/ _____) _ | |
( (____ _____ ____ _| |_ _____ ____| |__
\____ \| ___ | (_ _) ___ |/ ___) _ \
_____) ) ____| | | || |_| ____( (___| | | |
(______/|_____)_|_|_| \__)_____)\____)_| |_|
(C)2020 Semtech
LoRa concentrator HAL user manual
=================================
## 1. Introduction
The LoRa concentrator Hardware Abstraction Layer is a C library that allow you
to use a Semtech concentrator chip through a reduced number of high level C
functions to configure the hardware, send and receive packets.
The Semtech LoRa concentrator is a digital multi-channel multi-standard packet
radio used to send and receive packets wirelessly using LoRa or FSK modulations.
## 2. Components of the library
The library is composed of the following modules:
1. abstraction layer
* loragw_hal
* loragw_reg
* loragw_aux
* loragw_cal
* loragw_lbt
* loragw_sx1302
* loragw_sx1302_rx
* loragw_sx1302_timestamp
* loragw_sx125x
* loragw_sx1250
* loragw_sx1261
2. communication layer for sx1302
* loragw_com
* loragw_spi
* loragw_usb
3. communication layer for sx1255/SX1257 radios
* sx125x_com
* sx125x_spi
4. communication layer for sx1250 radios
* sx1250_com
* sx1250_spi
* sx1250_usb
5. communication layer for STM32 MCU (USB)
* loragw_mcu
6. communication layer for sx1261 radio (LBT / Spectral Scan)
* sx1261_com
* sx1261_spi
* sx1261_usb
7. peripherals
* loragw_i2c
* loragw_gps
* loragw_stts751
* loragw_ad5338r
The library also contains basic test programs to demonstrate code use and check
functionality.
### 2.1. loragw_hal
This is the main module and contains the high level functions to configure and
use the LoRa concentrator:
* lgw_board_setconf, to set the configuration of the concentrator
* lgw_rxrf_setconf, to set the configuration of the radio channels
* lgw_rxif_setconf, to set the configuration of the IF+modem channels
* lgw_txgain_setconf, to set the configuration of the concentrator gain table
* lgw_start, to apply the set configuration to the hardware and start it
* lgw_stop, to stop the hardware
* lgw_receive, to fetch packets if any was received
* lgw_send, to send a single packet (non-blocking, see warning in usage section)
* lgw_status, to check when a packet has effectively been sent
* lgw_get_trigcnt, to get the value of the sx1302 internal counter at last PPS
* lgw_get_instcnt, to get the value of the sx1302 internal counter
* lgw_get_eui, to get the sx1302 chip EUI
* lgw_get_temperature, to get the current temperature
* lgw_time_on_air, to get the Time On Air of a packet
* lgw_spectral_scan_start, to start scaning a particular channel
* lgw_spectral_scan_get_status, to get the status of the current scan
* lgw_spectral_scan_get_results, to get the results of the completed scan
* lgw_spectral_scan_abort, to abort curretn scan
For an standard application, include only this module.
The use of this module is detailed on the usage section.
/!\ When sending a packet, there is a delay (approx 1.5ms) for the analog
circuitry to start and be stable. This delay is adjusted by the HAL depending
on the board version (lgw_i_tx_start_delay_us).
In 'timestamp' mode, this is transparent: the modem is started
lgw_i_tx_start_delay_us microseconds before the user-set timestamp value is
reached, the preamble of the packet start right when the internal timestamp
counter reach target value.
In 'immediate' mode, the packet is emitted as soon as possible: transferring the
packet (and its parameters) from the host to the concentrator takes some time,
then there is the lgw_i_tx_start_delay_us, then the packet is emitted.
In 'triggered' mode (aka PPS/GPS mode), the packet, typically a beacon, is
emitted lgw_i_tx_start_delay_us microsenconds after a rising edge of the
trigger signal. Because there is no way to anticipate the triggering event and
start the analog circuitry beforehand, that delay must be taken into account in
the protocol.
### 2.2. loragw_reg
This module is used to access to the LoRa concentrator registers by name instead
of by address:
* lgw_connect, to initialise and check the connection with the hardware
* lgw_disconnect, to disconnect the hardware
* lgw_reg_r, read a named register
* lgw_reg_w, write a named register
* lgw_reg_rb, read a name register in burst
* lgw_reg_wb, write a named register in burst
* lgw_mem_rb, read from a memory section in burst
* lgw_mem_wb, write to a memory section in burst
This module handles read-only registers protection, multi-byte registers
management, signed registers management, read-modify-write routines for
sub-byte registers and read/write burst fragmentation to respect SPI/USB maximum
burst length constraints.
