[](https://travis-ci.org/marcopaz/pyjo_mongo)
# pyjo_mongo
A light wrapper around pymongo and pyjo to easily interact with MongoDB documents. See the following example.
## Install
```
pip install pyjo_mongo
```
## How to use
```python
from pymongo import MongoClient
from pyjo import Model, Field, RangeField, EnumField
from pyjo_mongo import Document
db_connection = MongoClient(MONGODB_URL)[DB_NAME]
class Gender(Enum):
female = 0
male = 1
class Address(Model):
city = Field(type=str)
postal_code = Field(type=int)
address = Field()
class User(Document):
__meta__ = {
'db_connection': lambda: db_connection,
'collection_name': 'users',
'indexes': [
{
'fields': ['username'],
'unique': True,
'index_background': True,
},
['first_name', 'last_name'],
],
}
username = Field(type=str, repr=True, required=True)
first_name = Field(type=str)
last_name = Field(type=str)
age = RangeField(min=18, max=120)
gender = EnumField(enum=Gender)
address = Field(type=Address)
active = Field(type=bool, default=True)
```
```python
u = User(username='mp')
u.id
# None
u.save()
u.id
# ObjectId('5a5ca86080a9b8291874f4db')
# objects is a class property built around a pymongo Cursor
# and will allow you to perform basic operations
u2 = User.objects.find_one({'username': 'mp'})
u2.gender = Gender.male
u2.save()
u.reload()
u.gender
# Gender.male
# queries use the same syntax of pymongo and automatically return pyjo data models
for user in User.objects.find({'active': True}):
print(user)
# <User(username=mp)>
# You can perform ordering operations on querysets
for user in User.objects.order_by('gender', '-age'):
print(user.username)
print(user.age)
```
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源分类:Python库 所属语言:Python 资源全名:pyjo_mongo-0.8.0.tar.gz 资源来源:官方 安装方法:https://lanzao.blog.csdn.net/article/details/101784059
资源推荐
资源详情
资源评论
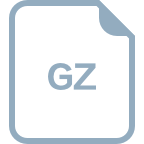
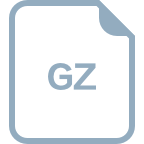
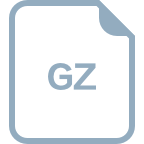
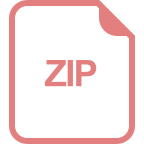
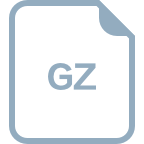
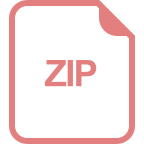
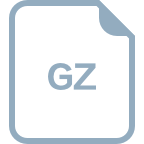
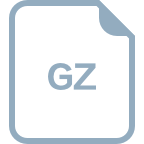
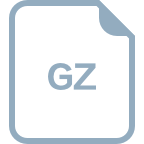
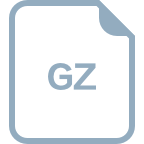
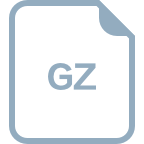
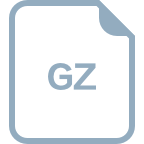
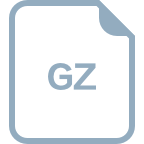
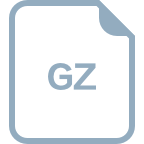
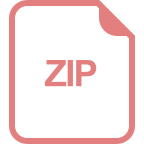
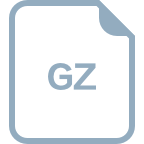
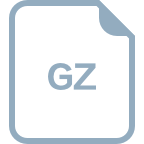
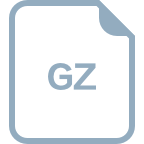
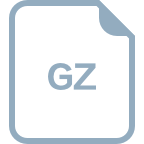
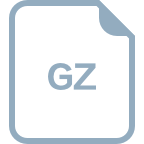
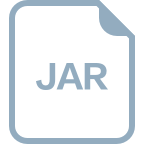
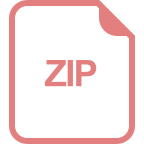
收起资源包目录


