<?php
#
# Markdown Extra - A text-to-HTML conversion tool for web writers
#
# PHP Markdown & Extra
# Copyright (c) 2004-2012 Michel Fortin
# <http://michelf.com/projects/php-markdown/>
#
# Original Markdown
# Copyright (c) 2004-2006 John Gruber
# <http://daringfireball.net/projects/markdown/>
#
define( 'MARKDOWN_VERSION', "1.0.1o" ); # Sun 8 Jan 2012
define( 'MARKDOWNEXTRA_VERSION', "1.2.5" ); # Sun 8 Jan 2012
#
# Global default settings:
#
# Change to ">" for HTML output
@define( 'MARKDOWN_EMPTY_ELEMENT_SUFFIX', " />");
# Define the width of a tab for code blocks.
@define( 'MARKDOWN_TAB_WIDTH', 4 );
# Optional title attribute for footnote links and backlinks.
@define( 'MARKDOWN_FN_LINK_TITLE', "" );
@define( 'MARKDOWN_FN_BACKLINK_TITLE', "" );
# Optional class attribute for footnote links and backlinks.
@define( 'MARKDOWN_FN_LINK_CLASS', "" );
@define( 'MARKDOWN_FN_BACKLINK_CLASS', "" );
#
# WordPress settings:
#
# Change to false to remove Markdown from posts and/or comments.
@define( 'MARKDOWN_WP_POSTS', true );
@define( 'MARKDOWN_WP_COMMENTS', true );
### Standard Function Interface ###
@define( 'MARKDOWN_PARSER_CLASS', 'MarkdownExtra_Parser' );
function Markdown($text) {
#
# Initialize the parser and return the result of its transform method.
#
# Setup static parser variable.
static $parser;
if (!isset($parser)) {
$parser_class = MARKDOWN_PARSER_CLASS;
$parser = new $parser_class;
}
# Transform text using parser.
return $parser->transform($text);
}
### WordPress Plugin Interface ###
/*
Plugin Name: Markdown Extra
Plugin URI: http://michelf.com/projects/php-markdown/
Description: <a href="http://daringfireball.net/projects/markdown/syntax">Markdown syntax</a> allows you to write using an easy-to-read, easy-to-write plain text format. Based on the original Perl version by <a href="http://daringfireball.net/">John Gruber</a>. <a href="http://michelf.com/projects/php-markdown/">More...</a>
Version: 1.2.5
Author: Michel Fortin
Author URI: http://michelf.com/
*/
if (isset($wp_version)) {
# More details about how it works here:
# <http://michelf.com/weblog/2005/wordpress-text-flow-vs-markdown/>
# Post content and excerpts
# - Remove WordPress paragraph generator.
# - Run Markdown on excerpt, then remove all tags.
# - Add paragraph tag around the excerpt, but remove it for the excerpt rss.
if (MARKDOWN_WP_POSTS) {
remove_filter('the_content', 'wpautop');
remove_filter('the_content_rss', 'wpautop');
remove_filter('the_excerpt', 'wpautop');
add_filter('the_content', 'mdwp_MarkdownPost', 6);
add_filter('the_content_rss', 'mdwp_MarkdownPost', 6);
add_filter('get_the_excerpt', 'mdwp_MarkdownPost', 6);
add_filter('get_the_excerpt', 'trim', 7);
add_filter('the_excerpt', 'mdwp_add_p');
add_filter('the_excerpt_rss', 'mdwp_strip_p');
remove_filter('content_save_pre', 'balanceTags', 50);
remove_filter('excerpt_save_pre', 'balanceTags', 50);
add_filter('the_content', 'balanceTags', 50);
add_filter('get_the_excerpt', 'balanceTags', 9);
}
# Add a footnote id prefix to posts when inside a loop.
function mdwp_MarkdownPost($text) {
static $parser;
if (!$parser) {
$parser_class = MARKDOWN_PARSER_CLASS;
$parser = new $parser_class;
}
if (is_single() || is_page() || is_feed()) {
$parser->fn_id_prefix = "";
} else {
$parser->fn_id_prefix = get_the_ID() . ".";
}
return $parser->transform($text);
