<!-- PROJECT SHIELDS -->
<!--
*** I'm using markdown "reference style" links for readability.
*** Reference links are enclosed in brackets [ ] instead of parentheses ( ).
*** See the bottom of this document for the declaration of the reference variables
*** for contributors-url, forks-url, etc. This is an optional, concise syntax you may use.
*** https://www.markdownguide.org/basic-syntax/#reference-style-links
-->
[![Contributors][contributors-shield]][contributors-url]
[![Forks][forks-shield]][forks-url]
[![Stargazers][stars-shield]][stars-url]
[![Issues][issues-shield]][issues-url]
[](https://anaconda.org/conda-forge/sanic-security)
[](https://pepy.tech/project/sanic-security)
[](https://github.com/psf/black)
<!-- PROJECT LOGO -->
<br />
<p align="center">
<h3 align="center">Sanic Security</h3>
<p align="center">
An effective, simple, and async security library for Sanic.
</p>
</p>
<!-- TABLE OF CONTENTS -->
## Table of Contents
* [About the Project](#about-the-project)
* [Getting Started](#getting-started)
* [Prerequisites](#prerequisites)
* [Installation](#installation)
* [Usage](#usage)
* [Initial Setup](#initial-setup)
* [Authentication](#authentication)
* [Captcha](#captcha)
* [Two Step Verification](#two-step-verification)
* [Authorization](#authorization)
* [Testing](#testing)
* [Tortoise](#tortoise)
* [Roadmap](#roadmap)
* [Contributing](#contributing)
* [License](#license)
* [Versioning](#Versioning)
<!-- ABOUT THE PROJECT -->
## About The Project
Sanic Security is an authentication, authorization, and verification library designed for use with [Sanic](https://github.com/huge-success/sanic).
This library contains a variety of features including:
* Login, registration, and authentication
* Two-step verification
* Two-factor authentication
* Captcha
* Wildcard and role based authorization
This repository has been starred by Sanic's core maintainer:
[](https://github.com/ahopkins)
Please visit [security.sunsetdeveloper.com](https://security.sunsetdeveloper.com) for more documentation.
<!-- GETTING STARTED -->
## Getting Started
In order to get started, please install pip.
### Prerequisites
* pip
```shell
sudo apt-get install python3-pip
```
### Installation
* Install the Sanic Security pip package.
```shell
pip3 install sanic-security
````
## Usage
Sanic Security setup and implementation is easy.
### Initial Setup
First you have to create a configuration file called security.ini in the working directory. Below is an example of its contents:
```ini
[SECURITY]
secret=05jF8cSMAdjlXcXeS2ZJUHg7Tbyu
captcha_font=captcha.ttf
cache_path=./resources/security-cache
session_samesite=strict
session_secure=true
[TORTOISE]
username=example
password=8UVbijLUGYfUtItAi
endpoint=example.cweAenuBY6b.us-north-1.rds.amazonaws.com
schema=exampleschema
models=sanic_security.models, example.models
engine=mysql
generate=true
```
Once you've configured Sanic Security, you can initialize Sanic with the example below:
```python
initialize_security_orm(app)
if __name__ == "__main__":
app.run(host="0.0.0.0", port=8000, debug=True)
```
The tables in the below examples represent example request `form-data`.
## Authentication
* Registration
Phone can be null or empty.
Key | Value |
--- | --- |
**username** | test
**email** | test@test.com
**phone** | 19811354186
**password** | testpass
**captcha** | Aj8HgD
```python
@app.post("api/auth/register")
@requires_captcha()
async def on_register(request, captcha_session):
account = await register(request)
two_step_session = await request_two_step_verification(request, account)
await email_code(two_step_session.code) #Custom method for emailing verification code.
response = json("Registration successful!", two_step_session.account.json())
two_step_session.encode(response)
return response
```
* Verify Account
Key | Value |
--- | --- |
**code** | G8ha9nVae
```python
@app.post("api/auth/verify")
async def on_verify(request):
two_step_session = await verify_account(request)
return json("You have verified your account and may login!", two_step_session.account.json())
```
* Login
Key | Value |
--- | --- |
**email** | test@test.com
**password** | testpass
```python
@app.post("api/auth/login")
async def on_login(request):
authentication_session = await login(request)
response = json("Login successful!", authentication_session.account.json())
authentication_session.encode(response)
return response
```
* Login (With two-factor authentication)
Key | Value |
--- | --- |
**email** | test@test.com
**password** | testpass
```python
@app.post("api/auth/login")
async def on_two_factor_login(request):
authentication_session = await login(request, two_factor=True)
two_step_session = await request_two_step_verification(request, authentication_session.account)
await email_code(two_step_session.code) #Custom method for emailing verification code.
