# Python Dictionary Representation
## Description
The `DictRepr` class is an abstract base class that enables any subclass to define its own dictionary representation. This makes it possible to do:
```python
dict_repr = dict(arbitrary_object)
```
The subclass only needs to do two things:
- Override the `keys()` instance method to return a list of strings
- Have the keys map to variables or properties on that object
## Example
```python
lass MyArbitraryClass(DictRepr):
_keys = ["uppercase", "length"]
def __init__(self, value: str):
self._value = value
@property
def uppercase(self):
return self._value.upper()
@property
def length(self):
return len(self._value)
def keys(self):
return self._keys
if __name__ == "__main__":
myobj = MyArbitraryClass("Hello World")
mydict = dict(myobj)
print("mydict type: {}".format(type(mydict)))
pprint(mydict)
```
```console
$ python3 examples/dict_repr_example.py
mydict type: <class 'dict'>
{'length': 11, 'uppercase': 'HELLO WORLD'}
```
## Mapping Keys to Object Attributes
It may be the case that a desired dicitonary key isn't the name of the corresponding attribute. For example, a string may have spaces or other characters that, while perfectly suitable as a dictionary key, make it illegal as an object attribute name.
In this case, the `KeyTuple` class provides a mapping between key string and attribute name. Simply include a KeyTuple object in place of the key string:
```Python
_keys = [
KeyTuple("string with spaces 1", "no_spaces_1"),
KeyTuple("string with spaces 2", "no_spaces_2")
]
```
### KeyTuple Example
Let's modify the previous example:
```python
from py_dict_repr import DictRepr, KeyTuple
class MyArbitraryClass(DictRepr):
_keys = [
KeyTuple("uppercase verion", "uppercase"),
KeyTuple("string length", "length"),
# KeyTuple may be mixed with regular key strings
"lowercase"
]
def __init__(self, value: str):
self._value = value
@property
def uppercase(self):
return self._value.upper()
@property
def lowercase(self):
return self._value.lower()
@property
def length(self):
return len(self._value)
def keys(self):
return self._keys
if __name__ == "__main__":
myobj = MyArbitraryClass("Hello World")
mydict = dict(myobj)
print("mydict type: {}".format(type(mydict)))
pprint(mydict)
```
```console
$ python3 examples/dict_repr_example.py
python3 ./examples/dict_repr_keytuple_example.py
mydict type: <class 'dict'>
{string length: 11, uppercase verion: 'HELLO WORLD', 'lowercase': 'hello world'}
```
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源分类:Python库 所属语言:Python 资源全名:py-dict-repr-0.1.1.tar.gz 资源来源:官方 安装方法:https://lanzao.blog.csdn.net/article/details/101784059
资源推荐
资源详情
资源评论
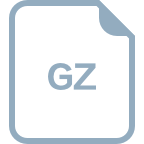
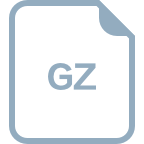
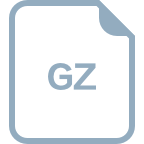
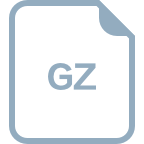
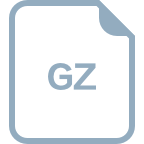
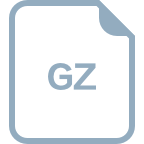
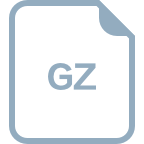
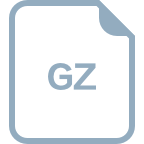
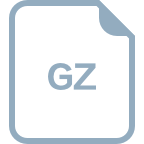
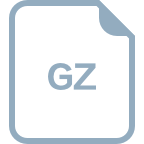
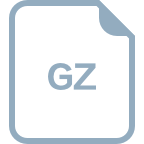
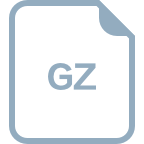
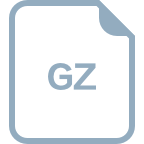
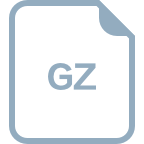
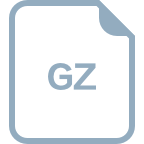
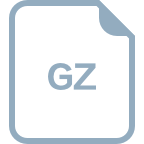
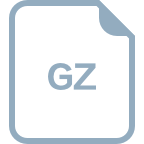
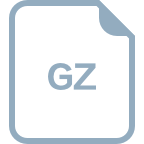
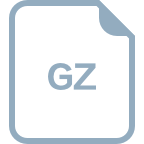
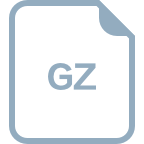
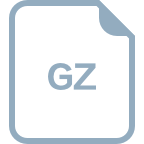
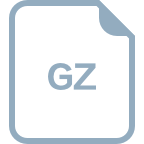
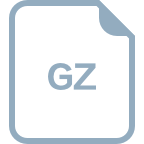
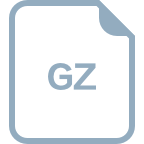
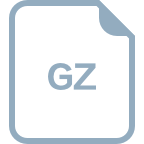
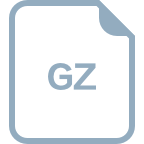
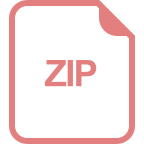
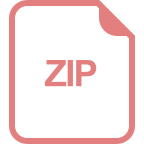
收起资源包目录


