# clj-memory-meter
This library is a thin wrapper
around [Java Agent for Memory Measurements](https://github.com/jbellis/jamm). It
allows to inspect at runtime how much memory an object occupies together with
all its child fields.
Extra features compared to **jamm**:
1. Easy runtime loading (you can start using it at any time at the REPL,
providing additional startup parameters is not necessary).
2. Human-readable size output.
**jamm** JAR file is shipped together with **clj-memory-meter** and unpacked at
runtime.
## Usage
**JDK9+:** you must start the JVM with option `-Djdk.attach.allowAttachSelf`,
otherwise the agent will not be able to dynamically attach to the running
process. For Leiningen, add `:jvm-opts ["-Djdk.attach.allowAttachSelf"]` to
`project.clj`. For Boot, start the process with environment variable
`BOOT_JVM_OPTIONS="-Djdk.attach.allowAttachSelf"`.
Add `com.clojure-goes-fast/clj-memory-meter` to your dependencies:
[](https://clojars.org/com.clojure-goes-fast/clj-memory-meter)
Once loaded, you can measure objects like this:
```clojure
(require '[clj-memory-meter.core :as mm])
;; measure calculates total memory occupancy of the object
(mm/measure "Hello, world!")
;=> "72 B"
(mm/measure [])
;=> "240 B"
(mm/measure (into {} (map #(vector % (str %)) (range 100))))
;=> "9.6 KB"
;; :shallow true calculates only memory occupied by the object itself,
;; without children
(mm/measure (object-array (repeatedly 100 #(String. "hello"))) :shallow true)
;=> "416 B"
(mm/measure (object-array (repeatedly 100 #(String. "hello"))))
;=> "2.8 KB"
;; :bytes true can be passed to return the raw number of bytes
(mm/measure (object-array (repeatedly 100 #(String. "hello"))) :bytes true)
;=> 2848
;; :debug true can be passed to print the object hierarchy. You can also pass an
;; integer number to limit the number of nested levels printed.
(mm/measure (apply list (range 4)) :debug true)
; root [clojure.lang.PersistentList] 256 bytes (40 bytes)
; |
; +--_first [java.lang.Long] 24 bytes (24 bytes)
; |
; +--_rest [clojure.lang.PersistentList] 192 bytes (40 bytes)
; |
; +--_first [java.lang.Long] 24 bytes (24 bytes)
; |
; +--_rest [clojure.lang.PersistentList] 128 bytes (40 bytes)
; |
; +--_first [java.lang.Long] 24 bytes (24 bytes)
; |
; +--_rest [clojure.lang.PersistentList] 64 bytes (40 bytes)
; |
; +--_first [java.lang.Long] 24 bytes (24 bytes)
;; Custom MemoryMeter object can be passed. See what you can configure here:
;; https://github.com/jbellis/jamm/blob/master/src/org/github/jamm/MemoryMeter.java
```
## License
jamm is distributed under Apache-2.0.
See [APACHE_PUBLIC_LICENSE](license/APACHE_PUBLIC_LICENSE) file. The location of the original
repository
is
[https://github.com/jbellis/jamm](https://github.com/jbellis/jamm).
---
clj-memory-meter is distributed under the Eclipse Public License.
See [ECLIPSE_PUBLIC_LICENSE](license/ECLIPSE_PUBLIC_LICENSE).
Copyright 2018-2020 Alexander Yakushev
没有合适的资源?快使用搜索试试~ 我知道了~
从 Clojure测量对象内存消耗_Clojure_代码_相关文件_下载
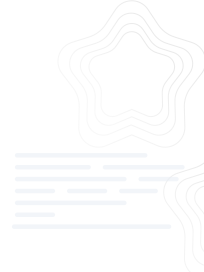
共8个文件
md:2个
jar:1个
boot:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 52 浏览量
2022-07-12
00:19:43
上传
评论
收藏 34KB ZIP 举报
温馨提示
这个库是Java Agent for Memory Measurements的一个瘦包装器。它允许在运行时检查对象及其所有子字段占用的内存量。 与jamm相比的额外功能: 轻松的运行时加载(您可以在 REPL 中随时开始使用它,无需提供额外的启动参数)。 人类可读的大小输出。 jamm JAR 文件与clj-memory-meter一起提供,并在运行时解压缩。 用法 JDK9+:必须使用 option 启动 JVM -Djdk.attach.allowAttachSelf,否则代理将无法动态附加到正在运行的进程。对于 Leiningen,添加:jvm-opts ["-Djdk.attach.allowAttachSelf"]到 project.clj. 对于 Boot,使用环境变量启动进程 BOOT_JVM_OPTIONS="-Djdk.attach.allowAttachSelf"。 添加com.clojure-goes-fast/clj-memory-meter到您的依赖项: 更多详情、使用方法,请下载后阅读README.md文件
资源推荐
资源详情
资源评论
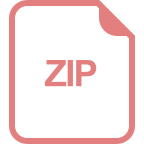
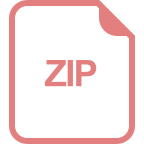
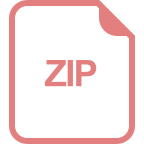
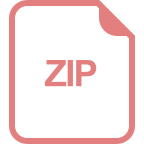
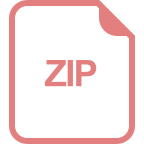
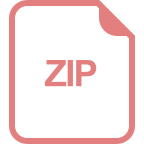
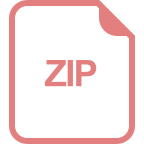
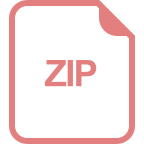
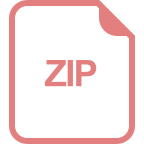
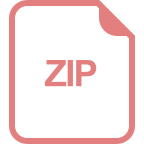
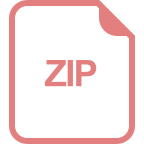
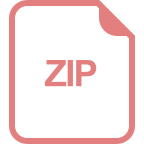
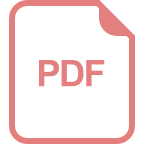
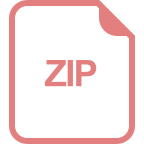
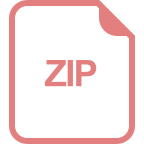
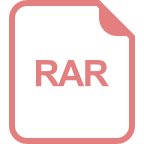
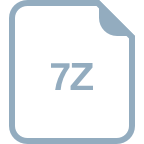
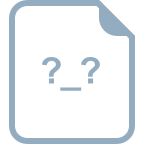
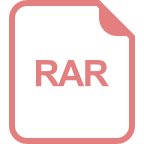
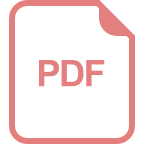
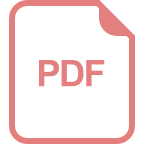
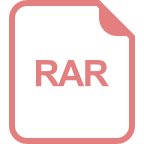
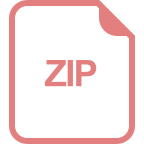
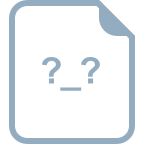
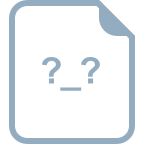
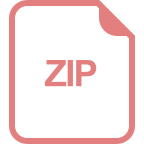
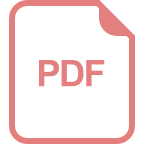
收起资源包目录



