package com.example.wxp.controller;
//hotel
import com.example.wxp.entity.Hotel;
import com.example.wxp.entity.Room;
import com.example.wxp.service.HotelService;
import com.example.wxp.service.RoomService;
import com.example.wxp.util.PageUtil;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletRequest;
import java.io.File;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.*;
@Controller
@RequestMapping("room")
public class RoomController {
@Autowired
private RoomService roomService;
@Autowired
private HotelService hotelService;
//查询全部房间信息
@RequestMapping("selectRoomList")
public String selectRoomList(Model model, String currentPage, String hid){
int currentpage = 0;
if (hid == null || hid.trim().equals("")) {
if (currentPage == null || currentPage.trim().equals("")) {
currentpage = 1;
} else {
currentpage = Integer.parseInt(currentPage);
}
Map<String, Object> map = new HashMap<String, Object>();
int count = roomService.getPageCount();
int pageSize = 2;
PageUtil<Room> param = new PageUtil<Room>();
param.setTotalCount(count);
param.setPageSize(pageSize);
param.setCurrentPage(currentpage);
int startRow = param.getStartRow();
map.put("startRow", startRow);
map.put("pageSize", pageSize);
List<Room> roomList = roomService.selectRoomByPage(startRow, pageSize);
param.setList(roomList);
model.addAttribute("roomList", roomList);
model.addAttribute("util", param);
model.addAttribute("currentPage", currentpage);
List<Hotel> hotelList = hotelService.selectHotel();
