# Regula Document Reader web API Python 3.5+ client
[](https://support.regulaforensics.com/hc/en-us/articles/115000916306-Documentation)
[](https://github.com/regulaforensics/DocumentReader-web-openapi)
[](https://support.regulaforensics.com/hc/en-us/articles/115000916306-Documentation)
[](https://api.regulaforensics.com/)
Documents recognition as easy as reading two bytes.
If you have any problems with or questions about this client, please contact us
through a [GitHub issue](https://github.com/regulaforensics/DocumentReader-web-python-client/issues).
You are invited to contribute [new features, fixes, or updates](https://github.com/regulaforensics/DocumentReader-web-python-client/issues?q=is%3Aissue+is%3Aopen+label%3A%22help+wanted%22), large or small;
We are always thrilled to receive pull requests, and do our best to process them as fast as we can.
See [dev guide](./dev.md)
## Install package
`regula.documentreader.webclient` is on the Python Package Index (PyPI):
```bash
pip install regula.documentreader.webclient
```
Or using `pipenv`
```bash
pipenv install regula.documentreader.webclient
```
## Example
Performing request:
```python
from regula.documentreader.webclient import *
with open("australia_passport.jpg", "rb") as f:
input_image = f.read()
with DocumentReaderApi(host='http://localhost:8080') as api:
params = ProcessParams(
scenario=Scenario.FULL_PROCESS,
result_type_output=[Result.DOCUMENT_IMAGE, Result.STATUS, Result.TEXT, Result.IMAGES]
)
request = RecognitionRequest(process_params=params, images=[input_image])
response = api.process(request)
```
Parsing results:
```python
# status examples
response_status = response.status
doc_overall_status = "valid" if response_status.overall_status == CheckResult.OK else "not valid"
# text fields example
doc_number_field = response.text.get_field(TextFieldType.DOCUMENT_NUMBER)
doc_number_mrz = doc_number_field.get_value()
doc_number_visual = doc_number_field.get_value(Source.VISUAL)
doc_number_visual_validity = doc_number_field.source_validity(Source.VISUAL)
doc_number_mrz_validity = doc_number_field.source_validity(Source.MRZ)
doc_number_mrz_visual_matching = doc_number_field.cross_source_comparison(Source.MRZ, Source.VISUAL)
# images fields example
normalized_input_image = response.images.document_image()
portrait_field = response.images.get_field(GraphicFieldType.PORTRAIT)
portrait_from_visual = portrait_field.get_value(Source.VISUAL)
portrait_from_rfid = portrait_field.get_value(Source.RFID, original=True)
```
You can find more detailed guide and run this sample in [example](./example) folder.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源分类:Python库 所属语言:Python 资源全名:regula.documentreader.webclient-5.7.0.tar.gz 资源来源:官方 安装方法:https://lanzao.blog.csdn.net/article/details/101784059
