<img alt="Starlite logo" src="./docs/images/SVG/starlite-banner.svg" width="100%" height="auto">
<div align="center">


[](https://sonarcloud.io/summary/new_code?id=Goldziher_starlite)
[](https://sonarcloud.io/summary/new_code?id=Goldziher_starlite)
[](https://sonarcloud.io/summary/new_code?id=Goldziher_starlite)
[](https://sonarcloud.io/summary/new_code?id=Goldziher_starlite)
[](https://sonarcloud.io/summary/new_code?id=Goldziher_starlite)
[](https://sonarcloud.io/summary/new_code?id=Goldziher_starlite)
[](https://discord.gg/X3FJqy8d2j)
[](https://itnext.io/introducing-starlite-3928adaa19ae)
</div>
# Starlite
Starlite is a light and flexible ASGI API framework. Using [Starlette](https://github.com/encode/starlette)
and [pydantic](https://github.com/samuelcolvin/pydantic) as foundations.
Check out the [Starlite documentation ð](https://starlite-api.github.io/starlite/)
## Core Features
- ð Class based controllers
- ð Decorators based configuration
- ð Extended testing support
- ð Extensive typing support including inference, validation and parsing
- ð Full async (ASGI) support
- ð Layered dependency injection
- ð OpenAPI 3.1 schema generation with [Redoc](https://github.com/Redocly/redoc) UI
- ð Route guards based authorization
- ð Simple middleware and authentication
- ð Support for pydantic models and pydantic dataclasses
- ð Support for standard library dataclasses
- ð Support for SQLAlchemy declarative classes
- ð Plugin system to allow extending supported classes
- ð Ultra-fast json serialization and deserialization using [orjson](https://github.com/ijl/orjson)
## Installation
```shell
pip install starlite
```
## Relation to Starlette and FastAPI
Although Starlite uses the Starlette ASGI toolkit, it does not simply extend Starlette, as FastAPI does. Starlite uses
selective pieces of Starlette while implementing its own routing and parsing logic, the primary reason for this is to
enforce a set of best practices and discourage misuse. This is done to promote simplicity and scalability - Starlite is
simple to use, easy to learn, and unlike both Starlette and FastAPI - it keeps complexity low when scaling.
Additionally, Starlite is [faster than both FastAPI and Starlette](https://github.com/Goldziher/api-performance-tests):