model.addAttribute("hotelList", hotelList);
return "empList";
}else{
int hotelId = Integer.parseInt(hid);
if (currentPage == null || currentPage.trim().equals("")) {
currentpage = 1;
} else {
currentpage = Integer.parseInt(currentPage);
}
Map<String, Object> map = new HashMap<String, Object>();
int count = roomService.selectcountById(hotelId);
int pageSize = 2;
PageUtil<Room> param = new PageUtil<Room>();
param.setTotalCount(count);
param.setPageSize(pageSize);
param.setCurrentPage(currentpage);
int startRow = param.getStartRow();
int totalPage = param.getTotalPage();
map.put("startRow", startRow);
map.put("pageSize", pageSize);
map.put("hotelId", hotelId);
List<Room> roomList = roomService.selectRoomByHId(map);
param.setList(roomList);
model.addAttribute("roomList", roomList);
model.addAttribute("util", param);
model.addAttribute("currentPage", currentpage);
List<Hotel> hotelList = hotelService.selectHotel();
model.addAttribute("hotelList", hotelList);
model.addAttribute("hid", hid);
return "empList";
}
}
//查询全部房间信息
@RequestMapping("addRoom")
public String addRoom(Room room,HttpServletRequest request, MultipartFile uploadFile) throws IOException {
if(uploadFile.getSize()!=0) {
String newFileName = uploadFile(request, uploadFile);
room.setRoomPicture(newFileName);
}
System.out.println(room.toString());
roomService.addRoom(room);
return "redirect:/room/selectRoomList";
}
//上传文件
public String uploadFile(HttpServletRequest request, MultipartFile uploadFile) throws IOException {