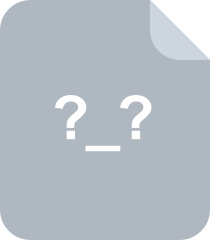
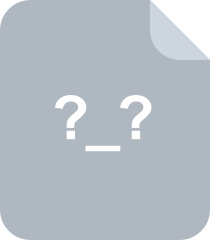

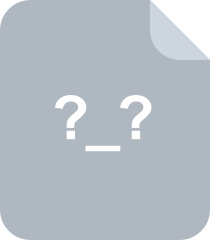
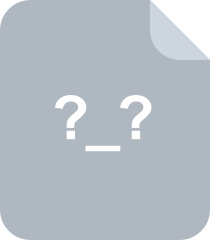
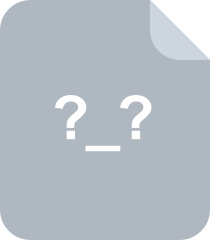

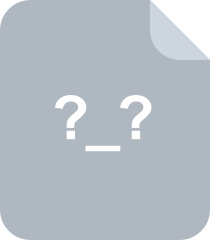
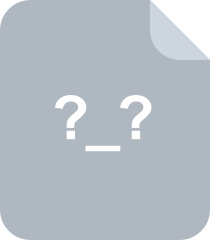
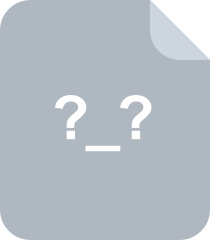

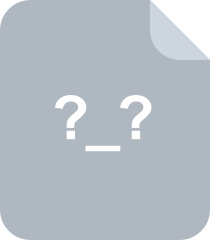
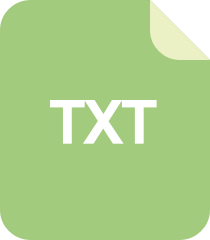
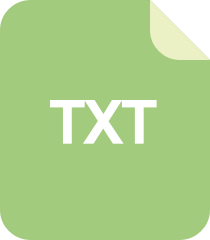
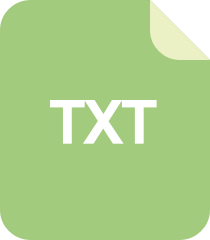
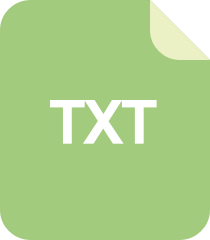
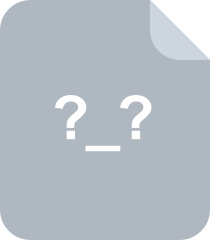
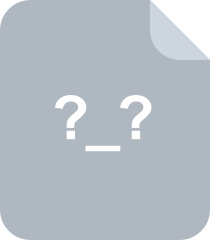
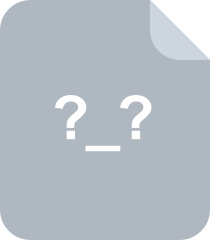
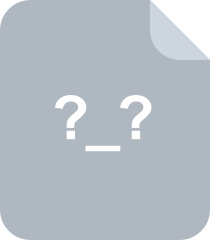
共 17 条
- 1
资源评论
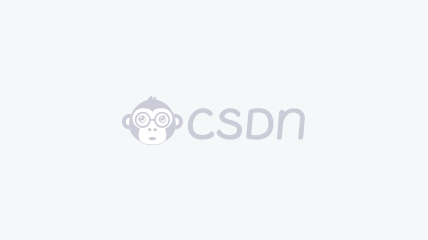

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

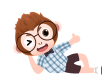
安全验证
文档复制为VIP权益,开通VIP直接复制