}
# Comments
# - Remove WordPress paragraph generator.
# - Remove WordPress auto-link generator.
# - Scramble important tags before passing them to the kses filter.
# - Run Markdown on excerpt then remove paragraph tags.
if (MARKDOWN_WP_COMMENTS) {
remove_filter('comment_text', 'wpautop', 30);
remove_filter('comment_text', 'make_clickable');
add_filter('pre_comment_content', 'Markdown', 6);
add_filter('pre_comment_content', 'mdwp_hide_tags', 8);
add_filter('pre_comment_content', 'mdwp_show_tags', 12);
add_filter('get_comment_text', 'Markdown', 6);
add_filter('get_comment_excerpt', 'Markdown', 6);
add_filter('get_comment_excerpt', 'mdwp_strip_p', 7);
global $mdwp_hidden_tags, $mdwp_placeholders;
$mdwp_hidden_tags = explode(' ',
'<p> </p> <pre> </pre> <ol> </ol> <ul> </ul> <li> </li>');
$mdwp_placeholders = explode(' ', str_rot13(
'pEj07ZbbBZ U1kqgh4w4p pre2zmeN6K QTi31t9pre ol0MP1jzJR '.
'ML5IjmbRol ulANi1NsGY J7zRLJqPul liA8ctl16T K9nhooUHli'));
}
function mdwp_add_p($text) {
if (!preg_match('{^$|^<(p|ul|ol|dl|pre|blockquote)>}i', $text)) {
$text = '<p>'.$text.'</p>';
$text = preg_replace('{\n{2,}}', "</p>\n\n<p>", $text);
}
return $text;
}
function mdwp_strip_p($t) { return preg_replace('{</?p>}i', '', $t); }
function mdwp_hide_tags($text) {
global $mdwp_hidden_tags, $mdwp_placeholders;
return str_replace($mdwp_hidden_tags, $mdwp_placeholders, $text);
}
function mdwp_show_tags($text) {
global $mdwp_hidden_tags, $mdwp_placeholders;
return str_replace($mdwp_placeholders, $mdwp_hidden_tags, $text);
}
}
### bBlog Plugin Info ###
function identify_modifier_markdown() {
return array(
'name' => 'markdown',
'type' => 'modifier',
'nicename' => 'PHP Markdown Extra',
'description' => 'A text-to-HTML conversion tool for web writers',
'authors' => 'Michel Fortin and John Gruber',
'licence' => 'GPL',
'version' => MARKDOWNEXTRA_VERSION,
'help' => '<a href="http://daringfireball.net/projects/markdown/syntax">Markdown syntax</a> allows you to write using an easy-to-read, easy-to-write plain text format. Based on the original Perl version by <a href="http://daringfireball.net/">John Gruber</a>. <a href="http://michelf.com/projects/php-markdown/">More...</a>',
);
}
### Smarty Modifier Interface ###
function smarty_modifier_markdown($text) {
return Markdown($text);
}
### Textile Compatibility Mode ###
# Rename this file to "classTextile.php" and it can replace Textile everywhere.
if (strcasecmp(substr(__FILE__, -16), "classTextile.php") == 0) {
# Try to include PHP SmartyPants. Should be in the same directory.
@include_once 'smartypants.php';
# Fake Textile class. It calls Markdown instead.
class Textile {
function TextileThis($text, $lite='', $encode='') {
if ($lite == '' && $encode == '') $text = Markdown($text);
if (function_exists('SmartyPants')) $text = SmartyPants($text);
return $text;
}
# Fake restricted version: restrictions are not supported for now.
function TextileRestricted($text, $lite='', $noimage='') {
return $this->TextileThis($text, $lite);
}
# Workaround to ensure compatibility with TextPattern 4.0.3.
function blockLite($text) { return $text; }
}
}
#
# Markdown Parser Class
#
class Markdown_Parser {
### Configuration Variables ###
# Change to ">" for HTML output.
var $empty_element_suffix = MARKDOWN_EMPTY_ELEMENT_SUFFIX;
var $tab_width = MARKDOWN_TAB_WIDTH;
# Change to `true` to disallow markup or entities.
var $no_markup = false;
var $no_entities = false;
# Predefined urls and titles for reference links and images.
var $predef_urls = array();
var $predef_titles = array();