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMddHHmmssSS");
String res = sdf.format(new Date());
//uploads文件夹位置
String rootPath =request.getServletContext().getRealPath("/hotelPictures");
//原始名称
String originalFilename = uploadFile.getOriginalFilename();
//新的文件名称
String newFileName = Math.random()+res+originalFilename.substring(originalFilename.lastIndexOf("."));
//创建年月文件夹
Calendar date = Calendar.getInstance();
File dateDirs = new File(date.get(Calendar.YEAR)
+ File.separator + (date.get(Calendar.MONTH)+1));
//新文件
File newFile = new File(rootPath+File.separator+dateDirs+File.separator+res);
//判断目标文件所在的目录是否存在
if(!newFile.getParentFile().exists()) {
//如果目标文件所在的目录不存在,则创建父目录
newFile.getParentFile().mkdirs();
}
//将内存中的数据写入磁盘
uploadFile.transferTo(newFile);
//完整的url
//String fileUrl = "/resource/uploads/"+date.get(Calendar.YEAR)+ "/"+(date.get(Calendar.MONTH)+1)+ "/"+ newFileName;
String fileUrl = date.get(Calendar.YEAR)+"/"+(date.get(Calendar.MONTH)+1)+ "/"+res;
return fileUrl;
}
//删除房间信息
@RequestMapping("delRoomById")
public String delRoomById(int roomId){
roomService.delRoomById(roomId);
return "redirect:/room/selectRoomList";
}
//跳转房源修改页面
@RequestMapping("toEditRoomById")
public String toEditRoomById(int roomId,Model model){
Room room = roomService.selectRoomByRId(roomId);
model.addAttribute("room", room);
List<Hotel> hotelList = hotelService.selectHotel();
model.addAttribute("hotelList", hotelList);
return "/backstage/updateRoominfo";
}
//房源修改
@RequestMapping("editRoom")
