springboot整合mybatis
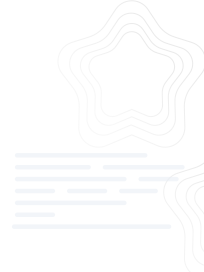

在IT行业中,Spring Boot是一个非常流行的微服务框架,它简化了Spring应用的初始搭建以及开发过程。而MyBatis作为一款强大的持久层框架,能够帮助开发者将SQL操作与Java代码紧密结合,实现灵活的数据访问。本篇文章将深入探讨如何在Spring Boot项目中整合MyBatis,以实现高效、简洁的数据库操作。 我们需要在Spring Boot项目的`pom.xml`或`build.gradle`文件中添加MyBatis和其Spring Boot starter的相关依赖。对于Maven用户,可以在`pom.xml`中添加以下依赖: ```xml <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.1.3</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency> ``` 这里我们使用了JPA依赖,以便于与MyBatis进行集成。同时,MyBatis的Spring Boot Starter用于快速配置MyBatis。添加了MySQL驱动依赖,因为很多项目中会使用MySQL作为数据库。 接下来,我们需要在`application.properties`或`application.yml`中配置数据库连接信息,例如: ```properties spring.datasource.url=jdbc:mysql://localhost:3306/mydb spring.datasource.username=root spring.datasource.password=root spring.datasource.driver-class-name=com.mysql.jdbc.Driver ``` 然后,创建MyBatis的配置文件`mybatis-config.xml`,并放在`src/main/resources`目录下。这个文件通常包含全局配置,例如别名、类型处理器等。一个简单的配置可能如下所示: ```xml <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <typeAliases> <package name="com.example.myproject.model"/> </typeAliases> </configuration> ``` 这里,我们指定了一个包名,MyBatis会自动为该包下的所有类创建别名。 接着,创建MyBatis的Mapper接口和XML映射文件。Mapper接口定义了SQL操作方法,XML文件则包含了具体的SQL语句。例如,有一个`UserMapper`接口: ```java package com.example.myproject.mapper; import com.example.myproject.model.User; import org.apache.ibatis.annotations.Select; import org.springframework.stereotype.Repository; import java.util.List; @Repository public interface UserMapper { @Select("SELECT * FROM user") List<User> getAllUsers(); } ``` 对应的`UserMapper.xml`文件位于`src/main/resources/mapper`目录下: ```xml <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.example.myproject.mapper.UserMapper"> <select id="getAllUsers" resultType="com.example.myproject.model.User"> SELECT * FROM user </select> </mapper> ``` 在这里,`namespace`属性应与Mapper接口的全限定名相同,`id`对应接口中的方法名,`resultType`是返回结果的Java类型。 Spring Boot会自动扫描`@Repository`注解的类,并通过`@Autowired`注入到需要的地方。这样,我们就可以在Service或Controller中直接使用Mapper来执行SQL了。 例如,在Service中: ```java package com.example.myproject.service; import com.example.myproject.mapper.UserMapper; import com.example.myproject.model.User; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import java.util.List; @Service public class UserService { private final UserMapper userMapper; @Autowired public UserService(UserMapper userMapper) { this.userMapper = userMapper; } public List<User> getAllUsers() { return userMapper.getAllUsers(); } } ``` 至此,我们就完成了Spring Boot与MyBatis的整合。通过这种方式,我们可以充分利用Spring Boot的自动化配置和MyBatis的灵活查询,实现高效、便捷的数据库操作。当然,实际项目中可能还需要考虑事务管理、分页查询、动态SQL等功能,但基础的整合步骤已经完成。
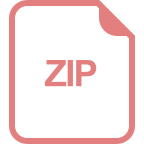
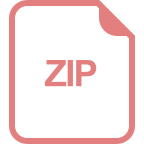
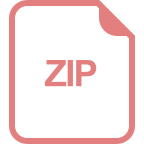
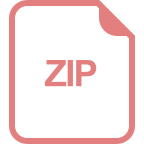
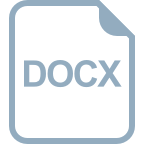
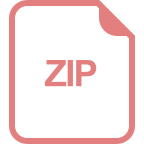