It make the code much easier to read and to debug.
Moreover, if registers are relocated between different hardware revisions but
keep the same function, the code written using register names can be reused "as
is".
If you need access to all the registers, include this module in your
application.
**/!\ Warning** please be sure to have a good understanding of the LoRa
concentrator inner working before accessing the internal registers directly.
### 2.3. loragw_com
This module contains the functions to access the LoRa concentrator register
array through the SPI or USB interfaces:
* lgw_com_r to read one byte
* lgw_com_w to write one byte
* lgw_com_rb to read two bytes or more
* lgw_com_wb to write two bytes or more
This modules is an abstract interface, it then relies on the following modules
to actually perform the interfacing:
* loragw_spi : for SPI interface
* loragw_usb : for USB interface
Please *do not* include that module directly into your application.
**/!\ Warning** Accessing the LoRa concentrator register array without the
checks and safety provided by the functions in loragw_reg is not recommended.
### 2.4. loragw_aux
This module contains a single host-dependant function wait_ms to pause for a
defined amount of milliseconds.
The procedure to start and configure the LoRa concentrator hardware contained in
the loragw_hal module requires to wait for several milliseconds at certain
steps, typically to allow for supply voltages or clocks to stabilize after been
switched on.
An accuracy of 1 ms or less is ideal.