public String editRoom(Room room1,HttpServletRequest request, MultipartFile uploadFile) throws IOException {
if(uploadFile.getSize()!=0) {
String newFileName = uploadFile(request, uploadFile);
room1.setRoomPicture(newFileName);
}
System.out.println(room1.toString());
roomService.editRoom(room1);
return "redirect:/room/selectRoomList";
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
springboot1.zip
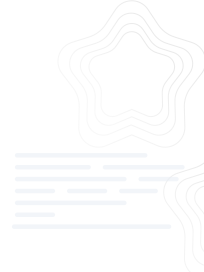
共871个文件
js:126个
gif:123个
xml:117个

需积分: 5 0 下载量 35 浏览量
2021-09-12
17:50:17
上传
评论
收藏 61.97MB ZIP 举报
温馨提示
简单酒店管理系统
资源推荐
资源详情
资源评论
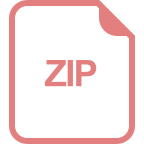
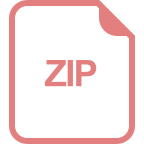
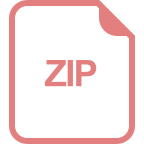
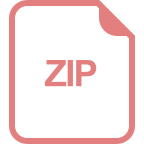
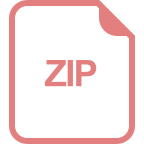
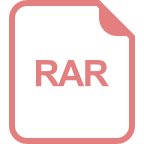
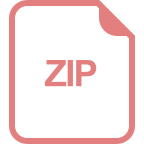
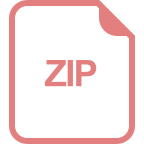
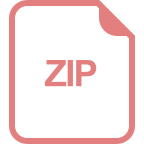
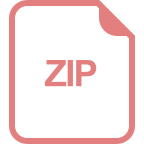
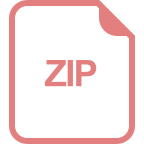
收起资源包目录

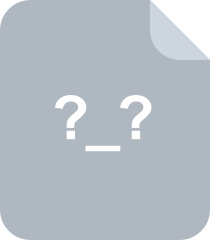
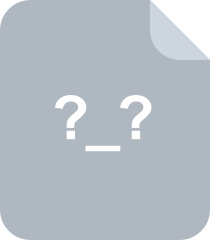
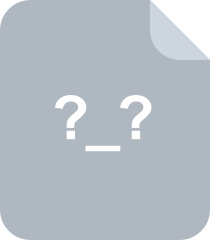
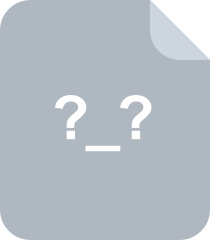
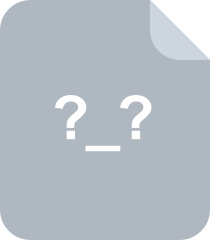
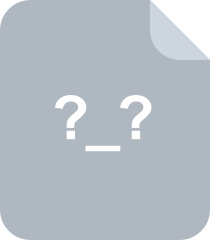
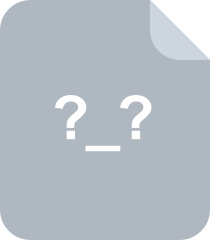
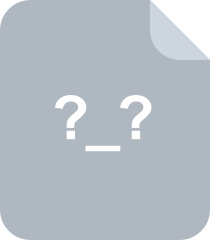
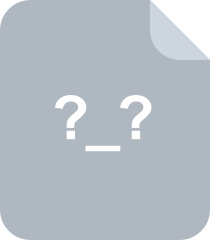
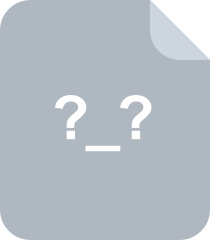
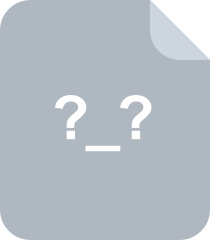
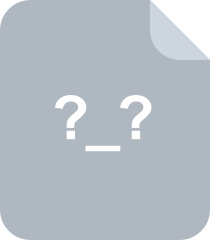
