# Copyright 2020 Q-CTRL Pty Ltd & Q-CTRL Inc. All rights reserved.
#
# Licensed under the Q-CTRL Terms of service (the "License"). Unauthorized
# copying or use of this file, via any medium, is strictly prohibited.
# Proprietary and confidential. You may not use this file except in compliance
# with the License. You may obtain a copy of the License at
#
# https://q-ctrl.com/terms
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS. See the
# License for the specific language.
"""
Functions for plotting control pulses.
"""
from collections import namedtuple
from typing import (
Any,
List,
)
import numpy as np
from matplotlib.ticker import ScalarFormatter
from .style import qctrl_style
@qctrl_style()
def plot_controls(figure, controls, polar=True, smooth=False):
"""
Creates a plot of the specified controls.
Parameters
----------
figure : matplotlib.figure.Figure
The matplotlib Figure in which the plots should be placed. The dimensions of the Figure
will be overridden by this method.
controls : dict
The dictionary of controls to plot. The keys should be the names of the controls, and the
values the list of segments representing the pulse of that control. Each such segment must
be a dictionary with 'value' and 'duration' keys, giving the value (in Hertz, possibly
complex) and duration (in seconds) of that segment of the pulse.
For example, the following would be a valid ``controls`` input::
{
'Clock': [
{'duration': 1.0, 'value': -0.5},
{'duration': 1.0, 'value': 0.5},
{'duration': 2.0, 'value': -1.5},
],
'Microwave': [
{'duration': 0.5, 'value': 0.5 + 1.5j},
{'duration': 1.0, 'value': 0.2 - 0.3j},
],
}
polar : bool, optional
The mode of the plot when the values appear to be complex numbers.
Plot magnitude and angle in two figures if set to True, otherwise plot I and Q
in two figures. Defaults to True.
smooth : bool, optional
Whether to plot the controls as samples joined by straight lines, rather than as
piecewise-constant segments. Defaults to False.
Raises
------
ValueError
If any of the input parameters are invalid.
"""
plots_data: List[Any] = []
for name, segments in controls.items():
durations = []
values = []
for segment in segments:
durations.append(segment["duration"])
values.append(segment["value"])
values = np.array(values)
plots_data = plots_data + _create_plots_data_from_control(
name, durations, values, polar
)
axes_list = _create_axes(figure, len(plots_data), width=7.0, height=2.0)
if smooth:
for axes, plot_data in zip(axes_list, plots_data):
# Convert the list of durations into a list of times at the midpoints of each segment,
# with a leading zero and trailing total time.
# Length of 'times' is m+2 (m is the number of segments).
end_points = np.cumsum(plot_data.xdata)
times = np.concatenate(
[[0.0], end_points - np.array(plot_data.xdata) * 0.5, [end_points[-1]]]
)
# Pad each values array with leading and trailing zeros, to indicate that the pulse is
# zero outside the plot domain. Length of 'values' is m+2.
values = np.pad(plot_data.values, ((1, 1)), "constant")
axes.plot(times, values, linewidth=2)
axes.fill(times, values, alpha=0.3)
axes.axhline(y=0, linewidth=1, zorder=-1)
axes.set_ylabel(plot_data.ylabel)
else:
for axes, plot_data in zip(axes_list, plots_data):
