# PHP Content Editable
<img align="right" width="250" src="https://github.com/acbrandao/templates/blob/master/img/html5.PNG">
This is an example of using PHP to update a contentEditable webpaget.
Basically this script allows the designated and tagged <Div> blocks to be edited and re-written
back into the HTML file.
## Installation
Simlply clone this folder, or click on the file you're interested in and just copy it to your working direcotry.
```bash
git clone git@github.com:acbrandao/PHP.git
```
### General Approach
- The plain HTML file we want to edit , which holds our content, we’ll call this content.html
- The PHP script named index.php which reads and write the content.html and saves the changes
We first begin by just reading and displaying the content.html , and we also have a few {{placeholders}} inside content.html to replace things like title .
We also add a little allowEdit javascript within the content.html to basically put it into edit mode. This examples uses a very simplistic password prompt, but its recommended you secure the page more carefully for production systems. The prompt can be initiated by a button (as in this example) or via a secret queryString parameter, use your best judgement how to enabled editing.
```javascirpt
<input type="hidden" name="pw" value="" id="pw"><button type="submit" class="btn btn-primary" onclick="return allowEdit()"> Edit </button>
<script>
function allowEdit()
{
var result= prompt('Credentials for editing. Make more secure. This is for demo purposes.');
document.getElementById('pw').value=result;
return result;
}
</script>
```
After the password prompt, we send the request back to the same index.php which checks that the user supplied password against our “secret” hash and if they match, reloads the content.html replacing the `<div class="editable" id="ce-1">` with tags with `<div class="editable" contentEditabe="true" id="ce-1">` This then allows the user to make the edits inside the browser.
Next we have an editable onBlur event handler which sends the changes back to the **index.php** to overwrite them to the content.html . The actual code that does this is below.
```php
//Ok lets re-write the content for this page
if ( isset($_REQUEST['action']) && $_REQUEST['action']=="write" && isset($_REQUEST['id']) ) //
{
//REQUIRES THAT DOMDocument is available
//https://www.php.net/manual/en/class.domdocument.php
//in order to properly parse HTML file and append to it.
$doc = new DOMDocument(); //instantiate the HTML
$doc->loadHTML($page_html); //load the HTML into the DomDocument Parser
$id=$_REQUEST['id'];
$replacement_html = htmlspecialchars( trim($_REQUEST['content']) );
echo "Editing content //*[@id='".$id."']"; //debug messages can be remove
echo "\n Replacing with contnet \n"; //debug messages can be remove
echo $replacement_html ;
//Now walk the matching Nodes and REPLACE with the new fragment
$xpath = new DOMXPath($doc); //setup an Xpath Query object
$nodes = $xpath->query("//*[@id='".$id."']"); //search for our id
foreach($nodes as $node) {
$newnode = $doc->createDocumentFragment();
if ( isset($replacement_html) )
{
$newnode->appendXML($replacement_html); //new content