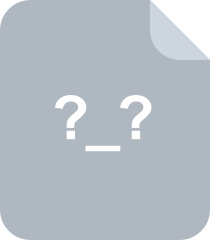
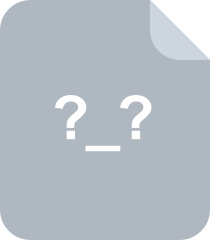
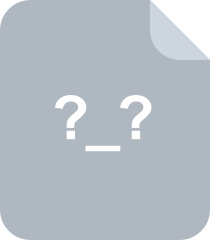
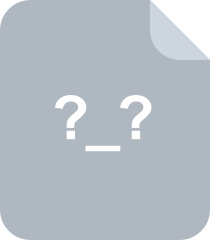
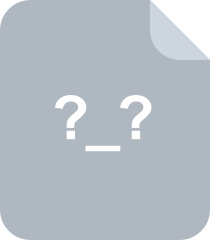
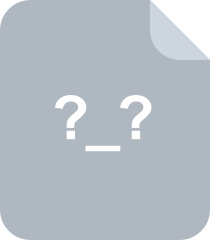
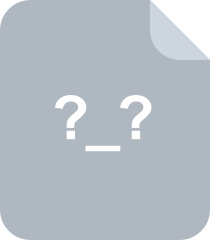
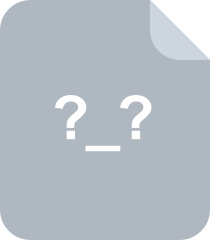
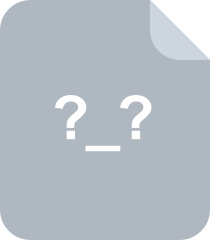
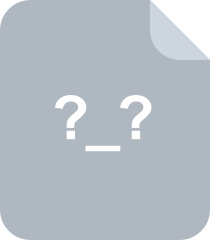
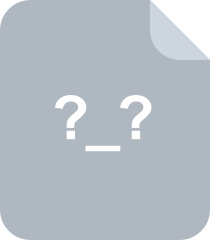
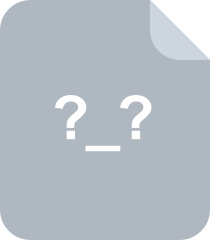
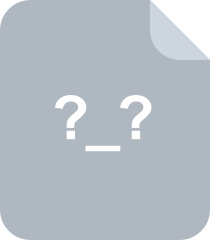
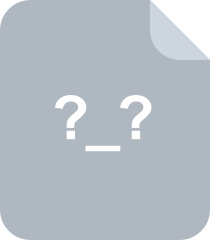
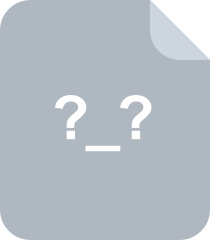
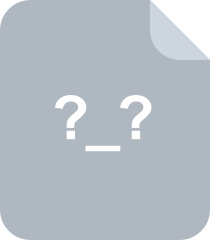
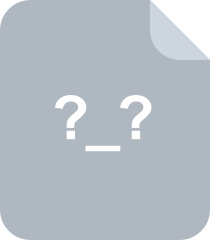
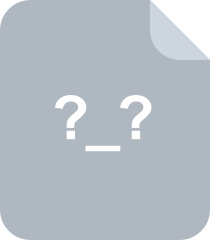
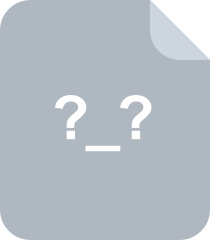
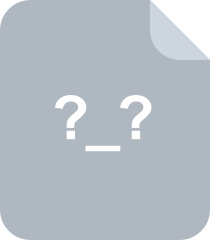
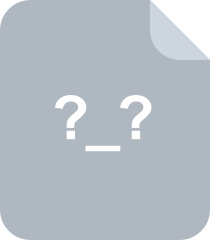
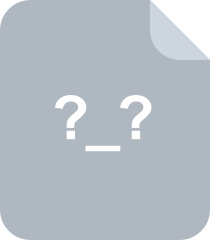
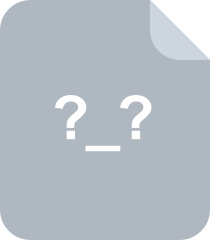
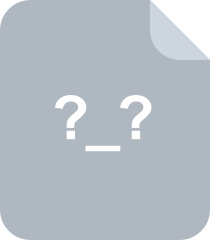
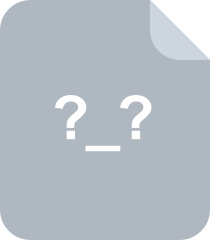
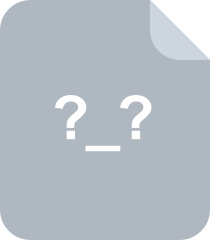
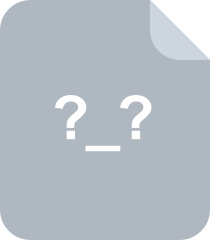
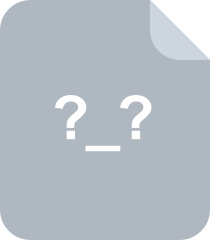
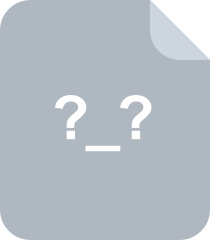
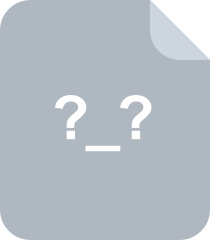
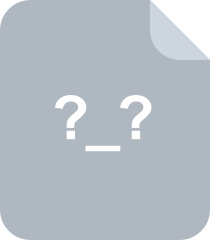
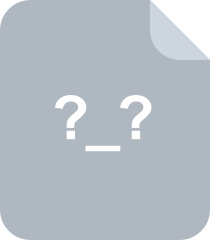
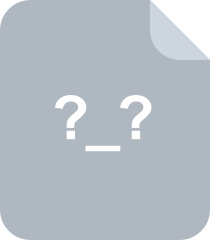
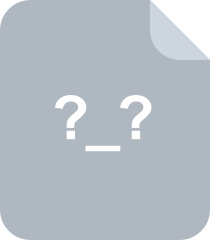
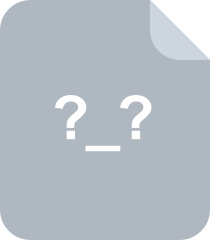
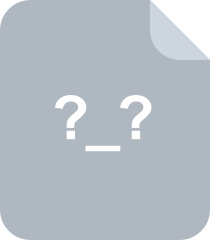