### Parser Implementation ###
# Regex to match balanced [brackets].
# Needed to insert a maximum bracked depth while converting to PHP.
var $nested_brackets_depth = 6;
var $nested_brackets_re;
var $nested_url_parenthesis_depth = 4;
var $nested_url_parenthesis_re;
# Table of hash values for escaped characters:
var $escape_chars = '\`*_{}[]()>#+-.!';
var $escape_chars_re;
function Markdown_Parser() {
#
# Constructor function. Initialize appropriate member variables.
#
$this->_initDetab();
$this->prepareItalicsAndBold();
$this->nested_brackets_re =
str_repeat('(?>[^\[\]]+|\[', $this->nested_brackets_depth).
str_repeat('\])*', $this->nested_brackets_depth);
$this->nested_url_parenthesis_re =
str_repeat('(?>[^()\s]+|\(', $this->nested_url_p
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
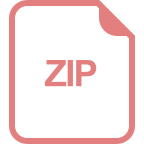
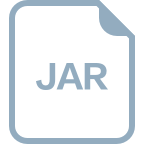
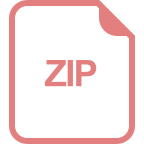
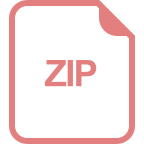
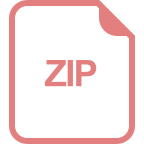
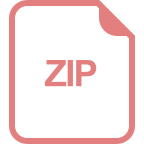
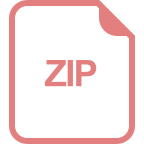
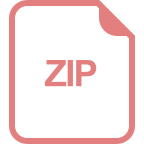
