# mypy-boto3-codedeploy
[](https://pypi.org/project/mypy-boto3-codedeploy)
[](https://pypi.org/project/mypy-boto3-codedeploy)
[](https://mypy-boto3-builder.readthedocs.io/)
Type annotations for
[boto3.CodeDeploy 1.14.62](https://boto3.amazonaws.com/v1/documentation/api/1.14.62/reference/services/codedeploy.html#CodeDeploy) service
compatible with
[VSCode](https://code.visualstudio.com/),
[PyCharm](https://www.jetbrains.com/pycharm/),
[mypy](https://github.com/python/mypy),
[pyright](https://github.com/microsoft/pyright)
and other tools.
Generated by [mypy-boto3-buider 3.1.0](https://github.com/vemel/mypy_boto3_builder).
More information can be found on [boto3-stubs](https://pypi.org/project/boto3-stubs/) page.
- [mypy-boto3-codedeploy](#mypy-boto3-codedeploy)
- [How to install](#how-to-install)
- [Usage](#usage)
- [VSCode](#vscode)
- [PyCharm](#pycharm)
- [Other IDEs](#other-ides)
- [mypy](#mypy)
- [pyright](#pyright)
- [Explicit type annotations](#explicit-type-annotations)
- [Client annotations](#client-annotations)
- [Paginators annotations](#paginators-annotations)
- [Waiters annotations](#waiters-annotations)
- [Service Resource annotations](#service-resource-annotations)
- [Other resources annotations](#other-resources-annotations)
- [Collections annotations](#collections-annotations)
- [Typed dictionations](#typed-dictionations)
## How to install
Install `boto3-stubs` for `CodeDeploy` service.
```bash
python -m pip install boto3-stubs[codedeploy]
```
## Usage
### VSCode
- Install [Python extension](https://marketplace.visualstudio.com/items?itemName=ms-python.python)
- Install [Pylance extension](https://marketplace.visualstudio.com/items?itemName=ms-python.vscode-pylance)
- Set `Pylance` as your Python Language Server
- Install `boto-stubs[codedeploy]` in your environment: `python -m pip install 'boto3-stubs[codedeploy]'`
Both type checking and auto-complete should work for `CodeDeploy` service.
No explicit type annotations required, write your `boto3` code as usual.
### PyCharm
- Install `boto-stubs[codedeploy]` in your environment: `python -m pip install 'boto3-stubs[codedeploy]'`
Both type checking and auto-complete should work for `CodeDeploy` service.
No explicit type annotations required, write your `boto3` code as usual.
Auto-complete can be slow on big projects or if you have a lot of installed `boto3-stubs` submodules.
### Other IDEs
Not tested, but as long as your IDE support `mypy` or `pyright`, everything should work.
### mypy
- Install `mypy`: `python -m pip install mypy`
- Install `boto-stubs[codedeploy]` in your environment: `python -m pip install 'boto3-stubs[codedeploy]'`
- Run `mypy` as usual
Type checking should work for `CodeDeploy` service.
No explicit type annotations required, write your `boto3` code as usual.
### pyright
- Install `pyright`: `yarn global add pyright`
- Install `boto-stubs[codedeploy]` in your environment: `python -m pip install 'boto3-stubs[codedeploy]'`
- Optionally, you can install `boto3-stubs` to `typings` folder.
Type checking should work for `CodeDeploy` service.
No explicit type annotations required, write your `boto3` code as usual.
## Explicit type annotations
### Client annotations
`CodeDeployClient` provides annotations for `boto3.client("codedeploy")`.
```python
import boto3
from mypy_boto3_codedeploy import CodeDeployClient
client: CodeDeployClient = boto3.client("codedeploy")
# now client usage is checked by mypy and IDE should provide code auto-complete
# works for session as well
session = boto3.session.Session(region="us-west-1")
session_client: CodeDeployClient = session.client("codedeploy")
```
### Paginators annotations
`mypy_boto3_codedeploy.paginator` module contains type annotations for all paginators.
```python
from mypy_boto3_codedeploy import CodeDeployClient
from mypy_boto3_codedeploy.paginator import (
ListApplicationRevisionsPaginator,
ListApplicationsPaginator,
ListDeploymentConfigsPaginator,
ListDeploymentGroupsPaginator,
ListDeploymentInstancesPaginator,
ListDeploymentsPaginator,
ListDeploymentTargetsPaginator,
ListGitHubAccountTokenNamesPaginator,
ListOnPremisesInstancesPaginator,
)
client: CodeDeployClient = boto3.client("codedeploy")
# Explicit type annotations are optional here
# Type should be correctly discovered by mypy and IDEs
# VSCode requires explicit type annotations
list_application_revisions_paginator: ListApplicationRevisionsPaginator = client.get_paginator("list_application_revisions")
list_applications_paginator: ListApplicationsPaginator = client.get_paginator("list_applications")
list_deployment_configs_paginator: ListDeploymentConfigsPaginator = client.get_paginator("list_deployment_configs")
list_deployment_groups_paginator: ListDeploymentGroupsPaginator = client.get_paginator("list_deployment_groups")
list_deployment_instances_paginator: ListDeploymentInstancesPaginator = client.get_paginator("list_deployment_instances")
list_deployment_targets_paginator: ListDeploymentTargetsPaginator = client.get_paginator("list_deployment_targets")
list_deployments_paginator: ListDeploymentsPaginator = client.get_paginator("list_deployments")
list_git_hub_account_token_names_paginator: ListGitHubAccountTokenNamesPaginator = client.get_paginator("list_git_hub_account_token_names")
list_on_premises_instances_paginator: ListOnPremisesInstancesPaginator = client.get_paginator("list_on_premises_instances")
```
### Waiters annotations
`mypy_boto3_codedeploy.waiter` module contains type annotations for all waiters.