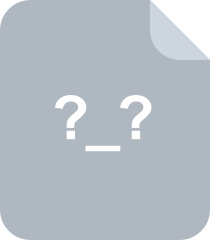
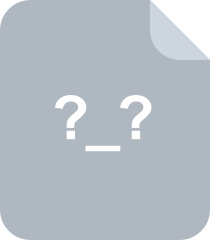
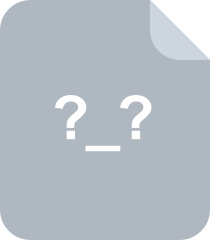
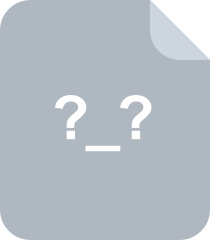
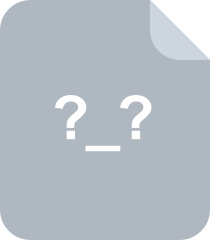
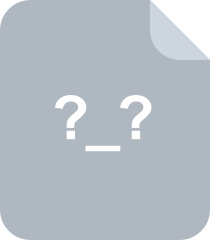
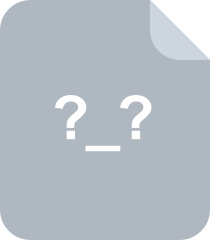
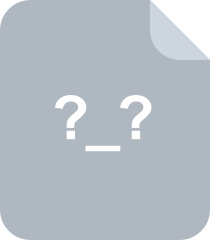
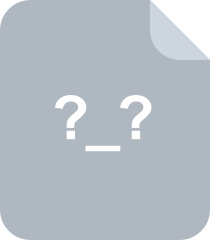
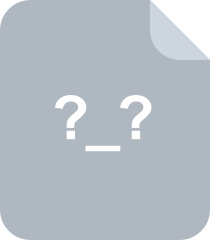
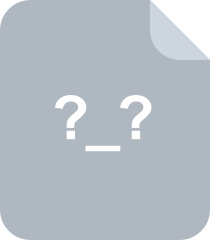
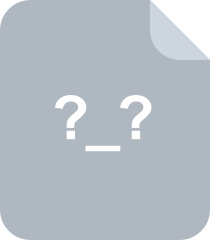
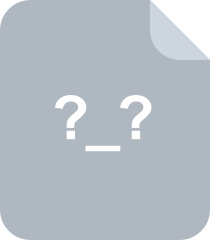
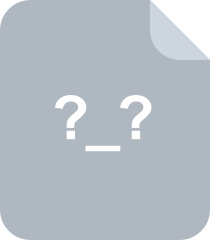
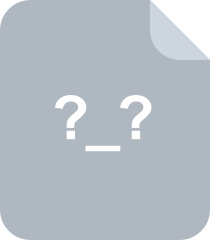
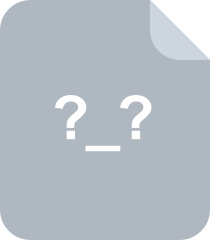
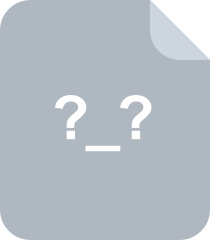
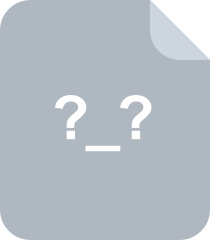
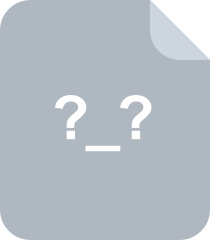
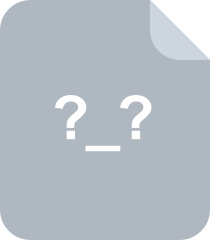
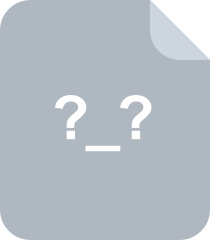
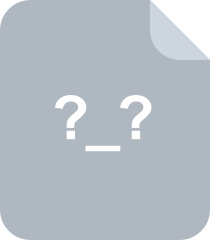
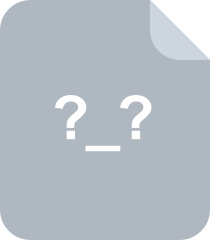
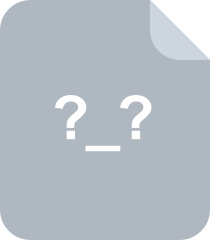
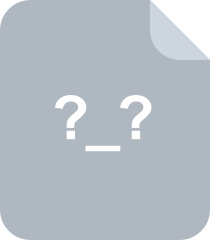
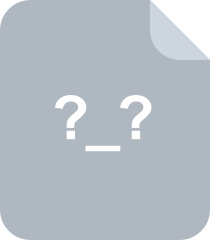
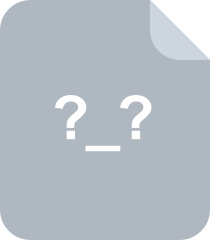
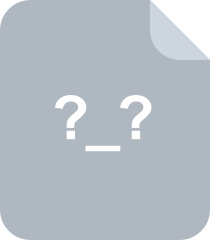
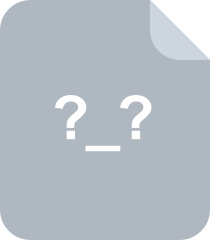
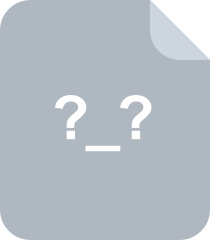
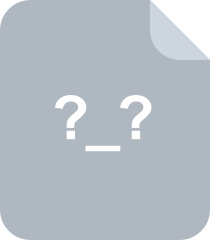
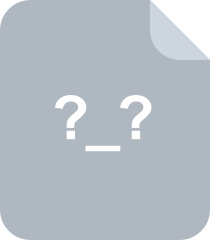
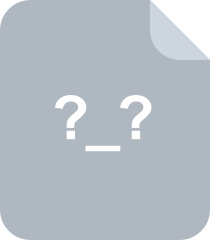
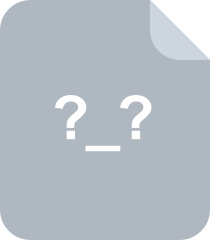
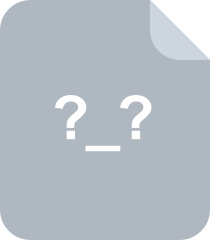
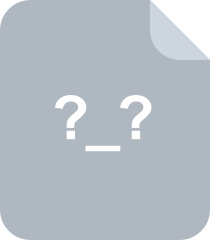
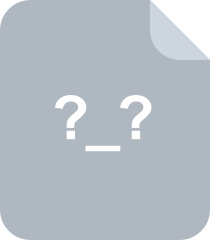
