="https://sindresorhus.com/assets/thanks/moxy-logo.svg" valign="middle" width="150"></a> for sponsoring Sindre Sorhus!
</p>
<p align="center"><sup>(they love Got too!)</sup></p>
<br>
<br>
</div>
> Human-friendly and powerful HTTP request library for Node.js
[](https://travis-ci.com/github/sindresorhus/got)
[](https://coveralls.io/github/sindresorhus/got?branch=master)
[](https://npmjs.com/got)
[](https://packagephobia.now.sh/result?p=got)
[Moving from Request?](documentation/migration-guides.md) [*(Note that Request is unmaintained)*](https://github.com/request/request/issues/3142)
[See how Got compares to other HTTP libraries](#comparison)
For browser usage, we recommend [Ky](https://github.com/sindresorhus/ky) by the same people.
## Highlights
- [Promise API](#api)
- [Stream API](#streams)
- [Pagination API](#pagination)
- [HTTP2 support](#http2)
- [Request cancelation](#aborting-the-request)
- [RFC compliant caching](#cache-adapters)
- [Follows redirects](#followredirect)
- [Retries on failure](#retry)
- [Progress events](#onuploadprogress-progress)
- [Handles gzip/deflate/brotli](#decompress)
- [Timeout handling](#timeout)
- [Errors with metadata](#errors)
- [JSON mode](#json-mode)
- [WHATWG URL support](#url)
- [HTTPS API](#advanced-https-api)
- [Hooks](#hooks)
- [Instances with custom defaults](#instances)
- [Types](#types)
- [Composable](documentation/advanced-creation.md#merging-instances)
- [Plugins](documentation/lets-make-a-plugin.md)
- [Used by 4K+ packages and 1.8M+ repos](https://github.com/sindresorhus/got/network/dependents)
- [Actively maintained](https://github.com/sindresorhus/got/graphs/contributors)
- [Trusted by many companies](#widely-used)
## Install
```
$ npm install got
```
## Usage
###### Promise
```js
const got = require('got');
(async () => {
try {
const response = await got('https://sindresorhus.com');
console.log(response.body);
//=> '<!doctype html> ...'
} catch (error) {
console.log(error.response.body);
//=> 'Internal server error ...'
}
})();
```
###### JSON
```js
const got = require('got');
(async () => {
const {body} = await got.post('https://httpbin.org/anything', {
json: {
hello: 'world'
},
responseType: 'json'
});
console.log(body.data);
//=> {hello: 'world'}
})();
```
See [JSON mode](#json-mode) for more details.
###### Streams
```js
const stream = require('stream');
const {promisify} = require('util');
const fs = require('fs');
const got = require('got');
const pipeline = promisify(stream.pipeline);
(async () => {
await pipeline(
got.stream('https://sindresorhus.com'),
fs.createWriteStream('index.html')
);
// For POST, PUT, PATCH, and DELETE methods, `got.stream` returns a `stream.Writable`.
await pipeline(
fs.createReadStream('index.html'),
got.stream.post('https://sindresorhus.com')
);
})();
```
**Tip:** `from.pipe(to)` doesn't forward errors. Instead, use [`stream.pipeline(from, ..., to, callback)`](https://nodejs.org/api/stream.html#stream_stream_pipeline_streams_callback).
**Note:** While `got.post('https://example.com')` resolves, `got.stream.post('https://example.com')` will hang indefinitely until a body is provided. If there's no body on purpose, remember to `.end()` the stream or set the [`body`](#body) option to an empty string.
### API
It's a `GET` request by default, but can be changed by using different methods or via [`options.method`](#method).
**By default, Got will retry on failure. To disable this option, set [`options.retry`](#retry) to `0`.**
#### got(url?, options?)
Returns a Promise giving a [Response object](#response) or a [Got Stream](#streams-1) if `options.isStream` is set to true.
##### url
Type: `string | object`
The URL to request, as a string, a [`https.request` options object](https://nodejs.org/api/https.html#https_https_request_options_callback), or a [WHATWG `URL`](https://nodejs.org/api/url.html#url_class_url).
Properties from `options` will override properties in the parsed `url`.
If no protocol is specified, it will throw a `TypeError`.