response = json("Login successful! A second factor is now required to be authenticated.", authentication_session.account.json())
authentication_session.encode(response)
two_step_session.encode(response)
return response
```
* Second Factor
Key | Value |
--- | --- |
**code** | G8ha9nVae
```python
@app.post("api/auth/login/second-factor")
@requires_two_step_verification()
async def on_login_second_factor(request, two_step_verification):
authentication_session = await on_second_factor(request)
response = json("Second factor attempt successful! You may now be authenticated!",
authentication_session.account.json())
return response
```
* Logout
```python
@app.post("api/auth/logout")
@requires_authentication()
async def on_logout(request, authentication_session):
await logout(authentication_session)
response = json("Logout successful!", authentication_session.account.json())
return response
```
* Requires Authentication
```python
@app.post("api/auth")
@requires_authentication()
async def on_authenticated(request, authentication_session):
return json(f"Hello {authentication_session.account.username}! You have been authenticated.",
authentication_session.account.json())
```
## Captcha
You must download a .ttf font for captcha challenges and define the file's path in security.ini.
[1001 Free Fonts](https://www.1001fonts.com/)
[Recommended Font](https://www.1001fonts.com/source-sans-pro-font.html)
Captcha challenge example:
[](https://github.com/sunset-developer/sanic-security/blob/main/images/captcha.png)
* Request Captcha
```python
@app.post("api/captcha/request")
async def on_request_captcha(request):
captcha_session = await request_captcha(request)
response = json("Captcha request successful!", captcha_session.json())
captcha_session.encode(response)
return response
```
* Captcha Image
```python
@app.get("api/captcha/img")
async def on_captcha_img(request):
captcha_session = await CaptchaSession.decode(request)
return await captcha_session.get_image()
```
* Requires Captcha
Key | Value |
--- | --- |
**captcha** | Aj8HgD
```python
@app.post("api/captcha")
@requires_captcha()
async def on_captcha_attempt(request, captcha_session):
return json("Captcha attempt successful!", captcha_session

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
最新资源
- 前端分析-2023071100789
- 基于纯手工HTML与CSS构建的田东家乡介绍网站设计源码
- 基于鸿蒙操作系统的芯片评估板自检系统源码
- manatee电磁噪声振动计算softwareManatee 1.09 电机电磁振动噪声NVH终结者 带教程,带教程,带教程重要的话说3遍 史上最强后处理软件,甩jmag、Maxwell、flux几
- 基于Python的Django框架开发的subaoApi设计源码
- 基于Owin+融云的LayIM3.0 .NET版本设计源码
- 单向光伏并网逆变器 图一单向光伏并网逆变器整体结构图 图二并网电流与电压曲线图 图三mppt控制最大功率追踪图 图四直流母线电压曲线图
- 基于Python Django框架的旅游网站后端设计源码
- 基于Kotlin语言的Gradle统一依赖管理设计源码
- 基于PyQt5框架的ExcelDiffer设计源码
- COMSOL裂缝地层的THM耦合,离散裂缝模型,随机复杂裂缝,适合地热能研究 增强地热系统,热流固耦合的开采过程
- 基于2020.02.06快照的xadmin JavaScript/Python/HTML/CSS/Shell五语言混合设计源码
- 基于Vue框架的计算机协会招新系统前端微服务设计源码
- BUCK多种控制策略对比 图一BUCK主电路图与控制策略方法 图二采用开环控制波形 图三开环调节过程 图四单电压闭环控制波形 图五单电压调节过程
- 永磁同步电机(PMSM)MATLAB仿真 直接转矩控制 转速外环 转矩跟磁链内环控制 转矩脉动去下图 能够明显减小电机转矩脉动
- 基于JavaScript的UscIoV跨链交互区块链平台设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


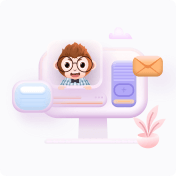