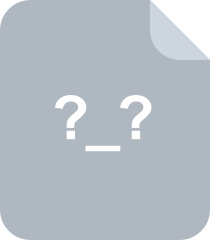

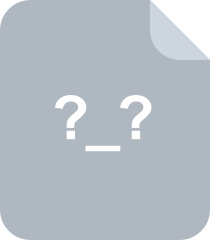
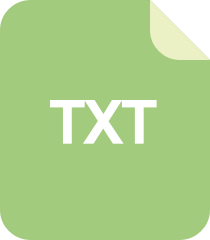
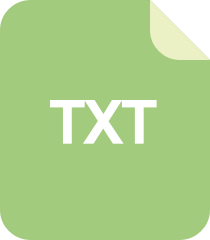
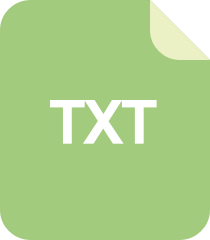
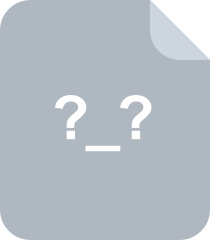

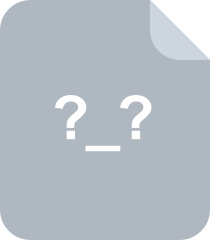
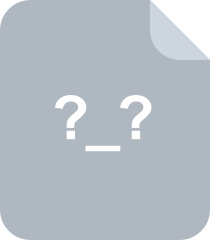
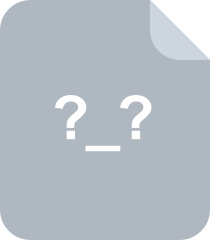
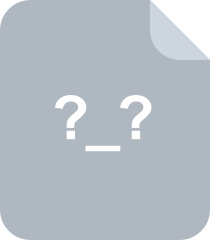
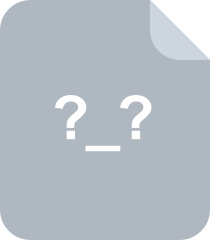
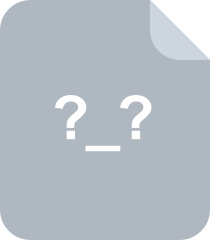
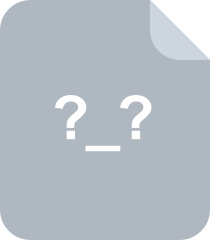
共 13 条
- 1
资源评论
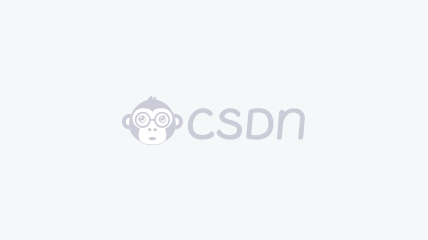

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

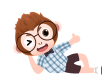
最新资源
- 基于Java Server Pages技术的CIMS课程设计源码
- 计组复习 4.docx
- 基于C语言核心的mGBA Game Boy Advance模拟器设计源码
- 基于Go语言的OAuth2 Server/Client自动同步设计源码库
- 基于Vue框架的水务局水资源管理系统设计源码
- 计组复习 3.docx
- 基于Vue框架的企业门户网站设计源码
- 计组 5.3 补码的加减法.docx
- 基于React和Vite的广西人才网手机端仿制设计源码
- 计组 5.2 浮点数据表示.docx
- 计组期末复习 2.docx
- 计组复习期末 1.docx
- 基于Gin、Vue2、ElementUI的EasyGoAdmin前后端分离权限管理系统设计源码
- 计组 10.1 微操作的节拍安排.docx
- 计组 8.2 指令周期,指令流水.docx
- 基于Python的时间序列分析交通流量预测与优化设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


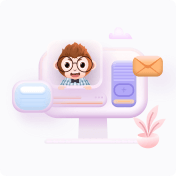
安全验证
文档复制为VIP权益,开通VIP直接复制