**Note:** The query string is **not** parsed as search params. Example:
```js
got('https://example.com/?query=a b'); //=> https://example.com/?query=a%20b
got('https://example.com/', {searchParams: {query: 'a b'}}); //=> https://example.com/?query=a+b
// The query string is overridden by `searchParams`
got('https://example.com/?query=a b', {searchParams: {query: 'a b'}}); //=> https://example.com/?query=a+b
```
##### options
Type: `object`
Any of the [`https.request`](https://nodejs.org/api/https.html#https_https_request_options_callback) options.
**Note:** Legacy URL support is disabled. `options.path` is supported only for backwards compatibility. Use `options.pathname` and `options.searchParams` instead. `options.auth` has been replaced with `options.username` & `options.password`.
###### method
Type: `string`\
Default: `GET`
The HTTP method used to make the request.
###### prefixUrl
Type: `string | URL`
When specified, `prefixUrl` will be prepended to `url`. The prefix can be any valid URL, either relative or absolute.\
A trailing slash `/` is optional - one will be added automatically.
**Note:** `prefixUrl` will be ignored if the `url` argument is a URL instance.
**Note:** Leading slashes in `input` are disallowed when using this option to enforce consistency and avoid confusion. For example, when the prefix URL is `https://example.com/foo` and the input is `/bar`, there's ambiguity whether the resulting URL would become `https://example.com/foo/bar` or `https://example.com/bar`. The latter is used by browsers.
**Tip:** Useful when used with [`got.extend()`](#custom-endpoints) to create niche-specific Got instances.
**Tip:** You can change `prefixUrl` using hooks as long as the URL still includes the `prefixUrl`. If the URL doesn't include it anymore, it will throw.
```js