Legend:
- a-: async, s-: sync
- np: no params, pp: path param, qp: query param, mp: mixed params
### Class Based Controllers
While supporting function based route handlers, Starlite also supports and promotes python OOP using class based
controllers:
```python title="my_app/controllers/user.py"
from typing import List, Optional
from pydantic import UUID4
from starlite import Controller, Partial, get, post, put, patch, delete
from datetime import datetime
from my_app.models import User
class UserController(Controller):
path = "/users"
@post()
async def create_user(self, data: User) -> User:
...
@get()
async def list_users(self) -> List[User]:
...
@get(path="/{date:int}")
async def list_new_users(self, date: datetime) -> List[User]:
...
@patch(path="/{user_id:uuid}")
async def partially_update_user(self, user_id: UUID4, data: Partial[User]) -> User:
...
@put(path="/{user_id:uuid}")
async def update_user(self, user_id: UUID4, data: User) -> User:
...
@get(path="/{user_name:str}")
async def get_user_by_name(self, user_name: str) -> Optional[User]:
...
@get(path="/{user_id:uuid}")
async def get_user(self, user_id: UUID4) -> User:
...
@delete(path="/{user_id:uuid}")
async def delete_user(self, user_id: UUID4) -> User:
...
```
### Data Parsing, Type Hints and Pydantic
One key difference between Starlite and Starlette/FastAPI is in parsing of form data and query parameters- Starlite
supports mixed form data and has faster and better query parameter parsing.
Starlite is rigorously typed, and it enforces typing. For example, if you forget to type a return value for a route
handler, an exception will be raised. The reason for this is that Starlite uses typing data to generate OpenAPI specs,
as well as to validate and parse data. Thus typing is absolutely essential to the framework.
Furthermore, Starlite allows extending its support using plugins.
### SQL Alchemy Support, Plugin System and DTOs
Starlite has a plugin system that allows the user to extend serialization/deserialization, OpenAPI generation and other
features. It ships with a builtin plugin for SQL Alchemy, which allows the user to use SQL Alchemy declarative classes
"natively", i.e. as type parameters that will be serialized/deserialized and to return them as values from route
handlers.
Starlite also supports the programmatic creation of DTOs with a `DTOFactory` class, which also supports the use of plugins.
### OpenAPI
Starlite has custom logic to generate OpenAPI 3.1.0 schema, the latest version. The schema generated by Starlite is
significantly more complete and more correct than those generated by FastAPI, and they include optional generation of
examples using the `pydantic-factories` library.
### Dependency Injection
Starlite has a simple but powerful DI system inspired by pytest. You can define named dependencies - sync or async - at
different levels of the application, and then selective use or overwrite them.
### Middleware
Starlite supports the Starlette Middleware system while simplifying it and offering builtin configuration of CORS and
some other middlewares.
### Route Guards
Starlite has an authorization mechanism called `guards`, which allows the user to define guard functions at different
level of the application (app, router, controller etc.) and validate the request before hitting the route handler
function.
### Request Life Cycle Hooks
Starlite supports request life cycle hooks, similarly to Flask - i.e. `before_request` and `after_request`
## Contributing
Starlite is open to contributions big and small. You can always [join our discord](https://discord.gg/X3FJqy8d2j) server
to discuss contributions and project maintenance. For guidelines on how to contribute, please
see [the contribution guide](CONTRIBUTING.md).
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源分类:Python库 所属语言:Python 资源全名:starlite-1.0.1.tar.gz 资源来源:官方 安装方法:https://lanzao.blog.csdn.net/article/details/101784059
资源推荐
资源详情
资源评论
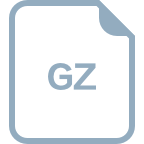
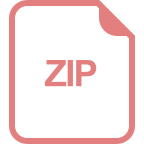
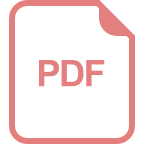
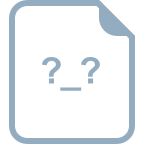
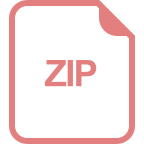
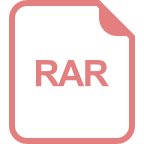
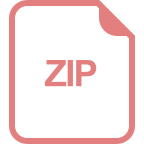
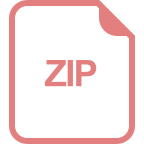
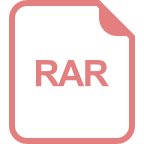
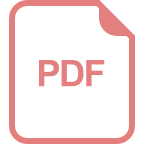
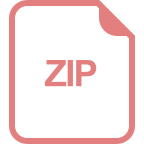
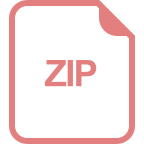
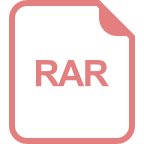
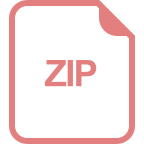
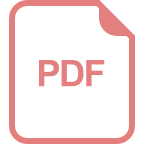
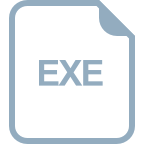
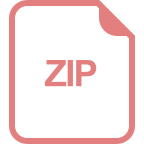
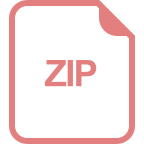
收起资源包目录