# Convert the list of durations into times, including a leading zero. Length of 'times'
# is m+1 (m is the number of segments).
times = np.insert(np.cumsum(plot_data.xdata), 0, 0.0)
# Pad each values array with leading and trailing zeros, to indicate that the pulse is
# zero outside the plot domain. Length of 'values' is m+2.
values = np.pad(plot_data.values, ((1, 1)), "constant")
# *---v2--*
# | |
# *--v1--* | *-v4-*
# | | | |
# | *---v3---* |
# --v0--* *---v5--
# t0 t1 t2 t3 t4
# To plot a piecewise-constant pulse, we need to sample from the times array at indices
# [0, 0, 1, 1, ..., m-1, m-1, m, m ], and from the values arrays at indices
# [0, 1, 1, 2, ..., m-1, m, m, m+1].
time_indices = np.repeat(np.arange(len(times)), 2)
value_indices = np.repeat(np.arange(len(values)), 2)[1:-1]
axes.plot(times[time_indices], values[value_indices], linewidth=2)
axes.fill(times[time_indices], values[value_indices], alpha=0.3)
axes.axhline(y=0, linewidth=1, zorder=-1)
for time in times:
axes.axvline(x=time, linestyle="--", linewidth=1, zorder=-1)
axes.set_ylabel(plot_data.ylabel)
axes_list[-1].set_xlabel(plots_data[-1].xlabel)
@qctrl_style()
def plot_smooth_controls(figure, controls, polar=True):
"""
Creates a plot of the specified smooth controls.
Parameters
----------
figure : matplotlib.figure.Figure
The matplotlib Figure in which the plots should be placed. The dimensions of the Figure
will be overridden by this method.
controls : dict
The dictionary of controls to plot. The keys should be the names of the controls, and the
values the list of samples representing the pulse of that control. Each such sample must
be a dictionary with 'value' and 'time' keys, giving the value (in Hertz, possibly complex)
and time (in seconds) of that sample of the pulse. The times must be in increasing order.
For example, the following would be a valid ``controls`` input::
{
'Clock': [
{'time': 0.0, 'value': -0.5},
{'time': 1.0, 'value': 0.5},
{'time': 3.0, 'value': -1.5},
],
'Microwave': [
{'time': 0.5, 'value': 0.5 + 1.5j},
{'time': 2.0, 'value': 0.2 - 0.3j},
],
}
polar : bool, optional
The mode of the plot when the values appear to be complex numbers.
Plot magnitude and angle in two figures if set to True, otherwise plot I and Q
in two figures. Defaults to True.
Raises
------
ValueError
If any of the input parameters are invalid.
"""
plots_data: List[Any] = []
for name, samples in controls.items():
times = []
values = []
for sample in samples:
times.append(sample["time"])
values.append(sample["value"])
values = np.array(values)
plots_data = plots_data + _create_plots_data_from_control(
name, times, values, polar
)
axes_list = _create_axes(figure, len(plots_data), width=7.0, height=2.0)
for axes, plot_data in zip(axes_list, plots_data):
# Pad with leading and trailing zeros, to indicate that the pulse is zero outside the plot
# domain.
times = np.pad(plot_data.xdata, ((1, 1)), "edge")
values = np.pad(plot_data.values, ((1, 1)), "constant")
axes.plot(times, values, linewidth=2)
axes.fill(times, values, alpha=0.3)
axes.axhline(y=0, linewidth=1, zorder=-1)
axes.set_ylabel(plot_data.ylabel)
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源分类:Python库 所属语言:Python 资源全名:qctrl-visualizer-2.4.0.tar.gz 资源来源:官方 安装方法:https://lanzao.blog.csdn.net/article/details/101784059
资源详情
资源评论
资源推荐
收起资源包目录