If your system does not allow that level of accuracy, make sure that the actual
delay is *longer* that the time specified when the function is called (ie.
wait_ms(X) **MUST NOT** before X milliseconds under any circumstance).
If the minimum delays are not guaranteed during the configuration and start
procedure, the hardware might not work at nominal performance.
Most likely, it will not work at all.
### 2.5. loragw_gps
This module contains functions to synchronize the concentrator internal
counter with an absolute time reference, in our case a GPS satellite receiver.
The internal concentrator counter is used to timestamp incoming packets and to
triggers outgoing packets with a microsecond accuracy.
In some cases, it might be useful to be able to transform that internal
timestamp (that is independent for each concentrator running in a typical
networked system) into an absolute GPS time.
In a typical implementation a GPS specific thread will be called, doing the
following things after opening the serial port:
* blocking reads on the serial port (using system read() function)
* parse UBX messages (using lgw_parse_ubx) to get actual native GPS time
* parse NMEA sentences (using lgw_parse_nmea) to get location and UTC time
Note: the RMC sentence gives UTC time, not native GPS time.
And each time an NAV-TIMEGPS UBX message has been received:
* get the concentrator timestamp (using lgw_get_trigcnt, mutex needed to
protect access to the concentrator)
* get the GPS time contained in the UBX message (using lgw_gps_get)
* call the lgw_gps_sync function (use mutex to protect the time reference that
