[](https://github.com/karolzak/keras-unet/actions?query=workflow%3A%22Python+package%22)
[](https://pypi.org/project/keras-unet/)
[](https://pepy.tech/project/keras-unet)
[](https://pepy.tech/project/keras-unet/month)
[](https://github.com/karolzak/keras-unet/blob/master/LICENSE)
<!--  -->
**Share**:
[](http://twitter.com/share?text=Check%20out%20Keras-Unet%20Python%20package%20which%20helps%20you%20to%20build%20image%20segmentation%20models%20in%20TF%20and%20Keras&url=https://github.com/karolzak/keras-unet/&hashtags=python,computervision,semanticsegmentation,unet,keras,tensorflow,deeplearning,ML,AI)
[](http://www.linkedin.com/shareArticle?mini=true&url=https://github.com/karolzak/keras-unet&title=Keras%20UNet%20python%20package)
# About
Helper package with multiple U-Net implementations in Keras as well as useful utility tools helpful when working with image segmentation tasks
# Features:
- [x] U-Net models implemented in Keras
- [x] Vanilla U-Net implementation based on [the original paper](https://arxiv.org/pdf/1505.04597.pdf)
- [x] Customizable U-Net
- [x] U-Net optimized for satellite images based on [DeepSense.AI Kaggle competition entry](https://deepsense.ai/deep-learning-for-satellite-imagery-via-image-segmentation/)
- [x] Utility functions:
- [x] Plotting images and masks with overlay
- [x] Plotting images masks and predictions with overlay (prediction on top of original image)
- [x] Plotting training history for metrics and losses
- [x] Cropping smaller patches out of bigger image (e.g. satellite imagery) using sliding window technique (also with overlap if needed)
- [x] Plotting smaller patches to visualize the cropped big image
- [x] Reconstructing smaller patches back to a big image
- [x] Data augmentation helper function
- [x] Notebooks (examples):
- [x] Training custom U-Net for whale tails segmentation
- [ ] Semantic segmentation for satellite images
- [x] Semantic segmentation for medical images [ISBI challenge 2015](https://biomedicalimaging.org/2015/program/isbi-challenges/)
# Installation:
```bash
pip install git+https://github.com/karolzak/keras-unet
```
or
```bash
pip install keras-unet
```
# Usage examples:
- U-Net implementations in Keras:
- [Vanilla U-Net](#Vanilla-U-Net)
- [Customizable U-Net](#Customizable-U-Net)
- [U-Net for satellite images](#U-Net-for-satellite-images)
- Utils:
- [Plot training history](#Plot-training-history)
- [Plot images and segmentation masks](#Plot-images-and-segmentation-masks)
- [Get smaller patches/crops from bigger image](#Get-smaller-patches/crops-from-bigger-image)
- [Plot small patches into single big image](#Plot-small-patches-into-single-big-image)
- [Reconstruct a bigger image from smaller patches/crops](#Reconstruct-a-bigger-image-from-smaller-patches/crops)
<br>
### Vanilla U-Net
[Model scheme can be viewed here](https://raw.githubusercontent.com/karolzak/keras-unet/master/docs/vanilla_unet.png)
```python
from keras_unet.models import vanilla_unet
model = vanilla_unet(input_shape=(512, 512, 3))
```
[[back to usage examples]](#usage-examples)
<br>
### Customizable U-Net
[Model scheme can be viewed here](https://raw.githubusercontent.com/karolzak/keras-unet/master/docs/custom_unet.png)
```python
from keras_unet.models import custom_unet
model = custom_unet(
input_shape=(512, 512, 3),
use_batch_norm=False,
num_classes=1,
filters=64,
dropout=0.2,
output_activation='sigmoid')
```
[[back to usage examples]](#usage-examples)
<br>
### U-Net for satellite images
[Model scheme can be viewed here](https://raw.githubusercontent.com/karolzak/keras-unet/master/docs/satellite_unet.png)
```python
from keras_unet.models import satellite_unet
model = satellite_unet(input_shape=(512, 512, 3))
```
[[back to usage examples]](#usage-examples)
<br>
### Plot training history
```python
history = model.fit_generator(...)
