package com.controller;
import java.text.SimpleDateFormat;
import com.alibaba.fastjson.JSONObject;
import java.util.*;
import org.springframework.beans.BeanUtils;
import javax.servlet.http.HttpServletRequest;
import org.springframework.web.context.ContextLoader;
import javax.servlet.ServletContext;
import com.service.TokenService;
import com.utils.StringUtil;
import java.lang.reflect.InvocationTargetException;
import org.apache.commons.lang3.StringUtils;
import com.annotation.IgnoreAuth;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.entity.YonghuEntity;
import com.service.YonghuService;
import com.entity.view.YonghuView;
import com.utils.PageUtils;
import com.utils.R;
/**
* 学生
* 后端接口
* @author
* @email
* @date 2021-03-02
*/
@RestController
@Controller
@RequestMapping("/yonghu")
public class YonghuController {
private static final Logger logger = LoggerFactory.getLogger(YonghuController.class);
@Autowired
private YonghuService yonghuService;
@Autowired
private TokenService tokenService;
//级联表service
//字典表map
Map<String, Map<Integer, String>> dictionaryMap;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params, HttpServletRequest request){
logger.debug("page方法:,,Controller:{},,params:{}",this.getClass().getName(),JSONObject.toJSONString(params));
PageUtils page = yonghuService.queryPage(params);
//字典表数据转换
List<YonghuView> list =(List<YonghuView>)page.getList();
ServletContext servletContext = ContextLoader.getCurrentWebApplicationContext().getServletContext();
dictionaryMap = (Map<String, Map<Integer, String>>) servletContext.getAttribute("dictionaryMap");
for(YonghuView c:list){
this.dictionaryConvert(c);
}
return R.ok().put("data", page);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
logger.debug("info方法:,,Controller:{},,id:{}",this.getClass().getName(),id);
YonghuEntity yonghu = yonghuService.selectById(id);
if(yonghu !=null){
//entity转view
YonghuView view = new YonghuView();
BeanUtils.copyProperties( yonghu , view );//把实体数据重构到view中
//字典表字典转换
ServletContext servletContext = ContextLoader.getCurrentWebApplicationContext().getServletContext();
dictionaryMap = (Map<String, Map<Integer, String>>) servletContext.getAttribute("dictionaryMap");
this.dictionaryConvert(view);
return R.ok().put("data", view);
}else {
return R.error(511,"查不到数据");
}
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
logger.debug("save方法:,,Controller:{},,yonghu:{}",this.getClass().getName(),yonghu.toString());
Wrapper<YonghuEntity> queryWrapper = new EntityWrapper<YonghuEntity>()
.eq("username", yonghu.getUsername())
.eq("password", yonghu.getPassword())
.eq("name", yonghu.getName())
.eq("phone", yonghu.getPhone())
.eq("id_number", yonghu.getIdNumber())
.eq("sex_types", yonghu.getSexTypes())
.eq("nation", yonghu.getNation())
.eq("politics_types", yonghu.getPoliticsTypes())
.eq("birthplace", yonghu.getBirthplace())
.eq("yonghu_types", yonghu.getYonghuTypes())
;
logger.info("sql语句:"+queryWrapper.getSqlSegment());
YonghuEntity yonghuEntity = yonghuService.selectOne(queryWrapper);
if(yonghuEntity==null){
yonghu.setCreateTime(new Date());
yonghu.setPassword("123456");
// String role = String.valueOf(request.getSession().getAttribute("role"));
// if("".equals(role)){
// yonghu.set
// }
yonghuService.insert(yonghu);
return R.ok();
}else {
return R.error(511,"表中有相同数据");
}
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
logger.debug("update方法:,,Controller:{},,yonghu:{}",this.getClass().getName(),yonghu.toString());
//根据字段查询是否有相同数据