// $node->appendChild($newnode); //replace the existing content
$node->parentNode->replaceChild($newnode, $node);
}
}
//write the update page (Entire Page back to disk)
if (is_writable($html_filename))
{
$bytes= $doc->saveHTMLFile($html_filename);
echo "\n Success wrote $html_filename with $bytes bytes \n";
}
else
die("File $html_filename cannot not be written to check permissions");
//return status and exit here.
die("\n Success AJAX Function write Content");
}
```
## Usage
Create a plain Plain HTML page then place your content inside structure like this
```html
<div class="editable" id="ce-1">Your content inside here </div>
```
the **class="editable"** will tell the PHP file to make the content located inside editable.
Thne assign an eventHanlder on Blur to write those changes back to the disk.
## Security Cosniderations
Please note using this technique on a live PUBLIC INTERNET site, with minimal security considerations is just inviting trouble, since, we’re allowing folks to **edit and overwrite** our html files system directly we are potentially exposing our site to some potential mischeif.
So in addition to the simplistic password prompt, we may want to sanitize the edited HTML prior to writing it to disk, escaping any offending or malicious user input. And generallly make editing these pages much more secure.
## Contributing
1. Fork it!
2. Create your feature branch: `git checkout -b my-new-feature`
3. Commit your changes: `git commit -am 'Add some feature'`
4. Push to the branch: `git push origin my-new-feature`
5. Submit a pull request :D
## License
This code is licensed under the MIT license. See https://opensource.org/licenses/MIT for details.
没有合适的资源?快使用搜索试试~ 我知道了~
PHP 相关项目:如简单的 PHP遗传 算法、LDAP 登录、Websockets 等_JavaScript_代码_下载
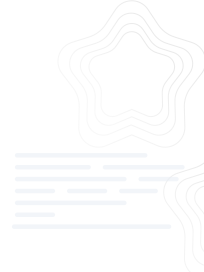
共60个文件
php:18个
md:10个
js:7个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 13 浏览量
2022-06-18
23:08:25
上传
评论
收藏 952KB ZIP 举报
温馨提示
引导网格 Bootstrap Bootgrid with PHP json server side script 是一个脚本,允许您使用 BootGrid 快速有效地渲染服务器端数据 PHP/引导程序 嘎 OOP 格式的 PHP 遗传算法代码 PHP wp_nas_rsync 用于将 Wordpress 与异地 nas 同步的 Shell 脚本 重击 ws PHP Websocket 客户端和服务器演示 PHP phpLDAPWindows PHP 示例连接到 LDAP 服务器 PHP phpDBLogEvent 如何将 CLI 事件记录到 MySQL 或 SQLite 的 PHP 示例 PHP simple_cahce PHP 如何轻松地将动态页面缓存到静态文本页面中 PHP phpContent编辑 使用带有 Ajax 和 PHP 后端的 HTML5 contentEditable 来更新网页 PHP 数学验证码 简单的 PHP 匹配基于 cpatcha 的输入检查 PHP
资源推荐
资源详情
资源评论
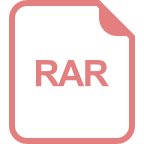
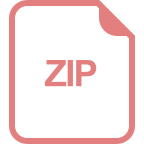
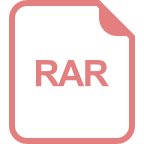
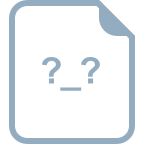
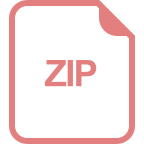
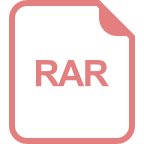
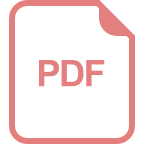
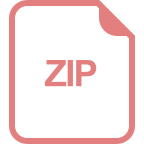
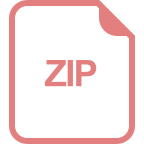
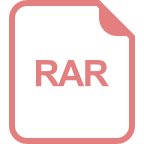
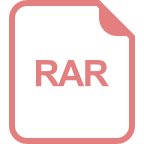
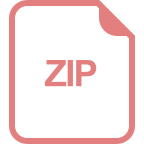
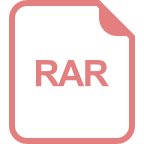
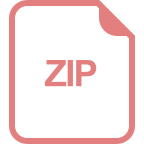
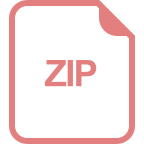
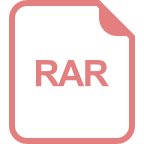
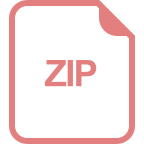
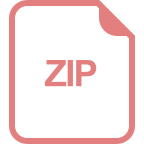
收起资源包目录