from keras_unet.utils import plot_segm_history
plot_segm_history(
history, # required - keras training history object
metrics=['iou', 'val_iou'], # optional - metrics names to plot
losses=['loss', 'val_loss']) # optional - loss names to plot
```
Output:
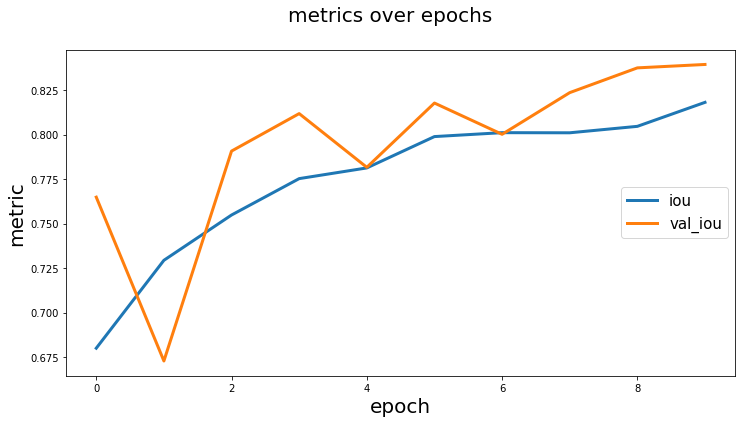
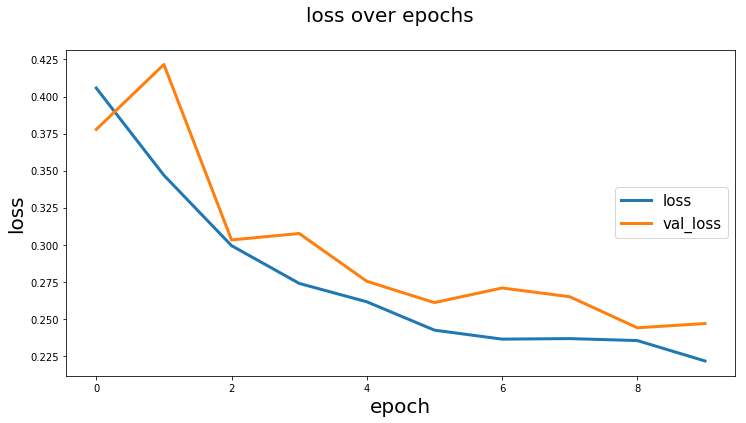
[[back to usage examples]](#usage-examples)
<br>
### Plot images and segmentation masks
```python
from keras_unet.utils import plot_imgs
plot_imgs(
org_imgs=x_val, # required - original images
mask_imgs=y_val, # required - ground truth masks
pred_imgs=y_pred, # optional - predicted masks
nm_img_to_plot=9) # optional - number of images to plot
```
Output:
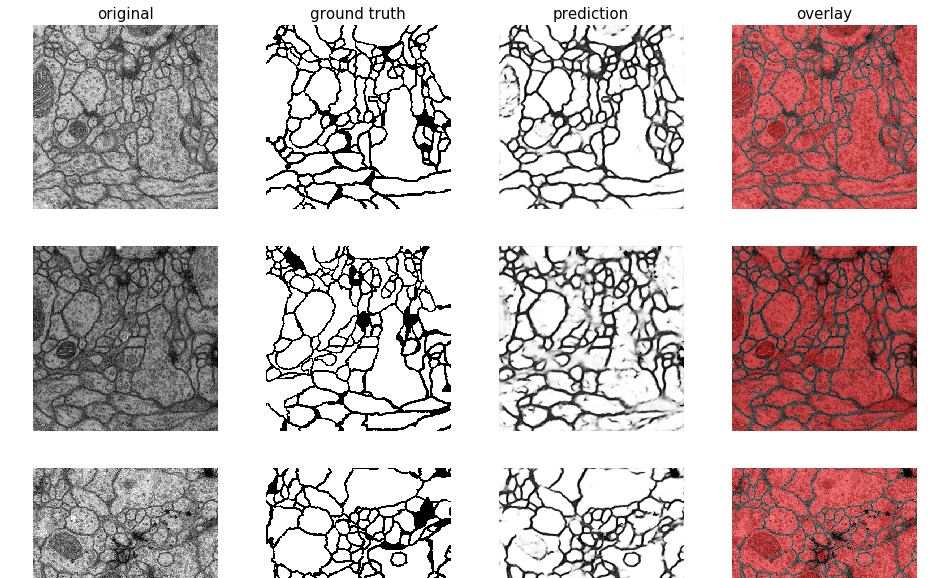
[[back to usage examples]](#usage-examples)
<br>
### Get smaller patches/crops from bigger image
```python
from PIL import Image
import numpy as np
from keras_unet.utils import get_patches
x = np.array(Image.open("../docs/sat_image_1.jpg"))
print("x shape: ", str(x.shape))
x_crops = get_patches(
img_arr=x, # required - array of images to be cropped
size=100, # default is 256
stride=100) # default is 256
print("x_crops shape: ", str(x_crops.shape))
```
Output:
```output
x shape: (1000, 1000, 3)
x_crops shape: (100, 100, 100, 3)
```
