# Torch Vectorized
> Batched and vectorized operations on volume of 3x3 symmetric matrices with Pytorch. The current Pytorch's implementation of batch eigen-decomposition is very slow when dealing with huge number of small matrices (e.g. 500k x 3x3). **This library offers some basic functions like vSymEig, vExpm and vLogm for fast computation (>250x faster) of huge number of small matrices with Pytorch using an analytical solution.**
#### Read the documentaton [HERE](https://torch-vectorized.readthedocs.io/en/latest/)
## vSymEig
> A quick closed-form solution for volumetric 3x3 matrices Eigen-Decomposition with Pytorch. Solves Eigen-Decomposition of data with shape Bx9xDxHxW, where B is the batch size, 9 is the flattened 3x3 symmetric matrices, D is the depth, H is the Height, W is the width. The goal is to accelerate the Eigen-Decomposition of multiple (>500k) small matrices (3x3) on GPU with Pytorch using an analytical solution.
<img src="https://raw.githubusercontent.com/banctilrobitaille/torch-vectorized/master/icons/vsymeig.png" width="100%" vertical-align="bottom">
## vExpm
> Based on vSymEig, computes the matrix exponential for batch of volumetric 3x3 matrices.
<img src="https://raw.githubusercontent.com/banctilrobitaille/torch-vectorized/master/icons/vexpm.png" width="100%" vertical-align="bottom">
## vLogm
> Based on vSymEig, computes the matrix logarithm for batch of volumetric 3x3 matrices.
<img src="https://raw.githubusercontent.com/banctilrobitaille/torch-vectorized/master/icons/vlogm.png" width="100%" vertical-align="bottom">
## Install me
> pip install torch-vectorized
## How to use
```python
from torchvectorized.vlinalg import vSymeig
# Random batch of volumetric 3x3 symmetric matrices of size 16x9x32x32x32
input = sym(torch.rand(16, 9, 32, 32, 32))
# Output eig_vals with size: 16x3x32x32x32 and eig_vecs with size 16,3,3,32,32,32
eig_vals, eig_vecs = vSymeig(input, eigen_vectors=True)
def sym(self, inputs):
# Ensure symmetry of randomly generated 3x3 matrix using (X + X.T) / 2.0
return (inputs + inputs[:, [0, 3, 6, 1, 4, 7, 2, 5, 8], :, :, :]) / 2.0
```
## Contributing
#### How to contribute ?
- [X] Create a branch by feature and/or bug fix
- [X] Get the code
- [X] Commit and push
- [X] Create a pull request
#### Branch naming
##### Feature branch
> feature/ [Short feature description] [Issue number]
##### Bug branch
> fix/ [Short fix description] [Issue number]
#### Commits syntax:
##### Adding code:
> \+ Added [Short Description] [Issue Number]
##### Deleting code:
> \- Deleted [Short Description] [Issue Number]
##### Modifying code:
> \* Changed [Short Description] [Issue Number]
##### Merging code:
> Y Merged [Short Description] [Issue Number]
Icons made by <a href="http://www.flaticon.com/authors/freepik" title="Freepik">Freepik</a> from <a href="http://www.flaticon.com" title="Flaticon">www.flaticon.com</a> is licensed by <a href="http://creativecommons.org/licenses/by/3.0/" title="Creative Commons BY 3.0" target="_blank">CC 3.0 BY</a>

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
最新资源
- 使用Python和Pygame实现圣诞节动画效果
- 数据分析-49-客户细分-K-Means聚类分析
- 企业可持续发展性数据集,ESG数据集,公司可持续发展性数据(可用于多种企业可持续性研究场景)
- chapter9.zip
- 使用Python和Pygame库创建新年烟花动画效果
- 国际象棋检测10-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- turbovnc-2.2.6.x86-64.rpm
- 艾利和iriver Astell&Kern SP3000 V1.30升级固件
- VirtualGL-2.6.5.x86-64.rpm
- dbeaver-ce-24.3.1-x86-64-setup.exe
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


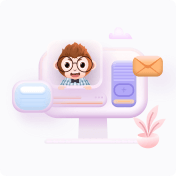