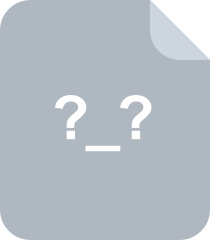
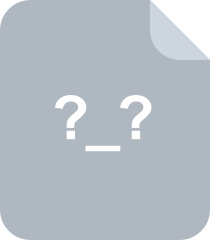
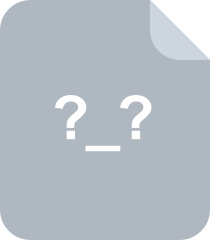
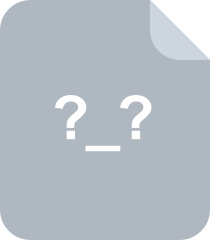

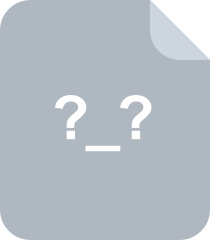
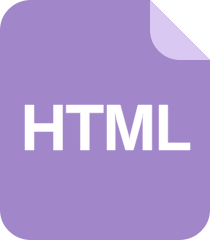
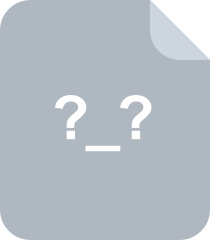
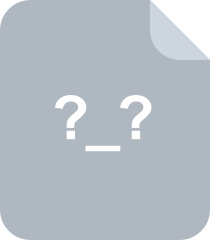

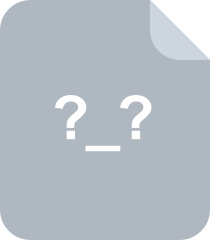
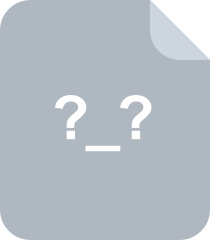
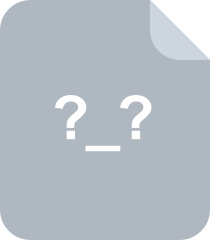
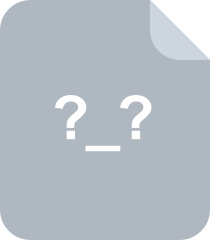
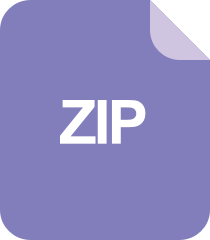
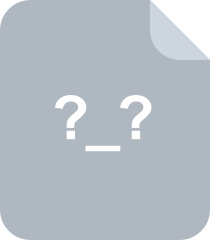
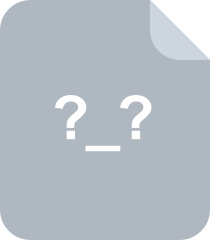
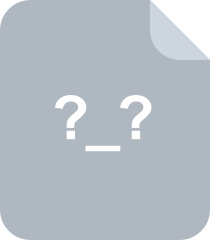
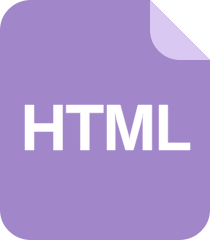
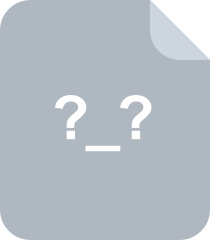

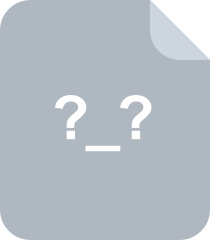
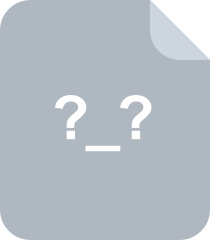
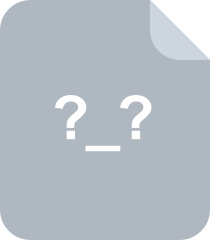
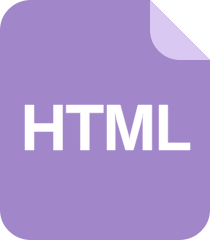
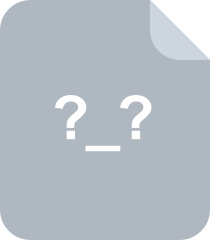