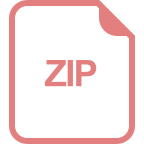
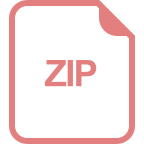
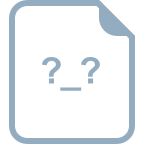
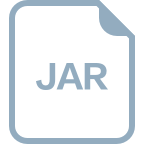
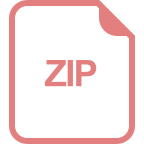
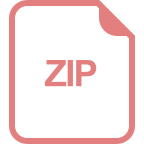
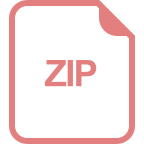
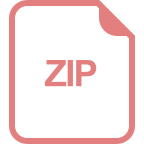
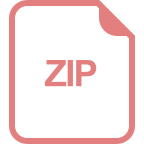
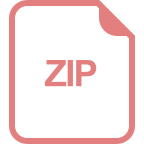
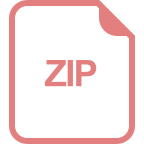
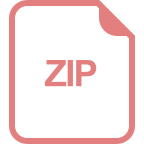
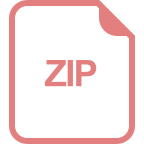
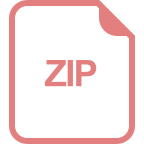
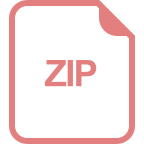
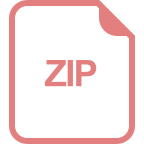
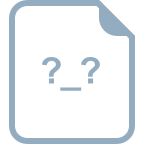
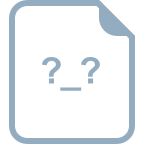
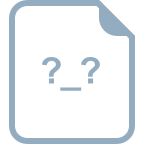
收起资源包目录

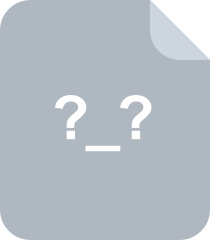
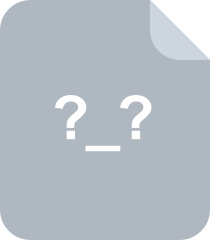
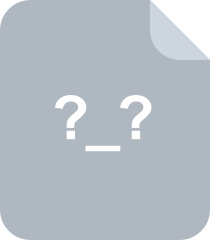
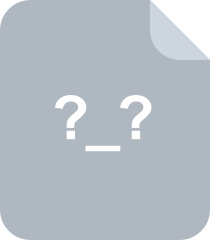
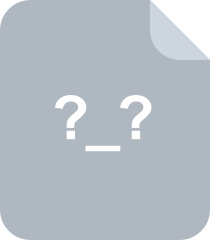
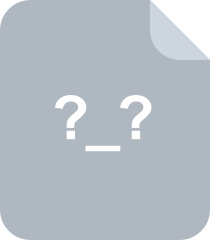
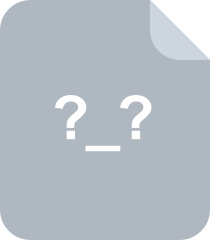
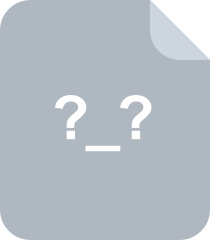
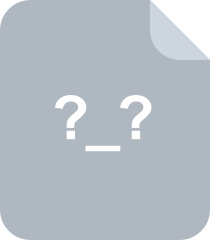