资源推荐
资源详情
资源评论
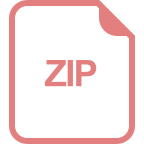
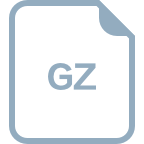
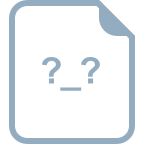
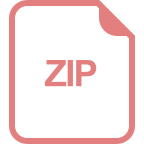
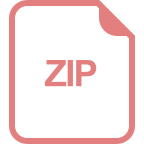
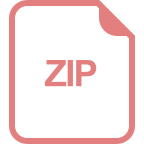
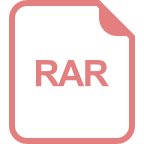
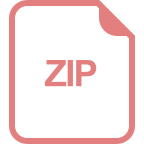
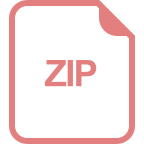
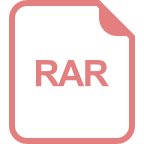
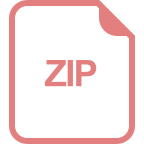
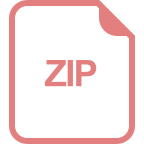
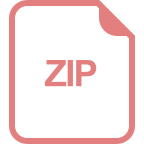
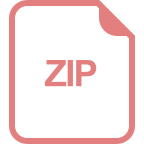
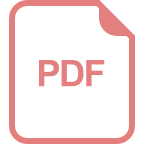
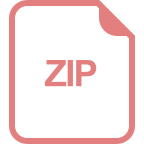
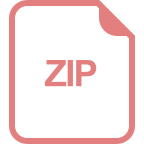
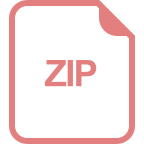
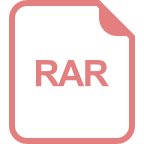
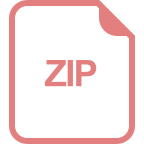
收起资源包目录

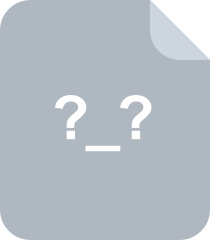
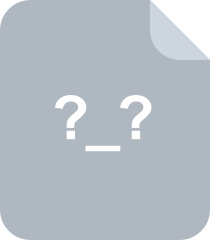
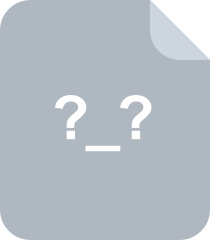
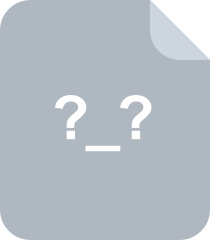
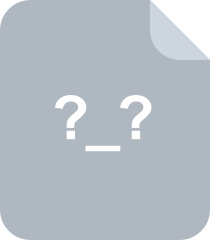
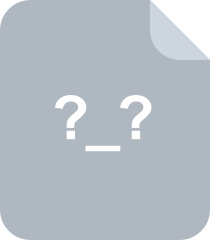
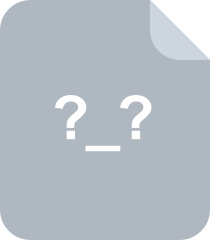
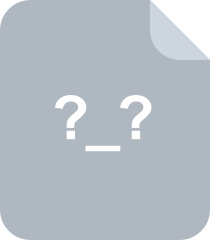
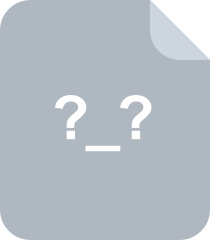