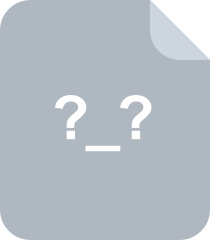

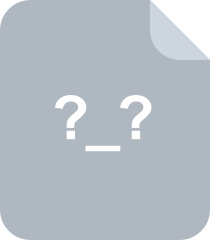

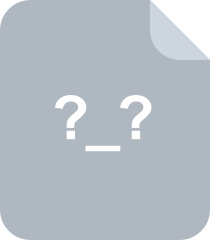
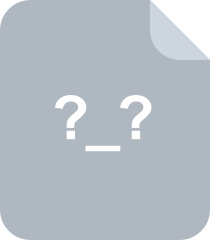
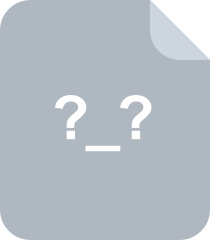
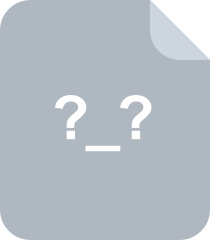
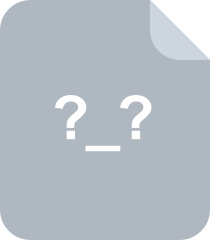
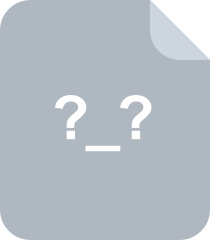
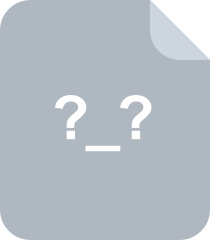
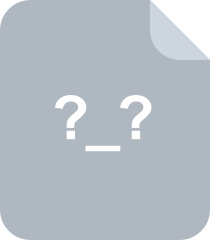
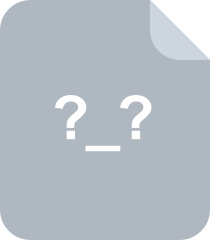
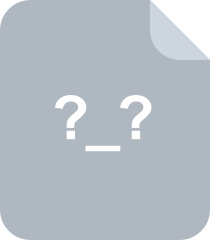
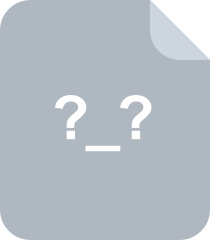
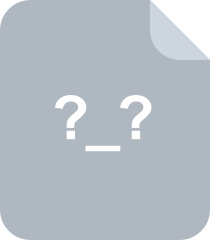
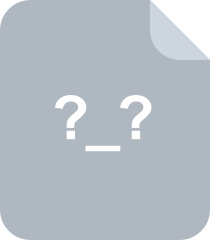

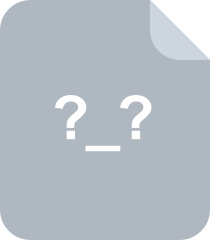
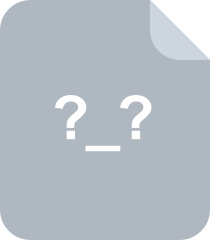
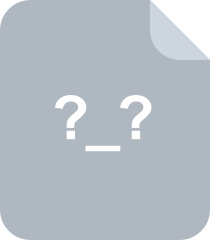
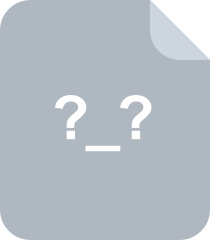
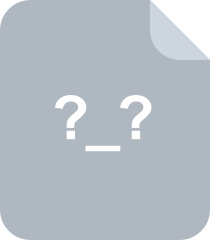
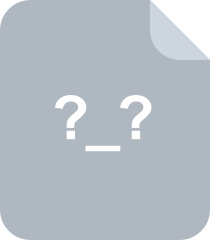
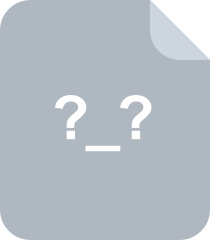
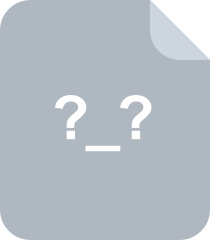
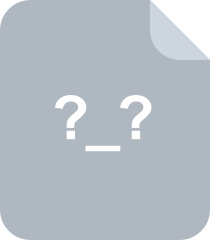

