# ���� Python FFmpeg Video Streaming
[](https://travis-ci.org/aminyazdanpanah/python-ffmpeg-video-streaming)
[](https://ci.appveyor.com/project/aminyazdanpanah/python-ffmpeg-video-streaming)
[](https://pepy.tech/project/python-ffmpeg-video-streaming)
[](https://badge.fury.io/py/python-ffmpeg-video-streaming)
[](https://github.com/aminyazdanpanah/python-ffmpeg-video-streaming/blob/master/LICENSE)
This package uses the **[FFmpeg](https://ffmpeg.org)** to package media content for online streaming(DASH and HLS)
**Contents**
- [Requirements](#requirements)
- [Installation](#installation)
- [Quickstart](#quickstart)
- [Opening a File](#opening-a-file)
- [DASH](#dash)
- [HLS](#hls)
- [Encrypted HLS](#encrypted-hls)
- [Progress](#progress)
- [Probe](#probe)
- [Several Open Source Players](#several-open-source-players)
- [Contributing and Reporting Bugs](#contributing-and-reporting-bugs)
- [Credits](#credits)
- [License](#license)
## Requirements
1. This version of the package is only compatible with **[Python 3.6](https://www.python.org/downloads/)** or higher.
2. To use this package, you need to **[install the FFMpeg](https://ffmpeg.org/download.html)**. You will need both FFMpeg and FFProbe binaries to use it.
## Installation
The latest version of `ffmpeg-streaming` can be acquired via pip:
```
pip install python-ffmpeg-video-streaming
```
## Quickstart
The best way to learn how to use this library is to review ****[the examples](https://github.com/aminyazdanpanah/python-ffmpeg-video-streaming/tree/master/examples)**** and browse the source code.
### opening a file
There are two ways to open a file:
#### 1. From a Local Path
```python
video = '/var/www/media/videos/test.mp4'
```
#### 2. From a cloud
```python
from ffmpeg_streaming.from_clouds import from_url
url = 'https://github.com/aminyazdanpanah/python-ffmpeg-video-streaming/blob/master/examples/_example.mp4?raw=true'
video = from_url(url)
```
**NOTE:** This package uses **[Requests](https://2.python-requests.org/en/master/)** to send and receive files.
### DASH
**[Dynamic Adaptive Streaming over HTTP (DASH)](https://dashif.org/)**, also known as MPEG-DASH, is an adaptive bitrate streaming technique that enables high quality streaming of media content over the Internet delivered from conventional HTTP web servers.
Similar to Apple's HTTP Live Streaming (HLS) solution, MPEG-DASH works by breaking the content into a sequence of small HTTP-based file segments, each segment containing a short interval of playback time of content that is potentially many hours in duration, such as a movie or the live broadcast of a sports event. The content is made available at a variety of different bit rates, i.e., alternative segments encoded at different bit rates covering aligned short intervals of playback time. While the content is being played back by an MPEG-DASH client, the client uses a bit rate adaptation (ABR) algorithm to automatically select the segment with the highest bit rate possible that can be downloaded in time for playback without causing stalls or re-buffering events in the playback. The current MPEG-DASH reference client dash.js offers both buffer-based (BOLA) and hybrid (DYNAMIC) bit rate adaptation algorithms. Thus, an MPEG-DASH client can seamlessly adapt to changing network conditions and provide high quality playback with fewer stalls or re-buffering events. [Learn more](https://en.wikipedia.org/wiki/Dynamic_Adaptive_Streaming_over_HTTP)
Create DASH Files:
```python
import ffmpeg_streaming
video = '/var/www/media/videos/test.mp4'
(
ffmpeg_streaming
.dash(video, adaption='"id=0,streams=v id=1,streams=a"')
.format('libx265')
.auto_rep()
.package('/var/www/media/videos/dash/test.mpd')
)
```
You can also create representations manually:
```python
import ffmpeg_streaming
from ffmpeg_streaming import Representation
from ffmpeg_streaming.from_clouds import from_url
url = 'https://github.com/aminyazdanpanah/python-ffmpeg-video-streaming/blob/master/examples/_example.mp4?raw=true'
video = from_url(url)
rep1 = Representation(width=256, height=144, kilo_bitrate=200)
rep2 = Representation(width=426, height=240, kilo_bitrate=500)
rep3 = Representation(width=640, height=360, kilo_bitrate=1000)
(
ffmpeg_streaming
.dash(video, adaption='"id=0,streams=v id=1,streams=a"')
.format('libx265')
.add_rep(rep1, rep2, rep3)
.package('/var/www/media/videos/dash/test.mpd')
)
```
See **[DASH examples](https://github.com/aminyazdanpanah/python-ffmpeg-video-streaming/tree/master/examples)** for more information.