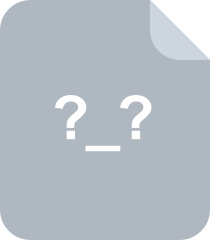
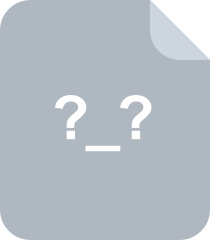
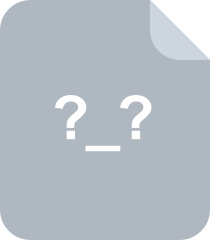
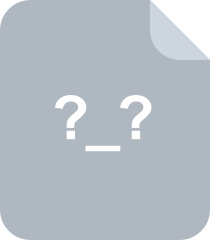
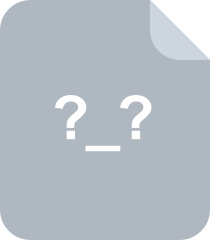
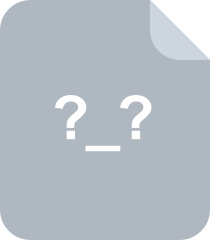
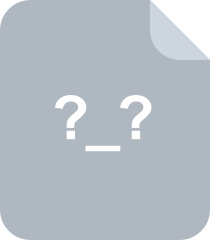
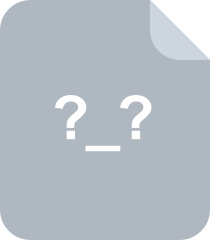
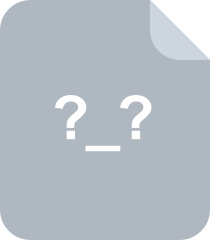
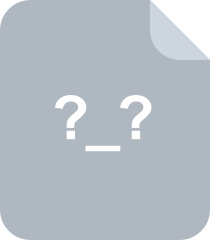
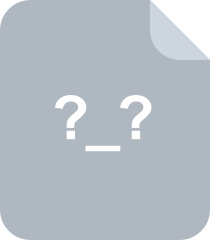
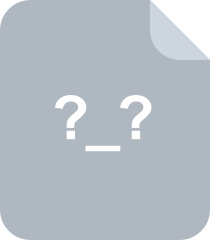
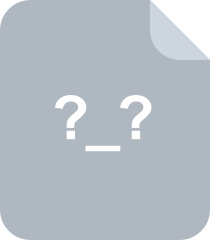
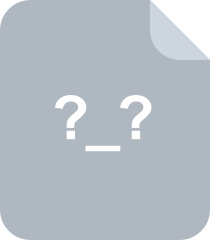
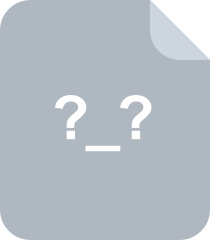
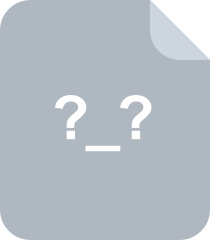
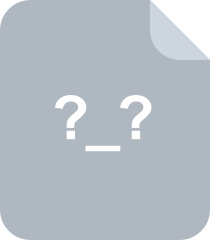
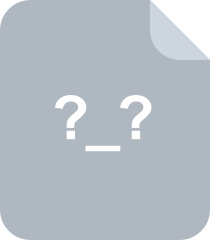
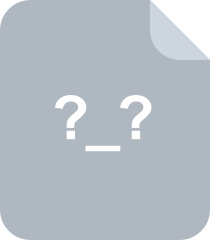
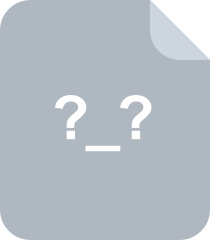
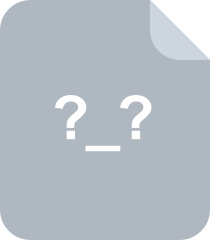
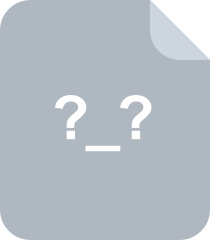
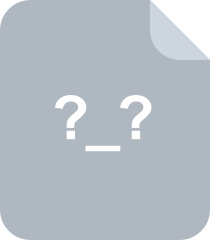
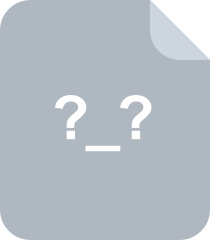
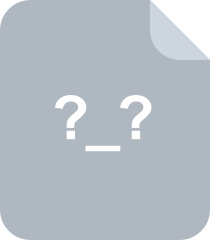
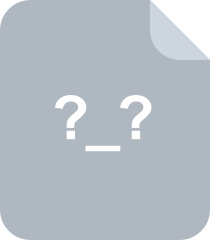
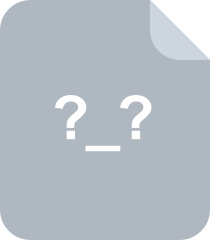
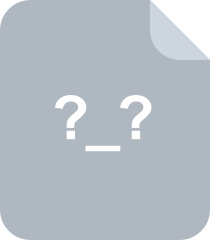
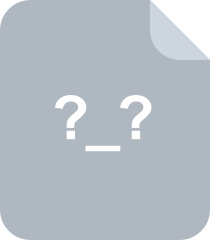
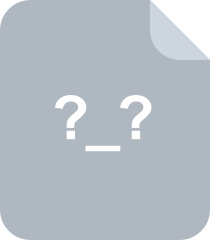
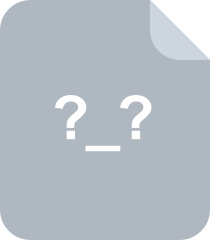
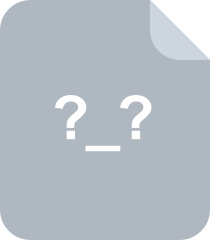
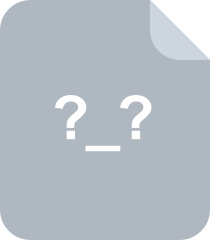