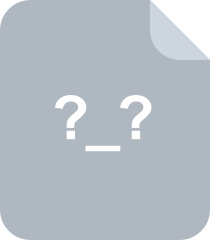
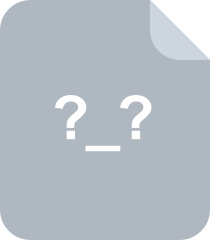
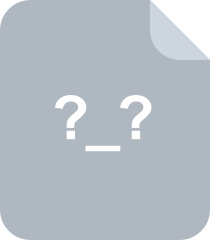
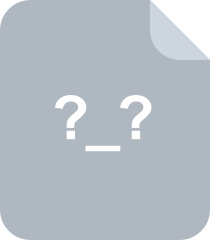
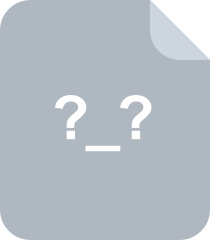
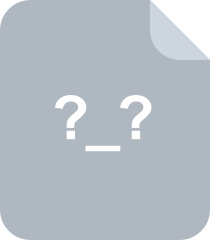
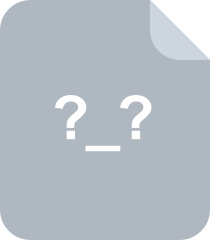
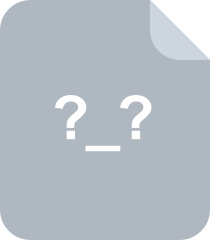
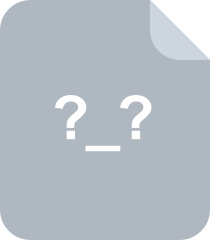
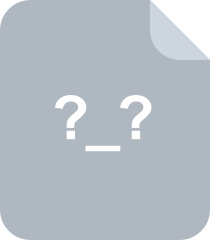
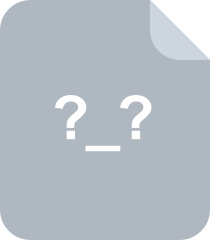
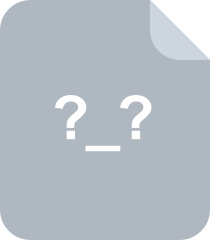
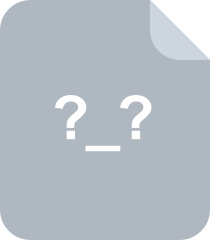
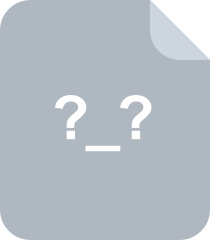
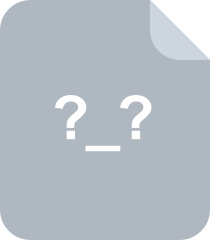
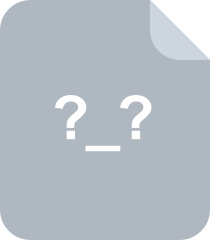
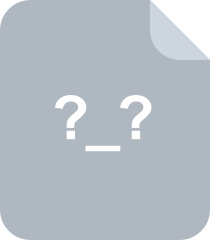
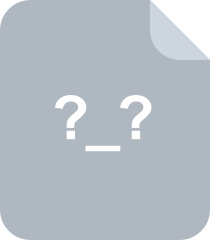
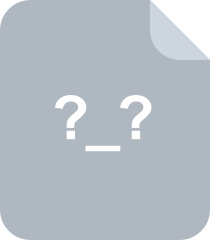
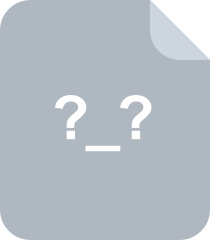
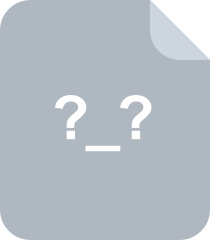
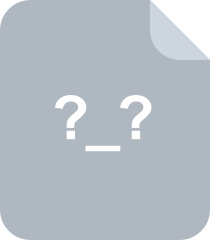
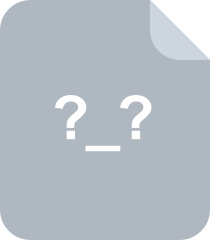
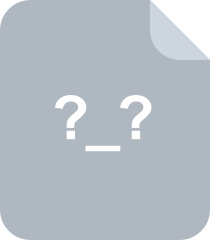
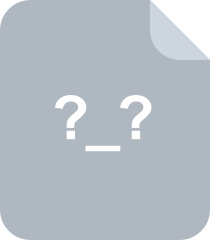
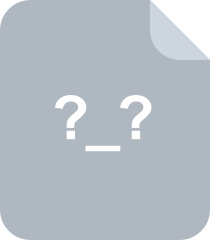
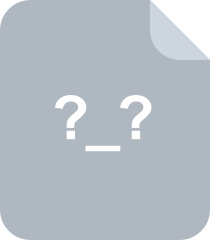