const got = require('got');
(async () => {
await got('unicorn', {prefixUrl: 'https://cats.com'});
//=> 'https://cats.com/unicorn'
const instance = got.extend({
prefixUrl: 'https://google.com'
});
await instance('unicorn', {
hooks: {
beforeRequest: [
options => {
options.prefixUrl = 'https://cats.com';
}
]
}
});
//=> 'https://cats.com/unicorn'
})();
```
###### headers
Type: `object`\
Default: `{}`
Request headers.
Existing headers will be overwritten. Headers set to `undefined` will be omitted.
###### isStream
Type: `boolean`\
Default: `false`
Returns a `Stream` instead of a `Promise`. This is equivalent to calling `got.stream(url, options?)`.
###### body
Type: `string | Buffer | stream.Readable` or [`form-data` instance](https://github.com/form-data/form-data)
**Note #1:** The `body` option cannot be used with the `json` or `form` option.
**Note #2:** If you provide this option, `got.stream()` will be read-only.
**Note #3:** If you provide a payload with the `GET` or `HEAD` method, it will throw a `TypeError` unless the method is `GET` and the `allowGetBody` option is set to `true`.
**Note #4:** This option is not enumerable and will not be merged with the instance defaults.
The `content-length` header will be automatically set if `body` is a `string` / `Buffer` / `fs.createReadStream` instance / [`form-data` instance](https://github.com/form-data/form-data), and `content-length` and `transfer-encoding` are not manually set in `options.headers`.