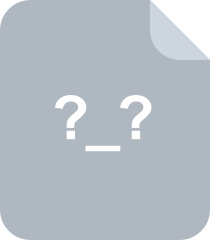
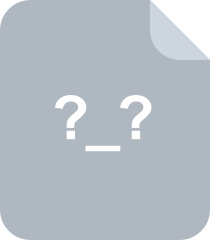
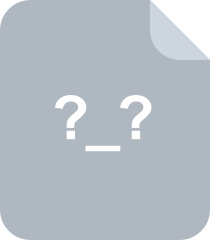
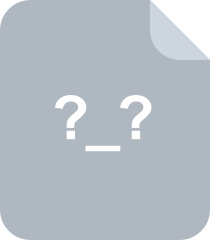
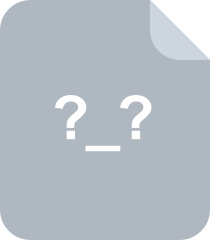
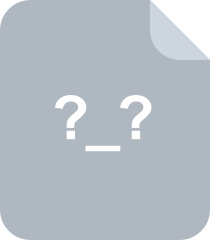
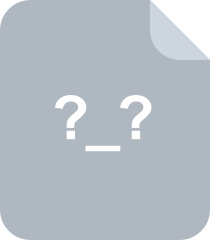
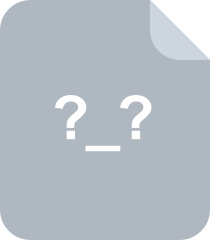
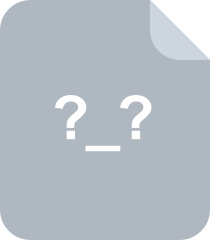
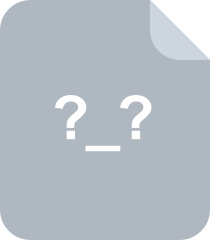
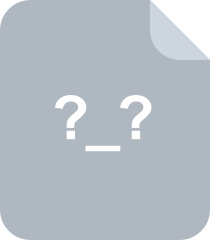
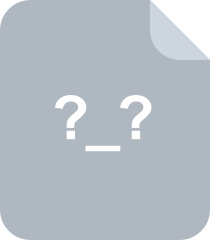
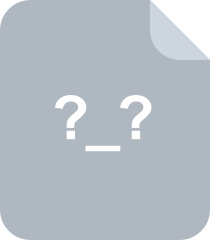
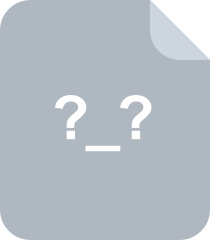
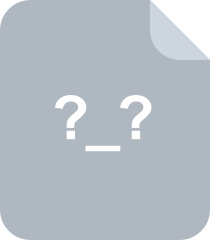
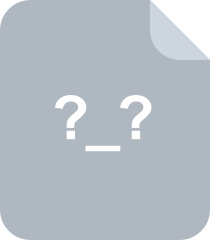
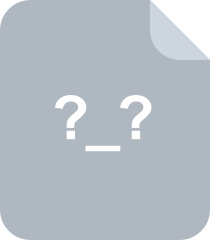
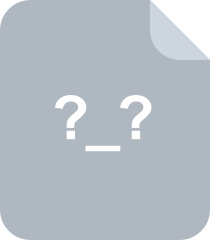
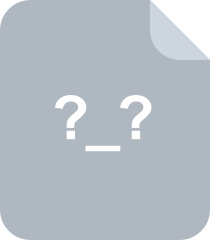
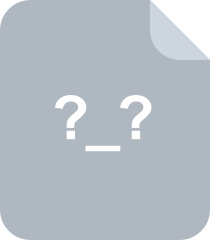
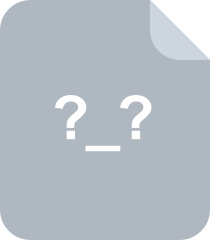
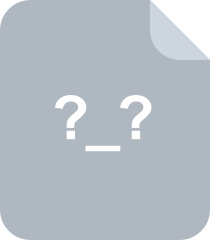
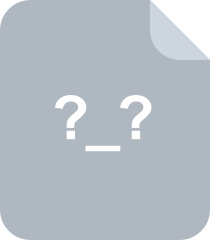
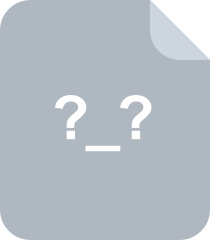
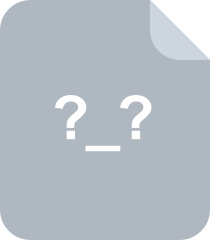
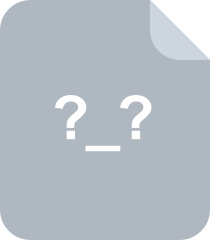
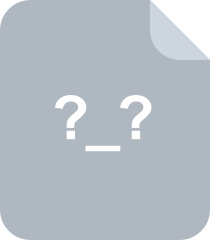
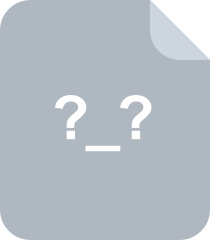
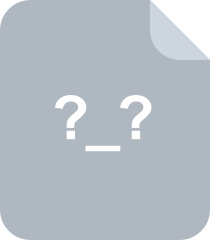
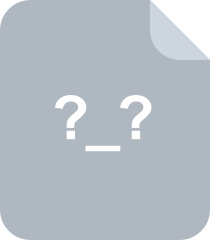
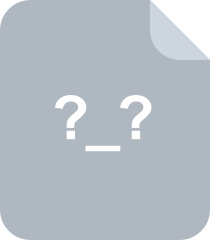
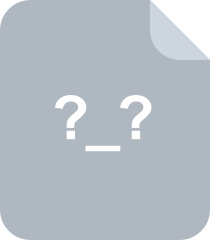