[[back to usage examples]](#usage-examples)
<br>
### Plot small patches into single big image
```python
from keras_unet.utils import plot_patches
print("x_crops shape: ", str(x_crops.shape))
plot_patches(
img_arr=x_crops, # required - array of cropped out images
org_img_size=(1000, 1000), # required - original size of the image
stride=100) # use only if stride is different from patch size
```
Output:
```output
x_crops shape: (100, 100, 100, 3)
```
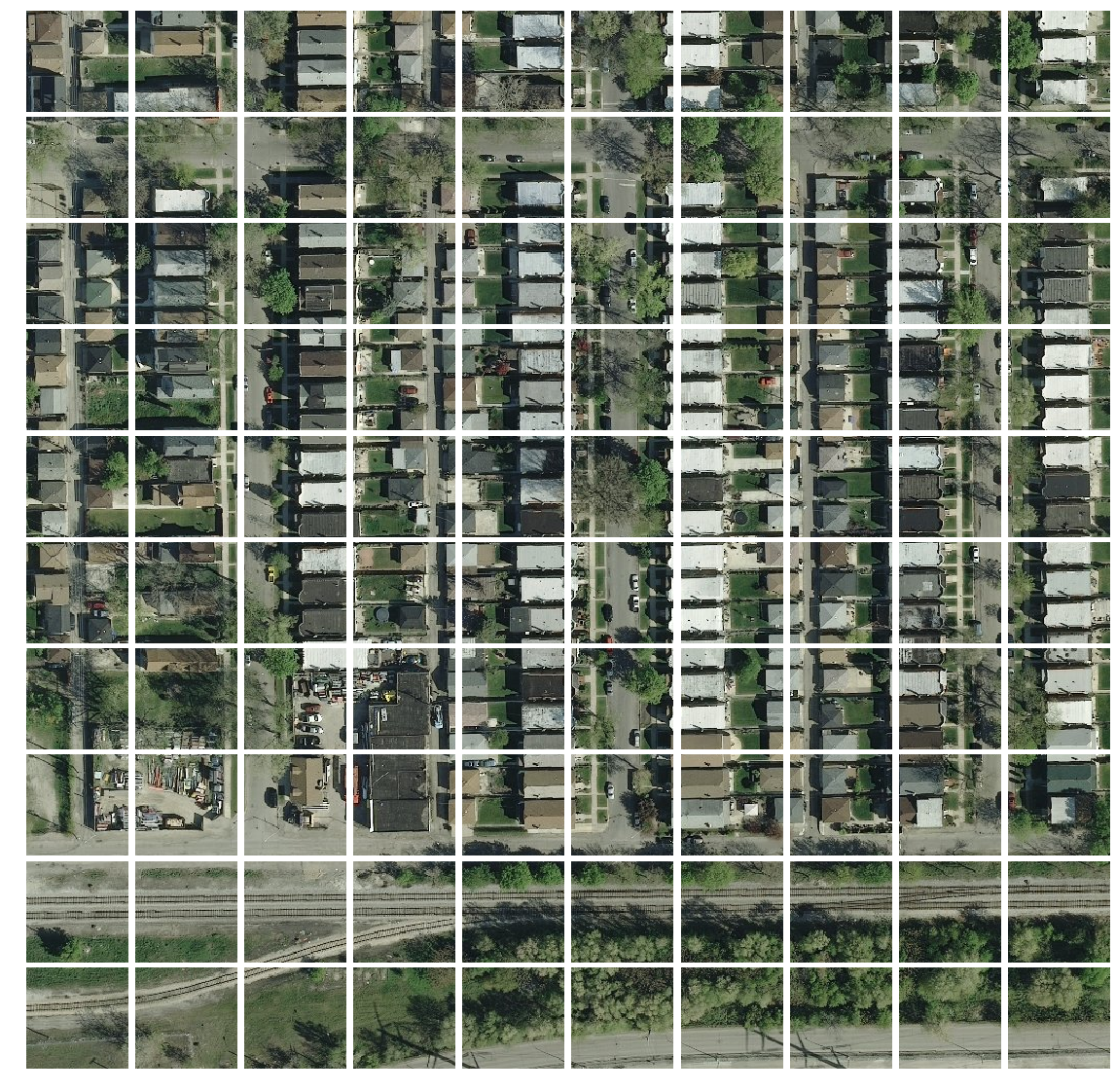
[[back to usage examples]](#usage-examples)
<br>
### Reconstruct a bigger image from smaller patches/crops
```python
import matplotlib.pyplot as plt
from keras_unet.utils import reconstruct_from_patches
print("x_crops shape: ", str(x_crops.shape))
x_reconstructed = reconstruct_from_patches(
img_arr=x_crops, # required - array of cropped out images
org_img_size=(1000, 1000), # required - original size of the image
stride=100) # use only if stride is different from patch size
print("x_reconstructed shape: ", str(x_reconstructed.shape))
plt.figure(figsize=(10,10))
plt.imshow(x_reconstructed[0])
plt.show()
```
Output:
```output
x_crops shape: (100, 100, 100, 3)
x_reconstructed shape: (1, 1000, 1000, 3)
```
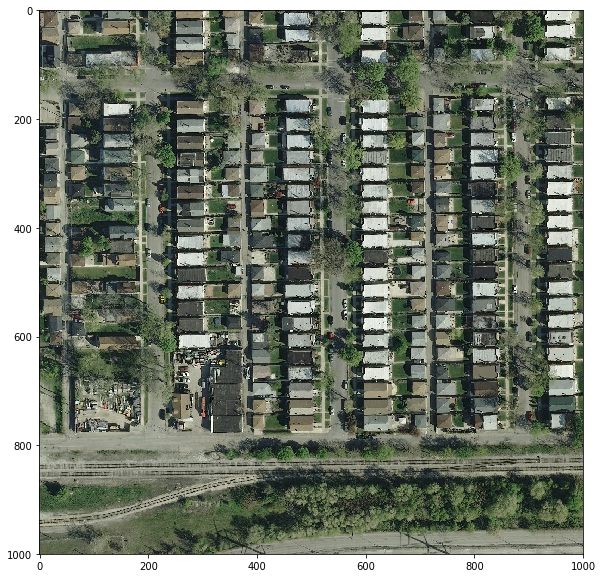
[[back to usage examples]](#usage-examples)

快撑死的鱼
- 粉丝: 2w+