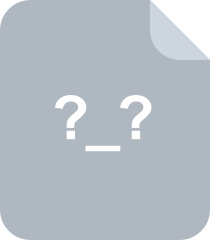




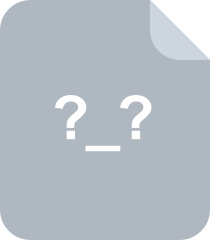



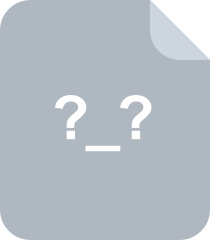



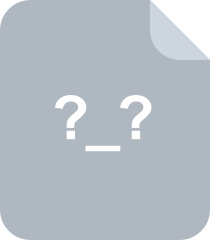
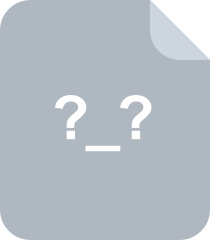

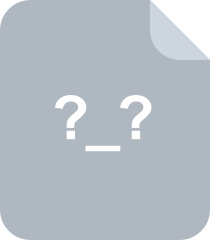

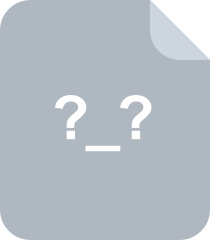

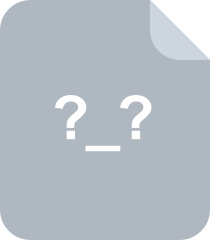


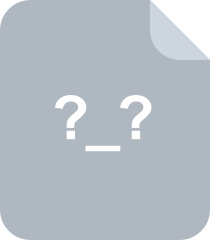
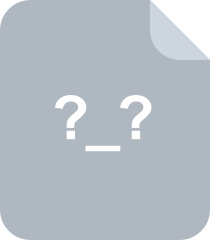





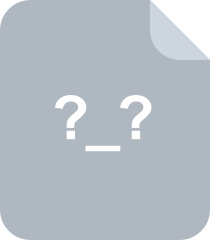


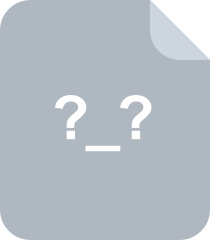






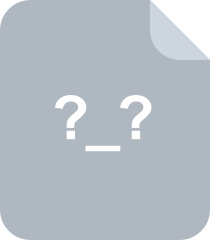
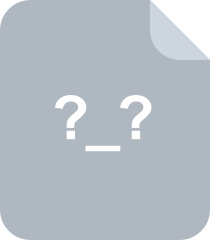

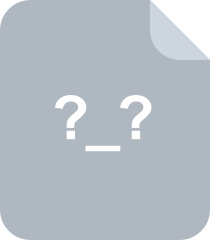

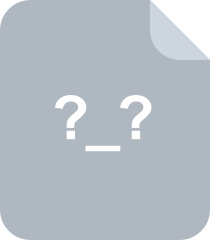

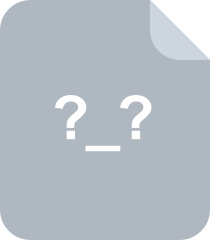

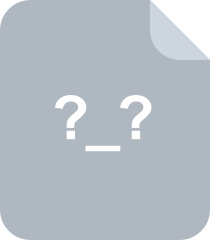
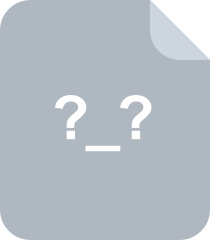
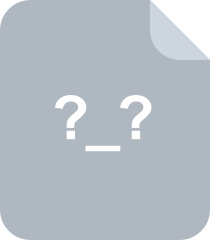
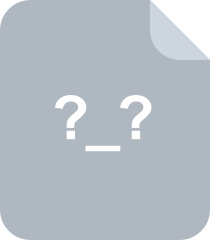
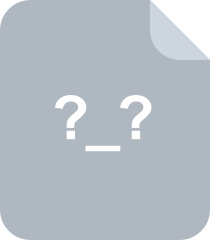