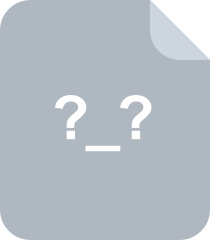
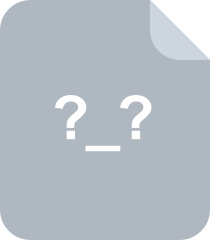
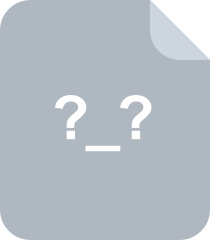
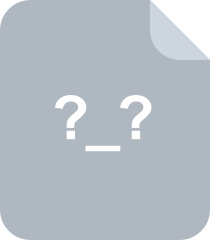
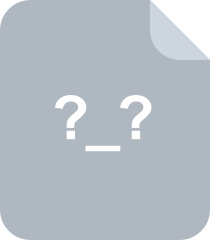
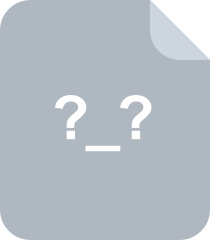
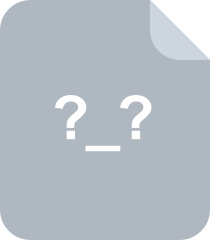
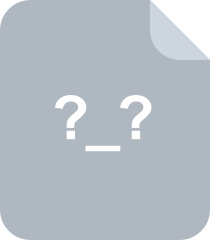
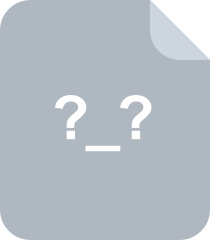
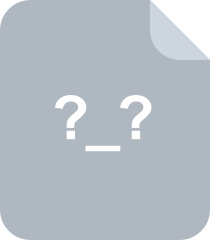
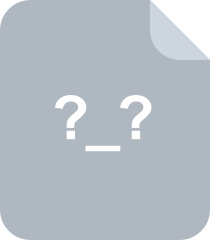
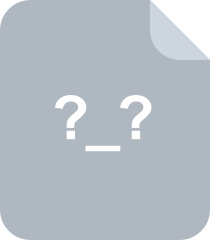
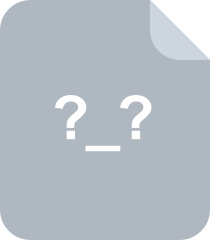
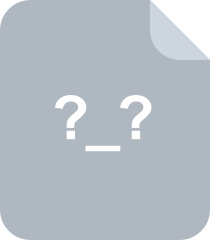
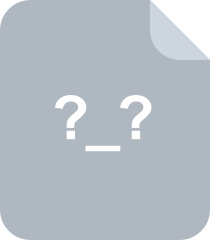
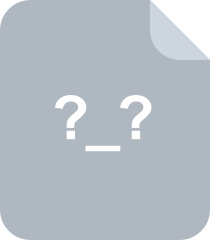
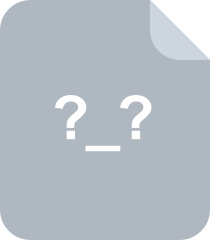