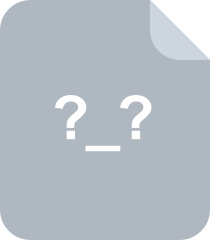
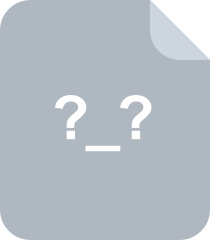
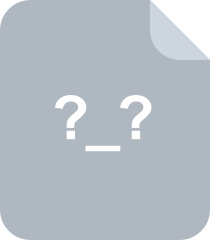
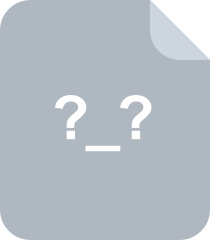
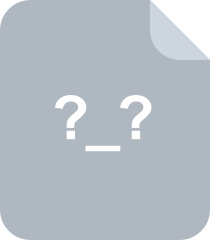
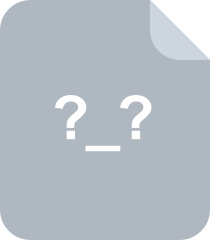
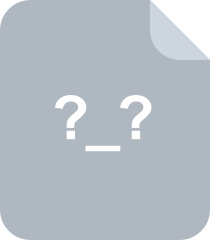
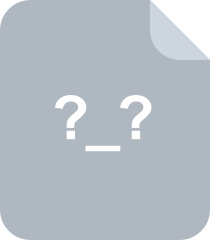
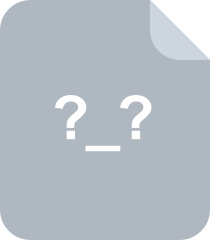
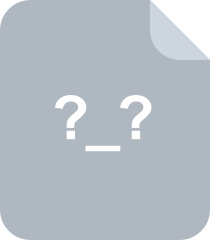
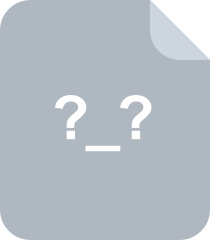
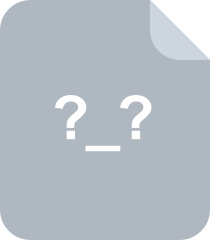
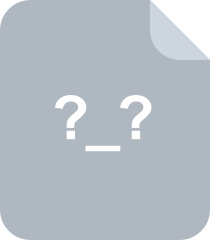
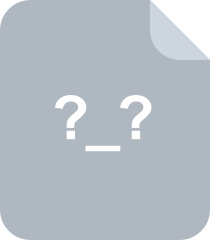
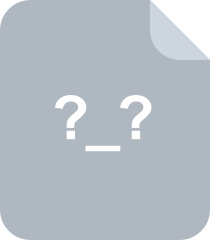
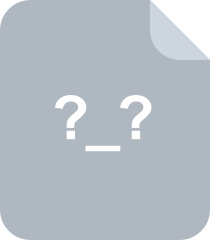
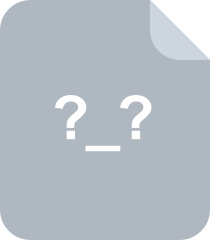
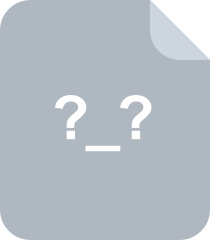
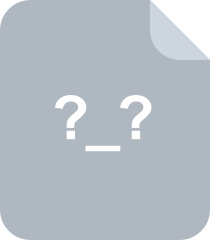
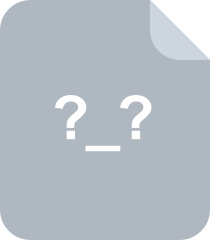
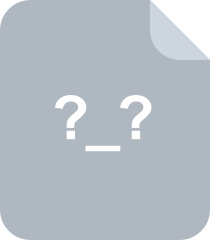
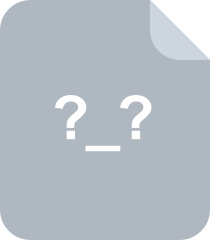
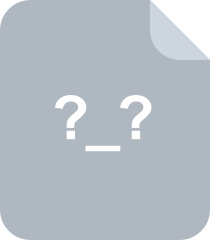
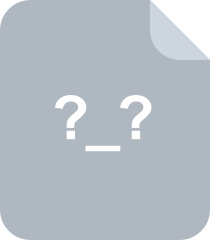
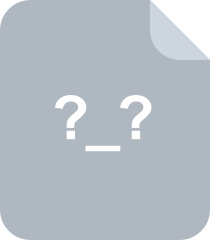
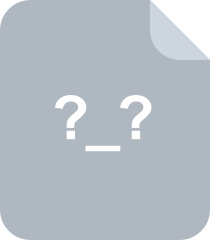
共 149 条
- 1
- 2
资源评论
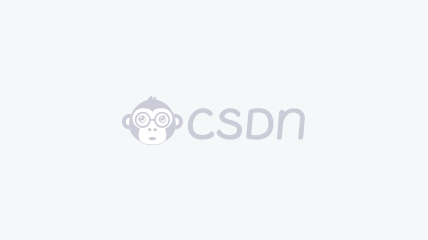

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

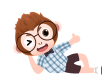
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


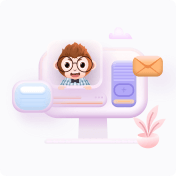
安全验证
文档复制为VIP权益,开通VIP直接复制