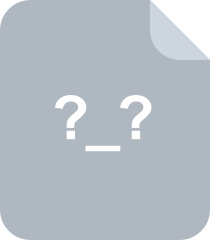
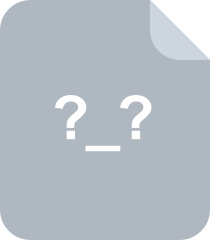
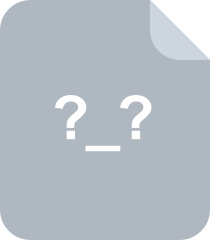
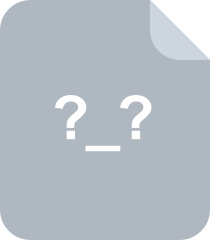
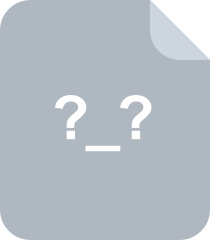
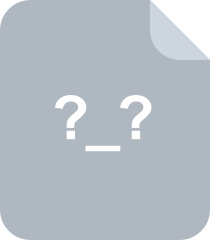
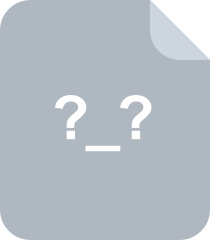
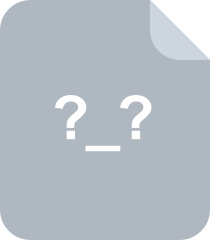
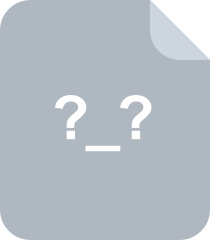
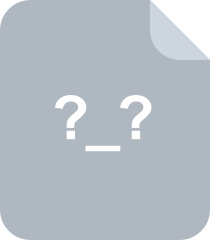
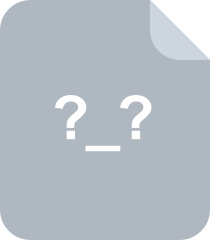
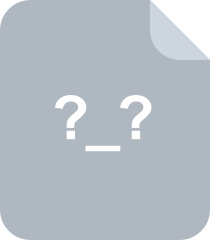
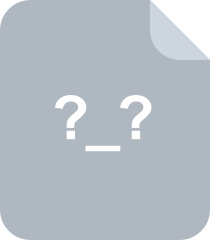
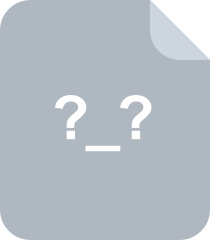
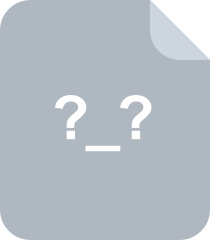
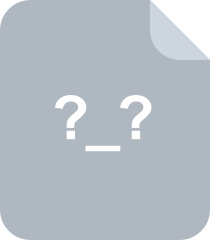
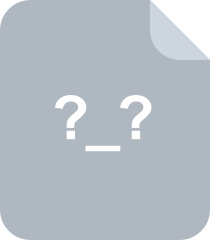
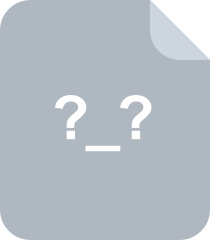
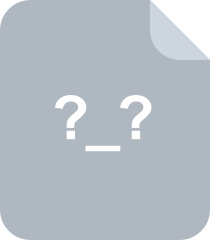
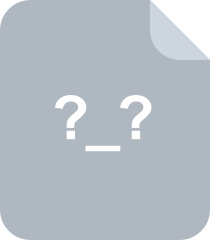
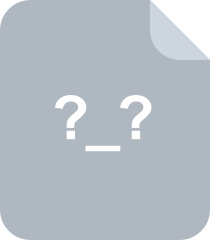
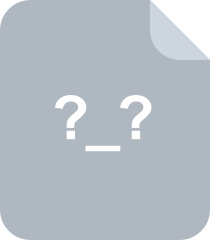
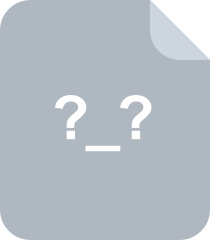
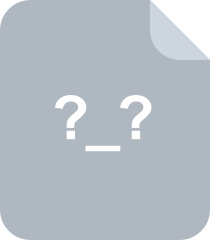
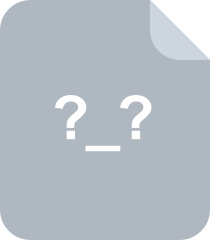
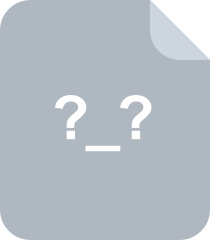
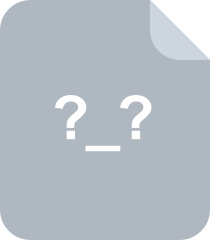
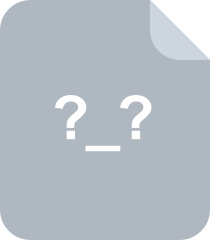
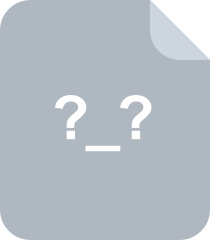
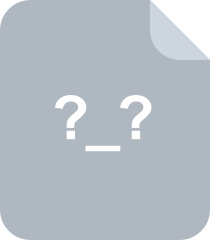
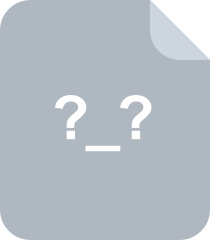
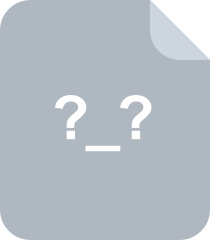
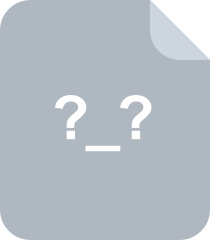