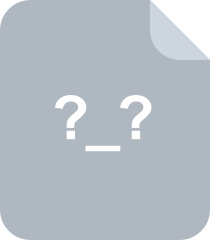
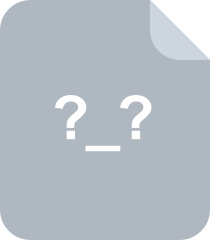
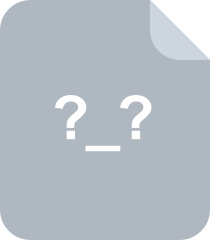
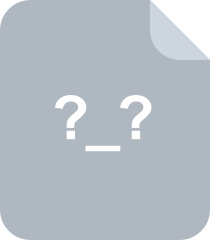

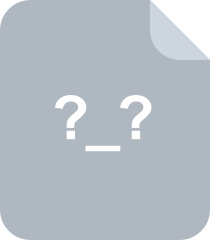
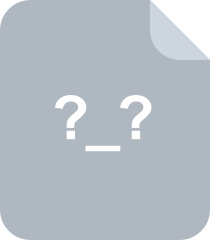
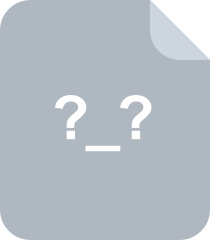
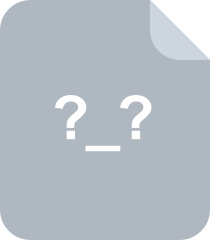
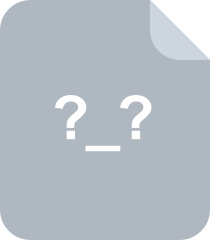
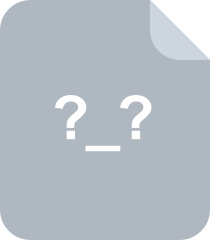
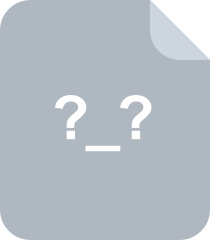
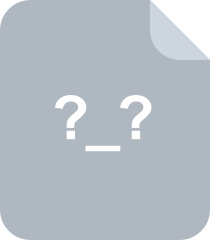
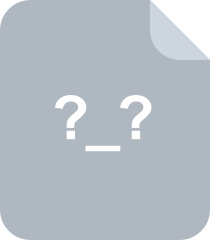
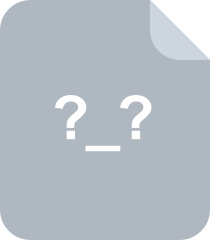
共 55 条
- 1
资源评论
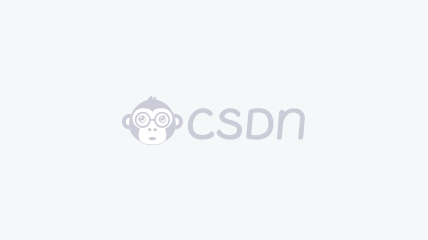

挣扎的蓝藻
- 粉丝: 13w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

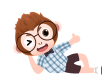
最新资源
- BmapExtras-国庆主题资源
- WanAndroid-master-国庆主题资源
- Qt5双缓冲机制实例程序源码
- java-leetcode题解之17-Letter-Combinations-of-a-Phone-Number
- java-leetcode题解之015-3Sum
- java-leetcode题解之014-Longest-Common-Prefix
- java-leetcode题解之013-Roman-to-Integer
- java-leetcode题解之012-Integer-to-Roman
- java-leetcode题解之011-Container-With-Most-Water
- java-leetcode题解之009-Palindrome-Number
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


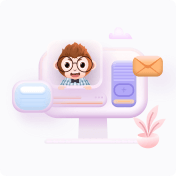
安全验证
文档复制为VIP权益,开通VIP直接复制