should be a global shared variable).
Then, in other threads, you can simply used that co
没有合适的资源?快使用搜索试试~ 我知道了~
SX1302/SX1303硬件抽象层和工具(包转发器...)_C语言_代码_相关文件_下载
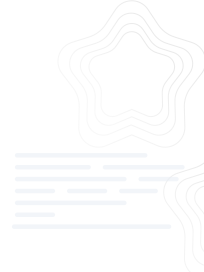
共132个文件
c:53个
h:36个
md:9个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
此目录包含库的源代码,用于构建基于 Semtech LoRa SX1302 集中器芯片(又名集中器)的网关。编译后,所有代码都包含在 libloragw.a 文件中,该文件将被静态链接(即集成到最终的可执行文件中)。 该库还附带了一些基本测试程序,用于测试库的不同子模块。 2. 帮助程序 这些程序包含在项目中,以提供有关如何使用 HAL 库的示例,并帮助系统构建者测试它的不同部分。 2.1。包转发器 包转发器是运行在 Lora 网关主机上的程序,它将集中器接收到的 RF 包通过 IP/UDP 链路转发到服务器,并发出服务器发送的 RF 包。 更多详情、使用方法,请下载后阅读README.md文件
资源推荐
资源详情
资源评论
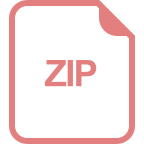
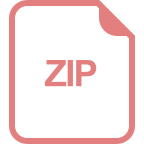
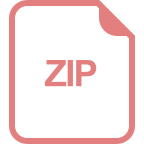
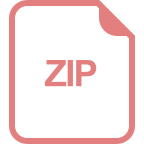
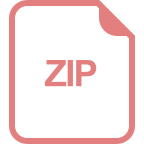
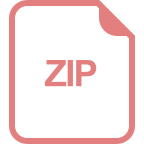
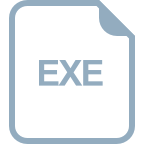
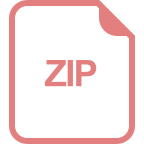
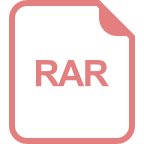
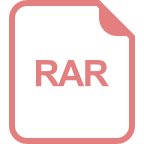
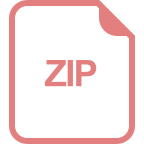
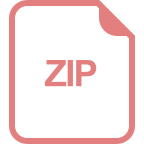
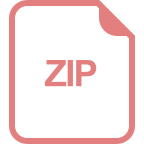
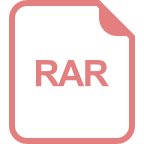
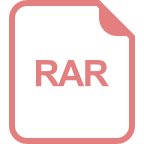
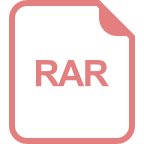
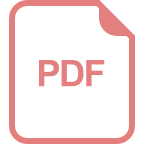
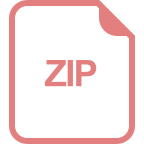
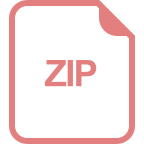
收起资源包目录

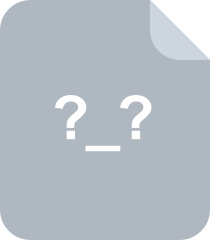
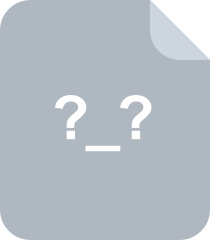
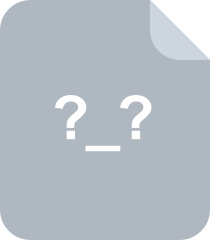
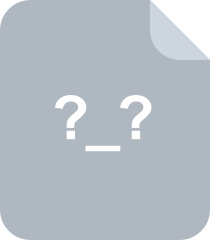