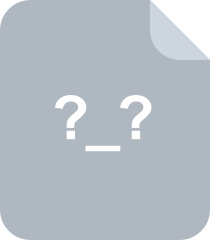
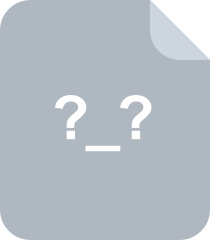
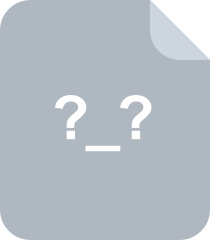
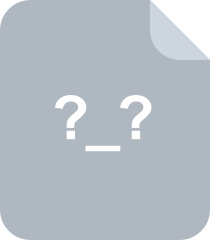
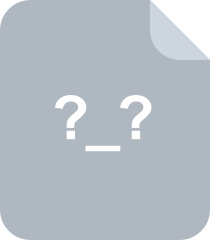
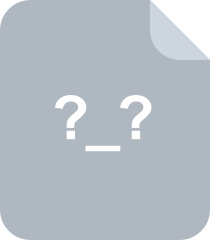
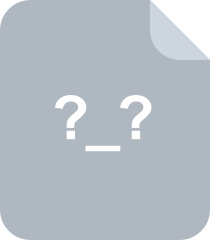
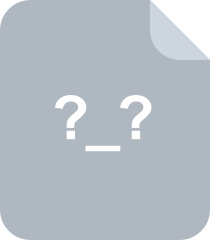
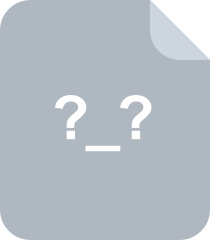
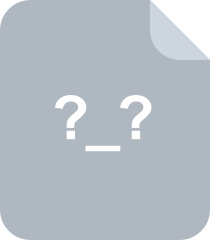
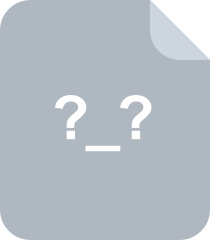
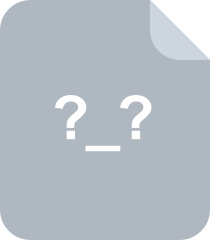
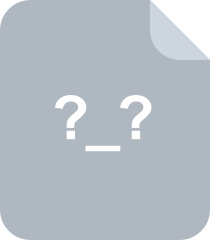
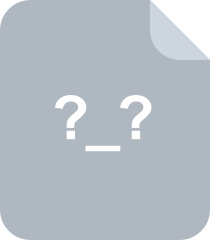
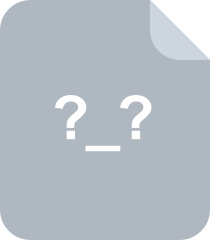
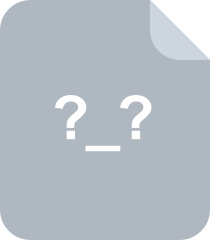
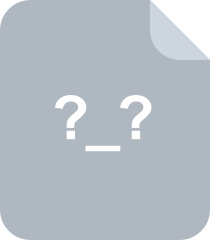
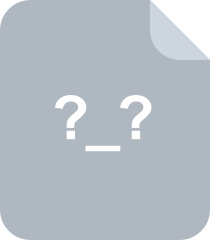
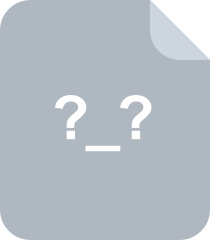
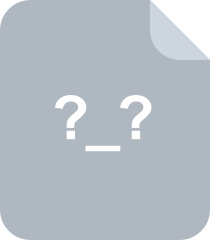
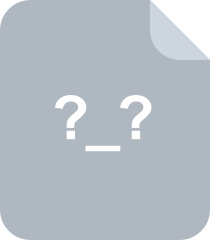
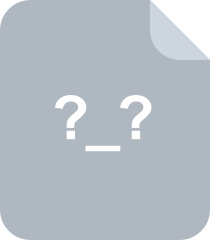
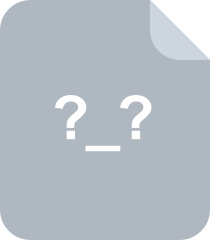
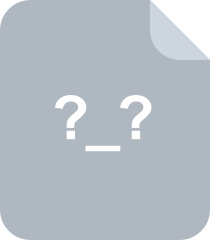
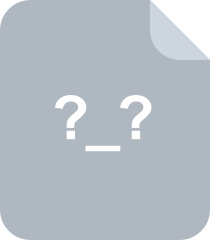
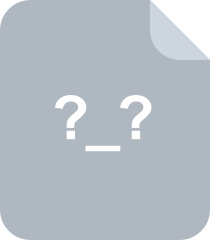
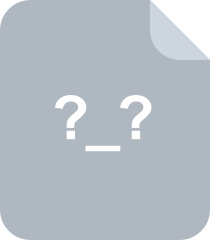
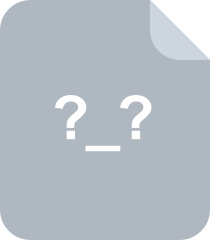
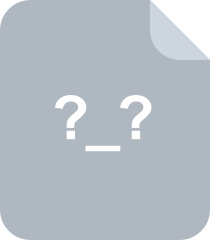
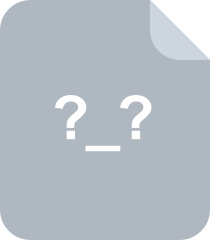
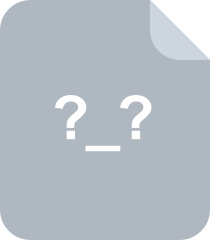
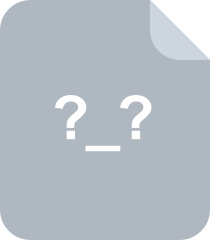
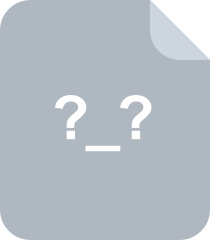