###### json
Type: `object | Array | number | string | boolean | null` *(JSON-serializable values)*
**Note #1:** If you provide thi
没有合适的资源?快使用搜索试试~ 我知道了~
electron框架包vue react前端页面
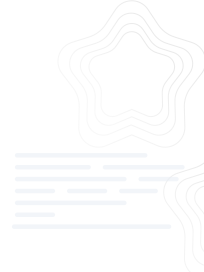
共2000个文件
js:1171个
md:511个
json:239个

0 下载量 111 浏览量
2023-11-04
20:07:13
上传
评论
收藏 78MB 7Z 举报
温馨提示
使用electron框架任意打包vue react前端页面,安装make即可使用,里面已经包含node_module,无需安装依赖 Electron是一个使用 JavaScript、HTML 和 CSS 构建桌面应用程序的框架。 嵌入 Chromium 和 Node.js 到 二进制的 Electron 允许您保持一个 JavaScript 代码代码库并创建 在Windows上运行的跨平台应用 macOS和Linux——不需要本地开发 经验。 ———————————————— 版权声明:本文为CSDN博主「yma16」的原创文章,遵循CC 4.0 BY-SA版权协议,转载请附上原文出处链接及本声明。 原文链接:https://blog.csdn.net/qq_38870145/article/details/134210786
资源推荐
资源详情
资源评论
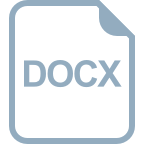
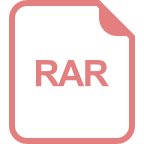
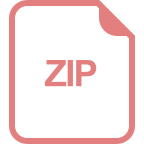
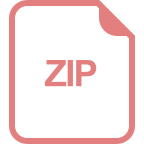
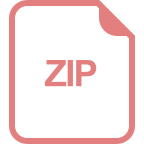
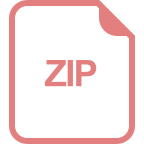
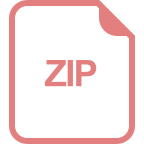
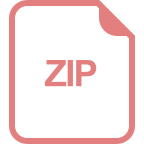
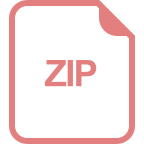
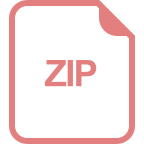
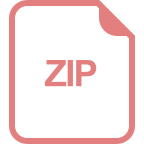
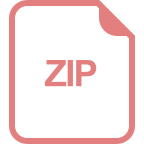
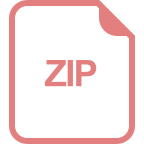
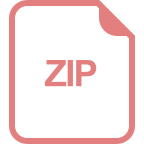
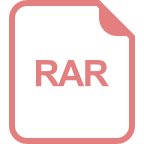
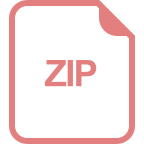
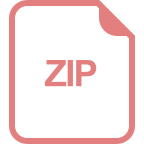
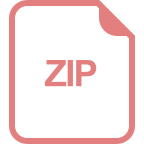
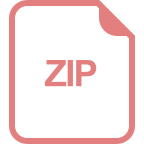