```python
from mypy_boto3_codedeploy import CodeDeployClient
from mypy_boto3_codedeploy.waiter import DeploymentSuccessfulWaiter
client: CodeDeployClient = boto3.client("codedeploy")
# Explicit type annotations are optional here
# Type should be correctly discovered by mypy and IDEs
# VSCode requires explicit type annotations
deployment_successful_waiter: DeploymentSuccessfulWaiter = client.get_waiter("deployment_successful")
```
### Typed dictionations
`mypy_boto3_codedeploy.type_defs` module contains structures and shapes assembled
to typed dictionaries for additional type checking.
```python
from mypy_boto3_codedeploy.type_defs import (
AlarmConfigurationTypeDef,
AlarmTypeDef,
ApplicationInfoTypeDef,
AppSpecContentTypeDef,
AutoRollbackConfigurationTypeDef,
AutoScalingGroupTypeDef,
BatchGetApplicationRevisionsOutputTypeDef,
BatchGetApplicationsOutputTypeDef,
BatchGetDeploymentGroupsOutputTypeDef,
BatchGetDeploymentInstancesOutputTypeDef,
BatchGetDeploymentsOutputTypeDef,
BatchGetDeploymentTargetsOutputTypeDef,
BatchGetOnPremisesInstancesOutputTypeDef,
BlueGreenDeploymentConfigurationTypeDef,
BlueInstanceTerminationOptionTypeDef,
CloudFormationTargetTypeDef,
CreateApplicationOutputTypeDef,
CreateDeploymentConfigOutputTypeDef,
CreateDeploymentGroupOutputTypeDef,
CreateDeploymentOutputTypeDef,
DeleteDeploymentGroupOutputTypeDef,
DeleteGitHubAccountTokenOutputTypeDef,
DeploymentConfigInfoTypeDef,
DeploymentGroupInfoTypeDef,
DeploymentInfoTypeDef,
DeploymentOverviewTypeDef,
DeploymentReadyOptionTypeDef,
DeploymentStyleTypeDef,
DeploymentTargetTypeDef,
DiagnosticsTypeDef,
EC2TagFilterTypeDef,
EC2TagSetTypeDef,
ECSServiceTypeDef,
ECSTargetTypeDef,
ECSTaskSetTypeDef,
ELBInfoTypeDef,
ErrorInformationTypeDef,
GenericRevisionInfoTypeDef,
GetApplicationOutputTypeDef,
GetApplicationRevisionOutputTypeDef,
GetDeploymentConfigOutputTypeDef,
GetDeploymentGroupOutputTypeDef,
GetDeploymentInstanceO

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
最新资源
- DSP280049C串口升级方案:全方位支持,包含Bootloader源码、上位机程序及用户示例工程,操作指南一览无余 ,DSP280049C串口升级方案:包含完整Bootloader源码与操作手册
- OBC&DCDC相关参数设计与计算
- 图书馆管理系统(SSH框架).zip(毕设&课设&实训&大作业&竞赛&项目)
- 基于BES秃鹰算法优化BP神经网络模型的多输入单输出拟合预测系统及其MATLAB程序实现,基于BES秃鹰智能算法的BP神经网络权值和阈值优化MATLAB实现,基于BES秃鹰智能算法优化BP神经网络模型
- JavaEE结课项目.zip(课设&实训&大作业&项目)
- 基于SSM+JSP的学生请假系统.zip(毕设&课设&实训&大作业&竞赛&项目)
- 全国大学生FPGA创新设计邀请赛的作品.zip
- 双层非线性优化模型:省内外电力市场及风险应对机制研究(以CVaR和线性转换为中心),基于CVaR方法的双层非线性优化模型在电力市场及省间交易中的研究与应用,主题:提出了一种双层非线性优化模型,将省内电
- 基于Qt的数据库应用课程设计-景点门票管理系统.zip(课设&实训&大作业&项目)
- Opencv实战基于python,银行卡识别、全景图片拼接、OCR图片识别.zip(毕设&课设&实训&大作业&竞赛&项目)
- 基于django的admin后台管理系统.zip(毕设&课设&实训&大作业&竞赛&项目)
- 前后端分离的简易博客项目(vue+springboot).zip(毕设&课设&实训&大作业&竞赛&项目)
- 基于西门子S7-1200的停车场车位智能控制系统:传感器检测、PLC控制及HM画面组态仿真,基于西门子S7-1200智能停车场的车位管理系统 - 传感器监控与PLC自动化控制方案 ,基于西门子120
- 基于Vue框架的Sparkle项目:TypeScript、JavaScript、HTML前端技术实践源码
- 基于AdminLTE +Django + Mysql 的会议室管理系统.zip(毕设&课设&实训&大作业&竞赛&项目)
- 西门子S7-1500 PLC在制药厂洁净空调系统中的精准控温控湿实践:包含冷水机组与洁净室空调机组案例研究,博图V15.1编程版本参考案例,西门子S7-1500 PLC在制药厂洁净空调系统中的精准控温
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


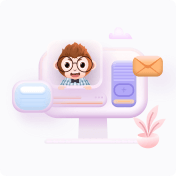