See also **[DASH options](https://ffmpeg.org/ffmpeg-formats.html#dash-2)** for more information about options.
### HLS
**[HTTP Live Streaming (also known as HLS)](https://developer.apple.com/streaming/)** is an HTTP-based adaptive bitrate streaming communications protocol implemented by Apple Inc. as part of its QuickTime, Safari, OS X, and iOS software. Client implementations are also available in Microsoft Edge, Firefox and some versions of Google Chrome. Support is widespread in streaming media servers.
HLS resembles MPEG-DASH in that it works by breaking the overall stream into a sequence of small HTTP-based file downloads, each download loading one short chunk of an overall potentially unbounded transport stream. A list of available streams, encoded at different bit rates, is sent to the client using an extended M3U playlist. [Learn more](https://en.wikipedia.org/wiki/HTTP_Live_Streaming)
Create HLS files based on original video(auto generate qualities).
```python
import ffmpeg_streaming
from ffmpeg_streaming.from_clouds import from_url
url = 'https://github.com/aminyazdanpanah/python-ffmpeg-video-streaming/blob/master/examples/_example.mp4?raw=true'
video = from_url(url)
(
ffmpeg_streaming
.hls(video, hls_time=10, hls_allow_cache=1)
.format('libx264')
.auto_rep()
.package('/var/www/media/videos/hls/test.m3u8')
)
```
You can also create representations manually:
```python
import ffmpeg_streaming
from ffmpeg_streaming import Representation
video = '/var/www/media/videos/test.mp4'
rep1 = Representation(width=256, height=144, kilo_bitrate=200)
rep2 = Representation(width=426, height=240, kilo_bitrate=500)
rep3 = Representation(width=640, height=360, kilo_bitrate=1000)
(
ffmpeg_streaming
.hls(video, hls_time=10, hls_allow_cache=1)
.format('libx264')
.add_rep(rep1, rep2, rep3)
.package('/var/www/media/videos/hls/test.m3u8')
)
```
**NOTE:** You cannot use HEVC(libx265) and VP9 formats for HLS packaging.
#### Encrypted HLS
The encryption process requires some kind of secret (key) together with an encryption algorithm. HLS uses AES in cipher block chaining (CBC) mode. This means each block is encrypted using the ciphertext of the preceding block. [Learn more](https://en.wikipedia.org/wiki/Block_cipher_mode_of_operation)
You need to pass both `URL to the key` and `path to save a random key` to the `encryption` method:
```python
import ffmpeg_streaming
from ffmpeg_streaming.from_clouds import from_url
url = 'https://github.com/aminyazdanpanah/python-ffmpeg-video-streaming/blob/master/examples/_example.mp4?raw=true'
video = from_url(url)
(
ffmpeg_streaming
.hls(video, hls_time=10, hls_allow_cache=1)
.encryption('https://www.aminyazdanpanah.com/k

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
最新资源
- 基于ssm的校园美食交流系统源码(java毕业设计完整源码+LW).zip
- 基于ssm的小学生课外知识学习网站源码(java毕业设计完整源码+LW).zip
- 卷盘式上料机sw18可编辑全套技术资料100%好用.zip
- 基于ssm的绿色农产品推广应用网站源码(java毕业设计完整源码+LW).zip
- 基于Vue.js的在线购物系统的设计与实现源码(java毕业设计完整源码+LW).zip
- 基于ssm的游戏攻略网站的设计与实现源码(java毕业设计完整源码+LW).zip
- 基于ssm的毕业管理系统源码(java毕业设计完整源码+LW).zip
- 基于ssm的智慧城市实验室主页系统设计与实现源码(java毕业设计完整源码+LW).zip
- 空气压缩包装机sw18全套技术资料100%好用.zip
- 基于ssm的高校共享单车管理系统的设计与实现源码(java毕业设计完整源码+LW).zip
- 空气增压泵AB-03(sw14可编辑+工程图)全套技术资料100%好用.zip
- 开关耐久性测试试验机sw20全套技术资料100%好用.zip
- 基于ssm的个人博客网站的设计与实现源码(java毕业设计完整源码+LW).zip
- 快递分拣机器人sw18可编辑全套技术资料100%好用.zip
- 快递分拣流水线sw18可编辑全套技术资料100%好用.zip
- 快递打包压缩机(sw18可编辑+工程图)全套技术资料100%好用.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


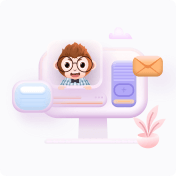