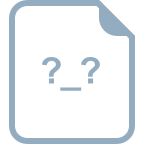
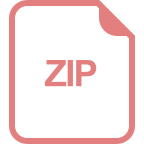
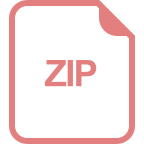
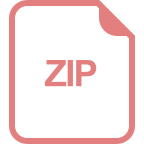
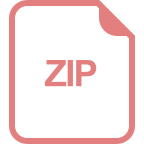
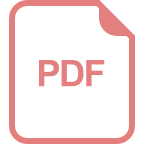
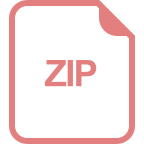
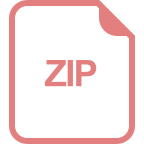
收起资源包目录

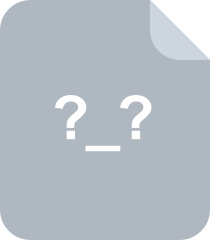
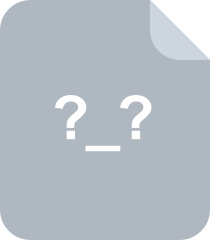
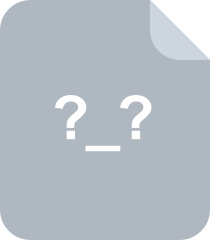
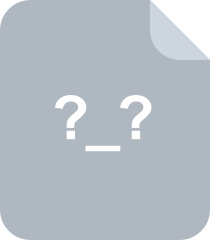
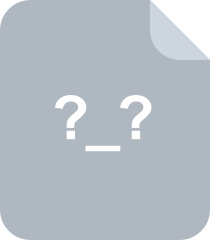
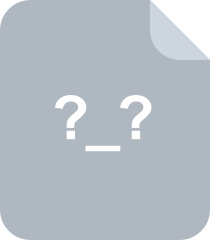
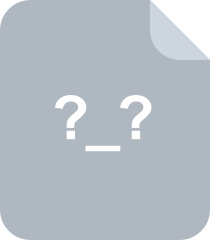
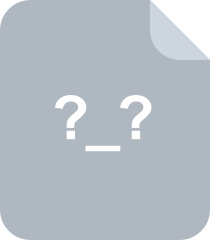
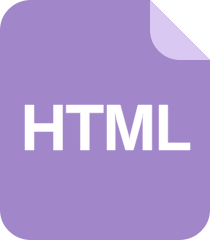
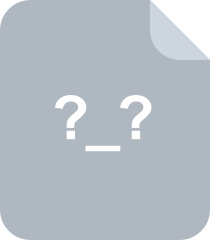
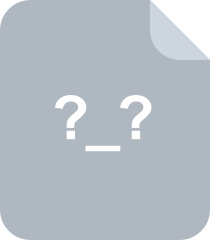
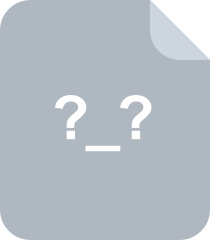
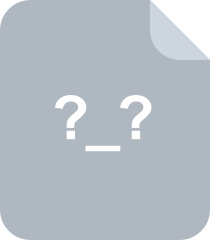
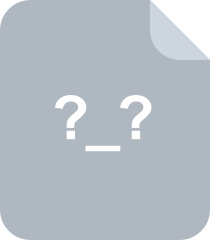
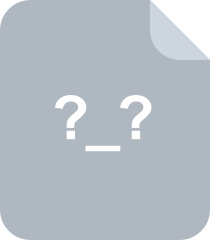
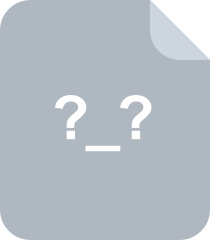
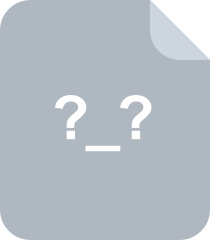
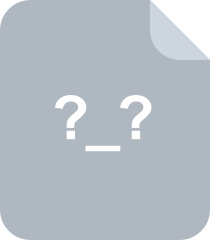
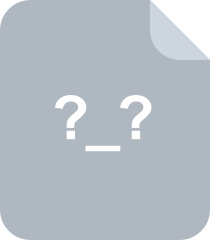
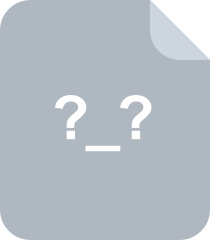
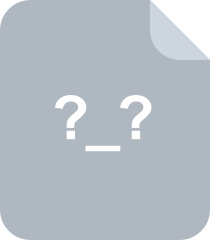
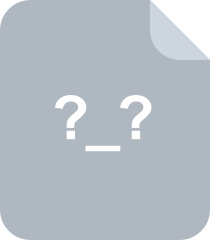
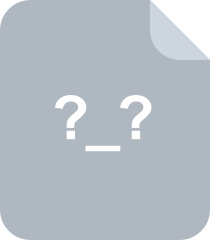
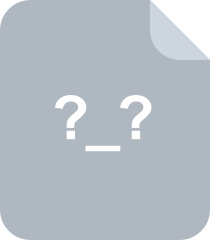
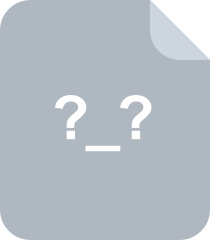
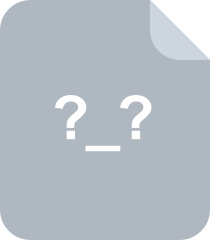
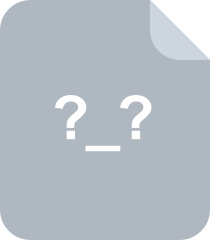
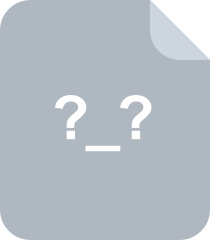
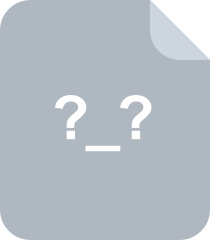
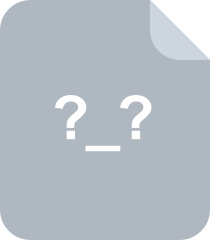
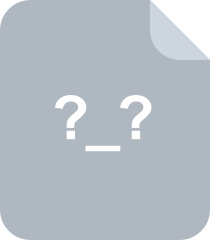