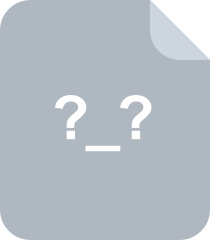
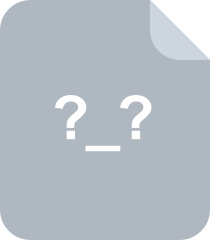


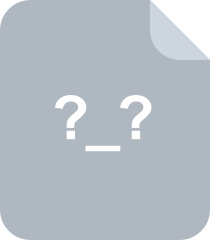
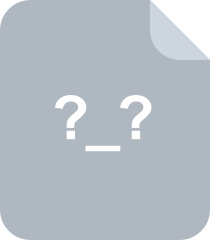
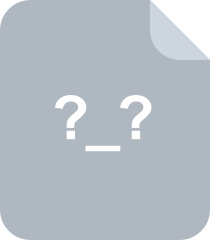
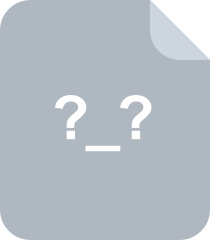
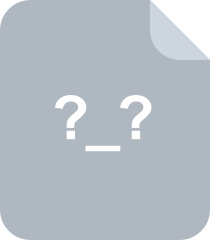
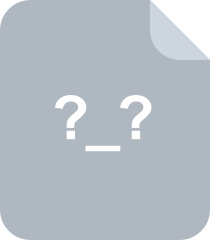
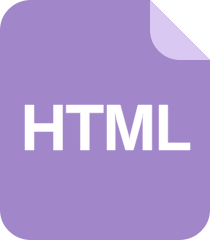
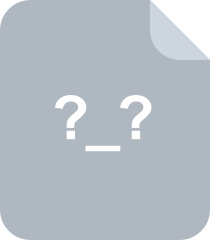

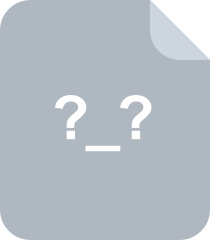
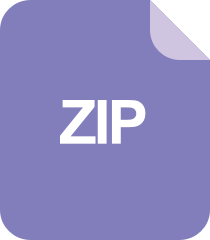

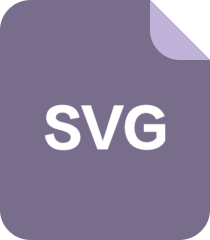
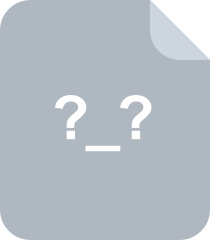
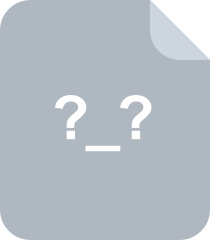
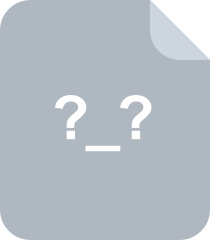
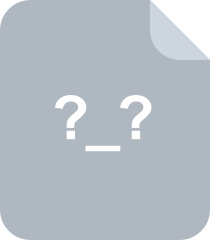