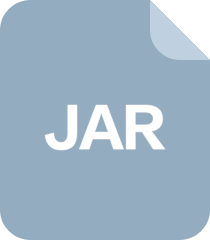
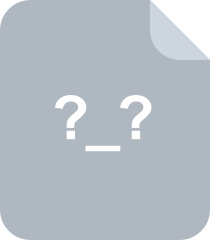
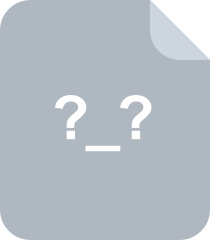
- 1
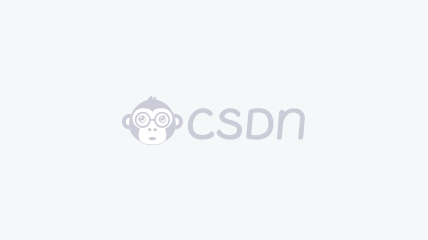

- 粉丝: 9
- 资源: 38
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

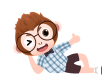
最新资源
- 毕设和企业适用springboot企业资源规划类及环境监控平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及酒店管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及旅游规划平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及食品配送管理平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及知识共享平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及智能图像识别系统源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及语音识别系统源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及信用评分平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及虚拟人类交互系统源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及信息管理系统源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及自动化控制系统源码+论文+视频.zip
- 毕设和企业适用springboot区块链技术类及工程管理平台源码+论文+视频.zip
- 毕设和企业适用springboot区块链技术类及城市智能运营平台源码+论文+视频.zip
- 毕设和企业适用springboot区块链技术类及民生服务平台源码+论文+视频.zip
- 毕设和企业适用springboot区块链技术类及供应链优化系统源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及远程医疗平台源码+论文+视频.zip

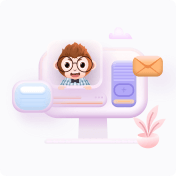