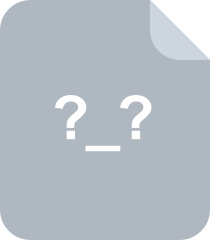
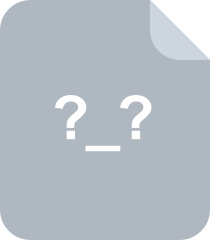
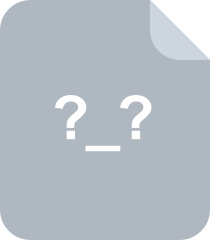
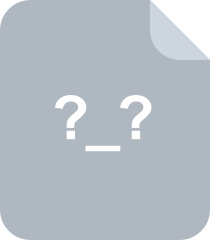
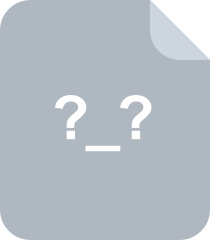
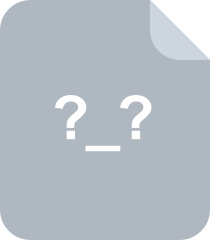
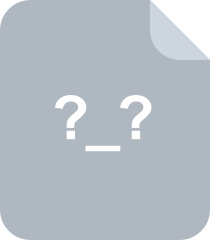
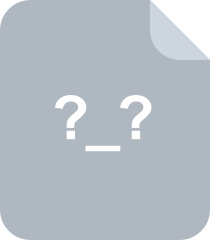
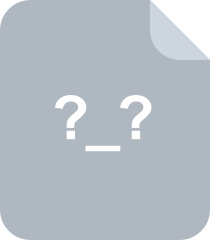
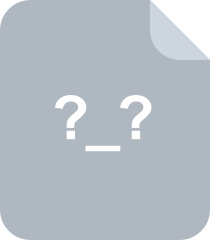
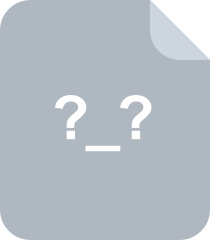
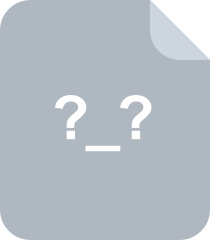
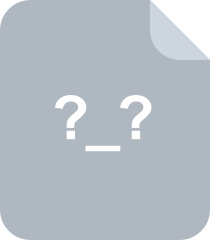
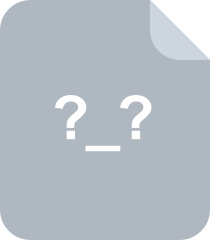
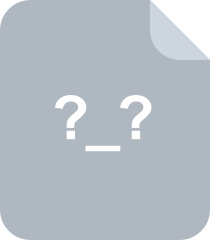
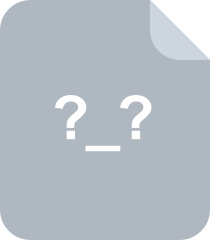
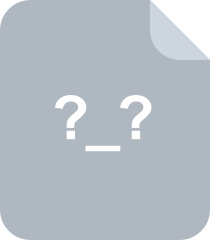
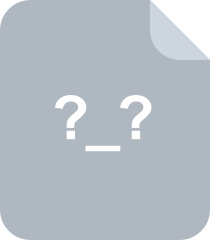
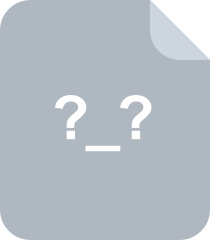
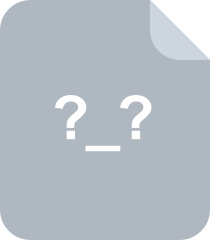
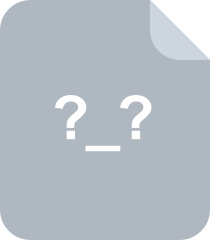
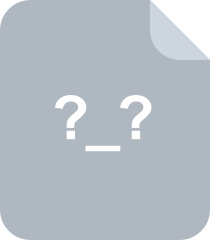
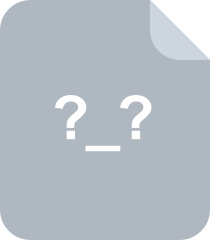
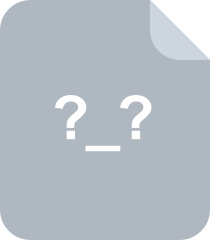
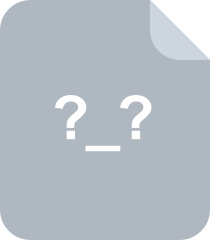
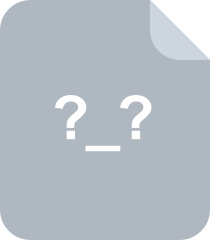
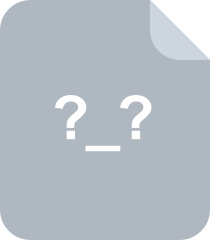
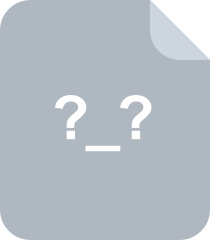
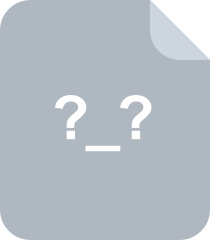
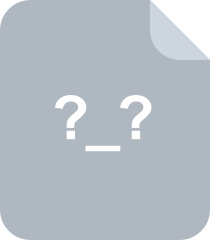
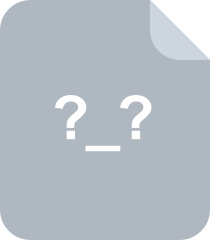
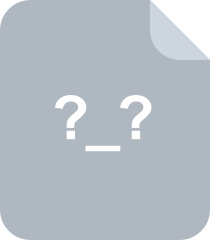
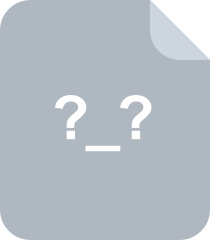
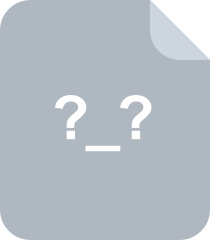
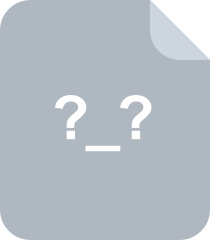
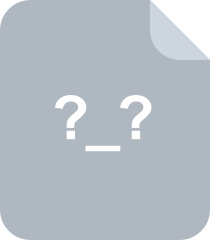
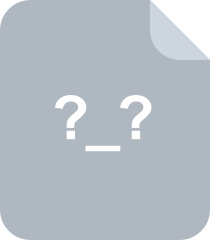
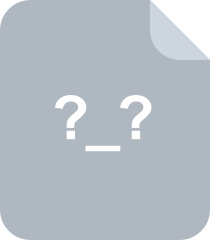