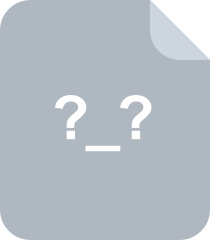
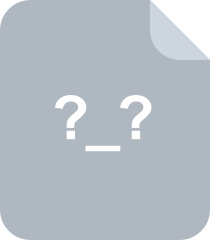
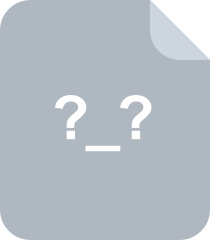
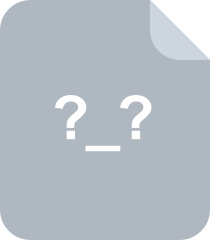
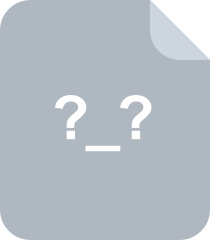
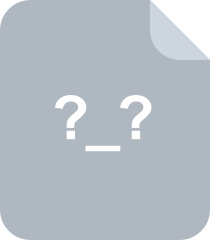
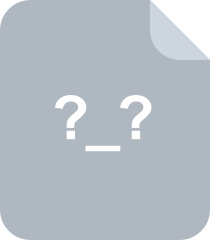
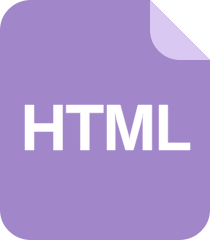
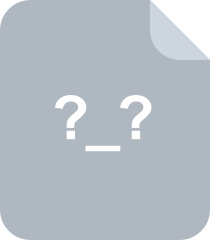

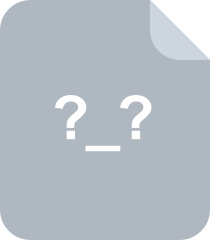
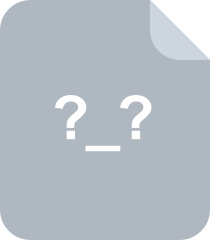

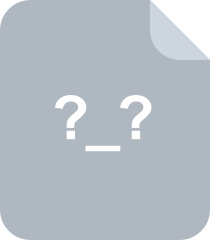
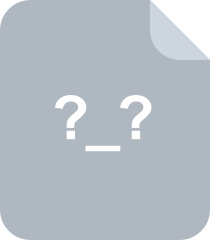
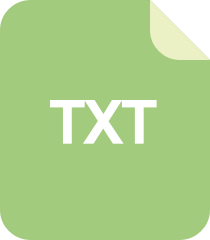

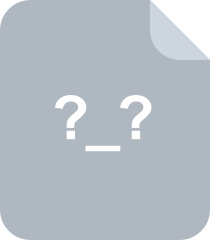
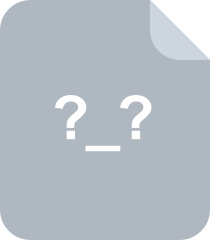
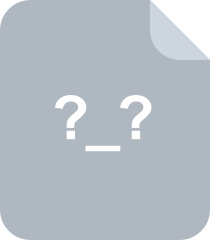
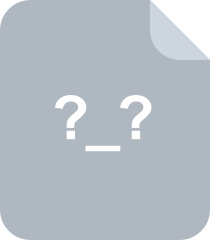
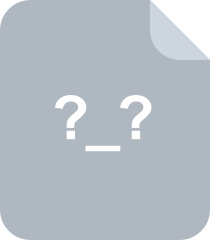
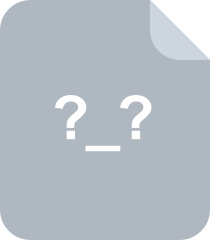
共 60 条
- 1
资源评论
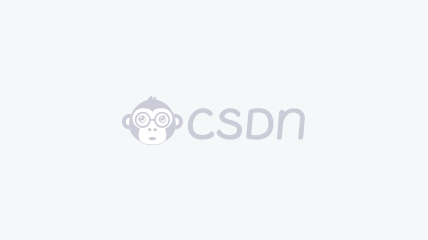

快撑死的鱼
- 粉丝: 1w+
- 资源: 9149
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

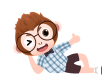
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


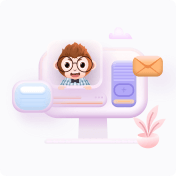
安全验证
文档复制为VIP权益,开通VIP直接复制
