
[](https://relevanceai.readthedocs.io/en/latest/?badge=latest)
[](https://img.shields.io/pypi/l/relevanceai)
For guides, tutorials on how to use this package, visit https://docs.relevance.ai/docs.
## ð¥ Features
Features of the library include:
- Quick vector search with free dashboard to preview results
- Vector clustering with support with built-in easy customisation
- Multi-vector search with filtering, facets, weighting
- Hybrid search (weighting exact text matching and vector search together)
... and more!
## ð§ Documentation
There are two main ways of documentations to take a look at:
| API type | Link |
| ------------- | ----------- |
| Guides | [Documentation](https://docs.relevance.ai/) |
| Python Reference | [Documentation](https://relevanceai.readthedocs.io/) |
## ð ï¸ Installation
```{bash}
pip install -U relevanceai
```
Or you can install it via conda to:
```{bash}
conda install pip
pip install -c relevanceai
```
You can also install on conda (only available on Linux environments at the moment): `conda install -c relevance relevanceai`.
## â© Quickstart
### Login into your project space
```{python}
from relevanceai import Client
client = relevanceai.Client(<project_name>, <api_key>)
```
This is a data example in the right format to be uploaded to relevanceai. Every document you upload should:
- Be a list of dictionaries
- Every dictionary has a field called _id
- Vector fields end in _vector_
```{python}
docs = [
{"_id": "1", "example_vector_": [0.1, 0.1, 0.1], "data": "Documentation"},
{"_id": "2", "example_vector_": [0.2, 0.2, 0.2], "data": "Best document!"},
{"_id": "3", "example_vector_": [0.3, 0.3, 0.3], "data": "document example"},
{"_id": "4", "example_vector_": [0.4, 0.4, 0.4], "data": "this is another doc"},
{"_id": "5", "example_vector_": [0.5, 0.5, 0.5], "data": "this is a doc"},
]
```
### Upload data into a new dataset
The documents will be uploaded into a new dataset that you can name in whichever way you want. If the dataset name does not exist yet, it will be created automatically. If the dataset already exist, the uploaded _id will be replacing the old data.
```{python}
client.insert_documents(dataset_id="quickstart", docs=docs)
```
### Perform a vector search
```{python}
client.services.search.vector(
dataset_id="quickstart",
multivector_query=[
{"vector": [0.2, 0.2, 0.2], "fields": ["example_vector_"]},
],
page_size=3,
query="sample search" # Stored on the dashboard but not required
```
## ð§ Development
### Getting Started
To get started with development, ensure you have pytest and mypy installed. These will help ensure typechecking and testing.
```{bash}
python -m pip install pytest mypy
```
Then run testing using:
Make sure to set your test credentials!
```{bash}
export TEST_PROJECT = xxx
export TEST_API_KEY = xxx
python -m pytest
mypy relevanceai
```
## ð§° Config
The config contains the adjustable global settings for the SDK. For a description of all the settings, see here.
To view setting options, run the following:
```{python}
client.config.options
```
The syntax for selecting an option is section.key. For example, to disable logging, run the following to modify logging.enable_logging:
```{python}
client.config.set_option('logging.enable_logging', False)
```
To restore all options to their default, run the following:
### Changing the base URL
You can change the base URL as such:
```{python}
client.base_url = "https://.../latest"
```
You can also update the ingest base URL:
```{python}
client.ingest_base_url = "https://.../latest
```

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
最新资源
- VmwareHardenedLoader.zip
- Labview通过FINS tcp协议与欧姆龙PLC通讯,支持CIO区,W区,D区,布尔量,整数,浮点数,字符串读写操作,软件无加密
- 英特尔2021-2024年网络连接性和IPU路线图
- Intouch2020R2SP1与西门子1500PLC通讯配置手册
- 电池组散热分析 ansys 流体 fluent
- 陀螺仪选型陀螺仪陀螺仪选型型陀螺仪选型
- 快速排序算法Python实现:详解分治法原理与高效排序步骤
- STM32F401,使用ST-link时候,不能识别,显示ST-LINK USB communication error
- Avue.js是基于现有的element-plus库进行的二次封装,简化一些繁琐的操作,核心理念为数据驱动视图,主要的组件库针对table表格和form表单场景,同时衍生出更多企业常用的组件,达到高复
- COMSOL 准 BIC控制石墨烯临界耦合光吸收 COMSOL 光学仿真,石墨烯,光吸收,费米能级可调下图是仿真文件截图,所见即所得
- Intel-633246-eASIC-PB-006-N5X-Product-Brief .pdf
- 家庭用具检测21-YOLO(v5至v11)、COCO、Paligemma、TFRecord、VOC数据集合集.rar
- 51单片机仿真摇号抽奖机源程序12864液晶显示仿真+程序
- Pear Admin 是 一 款 开 箱 即 用 的 前 端 开 发 模 板,提供便捷快速的开发方式,延续 Admin 的设计规范
- ECSHOP模板堂最新2017仿E宠物模板 整合ECTouch微分销商城
- 完结26章Java主流分布式解决方案多场景设计与实战
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


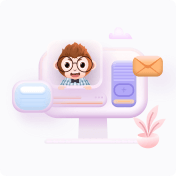