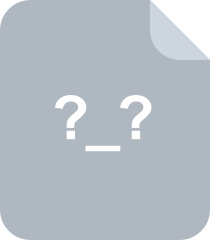
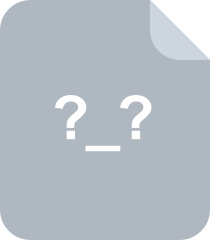
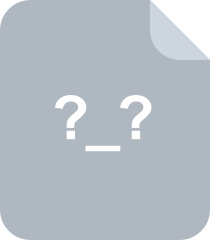
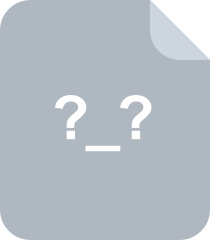
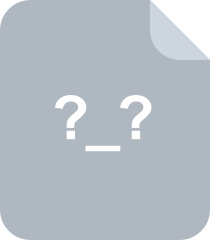
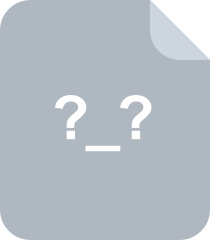
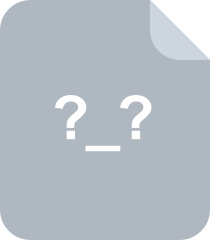
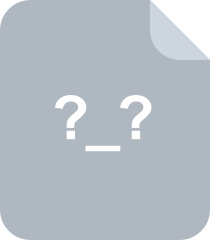
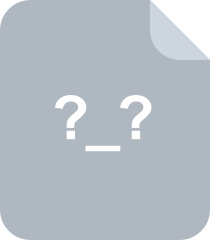
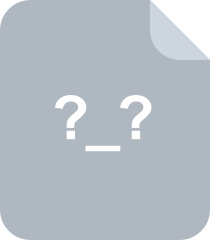
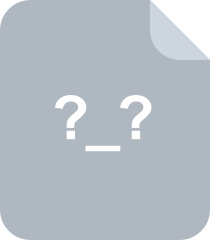
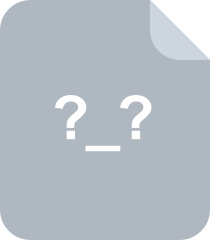
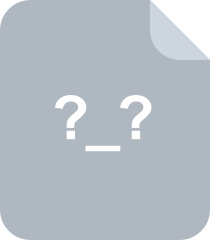
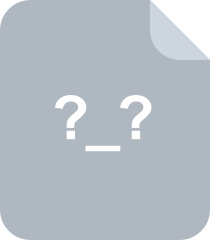
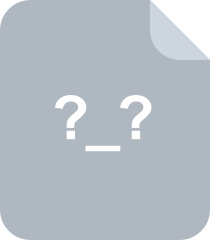
共 871 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
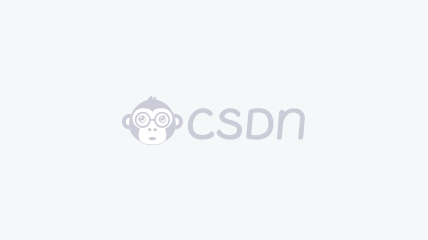

菜鸟小世界
- 粉丝: 1093
- 资源: 16
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

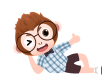
最新资源
- Python基础学习-12匿名函数lambda和map、filter
- MATLAB实现SSA-BP麻雀搜索算法优化BP神经网络多输入单输出回归预测(多指标,多图)(含完整的程序和代码详解)
- AMI aptio 5.x BIOS状态码(POST CODE)及开机Beep声含义表(Checkpoints & Beep Codes for Debugging R2.0)
- MATLAB实现POA-CNN-LSTM鹈鹕算法优化卷积长短期记忆神经网络多输入单输出回归预测(含完整的程序和代码详解)
- Matlab实现基于RF随机森林的电力负荷预测模型(含完整的程序和代码详解)
- Matlab实现基于GRNN广义回归神经网络的电力负荷预测模型(含完整的程序和代码详解)
- mmexport1732757977880.mp4
- MATLAB实现WOA-RBF鲸鱼优化算法优化径向基函数神经网络多输入单输出回归预测(多指标,多图)(含完整的程序和代码详解)
- MATLAB实现K折交叉验证GRNN广义回归神经网络多输入单输出回归预测(含完整的程序和代码详解)
- MATLAB实现基于RF随机森林的时间序列预测-递归预测未来(多指标评价)(含完整的程序和代码详解)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


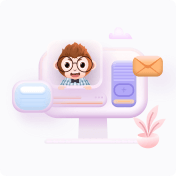
安全验证
文档复制为VIP权益,开通VIP直接复制