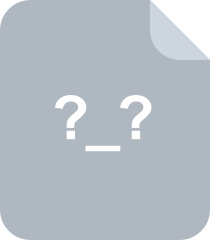
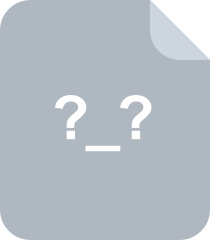
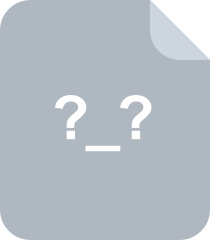
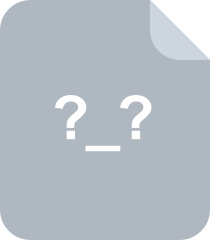
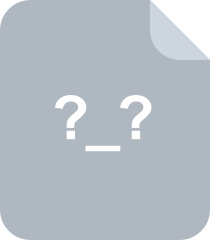
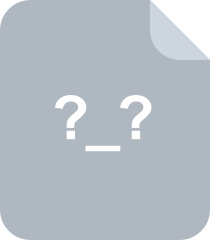
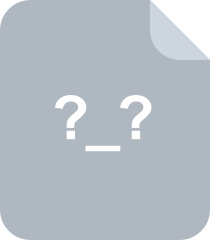
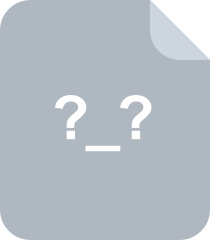
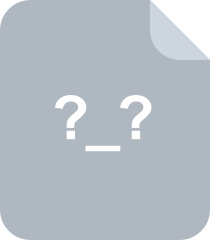
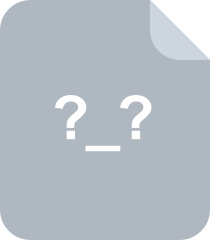
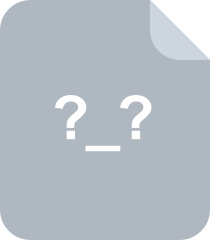
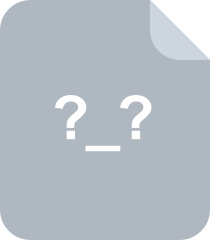
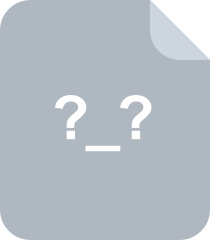
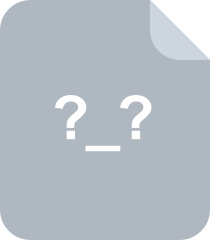
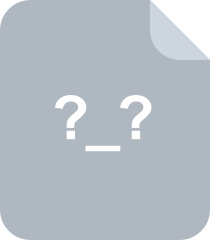
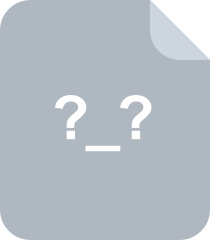
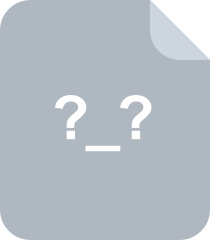
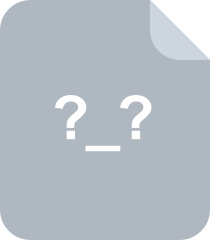
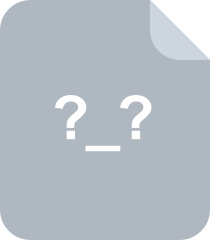
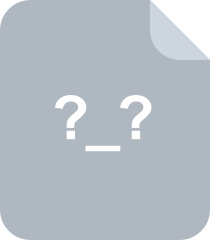
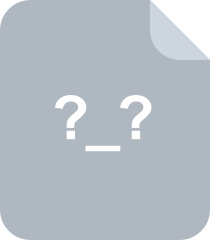
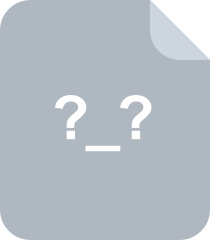
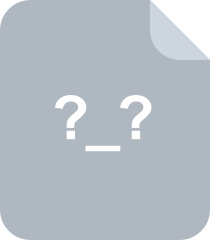
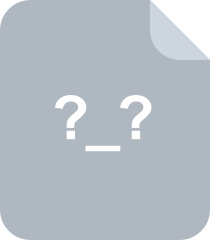
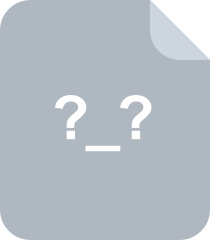
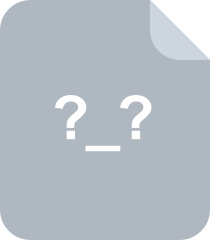
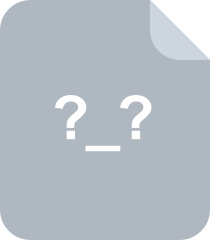
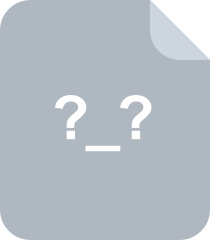