- 资源: 9155
最新资源
- 基于氩气、空气、氧气填充床的DBD介质阻挡放电仿真研究-Comsol等离子体模块应用与沿面放电特性分析,氩气、空气与氧气填充床DBD介质阻挡放电的Comsol等离子体模块仿真研究:沿面放电特性分析
- 三相短路计算与潮流分析:基于MATLAB的程序实现,三相短路计算与潮流分析:基于MATLAB的程序设计与实现,三相短路计算,潮流计算,MATLAB程序 ,三相短路计算; 潮流计算; MATLAB程序
- 双向Buck-Boost变换器与DC-DC变换器的电压外环电流内环控制及其三种工作模式解析:恒功率、恒电流与稳压模式应用,双向Buck-Boost变换器与DC-DC变换器的电压外环电流内环控制模式及其
- DSP28335驱动下的三相逆变器电路设计与高效代码实现,DSP28335驱动的三相逆变器电路设计与优化代码实践,dsp28335三相逆变器电路加代码 ,dsp28335; 三相逆变器电路; 代码,D
- 各省市区明清进士人数数据
- 基于Maxwell软件的450W功率、内置式永磁同步电机技术参数详解:12槽10极切向设计,定子直径40mm,额定电流与转速详述,CAD绘制转子设计文件 ,基于Maxwell软件的12槽10极切向内置
- office 2016 自定义安装的工具
- 利用激光雷达观测验证ERA5、NORA3和NEWA的大风廓线-Tall wind speed profiles with Doppler wind lidars
- Delphi 12.3控件之TMS FlexCel Studio for VCL 3.21.exe
- Delphi 7 创建Access 数据库源代码
- 蚁群算法融合动态窗口法的路径规划算法研究:多动态障碍物的应用与挑战,蚁群算法结合动态窗口路径规划,多动态障碍环境下策略优化,蚁群算法融合动态窗口法路径规划算法 多动态障碍物 ,蚁群算法;动态窗口法;路
- 《95015网络安全应急响应分析报告(2024)》
- 基于STM32单片机的多级PWM调速直流电机控制系统设计:集成L298N驱动器、霍尔测速模块、液晶显示及多模式控制功能,基于L298N驱动与霍尔测速的STM32单片机直流电机PWM调速控制系统设计:按
- 基于Comsol的精确人体皮肤温度场计算模型:揭示正常代谢下皮肤温度分布规律,基于Comsol的人体皮肤温度场计算模型:探究正常代谢下皮肤温度分布,comsol 人体皮肤温度场计算模型,可以得到人体皮
- 2024人才薪资报告行业报告2024人才薪资报告
- 直流微电网多储能单元均衡控制策略:改进下垂控制实现不同容量蓄电池协调,快速均衡SOC并维持母线电压稳定,直流微电网多储能单元均衡控制策略:改进下垂控制实现不同蓄电池协调与SOC均衡,增加母线电压补偿环
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


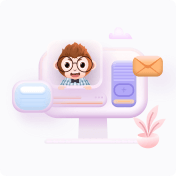