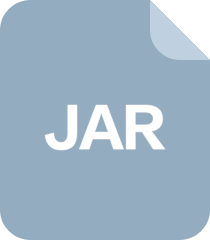

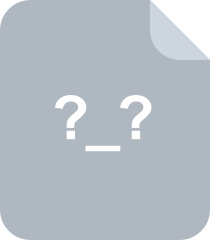
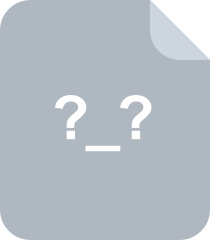


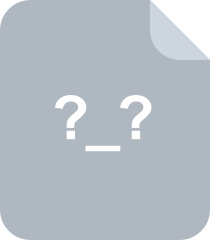
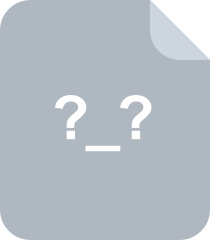
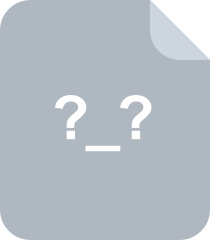
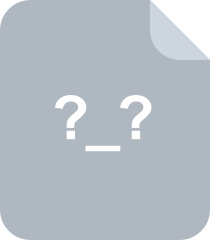
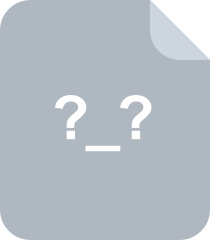
共 8 条
- 1
资源评论
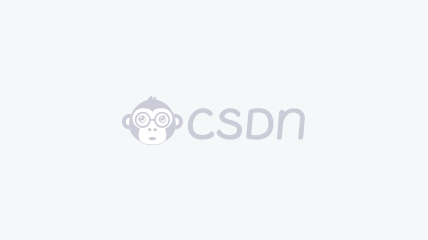

快撑死的鱼
- 粉丝: 1w+
- 资源: 9149
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

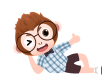
最新资源
- (源码)基于Spring Boot和Vue的后台管理系统.zip
- 用于将 Power BI 嵌入到您的应用中的 JavaScript 库 查看文档网站和 Wiki 了解更多信息 .zip
- (源码)基于Arduino、Python和Web技术的太阳能监控数据管理系统.zip
- (源码)基于Arduino的CAN总线传感器与执行器通信系统.zip
- (源码)基于C++的智能电力系统通信协议实现.zip
- 用于 Java 的 JSON-RPC.zip
- 用 JavaScript 重新实现计算机科学.zip
- (源码)基于PythonOpenCVYOLOv5DeepSort的猕猴桃自动计数系统.zip
- 用 JavaScript 编写的贪吃蛇游戏 .zip
- (源码)基于ASP.NET Core的美术课程管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


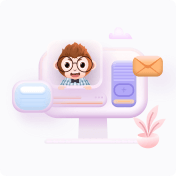
安全验证
文档复制为VIP权益,开通VIP直接复制