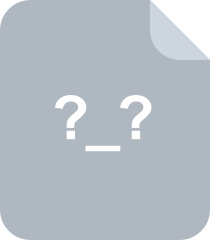
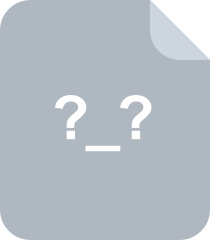
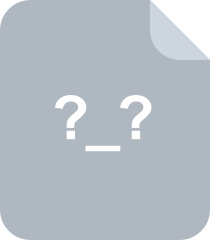
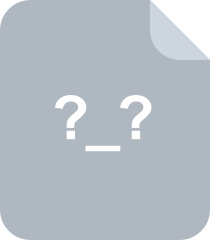
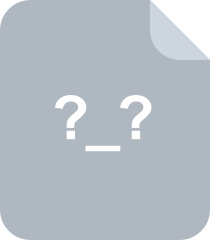
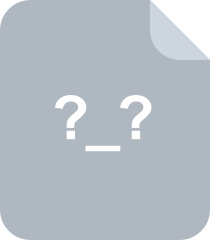
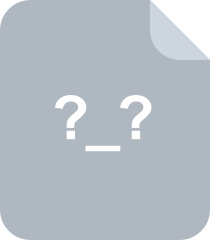
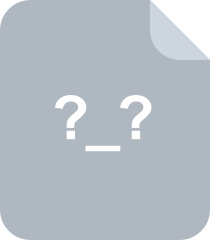
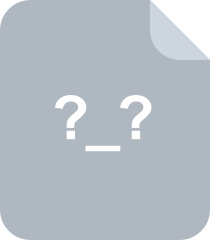
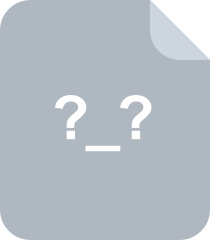
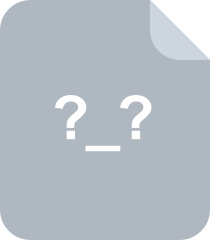
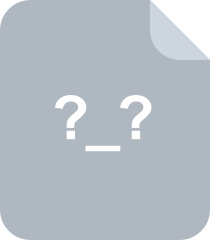
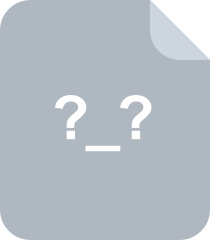
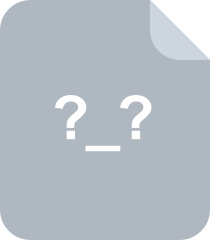
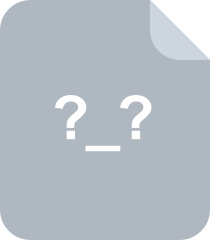
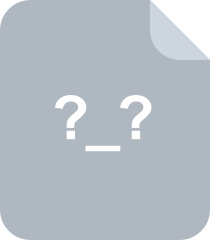
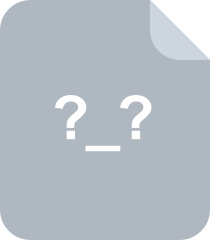
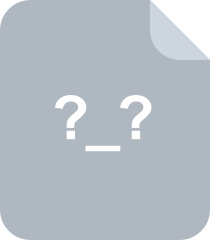
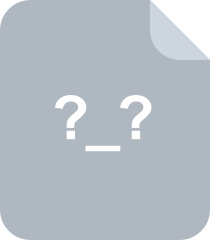
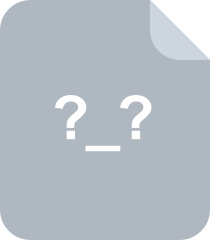
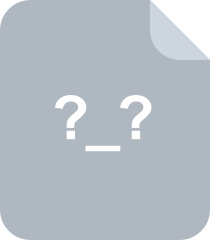
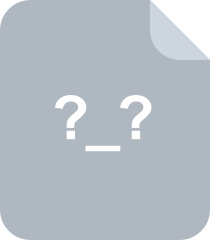
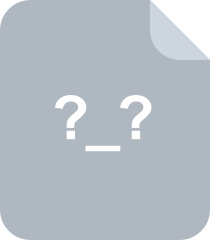
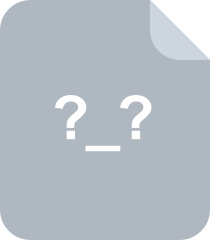
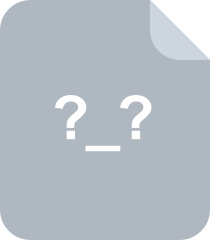
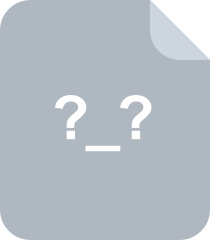
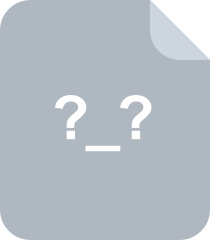
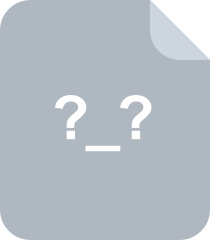