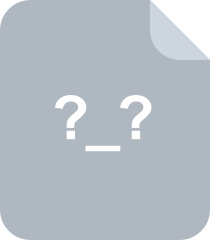
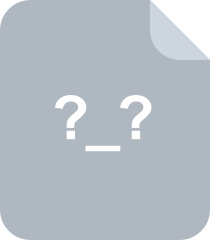
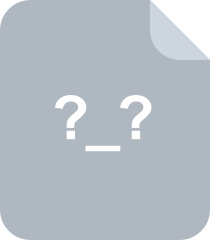
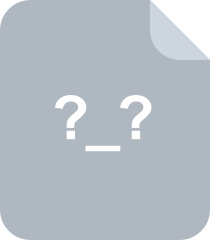

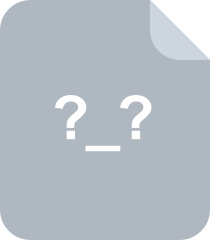
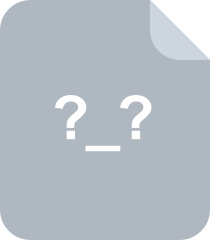
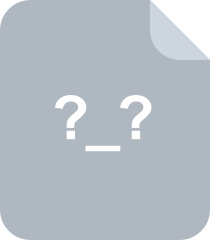
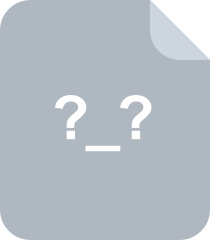
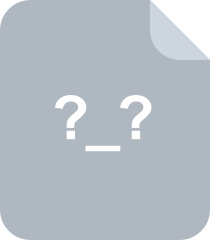
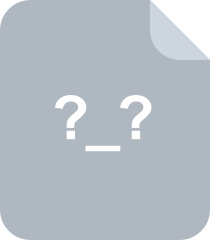
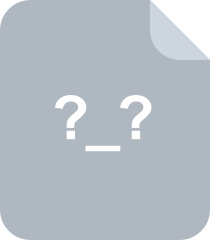
共 11 条
- 1
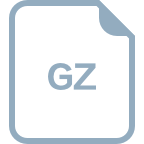
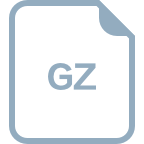
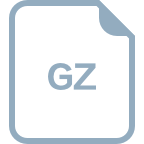
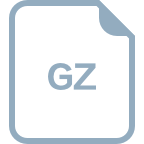
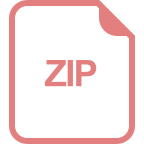
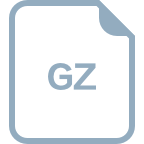
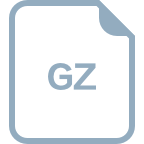
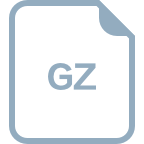
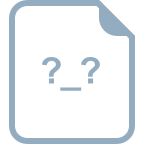
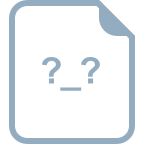
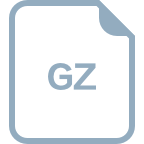
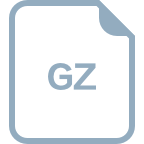
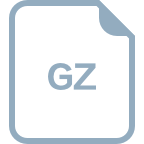
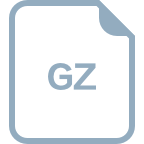
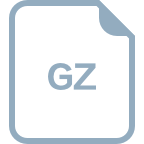
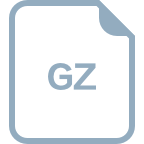
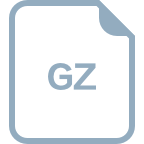
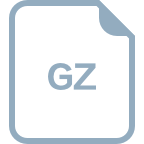
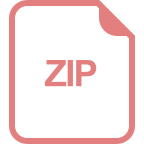
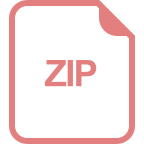
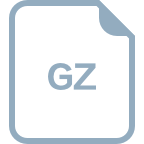
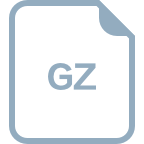
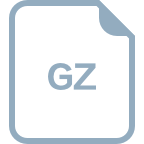
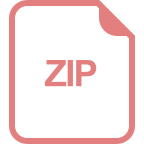

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

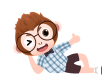
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


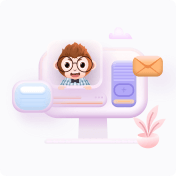
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0