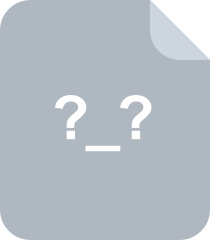
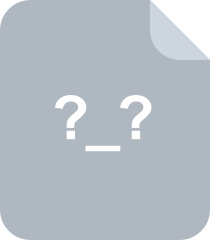
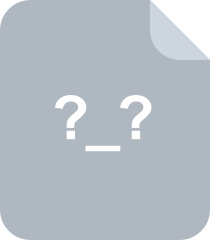
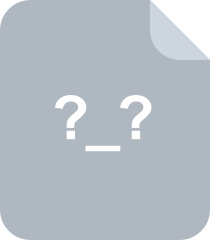
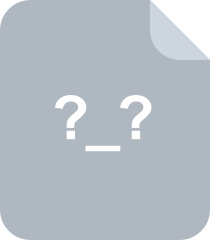
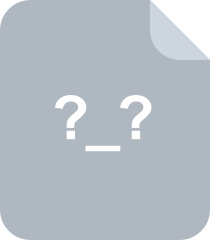
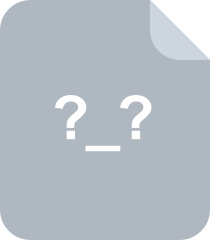
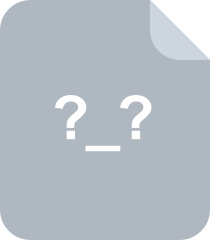
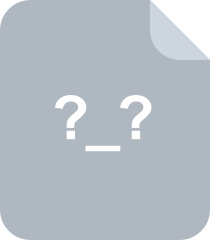
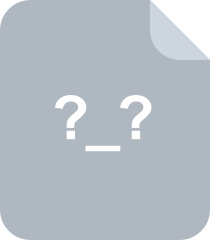
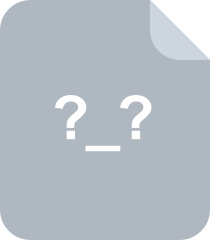
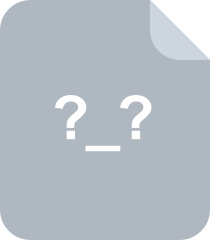
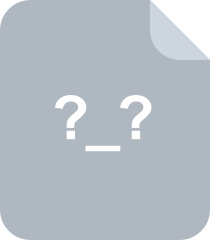
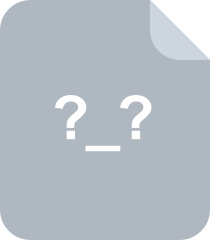
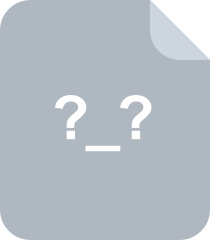
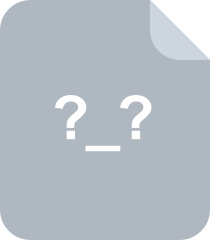
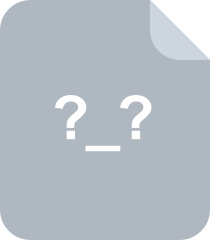
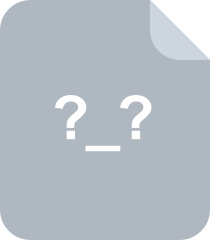
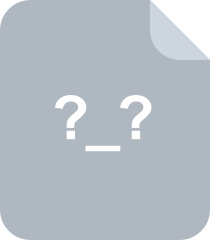
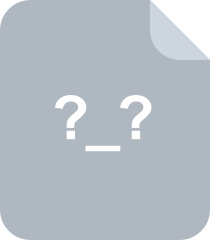
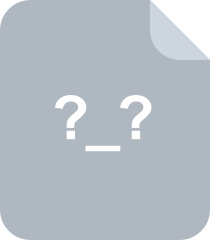
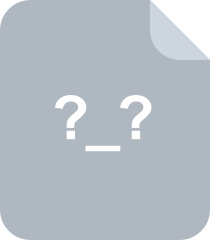
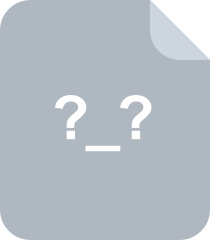
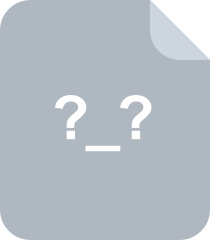
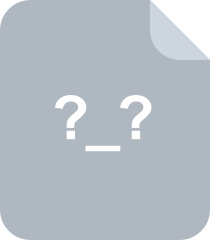
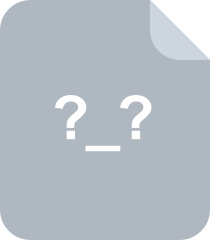
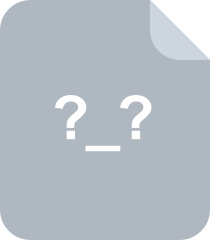
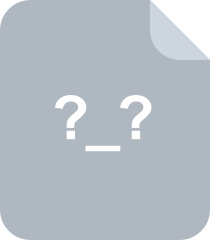
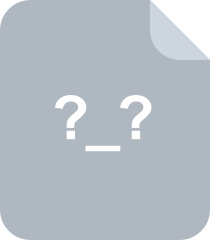
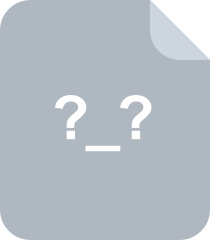
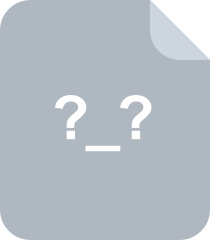
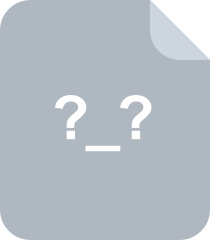
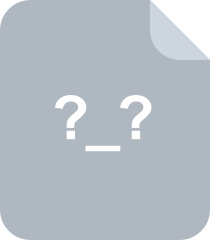
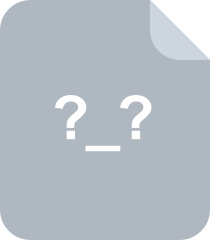
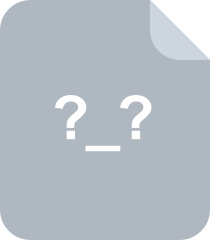
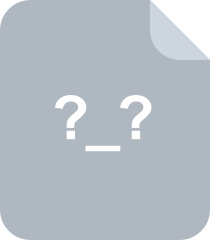
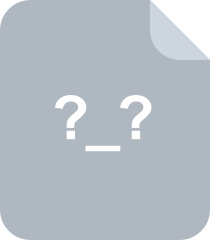
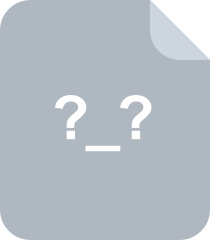
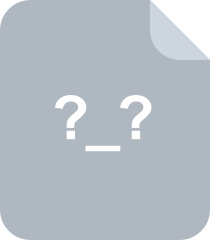