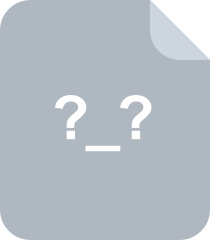
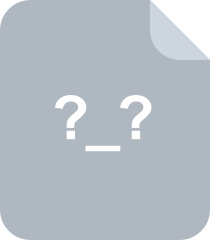
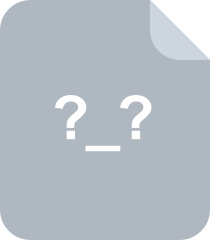
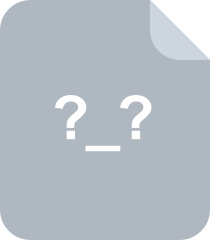
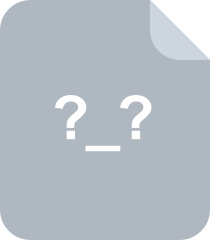
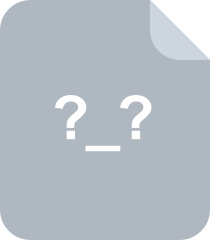
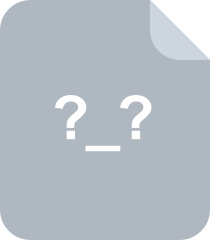
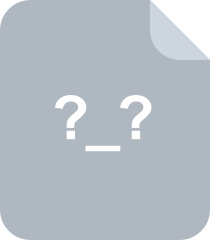
共 41074 条
- 1
- 2
- 3
- 4
- 5
- 6
- 411
资源评论
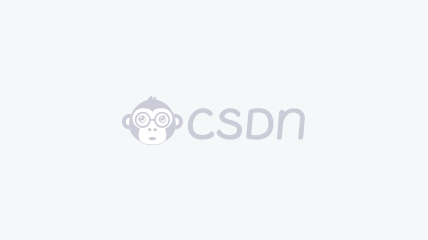

快撑死的鱼
- 粉丝: 2w+
- 资源: 9157
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

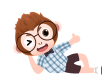
最新资源
- 浏览器新标签页个性化设置
- 1考试真题最近的t15.txt
- 1考试真题最近的t21.txt
- 硬币,骰子和网球检测12-YOLO(v5至v11)、COCO数据集合集.rar
- 浏览器插件-笔记高亮插件
- 1考试真题最近的t28.txt
- 1考试真题最近的t32.txt
- 1考试真题最近的t34.txt
- 1考试真题最近的t38.txt
- 【安卓源代码】数独联网对战APP(完整前后端+mysql+说明文档).zip
- 硬币,骰子和网球检测9-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 伦茨伺服PROFIBUS相关GSD
- 第八届蓝桥杯省赛单片机试题+基于CT107D单片机的电子钟程序设计与调试
- STM32F103RC-W5500实现8路TCP Client客户端并同时支持连接8路TCP Server服务端通讯.zip
- STM32F103RC-W5500实现DHCP自动获取IP地址.zip
- 硬币,骰子检测12-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


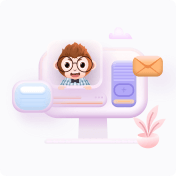
安全验证
文档复制为VIP权益,开通VIP直接复制