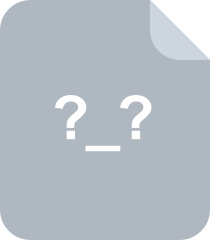
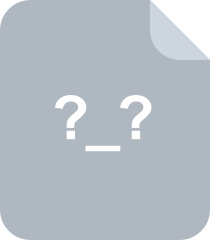
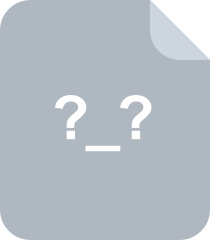
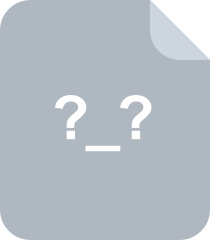
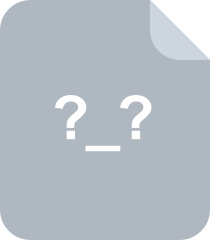
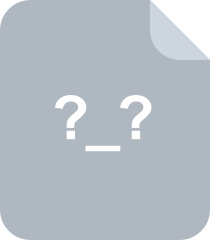
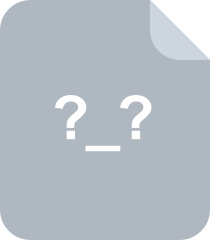
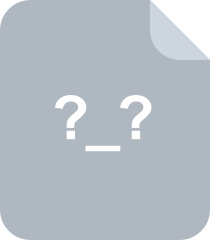
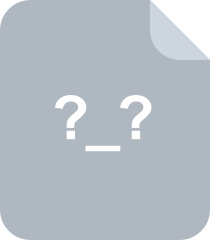
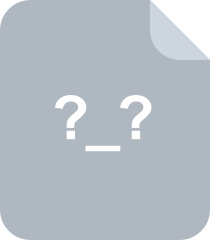
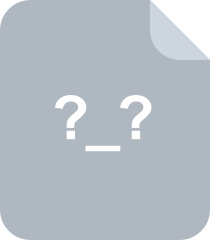
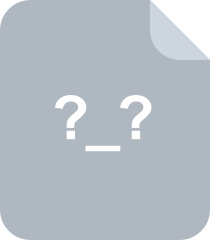
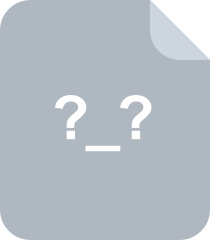
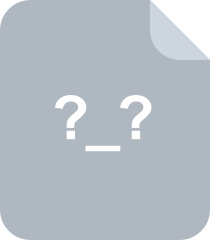
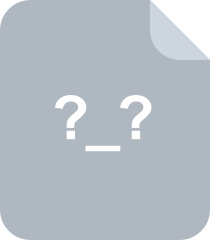
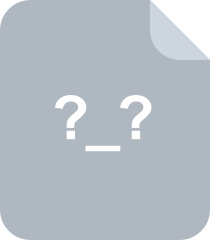
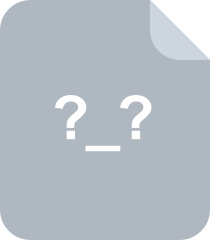
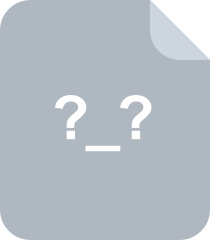
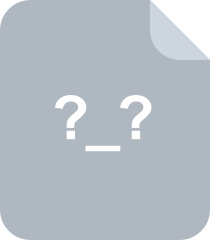
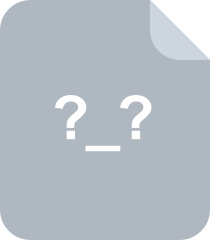
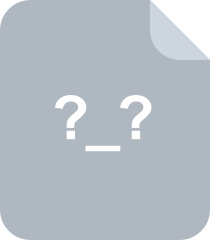
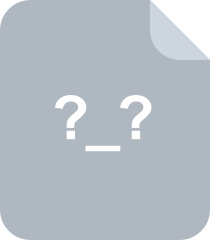
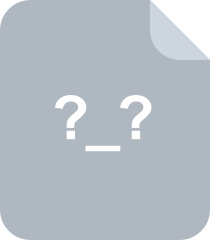
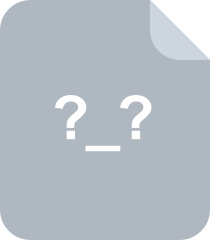
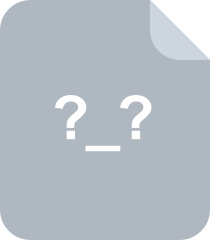
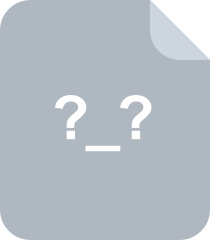
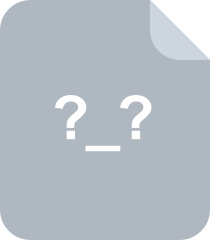
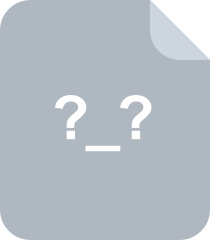
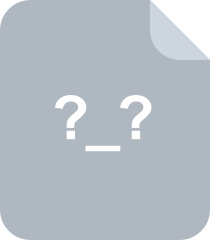
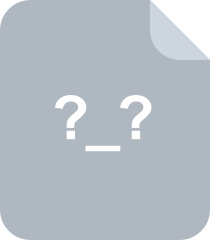
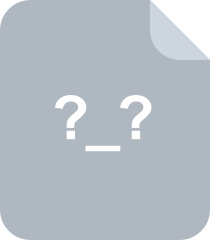
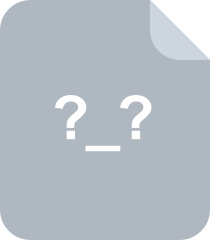
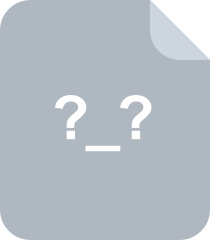
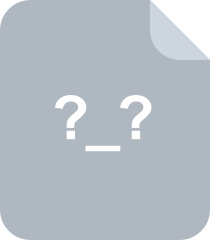
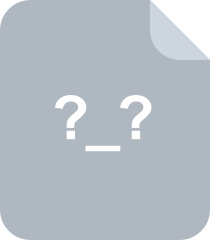
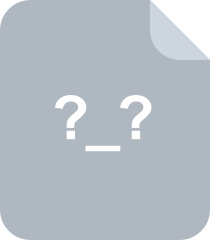
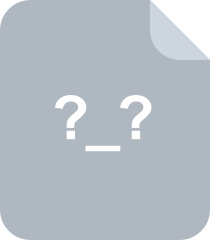
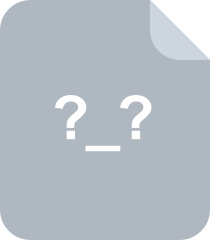