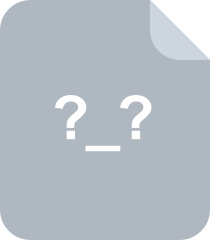
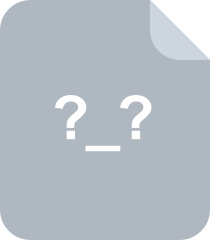
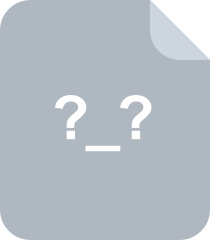
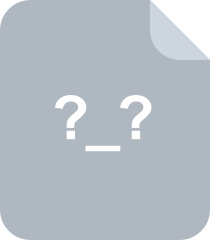
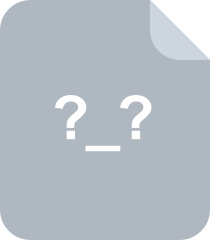
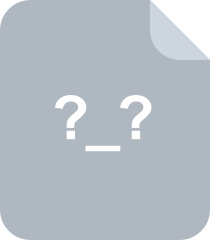
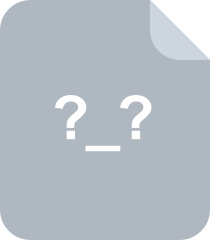
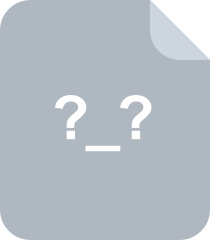
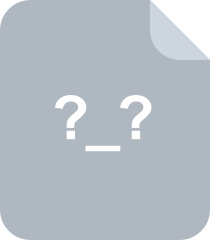
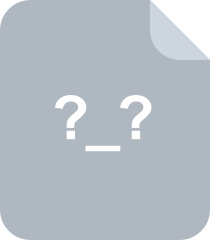
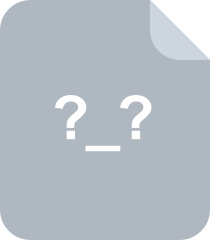
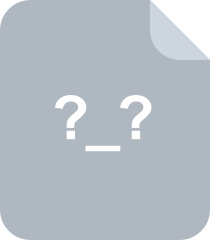
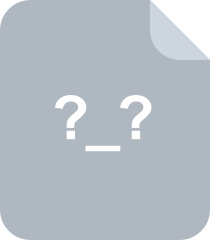
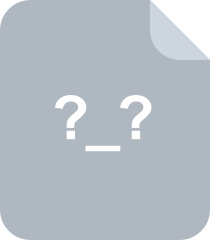
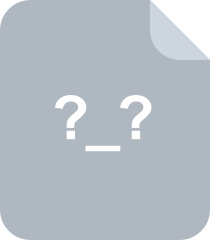
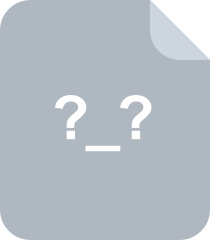
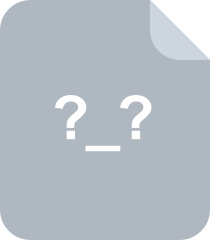
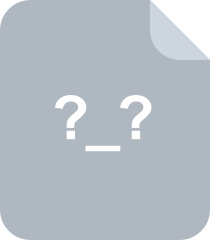
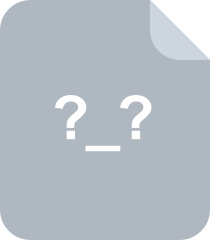
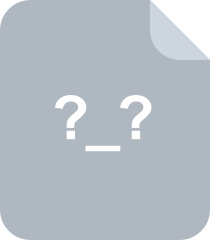
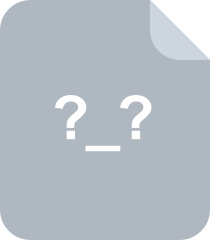
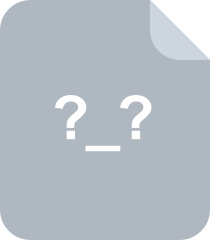
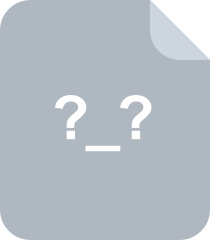
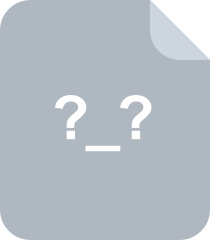
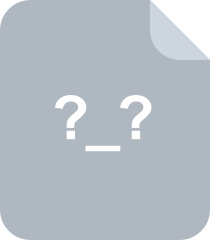
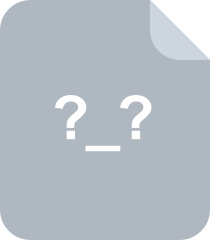
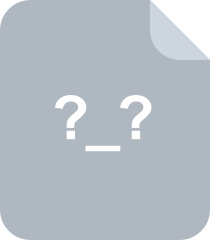
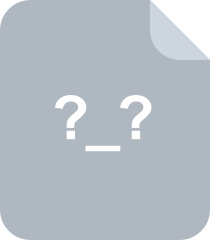
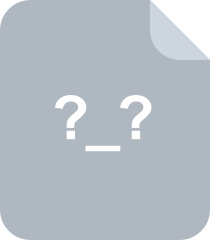
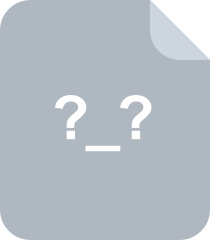
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
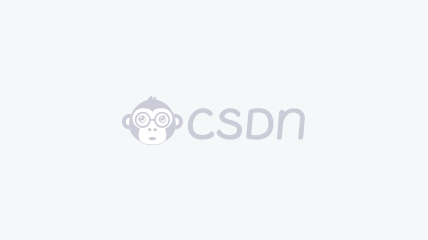

yma16
- 粉丝: 1w+
- 资源: 16
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

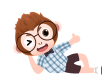
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


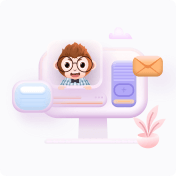
安全验证
文档复制为VIP权益,开通VIP直接复制