Wrapper<YonghuEntity> queryWrapper = new EntityWrapper<YonghuEntity>()
.notIn("id",yonghu.getId())
.eq("username", yonghu.getUsername())
.eq("password", yonghu.getPassword())
.eq("name", yonghu.getName())
.eq("phone", yonghu.getPhone())
.eq("id_number", yonghu.getIdNumber())
.eq("sex_types", yonghu.getSexTypes())
.eq("nation", yonghu.getNation())
.eq("politics_types", yonghu.getPoliticsTypes())
.eq("birthplace", yonghu.getBirthplace())
.eq("yonghu_types", yonghu.getYonghuTypes())
;
logger.info("sql语句:"+queryWrapper.getSqlSegment());
YonghuEntity yonghuEntity = yonghuService.selectOne(queryWrapper);
if("".equals(yonghu.getMyPhoto()) || "null".equals(yonghu.getMyPhoto())){
yonghu.setMyPhoto(null);
}
if(yonghuEntity==null){
// String role = String.valueOf(request.getSession().getAttribute("role"));
// if("".equals(role)){
// yonghu.set
// }
yonghuService.updateById(yonghu);//根据id更新
return R.ok();
}else {
return R.error(511,"表中有相同数据");
}
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Integer[] ids){
logger.debug("delete:,,Controller:{},,ids:{}",this.getClass().getName(),ids.toString());
yonghuService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
*字典表数据转换
*/
public void dictionaryConvert(YonghuView yonghuView){
//当前表的字典字段
if(StringUtil.isNotEmpty(String.valueOf(yonghuView.getSexTypes()))){
yonghuView.setSexValue(dictionaryMap.get("sex_types").get(yonghuView.getSexTypes()));
}
if(StringUtil.isNotEmpty(String.valueOf(yonghuView.getPoliticsTypes()))){
yonghuView.setPoliticsValue(dictionaryMap.get("politics_types").get(yonghuView.getPoliticsTypes()));
}
if(StringUtil.isNotEmpty(String.valueOf(yonghuView.getYonghuTypes()))){
yonghuView.setYonghuValue(dictionaryMap.get("yonghu_types").get(yonghuView.getYonghuTypes()));
}
//级联表的字典字段
}
/**
* 登录
*/
@IgnoreAuth
@PostMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
YonghuEntity yonghu = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("username", username));
if(yonghu==null || !yonghu.getPassword().equals(password)) {
return R.error("账号或密码不正确");
}
ServletContext servletContext = ContextLoader.getCurrentWebApplicationContext().getServletContext();
dictionaryMap = (Map<String, Map<Integer, String>>) servletContext.getAttribute("dictionaryMap");
String role = dictionaryMap.get("yonghu_types").get(yonghu.getYonghuTypes());
String token = tokenService.generateToken(yonghu.getId(),username, "yonghu",role );
R r = R.ok();
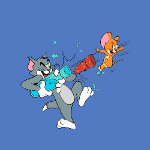
Java码库
- 粉丝: 2479
- 资源: 6186
最新资源
- 基于FPGA的Verilog实现FOC电流环控制系统设计与实践:集成PI控制器与SVPWM算法,ADC采样与串口通信,清晰代码层次结构,结合Simulink模型与RTL图解,适用于BLDC与PMSM的
- VESC STM32F4磁链观测器及无感正弦FOC控制实现与工程应用:代码、文档与仿真详解,VESC STM32F4磁链观测器与无感正弦FOC控制:实现0速闭环启动技术指南,VESC STM32F4磁
- 基于Vue全家桶的现代化家庭农场管理系统设计源码
- 基于Java开发的his门诊系统设计源码
- 基于海思硬件平台与华为云的智能饮食营养数据分析系统CaloScan设计源码
- 基于微信小程序和JavaScript的半开源soulmate设计源码
- 冲床自动化送料程序:双轴控制,FX1S PLC与昆仑通态触摸屏协同,通用型板材冲压冲裁自动送料系统,冲床自动化送料程序:双轴控制,FX1S PLC与昆仑通态触摸屏协同,适用于广泛板材冲压冲裁自动送料系
- 基于JavaScript的蓝牙在线版本蓝牙秤设计源码
- 贝叶斯优化SVM模型:多特征输入与输出数据的分类预测与迭代优化图解,基于多特征输入的Bayes-SVM数据分类预测模型:迭代优化与混淆矩阵图分析,bayes-SVM贝叶斯优化支持向量机的数据分类预测
- COMSOL 5.6模拟裂隙岩体注浆中渗透率演化:变质量渗流模型研究及不同压力下的封堵模拟,COMSOL 5.6模拟裂隙岩体注浆:压力变化下的渗透率演化及变质量渗流研究,comsol5.6,模拟裂隙岩
- 基于关键点检测技术的飞机仪表盘识别设计源码
- 基于Vue框架的献血系统前端页面设计源码
- 基于Java的MaaS-ABAC-DP多维数据安全共享隐私保护设计方案源码
- 模拟浆液黏度时空变化对裂隙注浆影响的研究-基于COMSOL 5.6的数值模拟分析,模拟裂隙注浆过程中浆液黏度时空变化特征-基于Comsol 5.6模拟研究,comsol5.6,模拟浆液黏度时空变化
- 基于Vue的纯Admin管理后台项目设计源码保姆级教程文档
- 基于GPT技术的Vue框架试验性辅助学习网站设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


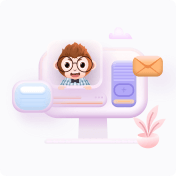