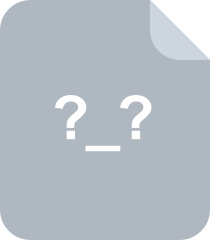
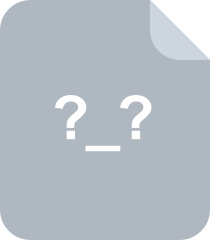
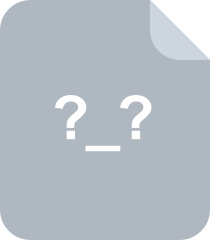
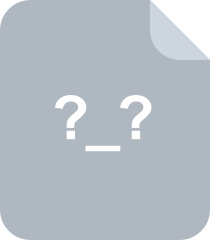
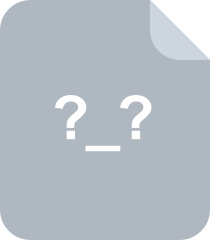
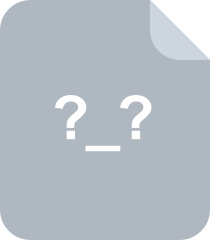
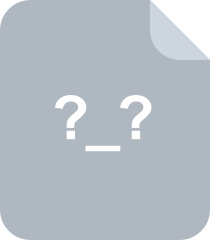
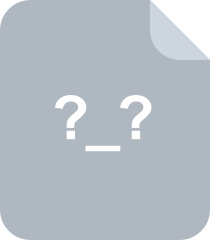
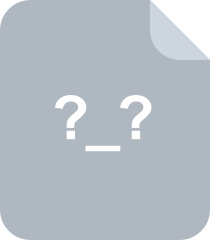
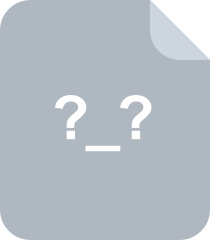
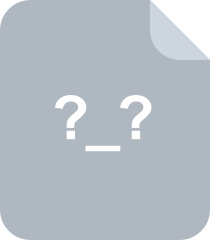
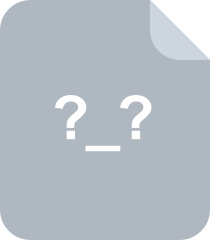
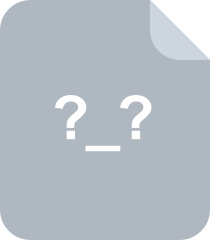
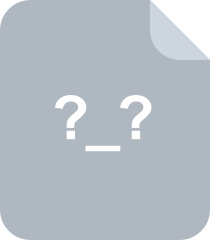
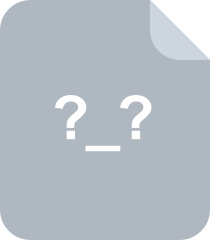
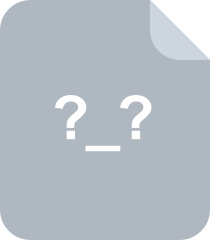
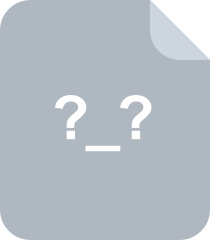
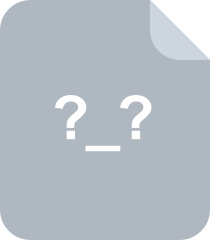
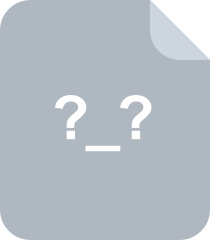
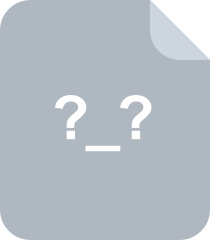
共 132 条
- 1
- 2

快撑死的鱼
- 粉丝: 1w+
- 资源: 9150

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

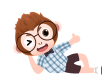
最新资源
- 【java毕业设计】springboot的租房管理系统(springboot+vue+mysql+说明文档).zip
- 【java毕业设计】论坛管理系统源码(springboot+vue+mysql+说明文档+LW).zip
- Stateflow简单实例
- 【java毕业设计】留守儿童爱心网站源码(springboot+vue+mysql+说明文档+LW).zip
- 基于PHP+MySql实现的图书管理系统+项目源码+文档说明
- 【java毕业设计】乐校园二手书交易管理系统源码(springboot+vue+mysql+说明文档+LW).zip
- DeBiFormer实战:使用DeBiFormer实现图像分类任务
- SunnyUI-786741.rar
- 【java毕业设计】篮球论坛系统源码(springboot+vue+mysql+说明文档+LW).zip
- 【java毕业设计】篮球竞赛预约平台源码(springboot+vue+mysql+说明文档+LW+LW).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


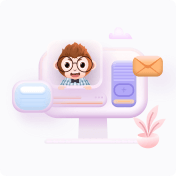
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页